LangChain is a framework that enables developers to harness the power of large language models, allowing them to build applications in C++ that can interact with and manipulate text-based data efficiently.
Here's a simple code snippet demonstrating how to integrate a basic LangChain command in C++:
#include <iostream>
#include <string>
// Example function to simulate a text generation
std::string generateText(const std::string& input) {
// This is a placeholder for actual LangChain integrations
return "Generated response based on: " + input;
}
int main() {
std::string userInput = "Tell me about LangChain in C++.";
std::string response = generateText(userInput);
std::cout << response << std::endl;
return 0;
}
Understanding LangChain
What is LangChain?
LangChain is a framework designed to facilitate the integration of language models into various applications. Its primary purpose is to enhance the interactivity and utility of AI through a structured and organized approach. With LangChain C++, developers can build high-performance applications that leverage the unique capabilities of C++ while utilizing the power of language models.
Architecture of LangChain
LangChain consists of several core components, which collectively form a versatile ecosystem for building applications.
- Agents: These are the decision-makers in LangChain, capable of executing commands based on user inputs.
- Memory: Memory allows LangChain applications to retain context and information, making interactions more relevant and personalized.
- LLMs (Large Language Models): These powerful models generate human-like text and understand complex queries, significantly enhancing the effectiveness of applications.
C++ plays a crucial role in LangChain's architecture because it allows for efficient memory management and execution speed, making it ideal for performance-critical applications.
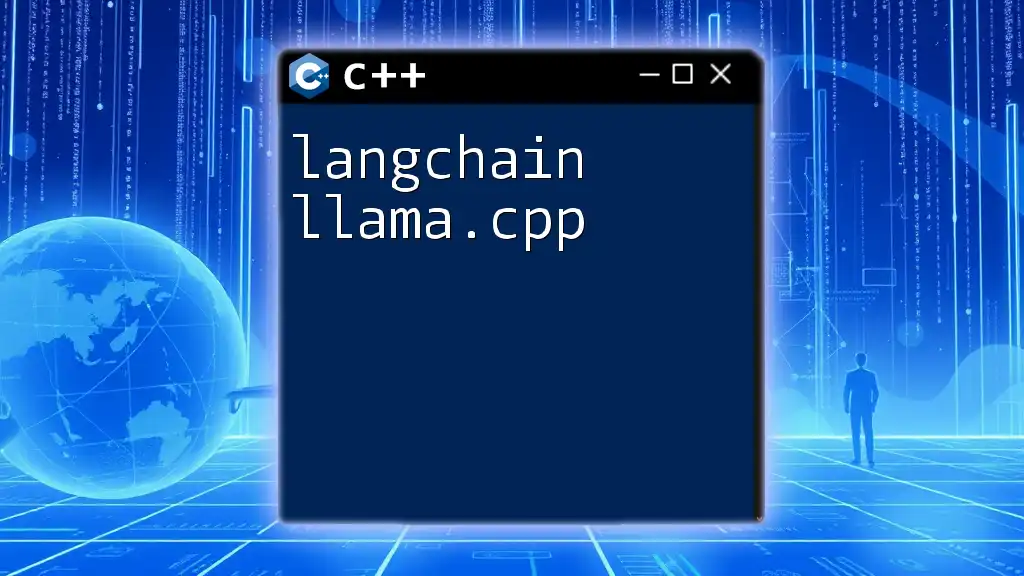
Setting Up Your C++ Environment
Essential Tools and Libraries
To get started with LangChain in C++, you need to set up a development environment that includes essential tools and libraries.
- C++ Compiler: Choose a suitable compiler such as GCC, Clang, or Visual Studio based on your operating system.
- LangChain Library: Ensure you have the LangChain library downloaded and referenced in your project.
- Additional Libraries: Use popular libraries like nlohmann/json for handling JSON data, which is common in API responses and configurations.
Installation Steps
- Download and Install C++ Compiler: Follow the guidelines specific to your OS for installation.
- Install LangChain: Visit the official LangChain website and follow the installation instructions tailored for C++.
- Test Your Environment: Run a simple 'Hello World' program to confirm that your development environment is set up correctly.
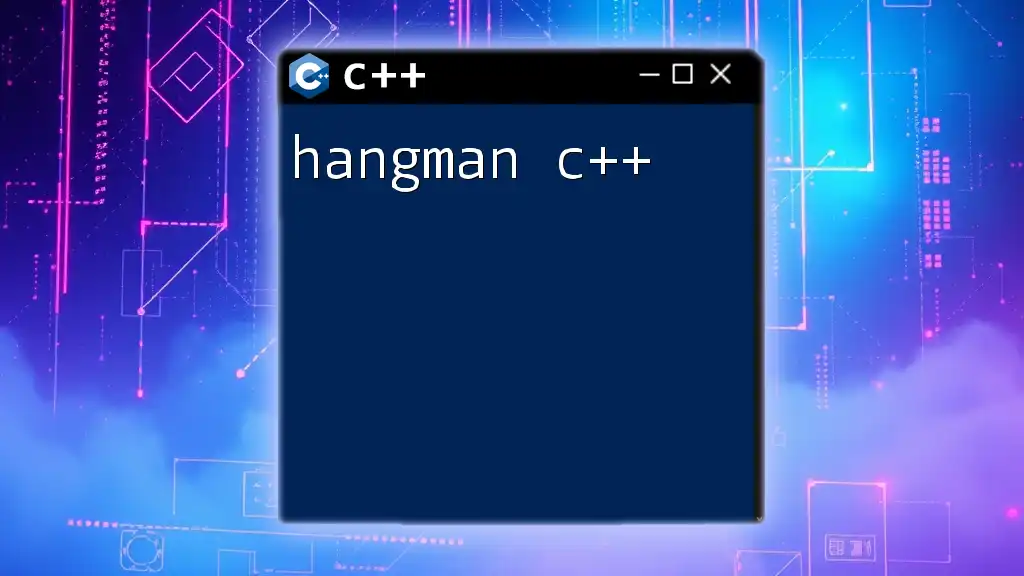
Core Commands in LangChain C++
Basic Concepts of Commands
In LangChain, commands are central to its functionality. They allow developers to create interactions that can respond to various inputs effectively. Understanding how commands work is fundamental to using LangChain efficiently in C++.
Example Commands
Creating a simple command in LangChain is quite straightforward. Below is a basic example demonstrating the creation and execution of a command:
#include <iostream>
#include "langchain/langchain.h"
int main() {
LangChain lc;
lc.add_command("greet", "Hello, World!");
lc.execute_command("greet");
return 0;
}
Explanation of the Code Snippet:
- #include: This directive includes the LangChain header.
- LangChain lc;: An instance of `LangChain` is created.
- add_command: This method adds a new command named "greet" with its associated response.
- execute_command: This method calls the command, producing the output "Hello, World!".
Advanced Command Use
For more engaging interactions, developers can handle user input dynamically, creating multi-step command sequences or including error handling mechanisms:
#include <iostream>
#include "langchain/langchain.h"
int main() {
LangChain lc;
lc.add_command("ask", "What's your name?");
std::string name;
std::cout << lc.execute_command("ask") << std::endl;
std::cin >> name;
lc.add_command("greet", "Hello, " + name + "!");
std::cout << lc.execute_command("greet") << std::endl;
return 0;
}
In this example, the program asks for the user’s name and greets them accordingly. User input handling enhances the interactivity of commands, making applications feel more personalized.
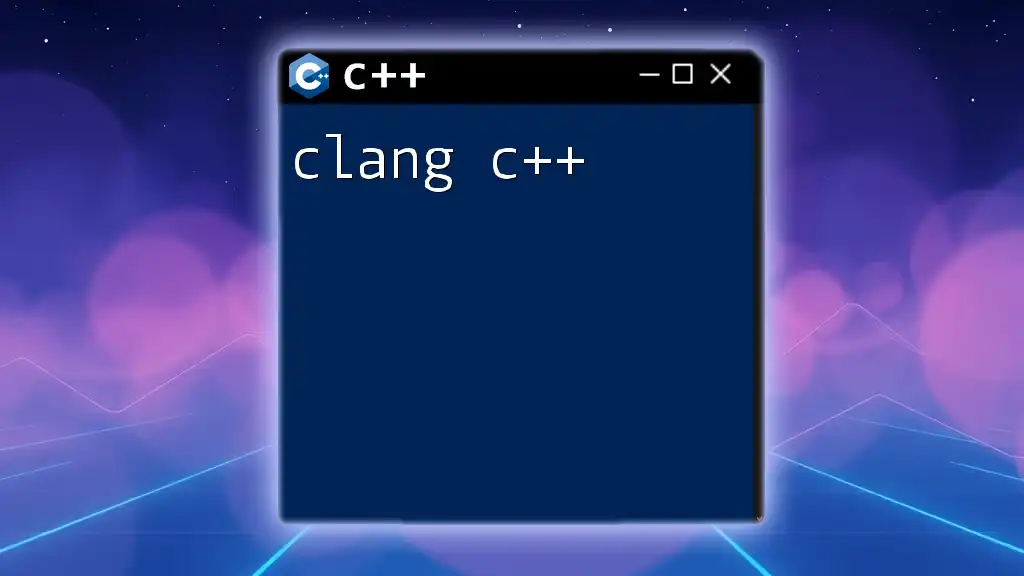
Memory Management in LangChain
Importance of Memory in LangChain Applications
Memory is a vital aspect of any interactive application. It enables the system to retain context, which leads to more relevant interactions over time. By managing memory effectively, developers can create applications that remember user preferences, past interactions, and other essential data.
Implementing Memory with C++
Below is an example of how to implement memory in LangChain:
Memory mem;
mem.save("my_data", "Some important data");
std::string retrieved_data = mem.get("my_data");
This code demonstrates the basic functionality of memory in LangChain. The `save` method stores data under a specified key, while `get` retrieves the stored data. Such functionality allows applications to become more context-aware, ultimately enhancing user experience.
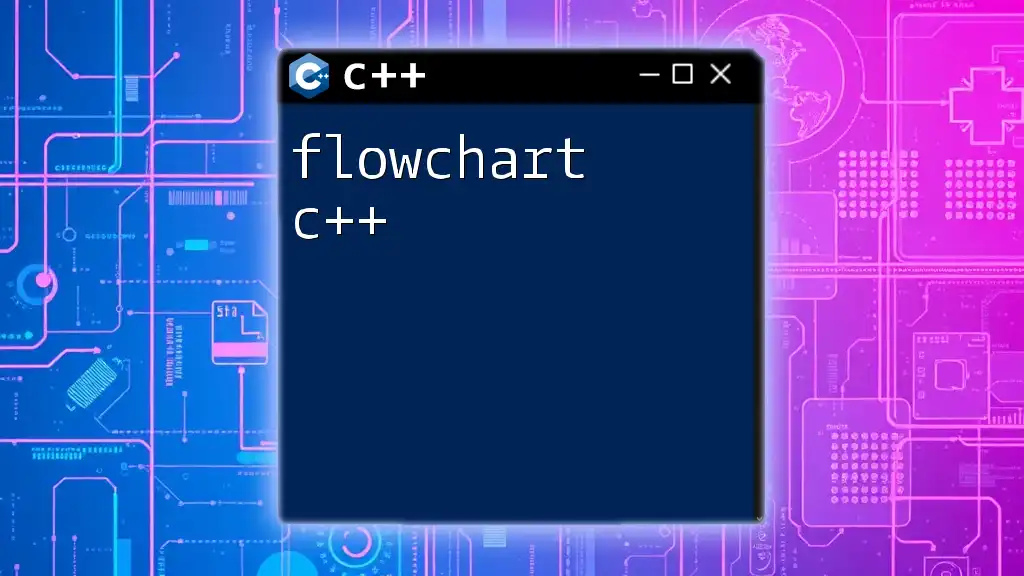
Building Agents Using LangChain C++
Understanding Agents
Agents in LangChain act as the orchestrators of commands and interactions. They interpret user requests and determine which commands to execute based on predefined logic. Agents are crucial for automating tasks and making applications more responsive.
Creating Your First Agent
To create an agent in LangChain, follow this example:
Agent my_agent("example_agent");
my_agent.add_task("task1", "some task details");
my_agent.run();
Walkthrough of the Code Snippet:
- Agent my_agent: This line invokes a new agent instance.
- add_task: Tasks associated with the agent are defined here, enabling the agent to execute specific operations.
- run: The agent begins processing its assigned tasks.
This fundamental example illustrates how to leverage agents in LangChain to build structured behaviors within applications.
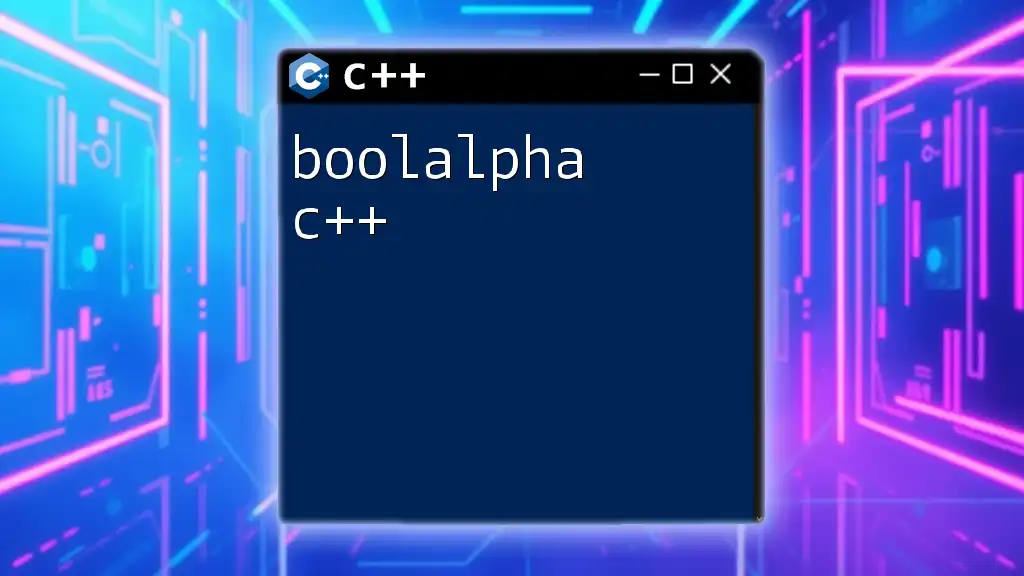
Integrating LLMs with C++
The Role of LLMs
Large Language Models (LLMs) provide the linguistic capabilities vital for natural language processing. They allow applications to engage users in a conversational manner, making them extremely useful for chatbots, automated assistants, and other interactive systems.
Example of LLM Integration
Integrating an LLM into a LangChain application can be done as follows:
LLM model("gpt3");
std::string response = model.generate_response("Hello, how can I help?");
Here, the LLM instance is created with a specific model (e.g., GPT-3), and the `generate_response` method is used to produce a contextually relevant reply based on user input. This allows for rich interactions powered by advanced linguistic capabilities.
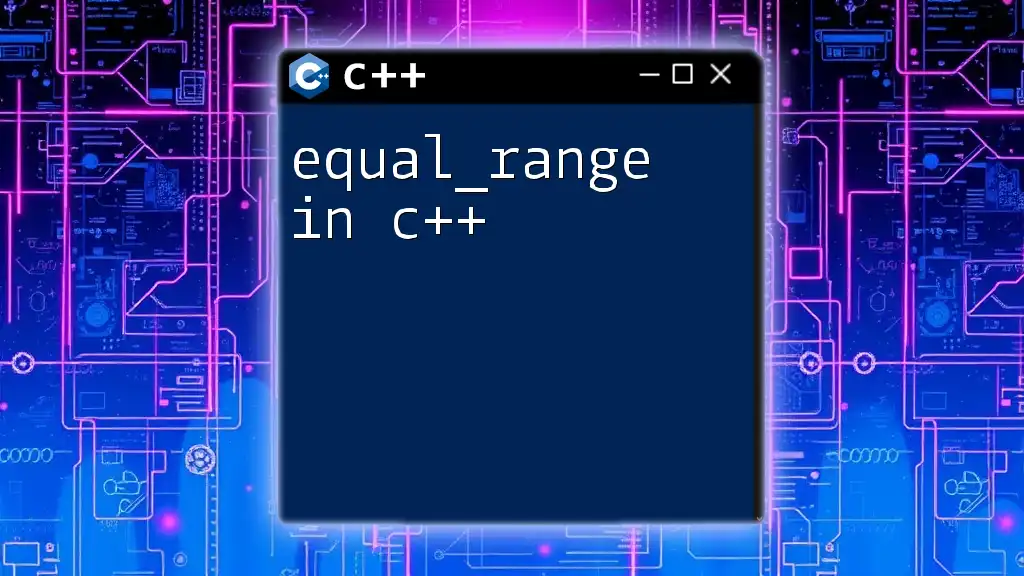
Best Practices for Using LangChain in C++
Code Readability and Maintainability
When developing with LangChain, following best practices for code readability and maintainability is essential. This includes using meaningful variable names, consistent indentation, and adequately commenting your code to make it easier for others (and your future self) to understand.
Performance Optimizations
C++ offers several methodologies for optimizing performance, such as minimizing memory usage and efficiently handling data structures. Profiling your application can help identify bottlenecks, allowing for targeted optimizations that enhance the user experience.
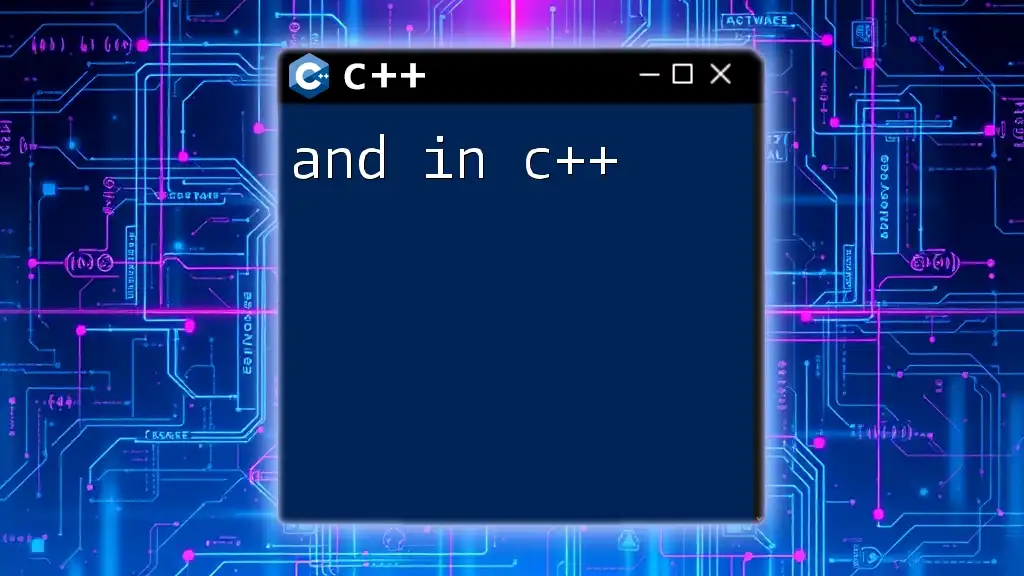
Troubleshooting Common Issues
Common Errors and Fixes
As with any programming effort, issues may arise when using LangChain with C++. Common compilation errors can stem from incorrect header inclusions or dependency misconfigurations. Runtime errors could include memory access violations or exceptions when handling invalid data.
Community and Support Resources
The LangChain community is a valuable resource for developers facing challenges. Online forums, official documentation, and community-contributed guides offer support and insights. Additionally, participating in these platforms allows individuals to learn from others' experiences and contribute their knowledge.
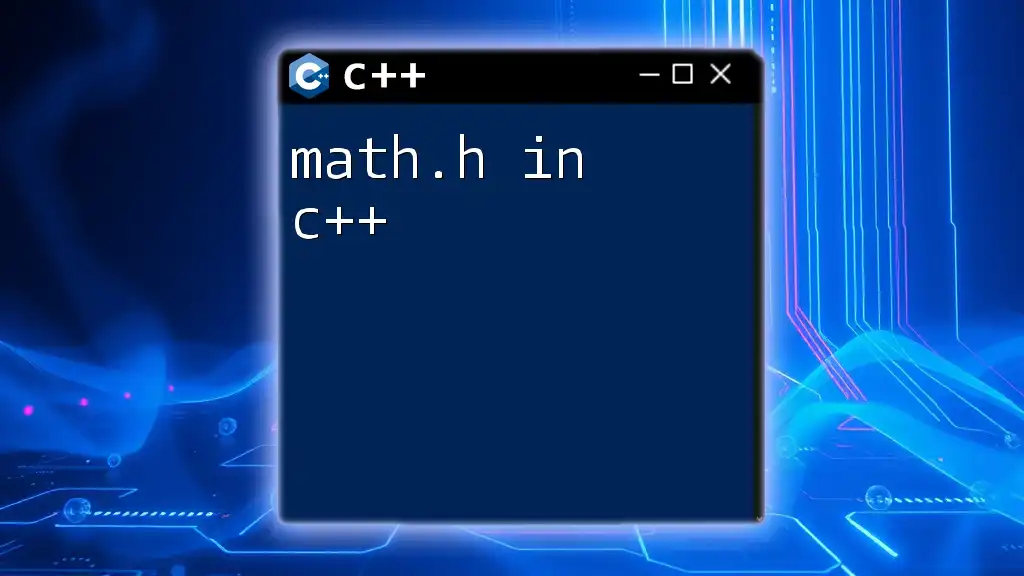
Conclusion
This guide has walked you through the essential facets of LangChain C++, highlighting its architecture, usage, and best practices. By understanding how to implement commands, memory management, agents, and LLM integration, you are better positioned to create powerful applications that utilize the strengths of both LangChain and C++.
Embark on your journey by experimenting with LangChain in your projects, and watch your development become more efficient and intuitive. With these foundational skills, you are well on your way to crafting innovative applications that leverage cutting-edge language processing technologies.
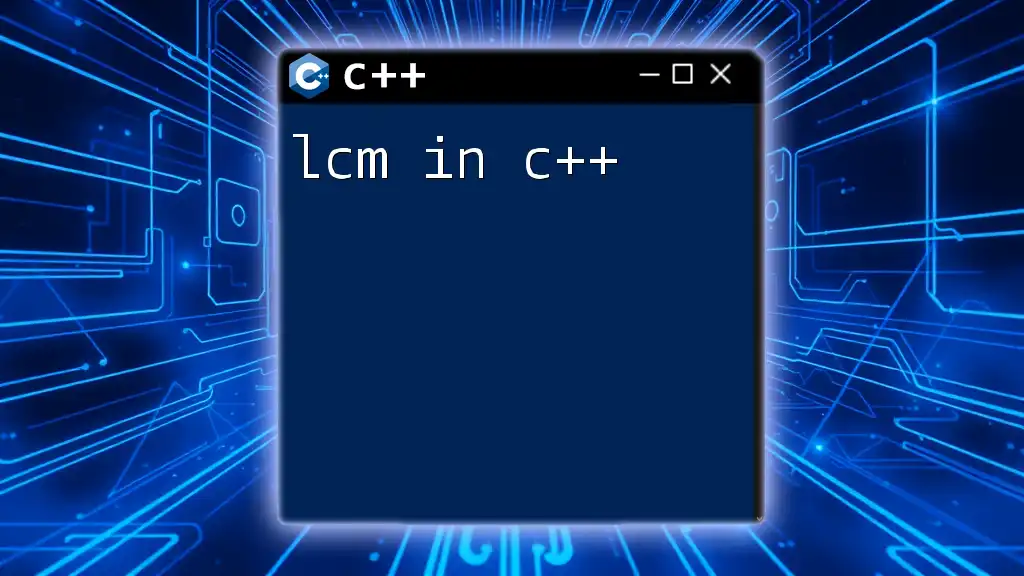
Additional Resources
For further learning, consider checking out the official LangChain documentation and exploring other resources such as recommended books on C++. Staying engaged with the community can also provide you with unique insights and future updates in this fast-evolving field.