ANSI C++ refers to the standardized version of the C++ programming language as defined by the American National Standards Institute, which ensures consistent syntax and behavior across different compilers.
Here's a simple code snippet that illustrates basic input and output in ANSI C++:
#include <iostream>
int main() {
std::cout << "Hello, ANSI C++!" << std::endl;
return 0;
}
What is ANSI C++?
Definition
ANSI C++ refers to the standardized version of C++, which was established by the American National Standards Institute (ANSI). This standardization ensures that C++ writing practices are consistent across different platforms and compilers. Unlike earlier versions of C++, ANSI C++ incorporates a wide array of improvements and features, making it more powerful and versatile for developers.
The Evolution of C++
C++ has evolved significantly since its inception. The initial version was developed by Bjarne Stroustrup in the late 1970s. Over the years, several updates have been made:
- C++98: The first standardized version adopted by ANSI in 1998.
- C++03: A bug-fix release of C++98 in 2003, with no new features added.
- C++11, C++14, C++17, and C++20: Subsequent updates that introduced numerous enhancements and features.
These updates built upon the principles laid out in ANSI C++, broadening its application and usability.
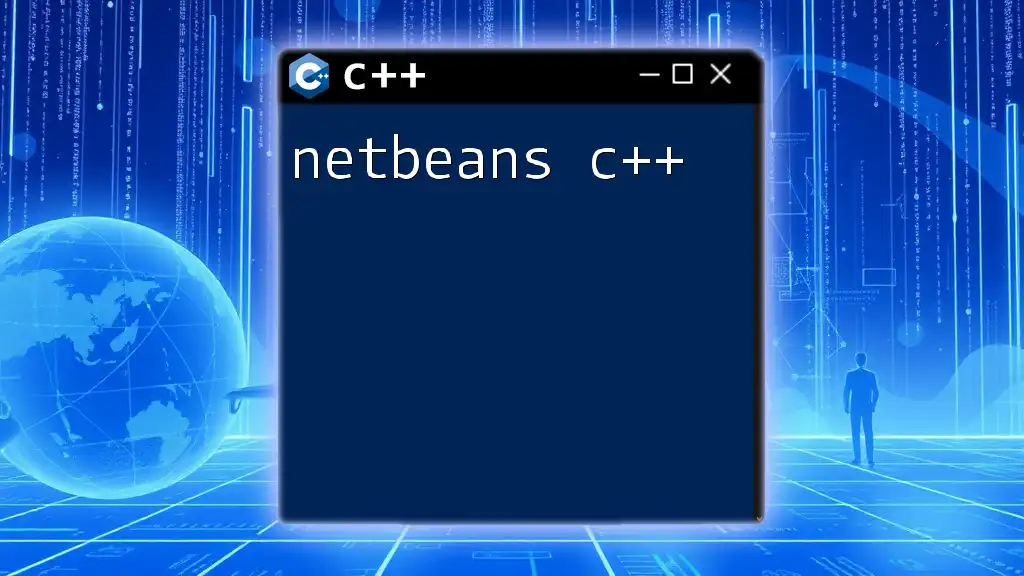
Key Features of ANSI C++
Object-Oriented Programming (OOP)
One of the cornerstones of ANSI C++ is Object-Oriented Programming (OOP). OOP facilitates the creation of applications that are modular, scalable, and maintainable. It encompasses four core concepts:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes that contain data and methods.
- Inheritance: Mechanism to create new classes based on existing ones, promoting code reusability.
- Polymorphism: Ability of different classes to be treated as instances of the same class through a common interface.
To illustrate these concepts, here is a simple code snippet:
class Animal {
public:
virtual void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() override {
std::cout << "Woof!" << std::endl;
}
};
In this example, `Animal` serves as the base class while `Dog` inherits from it. The `speak()` method demonstrates polymorphic behavior where the same method can exhibit different outcomes based on the object that invokes it.
Standard Template Library (STL)
ANSI C++ introduces the Standard Template Library (STL), which provides a robust framework for handling data structures and algorithms. STL enhances the language's capabilities by deploying templates, allowing developers to create generic and reusable code.
Key components of STL include:
- Containers: Basic data structures such as vectors, lists, and maps.
- Algorithms: Predefined procedures for sorting, searching, and manipulating data.
- Iterators: Objects that provide a way to access the data within containers.
Here’s an example showcasing the use of STL:
#include <vector>
#include <algorithm>
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n << " ";
});
In the above code, we utilize a vector as a container and the `std::for_each` algorithm to iterate through the collection, showcasing how STL streamlines data handling.
Exception Handling
Exception handling is a vital feature in ANSI C++ that helps maintain application stability by allowing developers to handle unforeseen errors gracefully. Using try-catch blocks, you can encapsulate code that may trigger exceptions and specify how the program should respond.
Here’s how you can implement exception handling:
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception &e) {
std::cout << e.what() << std::endl;
}
In this example, a runtime error is deliberately thrown. The catch block then handles the exception, printing an error message without crashing the program.
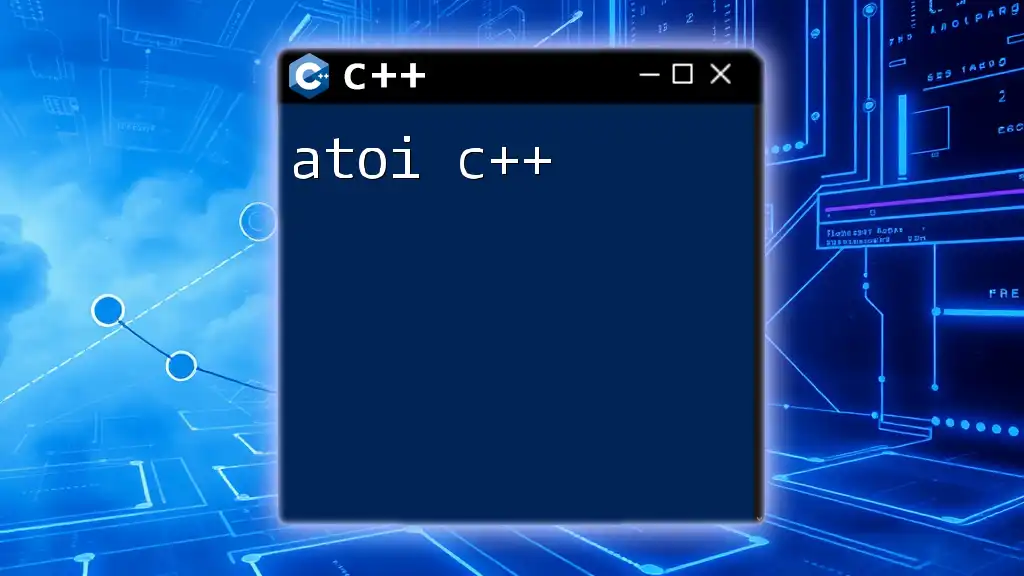
Advantages of Using ANSI C++
Portability
One of the greatest advantages of ANSI C++ is its portability. Programs written in ANSI C++ can be compiled and run on various platforms without significant modification. This cross-platform capability is crucial for developing applications that reach a wide audience and can operate in different environments.
Performance
ANSI C++ is designed with performance in mind. The language allows developers to write highly efficient code, optimized for speed and memory usage. Techniques such as careful memory management and operator overloading can significantly enhance application performance.
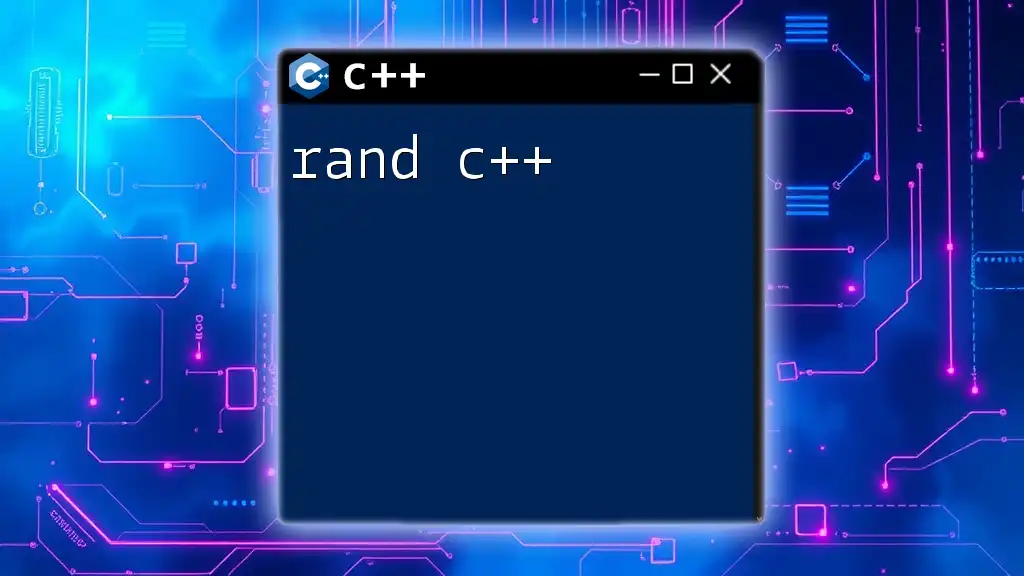
Common ANSI C++ Conventions
Naming Conventions
Adhering to naming conventions improves code readability and maintainability. Here are some guidelines to follow:
- Use camelCase for functions and variables (e.g., `calculateSum`).
- Use PascalCase for class names (e.g., `Animal`).
- Use UPPER_CASE for constants (e.g., `const int MAX_SIZE = 100;`).
Following these conventions makes it easier for you and others to understand your code at a glance.
Code Organization
Organizing your code logically is crucial for maintainability. Group related functions and classes, and structure files in a way that reflects the application’s architecture. For instance, a project might have separate directories for core logic, utilities, and user interface components, allowing for easier navigation.
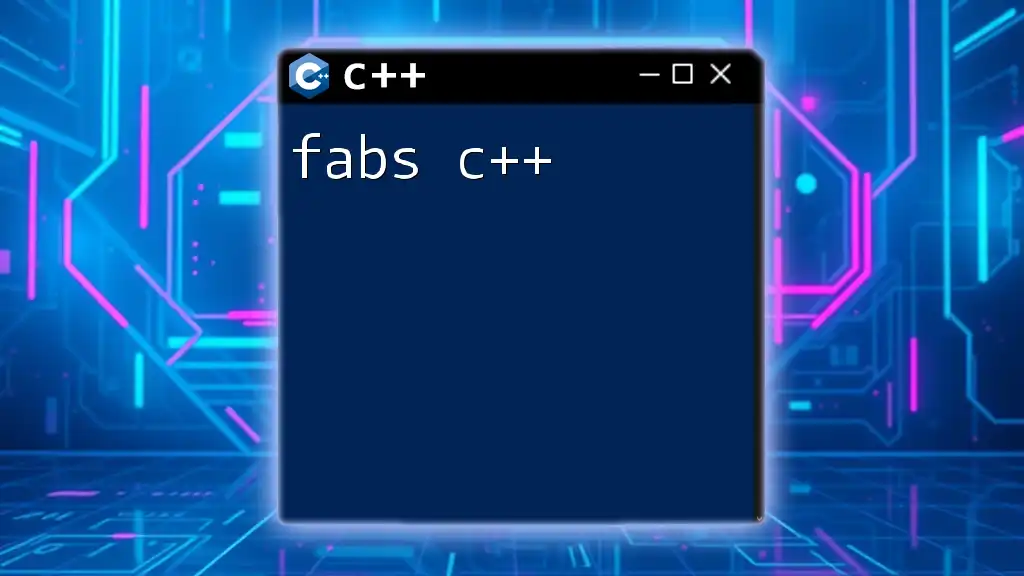
Best Practices in ANSI C++
Writing Maintainable Code
Maintaining code is often more challenging than writing it initially. Focusing on writing maintainable code involves adopting practices such as:
- Keeping functions short and focused on a single task.
- Using meaningful names for variables and functions to convey intent.
- Refactoring code regularly to improve clarity and efficiency.
Effective Use of Comments
Comments play a crucial role in documenting code. However, it’s important to strike a balance. Emphasizing self-documenting code by using clear and descriptive identifiers can reduce the need for excessive comments. When comments are necessary, ensure they explain why a decision was made rather than what the code is doing, as the latter should be clear from the code itself.
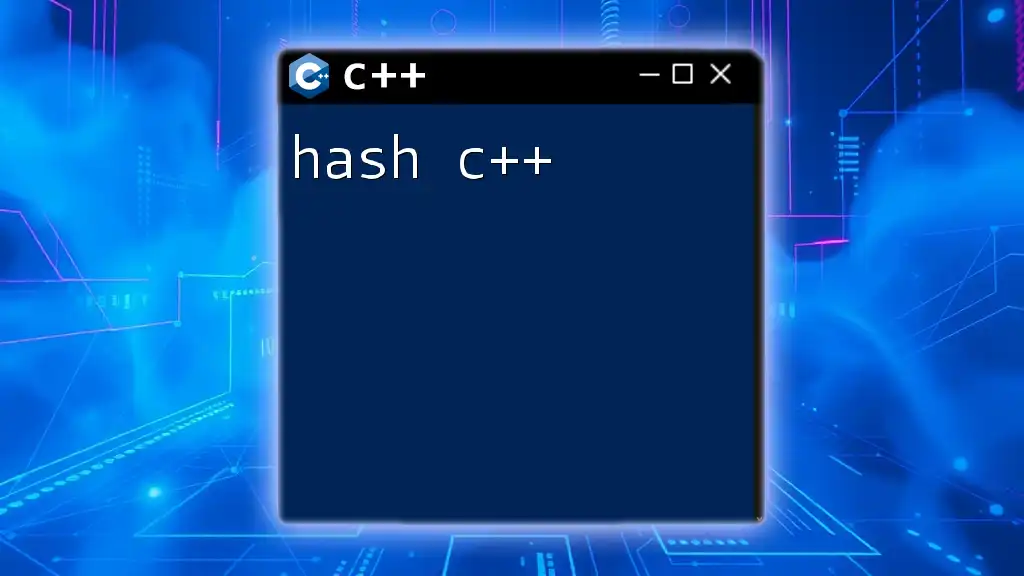
Tools and Resources for Learning ANSI C++
Compilers and IDEs
To get started programming in ANSI C++, selecting the right compiler and Integrated Development Environment (IDE) is essential. Popular choices include:
- Compilers: GCC, Clang, Microsoft Visual C++
- IDEs: Code::Blocks, Microsoft Visual Studio, Eclipse
These tools provide syntax highlighting, debugging capabilities, and other features that ease the coding experience.
Online Resources
Numerous online platforms offer additional learning resources for ANSI C++. Websites like Codecademy and Coursera provide structured courses, while community forums such as Stack Overflow foster discussions that can help clarify concepts. Books focusing on ANSI C++ further deepen your understanding of the language.
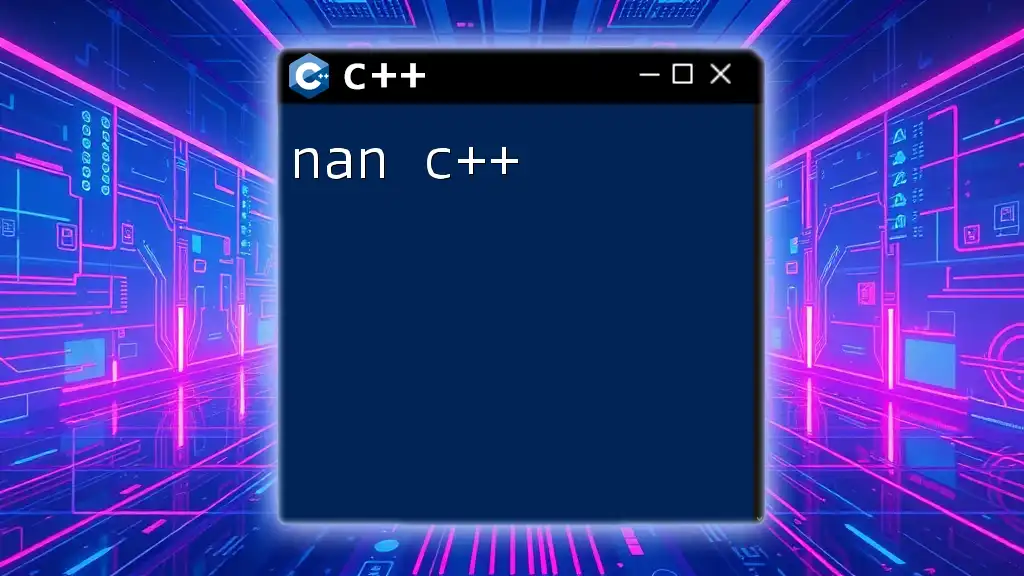
Conclusion
In summary, ANSI C++ forms the foundation of modern C++ programming, offering a wealth of features that facilitate robust, maintainable, and efficient application development. Whether you are a beginner or an experienced developer, diving into ANSI C++ will undoubtedly enhance your programming skills.
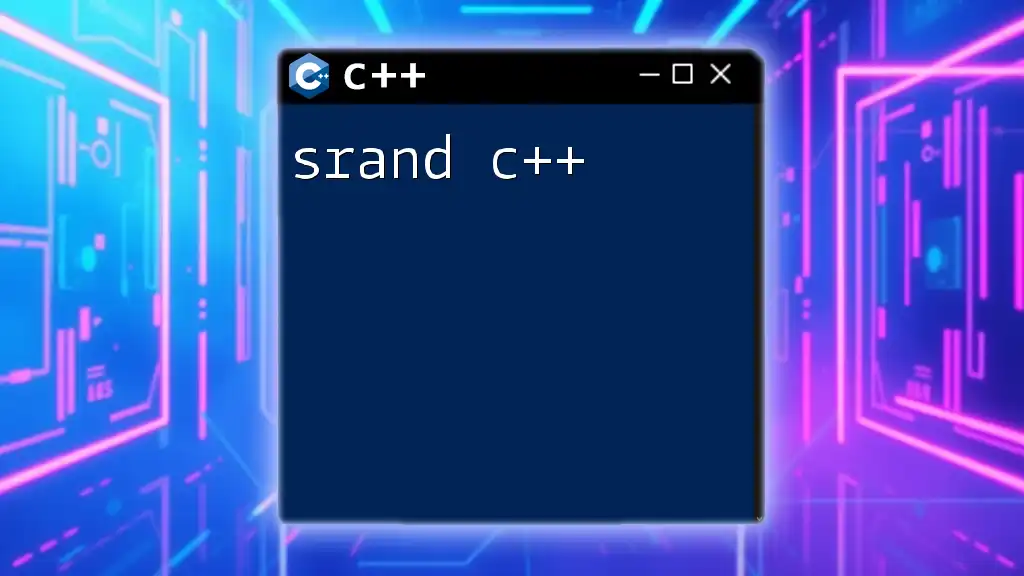
Call to Action
We encourage you to share your experiences with ANSI C++ in the comments below. What challenges have you faced, and how have you overcome them? If you’re looking to deepen your knowledge further, consider signing up for our upcoming courses or engage with our community for questions and collaborative learning opportunities!