Heaps in C++ are a type of data structure that allows for efficient priority queue operations, where elements can be added or removed based on their value, typically using a binary heap implementation.
Here's a simple example demonstrating a max heap using C++'s `std::priority_queue`:
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> maxHeap;
maxHeap.push(10);
maxHeap.push(5);
maxHeap.push(20);
std::cout << "Max element: " << maxHeap.top() << std::endl; // Outputs 20
maxHeap.pop();
std::cout << "Max element after pop: " << maxHeap.top() << std::endl; // Outputs 10
return 0;
}
What is a Heap?
A heap is more than just a pile; in the realm of computer science, it refers to a specialized tree-based data structure that satisfies the heap property. This property can be defined as follows: in a max-heap, for any given node, its value is greater than or equal to the values of its children, while in a min-heap, the value is less than or equal to those of its children. Understanding heaps is crucial as they are widely used in numerous algorithms and programming paradigms.
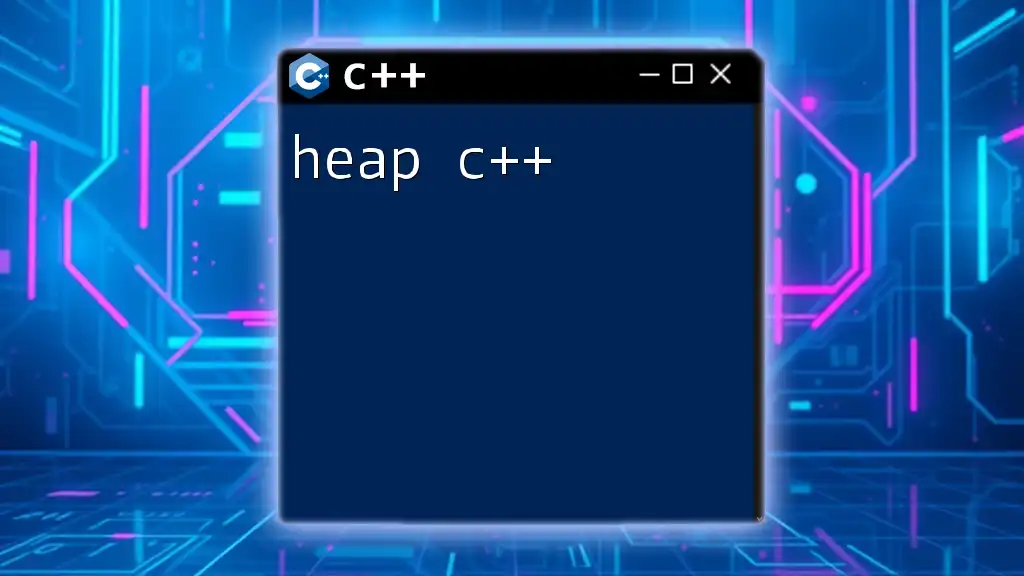
Types of Heaps
Binary Heap
The binary heap is one of the simplest and most commonly used heaps. It follows the following properties:
- It is a complete binary tree, meaning all levels are filled except possibly for the last level, which should be filled from left to right.
- The heap property must be maintained; thus, either a max-heap or a min-heap structure is upheld.
Binary heaps are advantageous due to their efficiency in insertion and deletion operations, both of which occur in O(log n) time.
Fibonacci Heap
A Fibonacci heap is a more advanced form of heap with a more relaxed structure. It allows for more efficient merging of heaps and operations like decrease-key and delete, making it particularly useful in network optimization applications.
Binomial Heap
A binomial heap consists of a collection of binomial trees. It supports efficient merging of two heaps, and like Fibonacci heaps, it allows for the decrease-key and delete operations to be executed more efficiently than binary heaps.
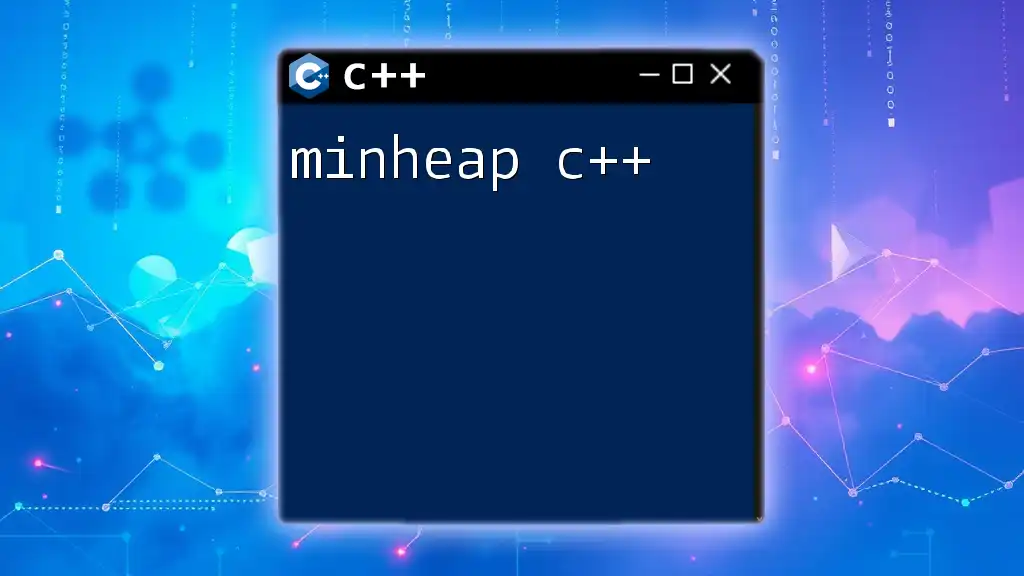
Understanding Binary Heaps
Structure of a Binary Heap
A binary heap is represented as a binary tree, where each node follows the heap property. A commonly seen representation is a complete binary tree. This structure allows an efficient traversal when inserting or deleting nodes.
Heap Properties
The two primary variants of binary heaps are:
- Max-Heap: The value of each node is greater than or equal to that of its children.
- Min-Heap: The value of each node is less than or equal to that of its children.
Understanding these properties is crucial for both the implementation and manipulation of heaps.
Array Representation of Heaps
Heaps can be efficiently represented using arrays. In this representation, for any element at index i, its children can be found at indices 2i + 1 and 2i + 2 (for the left and right children, respectively). The parent node can be located at index (i - 1) / 2.
// Example of array representation of a binary heap
int heap[] = {10, 15, 30, 40, 50, 100, 1000};
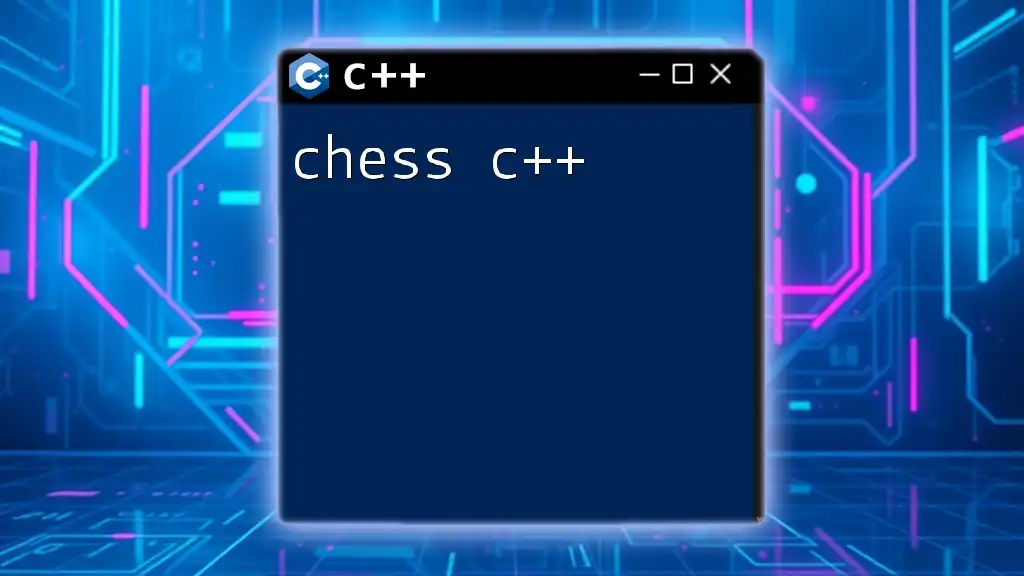
Basic Operations on Heaps
Insertion
Inserting an element into a binary heap involves adding it to the end of the heap (array representation) and then ensuring the heap property is maintained by "bubbling up" the new element.
void insert(int heap[], int& size, int value) {
heap[size] = value; // Add the new element at the end
size++; // Increase the size of the heap
int index = size - 1;
// Up-heapify or bubble up logic
while (index > 0) {
int parentIndex = (index - 1) / 2;
if (heap[parentIndex] >= heap[index]) break; // Maintain max-heap property
std::swap(heap[parentIndex], heap[index]);
index = parentIndex; // Move up the tree
}
}
Deletion
When deleting the root element from a binary heap (usually the maximum value in the case of a max-heap), the last element in the heap replaces the root. The heap must then be restructured through a process called "bubbling down."
void deleteRoot(int heap[], int& size) {
if (size <= 0) return; // Heap is empty
heap[0] = heap[size - 1]; // Replace root with the last element
size--; // Decrease the size
// Down-heapify or bubble down logic
int index = 0;
while (true) {
int largest = index;
int leftChild = 2 * index + 1;
int rightChild = 2 * index + 2;
if (leftChild < size && heap[leftChild] > heap[largest]) {
largest = leftChild;
}
if (rightChild < size && heap[rightChild] > heap[largest]) {
largest = rightChild;
}
if (largest == index) break;
std::swap(heap[index], heap[largest]);
index = largest; // Move down the tree
}
}
Heapify
The process of "heapifying" a section of a tree ensures that it satisfies the heap property. This action is key during building and adjusting heaps.
void heapify(int heap[], int size, int i) {
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < size && heap[left] > heap[largest]) {
largest = left;
}
if (right < size && heap[right] > heap[largest]) {
largest = right;
}
if (largest != i) {
std::swap(heap[i], heap[largest]);
heapify(heap, size, largest); // Recursive heapify
}
}
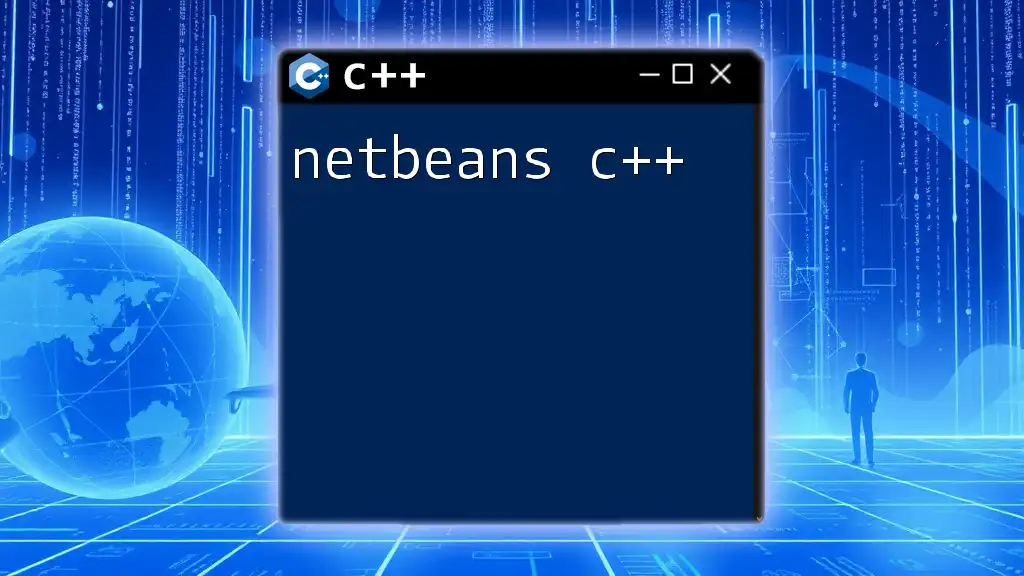
Advanced Heap Operations
Building a Heap
Constructing a binary heap from an unsorted array is done efficiently in linear time using the `heapify` function. Start from the last non-leaf node and work your way up, calling `heapify` on each node.
void buildHeap(int arr[], int n) {
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(arr, n, i);
}
}
Heap Sort
Heap sort is a highly efficient sorting algorithm that utilizes heaps. By turning an array into a max-heap, you can repeatedly remove the largest element and reconstruct the heap until the entire array is sorted.
void heapSort(int arr[], int n) {
buildHeap(arr, n); // Step 1: Build heap
for (int i = n - 1; i >= 0; i--) {
std::swap(arr[0], arr[i]); // Move current root to end
heapify(arr, i, 0); // Call heapify on the reduced heap
}
}
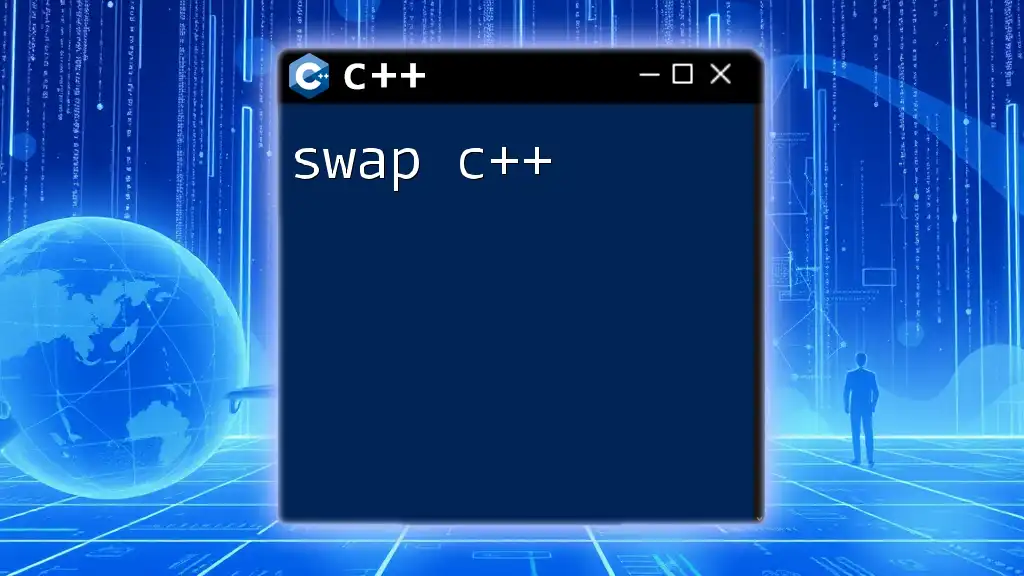
Use Cases of Heaps
Priority Queues
Priority queues are abstract data types that allow for the retrieval of the highest or lowest priority element efficiently. This is primarily accomplished using heaps, as they provide fast insertions and deletions while maintaining order.
Graph Algorithms
Heaps play an essential role in various graph algorithms, particularly Dijkstra's algorithm and Prim's algorithm, which utilize heaps for efficient shortest path calculations and minimum spanning tree computations. By employing heaps, these algorithms improve their efficiency dramatically.
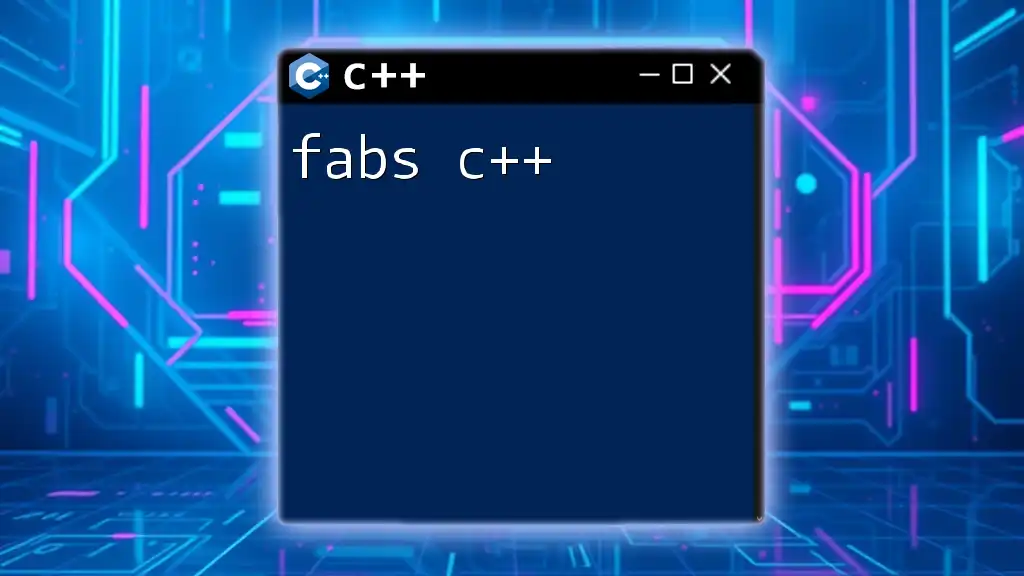
Conclusion
Understanding heaps in C++ equips programmers with a powerful tool for writing efficient algorithms and managing data structures effectively. Heaps are foundational for developing complex applications, from priority queues to graph algorithms. With the above insights and code examples, you now have a solid foundation to implement and work with heaps in your coding endeavors.
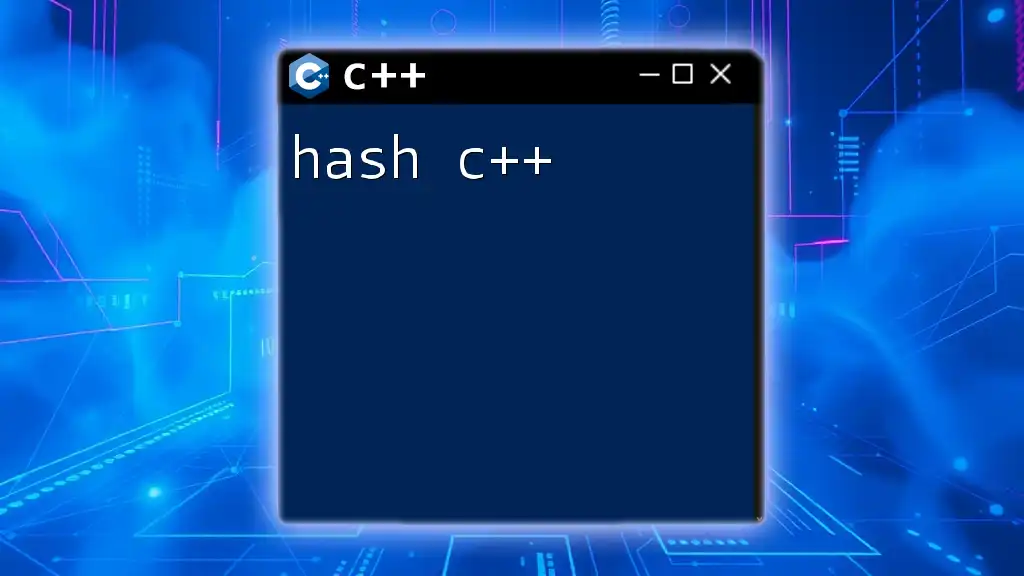
Further Readings and Resources
For those looking to deepen their knowledge on heaps and C++ programming further, consider exploring recommended books, online resources, and courses. Engaging with practical coding challenges will enhance your understanding and expertise.
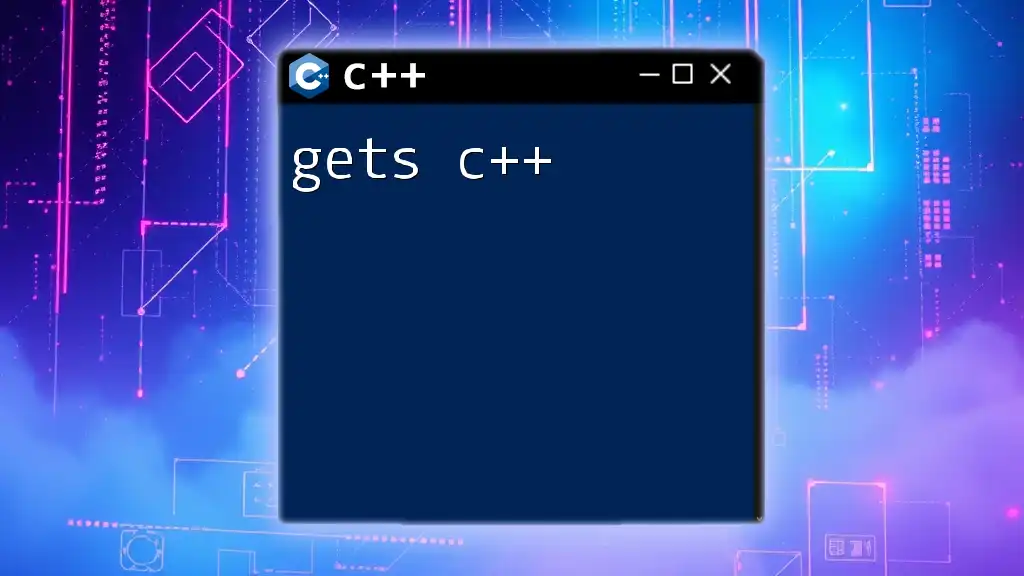
Call to Action
Start practicing heaps through engaging coding challenges and explore more resources tailored to your learning needs. Contact us for customized learning sessions designed to enhance your C++ skills efficiently!