In C++, `.hpp` files are typically used to contain header definitions, allowing for the declaration of classes, functions, and variables that can be included in multiple source files to promote code reuse and organization.
Here's a simple example of a header file (`example.hpp`):
#ifndef EXAMPLE_HPP
#define EXAMPLE_HPP
class Example {
public:
void sayHello();
};
#endif // EXAMPLE_HPP
Introduction to HPP Files
What is an HPP File?
An HPP file, or C++ header file, serves as a central component in C++ programming. Essentially, it defines the structure and interface of the classes, functions, and variables that will be used in your C++ source files. While the primary role of HPP files may resemble that of traditional .h files, they often include additional functionality, such as templates and inline function definitions, making them more versatile.
Importance of HPP Files in C++ Development
HPP files play an essential role in encapsulating and modularizing code. By providing a clear interface, they enable developers to engage with complex systems without delving into the underlying implementation. The benefits of modularity include simplified maintenance, where changes to one part of the codebase can be made independently of others. Furthermore, HPP files enhance code reuse, ensuring that consistent interfaces are available throughout your project.
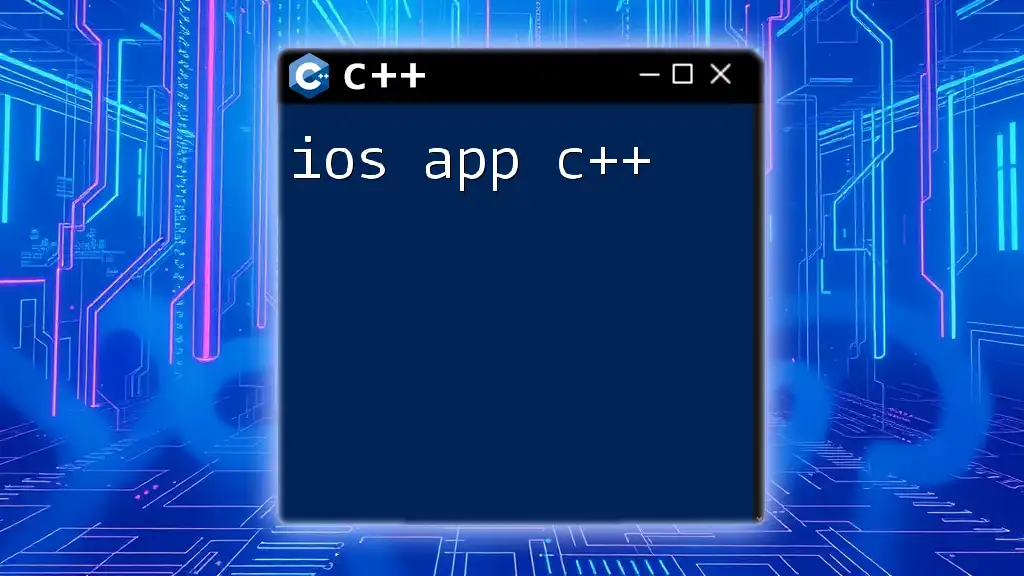
The Basics of Creating HPP Files
How to Create an HPP File
Creating an HPP file involves defining the declarations of your classes and functions. It is critical to follow naming conventions that reflect the file's purpose and content, keeping the filename descriptive.
Here is a basic structure of an HPP file:
// SampleClass.hpp
#ifndef SAMPLECLASS_HPP
#define SAMPLECLASS_HPP
class SampleClass {
public:
void sampleMethod();
};
#endif // SAMPLECLASS_HPP
In the example provided, we have opted for an include guard, which helps prevent multiple inclusions of the same header file in a single compilation.
Including HPP Files
To make use of an HPP file in your C++ program, simply include it at the beginning of your source file with the `#include` directive. This allows you to access the classes and functions defined in the HPP file seamlessly:
// main.cpp
#include "SampleClass.hpp"
int main() {
SampleClass obj;
obj.sampleMethod();
return 0;
}
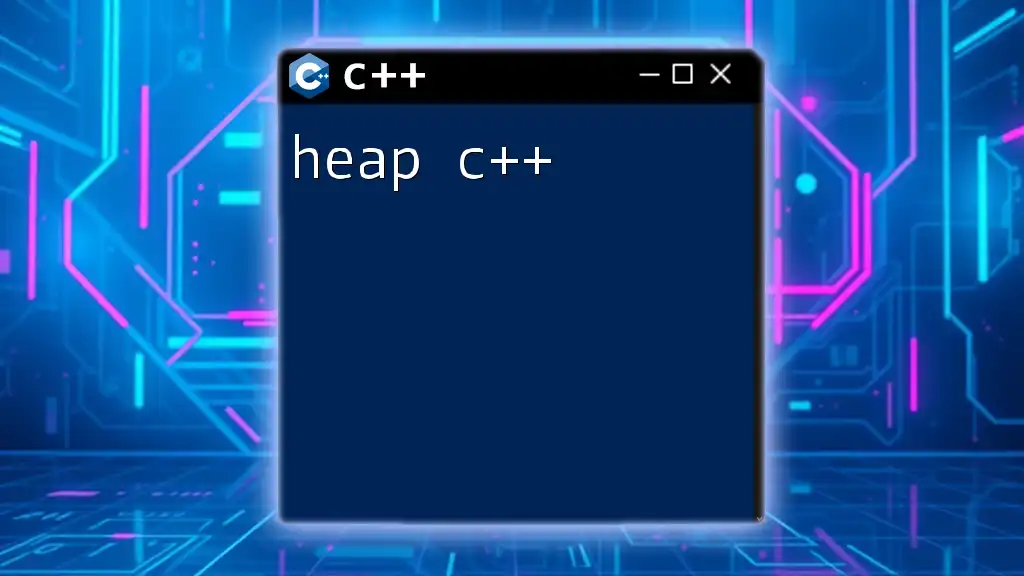
Benefits of Using HPP Files
Separation of Interface and Implementation
One of the most significant advantages of using HPP files is that they allow for a clear separation between the interface (declarations) and implementation (definitions). This structure is particularly advantageous in large projects where multiple developers collaborate. Each developer can work on implementation details in their respective source files (.cpp) while referencing the common interface provided by HPP files. This results in cleaner, more organized codebases.
Facilitating Code Organization
HPP files promote greater organization in C++ projects by enabling a modular programming approach. By grouping related functionality into HPP files, developers can manage complexity better. For example, a graphics program could have separate HPP files for shapes, text, and rendering functionality. This layered approach not only fosters readability but also aids in maintaining and updating the code as the project evolves.
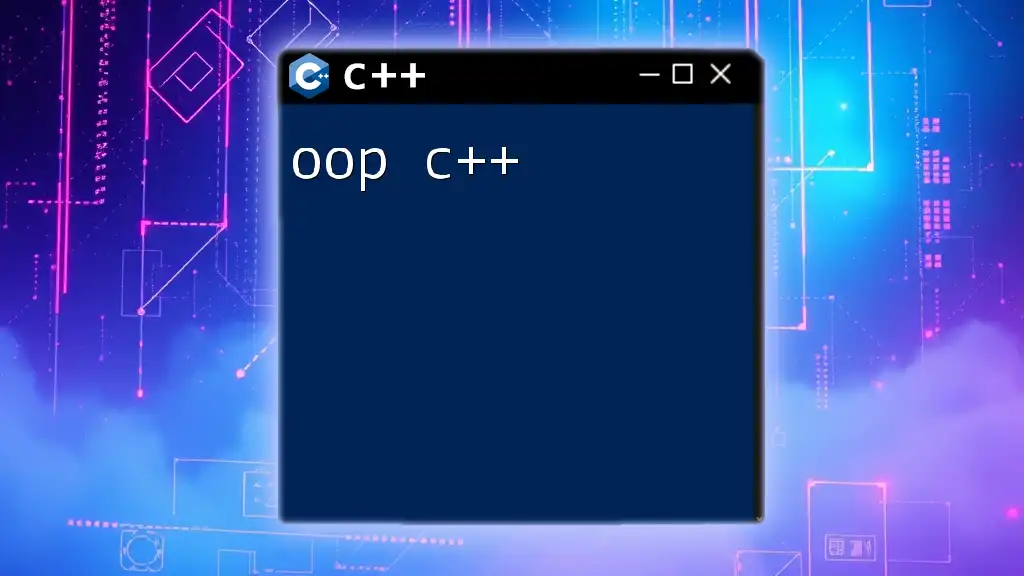
Advanced HPP File Techniques
Templates in HPP Files
Templates are a powerful feature in C++ that allows functions and classes to operate with any data type. This capability can be harnessed in HPP files to create versatile, reusable components. Below is an example of how to define a template function in an HPP file:
// TemplateFunction.hpp
#ifndef TEMPLATEFUNCTION_HPP
#define TEMPLATEFUNCTION_HPP
template<typename T>
T add(T a, T b) {
return a + b;
}
#endif // TEMPLATEFUNCTION_HPP
In this example, the `add` function can accept any data type that supports the addition operator, consolidating multiple function definitions into one.
Namespaces and HPP Files
Using namespaces in HPP files can prevent naming collisions, especially when integrating multiple libraries or large codebases. Here’s an example of how to effectively use namespaces in an HPP file:
// MyNamespace.hpp
#ifndef MYNAMESPACE_HPP
#define MYNAMESPACE_HPP
namespace MyNamespace {
class MyClass {
public:
void display();
};
}
#endif // MYNAMESPACE_HPP
By encapsulating `MyClass` within the `MyNamespace` namespace, you’re ensuring that it doesn’t clash with other class names in the global scope or in other libraries.
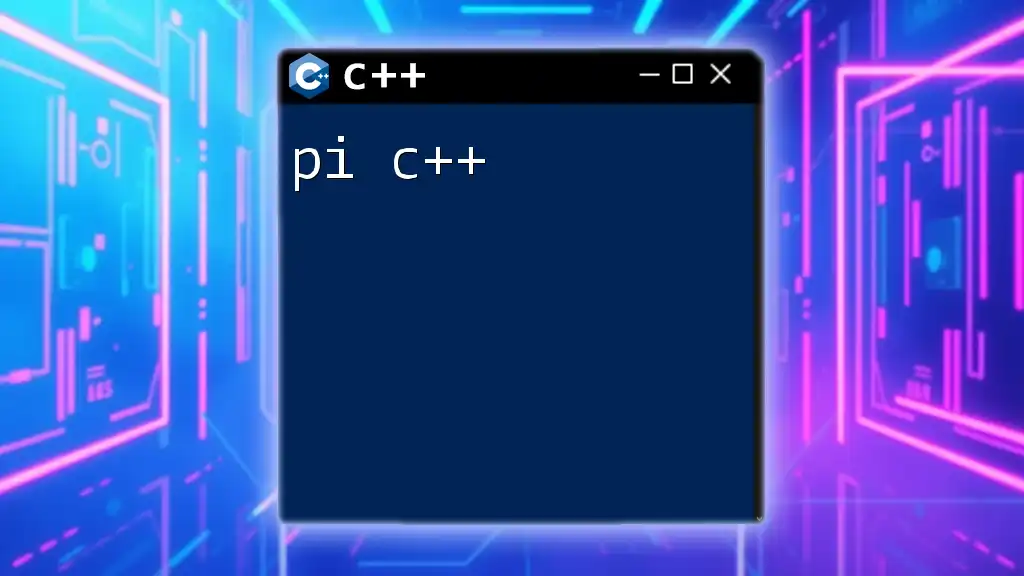
Common Mistakes When Using HPP Files
Neglecting Include Guards
A frequent pitfall among new C++ developers is neglecting to implement include guards. Failure to do so can lead to multiple definitions of the same class or function, resulting in compilation errors. Always remember to use include guards or `#pragma once` to ensure your HPP files are included only once per compilation.
Circular Dependencies
Circular dependencies occur when two or more headers include each other, creating a loop that the compiler cannot resolve. This situation can lead to incomplete type definitions or compilation errors. To avoid circular dependencies, structure your HPP files carefully and consider using forward declarations when necessary.
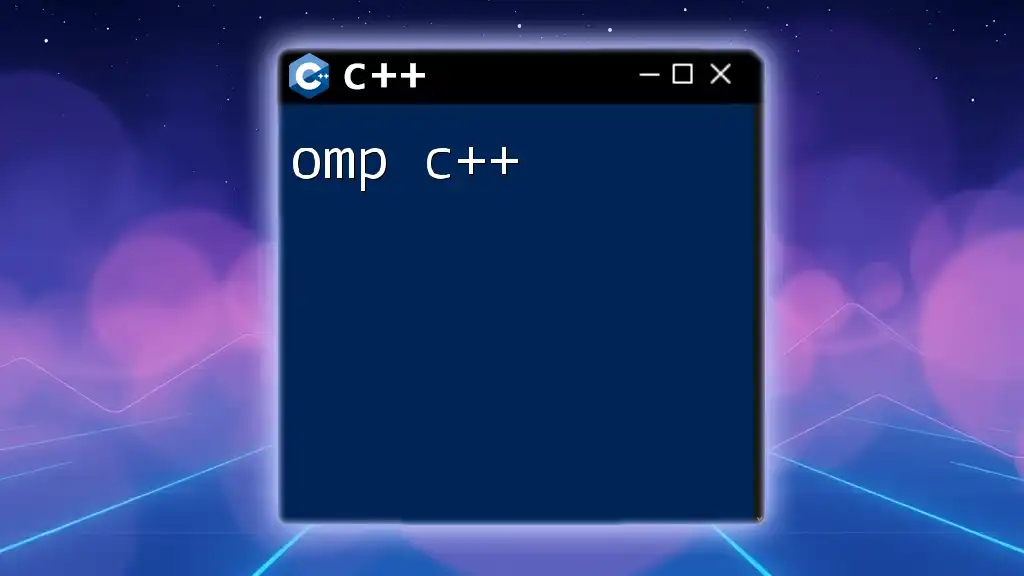
Best Practices for HPP Files
Keep HPP Files Clean and Concise
It is essential to maintain clean and concise HPP files. Each file should ideally contain related declarations, focusing on a specific area of functionality. This constraint helps keep the interface understandable and easy to use, minimizing the cognitive load on other developers.
Documenting Code in HPP Files
Proper documentation is vital in HPP files. Using in-line comments to explain complex logic or outlining the purpose of functions helps others (and your future self) grasp the design intentions quickly. Clear documentation contributes significantly to the maintainability of your codebase. Here’s an example of effective in-line comments:
// SampleClass.hpp
#ifndef SAMPLECLASS_HPP
#define SAMPLECLASS_HPP
class SampleClass {
public:
// Method to demonstrate sample operations
void sampleMethod();
};
#endif // SAMPLECLASS_HPP
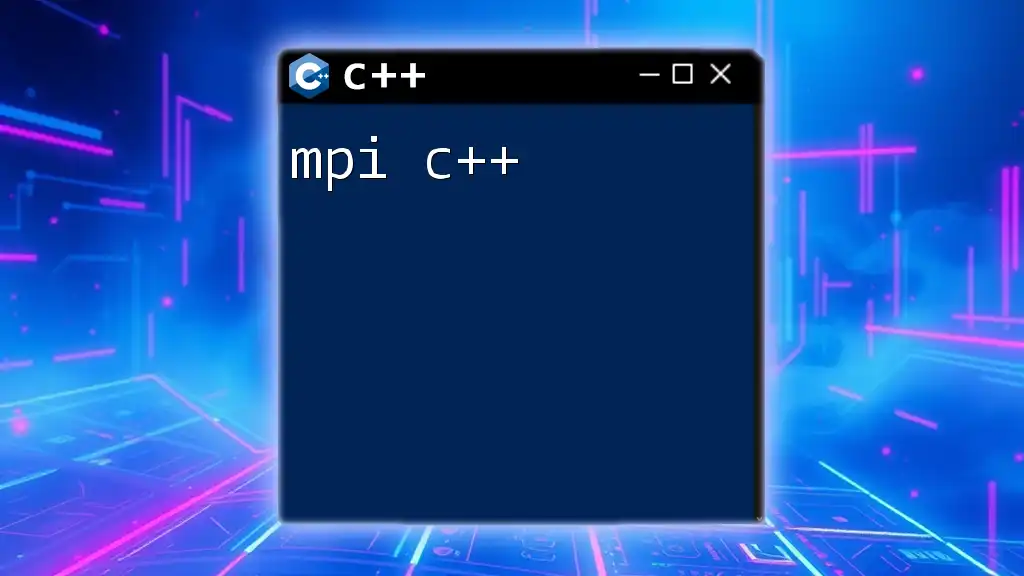
Conclusion
HPP files are an invaluable aspect of C++ programming, playing a crucial role in code organization, encapsulation, and reusability. Understanding how to create, utilize, and manage them effectively can significantly enhance your development process. As you progress in your C++ journey, experimenting with HPP files will deepen your understanding and improve your coding practices.
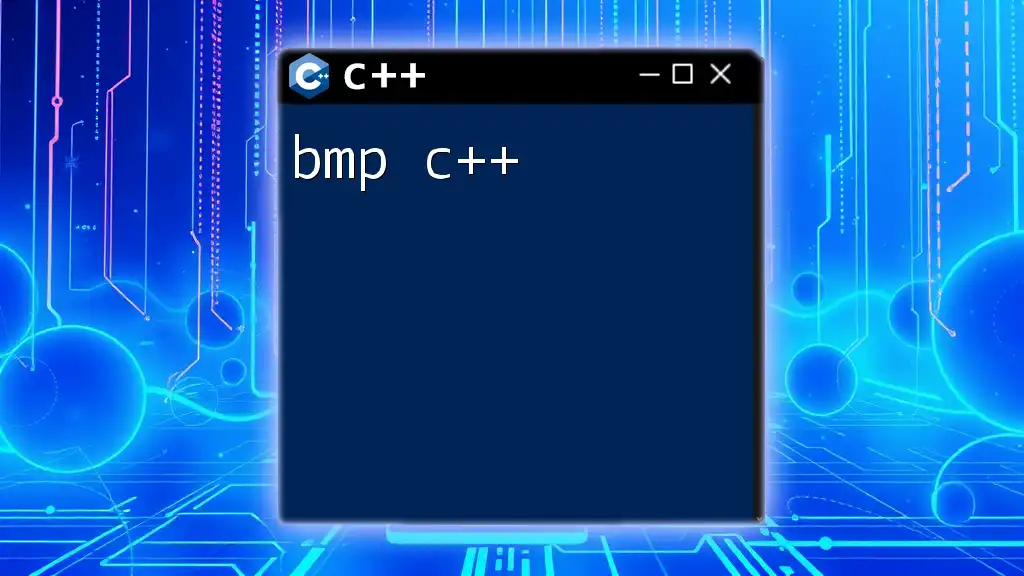
Additional Resources
Links to Further Reading
- Look for recommended resources online, including books and tutorials focused on advanced C++ programming techniques.
Community and Tutorials
Engaging with the C++ community through forums and attending online courses can provide additional insights and support as you continue to learn and grow as a C++ developer.