C++ can be used to develop iOS apps through the use of Objective-C++ or by integrating C++ libraries within a Swift or Objective-C project, allowing for performance-intensive tasks while leveraging the Cocoa Touch framework.
Here’s a simple code snippet that demonstrates how to create a basic C++ function that can be called from Objective-C++:
// MyCPPClass.cpp
#include "MyCPPClass.h"
#include <iostream>
void MyCPPClass::greet() {
std::cout << "Hello from C++!" << std::endl;
}
// MyCPPClass.h
#ifndef MyCPPClass_h
#define MyCPPClass_h
class MyCPPClass {
public:
void greet();
};
#endif /* MyCPPClass_h */
This setup allows you to have C++ functionality in your iOS app, enabling you to combine the strengths of both languages.
Understanding C++ in the iOS Ecosystem
What is C++?
C++ is a powerful, high-performance programming language that supports multiple programming paradigms, including procedural, object-oriented, and generic programming. Its efficiency, combined with the ability to manipulate system resources directly, makes it a preferred choice for applications requiring high performance. Unlike Objective-C and Swift, which are primarily used in iOS app development, C++ can be utilized to develop performance-intensive components.
Why Use C++ for iOS App Development?
There are several compelling reasons to employ C++ in your iOS app development:
-
Performance Benefits: C++ is often faster than Objective-C and Swift due to its compiled nature and fine-grained control over system resources. This is critical for applications that require high throughput and low latency, such as game engines or real-time data processing apps.
-
Code Reusability Across Platforms: By leveraging C++, developers can create code that runs on multiple platforms with minimal modifications. This is especially useful for teams working on cross-platform applications.
-
Large Existing Codebases: Many existing libraries and frameworks are written in C++. By integrating well-established C++ libraries into your iOS app, you can significantly reduce development time while enhancing functionality.
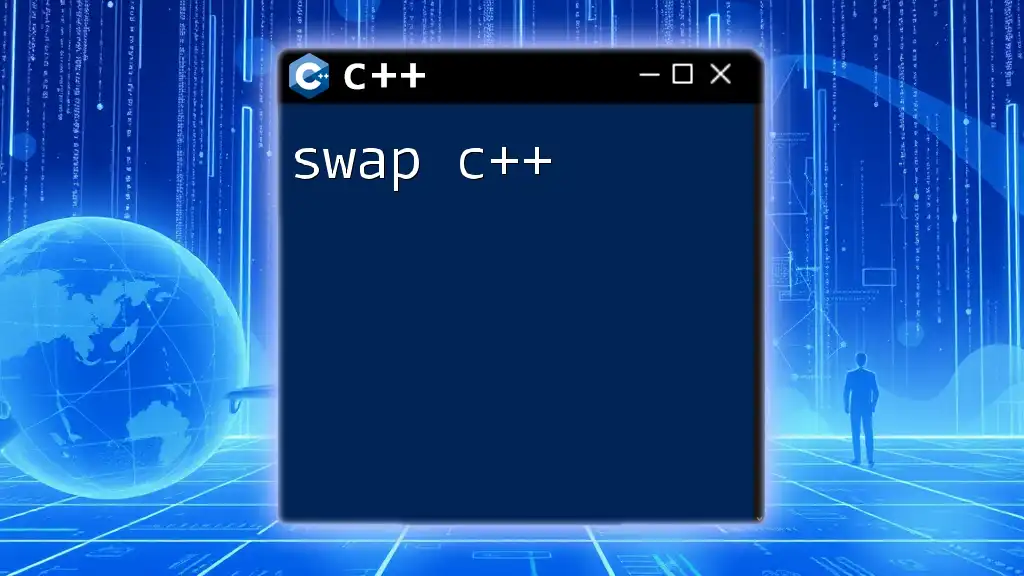
Setting Up Your Development Environment
Required Tools for C++ iOS Development
To get started with developing an iOS app using C++, certain tools are necessary:
-
Xcode: The primary integrated development environment (IDE) for iOS development, which includes support for C++ projects.
-
SDKs: Ensure the latest iOS SDK is installed for utilizing the latest APIs and features.
-
CMake: A cross-platform build system that can help manage builds for C++ projects more efficiently.
Configuring Xcode for C++ Projects
- Open Xcode and select Create a new Xcode project.
- Choose a macOS or iOS project template that best suits your needs. For iOS, select Single View App.
- Ensure to select C++ in the Language dropdown.
- After creating the project, configure build settings to support C++. Pay attention to the following:
- In Build Settings, set C++ Language Dialect and C++ Standard Library to desired options for better compatibility.
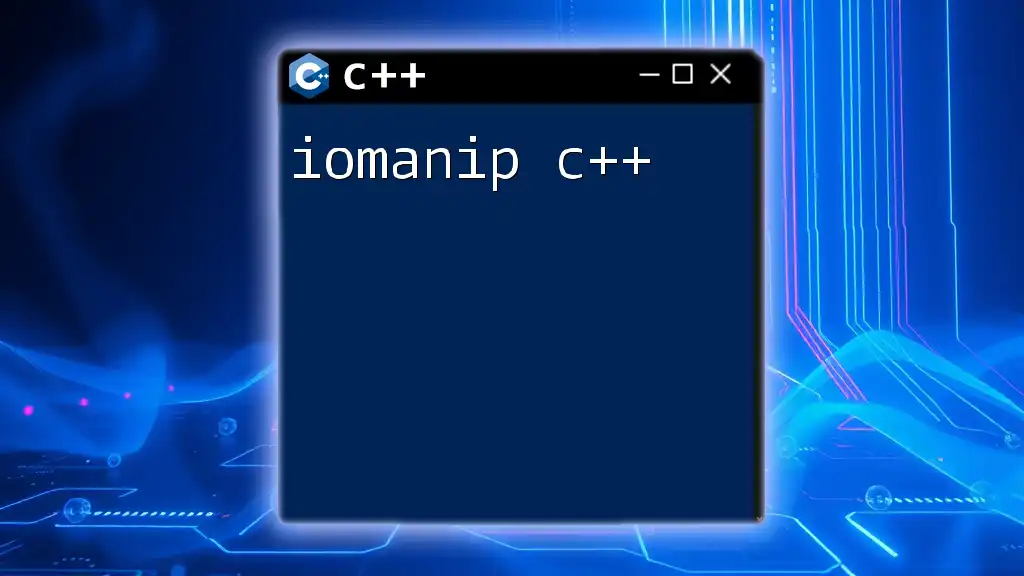
C++ Fundamentals for iOS Development
Key Concepts in C++
To effectively leverage C++ in your iOS app, understanding some core concepts is essential:
-
Object-Oriented Programming Principles: C++ supports encapsulation, inheritance, and polymorphism. Mastery of these concepts will help in creating clean, maintainable code.
-
Memory Management: Unlike garbage-collected languages, C++ requires you to manage memory manually. Utilizing smart pointers can mitigate some risks associated with memory leaks.
Basic C++ Syntax
Understanding C++ syntax is critical. Here’s a quick rundown:
-
Variables and Data Types: C++ supports types such as `int`, `double`, `char`, and complex data structures like structs and classes.
-
Control Structures: C++ includes standard constructs like `if`, `for`, and `while` that help control flow.
-
Functions and Classes: Functions encapsulate behavior, while classes allow you to create user-defined types. Here’s a snippet that demonstrates a simple class definition:
// Example of a simple C++ class
class SimpleCalculator {
public:
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
};
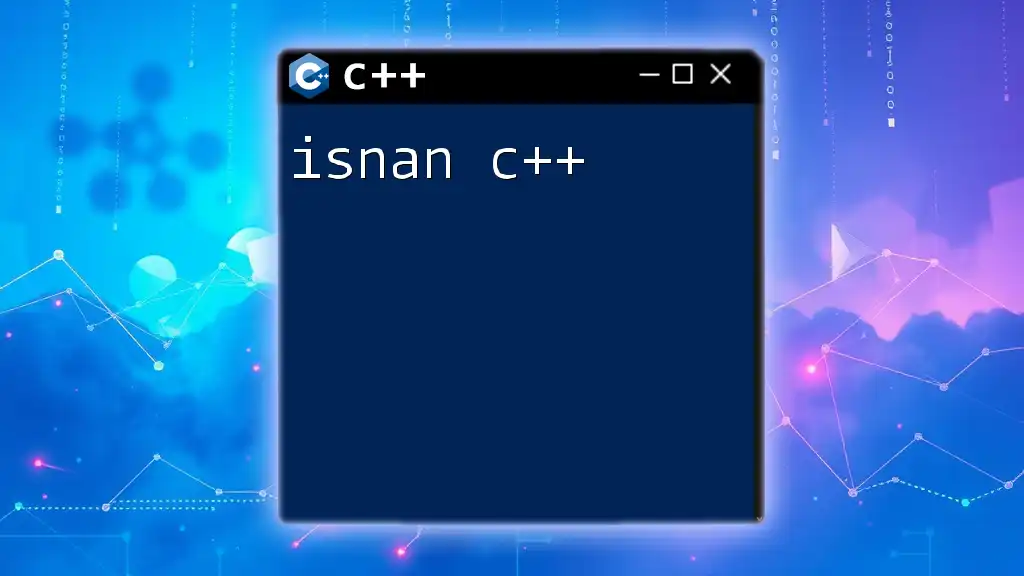
Building Your First iOS App with C++
Integrating C++ with Swift or Objective-C
To make C++ code accessible in Swift, you'll need a bridging header. This allows you to call C++ functions directly from Swift.
- Create a bridging header file in your Xcode project.
- Include C++ headers in the bridging header. Here’s an example:
// MyBridgingHeader.h
#ifndef MyBridgingHeader_h
#define MyBridgingHeader_h
#include "HelloWorld.h" // C++ header file
extern "C" {
void greet();
}
#endif /* MyBridgingHeader_h */
- Next, implement the `greet()` function in your C++ file:
// HelloWorld.cpp
#include "HelloWorld.h"
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, World from C++!" << endl;
}
- Finally, call the function from Swift using the bridging header.
Creating a Simple iOS App
To create a simple iOS app that uses your C++ code:
- Define your project structure with necessary C++ files organized in the appropriate directory.
- Link your C++ logic to the user interface designed using Storyboards in Xcode.
- Use swift code to call the C++ functions. For instance, the greeting function can be linked to a button action in Swift.
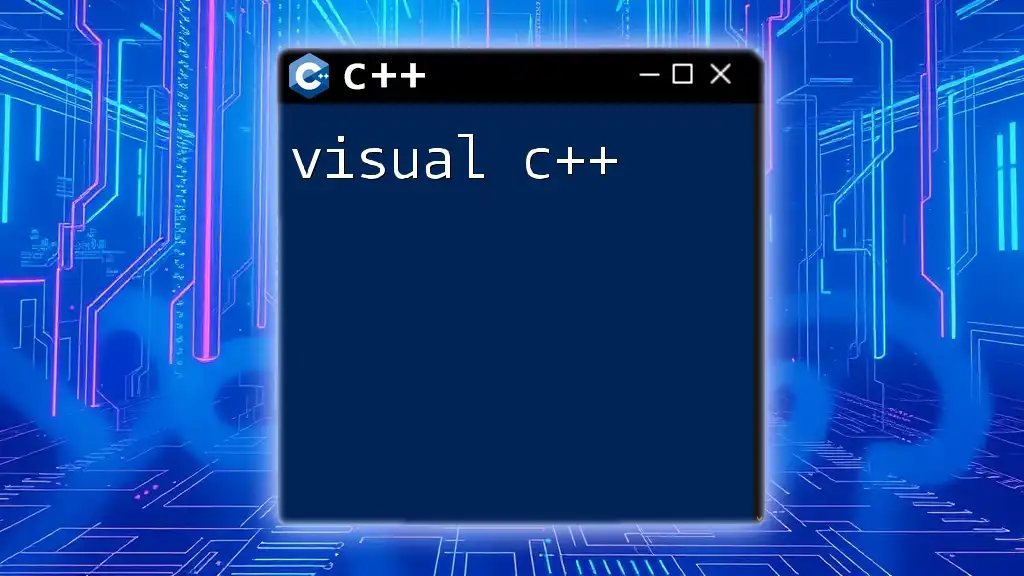
Advanced Topics in C++ iOS App Development
Performance Optimization Techniques
Analyzing and optimizing performance is crucial. Profiling tools within Xcode can help identify bottlenecks. Focus on:
- Memory Management: Utilize smart pointers (`std::shared_ptr` and `std::unique_ptr`) for automatic memory management.
- Multi-threading: Use libraries such as `<thread>` from the C++ standard library to handle concurrent operations and reduce load times for heavy processing tasks.
Leveraging C++ Libraries
Integrating popular third-party libraries such as Boost or OpenCV into your iOS app can expand functionality significantly. To integrate a library:
- Download the library and ensure it is compatible with iOS.
- Set up the library path in Xcode.
- Include the desired headers in your C++ files and use the library functions as needed.
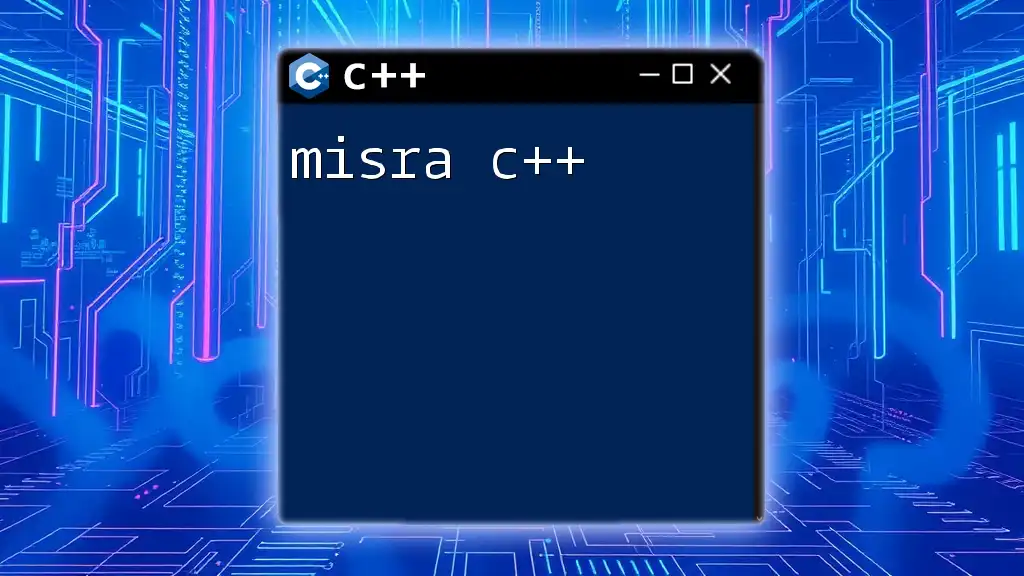
Testing and Debugging C++ Code in iOS Apps
Best Practices for Testing C++ Code
Employ unit testing frameworks such as Google Test to ensure your code remains robust. Create test cases to verify the functionality of your C++ methods, ensuring they behave as expected.
Debugging C++ Applications on iOS
Effective debugging requires understanding Xcode’s debugger. Key techniques:
- Set breakpoints to pause execution and examine variable states.
- Use `cout` for logging intermediate results. This can greatly assist in understanding flow and catching errors.
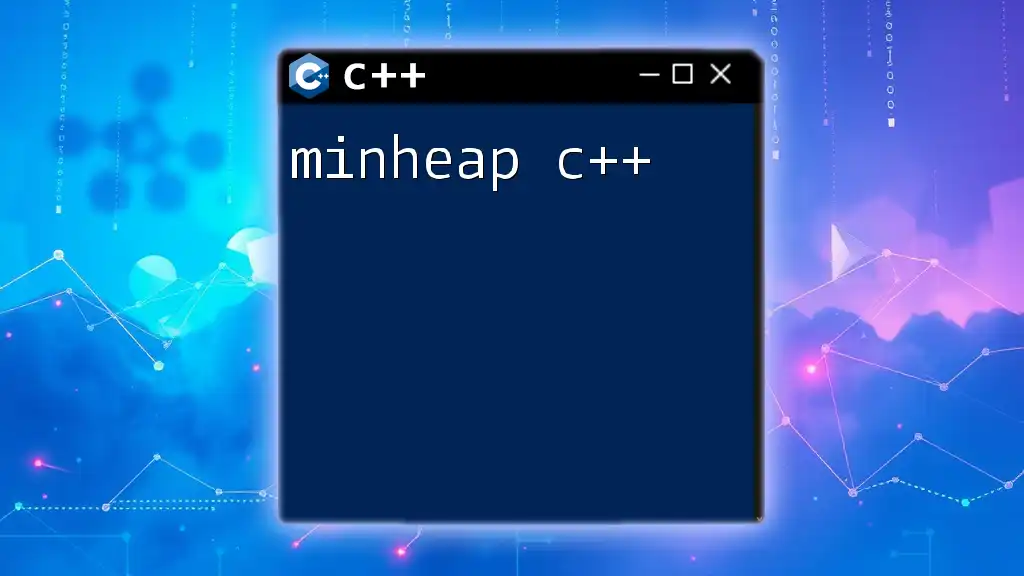
Deployment and Distribution
Preparing Your C++ iOS App for the App Store
Ensure compliance with Apple’s guidelines to avoid rejection. This includes creating a proper app sign and provisioning profile, as well as setting required app metadata.
Post-Launch Considerations
After deployment, focus on user feedback and systematic updates. Incorporate user suggestions into app enhancements, and remain proactive in addressing any bugs or issues.
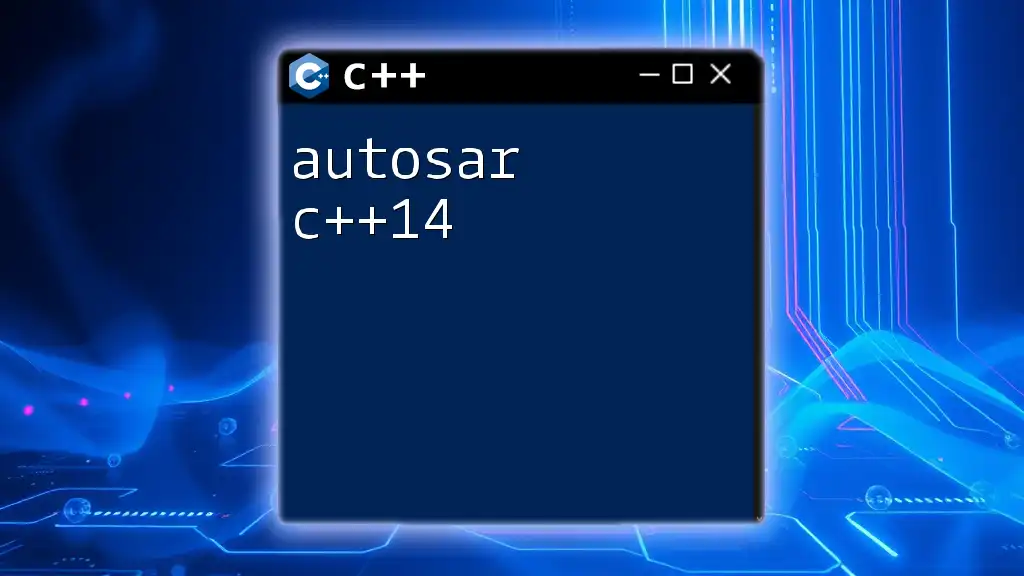
Conclusion: The Future of C++ in iOS Development
Incorporating C++ into iOS app development opens new horizons by blending high performance with robust code management. As the tech landscape evolves, C++ will continue to play a pivotal role in developing powerful, efficient applications across platforms. Engaging with further learning resources will deepen your understanding and expertise in this area, preparing you for the future of app development.
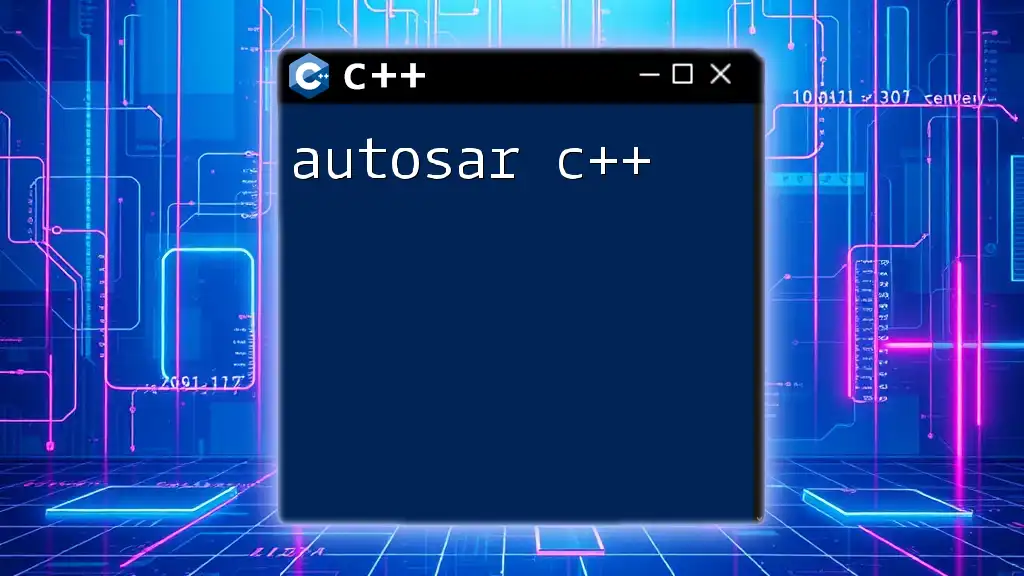
Additional Resources
Build a solid foundation with recommended books, online courses, and active community forums dedicated to C++ and iOS development. These resources can provide invaluable knowledge and opportunities for growth in your programming journey.