In C++, the term "alpha" can refer to a specific check for whether a character is an alphabetic letter, which can be easily performed using the `isalpha()` function from the `<cctype>` library.
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabetic character." << std::endl;
} else {
std::cout << ch << " is not an alphabetic character." << std::endl;
}
return 0;
}
Understanding C++ Fundamentals
What is C++?
C++ is a powerful, high-performance programming language that extends the C programming language by adding object-oriented features. It was developed in the early 1980s by Bjarne Stroustrup at Bell Labs. C++ has undergone significant evolution since its inception, with many updates that have introduced new features and enhancements aimed at improving developer productivity and software performance.
Key Features of C++
C++ stands out in the programming landscape due to several key features:
-
Object-Oriented Programming (OOP): C++ is fundamentally anchored in OOP principles. This approach allows for modular program design, leading to code reusability, scalability, and easier maintenance. Concepts such as encapsulation, inheritance, and polymorphism are pivotal in utilizing C++ effectively.
-
Performance and Efficiency: One of C++'s core advantages over many other languages is its capability for low-level hardware manipulation. Developers have precise control over memory management, making it an excellent choice for performance-critical applications.
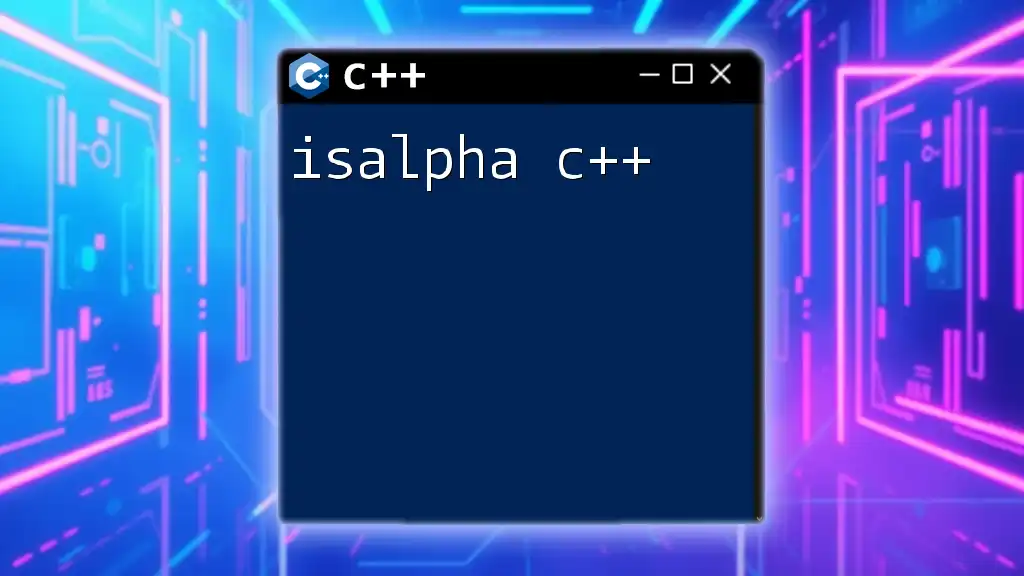
Exploring Alpha C++
What is Alpha C++?
The term "Alpha C++" can refer to developmental stages of C++ features or experimental libraries and frameworks within the C++ ecosystem. Generally, in software development, "alpha" indicates an early version of a program that is not yet stable and may contain a significant number of bugs or incomplete features.
Key Characteristics of Alpha C++
Experimental Features
Alpha versions of C++ might include new syntax or features that have not yet been fully integrated into the official language specification. For instance, developers may experiment with new data structures, algorithms, or paradigms that could enhance performance or ease of use.
Stability and Reliability
It's crucial to understand that using Alpha versions comes with risks. Because Alpha versions are in early development, they may experience frequent changes, leading to potential instability. Developers need to exercise caution and consider the impact of bugs on their projects.
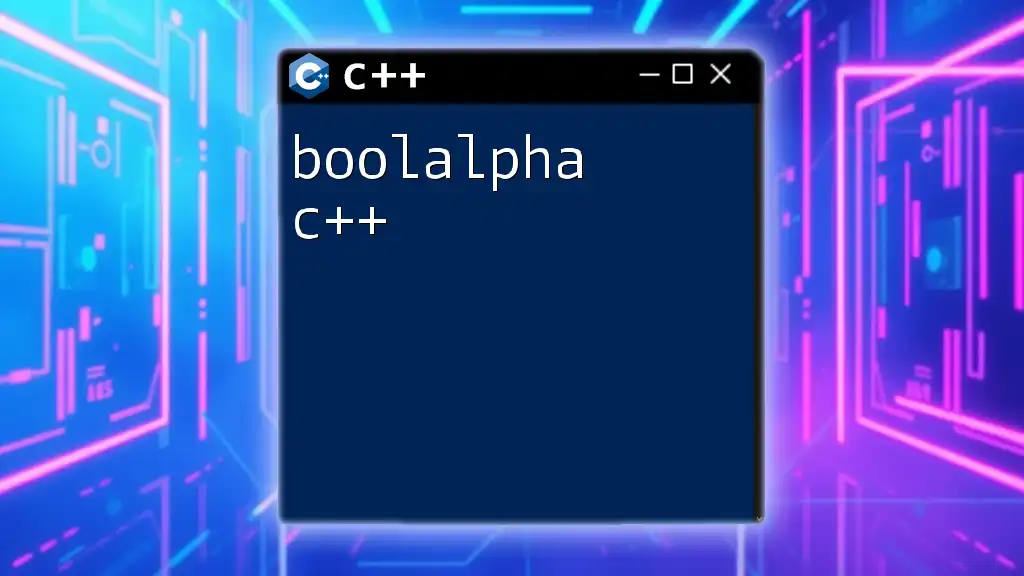
Practical Application of C++
Code Snippet: An Example of C++ Implementing Basic Functions
Let's start with a foundational example—a simple "Hello World" program in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code snippet, we include the `<iostream>` library to use standard input and output features. The `main` function serves as the entry point of the program, where `cout` outputs the "Hello, World!" text to the console. This simple structure showcases C++’s syntax and its execution flow.
Engaging with Alpha C++
Installation and Setup
To engage experimentally with Alpha C++, you need to set up a suitable programming environment. Installation steps may vary based on the specific Alpha features you want to explore. Often, this involves downloading an unsupported or development version of a C++ compiler or integrated development environment (IDE).
Example Code Snippet: Using Alpha Features
Here's a hypothetical example that illustrates the use of an experimental Alpha feature.
#include <alpha_library.h> // Hypothetical example
AlphaClass instance;
instance.alphaFunction();
In this snippet, we hypothetically include a library named `alpha_library` which contains classes and functions characteristic of Alpha C++. The `AlphaClass` could represent new functionality under development, with `alphaFunction` demonstrating how new methods may operate. Such features could be groundbreaking, and experimenting with them can offer insights into what the future of C++ might look like.
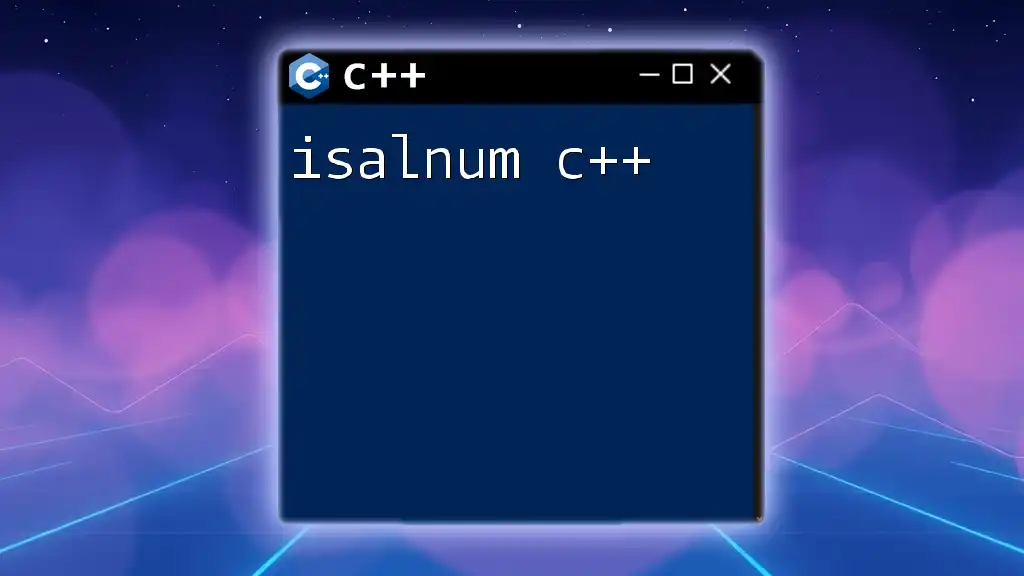
Benefits of Using Alpha C++
Advantages
Engaging with Alpha C++ allows developers to tap into innovative and cutting-edge features. While undergoing the growing pains of new developments, programmers can explore features and functionalities that may greatly enhance their expertise and approach to solving problems.
Community Contributions
When developers use Alpha versions, they can play a crucial role in shaping the language's future. Your feedback can directly influence the final, stable iteration of a feature. You become a part of the community that pushes C++ forward, working collaboratively to refine and improve its capabilities.
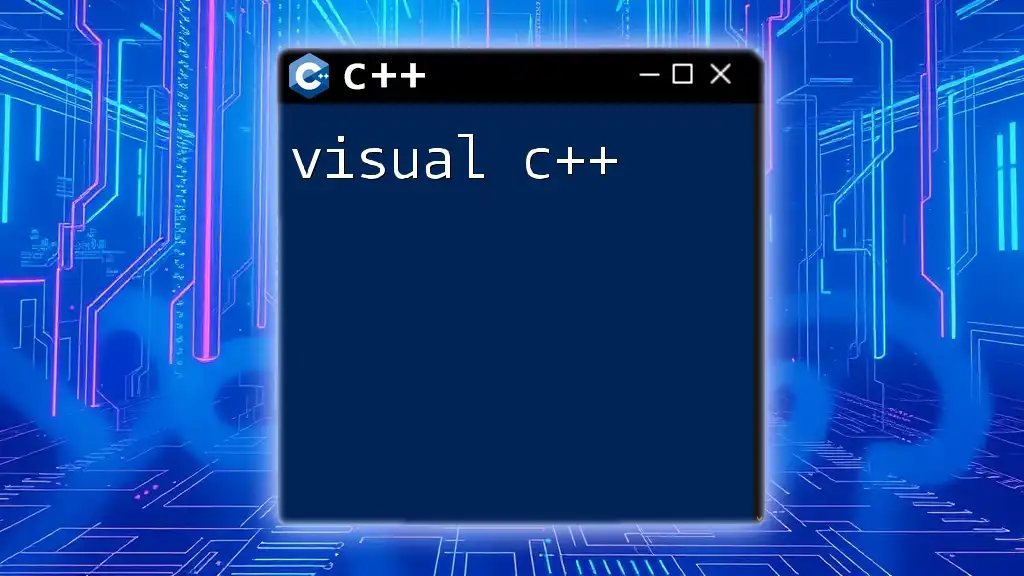
Challenges of Using Alpha C++
Potential Drawbacks
While there are significant advantages to using Alpha C++, it's essential to acknowledge the risks associated with instability and bugs. Because these versions may not have undergone exhaustive testing, they can frustrate developers seeking reliability in their applications.
Compatibility Concerns
As Alpha features evolve, they may be subject to significant changes, which can lead to versioning issues down the line. Code designed for an Alpha version may not be compatible with future iterations. Developers must remain vigilant and be prepared for such transitions.
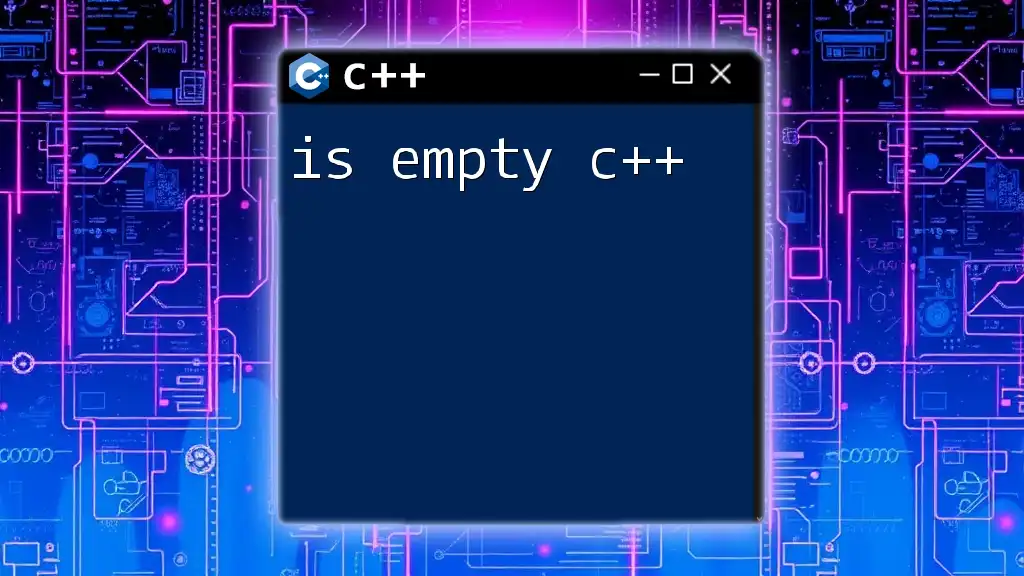
Conclusion
In conclusion, while the question of "is alpha C++?" delves into an area filled with potential challenges, it also opens the door to exciting opportunities. With stability comes innovation, and by engaging with Alpha features, developers can stay on the cutting edge of C++ programming.
Call to Action
We encourage you to explore C++ fully, including any innovative Alpha features that may soon become staples of the language. Through experimentation and curiosity, you can cultivate your skills and contribute to the ever-evolving world of software development.
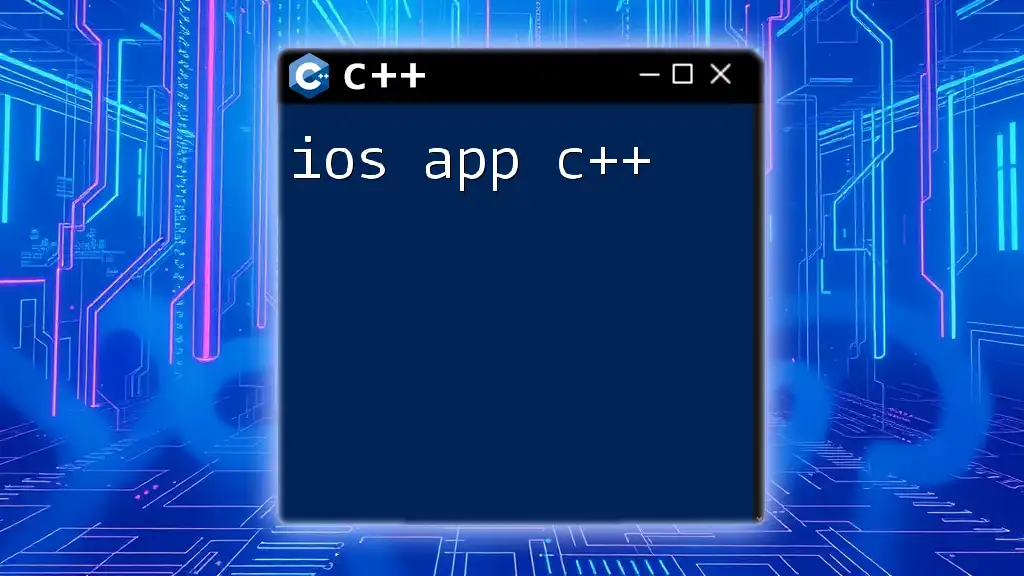
Additional Resources
To deepen your understanding of C++ and engage with its broader community, explore the following resources:
- Official C++ documentation
- C++ forums and discussion boards
- Recommended books and tutorials that cater to varying levels of expertise