Visual C++ 2023 is an integrated development environment (IDE) from Microsoft that enables developers to create and manage C++ applications efficiently using the latest standards and features.
Here’s a simple code snippet demonstrating a "Hello World" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Visual C++ 2023: A Comprehensive Guide
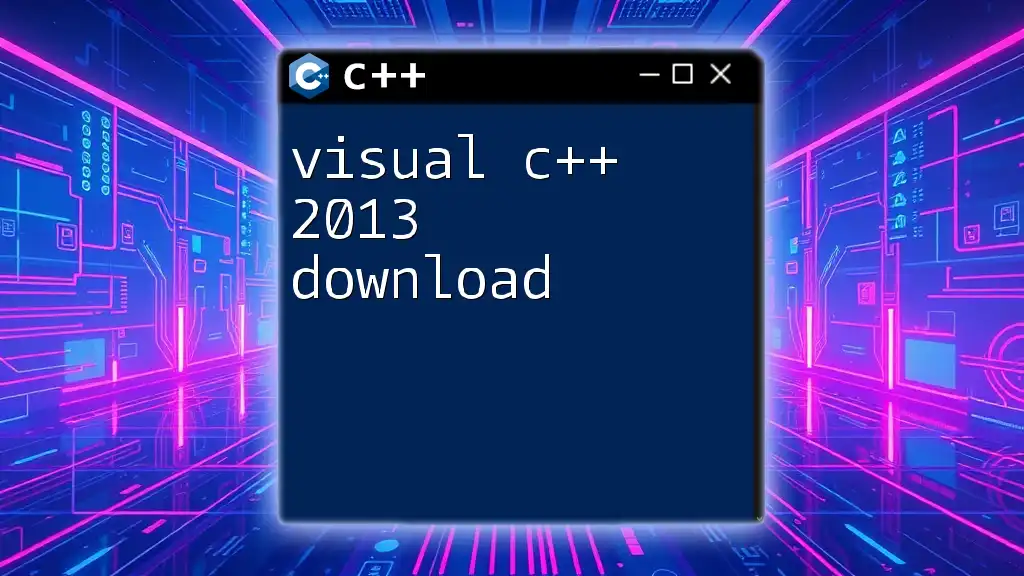
Setting Up Visual C++ 2023
System Requirements
Before diving into Visual C++ 2023, ensure that your development environment meets the necessary system requirements. This includes:
- An operating system: Windows 10 version 1909 or later, or Windows 11
- A minimum of 4 GB RAM (8 GB recommended)
- At least 10 GB of free disk space for Visual Studio installation
Installation Process
To get started with Visual C++ 2023, follow these steps:
-
Download Visual Studio: Visit the official Microsoft Visual Studio website to download the installer for Visual Studio 2023. Choose the Community Edition if you are looking for a free option.
-
Select C++ Workloads: During the installation process, select the "Desktop Development with C++" workload. This package includes the necessary tools and libraries for C++ development.
-
Optional Components: Consider selecting additional workloads or components related to game development or mobile app development depending on your needs.
-
Complete Installation: Finalize the installation by clicking the Install button. This process may take some time depending on your internet speed and system capabilities.
Configuring Your Environment
Once installed, customizing your Visual C++ environment will enhance your coding experience:
-
Workspace Setup: Adjust the layout of the IDE to feature the tools you use most. Arrange the Solution Explorer, Properties, and Output windows to suit your workflow.
-
Theme Customization: Change the theme of the IDE to reduce eye strain, especially if you spend long hours coding. You can choose between the default light theme, the dark theme that minimizes glare, or a high-contrast option for better visibility.
-
Important Settings: Check the options for IntelliSense and code formatting according to your style. It's beneficial to enable automatic formatting on paste, which can help maintain code consistency.
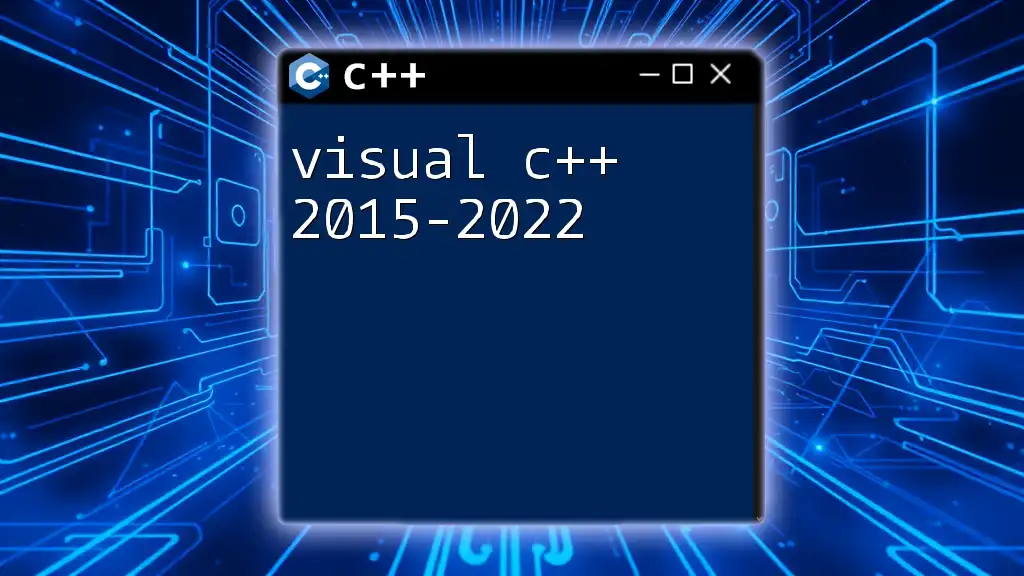
Key Concepts in Visual C++
Understanding Projects and Solutions
A fundamental concept in Visual C++ 2023 is the distinction between projects and solutions. A project typically refers to a single application, while a solution can contain multiple projects. This structure allows you to manage related code efficiently.
To create a new project, navigate to File > New > Project and select "Console Application" (for example) in the C++ options. Follow the prompts to name your project and select a location for saving it.
Basic C++ Command Structure
Understanding the syntax of C++ is essential. Here’s a basic overview of how to declare variables, manage data types, and execute operations.
Consider the following code snippet:
int main() {
int a = 5; // Integer variable
float b = 4.2f; // Float variable
// Basic arithmetic operation
int sum = a + (int)b; // Casting float to integer
return 0;
}
In this example, you create two variables, `a` and `b`, and perform a simple addition, highlighting the importance of variable types and casting.
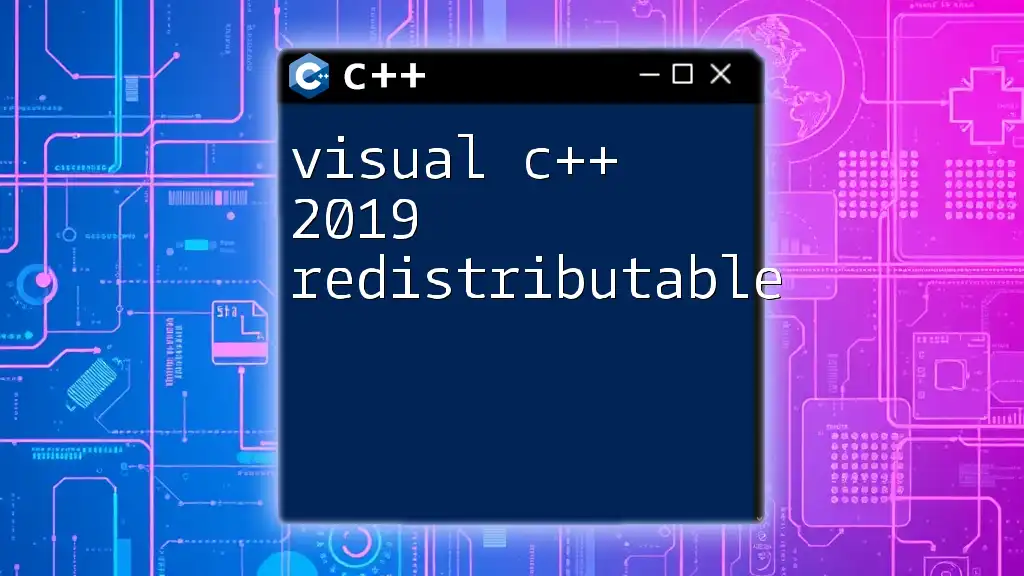
Advanced Features
Enhanced Code Analysis
Visual C++ 2023 comes equipped with advanced code analysis tools that can greatly enhance the quality of your code. These tools analyze your code to identify potential errors, inefficiencies, or non-compliance with best practices.
To run code analysis, right-click on your project in the Solution Explorer, select Analyze > Run Code Analysis on Solution. This feature will generate a report indicating warnings and suggestions for improvement.
Utilizing C++20 Features
With C++20, several new features have been introduced that make coding more expressive and efficient. Key highlights include:
-
Concepts: This is a way to specify constraints on template parameters, allowing for cleaner and more predictable generic programming.
-
Ranges: These allow for more readable iteration compared to traditional loops.
Here’s an example using concepts:
#include <concepts>
template<typename T>
requires std::integral<T>
void printIntegral(T value) {
std::cout << value << std::endl;
}
In this example, the `printIntegral` function can only accept integral types, thereby filtering ineligible types at compile time.
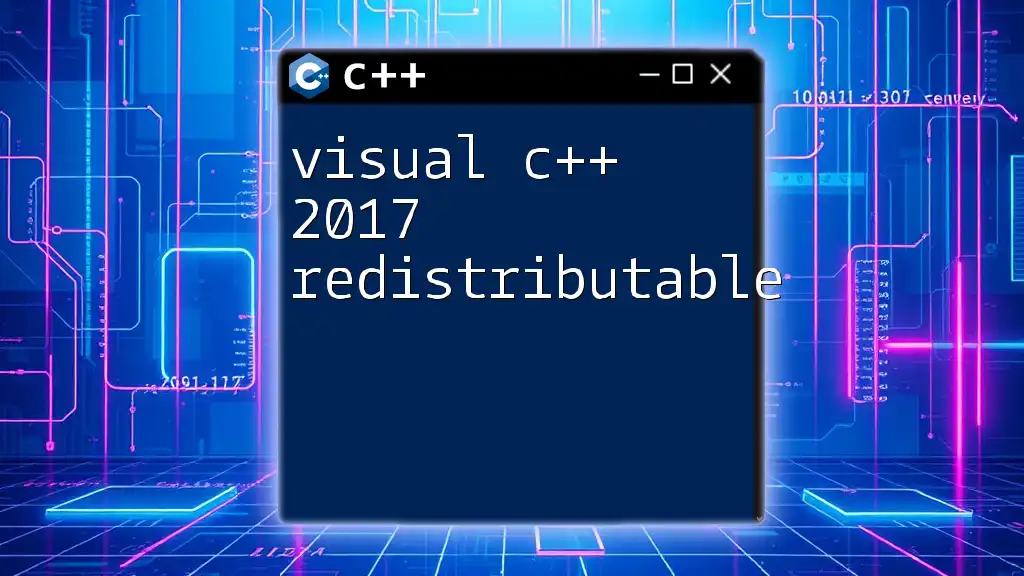
Debugging and Diagnostics
Using the Debugger in Visual C++
One of the standout features of Visual C++ 2023 is its integrated debugger, making it easier to trace and fix issues in your code. Throughout your coding session, you can set breakpoints by clicking the left margin of the code window. This allows you to pause the execution at specific lines and inspect variables at that point.
To view variable values or step through your code line-by-line, use the Debug menu to navigate through the options like Step Into, Step Over, and Continue. Each step allows you to monitor the flow of logic in your application.
Error Handling Techniques
Effective error handling is paramount in C++. By utilizing exceptions, you can catch and manage errors gracefully. The following code exemplifies this practice:
try {
int* arr = new int[99999999999]; // Attempt to allocate a large array
} catch (std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
In this snippet, if the memory allocation fails, a `bad_alloc` exception is thrown, and the relevant error message is printed, preventing the program from crashing ungracefully.
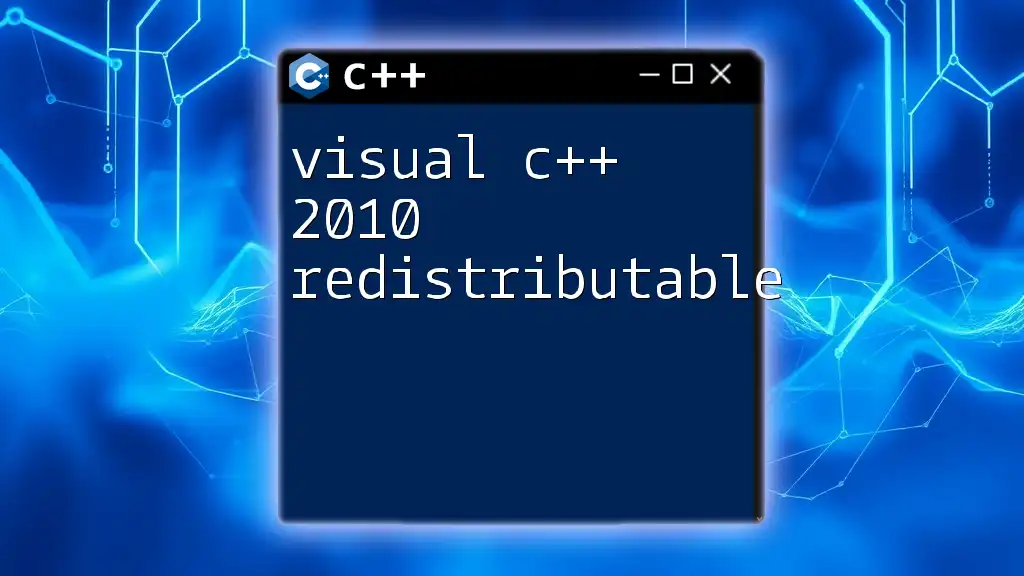
Working with Libraries and APIs
Integrating Standard Library and Boost
Visual C++ 2023 seamlessly integrates with the Standard Template Library (STL), which provides ready-to-use classes and functions. For instance, using `std::vector`.
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Adding a new number
numbers.push_back(6);
`std::vector` offers dynamic resizing, which simplifies management of collections of data.
Using Third-Party Libraries
Boost is among the most popular libraries that extend C++ capabilities. To include Boost in your project:
- Download the Boost library from the official website.
- Unzip and place it in your local directory.
- Add the include directory under your project properties in C/C++ > General > Additional Include Directories.
With Boost available, you can utilize its powerful features for tasks ranging from smart pointers to multi-threading.
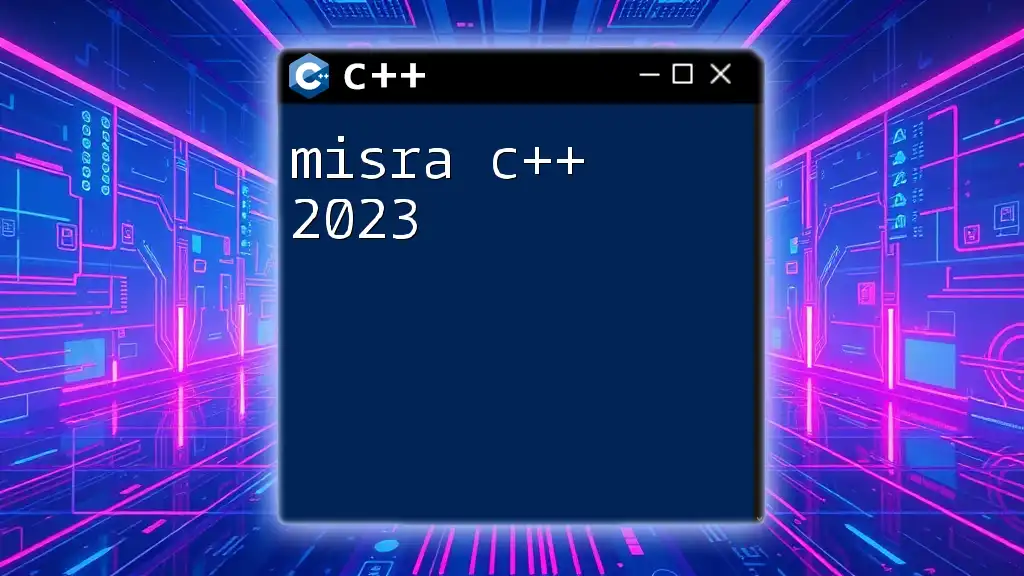
Best Practices for Visual C++ Development
Code Organization and Management
Maintaining a clean code structure is crucial for long-term project success. Organize your code into multiple source and header files, separating functionality (e.g., splitting classes into their respective files) to enhance readability and maintainability.
Writing Efficient C++ Code
In Visual C++, writing efficient code involves using appropriate algorithms, minimizing resource usage, and optimizing loops. Whenever possible, prefer using algorithms from the STL, as they are often more efficient than custom implementations.
Version Control with C++ Projects
Incorporating version control in your projects is essential for managing changes effectively. Git is the most commonly used system. In your terminal or command prompt:
git init
git add .
git commit -m "Initial commit."
Establishing a version control system will significantly enhance collaboration and code integrity throughout the development lifecycle.
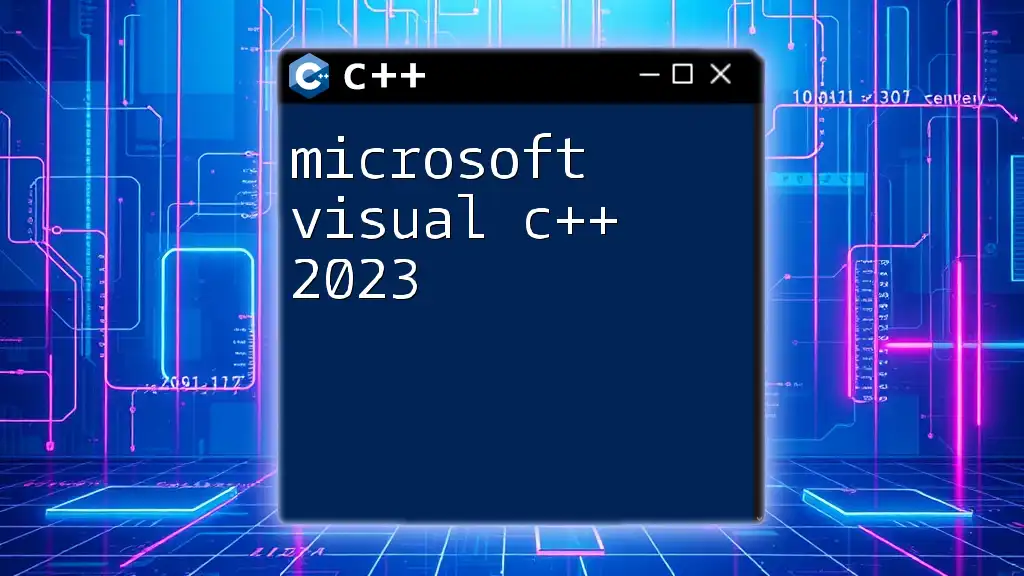
Resources and Community
Learning Resources
While Visual C++ 2023 is a robust tool, continuous learning is vital. Consider engaging with:
- Books: Titles like "The C++ Programming Language" by Bjarne Stroustrup provide in-depth knowledge.
- Online Courses: Websites like Coursera, Udemy, and LinkedIn Learning offer curated video series on C++.
Joining the C++ Community
Becoming part of the C++ community can open up new opportunities for collaboration and professional growth. Join forums like Stack Overflow, participate in relevant subreddits, and consider attending C++ conferences to network with fellow developers.
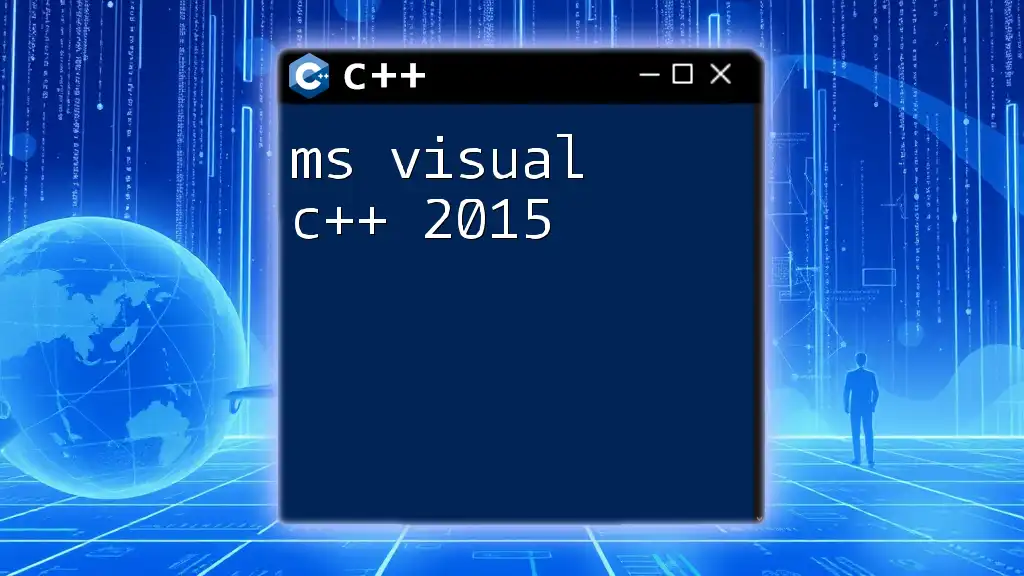
Conclusion
Visual C++ 2023 offers extensive features and capabilities for both novice and experienced developers. By understanding the robust development environment, you can harness its power to create efficient, high-quality applications. Embarking on a journey of continuous learning and community engagement will not only enhance your proficiency but also contribute to your growth within this exciting field.