MISRA C++ 2023 is a set of guidelines designed to promote safety and reliability in C++ programming, particularly in embedded systems and critical applications.
Here's a simple code snippet that demonstrates a basic C++ structure while adhering to some MISRA C++ guidelines:
#include <iostream>
class HelloWorld {
public:
void greet() const;
};
void HelloWorld::greet() const {
std::cout << "Hello, World!" << std::endl;
}
int main() {
HelloWorld hw;
hw.greet();
return 0;
}
What is MISRA C++?
MISRA C++ refers to a set of guidelines aimed at ensuring the safety, reliability, and maintainability of C++ software, particularly in the automotive industry and other safety-critical environments. Established to address the unique challenges posed by the C++ language, these guidelines serve as a critical benchmark for developers who wish to create high-quality software.
Historically, the MISRA C standards originated in the 1990s, reflecting the growing necessity for coding conventions in embedded systems. As programming paradigms evolved, so too did the guidelines, culminating in the release of MISRA C++ 2023. The necessity for these guidelines cannot be overstated, as they help organizations mitigate risks associated with software defects, which can lead to catastrophic results in high-stakes environments.
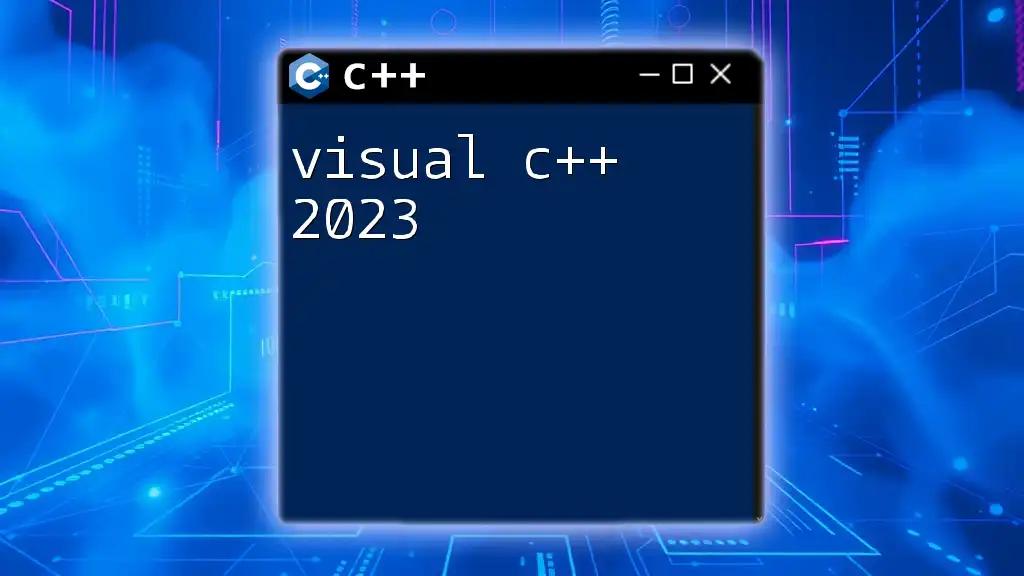
Evolution of MISRA C++
To fully comprehend MISRA C++ 2023, it is essential to examine its evolutionary journey. Previous versions of the guidelines, particularly MISRA C++ 2008, laid the foundation for safer programming practices but required updates to keep pace with advancements in the C++ language itself and the changing landscape of software development.
Key changes in the development of MISRA C++ have often stemmed from real-world feedback and emerging industry trends. As developers began to adopt more advanced features of C++, such as templates and exceptions, the need for guidance addressing these constructs became increasingly evident. MISRA C++ 2023 aims to address these evolving needs while retaining the core ethos of safety and reliability.
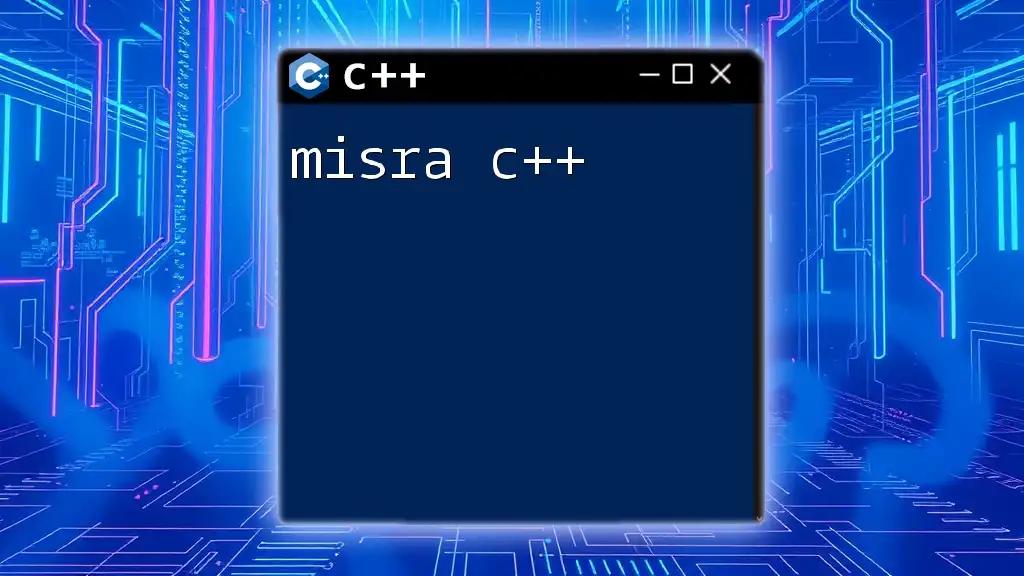
Objectives of MISRA C++ 2023
Safety and Reliability
At the core of MISRA C++ 2023 lies the objective of ensuring code safety. Safety-critical applications demand stringent adherence to guidelines that prevent undefined behavior, data corruption, and runtime errors. By focusing on risk reduction through comprehensive coding practices, MISRA C++ establishes a framework within which safe and reliable software can thrive.
Maintainability
Maintainability is another crucial objective. Well-structured and readable code simplifies the task of updating and modifying existing software, particularly in large projects with multiple developers. MISRA C++ emphasizes adherence to uniform coding practices, enabling any developer to understand and work with the codebase with minimal ramp-up time.
Portability
Portability ensures that code can be easily adapted across various platforms and environments, which is essential for distributed systems in automotive and other sectors. By adhering to MISRA C++ guidelines, developers can help avoid platform-specific features that can hinder broader applicability.
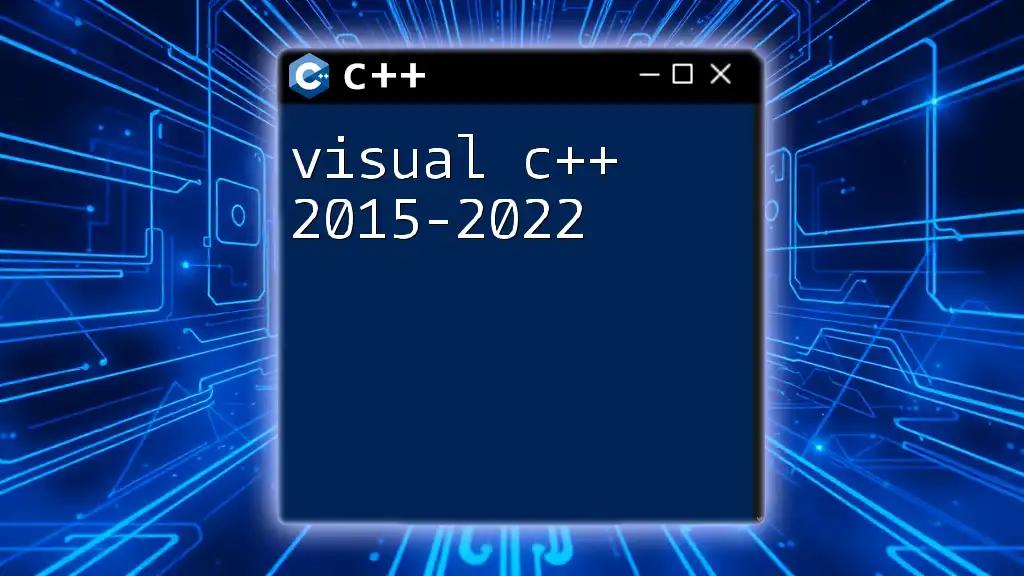
Key Principles of MISRA C++ 2023
Overview of Core Principles
The core principles of MISRA C++ are anchored in promoting clarity, consistency, and simplicity in programming. These principles are the bedrock for reducing complexity and creating predictable, maintainable code. The guidelines offer a robust framework for establishing coding standards that positively impact the development cycle.
Structure of the Guidelines
Mandatory Rules
Mandatory rules are those that must be strictly adhered to without exception. Non-compliance with these rules can result in significant safety concerns. For example, using an uninitialized variable can lead to undefined behavior, making adherence to this rule non-negotiable.
Required Rules
Required rules represent practices that should generally be followed, though exceptions may be justified under certain circumstances. For instance, while it is advisable to use `nullptr` instead of a raw pointer for null checks, instances may arise where an exception can be warranted due to legacy code constraints.
Advisory Rules
Advisory rules serve as recommendations meant to enhance code quality, but they are not strictly enforced. They provide valuable insights on best practices that can strengthen the overall reliability of the application.
Example Code Snippets
Violations of Mandatory Rules
Consider the following example that demonstrates a violation of mandatory rules:
int main() {
int *ptr; // MISRA violation - variable is uninitialized
// Using ptr without assigning a value leads to undefined behavior.
}
In this case, failing to initialize a pointer can lead to unpredictable outcomes, making it a crucial point of attention.
Adhering to Required Rules
Now let’s look at an example that aligns with the required rules:
const int MAX_SIZE = 100; // Define a constant for array size
int myArray[MAX_SIZE]; // Use MAX_SIZE consistently
By employing constants instead of magic numbers throughout the code, we enhance readability and facilitate easier maintenance.
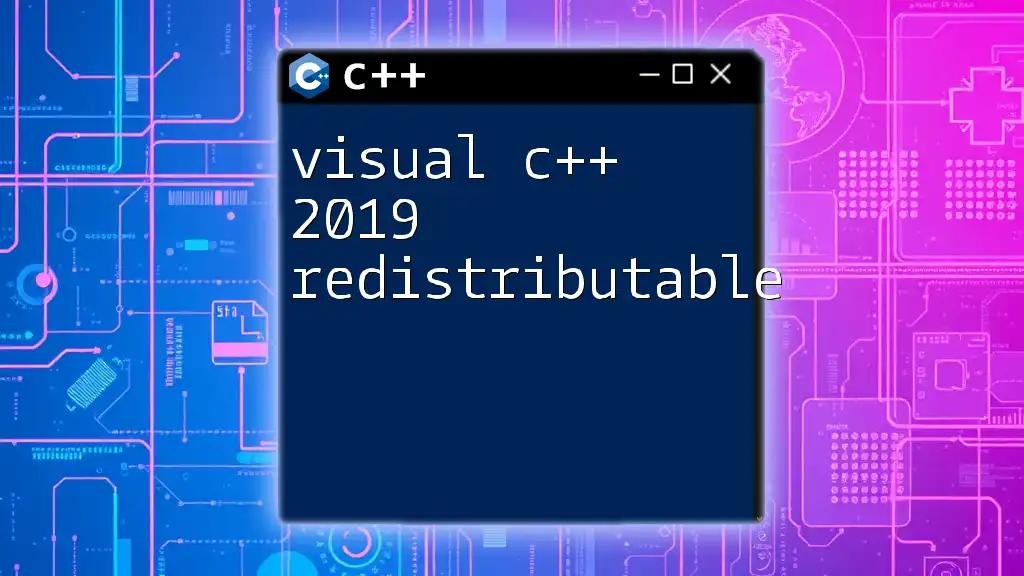
Major Changes in MISRA C++ 2023
Key Updates from Previous Versions
MISRA C++ 2023 introduces several key updates when compared to its predecessor, MISRA C++ 2008. These updates are largely influenced by advancements in C++ standards, including features from C++11 and onwards. The evolution of MISRA reflects a commitment to address contemporary programming practices and bolster compliance with modern language features.
New Concepts Introduced in 2023
The latest iteration introduces several new concepts designed to provide clearer guidance on advanced features such as templates, lambda expressions, and exception handling. These additions are significant as they acknowledge the practical realities encountered by developers who are adopting newer paradigms within C++.
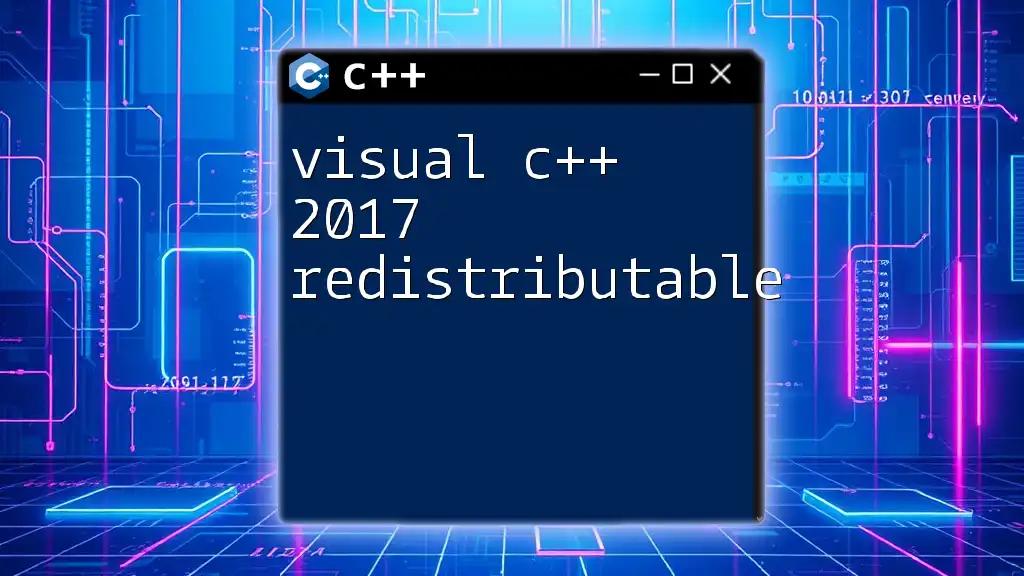
Practical Implementation of MISRA C++ 2023
Tools for Compliance
A multitude of tools exist to aid developers in complying with the MISRA C++ 2023 guidelines. These tools, such as static code analyzers, automate the identification of rule violations and suggest corrective actions. Popular tools include:
- PC-lint: Offers robust features for statically analyzing C++ code.
- CPPcheck: An open-source static analysis tool that identifies common mistakes and allows for MISRA C++ checks.
- ESLint: Though primarily known for JavaScript, it can be configured for C++ with appropriate plugins.
Choosing the right tool involves balancing factors like cost, ease of integration, and specific project requirements.
Best Practices for Adoption
Adopting MISRA C++ 2023 within a project is best accomplished through a structured approach:
- Assessment: Begin by assessing the current codebase to identify existing violations.
- Planning: Create a detailed plan for systematic implementation, setting clear milestones.
- Integration: Incorporate compliance checks into your CI/CD pipeline to ensure continuous adherence during development.
- Monitoring: Continuously monitor code quality and compliance through regular assessments.
This comprehensive process minimizes disruption while maximizing compliance effectiveness.
Training and Education
Understanding and implementing MISRA C++ guidelines is a collaborative company effort that requires proper training and education. Investing in team seminars, hands-on workshops, and access to extensive documentation will equip your developers to utilize the guidelines effectively. Consider providing resources that include online courses and books dedicated to MISRA standards.
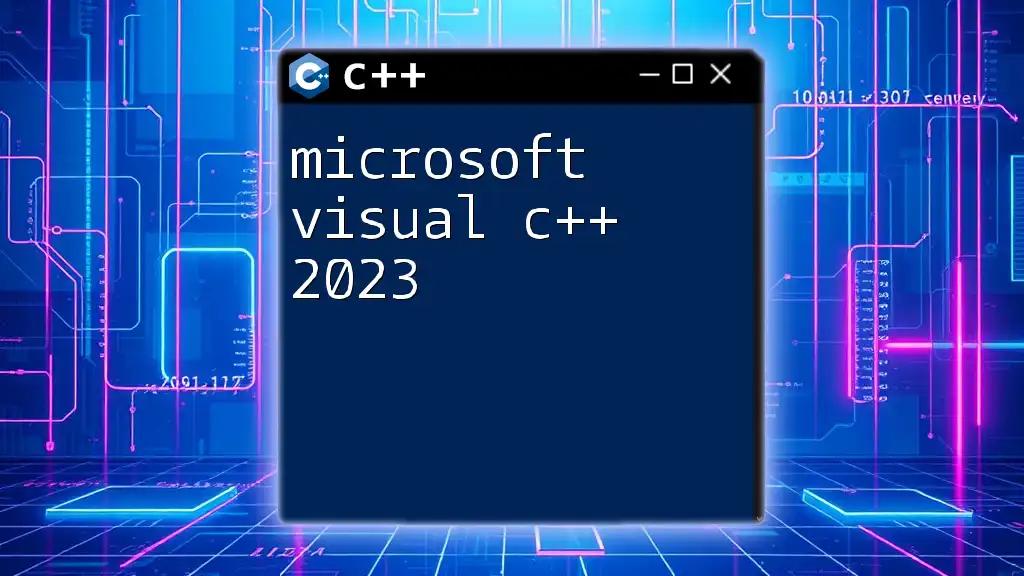
Challenges and Considerations
Common Pitfalls When Following MISRA C++
Developers often encounter challenges when adhering to MISRA C++ guidelines. Common pitfalls include:
- Misunderstanding Requirements: Many developers may misinterpret the guidelines, leading to inadequate implementation.
- Resistance to Change: Depending on team dynamics, some developers may resist transitioning to MISRA practices, creating friction.
- Overhead: The perception that MISRA compliance adds unnecessary overhead can deter teams from fully embracing the guidelines.
Awareness of these pitfalls can facilitate better strategies for overcoming them.
Balancing Compliance with Productivity
One of the quintessential challenges of MISRA C++ 2023 is finding a balance between strict compliance and productivity. Developers often wrestle with the need to follow guidelines meticulously while achieving deadlines.
Real-life scenarios indicate that a blanket adherence to all guidelines may stifle creativity and reduce agility. Organizations must engage in flexible strategies, where critical guidelines are prioritized without compromising essential project timelines and objectives.
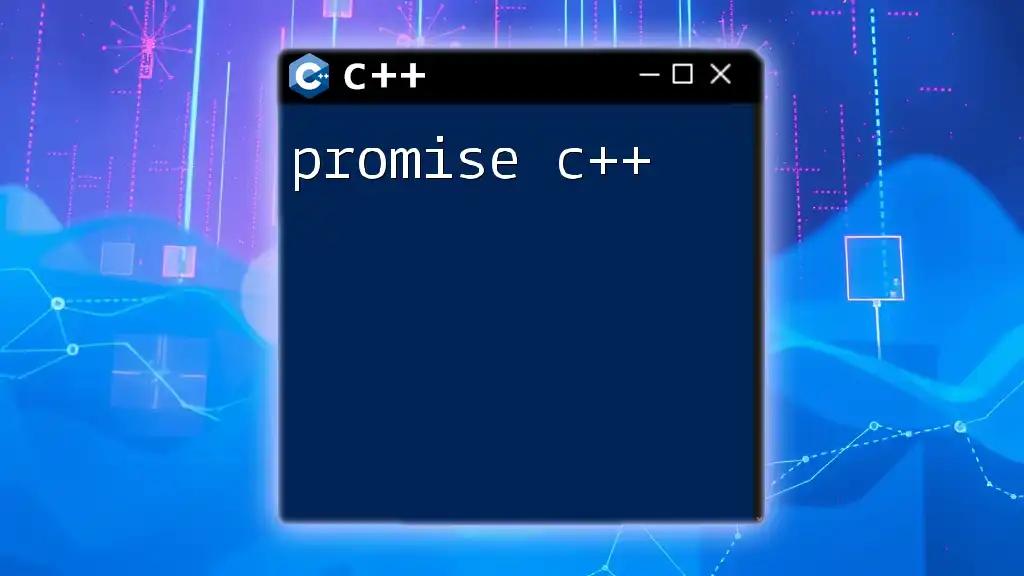
Conclusion
The Future of MISRA C++ Standards
As programming languages and development practices continue to evolve, so too will the MISRA C++ standards. Future updates will likely reflect ongoing trends in software engineering while incorporating lessons learned from real-world implementations.
Final Thoughts on MISRA C++ 2023
In summary, adopting MISRA C++ 2023 is a proactive approach to crafting safe, reliable, and maintainable software. The guidelines provide a solid foundation for developers committed to producing quality code. By understanding and implementing these standards, developers can enhance the robustness and reliability of their software.
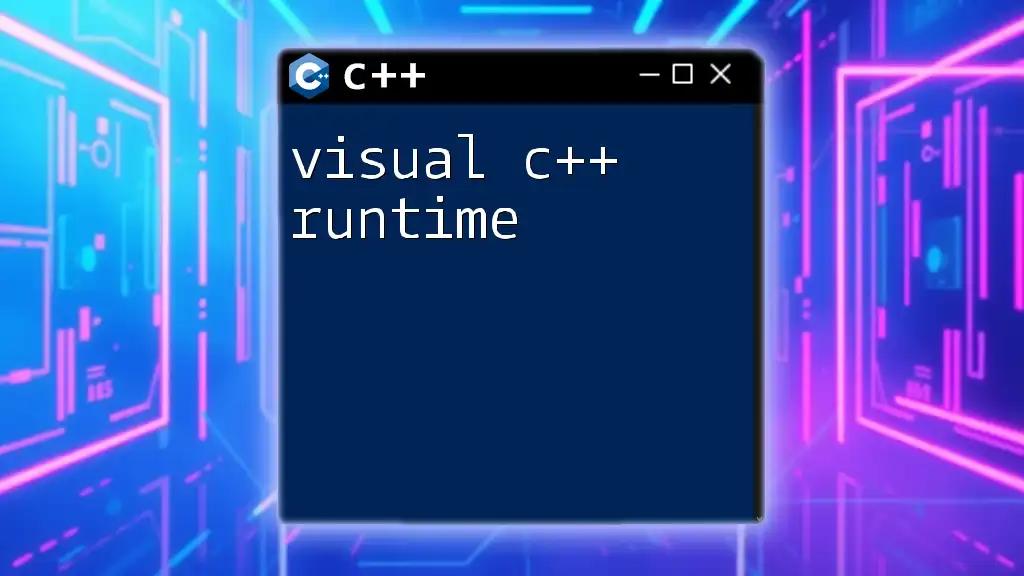
Additional Resources
Recommended Readings
Developers looking to immerse themselves in MISRA C++ would benefit from literature focused on coding standards, safety-critical programming, and project management best practices. Books that delve into MISRA processes or compile case studies can provide invaluable insights.
Related Standards and Guidelines
Familiarity with other coding standards, such as AUTOSAR or ISO C++, can furnish a broader context for MISRA C++ 2023. Understanding this wider framework not only enriches a developer's skill set but also promotes a culture of safety and quality within the industry.
In conclusion, MISRA C++ 2023 is not just a set of guidelines but a pathway to elevating programming practices to new heights. Embracing these principles forms a cornerstone for developing high-quality, safety-critical software.