C++20 introduces several exciting features and enhancements, such as concepts, ranges, and coroutines, elevating the language's usability and performance.
Here's a simple example using a concept in C++20:
#include <iostream>
#include <concepts>
template<typename T>
concept Integral = std::is_integral_v<T>;
void printIntegral(Integral auto value) {
std::cout << "The integral value is: " << value << std::endl;
}
int main() {
printIntegral(42); // Valid
// printIntegral(3.14); // Invalid, would cause a compilation error
return 0;
}
What is C++20?
C++20 marks a significant milestone in the evolution of the C++ programming language. It introduces a host of groundbreaking features and improvements that enhance productivity, code maintenance, and performance. With this version, developers gain more expressive tools and paradigms, paving the way for writing cleaner and more efficient code.

Key Features Added in C++20
C++20 brings many notable features that address common programming tasks. Below is an outline of the key additions:
- Concepts
- Ranges
- Calendar and Time Zone Library
- Format Library
- Coroutines
- Modules
- Three-Way Comparison (Spaceship Operator)
- Constexpr Enhancements

Core Language Enhancements
Concepts
C++20 introduces concepts, a powerful way to specify template requirements. They allow programmers to enforce constraints on template parameters, making error messages clearer and providing better type-checking at compile time.
For example, you can define a concept to validate if a type is incrementable:
template<typename T>
concept Incrementable = requires(T x) {
{ ++x } -> std::same_as<T&>;
{ x++ } -> std::same_as<T>;
};
This makes it possible to write cleaner template code while ensuring that only types fulfilling the specified criteria can be used.
Ranges
The ranges library streamlines the way you work with sequences of data. With ranges, you can express operations on collections with a more readable and expressive syntax. This feature reduces the need for boilerplate code and enhances clarity.
Here's a simple usage of ranges to filter even numbers from a vector:
#include <ranges>
#include <vector>
std::vector<int> v = {1, 2, 3, 4, 5};
auto even_numbers = v | std::views::filter([](int n) { return n % 2 == 0; });
This concise formulation demonstrates how ranges can minimize code clutter while enhancing readability and maintainability.
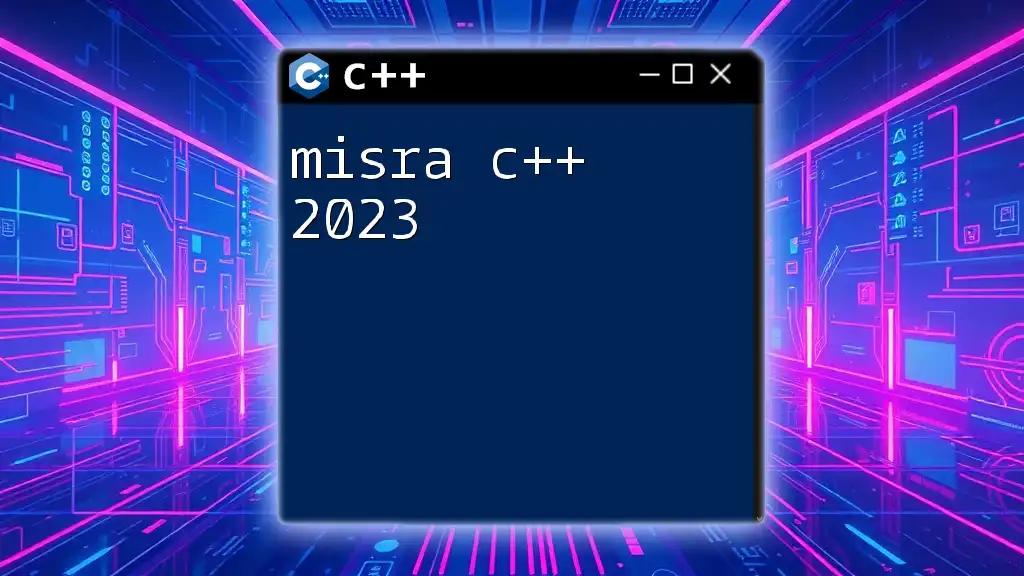
New Standard Library Features
Calendar and Time Zone Library
C++20 brings the much-anticipated calendar and time zone library, providing developers with standardized facilities for date and time manipulation. This library simplifies working with dates and time zones, making it easier to manage time-sensitive applications.
Here’s a simple example demonstrating how to get the current system time:
#include <chrono>
using namespace std::chrono;
auto current_time = system_clock::now();
Format Library
The new format library allows developers to format strings with ease, introducing a type-safe way to handle string formatting compared to traditional methods. The library supports various format specifiers, making string interpolation intuitive and straightforward.
Here’s a quick example of using the format library:
#include <format>
std::string formatted_string = std::format("Hello, {}!", "World");
This feature reduces the risk of errors while formatting strings and improves code readability.
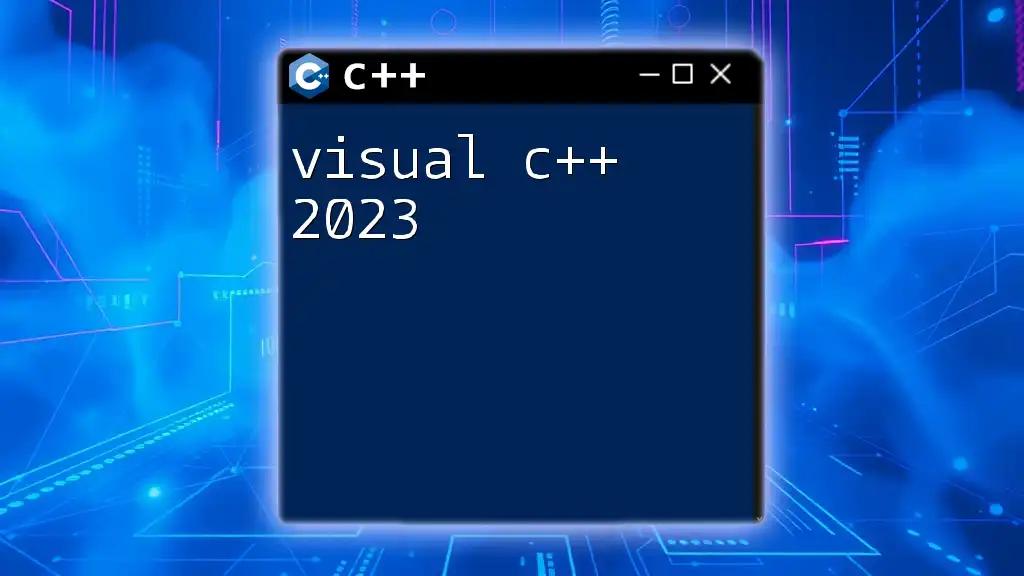
Expanded Functionalities
Coroutines
C++20 introduces coroutines, a revolutionary construct that simplifies asynchronous programming. Coroutines allow functions to yield and resume execution, enabling cleaner flow control. This is particularly helpful for tasks such as asynchronous I/O or managing state transitions.
Here is a simple coroutine example:
std::generator<int> count_up_to(int n) {
for (int i = 1; i <= n; ++i)
co_yield i;
}
Coroutines allow you to handle asynchronous code in a more linear fashion, improving maintainability.
Modules
The modules feature shifts the paradigm of C++ programming. They provide a way to manage code in a more organized manner, reducing compilation dependencies and speeding up build times. With modules, code can be split into distinct units, improving encapsulation and limiting the visibility of implementation details.
Here’s a basic structure of a module:
export module math_utils;
export int add(int a, int b) { return a + b; }
Modules help developers manage large codebases better while minimizing issues related to header file dependencies.
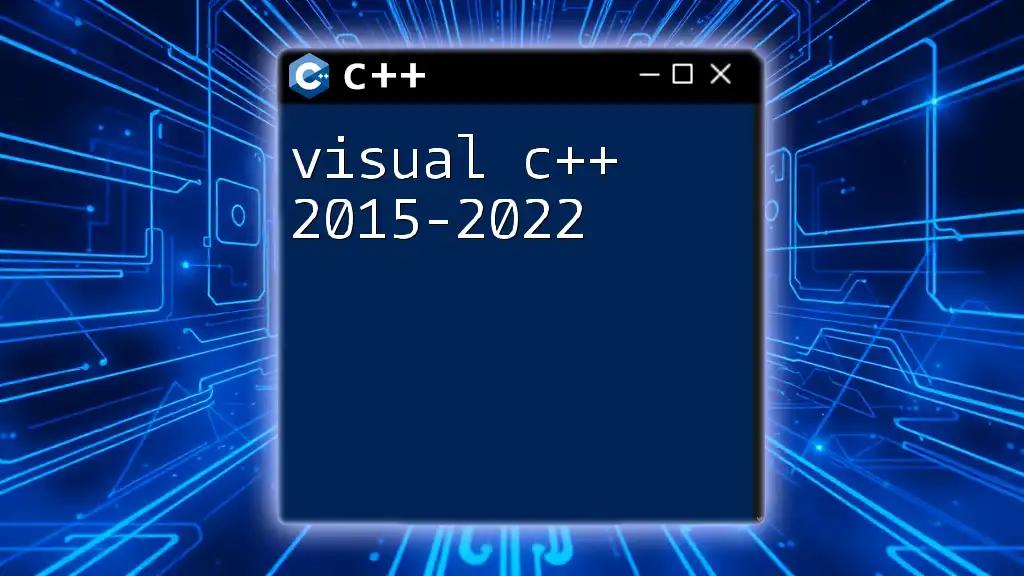
Additional Language Features
Three-Way Comparison (Spaceship Operator)
The spaceship operator (`<=>`) simplifies comparisons between types, allowing C++ developers to easily implement ordering for user-defined types. This operator generates default comparison functions, reducing boilerplate code.
Here’s how you can implement it:
struct Point {
int x, y;
auto operator<=>(const Point&) const = default;
};
This encapsulation simplifies the comparison process and enhances code clarity and maintenance.
Constexpr Enhancements
With C++20, `constexpr` capabilities are significantly enhanced, enabling the use of dynamic memory allocation in `constexpr` functions and more complex logical structures. This enhancement allows developers to perform computations at compile time, leading to more efficient code execution.
Here is an example of a `constexpr` function:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
This allows the compiler to calculate results at compile time, optimizing runtime performance.
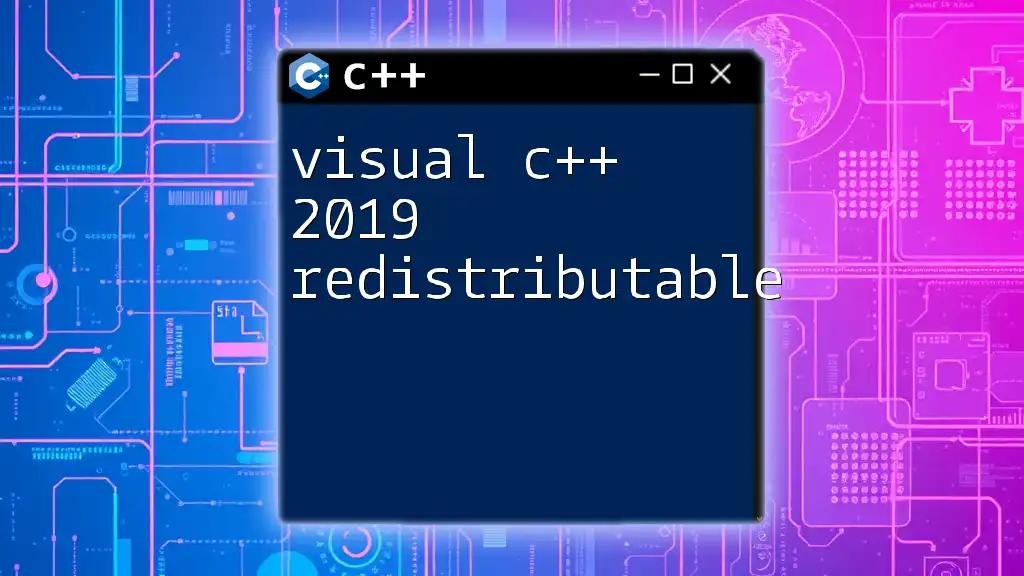
Best Practices for Using C++20 Features
Adopting Concepts for Template Code
When using concepts, leverage them to create clear interfaces in your template code. Doing so dramatically reduces compile-time errors and improves the overall robustness of your templates.
When to Use Coroutines
Utilize coroutines when dealing with asynchronous operations or when you need to manage state across multiple function calls. They offer a cleaner and more intuitive approach to handle such scenarios, making your code easier to reason about.
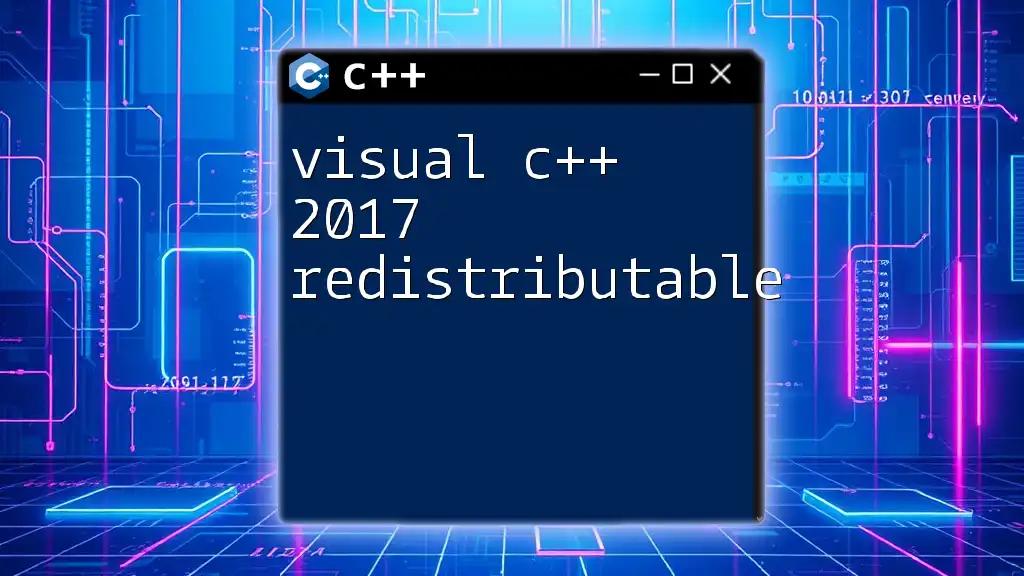
Future Trends of C++ Beyond C++20
Looking ahead, the future of C++ appears promising, with ongoing discussions in the community regarding enhancements and directions for future versions. Concepts for better type-checking and the evolution of modules are just the beginning of a shift towards a more robust and maintainable C++.

Resources for Continued Learning
To enhance your understanding of C++20, consider exploring the following resources:
- Online courses and tutorials focused specifically on C++20 features.
- Books that delve deeper into core functionalities and applications of the latest standards.
- Community forums and discussion boards to share experiences and seek assistance.

Frequently Asked Questions
What are the most valuable additions in C++20?
C++20’s highlights include concepts, ranges, coroutines, modules, and the revamped standard library. Each addition offers developers tools to write cleaner, more efficient code.
How can I start using C++20 features today?
To begin utilizing C++20 features, ensure you have a compatible compiler that supports C++20. Familiarize yourself with the new features through tutorials and practice implementing them in your projects.