C++20 introduces several powerful features, including concepts which allow for more expressive template programming and better type-checking.
#include <iostream>
#include <concepts>
template<typename T>
concept Incrementable = requires(T x) { ++x; };
template<Incrementable T>
void increment(T& value) {
++value;
}
int main() {
int num = 5;
increment(num);
std::cout << "Incremented value: " << num << std::endl; // Output: Incremented value: 6
return 0;
}
What is C++20?
C++20 is the latest major release of the C++ programming language, introducing numerous features that aim to improve the programming experience. It builds upon its predecessor versions, enhancing usability and offering new tools for developers. Understanding C++20 is crucial for anyone engaged in modern software development, as it optimizes performance and boosts productivity.
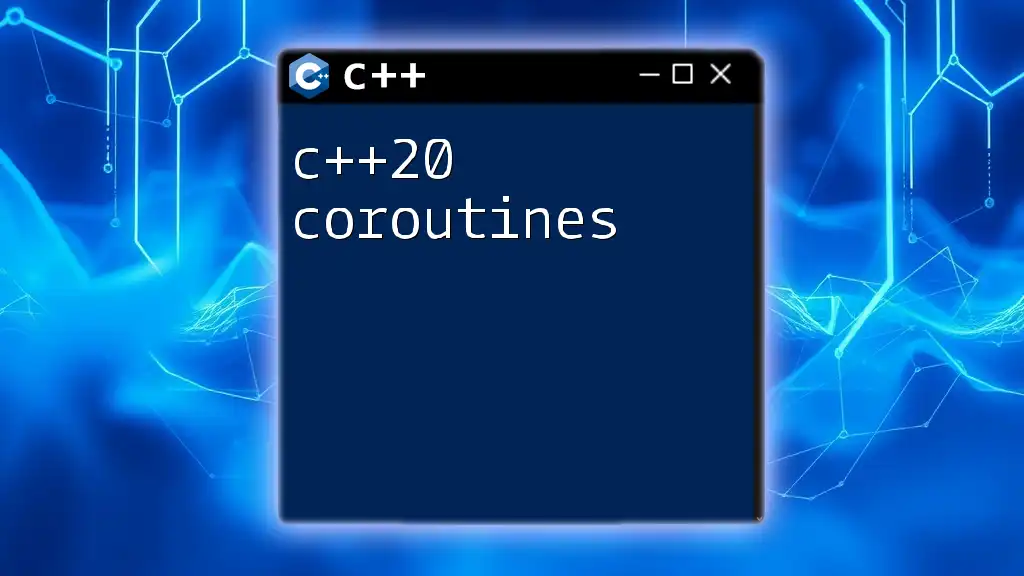
Evolution of C++
C++ has a rich history, with each version bringing vital enhancements. The path leading to C++20 involved significant steps such as:
- C++11: Introduced lambda expressions, smart pointers, and move semantics.
- C++14: Added features like binary literals and variable templates.
- C++17: Brought in structured bindings and std::optional.
C++20 expands upon these foundations, providing tools that cater to the challenges faced by modern developers.
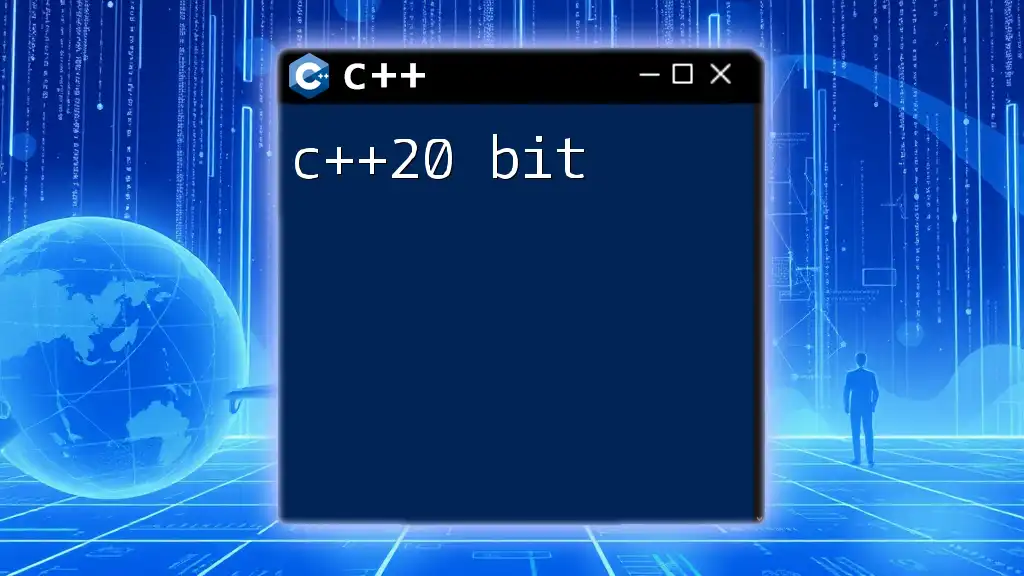
New Features of C++20
Concepts
Concepts are a powerful addition to template programming in C++20. They define constraints on template parameters, making code cleaner and errors easier to spot.
By implementing concepts, developers can express requirements for templates in a clear manner, enhancing code readability.
Example:
template<typename T>
concept Addable = requires(T a, T b) {
{ a + b } -> std::same_as<T>;
};
The above code defines a concept that requires its type parameter to support addition. This means you can create template functions that only accept types satisfying the Addable constraint.
Ranges
Ranges simplify working with collections and sequences in C++20. Traditionally, iterating through collections involved cumbersome loops and iterator handling. With ranges, developers can apply operations in a more readable fashion.
Example:
#include <ranges>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto squares = vec | std::views::transform([](int i) { return i * i; });
for (int square : squares) {
std::cout << square << " "; // Output: 1 4 9 16 25
}
}
In this example, the `std::views::transform` function is applied to generate a transformed view of the original vector without the complexities associated with traditional loops.
Coroutines
Coroutines facilitate asynchronous programming by allowing functions to be paused and resumed, giving developers finer control over operations like I/O tasks or complex calculations.
They introduce a new style of programming that can improve performance and responsiveness in applications.
Example:
#include <iostream>
#include <coroutine>
struct generator {
struct promise_type {
int current_value;
auto get_return_object() { return generator{this}; }
auto initial_suspend() { return std::suspend_always{}; }
auto final_suspend() noexcept { return std::suspend_always{}; }
void unhandled_exception() {}
auto yield_value(int value) {
current_value = value;
return std::suspend_always{};
}
};
promise_type* promise;
generator(promise_type* promise) : promise(promise) {}
// Coroutine continuation function
int getNext() {
promise->current_value = 0;
return promise->current_value;
}
};
generator myCoroutine() {
for (int i = 0; i < 5; ++i) {
co_yield i; // Pauses here and returns the value
}
}
The `co_yield` keyword allows the coroutine to pause its execution and return a value, providing a clean way to manage state over multiple calls.
Modules
Modules introduce a new way of structuring C++ code, enhancing encapsulation and significantly reducing compilation times. This feature allows you to organize your project in a more logical manner while also improving the performance of large applications.
Example:
// In module.cppm
export module math;
export int square(int x) { return x * x; }
// In main.cpp
import math;
int main() {
std::cout << square(5); // Outputs 25
}
This modular approach eliminates the need for header files, making code maintenance easier.
Calendar and Time Zone Library
C++20 introduces a comprehensive calendar and time zone library, enhancing the `<chrono>` header with new features to facilitate management of date and time-related functionalities. This provides developers with tools to handle time in a more accurate and straightforward manner.
Example:
#include <chrono>
#include <iostream>
int main() {
using namespace std::chrono;
auto today = floor<days>(system_clock::now());
std::cout << "Today: "
<< duration_cast<seconds>(today.time_since_epoch()).count();
}
In this snippet, the current date is retrieved and formatted, showcasing the ease of working with the new library.
New Standard Attributes
C++20 also introduces several new standard attributes that enhance the already existing set of attributes. These attributes can provide additional information to the compiler, aiding optimization and improving type safety.
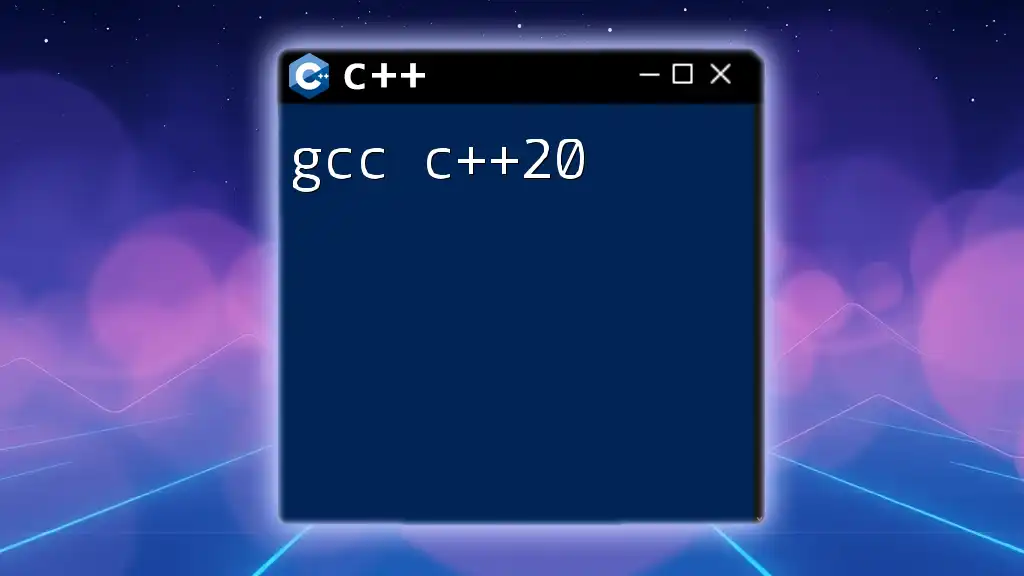
Enhancements to Existing Features
Improved Lambdas
C++20 improves lambda expressions by allowing them to take template parameters directly. This provides greater flexibility in creating modular and reusable code.
Example:
auto sum = [](auto a, auto b) { return a + b; };
std::cout << sum(3, 4); // Outputs 7
This new feature streamlines the process of creating inline functions that can be customized based on their input types.
Dynamic Memory Management Improvements
C++20 includes various improvements in dynamic memory management, specifically in how smart pointers interact and optimize resource management. This leads to fewer memory leaks and easier management of dynamic resources.
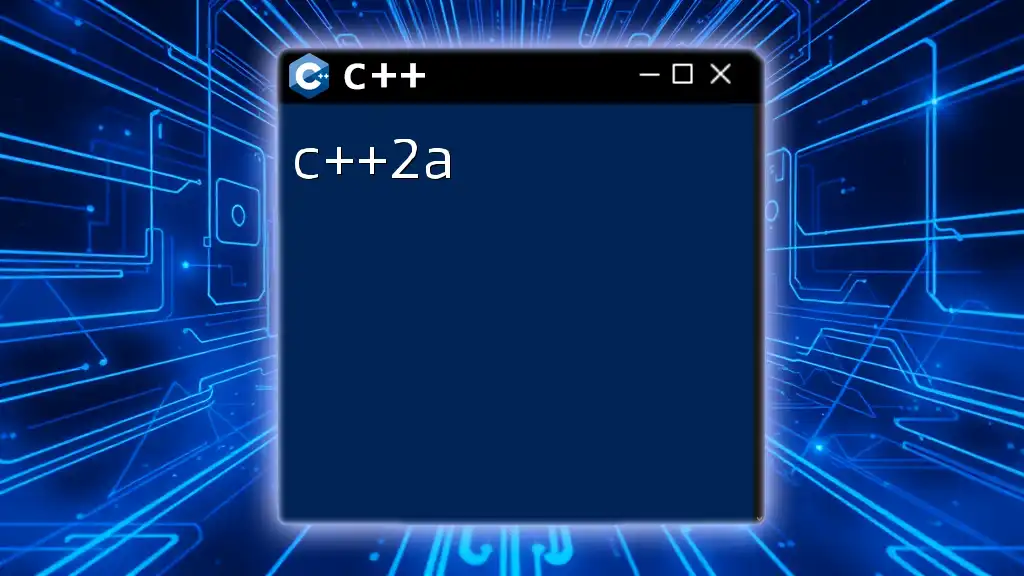
Backward Compatibility
One vital aspect of C++20 is its backward compatibility. New features are designed to integrate seamlessly with existing codebases, preventing disruption of established projects. This makes adopting C++20 more appealing, as developers can transition to the new standard without the fear of breaking legacy code.
Best Practices for Transitioning Projects to C++20
- Conduct comprehensive testing to ensure existing functionalities remain intact.
- Gradually introduce new features by refactoring components of the codebase.
- Use static analysis tools to identify potential issues during the transition.
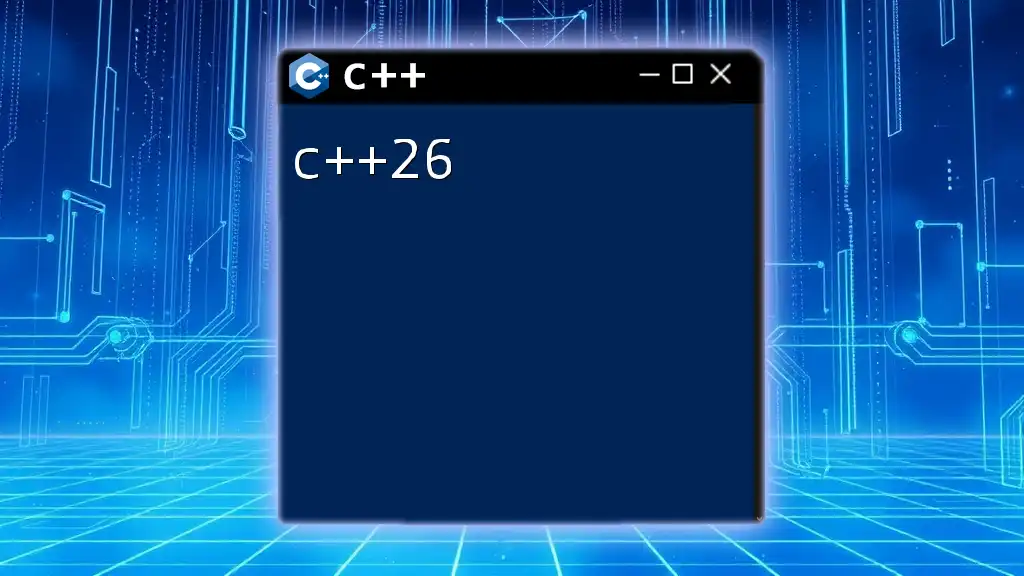
Conclusion
As we look to the future, C++20 sets the stage for further advancements in the language, making it crucial for developers to familiarize themselves with its features. Learning and integrating C++20 into projects will empower programmers, allowing them to write more efficient and effective code.
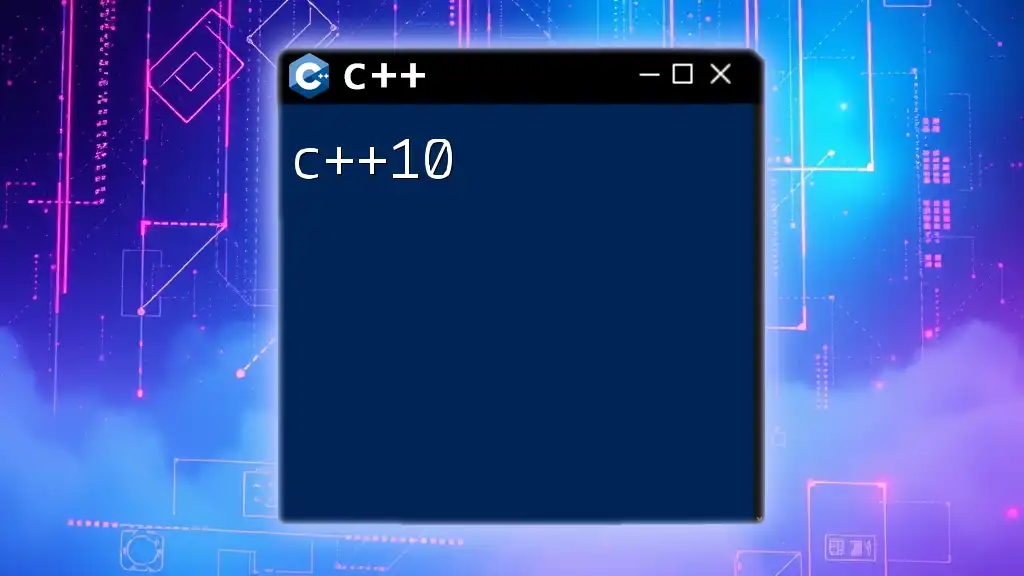
Additional Resources
To deepen your knowledge on C++20, consider these resources:
- Books: Look for titles that focus specifically on C++20 features and best practices.
- Online Courses: Platforms like Coursera, Udemy, and others offer tailored courses that dive into C++20.
- Documentation: The official C++20 documentation provides comprehensive guidelines and references.
By embracing C++20, you enhance your toolkit and prepare yourself for the evolving landscape of modern software development.