C++ 2023 introduces key enhancements such as modules and coroutines, streamlining code organization and improving asynchronous programming.
Here's a simple example demonstrating the use of a coroutine in C++20:
#include <iostream>
#include <coroutine>
struct SimpleCoroutine {
struct promise_type {
SimpleCoroutine get_return_object() { return {}; }
std::suspend_never initial_suspend() { return {}; }
std::suspend_never final_suspend() noexcept { return {}; }
void unhandled_exception() {}
void return_void() {}
};
};
SimpleCoroutine exampleCoroutine() {
std::cout << "Hello from Coroutine!" << std::endl;
co_return;
}
int main() {
exampleCoroutine();
return 0;
}
What’s New in C++ 2023
Overview of C++ Standards
C++ has a rich history that dates back to the early 1980s, evolving through various versions and standards like C++98, C++11, C++14, C++17, and C++20. Each iteration has built upon the last, introducing features that aim to make programming more efficient and powerful. As we review C++ 2023, it is essential to grasp the innovations that have been incorporated, ensuring developers are well-prepared to leverage the latest advancements.
Key Features Introduced in C++23
C++23 introduces several critical enhancements. As a developer, familiarizing yourself with these features can greatly improve your coding efficiency and lead to cleaner, more maintainable applications. Here are some of the standout additions:
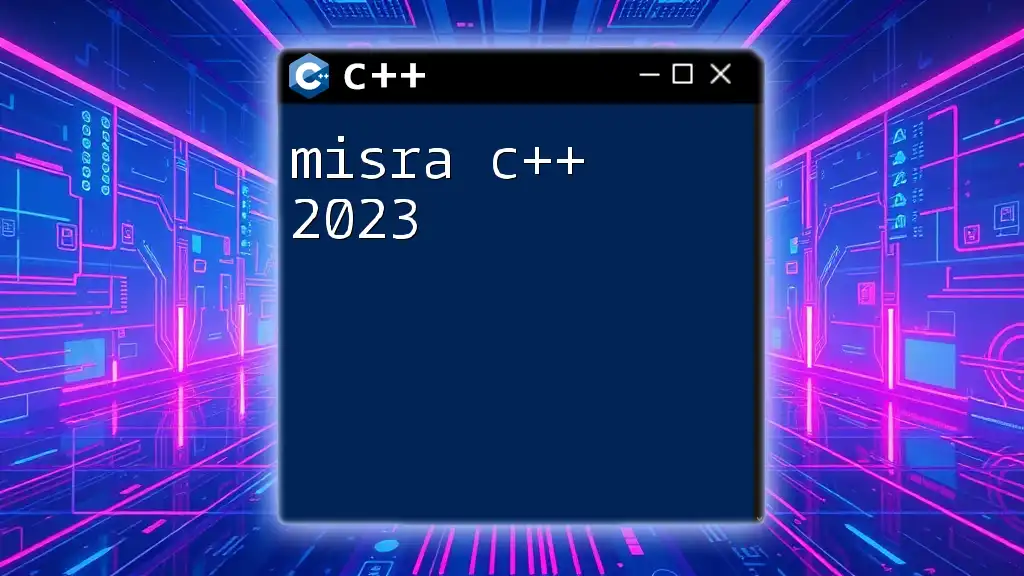
Language Enhancements
Deduction Guides for Class Templates
Another significant feature introduced in C++ 2023 is the concept of deduction guides for class templates. This feature simplifies the syntax when creating instances of template classes where the type can be deduced automatically, thus enhancing usability.
For example:
template<typename T>
struct MyContainer {
using value_type = T;
};
MyContainer auto myContainer = MyContainer<int>(); // Deduces T as int
In this snippet, `myContainer` is automatically deduced to be of type `MyContainer<int>`, improving code clarity and reducing boilerplate.
Expanded constexpr Capabilities
C++ 2023 expands the capabilities of `constexpr`, allowing developers to perform more operations at compile-time. Function arguments and more complex data structures can now be declared as `constexpr`, enabling potential performance gains.
Consider the following example:
constexpr int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
static_assert(factorial(5) == 120);
Here, `factorial` can be evaluated at compile-time, which can significantly speed up programs by minimizing runtime computations.
`std::expected` and Error Handling
Error handling has always been a challenging aspect of C++ development. C++ 2023 introduces `std::expected`, a standard way to represent values that might fail to compute successfully. This can effectively replace traditional error handling methods, such as using exceptions, leading to cleaner code.
Here’s an example:
#include <expected>
std::expected<int, std::string> find_value(int key) {
if (key < 0) {
return std::unexpected("Key must be non-negative");
}
return key * 2; // Just a sample computation
}
With `std::expected`, error cases can be handled more explicitly and elegantly.
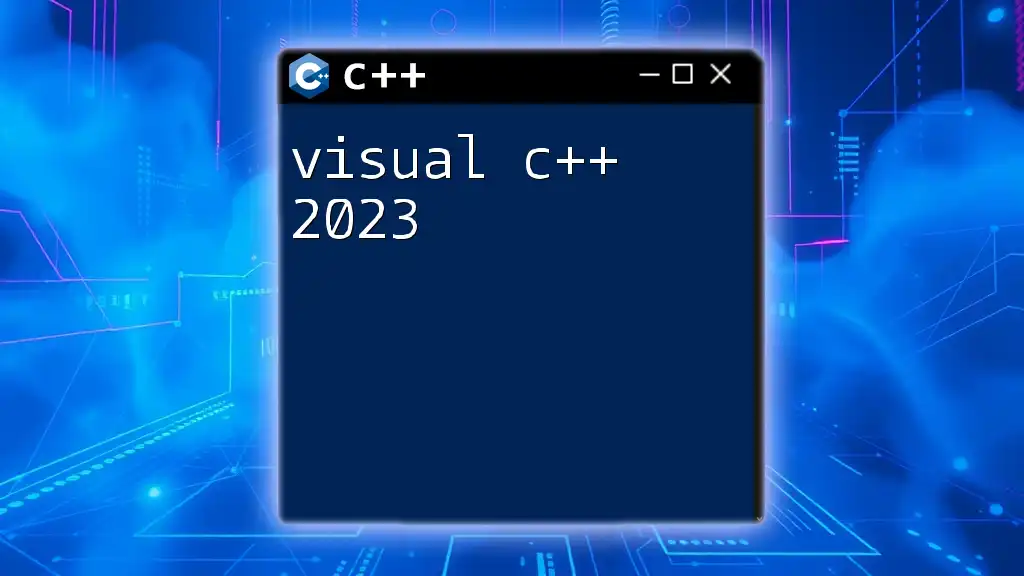
New Library Features
Ranges and View Libraries Enhancements
The ranges library has received substantial upgrades in C++ 2023, improving the way we manipulate collections. With new range-based operations and views, developers can write clearer and more functional-style code.
For instance:
#include <ranges>
auto nums = std::views::iota(1, 10) | std::views::filter([](int n) { return n % 2 == 0; });
This code snippet creates a range of numbers from 1 to 10 and filters out the even numbers seamlessly, demonstrating the power of ranges.
Synchronization and Concurrency Features
C++ 2023 also brings improvements in concurrency, catering to the growing need for efficient multi-threading. New synchronization features have been added to facilitate easier and safer concurrent programming.
Here’s an example to illustrate this:
#include <syncstream>
std::osyncstream out(std::cout);
out << "Thread-safe output" << std::endl;
This enhancement allows you to output to streams in a thread-safe manner, minimizing data races and potential output issues.
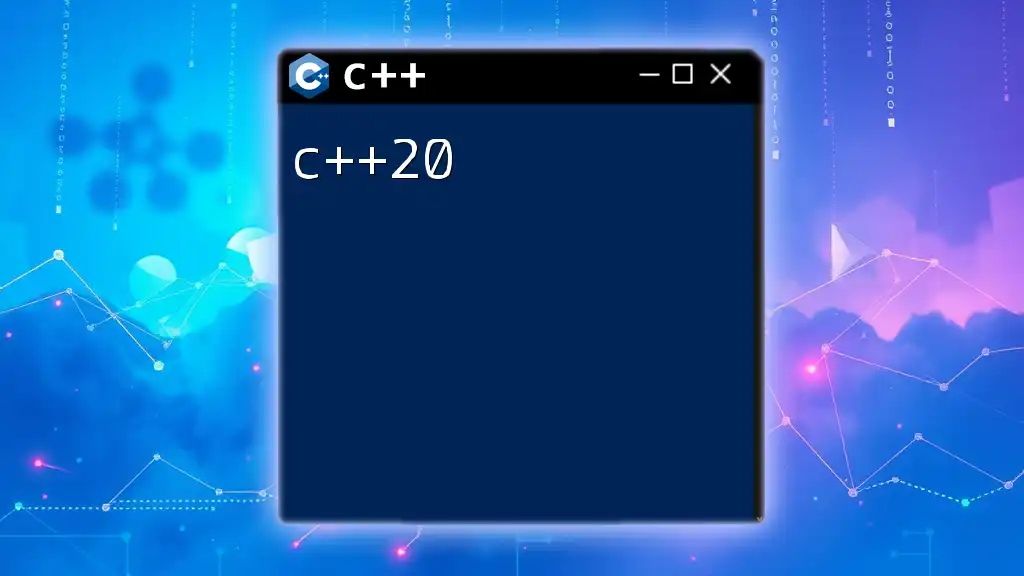
Performance Improvements
Compile-Time Optimizations
Performance is always a significant focus for C++ development. With C++ 2023, improvements have been made to compile-time optimizations. The compiler's speed and efficiency have been enhanced, reducing build times significantly, which can be a game-changer for large projects.
Execution Speed Enhancements
C++ 2023 also offers various changes designed to improve runtime performance. By fine-tuning language constructs and introducing more efficient algorithms, developers can expect better execution speeds in applications. These changes can directly impact performance-critical applications, making them faster and more responsive.
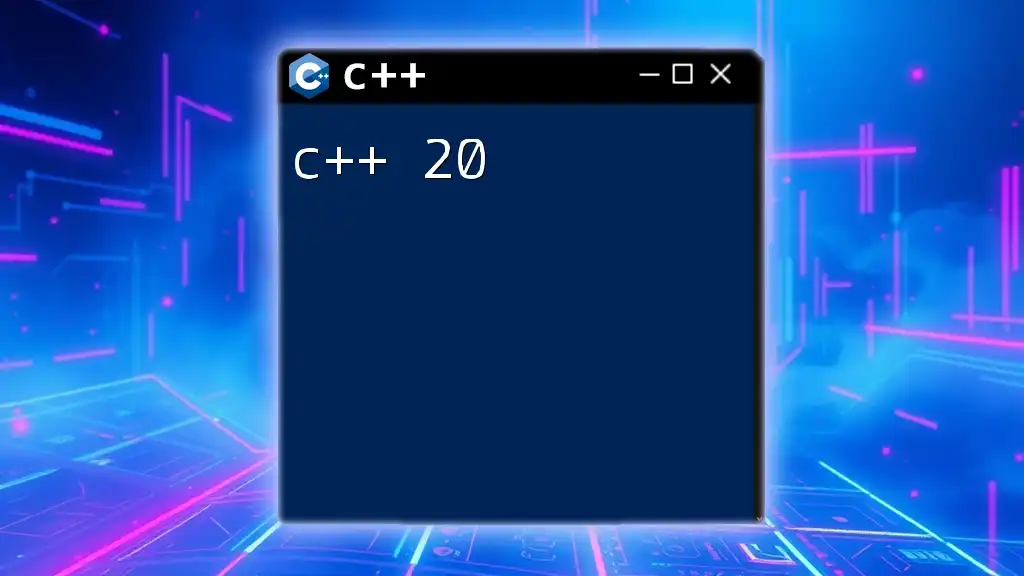
Best Practices for Using C++ 2023
Writing Clean and Maintainable Code
The introduction of new features in C++ 2023 emphasizes the need for writing clean and maintainable code. Using modern practices such as expressive naming, logical structure, and appropriate comments will go a long way in ensuring your code remains accessible and understandable.
Leveraging New Features
To fully benefit from C++ 2023, developers should actively adopt and leverage new features in their projects. Experimenting with these features in smaller, manageable projects can help solidify understanding. For instance, embracing `std::expected` for error handling can lead to more robust applications.
Backward Compatibility Considerations
As with any new standard, understanding backward compatibility is critical. C++ 2023 maintains a strong degree of compatibility with previous standards, allowing developers to integrate new features without significant overhaul of existing codebases. When transitioning, it’s advisable to conduct thorough testing to ensure functionalities remain consistent.
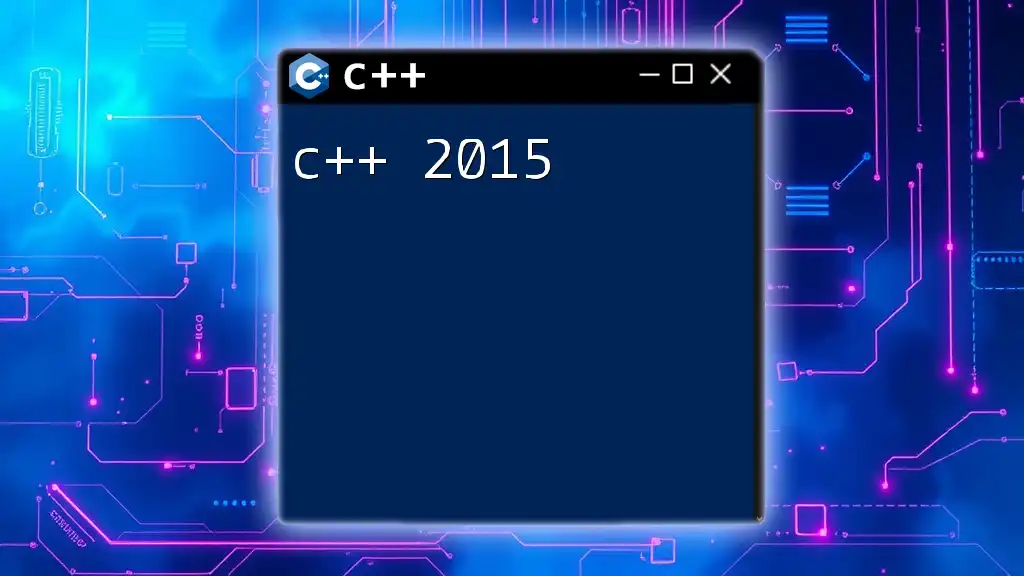
Tips and Resources
Online Tools and Compilers for C++ 2023
Several online tools and compilers have emerged that support C++ 2023 features. Popular IDEs like Visual Studio, CLion, and online platforms such as Repl.it or Compiler Explorer are valuable resources for experimenting with new features without needing a local setup.
Community and Learning Resources
Engaging with the C++ community is incredibly beneficial. Developers should explore forums like Stack Overflow, join C++ groups on social media, and follow reputable blogs and channels focused on C++ education. Tutorials, documentation, and courses are often updated to reflect the latest standards, providing continuing education opportunities.
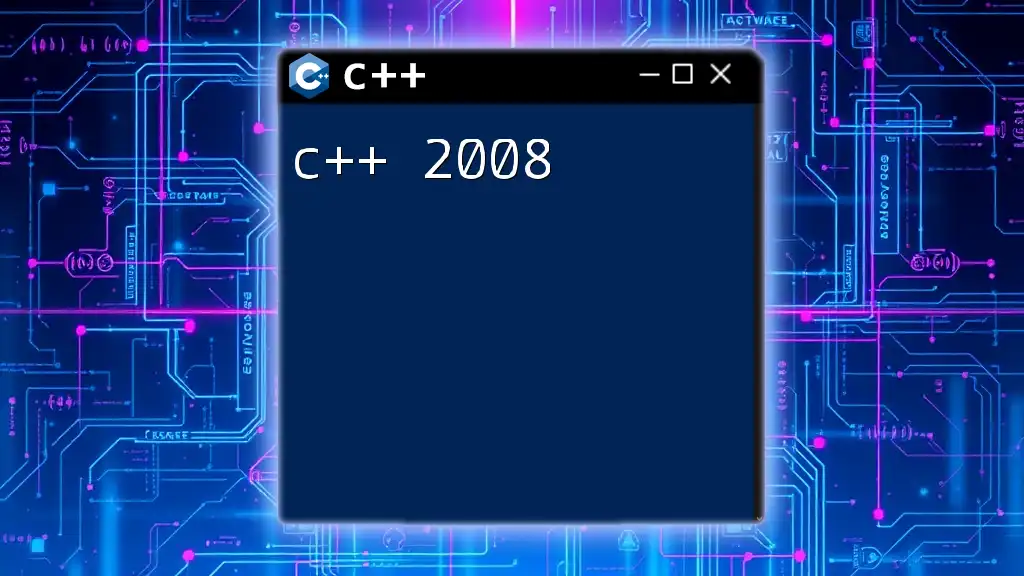
Conclusion
The advancements in C++ 2023 come with a promise of enhanced efficiency, clarity, and usability. With features designed to streamline coding practices while supporting modern programming paradigms, adopting these updates will serve developers well. As C++ evolves, staying updated and skilled with its features will ensure continued growth and success in your programming endeavors.