C++17 introduced several enhancements to the language, including improved support for parallelism and new standard library features such as `std::optional` and `std::variant` for more robust type handling.
Here's a code snippet demonstrating `std::optional`:
#include <iostream>
#include <optional>
std::optional<int> findValue(bool shouldFind) {
if (shouldFind) {
return 42; // Found the value
}
return std::nullopt; // No value found
}
int main() {
auto value = findValue(true);
if (value) {
std::cout << "Value found: " << *value << std::endl;
} else {
std::cout << "No value found." << std::endl;
}
return 0;
}
What is C++ 2017?
C++ 2017, also known as C++17, is an update to the C++ programming language that introduces several new features and improvements over its predecessors. It aims to enhance the efficiency, performance, and usability of the language, making it even more valuable in modern software development. C++17 is significant not only for its technical advancements but also for how it influences development practices across various industries such as gaming, systems programming, and real-time applications.
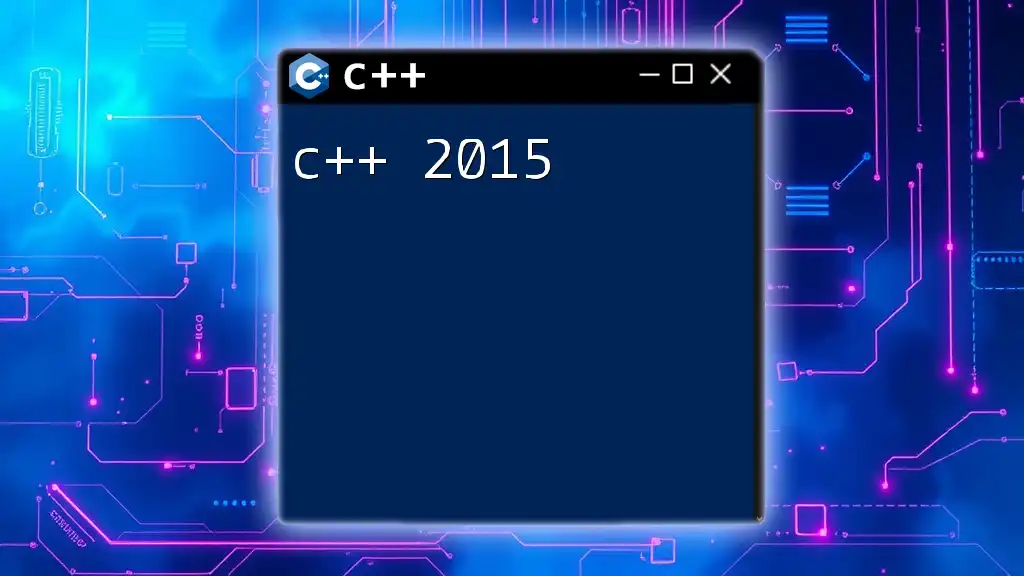
What’s New in C++ 2017
Key Features of C++ 2017
C++17 introduces several key enhancements and features that promote better coding practices, improved safety, and increased performance. Among these enhancements, particular attention is given to simplifying complex code structures and making the language more expressive.
Language Features
Inline Variables
This feature allows you to declare variables that can be defined inline in a header file without the need for extern storage duration. This makes header-only libraries easier to manage.
Example:
inline constexpr double PI = 3.14159265358979323846;
Structured Bindings
Structured bindings provide a more intuitive way of unpacking tuples, pairs, or user-defined types, making it easier to deal with multiple return values.
Example:
std::tuple<int, double, std::string> getData() {
return {1, 3.14, "Hello"};
}
auto [id, value, message] = getData();
Constexpr If
The `if constexpr` feature introduces compile-time branching, allowing you to enable or disable parts of your code based on conditions evaluated at compile time. This is particularly useful in template programming to reduce code bloat.
Example:
template<typename T>
void process(T value) {
if constexpr (std::is_integral<T>::value) {
// Handle integer
} else {
// Handle non-integer
}
}
Library Features
New Standard Library Features
C++17 enriches the Standard Library with new components that simplify everyday programming tasks.
- std::optional: Represents optional values that can be empty, encapsulating the mechanism of handling absence.
Example:
std::optional<int> maybeGetValue(bool shouldReturn) {
if (shouldReturn) return 42;
return std::nullopt;
}
- std::variant: A type-safe union that can hold one of several types, providing a safer alternative to unions.
Example:
std::variant<int, std::string> var;
var = 10; // now var holds an int
- std::any: A type-safe container that can contain values of any type.
Example:
std::any a = 42;
a = std::string("Hello");
Filesystem Library
C++17 includes a new filesystem library that provides convenient methods for file system manipulation. This makes it easy to perform file operations such as reading, writing, and manipulating file paths.
Example:
#include <filesystem>
namespace fs = std::filesystem;
fs::path path = "example.txt";
if (fs::exists(path)) {
std::cout << path << " exists." << std::endl;
}
Parallel Algorithms
C++17 introduces parallel execution policies for algorithms, allowing developers to take advantage of multi-core architectures seamlessly.
Example:
#include <algorithm>
#include <vector>
#include <execution>
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(std::execution::par, numbers.begin(), numbers.end(), [](int& n) { n *= 2; });
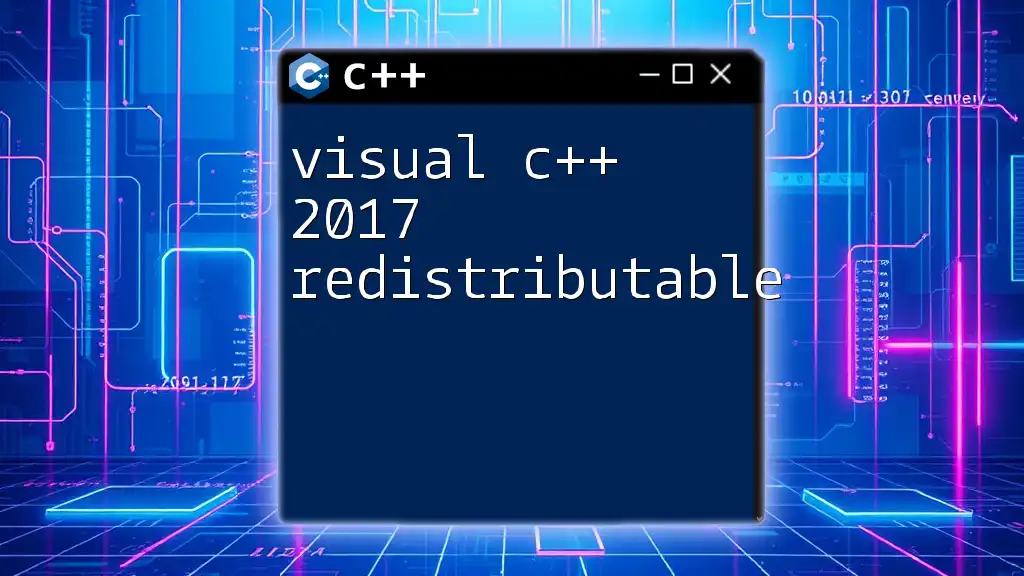
Using Visual C++ 2017
Getting Started with Visual C++ 2017
Visual C++ 2017 is a robust Integrated Development Environment (IDE) that provides extensive support for C++ development, making it easier to create and manage C++ projects.
To get started, you must install Visual Studio and select the C++ development workload. This process enables various tools and features for C++17 development.
Development Environment
The development environment is user-friendly, featuring an intuitive interface with easily accessible project tools. One of its standout features is the integrated debugger, which provides an effective way to troubleshoot and analyze code.
Code Samples in Visual C++ 2017
A practical way to comprehend capabilities offered by C++17 is through example projects. Below is a simple demonstration that showcases several C++17 features:
#include <iostream>
#include <tuple>
#include <optional>
inline constexpr double PI = 3.14159265358979323846;
std::tuple<int, double, std::string> getData() {
return {1, 3.14, "Example"};
}
std::optional<int> maybeGetValue(bool condition) {
return condition ? 42 : std::nullopt;
}
int main() {
// Using structured binding
auto [id, pi_val, message] = getData();
std::cout << "ID: " << id << ", Value: " << pi_val << ", Message: " << message << std::endl;
// Using optional
if (auto maybe_value = maybeGetValue(true)) {
std::cout << "Value: " << *maybe_value << std::endl;
} else {
std::cout << "No value returned!" << std::endl;
}
}
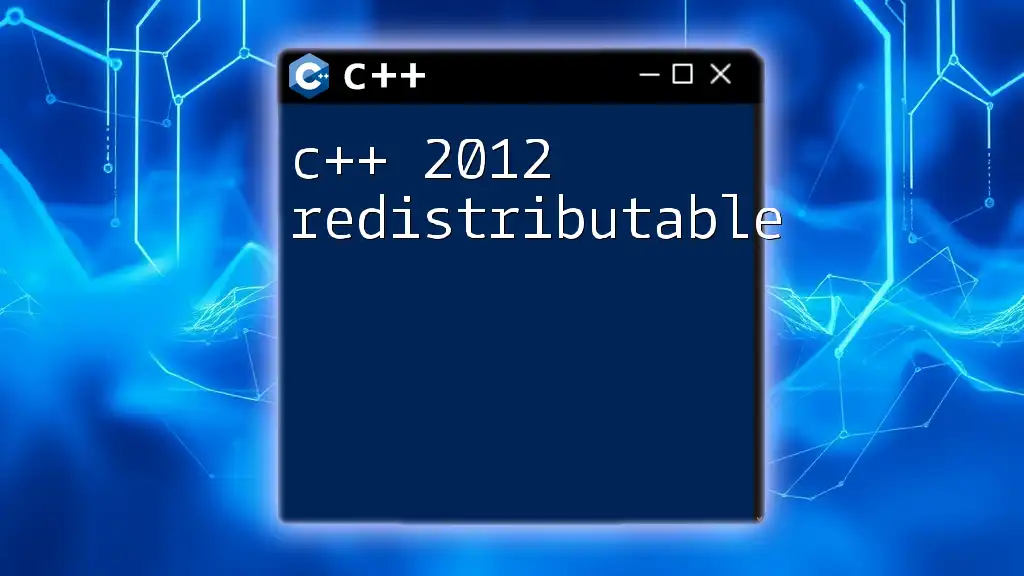
Best Practices for Writing C++ 2017 Code
Coding Standards and Style Guide
Maintaining coding standards is crucial for ensuring that your code is readable and maintainable. Adhering to best practices encourages consistency and collaborativeness in development teams. Common practices include:
- Using meaningful variable names
- Keeping functions short and focused
- Embracing RAII (Resource Acquisition Is Initialization)
Performance Considerations
Optimizing the performance of your C++ code is vital, especially in performance-critical applications. Here are some essential tips:
- Utilize move semantics to avoid unnecessary copying.
- Minimize dynamic memory allocations.
- Use `std::array` instead of raw arrays where possible for better safety.
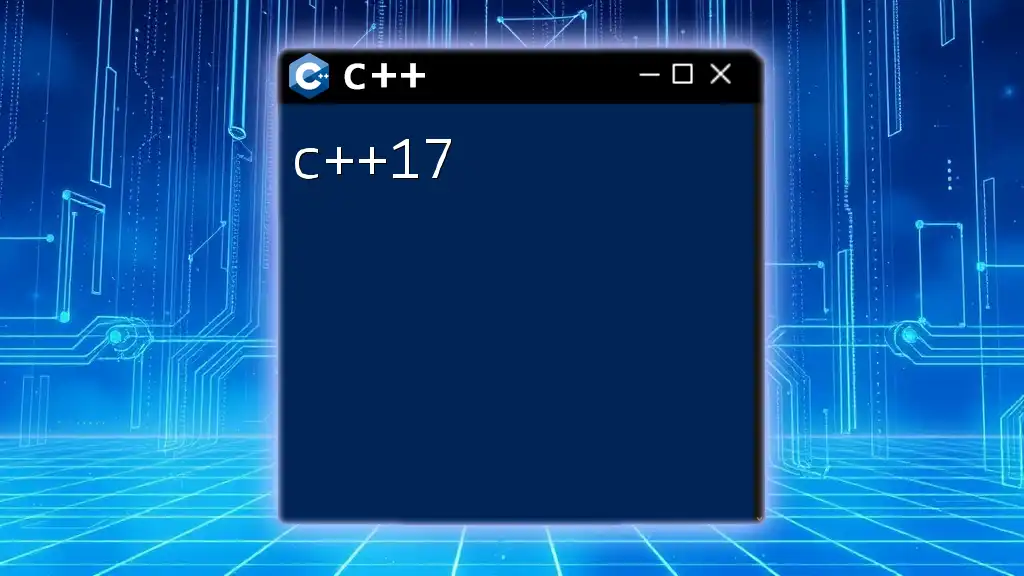
Migrating to C++ 2017
Preparing for Migration
When considering migration from older versions of C++, developers should assess the compatibility of existing codebases with C++17 features. Several tools can assist in identifying discrepancies and potential issues.
Challenges of Migration
Common Pitfalls
During migration, developers may encounter several challenges, such as deprecated features or changes in behavior. Thorough testing and code reviews can help detect and address these challenges early.
Testing and Validation
After migrating, it is essential to validate and test the software rigorously. Using unit tests or frameworks like Google Test can ensure that the application behaves as expected.
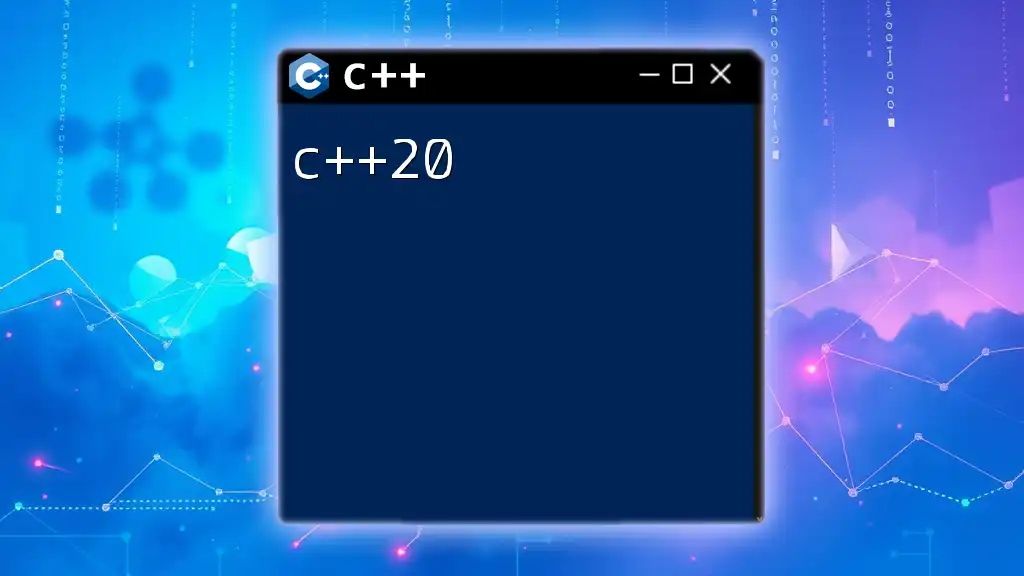
Conclusion
C++ 2017 brings an array of innovative features that make it an essential upgrade for modern programming. By embracing these enhancements, developers can write cleaner code, leverage advanced capabilities, and build efficient applications. As C++ continues to evolve, staying informed and adaptive will empower you to harness its full potential.
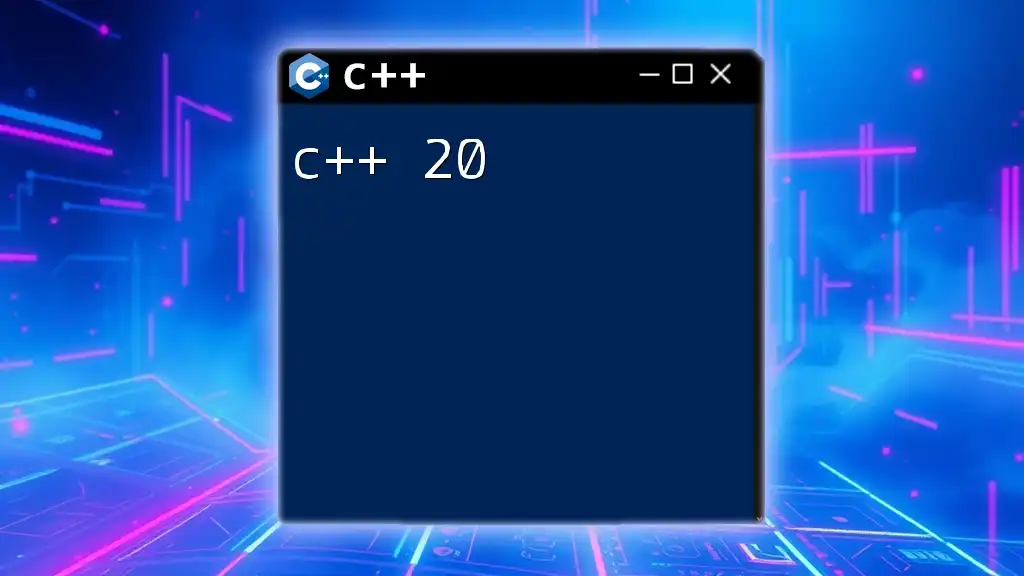
Additional Resources
To further your understanding of C++ 2017, consider exploring online platforms that offer tutorials and classes specifically tailored to C++ programming. Additionally, online forums and programming communities can be significant sources of support as you navigate through learning and implementing C++17 features.