Microsoft Visual C++ 2015 is an integrated development environment (IDE) that allows developers to create, debug, and compile C++ applications efficiently on the Windows platform.
Here's an example of a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Features of Visual C++ 2015
Key Enhancements
MS Visual C++ 2015 introduced a host of features designed to improve both performance and usability. These enhancements include improved performance and optimization capabilities, allowing developers to create faster and more efficient applications. The IDE (Integrated Development Environment) includes support for the latest C++ standards, enabling programmers to utilize advanced features like lambdas and smart pointers.
Another significant upgrade was the enhanced debugging and profiling tools, allowing developers to identify and resolve issues faster. These tools provide insights into memory usage, CPU performance, and help streamline the testing process.
Development Environment Improvements
The user interface of the Visual C++ 2015 IDE has been designed for simplicity and efficiency. It has been enhanced for better usability, allowing developers to navigate their codebases more easily. Among the improvements are better code refactoring tools, which help developers to restructure existing code without altering its external behavior, thereby making the maintenance of large codebases less daunting.
In addition, new features for code navigation and IntelliSense have been incorporated. IntelliSense, a code-completion aid, provides valuable suggestions as you type, improving productivity by reducing the effort needed to recall syntax and function names.
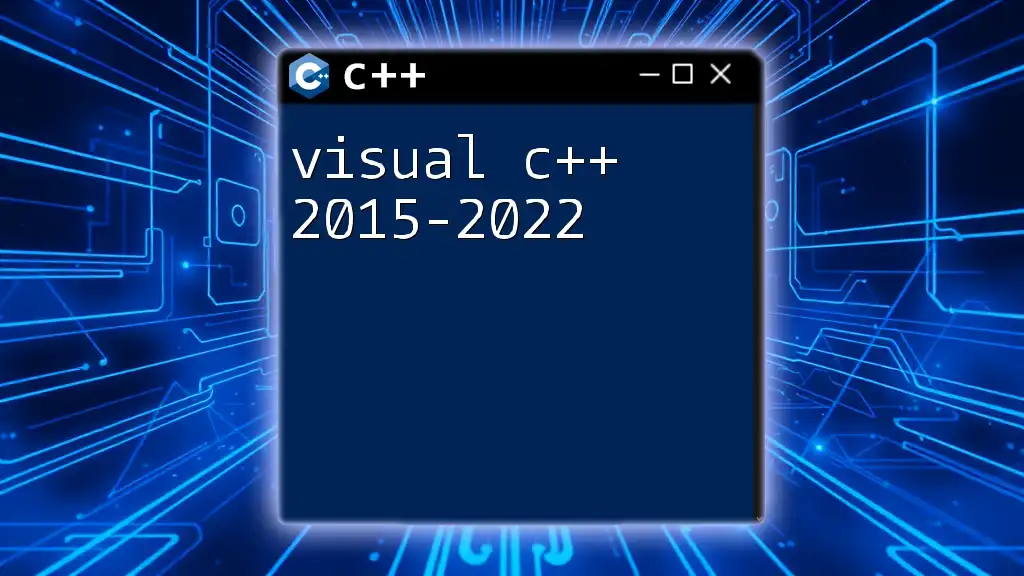
MS Visual C++ 2015 Redistributable
What is the Visual C++ 2015 Redistributable?
The Visual C++ 2015 Redistributable is a crucial component for anyone developing or using software built with MS Visual C++ 2015. It includes runtime libraries necessary for executable files and DLLs compiled with Visual C++. Unlike the full installation of Visual C++ 2015, the redistributable allows end-users to run applications without needing the entire development environment installed on their machines.
Importance of the Redistributable Package
The importance of the redistributable cannot be overstated. Applications that are built using MS Visual C++ 2015 depend on specific libraries included in the redistributable package. If these libraries are missing, users may encounter errors such as “Missing MSVCP140.dll.” Software developers should ensure that the redistributable is installed on any system that will run their applications to avoid these common pitfalls.
Installation Steps
Installing the Visual C++ 2015 Redistributable is straightforward:
- Download the appropriate package from the official Microsoft website.
- Run the installer and follow the on-screen instructions.
- To check if the redistributable is already installed, open the command prompt and execute the following command:
wmic product get name, version
Look for "Microsoft Visual C++ 2015 Redistributable" in the list to verify successful installation.
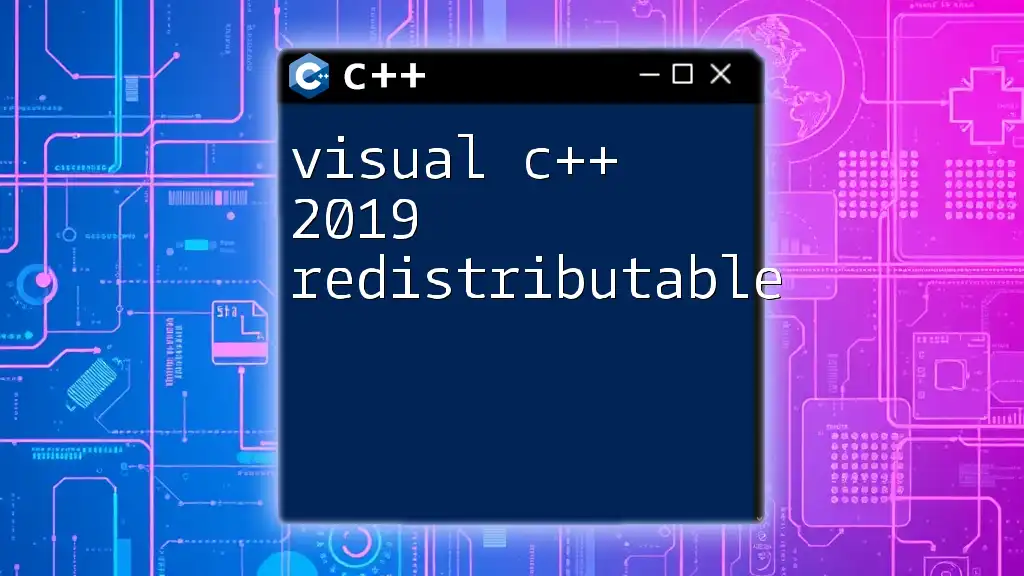
Developing Applications with Visual C++ 2015
Setting Up Your Development Environment
Getting started with MS Visual C++ 2015 involves a few simple steps. First, download and install Visual C++ 2015 Community Edition from the Microsoft website. Once installed, create a new project by selecting "File" > "New" > "Project." Choose one of the C++ templates according to the type of application you wish to build.
Writing Your First C++ Program
After setting up your environment, you can try compiling a simple program. Below is a sample code snippet that displays a classic "Hello, World!" message on the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code snippet demonstrates basic syntax: it includes the iostream library necessary for input-output operations, defines the main function, and outputs "Hello, World!" to the console.
Compiling and Running Your Application
To compile and run your newly created program, click on the "Local Windows Debugger" button in the toolbar. Visual C++ 2015 will compile your code, and if there are no errors, the output window will display your "Hello, World!" message. If you encounter compilation errors, pay attention to the error messages in the output pane, which will guide you in diagnosing issues such as syntax errors or missing semicolons.
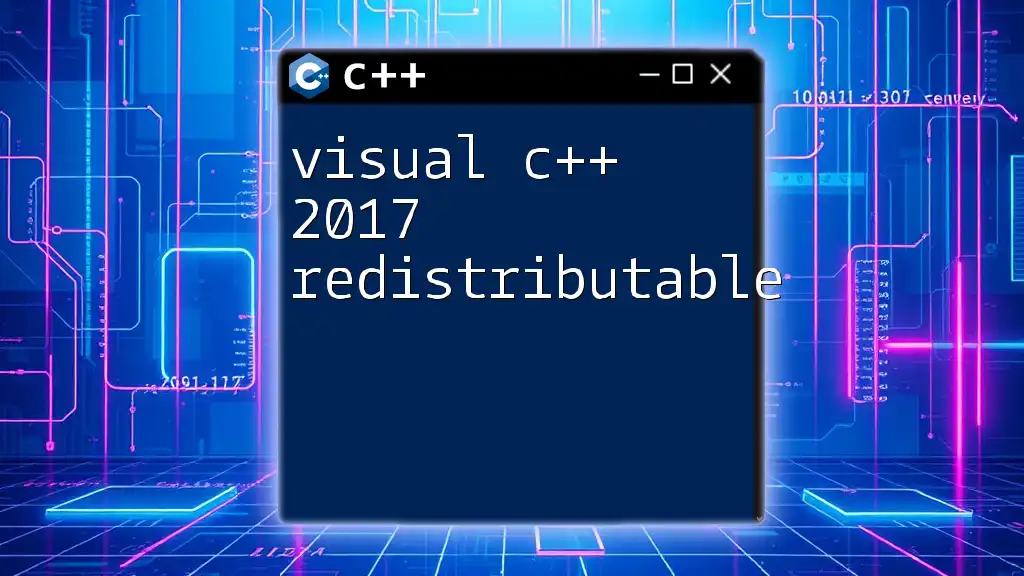
Leveraging Visual C++ 2015 Features
C++ Standard Library Features in 2015
Visual C++ 2015 supports new additions to the C++ standard library. Some notable features include `std::shared_ptr` and `std::make_unique`, which are critical for effective memory management. These features reduce the risk of memory leaks by ensuring automatic cleanup of resources, making applications more robust and efficient.
Here’s an example of using `std::shared_ptr`:
#include <iostream>
#include <memory>
class Demo {
public:
Demo() { std::cout << "Demo created!" << std::endl; }
~Demo() { std::cout << "Demo destroyed!" << std::endl; }
};
int main() {
std::shared_ptr<Demo> ptr1(new Demo());
{
std::shared_ptr<Demo> ptr2 = ptr1; // Shared ownership
std::cout << "Inside block." << std::endl;
} // ptr2 goes out of scope, but ptr1 still exists
return 0;
}
In this example, memory management is handled by `std::shared_ptr`, which provides automatic cleanup when the last pointer to the object is out of scope.
Profiling and Debugging Your Code
Debugging is an essential part of software development, and the Visual C++ 2015 debugger makes it efficient and user-friendly. You can set breakpoints by clicking in the margin next to a line of code, allowing your application to pause execution there. From this point, you can inspect variables to understand the program state.
For example, if your code isn’t producing the expected results, you might consider checking the values stored in variables by hovering over them or using the Watch Window.
Performance Optimization Techniques
To ensure your applications run efficiently, implementing performance optimization practices is fundamental. Use profiling tools integrated into Visual C++ 2015 to analyze where your application spends most of its time and identify bottlenecks.
Here are key practices to follow:
- Write efficient algorithms and choose data structures wisely.
- Minimize resource allocation and reuse objects instead.
- Eliminate unnecessary calculations inside loops.
Employing even basic optimization techniques can significantly enhance the performance of your application.
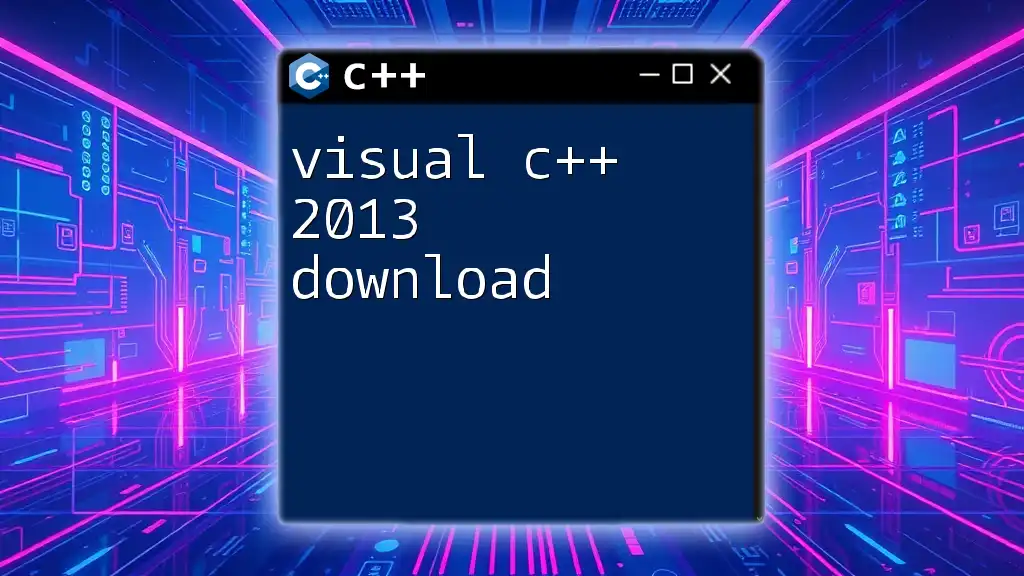
Visual C++ 2015 on Windows
Compatibility with Windows Platforms
MS Visual C++ 2015 is compatible with various Windows versions, from Windows 7 to Windows 10. This wide compatibility allows developers to create applications tailored to specific Windows environments, ensuring usability across many systems.
Deploying Your Application
Once you've developed your application, deploying it requires packaging it with the necessary files, including the Visual C++ 2015 Redistributable. Utilize Setup Projects in Visual Studio to create installation packages that can be distributed to end users. This ensures that all dependencies, including the Visual C++ libraries, are included for seamless installation.
Common deployment issues involve missing components, so always verify that those components are installed on the target system before final deployment.
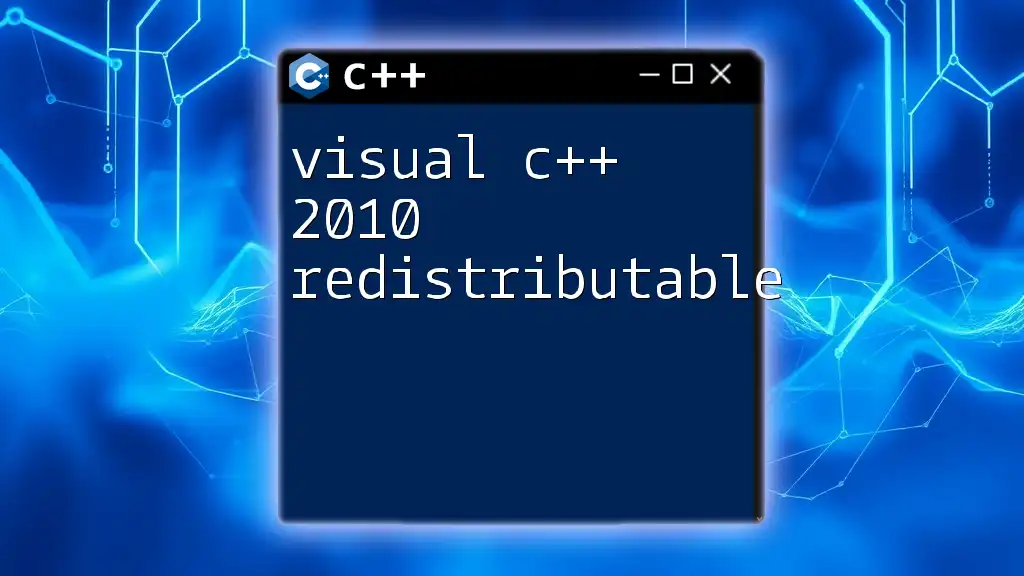
Conclusion
In summary, MS Visual C++ 2015 represents a powerful tool for developers, offering enhanced functionalities and optimizations. With a user-friendly IDE, a robust set of features, and strong community support, it remains a relevant choice in the C++ development landscape. As you gain experience, continue to explore its capabilities to unlock the full potential of your programming endeavors.
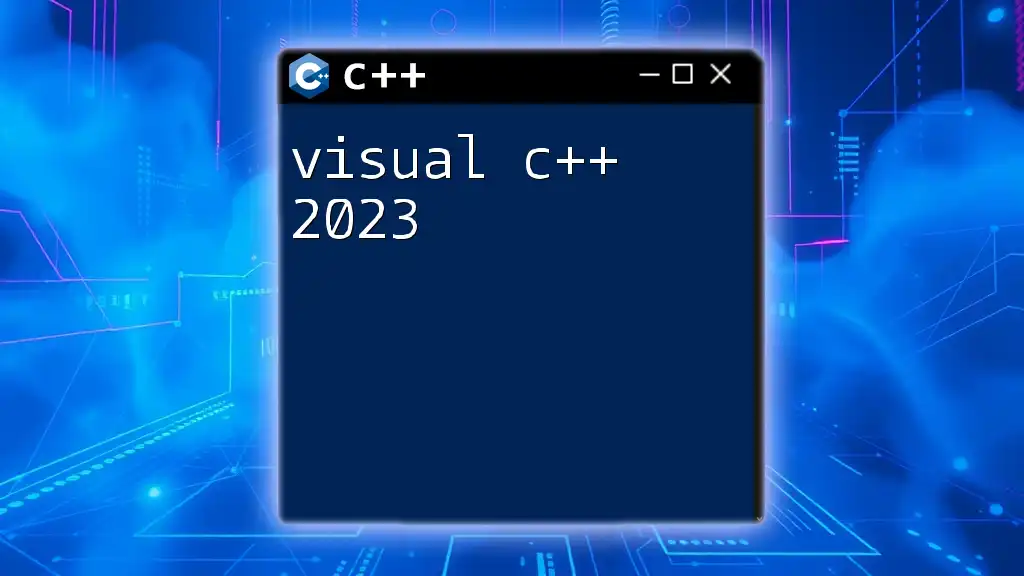
Additional Resources
For further knowledge enhancement, make sure to explore Microsoft’s official documentation. Websites and resources such as online courses, forums, and C++ programming communities can be invaluable in sharpening your skills and addressing any challenges you encounter.
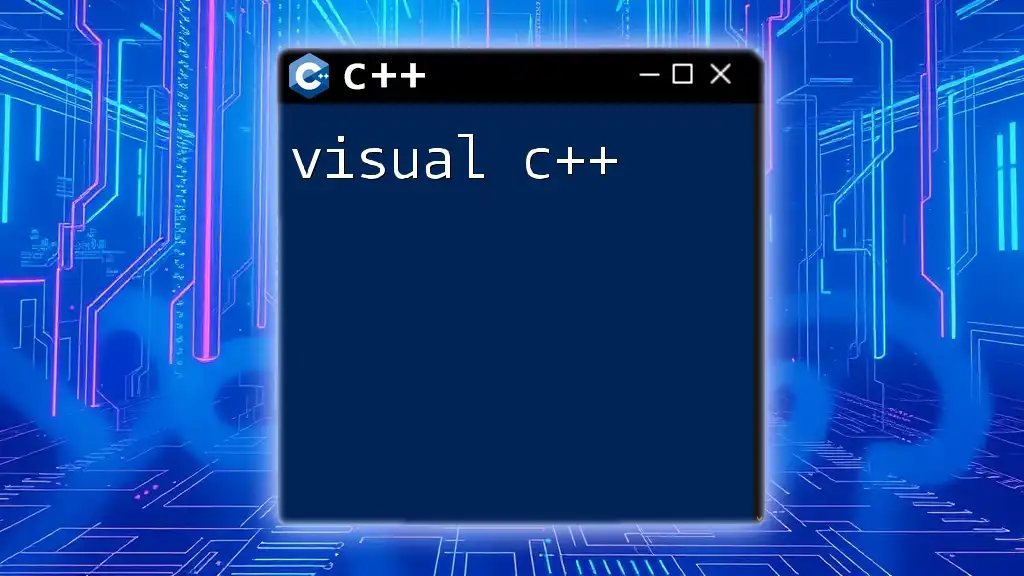
Call to Action
Take the next step in your C++ journey by joining our newsletter or participating in our courses designed to deepen your understanding of MS Visual C++ 2015. Share your experiences, questions, or tips in the comments section below, and engage with fellow programmers to foster a thriving community of learners!