The Visual C++ 2010 Redistributable is a package that installs the runtime components required to run applications developed with Visual C++ 2010 on a computer that does not have Visual Studio 2010 installed.
Here’s a simple example to demonstrate how to use a command in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Redistributable Packages
What is a C++ Redistributable Package?
A C++ redistributable package is a collection of runtime components necessary for running applications developed with Visual C++. When a program is built using Visual Studio, it often relies on specific libraries from the C++ runtime. The redistributable package ensures that these libraries are available on users' systems, allowing applications to function correctly without needing each user to install Visual Studio.
Why Do You Need the C++ Redistributable Package 2010?
The Visual C++ 2010 Redistributable is crucial for developers and users alike. Many applications built on Visual C++ 2010 require this package to run, especially if:
- The program uses dynamic memory allocation, exception handling, or STL (Standard Template Library) features that rely on the runtime.
- End-users need to run the application on machines that don’t have the development environment installed.
Incompatibility issues may arise if the runtime components are missing, leading to runtime errors that can frustrate users.
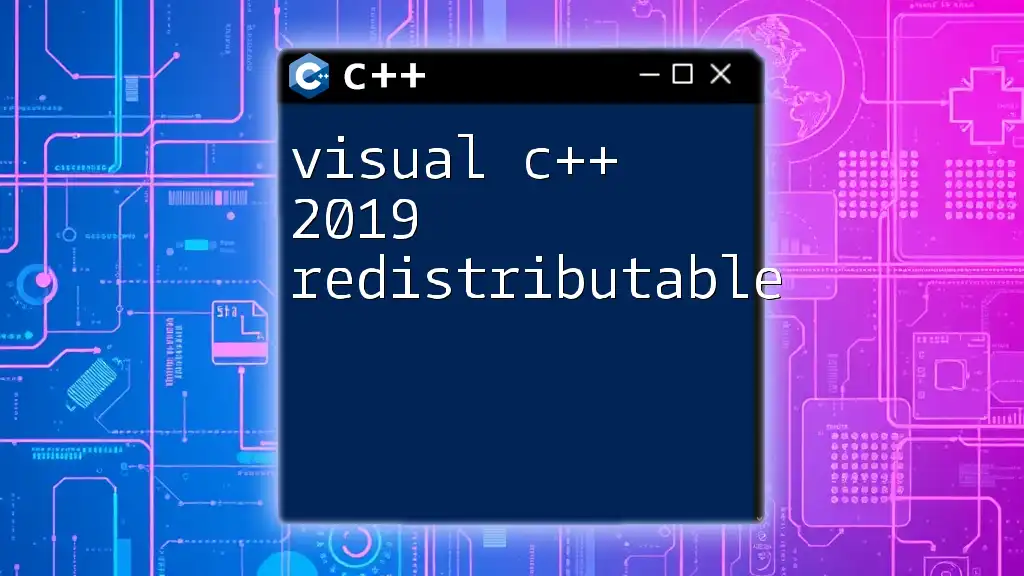
Visual C++ 2010 Redistributable
Overview of Visual C++ 2010
Visual C++ 2010 introduced several enhancements over its predecessors, including improved compiler optimizations and better support for C++ standards. This version supports both 32-bit and 64-bit applications, making it flexible for various development needs. The major features include:
- Better debugger and profiling tools
- Support for the C++0x features (now known as C++11)
- Enhanced performance for managed code
Core Components of Visual C++ 2010 Runtime
The Visual C++ 2010 runtime includes various components, such as:
- C runtime library (CRT): Provides fundamental routines for handling tasks such as input/output and memory management.
- Standard C++ Library: Includes features for object-oriented programming, exceptions, and STL containers.
- MFC (Microsoft Foundation Classes): For developing Windows applications.
Here’s a simple example showing how to allocate memory dynamically, demonstrating how the runtime handles memory with proper error checking.
#include <iostream>
#include <cstdlib>
int main() {
int* arr = (int*)malloc(5 * sizeof(int));
// Check allocation success
if(arr == nullptr) {
std::cerr << "Memory allocation failed." << std::endl;
return 1;
}
// Usage of arr
// Free allocated memory
free(arr);
return 0;
}
Installing Visual C++ 2010 Redistributable
Step-by-Step Installation Guide
Installing the Visual C++ 2010 Redistributable is straightforward:
- System requirements: Ensure that your operating system supports it. Generally, it's compatible with Windows Vista, Windows 7, and Windows 8.
- Downloading the redistributable package: You can find it on Microsoft’s official website. Be sure to choose the correct architecture (x86 for 32-bit or x64 for 64-bit).
- Installation process:
- Launch the downloaded installer and follow the prompts.
- Choose to install either the x86 version for 32-bit applications or the x64 version for 64-bit applications.
Common Installation Issues
Sometimes users face installation errors, mostly due to the following:
- Previous versions: Ensure that older versions of the redistributable are uninstalled before installing a new version.
- Administrative rights: Run the installer with administrator privileges to prevent permission-related failures.
To verify the installation, check the Control Panel > Programs and Features for the Visual C++ 2010 Redistributable.
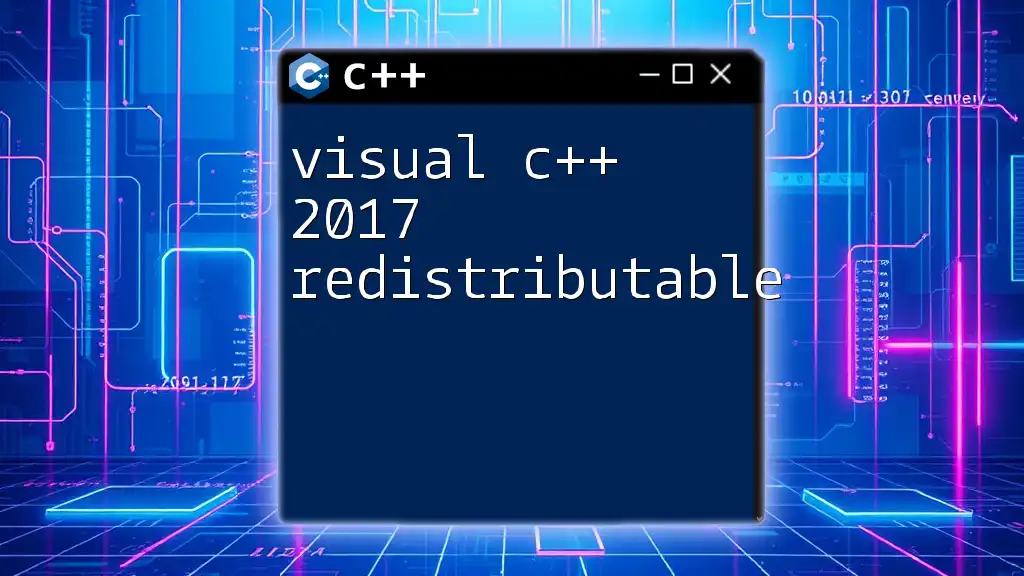
Using the Visual C++ 2010 Runtime in Your Applications
Setting Up Your Project
When developing in Visual Studio 2010, it’s essential to configure your project to link against the runtime libraries. Here’s how to set it up:
- Open the project properties.
- Navigate to Configuration Properties > C/C++ > Code Generation.
- Ensure that the Runtime Library is set appropriately (e.g., Multi-threaded DLL).
Code Examples
Basic Example of Exception Handling
Handling exceptions properly is a critical aspect of robust C++ code. The following example illustrates a simple throw/catch mechanism using runtime features:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An example exception");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
This example shows how utilizing standard exception handling enhances application reliability and user experience.
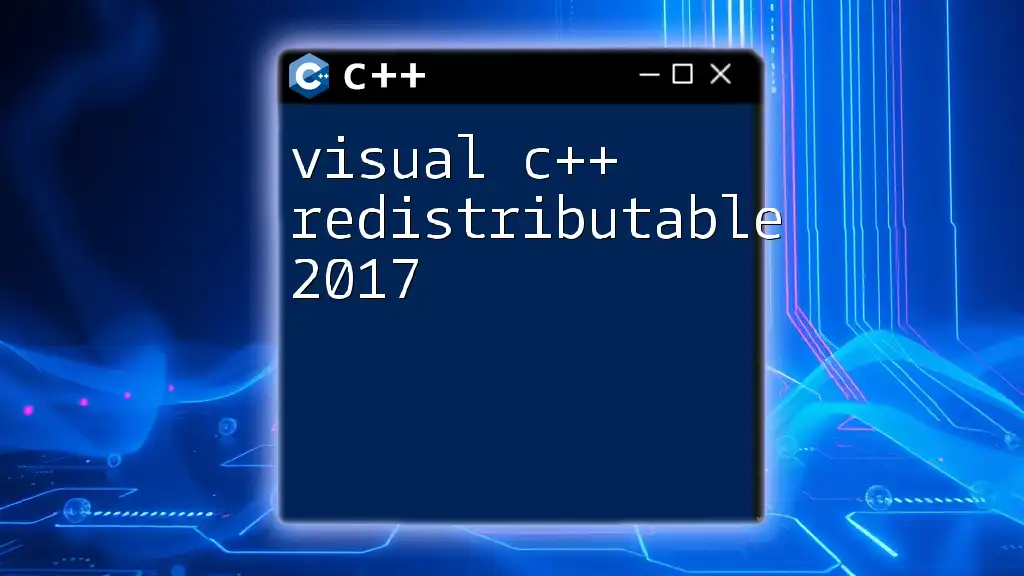
Differences Between Visual C++ Redistributable Versions
Comparing Versions
The landscape of Visual C++ redistributables has evolved over the years. Key differences between Visual C++ 2010 and other versions include:
- Performance improvements in runtime operations, including more efficient memory management in newer versions.
- Support for additional language features in later releases, making them more suitable for modern C++ development.
Choosing the Right Redistributable Version
Selecting the appropriate redistributable depends largely on your development environment and target audience. If you're working on legacy applications, sticking with the Visual C++ 2010 Redistributable may be essential. However, for new projects, consider using the latest version that aligns with your application requirements, as it will provide better features and support.
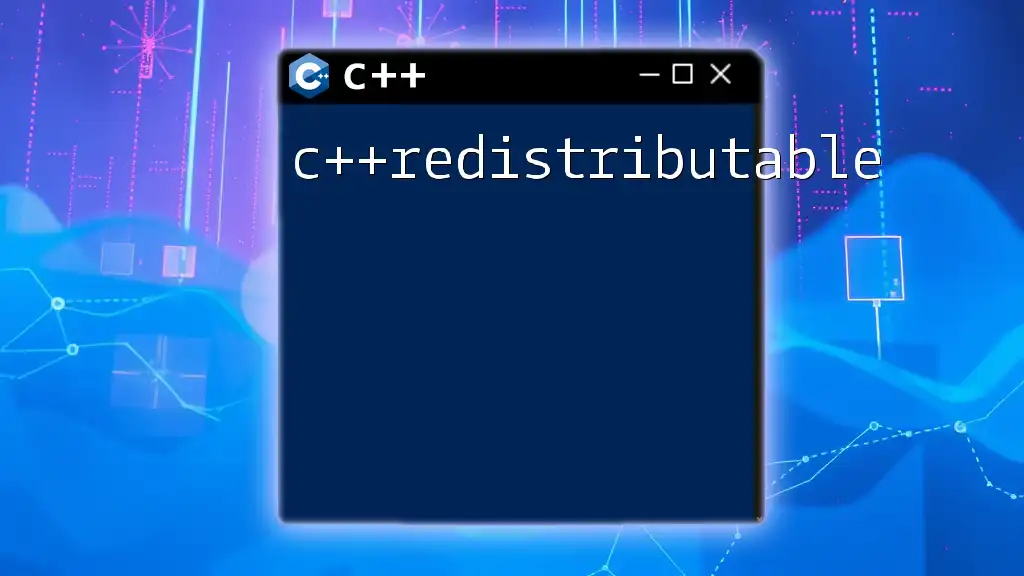
Conclusion
The Visual C++ 2010 Redistributable plays a fundamental role in ensuring that applications built with Visual C++ can run seamlessly on user machines. By comprehensively understanding and utilizing this redistributable, developers can swiftly tackle common issues and enhance application performance. As you integrate the knowledge of redistributables into your development practices, remember that a well-configured environment can significantly impact your application's success.
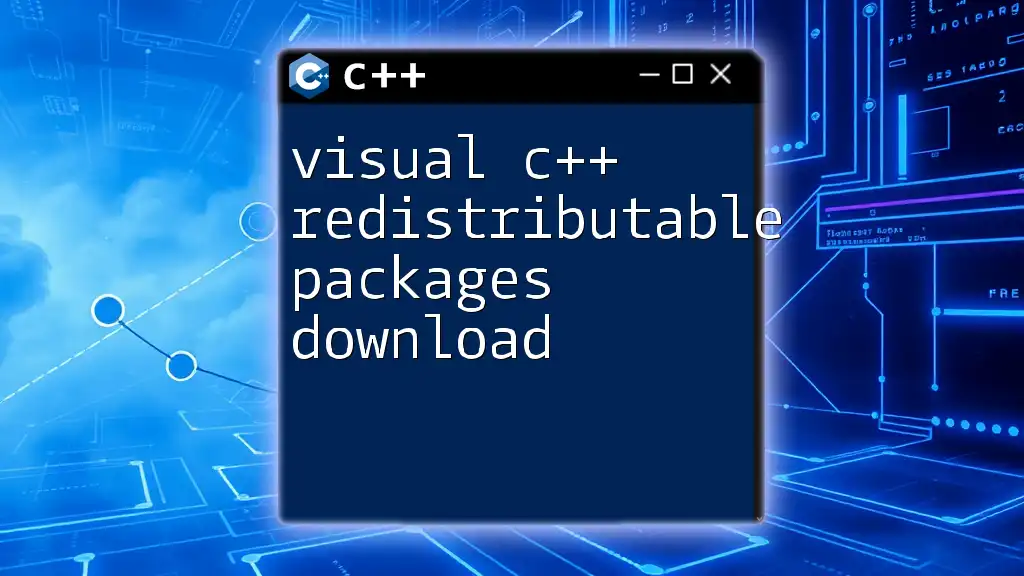
Additional Resources
For further insights, consider exploring official Microsoft documentation, recommended development tools, and engaging with online communities that focus on C++ development for more support and knowledge sharing.