C++17 introduced several features, such as structured bindings and optional types, which simplify code and enhance functionality.
Here's a code snippet demonstrating structured bindings:
#include <tuple>
#include <iostream>
int main() {
auto person = std::make_tuple("Alice", 30);
auto [name, age] = person; // Structured binding
std::cout << "Name: " << name << ", Age: " << age << std::endl;
return 0;
}
What is C++ 17?
C++ 17 represents a significant advancement in the C++ programming language, introducing new features that enhance its usability, performance, and expressiveness. Building upon the previous versions (C++ 11 and C++ 14), C++ 17 focuses on improving the standard library, simplifying code, and optimizing performance. Understanding these features is crucial for modern C++ development, allowing developers to write more efficient and maintainable code.
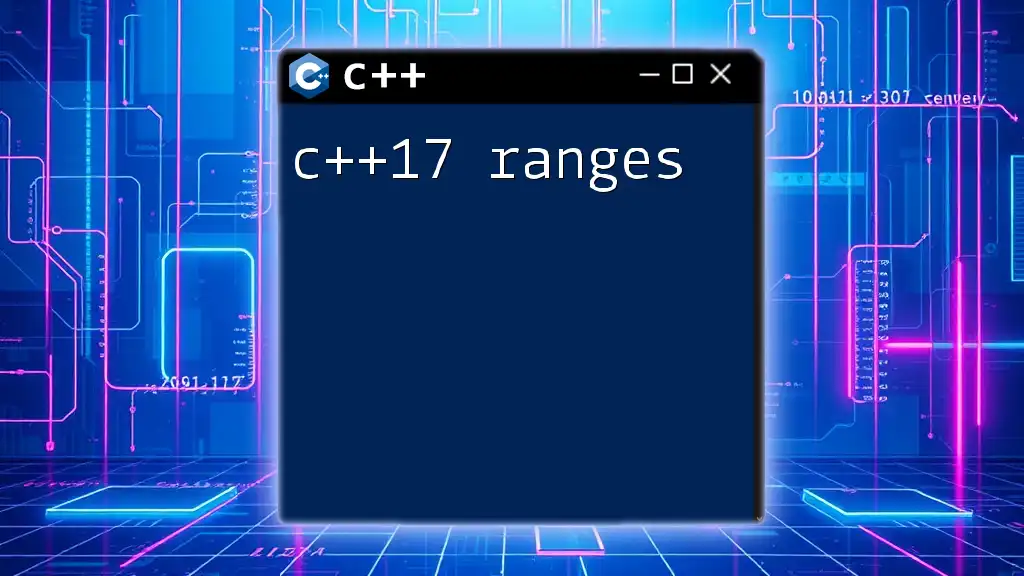
Key Features of C++ 17
Structured Bindings
One of the standout features in C++ 17 is structured bindings, which allow for more intuitive syntax when unpacking values from tuples, pairs, or arrays. Instead of accessing elements one by one, you can simultaneously declare multiple variables.
Example:
auto [x, y] = std::make_tuple(1, 2);
In this code, the pair of values returned by `std::make_tuple` is unpacked directly into the variables `x` and `y`. This feature not only simplifies the code but also significantly enhances readability, making it easier to maintain and understand at a glance.
If constexpr
The `if constexpr` statement enables compile-time conditional execution, allowing different code paths to be chosen based on whether a certain condition is true during compilation. This feature is particularly useful in template metaprogramming, offering a powerful mechanism for avoiding unnecessary code execution.
Example:
template <typename T>
void process(T value) {
if constexpr (std::is_integral_v<T>) {
// Code for integral types
} else {
// Code for other types
}
}
With `if constexpr`, only the branch that matches the type will be included in the final compiled code. This leads to more optimized binaries and can reduce runtime overhead.
Inline Variables
C++ 17 introduces the concept of inline variables, which allow for variable definitions to be shared across multiple translation units without violating the One Definition Rule. This feature is particularly beneficial for defining constants.
Example:
inline constexpr double pi = 3.14159;
Using inline variables means you can define `pi` in a header file without worrying about multiple definitions, all while maintaining the clarity of a single declaration.
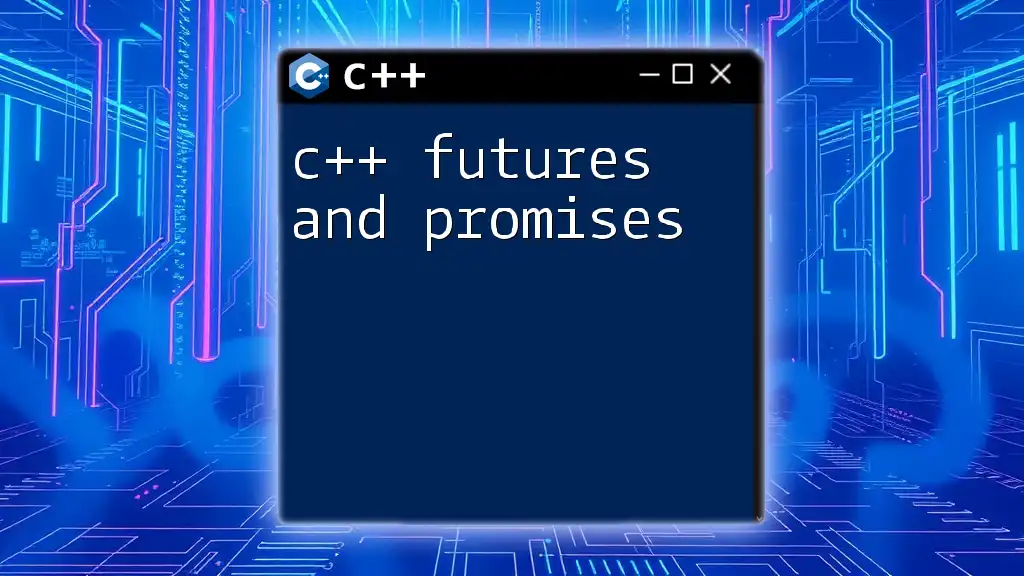
New Container Features
std::optional
The `std::optional` is a powerful utility that represents an object that may or may not contain a value. It is particularly useful for functions that may not have a return value and can help avoid using pointers or special sentinel values to indicate "no value."
Example:
std::optional<int> GetNumber(bool returnNumber) {
if (returnNumber) return 42;
return std::nullopt;
}
Here, `GetNumber` returns an `int` wrapped in `std::optional`, allowing the caller to check if a number was returned without needing to deal with null pointers.
std::variant
Another addition to the STL is `std::variant`, a type-safe union that can hold one of several types. This feature brings type safety to situations where a variable may need to adapt its type based on different conditions.
Example:
std::variant<int, std::string> var;
var = 42; // valid assignment
var = "Hello"; // valid assignment
By utilizing `std::variant`, developers can manage multiple types without the risks associated with traditional unions.
std::any
The `std::any` type is a container for single values of any type, providing a means to store and manipulate heterogeneous data.
Example:
std::any myData = 42;
myData = std::string("Hello, World!");
The ability to store any type of value makes `std::any` particularly useful in situations where the type is not known at compile time, such as with containers that need to store objects of different types.
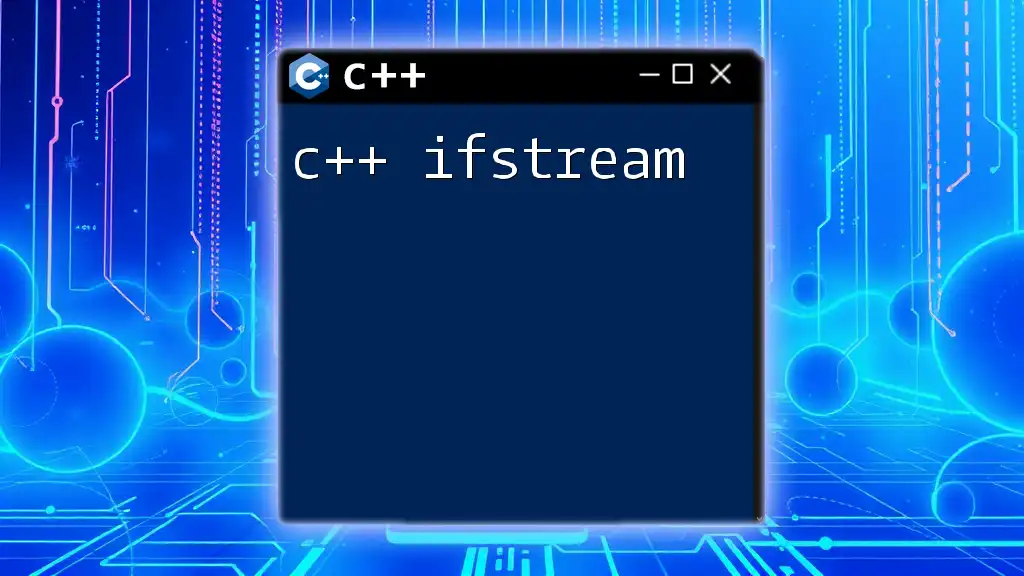
Enhancements in STL
New String and Container Functions
C++ 17 adds several new functions to strings and containers, improving their usability and flexibility.
For instance, `std::string` now includes the `starts_with` and `ends_with` member functions, allowing developers to check the beginning and end of strings without reverting to cumbersome methods.
Examples:
std::string str = "Hello, World!";
bool starts = str.starts_with("Hello"); // returns true
bool ends = str.ends_with("World!"); // returns true
These new string methods streamline string manipulations, leading to cleaner and more expressive code.
std::filesystem
The introduction of the file system library, `std::filesystem`, provides a standardized platform for file and directory manipulation. It allows developers to easily work with paths, files, and directories in a way that's both intuitive and portable.
Example:
namespace fs = std::filesystem;
fs::path p = "test.txt";
if (fs::exists(p)) {
// File processing logic here
}
With `std::filesystem`, file management becomes significantly more straightforward and reduces the risk of errors compared to traditional methods.
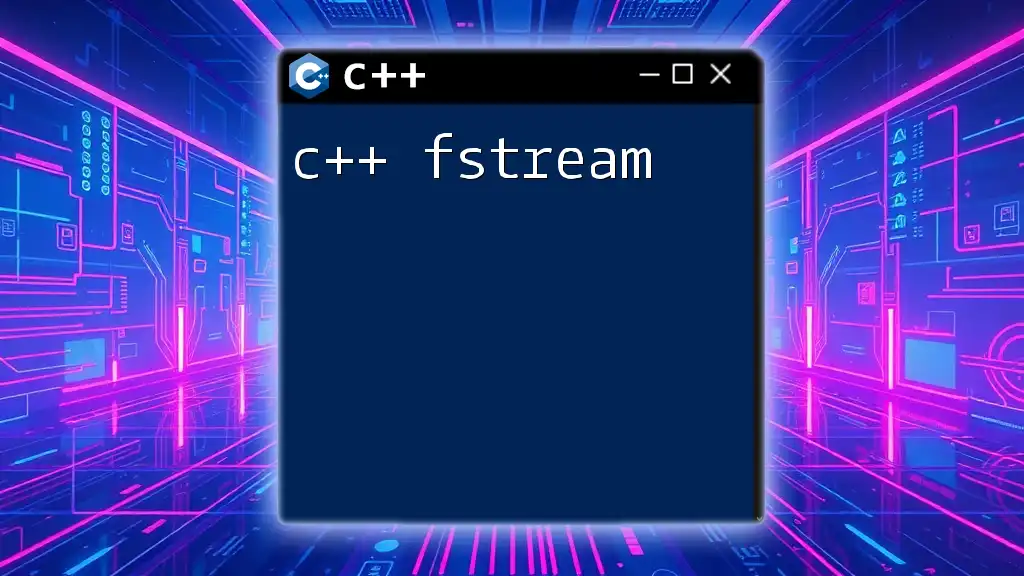
C++ 17 Improvements in Lambdas
C++ 17 has introduced important improvements to lambda expressions, making them even more powerful and versatile.
Lambda Expressions: The syntax for defining and using lambda expressions remains unchanged, but the features surrounding them have evolved. One notable improvement is the capability for lambdas to capture variables by move.
Example:
auto lambda = [](int a, int b) { return a + b; };
Capture by Move:
auto lambda = [data = std::move(some_data)] { /* ... */ };
These enhancements allow developers to write more robust and efficient lambda expressions, making them an integral part of modern C++ programming.
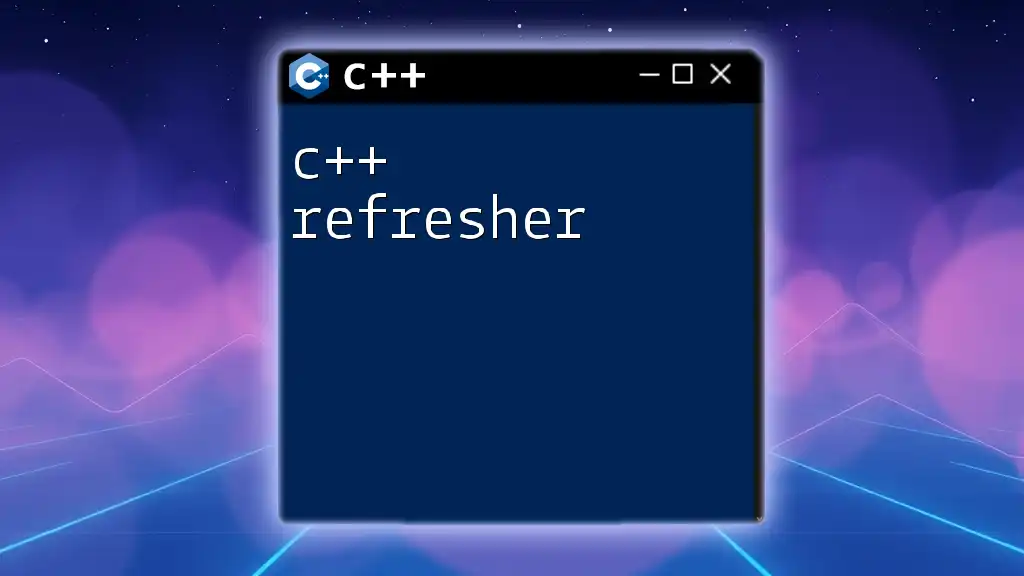
Parallel Algorithms
C++ 17 introduces parallel algorithms, providing easy-to-use interfaces that can enhance performance by leveraging multi-core processors. The standard library now supports execution policies that specify whether algorithms should operate sequentially or in parallel.
Example:
#include <execution>
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
std::transform(std::execution::par, vec.begin(), vec.end(), vec.begin(), [](int x) { return x * x; });
By using `std::execution::par`, this code takes advantage of parallel processing, which can dramatically reduce computation time for large datasets.
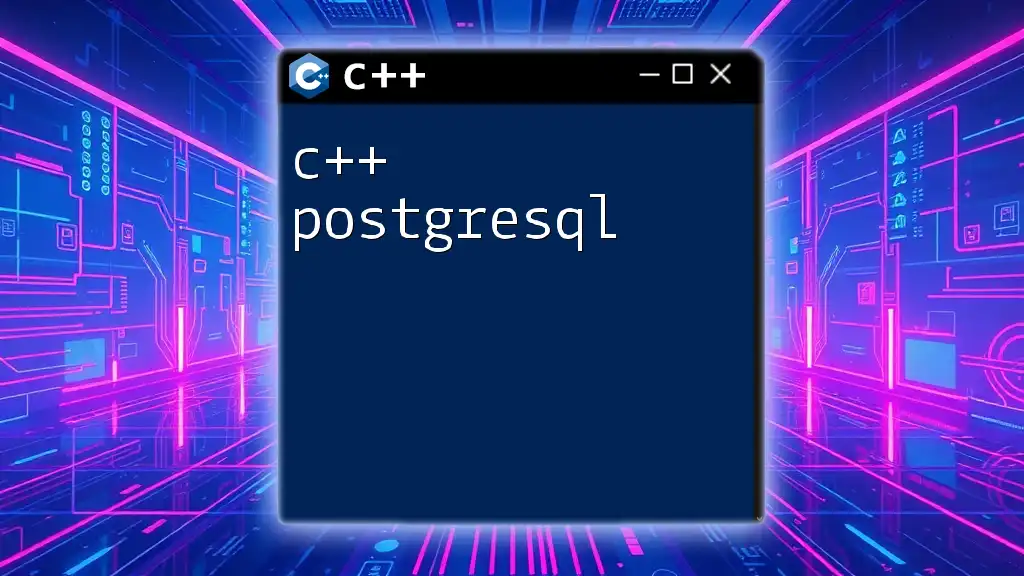
Conclusion
C++ 17 features significantly enhance the language's flexibility, efficiency, and ease of use. By adopting principles such as structured bindings, `std::optional`, `std::variant`, and parallel algorithms, developers can write cleaner and more efficient code. Familiarizing yourself with these features is essential for programmers aiming to leverage the full power of modern C++ effectively. Embracing these advancements not only improves individual projects but also fosters better practices in the C++ development community.
Further Reading and Resources
To dive deeper into C++ 17 features, consult the official C++ documentation, practice coding through tutorials, and explore comprehensive books that cover these advancements in detail. Understanding C++ 17 features will empower you to enhance your programming skills and make substantial contributions to modern software development.