The `std::reverse` function in C++ is used to reverse the order of elements in a range, such as an array or a vector.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::reverse(vec.begin(), vec.end());
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
Understanding the Reverse Function in C++
The reverse function in C++ is an essential tool for manipulating sequences in a quick and efficient manner. It provides a way to reverse elements within a container, making it easier to work with data structures without having to implement complex logic.
How the Reverse Function Works
The reverse function operates by swapping elements in a sequence, effectively repositioning them from their original order to the opposite end. Each element at position `i` will be swapped with the element at position `n-i-1`, where `n` is the total number of elements. This simple mechanism allows for in-place reversal, meaning that it does not require additional storage for another copy of the data.
The time complexity of the reversal operation is O(n), which is efficient as it only requires a single pass through the data.
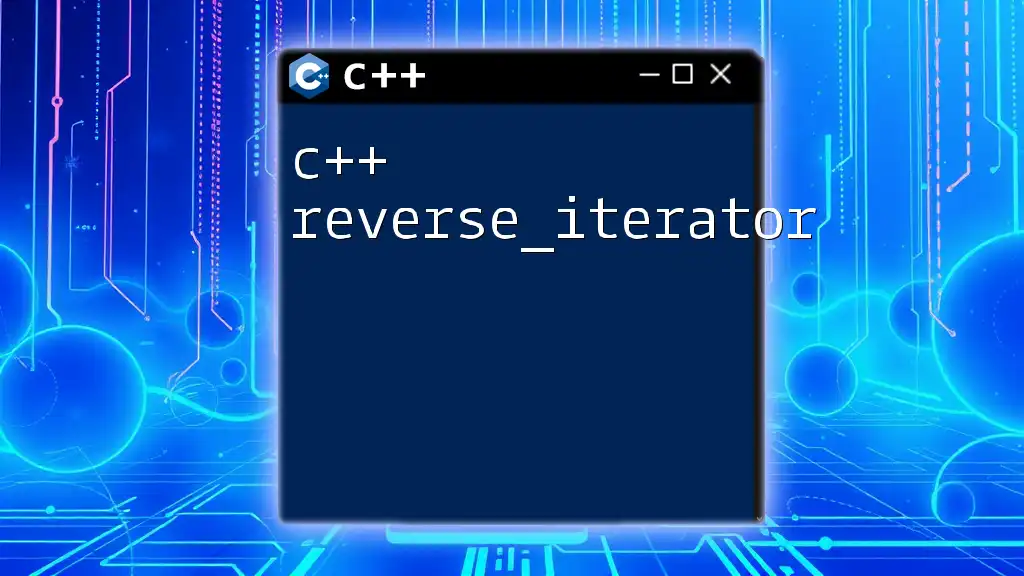
Implementing the Reverse Function in C++
When using C++, the reverse function is found in the `<algorithm>` header. This easily accessible library offers a wide range of functionalities, including our focus: reversing sequences.
Syntax and Parameters
The syntax for the `std::reverse` function is straightforward:
void reverse(RandomAccessIterator first, RandomAccessIterator last);
The parameters `first` and `last` define the range of the sequence to be reversed, from the beginning to just past the last element.
Example Usage of std::reverse
Example 1: Reversing a Simple Array
Let's look at a simple example of reversing an array:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::reverse(arr, arr + 5);
for(int i : arr) {
std::cout << i << " ";
}
return 0;
}
In this code snippet, we include the necessary headers and define an array. We then call `std::reverse`, passing the start and end of the array to reverse its elements. The output will be `5 4 3 2 1`, demonstrating a successful reversal.
Example 2: Reversing a Vector
Vectors are another common data structure in C++. Here’s how to reverse a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::reverse(vec.begin(), vec.end());
for(int i : vec) {
std::cout << i << " ";
}
return 0;
}
In this example, we use `std::reverse` with `vec.begin()` and `vec.end()` to specify the complete range of the vector. The output will be `50 40 30 20 10`, confirming that the sequence has been effectively reversed.
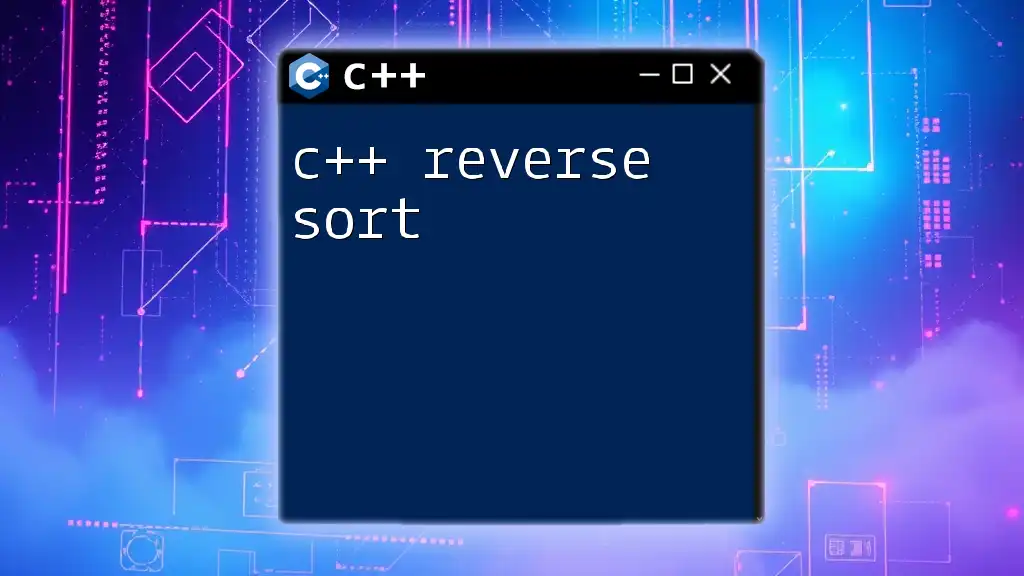
Reversing a String in C++
Reversing a string can be just as straightforward with the `std::reverse` function. This functionality is crucial when you need to manipulate textual data or require a reversed representation of a string.
Example: Reversing a String
Here’s how we can reverse a string using `std::reverse`:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end());
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
In the above code, we create a string and apply `std::reverse` to the entire string. The output shows `!dlroW ,olleH`, indicating that our operation was successful.
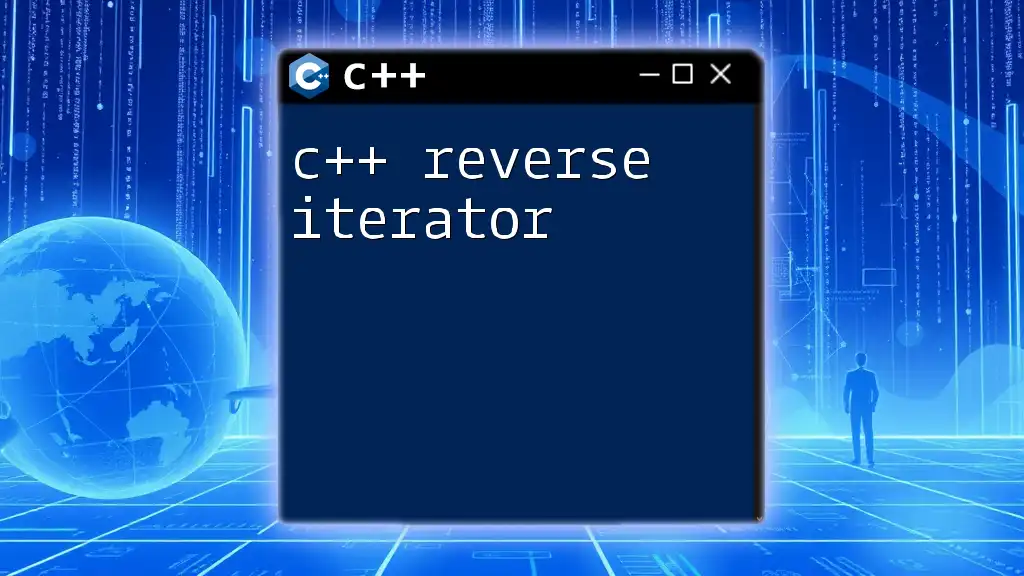
Custom Reverse Function Implementation
Sometimes, you might want to create your own reverse function, especially if you want to understand the underlying mechanics or if you are dealing with specific situations. This can improve your understanding of how data can be manipulated programmatically.
Example: Custom Reverse Function
Here is a simple custom implementation of a reverse function:
#include <iostream>
#include <vector>
void custom_reverse(std::vector<int>& vec) {
int n = vec.size();
for(int i = 0; i < n / 2; ++i) {
std::swap(vec[i], vec[n - i - 1]);
}
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
custom_reverse(vec);
for(int num : vec) {
std::cout << num << " "; // Output: 5 4 3 2 1
}
return 0;
}
In this custom implementation, we loop through half the vector and swap each element with its counterpart from the end. This method is effective and reinforces understanding of the reversal process. The output confirms that the reversal was successful with `5 4 3 2 1`.
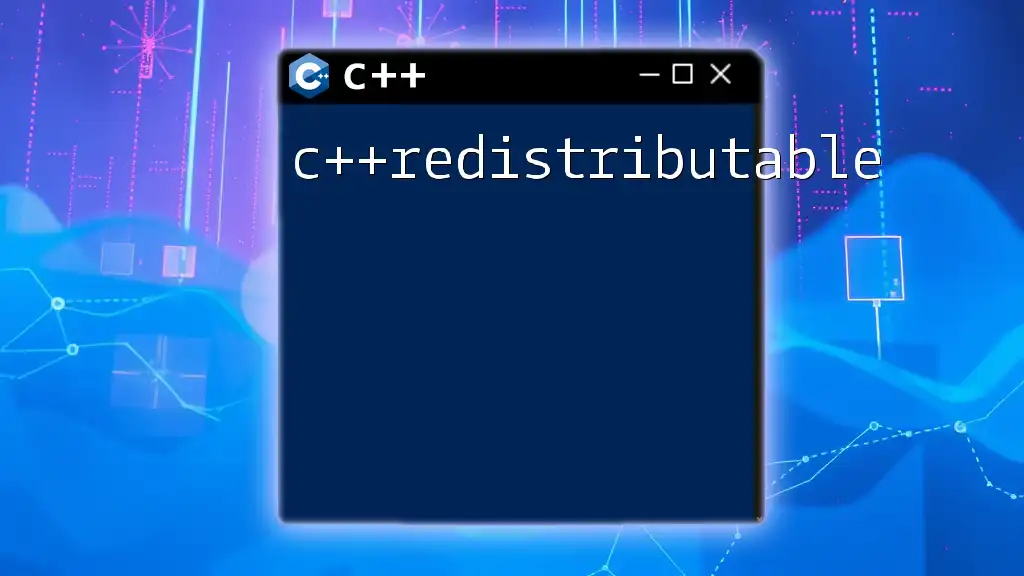
Common Pitfalls and How to Avoid Them
While working with the reverse function, it’s essential to be aware of certain common pitfalls.
- Data Types: Ensure you are working with containers that support random access iterators (e.g., arrays, vectors). If you attempt to reverse a type that does not support this, you will encounter compilation errors.
- Off-by-One Errors: Be cautious of index boundaries. When manually implementing reversal, off-by-one errors can lead to unexpected behavior or segmentation faults.
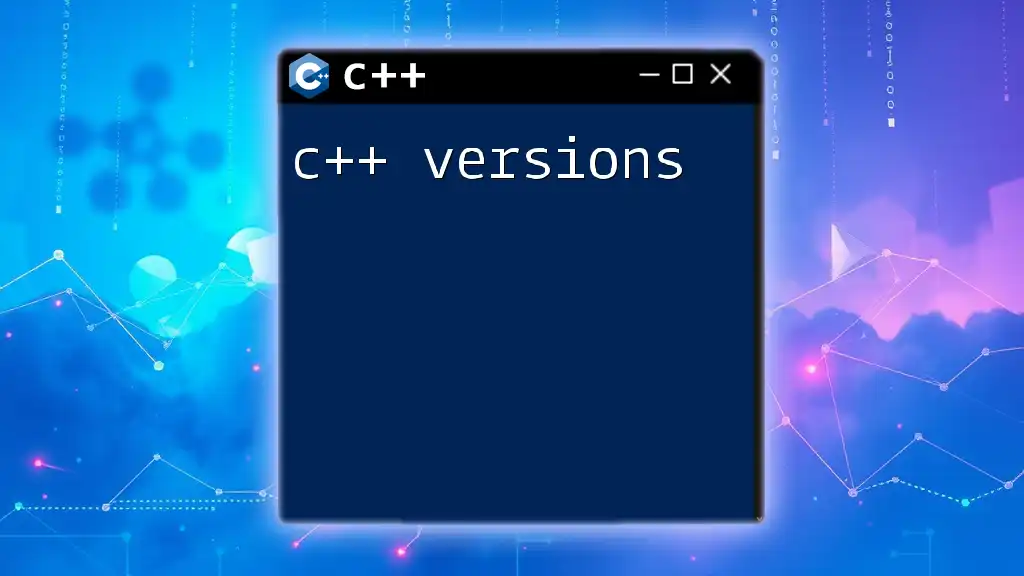
Practical Applications of C++ Reverse
The applications of reversing sequences are vast and varied. Reversal is often necessary in many algorithms, such as sorting and searching methods, enabling effective data manipulation.
In game development, for instance, you may need to reverse the order of elements, such as sprite lists or game objects, for visual or logical representation during gameplay.
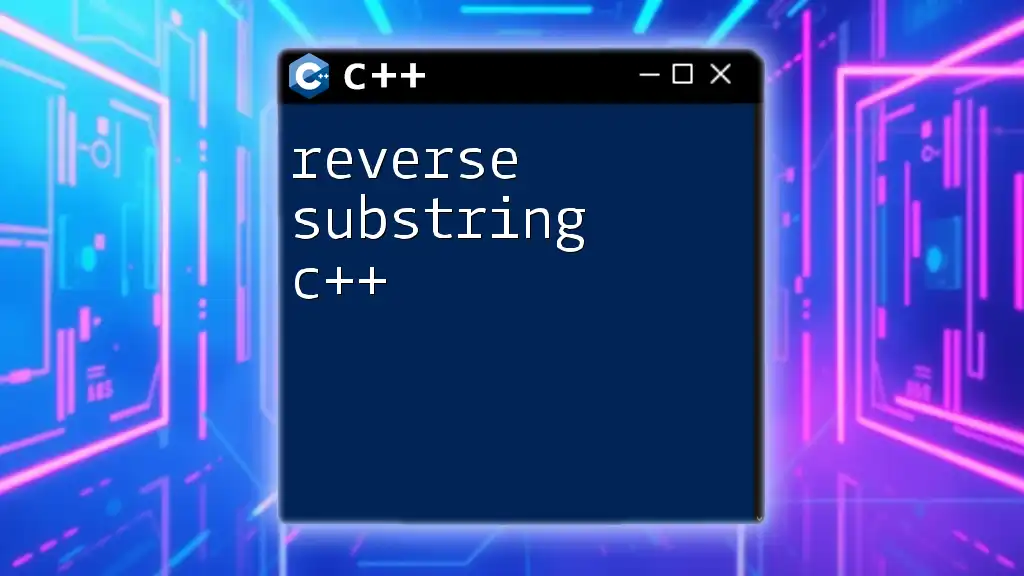
Conclusion
The C++ reverse function is a powerful and efficient tool for manipulating sequences effortlessly. Mastering its usage is essential for anyone looking to excel in C++ programming. By practicing with various data types and creating custom implementations, you can build a robust understanding of this versatile function.
Keep exploring more C++ commands and tools to further enhance your programming skill set!
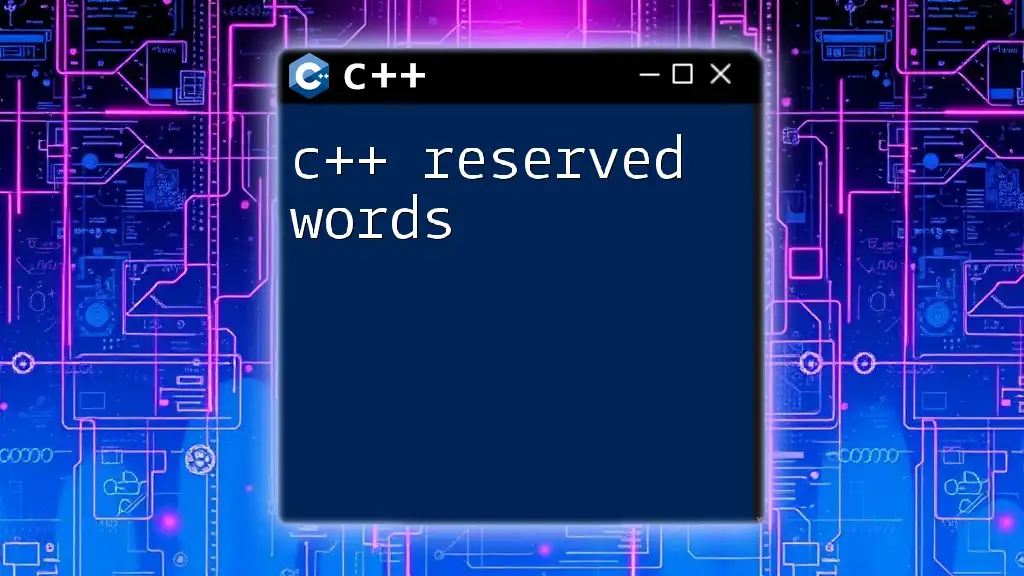
Additional Resources
To dive deeper into C++ and the reverse functionality, consider referring to the official C++ documentation or exploring online courses that focus on algorithm manipulation and data structure handling. Books and tutorials that emphasize practical coding exercises can also provide great value in your learning journey.