A C++ parser is a tool that analyzes and interprets C++ code to validate syntax, generate abstract syntax trees, or transform code into a different representation.
Here's a simple example of how a basic C++ parser might be structured:
#include <iostream>
#include <string>
void parse(const std::string& code) {
// A simplistic parser example
if (code.find("int") != std::string::npos) {
std::cout << "This code contains an integer declaration." << std::endl;
} else {
std::cout << "No integer declaration found." << std::endl;
}
}
int main() {
std::string cppCode = "int main() { return 0; }";
parse(cppCode);
return 0;
}
Introduction to C++ Parsers
A parser is a vital component in many programming and data processing systems. It takes structured input (such as source code or data files) and transforms it into a format that is easy to manipulate or understand by computers. Specifically in C++, parsing is critical for developing compilers, interpreters, and other utilities that require structured data interpretation.
Real-world applications of C++ Parsers
C++ parsers find applications in various fields:
- Compilers and Interpreters: These systems use parsers to read source code, check for syntax rules, and convert the code into a form the machine can understand.
- Data Processing Applications: Parsing allows for extraction and manipulation of data from files or streams, making it essential in data analysis and transformation tasks.
- Configuration File Parsing: Configuration files often require reading, interpreting, and converting into usable data structures, where C++ parsers excel.

Understanding the Parsing Process
The parsing process can be broken down into several key stages:
Lexical Analysis
Lexical analysis is the first stage where the input data is divided into tokens. Tokens are meaningful sequences of characters, such as keywords, identifiers, and symbols.
For example, in C++, the code snippet:
int main() { return 0; }
is analyzed into tokens: `int`, `main`, `(`, `)`, `{`, `return`, `0`, and `}`.
Syntax Analysis
In the syntax analysis stage, the parser checks if the sequence of tokens follows the grammatical rules of the language. This ensures that the structure of the code is valid.
For instance, if a parser encounters `int 42;`, it would raise an error, as declaring an integer variable without an identifier is syntactically incorrect.
Semantic Analysis
Once the syntax is deemed correct, semantic analysis takes place. This phase verifies the meaning of the statements, ensuring that actions in the code are permissible. For example, it checks if variables are declared before being used.

Types of Parsers in C++
When working with C++, you can encounter various types of parsers, primarily categorized into top-down and bottom-up parsers.
Top-Down Parsers
Top-down parsers construct the parse tree from the top (root) down to the leaves. They predict the structure of the input based on grammar rules.
Recursive Descent Parser
A common top-down parsing strategy is the recursive descent parser. It uses a set of recursive procedures for parsing the grammar rules.
Here’s a brief look at how it might be structured:
void parseExpression() {
if (token == NUMBER) {
consume(NUMBER);
} else {
throw std::runtime_error("Expected a number.");
}
}
Bottom-Up Parsers
Bottom-up parsers build the parse tree from the leaves up to the root. These parsers try to reduce the input to the start symbol.
Shift-Reduce Parser
The shift-reduce parser is an illustrative example of a bottom-up parser. It works by shifting tokens onto a stack and then reducing them into non-terminals.
A hypothetical code snippet might look like this:
void shift() {
// code to shift the current token onto the stack
}

Implementing a Basic C++ Parser
To create a C++ parser, you must start with two primary components: the lexer and the parser.
Building the Lexer
A lexer is responsible for tokenizing the input. It reads the input string and outputs a vector of tokens.
Here’s a simple structure for a token:
struct Token {
std::string type;
std::string value;
};
The lexer function would define the logic for splitting input:
std::vector<Token> lex(const std::string& input) {
// Tokenization logic here
}
Constructing the Parser
The parser connects with the lexer to parse the tokens produced. It uses a specific algorithm to interpret the series of tokens and generates a parse tree or an abstract syntax tree.
You may start with a simple function for parsing:
void parse(const std::vector<Token>& tokens) {
// Parsing logic here
}
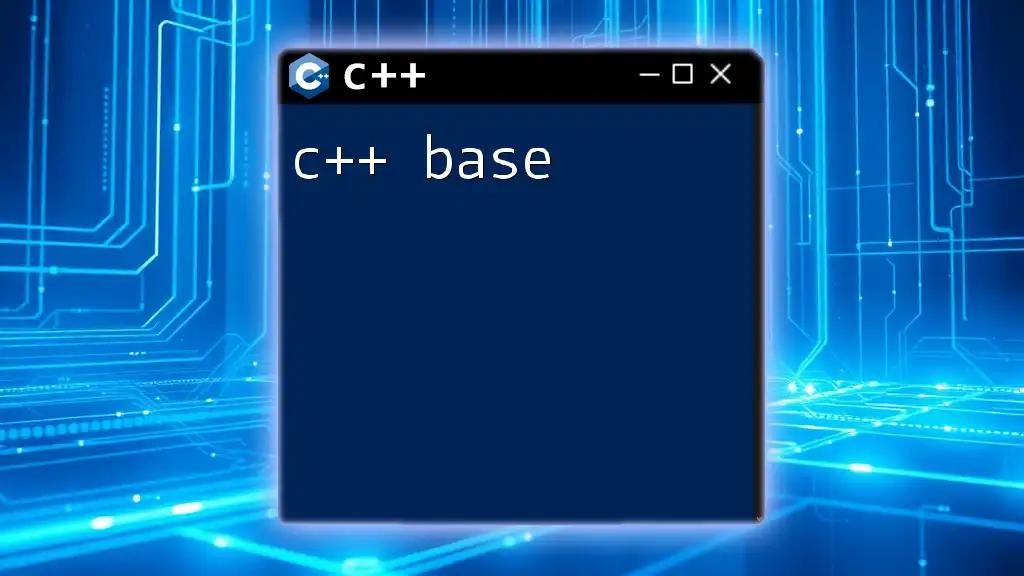
Error Handling in Parsing
Error handling is crucial in parsing. The parser must gracefully manage various errors to provide meaningful feedback.
Common Parsing Errors
- Syntax Errors: These occur when the token sequence does not conform to the expected grammar, such as missing semicolons or unmatched parentheses.
- Semantic Errors: Occur when valid syntax doesn't make logical sense, like using a variable before declaring it.
Strategies for Error Detection and Recovery
-
Reporting Informative Error Messages: Provide context for where the error occurred, allowing users to debug effectively.
-
Simple Fallback Strategies: Implement strategies, such as skipping certain tokens and continuing parsing to collect multiple errors in a single run.

Optimizing Your C++ Parser
Performance Considerations
In any C++ parser, performance is a priority. Consider the following aspects:
-
Time Complexity: Review the parsing algorithms you implement, as some are inherently more efficient than others.
-
Memory Usage Optimization: Minimize memory footprints, especially when handling large input files or extensive token streams.
Extending Your Parser
Once your initial parser is ready, consider:
- Adding New Features: Implement support for additional grammars or expressions.
- Handling Larger Languages: Adapt your parsing strategies for scalability when working with larger programming languages or complex data structures.
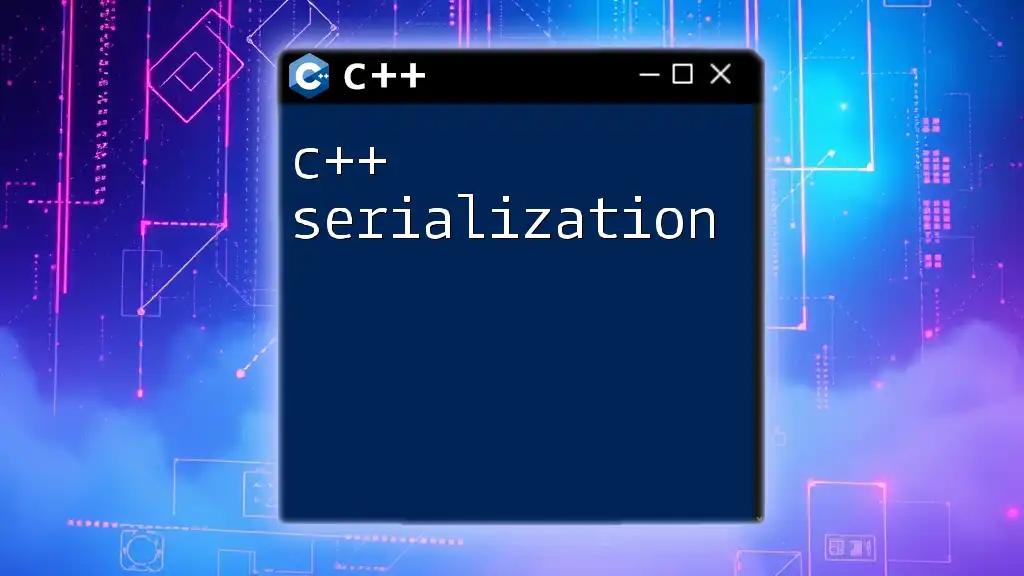
Testing Your Parser
Developing a parser without proper testing can lead to unexpected behavior and errors in real-world applications. Implement unit tests to ensure each component behaves as expected.
Writing Test Cases for Parsers
Use a testing framework to create test cases that validate the functionality of both the lexer and parser. For example:
TEST(ParserTests, SimpleExpression) {
std::vector<Token> tokens = lex("a = b + c;");
ASSERT_NO_THROW(parse(tokens));
}
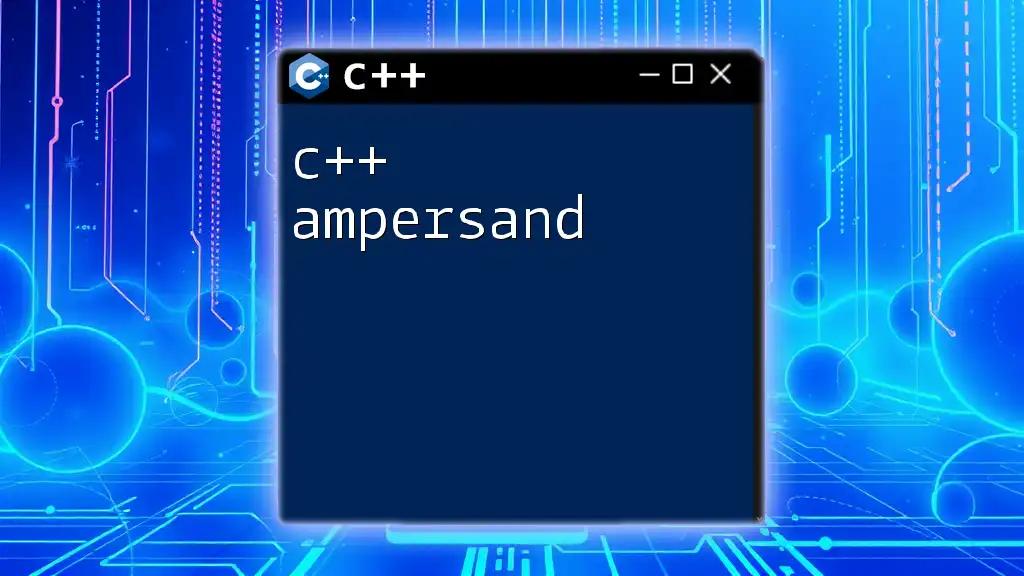
Conclusion
In conclusion, mastering the concept of a C++ parser is fundamental for anyone looking to delve deeper into programming languages or data manipulations. Understanding the intricacies of parsing will empower you to develop robust applications. As you continue your learning journey, explore additional resources and stay connected with programming communities.