In C++, the `pause` functionality can be achieved by using the `system("pause")` command, which temporarily halts program execution until the user presses a key.
Here’s an example:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Press any key to continue..." << std::endl;
system("pause");
return 0;
}
What is C++ Pause?
C++ pause refers to methods used within C++ programs to temporarily halt execution until a specific condition is met, typically until the user provides input. Understanding how to properly implement a pause can enhance your program's interactivity and debugging capabilities.

Why Pause a Program?
Pausing a program serves several important functions. It can provide the developer with an opportunity to examine the state of the program at specific moments, making it an essential feature during debugging. Additionally, pauses can create a more user-friendly experience by allowing users to read outputs or complete a specific action before the program proceeds.

Common Ways to Implement Pause in C++
Using `system("pause")`
One of the simplest methods to pause a C++ program is by using the command `system("pause")`. This command works on Windows systems and prompts the user to press any key to continue.
#include <iostream>
#include <cstdlib> // For system()
int main() {
std::cout << "Press Enter to continue...";
system("pause");
return 0;
}
Pros and Cons
Pros:
- Simplicity: This method is straightforward and easy to implement in any application.
Cons:
- Cross-platform Issues: The `system("pause")` command is specific to Windows, meaning your code may not function as intended on Unix/Linux systems. This reduces its portability, which is a significant drawback when developing more complex applications that may need to run on multiple platforms.
Using `std::cin` to Pause
Another method involves using `std::cin` to wait for user input. This approach is more versatile and compatible across different operating systems.
#include <iostream>
int main() {
std::cout << "Press Enter to continue...";
std::cin.get(); // Waits for user input
return 0;
}
Advantages of Using `std::cin`
Using `std::cin` to implement a pause is highly beneficial for several reasons:
- Cross-platform Compatibility: Unlike `system("pause")`, this method works seamlessly on any operating system supporting C++.
- Standard Practice: It adheres to established coding conventions, promoting better programming habits.
Creating a Custom Pause Function
Creating your own reusable pause function is a fantastic way to encapsulate and standardize the process throughout your code.
#include <iostream>
void pause() {
std::cout << "Press Enter to continue...";
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Clear input buffer
std::cin.get(); // Waits for user input
}
int main() {
std::cout << "Doing some work..." << std::endl;
pause();
return 0;
}
Benefits of Custom Functions
By implementing a custom pause function, you gain several advantages:
- Encapsulation: The logic for pausing is neatly contained within a function, reducing redundancy.
- Improved Readability: Using a function named `pause()` improves the clarity of your code, allowing readers to quickly understand the context of where and why the pause occurs.

Special Considerations
Handling Input Errors
When using `std::cin`, it's crucial to address potential input errors. Users may inadvertently input incorrect data, leading to unexpected behavior. To safeguard against this, you can add checks to clean the input stream before calling `std::cin.get()`. The method `std::cin.ignore()` is effective in clearing the buffer of any unwanted input, which helps maintain the integrity of your pauses.
Performance Considerations
While pauses are useful, they can also introduce performance bottlenecks if not managed carefully. Unnecessary pauses can disrupt the flow of your application, resulting in sluggish behavior. Understanding when and where to effectively insert pauses can significantly improve user experience while maintaining performance.
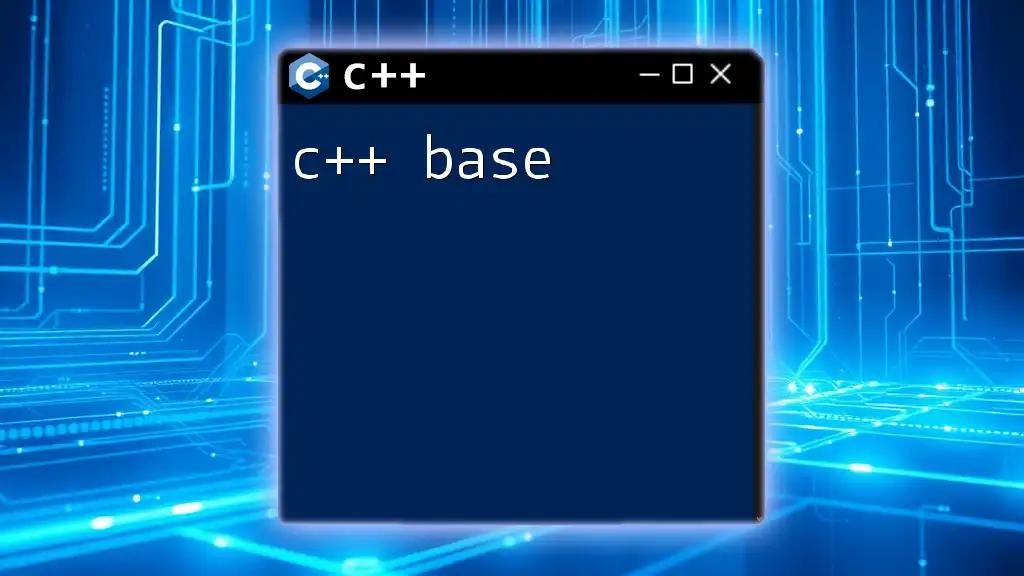
Best Practices
When to Use Pause Commands
Pauses should be deployed judiciously. They are particularly beneficial in scenarios requiring user interaction or during a development phase for debugging. Understand that overuse of pauses can lead to frustration, especially in production environments where efficiency is paramount.
Debugging with Pauses
Strategic pauses can be a developer's best friend. Placing pause commands at critical points in your code allows for examination of the program state—variable values, control flow, and system behaviors—making it an excellent practice for effective debugging.
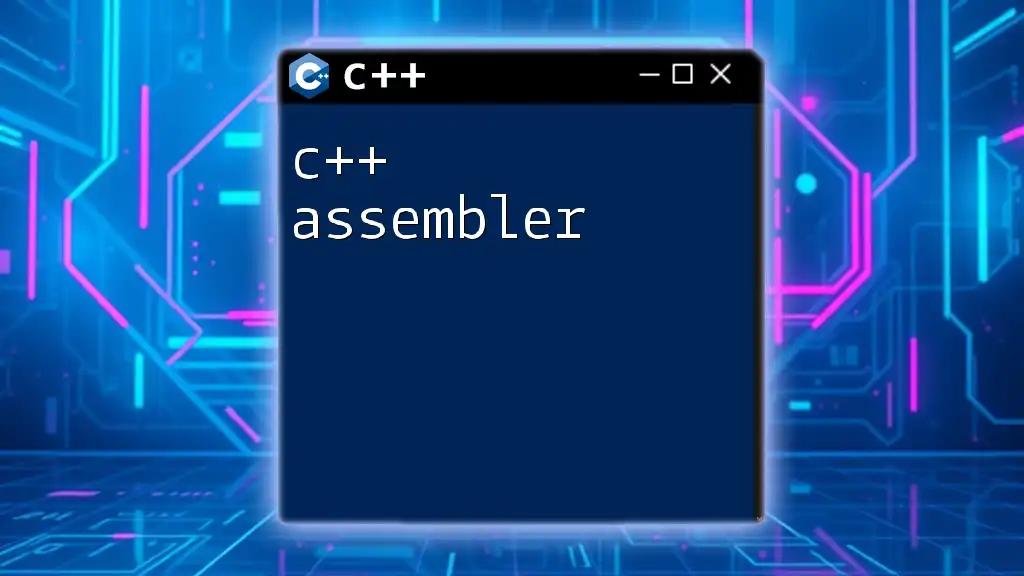
Recap of C++ Pause Methods
To summarize, there are multiple techniques to implement pausing in C++ programs, ranging from `system("pause")` to using `std::cin` and custom functions. Each method has its pros and cons, and understanding these can greatly enhance your programming efficiency and effectiveness.

Encouragement to Experiment
Don’t hesitate to experiment with pause commands in your own C++ projects. Each method has its unique benefits, and finding the best fit will improve both your coding skills and the quality of your applications. Developing a strong command over these aspects will serve you well in your programming journey.
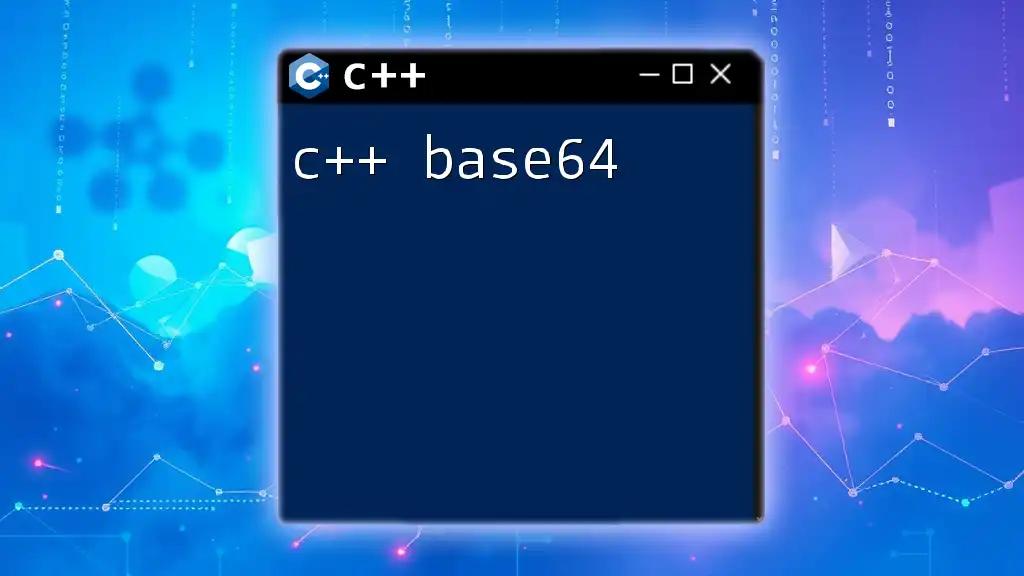
Additional Resources
For deeper insights into C++ programming, consult relevant documentation, tutorials, and literature that focus specifically on system commands, input handling, and effective debugging.
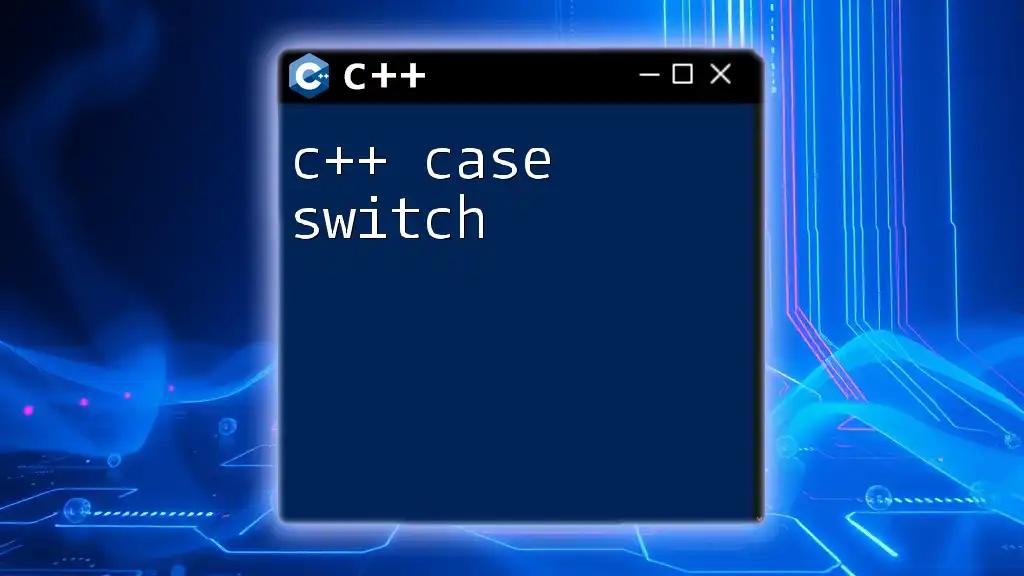
Common Questions Around C++ Pause
Finally, let’s address some common questions that arise concerning the `c++ pause` topic:
-
How to pause a C++ program on Mac/Linux?
- Utilize `std::cin` as shown, as it works universally.
-
Is using `system("pause")` safe in production code?
- Generally, it's best to avoid it in production due to portability concerns. Instead, opt for `std::cin` or a custom pause function.