In C++, parsing a CSV (Comma-Separated Values) file can be done by reading the file line by line and splitting each line by commas to extract individual values.
Here’s a simple example code snippet that demonstrates how to parse a CSV file:
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
void parseCSV(const std::string& fileName) {
std::ifstream file(fileName);
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string value;
std::vector<std::string> row;
while (std::getline(ss, value, ',')) {
row.push_back(value);
}
// Process the row (For demonstration, we print it)
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
}
int main() {
parseCSV("data.csv");
return 0;
}
Understanding CSV Files
What is a CSV File?
A CSV file, or Comma-Separated Values file, is a simple text file that uses a specific structure to organize tabular data. Each line in the file represents a new row of data, while values within a row are separated by commas. This format is widely used for data interchange because it is both human-readable and machine-readable.
Common use cases for CSV files include data import and export in applications like spreadsheets, databases, and statistical analysis tools.
Structure of a CSV File
The basic structure of a CSV file consists of multiple lines, where each line corresponds to a row of data. A header row commonly exists at the beginning, specifying the names of each column. Each subsequent line represents a data record corresponding to the columns defined in the header.
A typical CSV might look like this:
Name,Age,Country
Alice,30,USA
Bob,25,UK
Charlie,35,Canada
In this example:
- The first row is the header that labels each column.
- Rows following the header are data records.
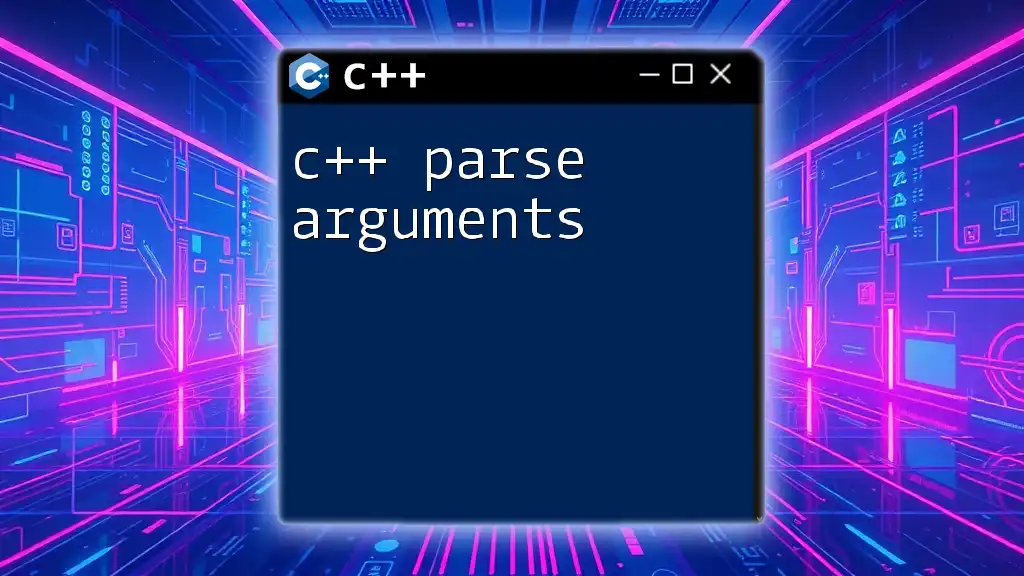
Why Use C++ for CSV Parsing?
Advantages of C++ in Handling CSV Files
Using C++ to parse CSV files offers several advantages:
- Performance Benefits: C++ is a compiled language, which typically leads to faster execution speeds compared to interpreted languages. This is essential for parsing large datasets.
- Flexibility and Control: C++ provides a wide array of tools to manipulate data structures, giving you customized control over how data is handled during the parsing process.
- Compatibility: C++ can easily interact with other programming languages and systems, making it a versatile choice for projects that require data interchange.
When to Choose C++ Over Other Languages
C++ should be considered for CSV parsing when performance is a key factor, especially in applications dealing with big data. While languages like Python and Java offer excellent CSV libraries, they might not match the efficiency of well-optimized C++ code in handling large files or complex data processing tasks.
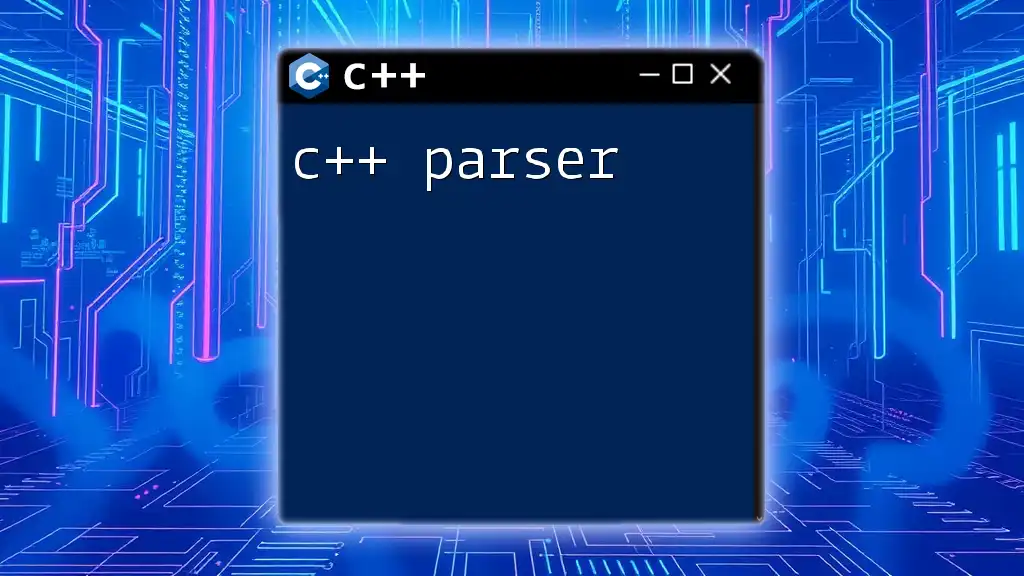
Setting Up Your C++ Environment
Required Tools and Libraries
To get started with C++ for CSV parsing, you will need:
- A C++ compiler (e.g., GCC, Clang, or MSVC).
- An Integrated Development Environment (IDE), with options like Visual Studio, Code::Blocks, or Eclipse.
Additionally, you might consider using standard libraries such as `<fstream>` for file operations and `<sstream>` for string manipulation, both of which are included in the C++ Standard Library.
Installing a C++ Development Environment
Installing a C++ development environment is straightforward. Follow these general steps:
- Choose a Compiler and IDE: Select a compiler matching your operating system, and download an IDE that suits your needs.
- Install the Software: Follow installation instructions for the chosen IDE or compiler specific to your platform (Windows, macOS, or Linux).
- Compile and Run a Sample C++ Program: Test your installation by compiling and running a simple C++ program. This ensures everything is set up correctly and ready for CSV parsing tasks.
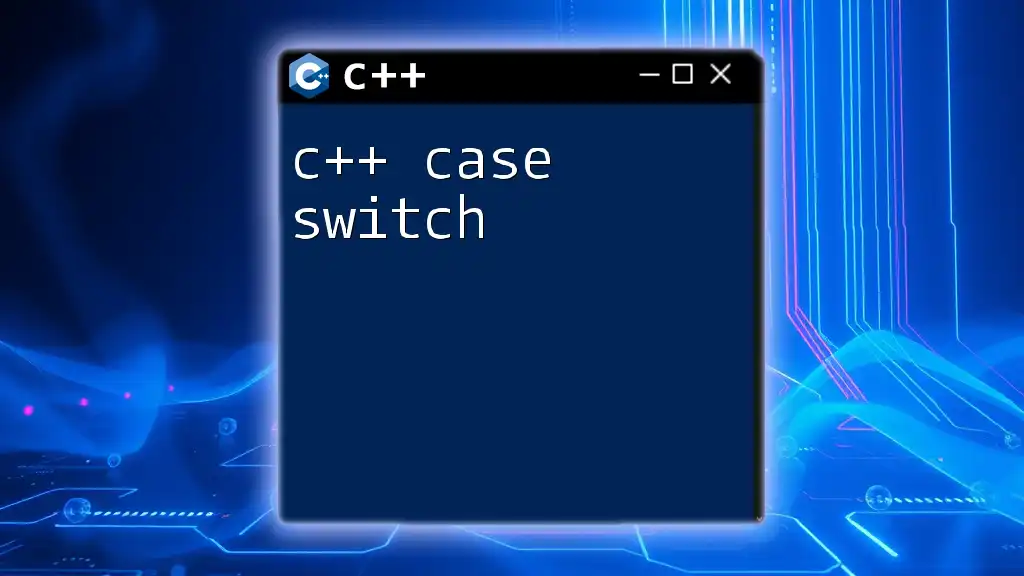
Writing a Basic CSV Parser in C++
Reading a CSV File
The first step in parsing a CSV file is to read its content. The `<fstream>` library provides support for file input and output in C++. You can read your CSV file line by line using the `ifstream` class.
Here's a simple example of how to read a CSV file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("sample.csv");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl; // Output each line read
}
file.close();
return 0;
}
Parsing CSV Lines
After reading the content of the CSV file, the next step is to split each line into individual values. For this, we can use the `<sstream>` library, which allows us to process string streams efficiently.
Here’s how to implement a function that splits a line into values:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> parseLine(const std::string &line) {
std::stringstream ss(line);
std::string value;
std::vector<std::string> parsedValues;
while (std::getline(ss, value, ',')) {
parsedValues.push_back(value);
}
return parsedValues;
}
Putting It All Together: Full CSV Parser
Now that we have both the file reading and line parsing mechanisms in place, we can combine them into a complete CSV parser. Here’s the code that brings everything together:
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
std::vector<std::string> parseLine(const std::string &line) {
std::stringstream ss(line);
std::string value;
std::vector<std::string> parsedValues;
while (std::getline(ss, value, ',')) {
parsedValues.push_back(value);
}
return parsedValues;
}
int main() {
std::ifstream file("sample.csv");
std::string line;
while (std::getline(file, line)) {
std::vector<std::string> values = parseLine(line);
for (const auto& val : values) {
std::cout << val << ' ';
}
std::cout << std::endl;
}
file.close();
return 0;
}
This code snippet reads a CSV file, parses each line into separate values, and outputs them.
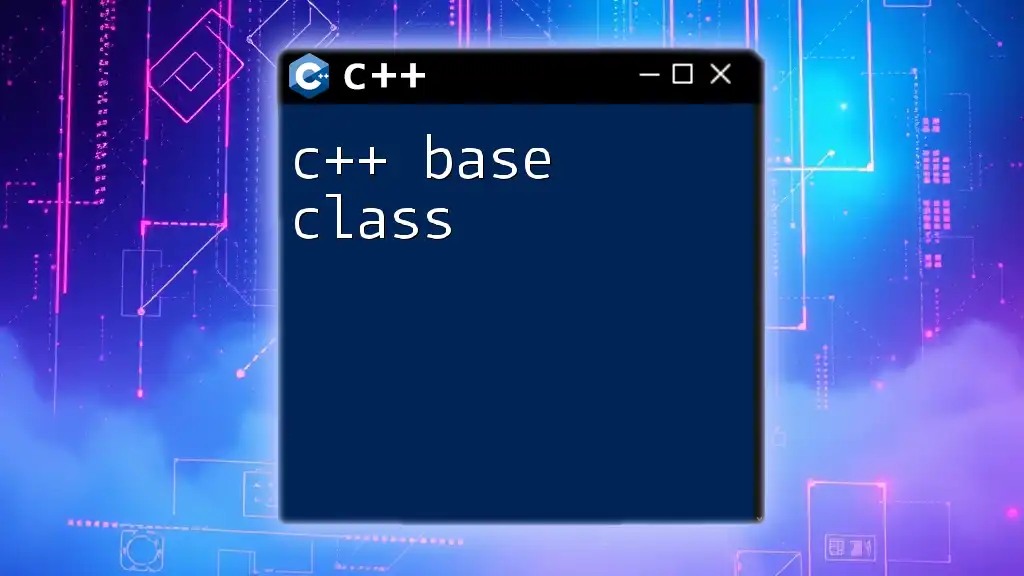
Advanced CSV Parsing Techniques
Handling Complex CSV Formats
While simple CSV files are easy to parse, real-world scenarios often present challenges—like handling quoted fields or commas embedded within quotes. To address these issues effectively, you may consider using regular expressions, which can help identify and capture complex patterns within your CSV data.
Tips for Error Handling
Parsing CSV is not without its pitfalls. Anticipate common errors such as malformed lines, unexpected symbols, or inconsistent column counts. Implement robust error handling to check for these issues during parsing:
- Verify that each line has the correct number of columns compared to the header.
- Handle exceptions gracefully by using try-catch blocks to manage errors in file I/O operations.
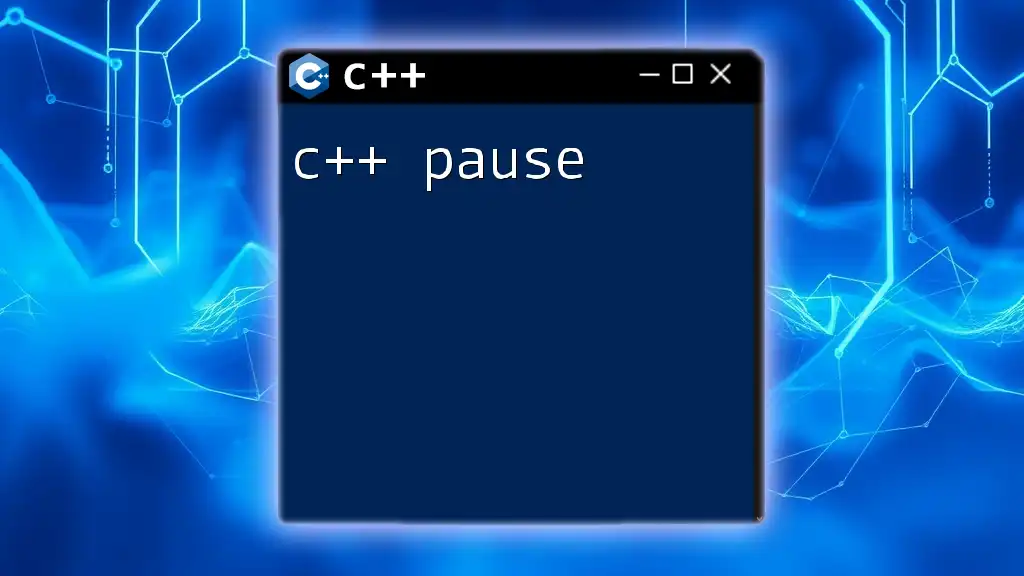
Best Practices for CSV Parsing in C++
Writing Clean and Maintainable Code
Strive to maintain clarity in your code. Using meaningful variable names, and concise comments will significantly enhance the readability and maintainability of your parser. Always document your methods so readers (or future you!) can understand their purpose quickly.
Performance Considerations
As your dataset grows, performance may become a concern. Optimize your parser by minimizing unnecessary object creations and reallocations. For example, preallocate memory for vectors if the number of elements is known ahead of time. Additionally, you could consider benchmarking different parsing strategies to see which performs best for your use case.
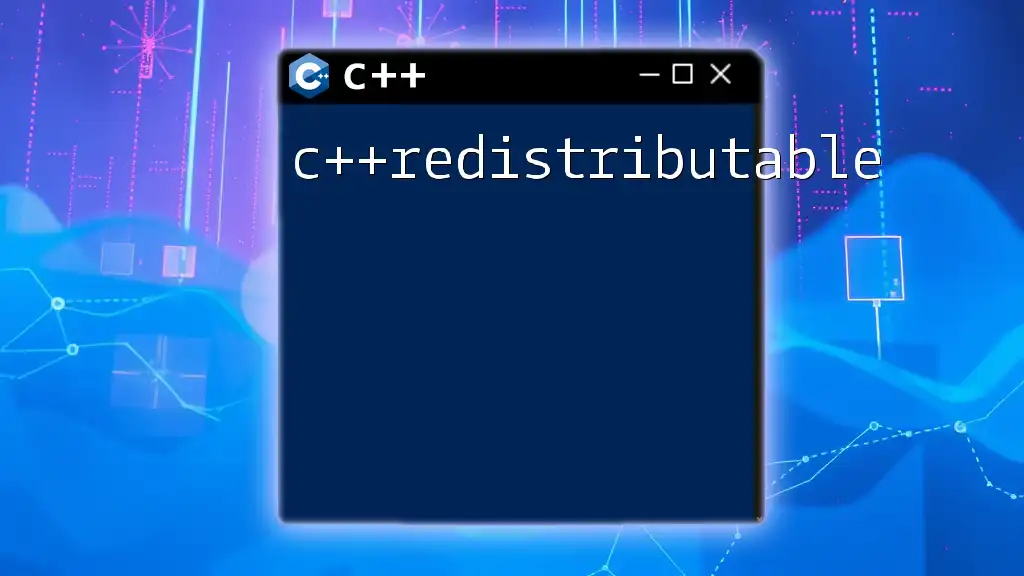
Conclusion
In this comprehensive guide, you learned how to C++ parse CSV files effectively, from reading files and parsing lines, to dealing with complex formats. C++'s performance benefits and control over data structures make it an excellent choice for CSV parsing, particularly for applications that require efficiency and reliability.
As you embark on your journey with CSV parsing in C++, remember to experiment, optimize, and keep your code clean. The skills you've obtained here can pave the way for working with broader dataset management tasks in the future.
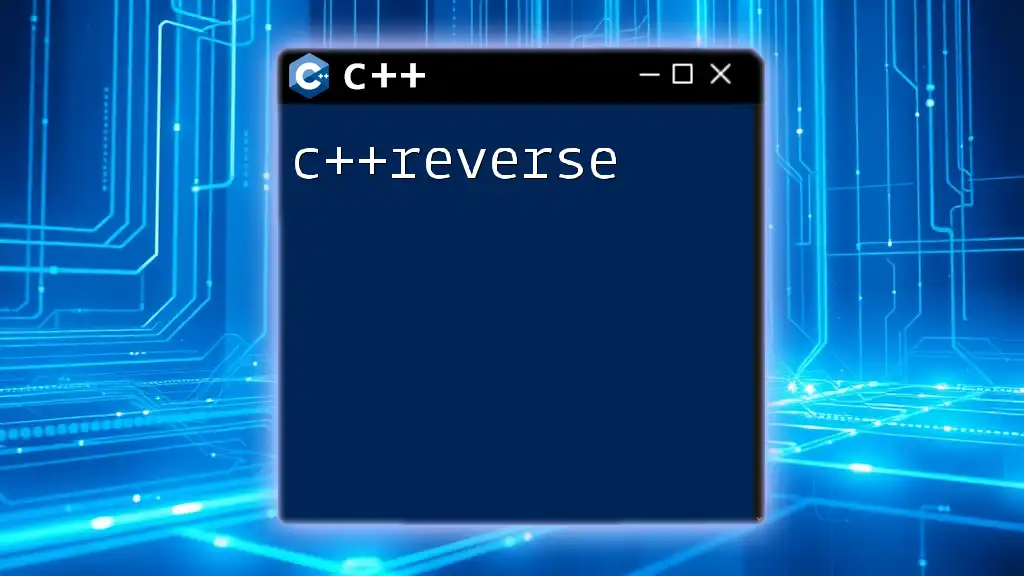
Call to Action
We encourage you to share your experiences and challenges in CSV parsing. What techniques did you find most effective? Did you encounter unexpected issues? Join the conversation and let us know your thoughts and questions!
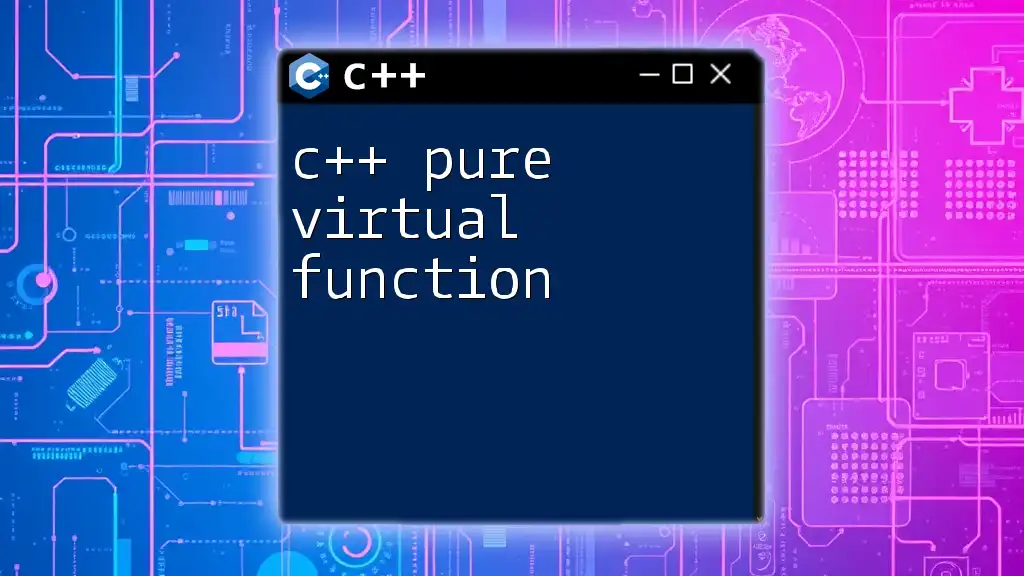
Additional Resources
To further enhance your understanding, consider reading the documentation on the libraries discussed, and explore more advanced C++ techniques in data manipulation. Happy coding!