A C++ pure virtual function is a virtual function that has no implementation in the base class and must be overridden in any derived class, enabling the creation of abstract classes.
Here's a code snippet demonstrating a pure virtual function:
#include <iostream>
class Shape {
public:
// Pure virtual function
virtual void draw() = 0;
};
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing a circle" << std::endl;
}
};
int main() {
Circle circle;
circle.draw(); // Outputs: Drawing a circle
return 0;
}
Understanding C++ Pure Virtual Functions
What are Pure Virtual Methods?
A pure virtual function is a function declared in a base class that has no implementation and is meant to be overridden in derived classes. It identifies the class as an abstract class, preventing instances of this class from being created directly. The syntax for declaring a pure virtual function involves appending `= 0` to its declaration, signifying that the function must be implemented in any derived class.
Example Code Snippet:
class Base {
public:
virtual void display() = 0; // pure virtual function
};
In the example above, `display()` is a pure virtual function. Any class derived from `Base` must provide an implementation for `display()`, or it too will be considered abstract.
How Pure Virtual Functions Differ from Regular Functions
A regular virtual function in C++ has an implementation in the base class, which can be optionally overridden by derived classes. In contrast, a pure virtual function stands as a placeholder without any definition in the base class, forcing derived classes to implement it. This significant difference is critical for creating a polymorphic interface, where the specific implementation can vary based on the object type that is being referenced.
The Role of Pure Virtual Functions in Inheritance
Pure virtual functions are integral to establishing a consistent interface across various derived classes. When a base class contains pure virtual functions, any class inheriting from it must provide a concrete implementation for each pure virtual function. This enforces a contract between the base class and derived classes, enhancing the integrity and adaptability of codebases, especially as systems grow in complexity.
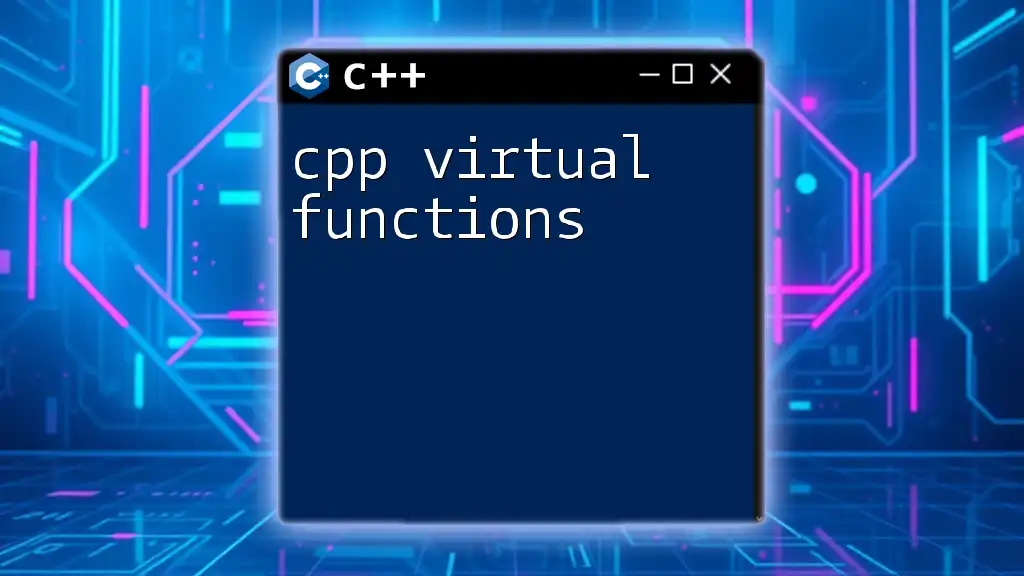
Creating Pure Virtual Functions in C++
Syntax and Structure
To declare a pure virtual function, you define it in the base class using the `virtual` keyword followed by the function name and parameters, finished with `= 0`.
Example Code Snippet:
class Shape {
public:
virtual void draw() = 0; // pure virtual function
};
This `Shape` class serves as an abstract base class for various shapes.
Implementing Pure Virtual Functions in Derived Classes
When a derived class inherits from a base class containing pure virtual functions, it must provide implementations for these functions; otherwise, the derived class will also be abstract.
Example Code Snippet:
class Circle : public Shape {
public:
void draw() override { // overriding the pure virtual function
std::cout << "Drawing a Circle." << std::endl;
}
};
class Square : public Shape {
public:
void draw() override {
std::cout << "Drawing a Square." << std::endl;
}
};
In this example, both `Circle` and `Square` provide specific implementations of the `draw()` function.
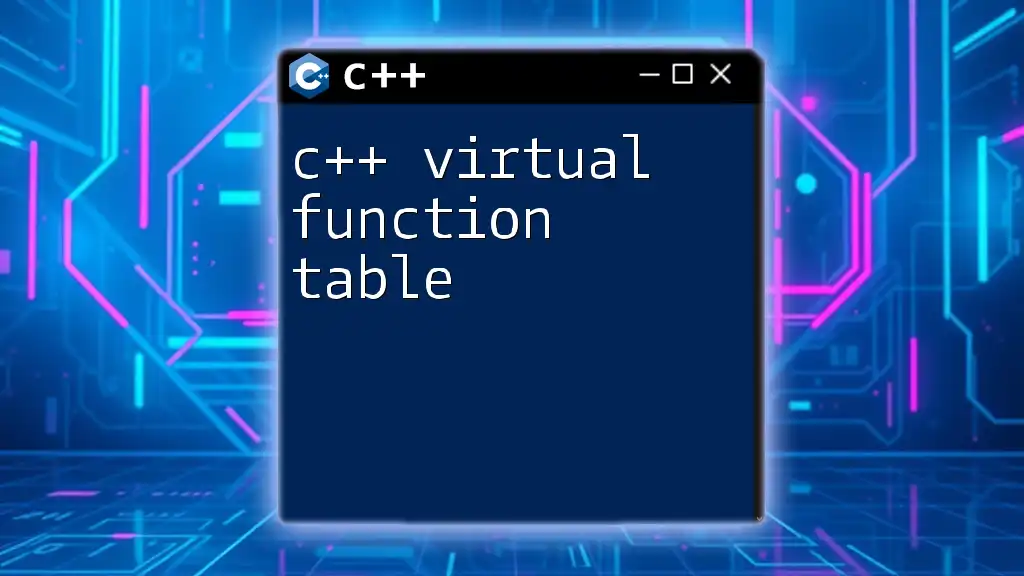
Use Cases and Advantages of Pure Virtual Functions
Use Cases in Real-world Applications
The utility of pure virtual functions shines brightly in scenarios requiring a flexible and extensible design. Consider a graphics application where multiple shapes need to be drawn. By utilizing a pure virtual base class, you can declare an interface that various shape classes can adhere to, allowing polymorphic behavior.
Advantages of Using Pure Virtual Functions
Using pure virtual functions offers several advantages:
- Promotes Clean Code Architecture: By defining abstract interfaces, it establishes clear contracts for derived classes, contributing to code that is easier to read and maintain.
- Encourages Implementation Diversity: Different derived classes can offer their unique implementations, allowing for greater flexibility and adaptability in how functions behave based on specific class types.
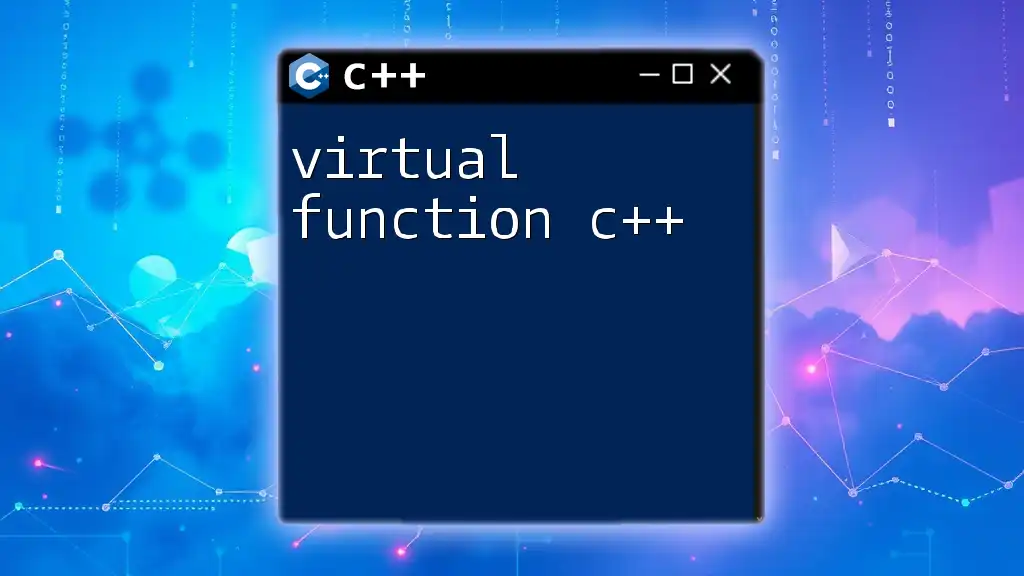
Potential Pitfalls When Using Pure Virtual Functions
Common Mistakes to Avoid
Several common mistakes can lead to confusion or errors in C++ when dealing with pure virtual functions:
- Forgetting to implement pure virtual functions in derived classes, resulting in compilation errors.
- Attempting to instantiate an abstract class directly, which leads to an error since abstract classes cannot be instantiated.
Error Handling and Debugging Tips
When encountering an issue related to pure virtual functions, the compiler error messages usually provide guidance about missing implementations. Ensure that all derived classes implementing the abstract interface fulfill their obligations by adequately defining all pure virtual functions.
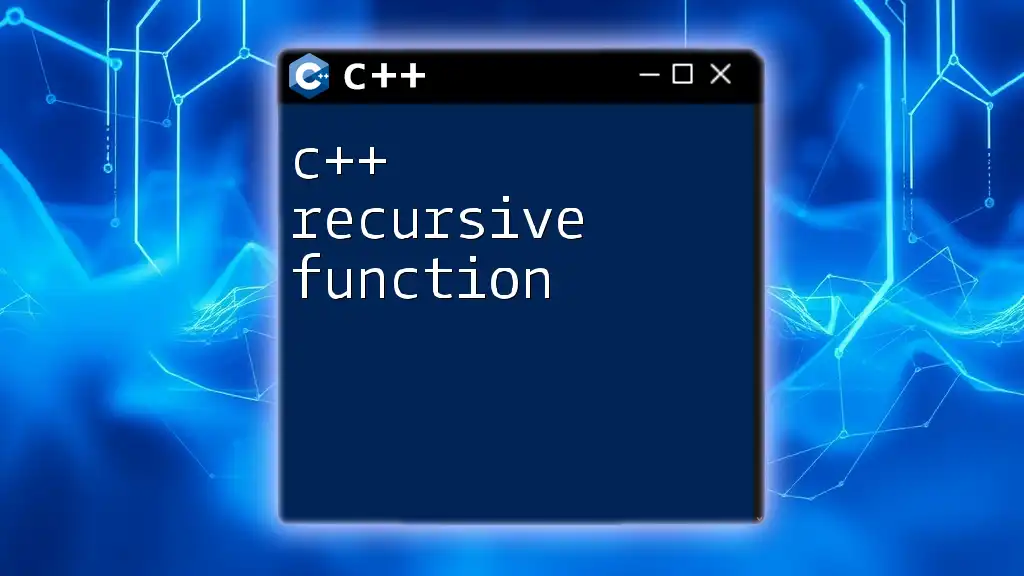
Best Practices for Implementing Pure Virtual Functions
To effectively use pure virtual methods in C++, consider the following best practices:
Guidelines for Using Pure Virtual Methods
- Use pure virtual functions when you want to define an interface intended for multiple derived classes and ensure they all provide their distinct implementations.
- Understand when to use pure virtual functions versus regular virtual functions. Use pure virtual when you want to enforce the implementation of the method in every derived class.
Documentation and Code Comments
Documenting your pure virtual functions is essential for maintainability. Clearly state what each function is intended to do, and consider providing examples if the functions behave differently in various derived classes. This documentation will aid other developers who may use or modify your code.
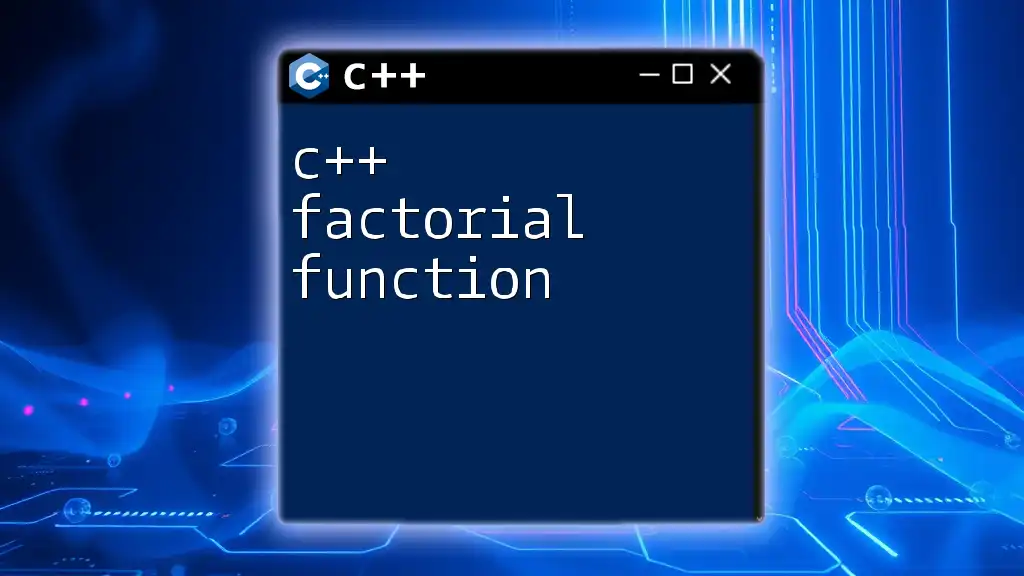
Conclusion
In summary, the C++ pure virtual function provides a powerful mechanism for achieving polymorphism in object-oriented programming. By requiring derived classes to implement specific behaviors, you can craft systems that are both flexible and robust.
Encourage further exploration by practicing with examples and engaging in projects involving pure virtual functions. C++ offers a wealth of resources to deepen your understanding, so jump in and start implementing pure virtual functions to unleash the full potential of your code!
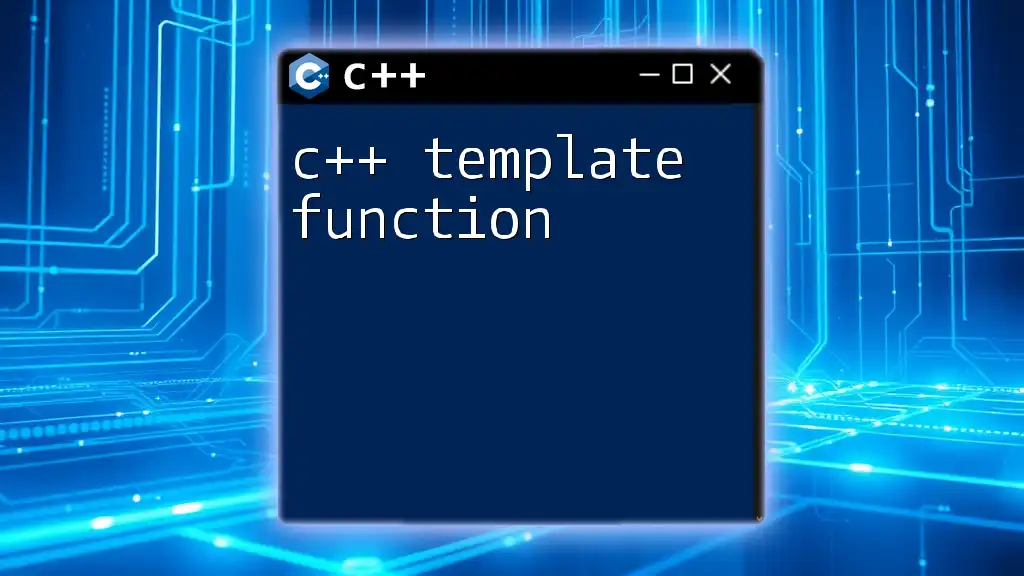
Frequently Asked Questions (FAQs)
What is the difference between a pure virtual function and a virtual function?
The primary distinction lies in their presence in the class. A virtual function has an implementation in its class, while a pure virtual function has no implementation and must be overridden in derived classes. Thus, a derived class can choose not to override virtual functions, but it must do so for pure virtual functions.
Can a class have both virtual and pure virtual methods?
Yes, a class can contain both virtual and pure virtual methods. This allows for a mixture of defined implementations and enforced abstract methods within the same class, providing flexibility in design.
How do pure virtual functions affect object-oriented design in C++?
They aid in establishing a clean interface blueprint across different components of your application, promoting a strong sense of encapsulation and polymorphic behavior. This helps in creating systems that are easier to maintain, understand, and extend.
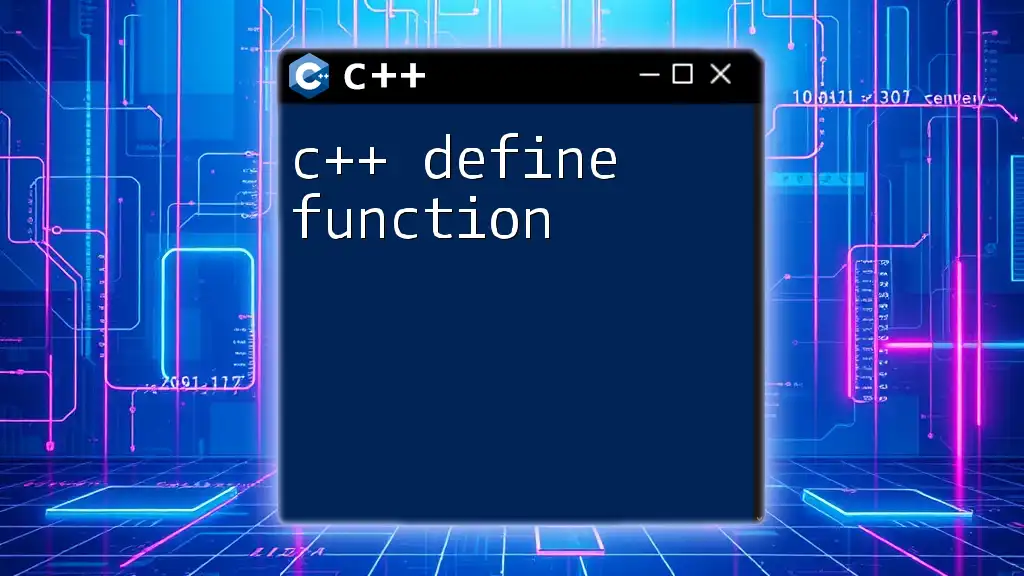
Additional Resources
Familiarize yourself with related materials, such as recommended books and online courses focused on object-oriented programming with C++. Exploring C++ documentation and engaging with community forums can also significantly enhance your understanding of pure virtual functions and improve your overall coding proficiency.