The C++ mean function calculates the average value of a set of numbers by summing them up and dividing by their count.
#include <iostream>
#include <vector>
double mean(const std::vector<double>& numbers) {
double sum = 0.0;
for (double num : numbers) {
sum += num;
}
return numbers.empty() ? 0 : sum / numbers.size();
}
int main() {
std::vector<double> data = {10, 20, 30, 40, 50};
std::cout << "Mean: " << mean(data) << std::endl;
return 0;
}
Understanding the Basics
What is a Mean?
The mean, often referred to as the average, is a fundamental statistical concept that provides a measure of central tendency in a data set. It is calculated by summing all the values and dividing by the count of values. While there are various types of means—arithmetic, geometric, and harmonic—in this article, we will focus specifically on the arithmetic mean, which is the most commonly used.
Why Use a Mean Function in C++?
Incorporating a mean function in your C++ programs is essential for various reasons:
- Streamlined Calculations: By encapsulating the calculation of the mean into a function, you can simplify your code, making it more readable and maintainable.
- Reusability: A well-defined function allows you to reuse the mean calculation across different parts of your program, avoiding code duplication.
- Error Handling: Implementing a dedicated function allows for better control over input validation and error management, ensuring that your program behaves predictably and handles edge cases gracefully.
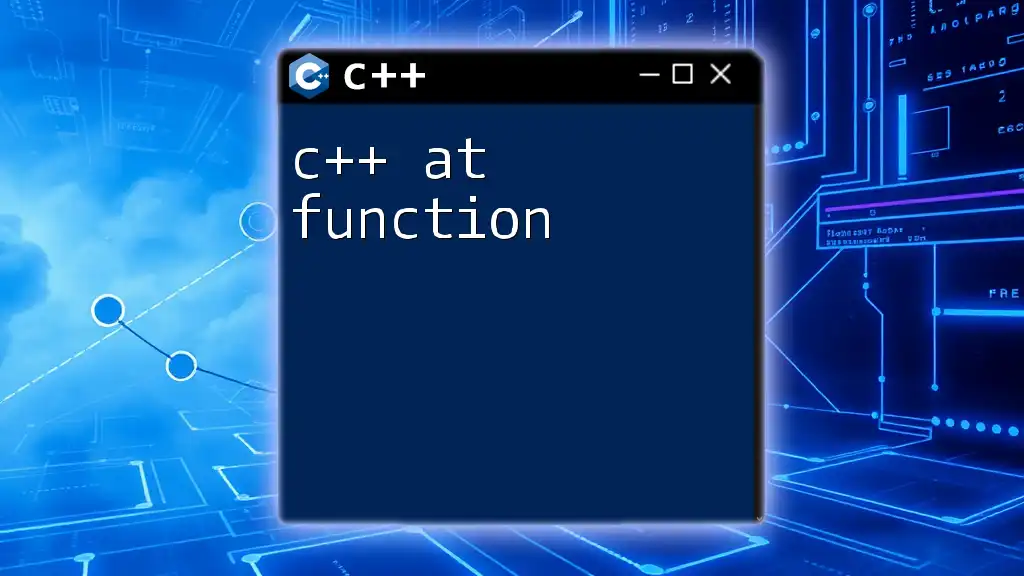
Implementing the Mean Function in C++
Basic Syntax of a Function in C++
Understanding the syntax of a function is crucial before diving into creating a mean function. The basic structure consists of the return type, function name, and parameters. For example, a typical function signature looks like this:
return_type function_name(parameter_list) {
// function body
}
Creating a Simple Mean Function
To begin, let's implement a straightforward mean function that takes a vector of double values and returns the mean. Here’s how you can do it:
double mean(const std::vector<double>& numbers) {
double sum = 0.0;
for (const auto& num : numbers) {
sum += num;
}
return sum / numbers.size();
}
In this code snippet:
- We define our function `mean`, which accepts a constant reference to a vector of doubles.
- We initialize a variable sum to 0.0, which will accumulate the values from the vector.
- A for loop iterates through each number in the vector, adding it to the sum.
- Finally, the mean is calculated by dividing the sum by the vector's size, which gives us the average of the numbers.
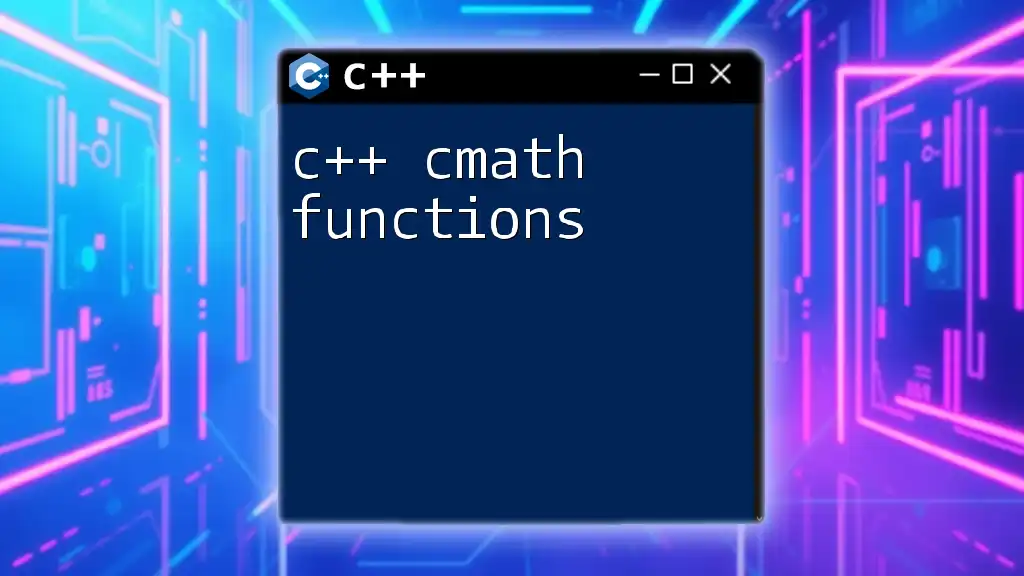
Enhancing the Mean Function
Handling Edge Cases
When creating a mean function, you must consider edge cases—situations that might cause your function to behave unexpectedly. A common edge case is when the vector is empty. In such cases, attempting to calculate the mean would result in a division by zero. Here’s how to handle this:
double mean(const std::vector<double>& numbers) {
if (numbers.empty()) {
throw std::invalid_argument("Vector is empty!");
}
double sum = 0.0;
for (const auto& num : numbers) {
sum += num;
}
return sum / numbers.size();
}
In the modified function:
- Before calculating the mean, we check if the vector is empty and throw an `std::invalid_argument` exception if it is. This ensures that users of the function receive meaningful feedback when they input invalid data, rather than encountering a runtime error.
Using Function Templates to Calculate Mean
To make our mean function more versatile, we can employ function templates, which allow our function to accept different numeric types. This is desirable because C++ has various numeric types, including `int`, `float`, and `double`. Here’s an enhanced version of the mean function using templates:
template <typename T>
double mean(const std::vector<T>& numbers) {
if (numbers.empty()) {
throw std::invalid_argument("Vector is empty!");
}
T sum = 0;
for (const auto& num : numbers) {
sum += num;
}
return static_cast<double>(sum) / numbers.size();
}
In this example:
- The function is declared as a template that can handle any type `T`, allowing it to compute the mean for various numeric types.
- The vector parameter is now a vector of type `T`.
- The sum is also declared as type `T`, ensuring type compatibility and correctness.
- Finally, we cast the sum to `double` when returning the mean to maintain precision in the result.
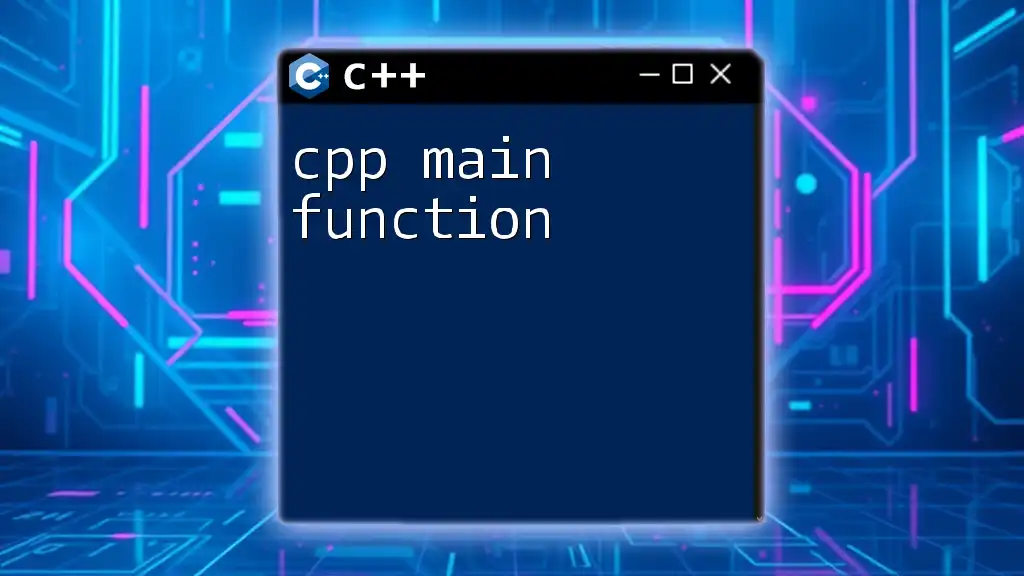
Practical Applications of the Mean Function
Mean Function in Real-Life Scenarios
One common use case for the C++ mean function is in educational contexts, such as calculating the average score of students in a class. Suppose we have a vector representing the scores of students:
std::vector<double> scores = {85.5, 90.0, 78.5, 88.0, 92.5};
double averageScore = mean(scores);
In this example, the mean function will compute the average score from the vector, providing an essential metric for performance evaluation.
Using Mean in Data Analysis
The mean function also plays a critical role in data analysis, whether it’s for statistical reporting or preliminary data characterization. For larger datasets, performance becomes crucial. The efficiently implemented mean function will execute quickly, even with large volumes of data, making it suitable for complex applications such as data mining or machine learning.
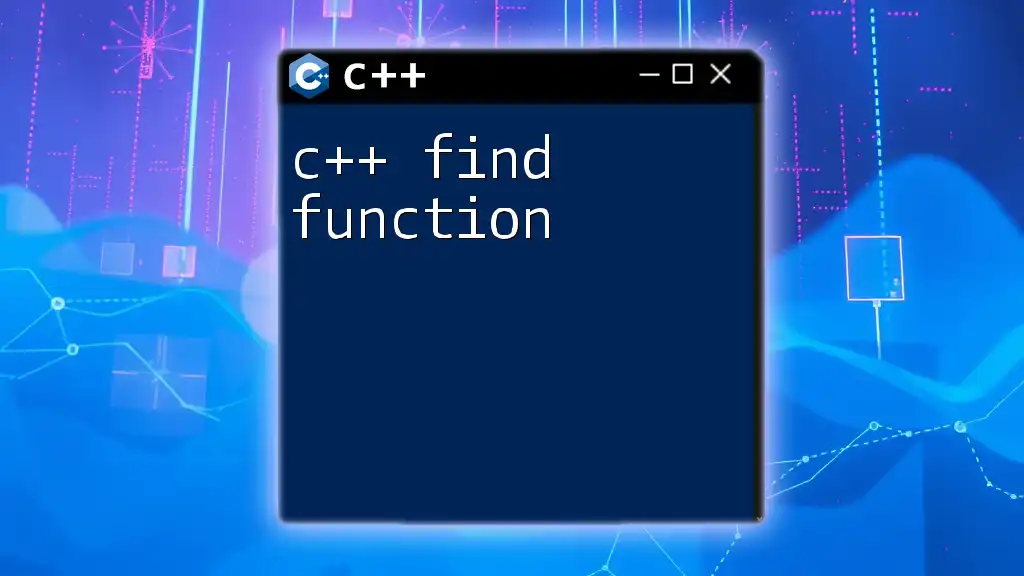
Conclusion
In summary, understanding and implementing the C++ mean function is vital for developers and analysts alike. A well-realized mean function provides a foundation for statistical calculations, enhances code readability, and promotes reusability. By considering error handling and adopting templates, you can create robust solutions tailored to a variety of applications.
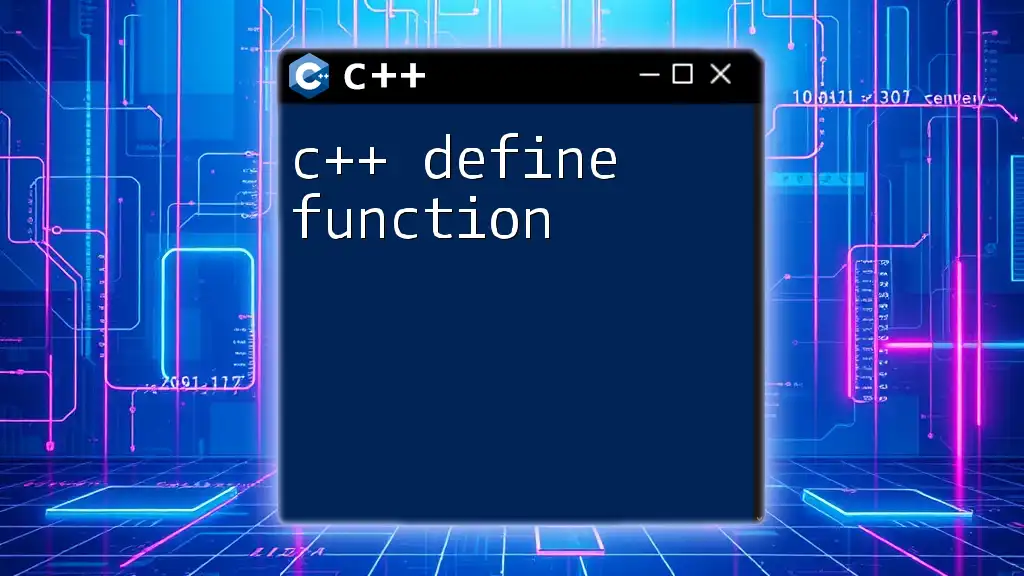
FAQs
Common Questions About the C++ Mean Function
-
What if I pass a non-numeric vector to the mean function?
- The current implementation expects numeric types and attempting to pass incompatible types will result in a compile-time error. Be sure to use a vector of a numeric type to avoid such issues.
-
How to calculate the weighted mean in C++?
- To calculate the weighted mean, you would require a second vector representing the weights, implementing a similar iteration to compute the sum of the products of values and their weights, followed by dividing by the total weight.
-
What are some advanced statistics functions I can implement in C++?
- Beyond the mean, consider implementing functions for median, mode, standard deviation, and more complex statistical analyses to enrich your data processing capabilities.
Encouragement to explore writing your own mean functions, experimenting with diverse data types and edge cases will empower you to enhance your skills and deepen your understanding of C++. With a solid grasp of these concepts, you can tackle more complex statistical calculations in your C++ projects effectively.