An inline function in C++ is a function that is expanded in line when it is invoked, which can reduce the overhead of function calls for small, frequently called functions.
Here’s a simple example of an inline function in C++:
#include <iostream>
inline int square(int x) {
return x * x;
}
int main() {
std::cout << "Square of 5 is: " << square(5) << std::endl;
return 0;
}
What is an Inline Function?
A C++ inline function is a special type of function that requests the compiler to insert the function’s body directly into each location where the function is called. This minimizes the overhead associated with a traditional function call, potentially leading to performance enhancements in execution speed, especially for small, frequently used functions.
Differences Between Inline Functions and Regular Functions
Regular functions incur a performance cost each time they are called due to the overhead of setting up the call and returning from it. In contrast, inline functions aim to eliminate this overhead by replacing the call with the actual code of the function itself. However, it is important to note that the compiler may ignore the inline request, particularly for larger functions or those that cannot be trivially inlined.
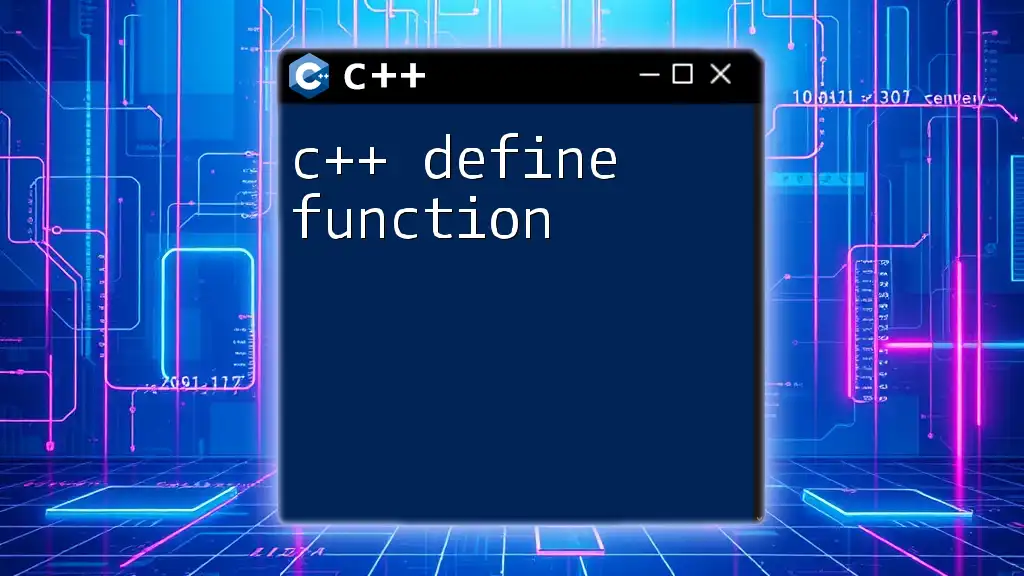
Why Use Inline Functions?
Performance Benefits
The primary advantage of using a C++ inline function is increased performance. When a function is small and called frequently, each call's overhead can accumulate and negatively affect performance. By using inline functions, the execution time can be significantly reduced.
Situations Where Inline Functions Are Appropriate
Inline functions work best for:
- Simple operations like arithmetic or logical comparisons.
- Frequent calls in tight loops.
- Short functions that do not exceed a few lines of code.
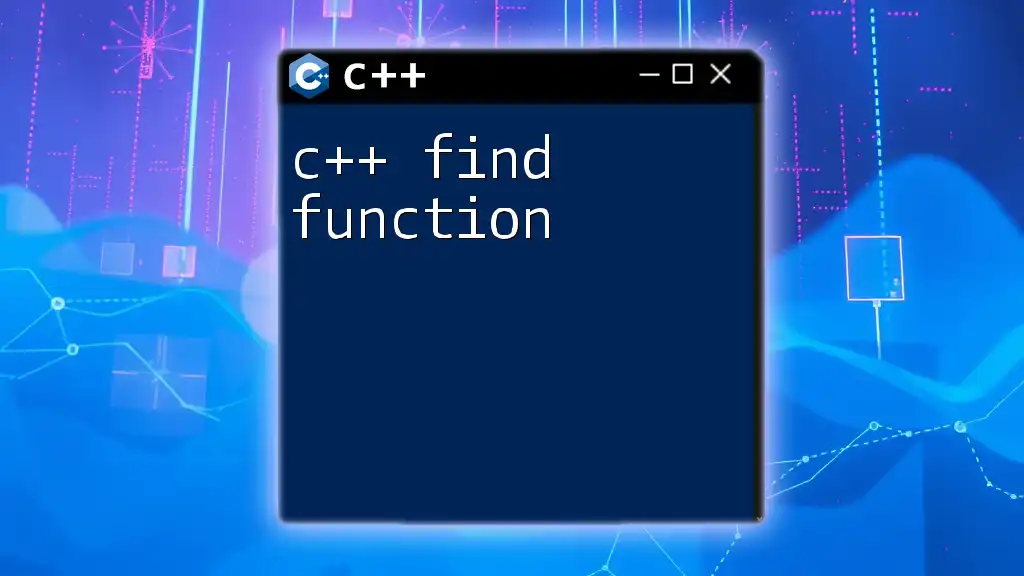
Understanding the Syntax of Inline Functions
How to Define an Inline Function
The syntax for defining an inline function is straightforward. You simply precede the function’s return type with the keyword `inline`. Here’s a basic example:
inline int add(int a, int b) {
return a + b;
}
Where to Declare Inline Functions
Inline functions are typically defined in header files. This allows the definitions to be included wherever needed throughout the project. If defined in a `.cpp` file and not included, the compiler may not have access to the function body at the point of call.
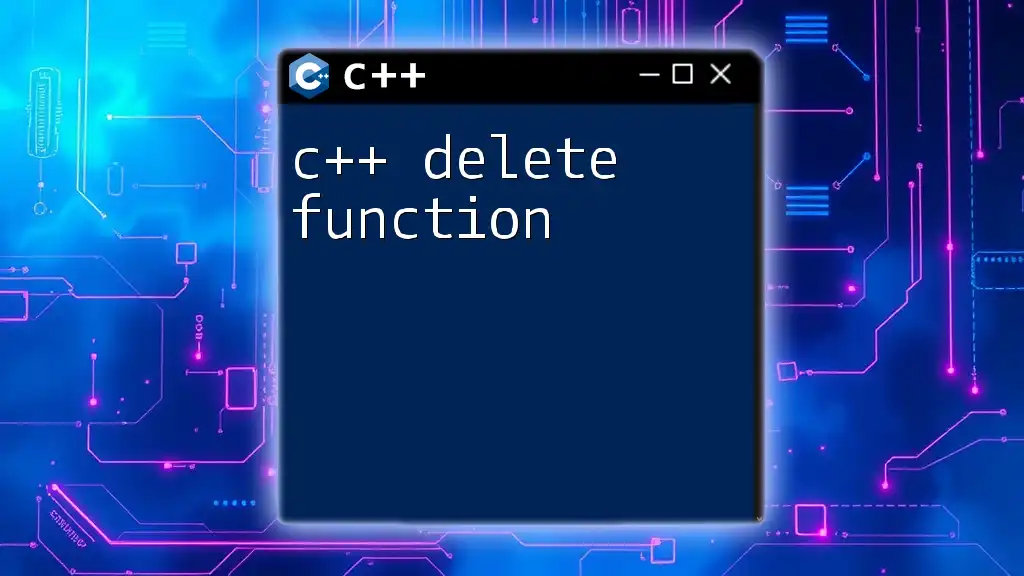
Benefits of Using Inline Functions
Improved Performance
An inline function can significantly reduce function call overhead. When a function is inlined, it can eliminate the need for pushing arguments onto the stack and jumping to the function’s code, leading to faster execution.
Enhanced Code Readability
Inline functions contribute to better code organization. They allow programmers to create descriptive function names for common tasks, which enhances overall code readability without sacrificing performance.
Compiler Optimizations
Modern C++ compilers are well-optimized and often make intelligent decisions regarding inlining. They may decide to inline functions that have not been explicitly marked as inline if they find that doing so would improve performance.
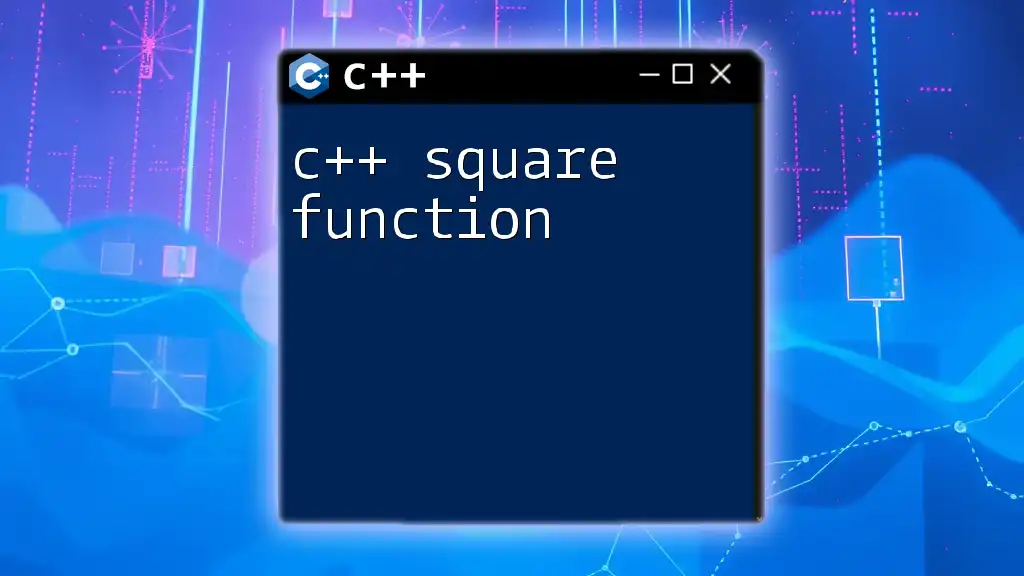
Drawbacks of Inline Functions
Increased Code Size
One significant drawback of inline functions is potential code bloat. Since the body of the inline function is duplicated at each call site, this can lead to larger binaries, particularly if the inline function is called frequently.
Limitations on Use
Inline functions are not suitable in all scenarios. Some limitations include:
- Complex logic: If the function is complex or involves loops, the compiler is likely to ignore the inline request.
- Recursion: Inline functions cannot be recursive, as this would complicate their expanded form.
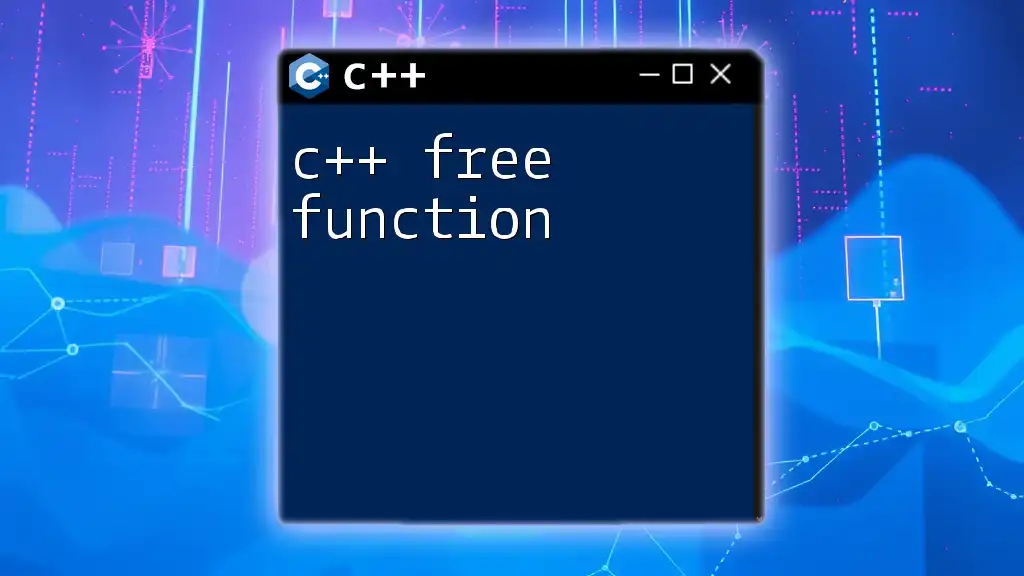
Best Practices for Inline Functions
When to Choose Inline
Consider using inline functions in scenarios where:
- The function consists of only a few simple operations.
- It is called frequently within a small scope.
Maintaining Readability
While optimizing for performance, it is crucial to keep inline functions concise. Avoid intricate algorithms or excessive logic flow within inline functions to ensure they remain legible.
Avoiding Complex Logic
The golden rule is to keep inline functions simple and focused on achieving a single task. Avoid nested calls or multi-step processes to enhance the effectiveness of the inlining.
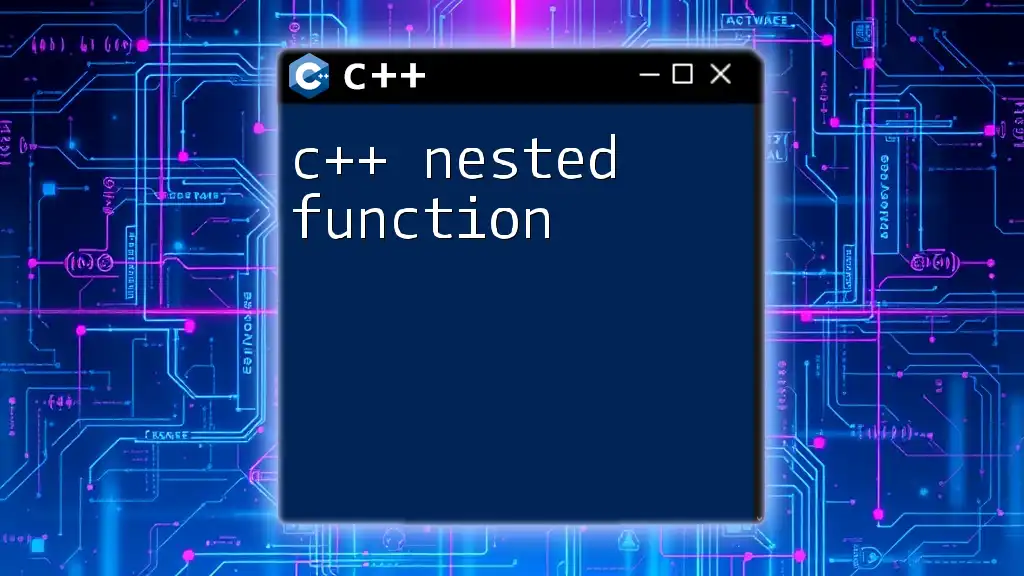
Examples of Inline Functions
Example 1: Simple Calculation
Here’s a simple inline function that computes the square of a number:
inline int square(int x) {
return x * x;
}
This function is straightforward, making it a perfect candidate for inlining.
Example 2: Multiple Parameters
Let’s consider an inline function that returns the maximum of two integers:
inline int max(int a, int b) {
return (a > b) ? a : b;
}
This inline function uses a conditional operator to determine the maximum, maintaining simplicity and efficiency.
Example 3: Template Inline Function
Using templates with inline functions promotes flexibility. Here’s an example:
template <typename T>
inline T getMax(T a, T b) {
return (a > b) ? a : b;
}
In this case, the function can handle various data types, making it versatile and reusable.
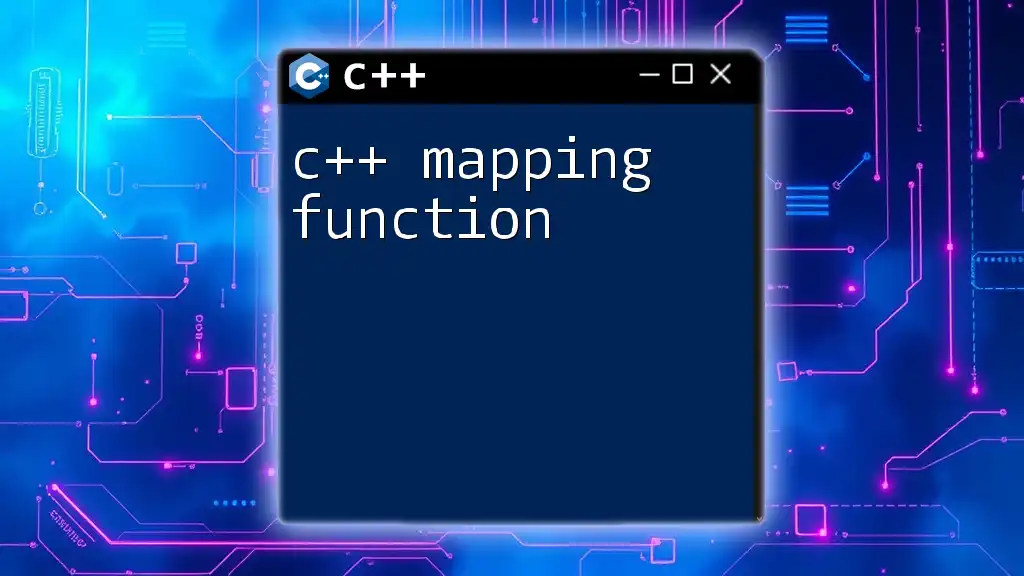
Inline Functions vs Macros
Comparison of Performance
When comparing inline functions to macros, inline functions generally provide better performance due to type safety and the compiler's optimization capabilities. Macros do not respect variable types and can lead to unpredictable behavior if not carefully managed.
Safety and Debugging
Inline functions offer type safety, making them more reliable than macros. This increases code robustness and eases debugging since inline functions are checked during compilation.
Code Maintenance
Another significant advantage of inline functions over macros is improved code maintenance. Changes made to an inline function automatically propagate throughout the program, whereas macros require manual updates in each invocation, increasing the chance of errors.
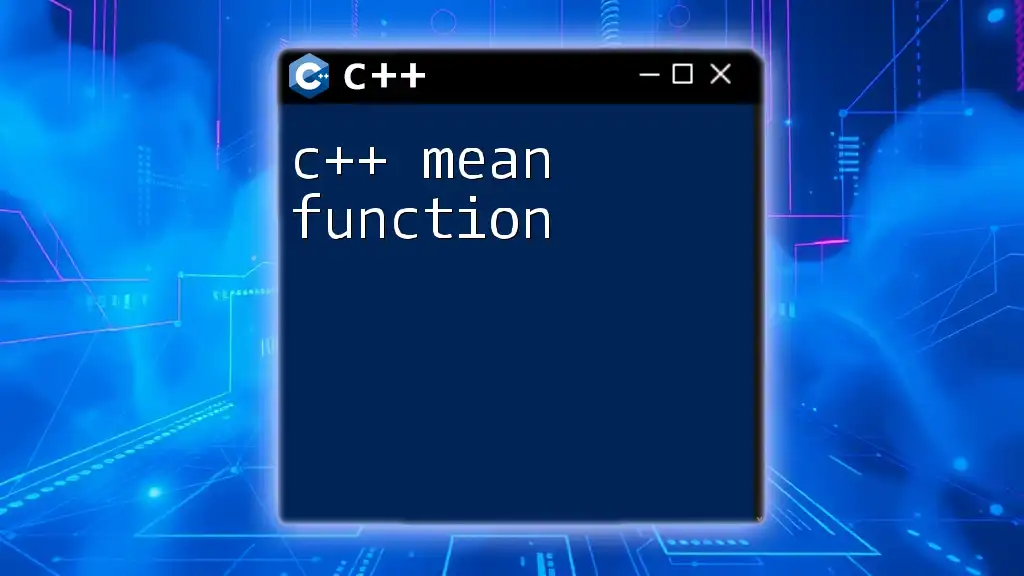
Conclusion
C++ inline functions are a powerful tool that can enhance performance, readability, and maintainability of code. By understanding when and how to use inline functions, programmers can write more efficient, cleaner C++ code. Whether you’re optimizing frequently called arithmetic functions or creating template-based utilities, inline functions are an essential part of every C++ developer's toolkit.
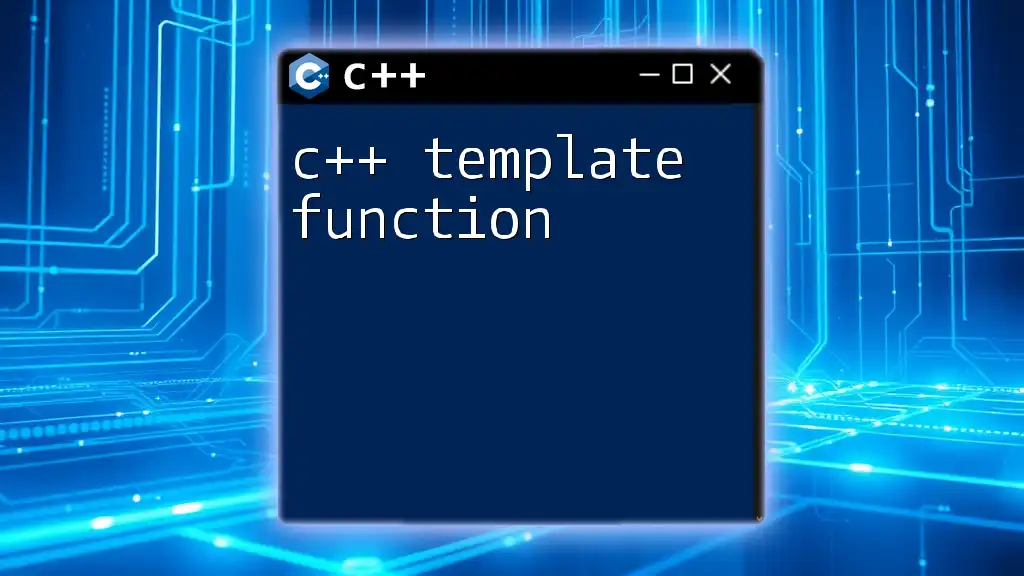
Call to Action
For those looking to deepen their understanding of C++, exploring additional resources on inline functions is highly encouraged. Share your experiences and questions regarding the use of inline functions, and let’s continue to enhance our coding skills together!