The C++ square function takes an integer input and returns its square by multiplying the number by itself.
Here’s a simple implementation in C++:
int square(int num) {
return num * num;
}
Understanding the Square Function in C++
What is Squaring in C++?
Squaring in C++ is the mathematical operation that involves multiplying a number by itself. For example, the square of 4 is \(4 \times 4 = 16\). This simple operation has various applications in programming, from mathematical calculations in algorithms to complex simulations in engineering and sciences.
Why Use a Square Function?
Using a square function encapsulates the logic of squaring a number into a reusable component. This promotes code readability and maintainability—important principles in software development. Rather than repeatedly writing the squaring operation wherever needed, encapsulating it into a function allows you to easily invoke it, making the code cleaner and simpler to understand.
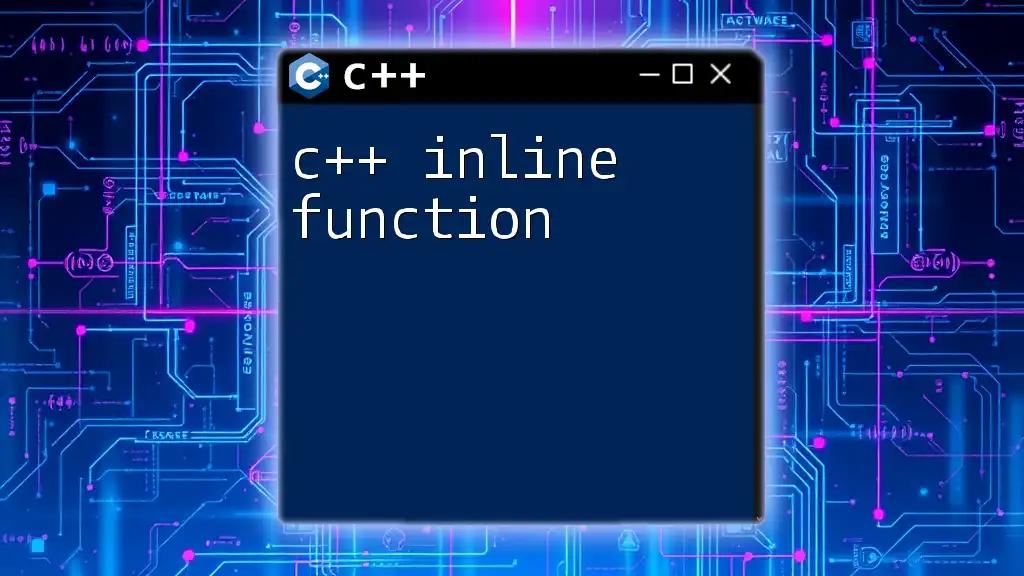
How to Square a Number in C++
Creating Your First C++ Square Function
To start with, let's define a basic function that squares a number. The function can be defined to handle different data types, but we'll focus first on a double, which is sufficient for many scenarios.
Code Example:
double square(double num) {
return num * num;
}
Understanding Data Types
C++ offers a variety of data types including `int`, `float`, and `double`, each serving different purposes based on the range and precision needed.
- `int`: Best for whole numbers without fractions.
- `float`: Suitable for single-precision floating-point numbers, useful when you're dealing with numbers that require decimal points but less precision.
- `double`: Ideal for double-precision floating-point numbers, needed when calculations demand higher accuracy.
Choosing the right data type for your square function is crucial, depending on whether you're working with integers or decimal numbers.
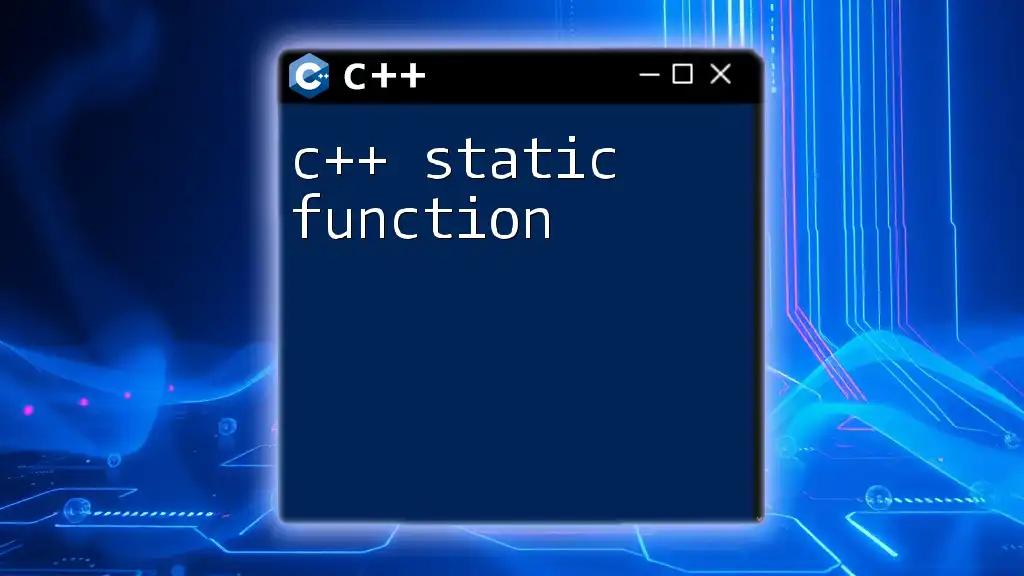
Squaring Numbers in C++: Step-by-Step
Step 1: Writing the Square Function
A complete square function structure includes specifying the return type, the function name, and the parameters. Here’s how you can define a function that accepts an `int`:
Code Example:
int square(int num) {
return num * num;
}
Step 2: Calling the Square Function
To utilize your square function, it needs to be called within the main function. Here's a complete program demonstrating this:
#include <iostream>
using namespace std;
int square(int num) {
return num * num;
}
int main() {
int value = 5;
cout << "The square of " << value << " is " << square(value) << endl;
return 0;
}
Step 3: Testing the Square Function
Testing is vital to ensure that your function behaves as expected under different conditions. Here are a few examples of invoking the square function with various inputs:
cout << "The square of -3 is " << square(-3) << endl; // Expect 9
cout << "The square of 0 is " << square(0) << endl; // Expect 0
Running these tests allows you to verify the behavior of your function and ensures that it handles different types of input correctly.
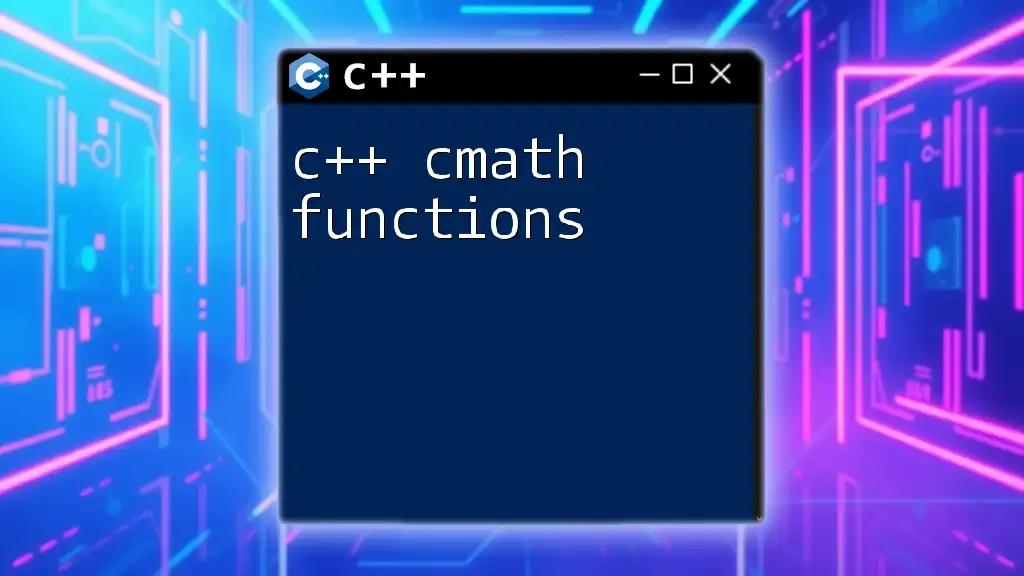
Advanced Concepts: C++ Square Function Variations
Using Templates for Flexibility
To make your square function more flexible and able to handle a variety of data types, you can utilize C++ templates. By doing this, you allow the function to accept any data type that supports multiplication.
Code Example:
template <typename T>
T square(T num) {
return num * num;
}
Overloading the Square Function
Function overloading offers a way to define multiple functions with the same name but differing parameters. This can be particularly useful for squaring different data types. Here’s how to overload the square function to handle both `int` and `double` types:
int square(int num);
double square(double num);
In this case, C++ will automatically call the correct function based on the argument type passed during the function call.
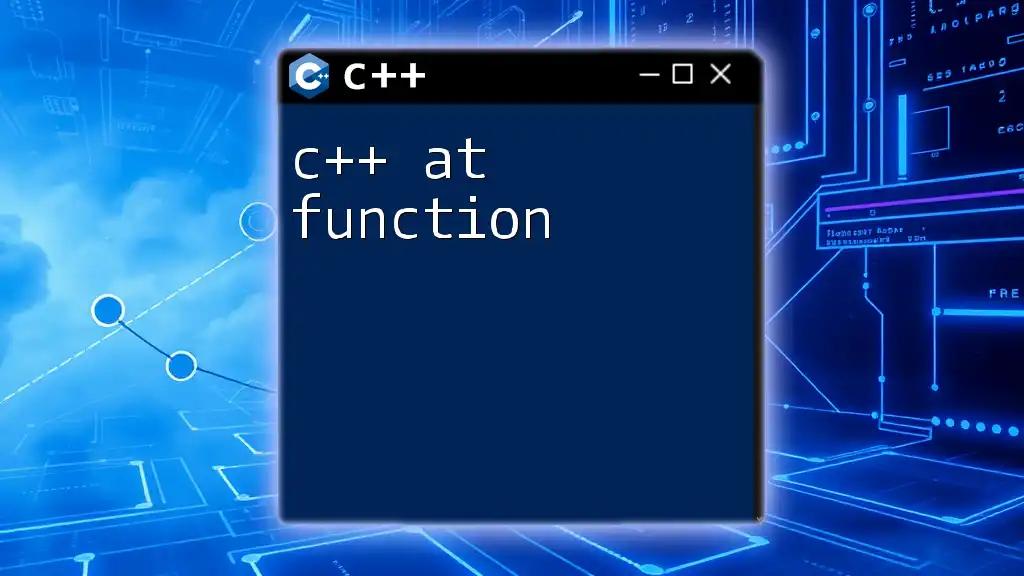
Performance Considerations in Squaring Numbers in C++
Efficiency of Squaring in C++
The efficiency of your squaring function can significantly impact performance, especially in high-performance computing scenarios. Using multiplication is typically efficient, but if you find that you need to square numbers in a high-frequency loop, it may be worth considering optimizations.
Library Alternatives for Mathematical Operations
While squaring a number is straightforward, C++ also provides several libraries (like `<cmath>`) that include mathematical functions. Depending on your needs, these libraries can provide more robust mathematical capabilities. However, for simple squaring, writing your own function is perfectly acceptable and often more readable.
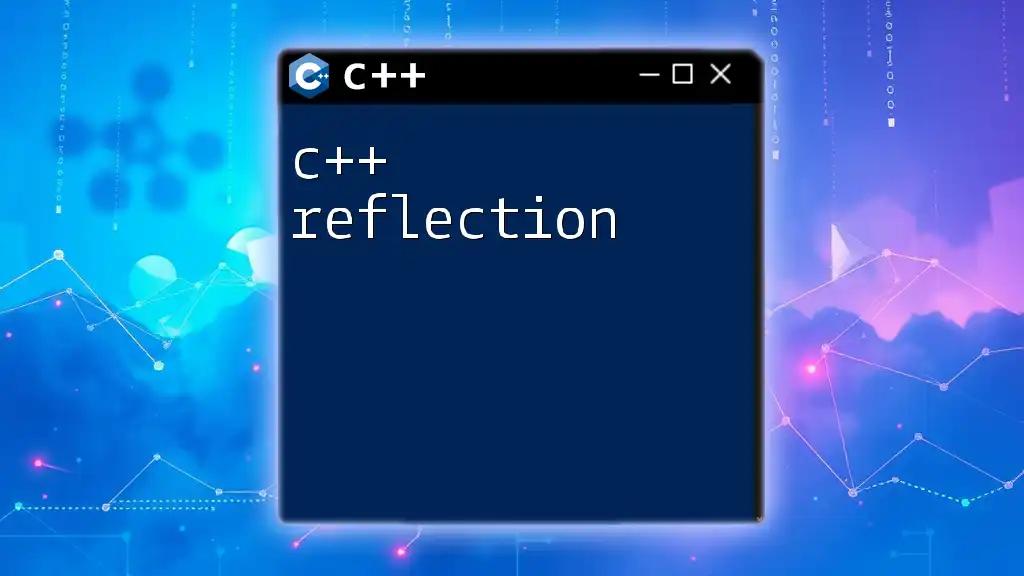
Conclusion
Understanding how to implement the C++ square function is a fundamental skill for any programmer, enhancing both your coding efficiency and capability to write maintainable software. By practicing writing this function and exploring its variations, you further your knowledge in C++ programming.
Explore beyond the square function into other mathematical operations in C++, and consider how encapsulating these operations can foster clean, effective coding.