A C++ function library is a collection of pre-defined functions that users can call in their programs to perform specific tasks, enhancing code reusability and efficiency.
#include <iostream>
#include <cmath> // Example of including a function library
int main() {
double result = sqrt(25.0); // Using a function from the cmath library
std::cout << "Square root of 25 is: " << result << std::endl;
return 0;
}
Introduction to C++ Function Libraries
A C++ function library is a collection of pre-written functions that provide various functionalities that can be reused across multiple programs. These libraries save developers considerable time and effort by allowing them to leverage existing, well-tested code rather than writing everything from scratch. Utilizing C++ library functions is integral for efficient coding, promoting code reusability, reliability, and maintainability.
Benefits of Utilizing C++ Library Functions
- Code Reusability: By using C++ library functions, you reduce redundancy in your code. They allow you to focus on specific program logic while relying on off-the-shelf solutions for common tasks.
- Time-Saving: Function libraries have been optimized and tested, thus developers can implement solutions faster without delving into the intricacies.
- Reliability: Libraries like the C++ Standard Library are built and maintained by experts, ensuring reliability and robustness, which would take considerable effort to replicate.
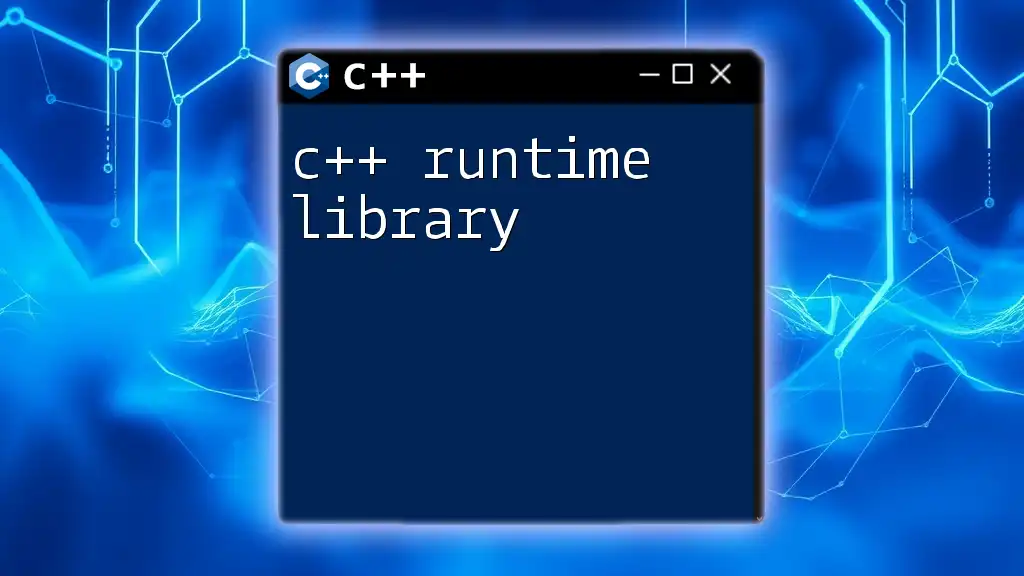
Overview of C++ Standard Library
What is the Standard Library?
The C++ Standard Library provides a wealth of pre-written code that can be used across various platforms. It consists of various components, such as headers and functions, organized to facilitate programming tasks.
Categories of the Standard Library
- Containers: These include data structures such as vectors, lists, and maps, which help in organizing data efficiently.
- Algorithms: A powerful collection of algorithms for searching, sorting, and manipulating data within containers.
- Input/Output: Handling data coming into and going out of a program through streams.
- Strings and Streams: Functions and classes dedicated to string manipulation and data stream processing.
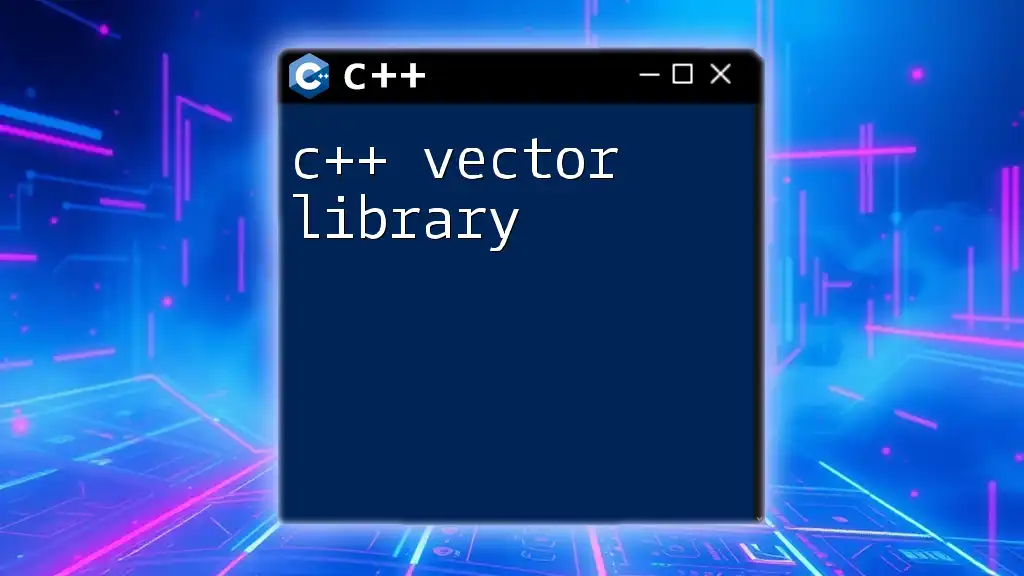
Core C++ Library Functions
Mathematical Functions
The `cmath` library provides a comprehensive suite of mathematical functions.
Common Functions
Among the most utilized functions from the `cmath` library are `sqrt()`, `pow()`, `sin()`, and `cos()`.
Example: Here’s a simple code snippet showing how to use one of these functions:
#include <cmath>
#include <iostream>
int main() {
double number = 16.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
Explanation: The above code snippet demonstrates how to calculate the square root of 16 using the `sqrt()` function from the `cmath` library. The result will be displayed in the console as "The square root of 16 is 4".
Input and Output Functions
The `iostream` library is fundamental in C++ for handling input and output operations.
Critical Functions
Functions like `std::cout` and `std::cin` are essential for displaying messages and retrieving user inputs, respectively.
Example: Below is a simple program that uses these functions:
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
Explanation: This snippet captures user input from the console, storing it in the variable `number`, which is then displayed back to the user.
String Manipulation Functions
The `string` library in C++ presents a set of functions that enhance the handling and manipulation of strings.
Essential Functions
Key string functions include `size()`, `substr()`, and `append()`.
Example: Here’s how the `append()` function works:
#include <string>
#include <iostream>
int main() {
std::string text = "Hello";
text.append(" World");
std::cout << text << std::endl;
return 0;
}
Explanation: This code showcases concatenating the string " World" to "Hello" utilizing the `append()` function, resulting in the console displaying "Hello World".
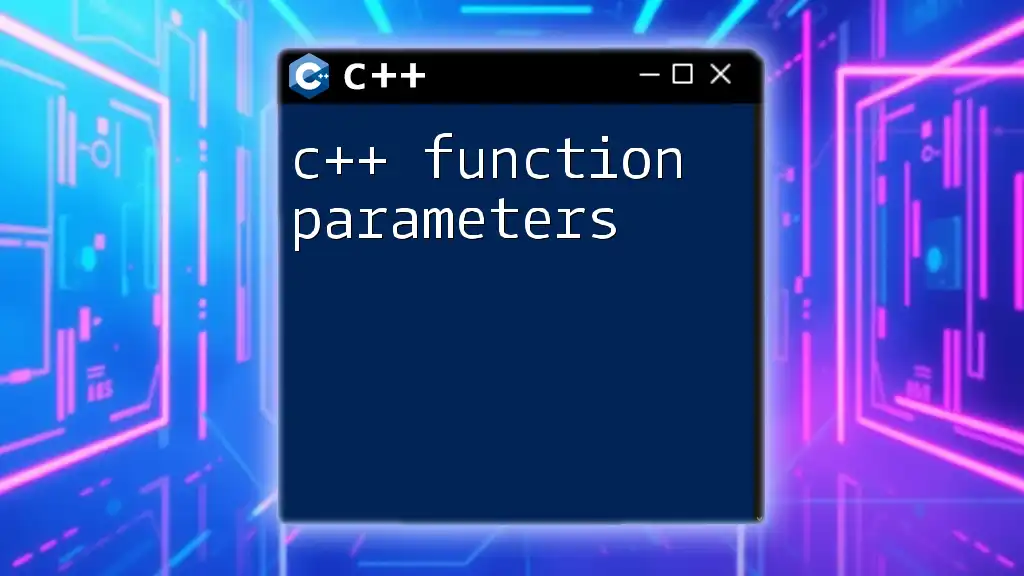
Exploring Custom Function Libraries
Creating Your Own Function Library
Building a custom function library allows for tailored functionalities that can suit specific programming needs. The creation process generally involves creating a header file containing function declarations.
Example: A basic math function library can look like this:
// math_functions.h
double add(double a, double b);
double subtract(double a, double b);
Explanation: This example defines a header file named `math_functions.h` that declares two functions, `add` and `subtract`, for use in various programs.
Linking Custom Libraries
After creating a custom library, it’s essential to compile and link it with your main program. This process varies depending on the compiler but usually involves specifying the path to the library files during the compilation step.
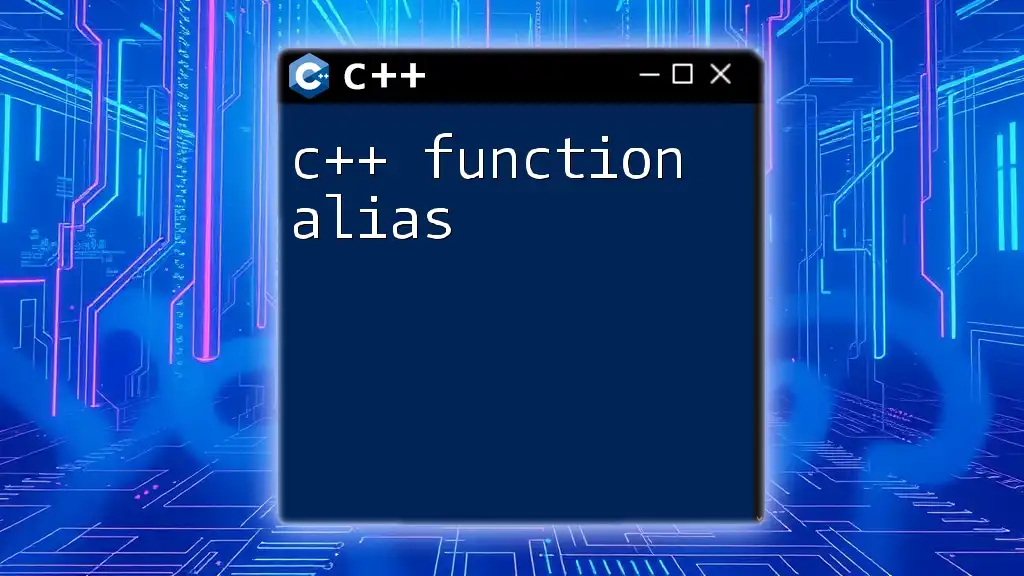
Popular C++ Libraries and Frameworks
Boost: A Detailed Look
Boost is a highly regarded C++ library that provides numerous functionalities including support for smart pointers, regular expressions, and many more. It simplifies complex tasks, ultimately enhancing productivity and code quality.
Qt: GUI Library
Qt is a versatile library primarily designed for developing graphical user interfaces. It provides tools for creating responsive and visually appealing applications, making it easier to build cross-platform applications.
OpenCV: Computer Vision Library
OpenCV is an essential library for computer vision and image processing tasks. It comes with functions that help in tasks such as image filtering, object detection, and video analysis, enabling developers to integrate advanced visual capabilities within their applications.
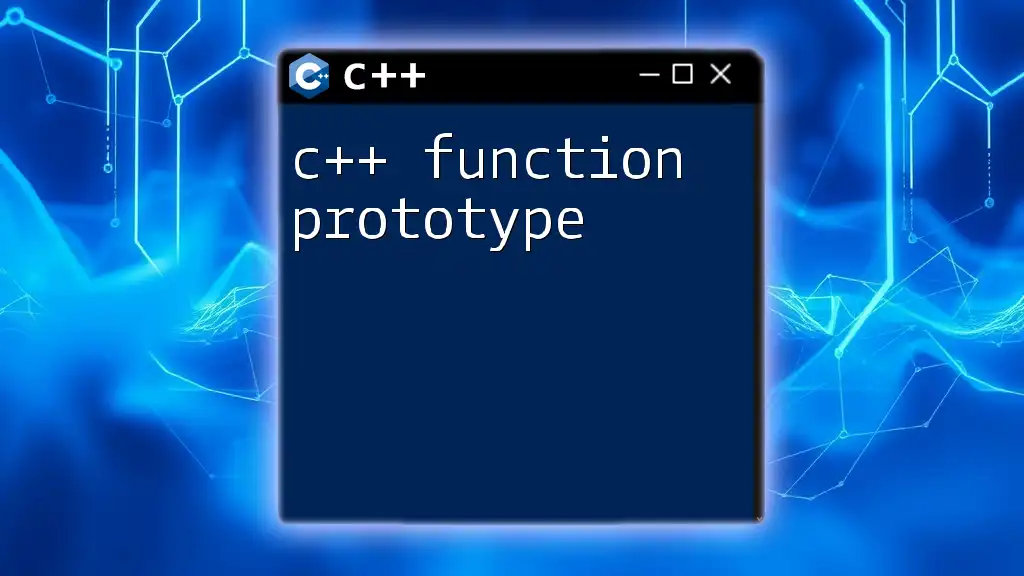
Best Practices for Using C++ Function Libraries
Organizing Your Code
Structured code organization is vital for enhancing readability and maintainability. Keeping functions logically grouped and adhering to coding standards will simplify future debugging and collaboration.
Using Namespaces
Namespaces help avoid conflicts among identifiers in different libraries or sections of code. This practice is crucial when using multiple libraries that may contain functions with the same names.
Documentation and Comments
Documenting your functions and libraries is crucial for both your understanding and for others who may use your code later on. Comments should explain the purpose of functions, parameters, and expected output, ensuring easier maintenance and usability.
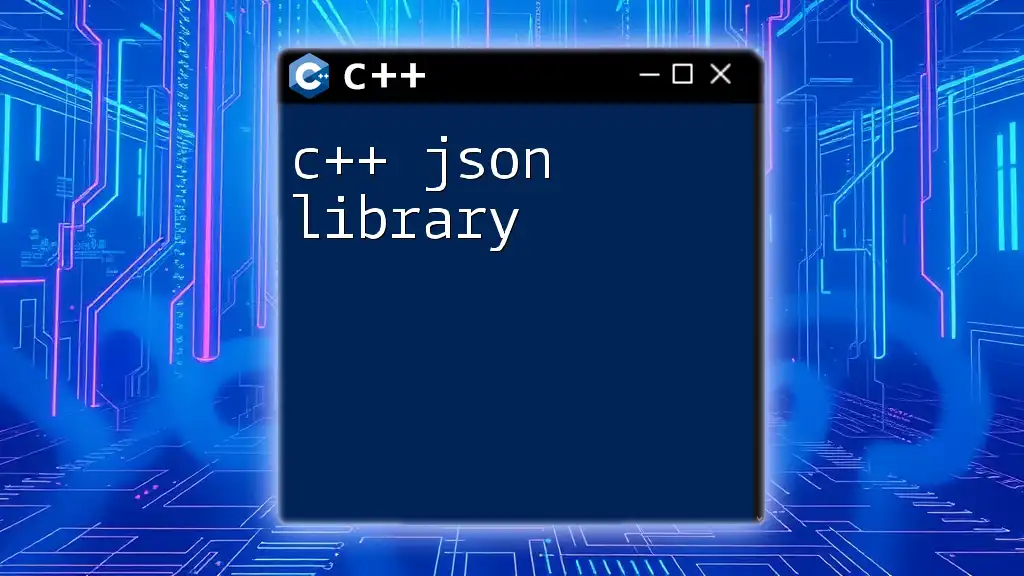
Conclusion
The C++ function libraries offer invaluable resources that enhance programming productivity through established, reusable code. Building and utilizing both standard and custom libraries enables developers to create robust and efficient applications. The future of C++ function libraries will continue to evolve, encouraging programmers to leverage these tools to push the boundaries of what’s possible in C++. Exploring and experimenting with libraries is not just beneficial; it is essential for mastering C++ programming.
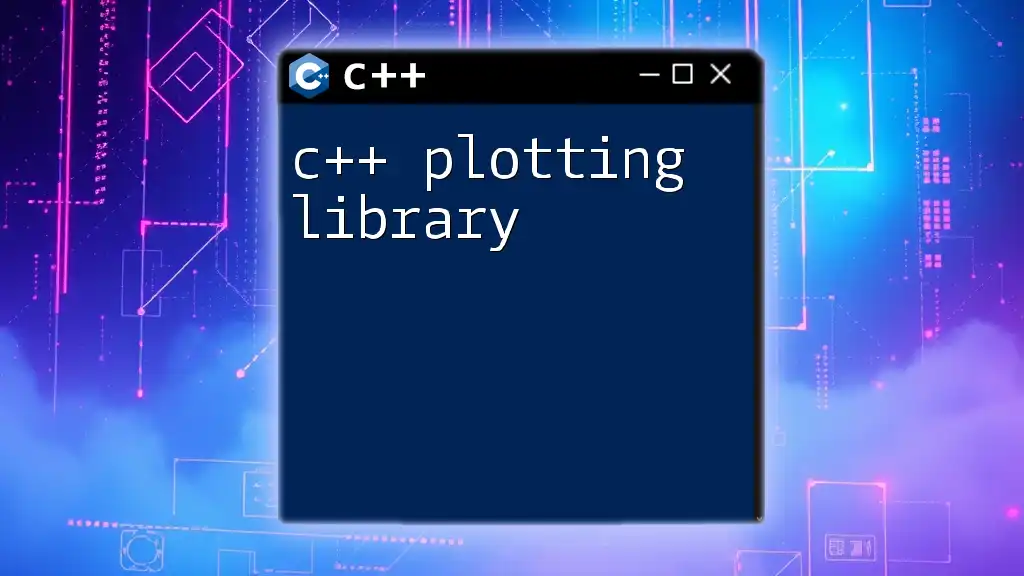
Further Resources
For those looking to deepen their understanding of C++, numerous books and online tutorials are available. Additionally, participating in online forums and communities can provide further insights, support, and collaboration opportunities in this vast programming landscape.