A C++ plotting library enables developers to create visual representations of data through graphs and charts, enhancing data analysis and presentation. Here's a simple code snippet using the popular `matplotlibcpp` library for plotting a sine wave:
#include <iostream>
#include "matplotlibcpp.h"
#include <cmath>
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x, y;
for (double i = 0; i < 100; i++) {
x.push_back(i);
y.push_back(sin(i * M_PI / 50)); // sine function
}
plt::plot(x, y);
plt::title("Sine Wave");
plt::xlabel("x");
plt::ylabel("sin(x)");
plt::show();
return 0;
}
Why Use a Plotting Library in C++?
Using a C++ plotting library has distinct advantages that cater to different needs across industries. One of the primary benefits is efficiency in data handling. C++ excels in processing vast datasets owing to its speed and performance. Compared to other languages like Python or R, where data is often interpreted at runtime, C++ compiles to machine code that runs directly on hardware, making it exceptionally efficient for scientific computing.
Another significant advantage is integration with existing C++ code. Many applications in fields such as physics, engineering, and finance require data visualization tools that can seamlessly integrate with their backend systems. Leveraging C++ libraries allows developers to create plots without switching languages or frameworks, facilitating smoother workflows and optimized performance.
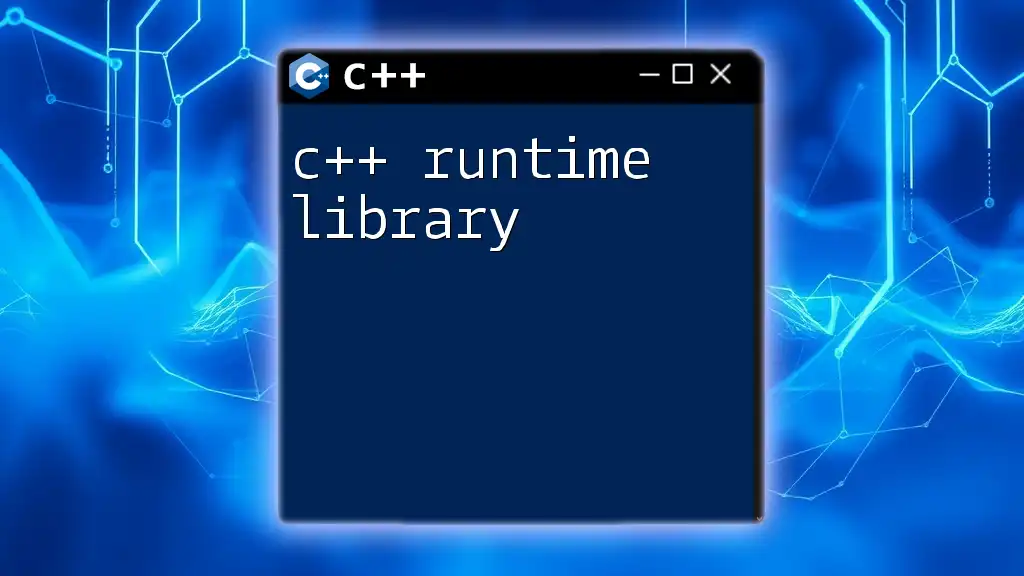
Choosing the Right C++ Plotting Library
When venturing into the world of C++ plotting libraries, it’s essential to consider various options. Here’s a quick overview of some popular libraries:
- Matplotlib (CPPPlot): A C++ wrapper for Python’s Matplotlib, particularly useful for users familiar with Python syntax.
- QCustomPlot: An easy-to-use option for creating highly customizable 2D plots in Qt applications.
- ROOT: Originally designed for particle physics, ROOT excels in large datasets, enabling complex data analysis and visualization.
When choosing a library, consider factors such as the complexity of data, the types of plots you need (like line graphs, scatter plots, or bar charts), and performance and optimization requirements. Libraries vary in ease of use and computational efficiency; hence, selecting one that aligns with your project goals is crucial.
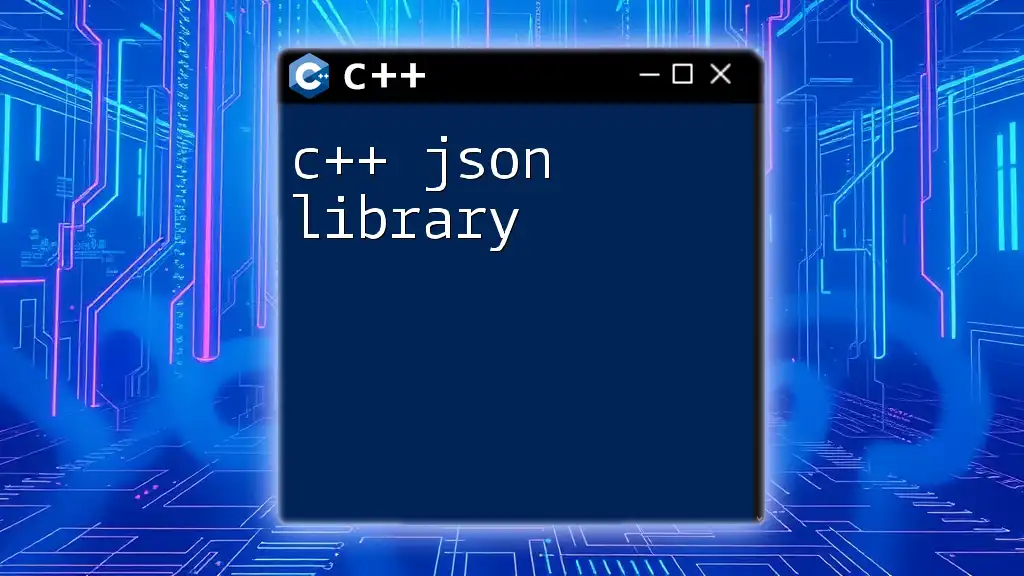
Getting Started with C++ Plotting Libraries
To get started with a C++ plotting library, the first step is installation and setup. For instance, if you choose QCustomPlot, you can follow these steps:
- Download the library: Visit the QCustomPlot website and download the latest version.
- Include the library in your project: Copy the QCustomPlot files into your project directory.
- Set up the development environment: Make sure your IDE supports C++. If using Qt, ensure the Qt framework is correctly configured.
Here’s a straightforward example of how to install QCustomPlot using CMake:
find_package(Qt5Widgets REQUIRED)
include_directories(${CMAKE_CURRENT_SOURCE_DIR}/include)
add_executable(MyPlottingApp main.cpp)
target_link_libraries(MyPlottingApp Qt5::Widgets)
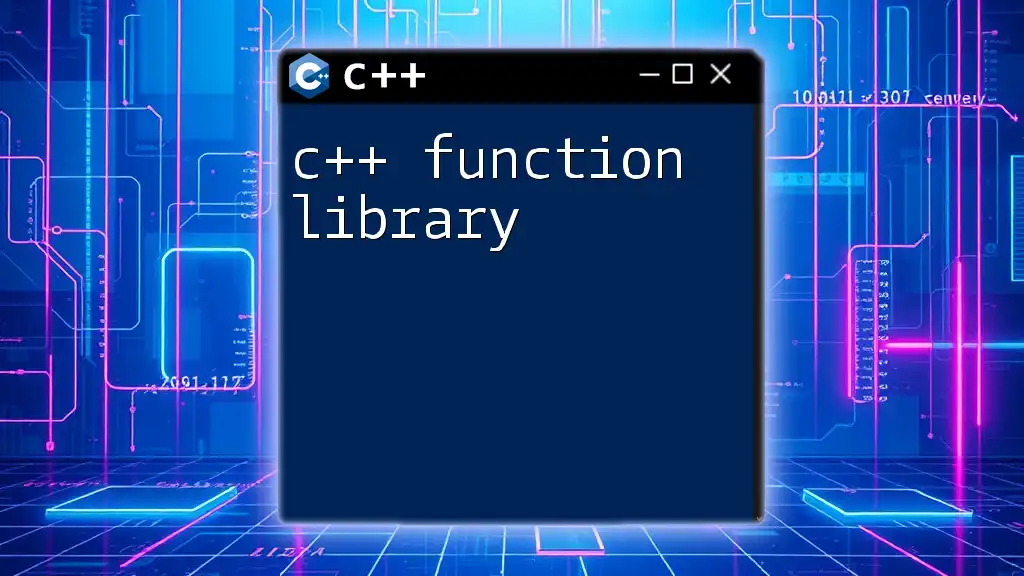
Basic Plotting Example
Creating your first plot can be an exciting venture! Here’s a step-by-step guide to making a basic line plot using QCustomPlot.
First, you need to set up your plotting canvas:
#include <QCustomPlot.h>
void ExamplePlot() {
QCustomPlot *customPlot = new QCustomPlot();
QVector<double> x(101), y(101); // initialize vectors
for (int i = 0; i < 101; ++i) {
x[i] = i / 50.0 - 1; // x ranges from -1 to 1
y[i] = x[i] * x[i]; // function y=x^2
}
customPlot->addGraph(); // Create a new graph
customPlot->graph(0)->setData(x, y); // Set the data
customPlot->xAxis->setLabel("x"); // Label for x-axis
customPlot->yAxis->setLabel("y"); // Label for y-axis
customPlot->replot(); // Refresh the plot to display the data
}
In the snippet above, we initialize two vectors for our x and y coordinates and then populate them. The significant function here is `setData()`, where the x and y values are assigned to the plot. After labeling the axes, `replot()` gets called to render the graph.
Explanation of the Code
- `QCustomPlot *customPlot`: Declares a new instance of the plotting object.
- `QVector<double> x(101), y(101)`: Initializes arrays to hold data points.
- The loop iterates to populate x with values ranging from -1 to 1, while y computes the square of each x value.
- `addGraph()`: Creates a new data series in the plot.
- `setLabel()`: Sets labels for the x and y axes, providing clear information on the data being presented.
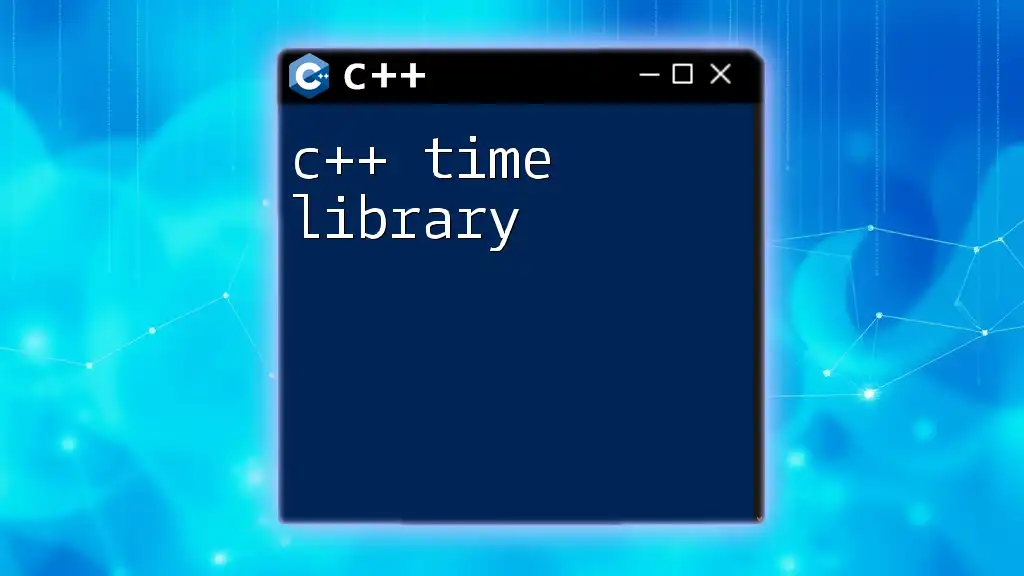
Advanced Plotting Techniques
Once you are comfortable with basic plotting, you can explore advanced techniques such as overlaying multiple plots or customizing the appearance of your graphs.
Multiple Plots
Overlaying graphs can provide insights into multiple datasets simultaneously. Here’s how you can add an extra graph:
QVector<double> x2(101), y2(101);
for (int i = 0; i < 101; ++i) {
y2[i] = std::sin(x[i]); // Another function y=sin(x)
}
customPlot->addGraph(); // Add a second graph
customPlot->graph(1)->setData(x, y2); // Setting data for the second graph
Customization of Plots
One of the most appealing features of a C++ plotting library is the ability to customize your plots. You can modify styles, colors, and annotations. For instance, you may want to change the line colors:
customPlot->graph(0)->setPen(QPen(Qt::blue)); // Change line color for first graph
customPlot->graph(1)->setPen(QPen(Qt::red)); // Set color for the second graph
customPlot->replot();
This allows you to enhance visual clarity and aesthetics, making your data representation more appealing and easier to interpret.
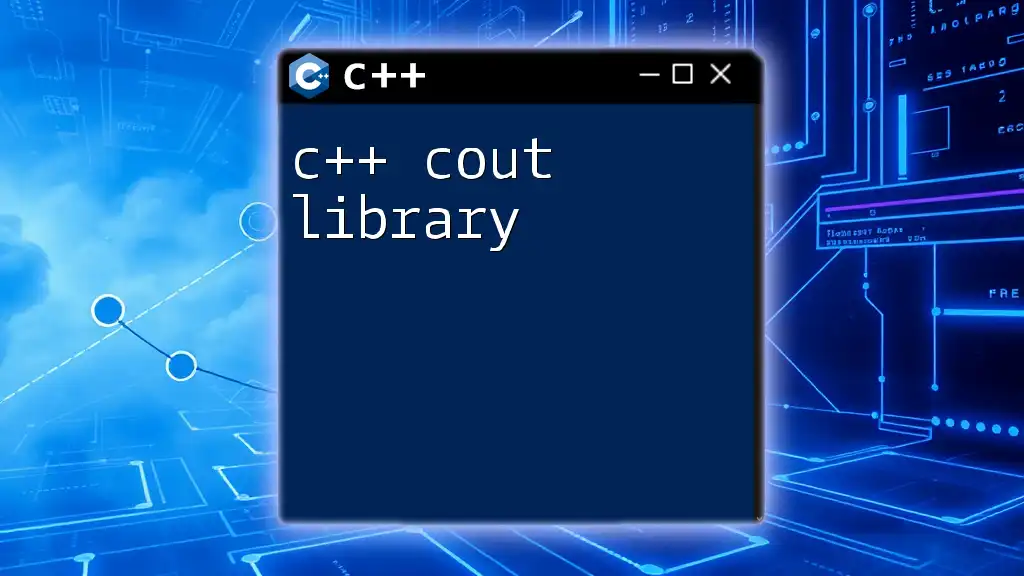
Exploring Different Plot Types
Expanding on the basic line plot, other plot types can be utilized for various data representations:
Bar Charts
Bar charts are effective for categorical data. To implement a bar chart in QCustomPlot, use the `QCPBars` class to visualize your dataset efficiently.
Scatter Plots
Scatter plots are ideal for visualizing relationships between two numerical variables. You can create them by adding points to your plot rather than connecting them with lines.
Heatmaps
Heatmaps represent data density or values over a two-dimensional area, offering a compelling way to visualize large data sets that exhibit patterns.
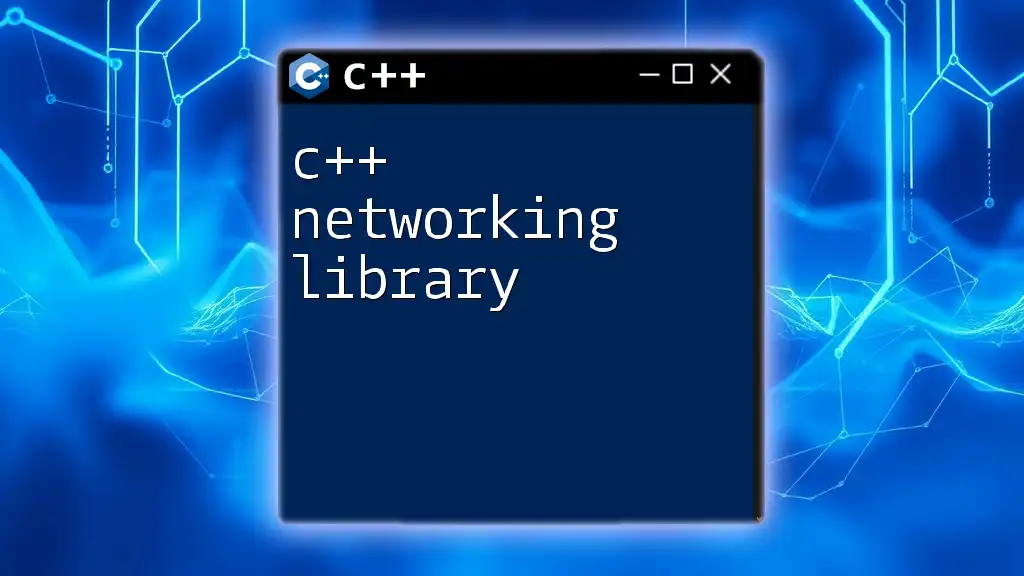
Troubleshooting Common Issues
You may encounter some challenges when using C++ plotting libraries, especially during the initial setup. Common problems can include compilation issues where required dependencies are not correctly linked. To resolve this, double-check your project settings and ensure all libraries are included.
Runtime errors may arise from incorrectly defined data ranges or mismatched functions and datasets. Always validate your data input and ascertain that the arrays you provide align with your plotting expectations.
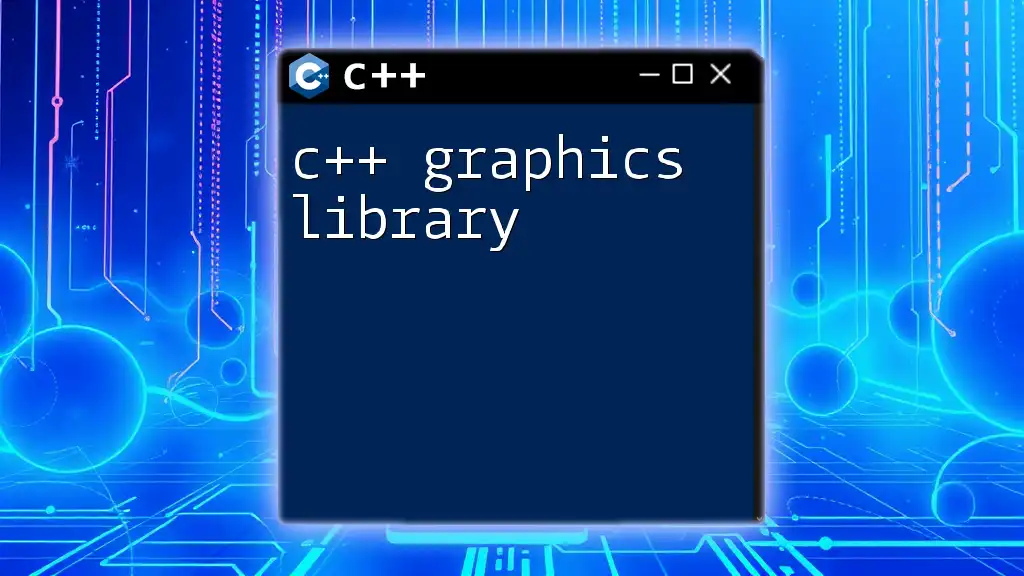
Real-World Applications
Using C++ plotting libraries is not just an academic exercise; they're widely used in various domains. In scientific research, for instance, researchers utilize these libraries to visualize experimental data, derive conclusions, and present findings effectively.
In the realm of business analytics, visualizing sales trends, customer behavior, and operational metrics can significantly aid in data-driven decision-making.
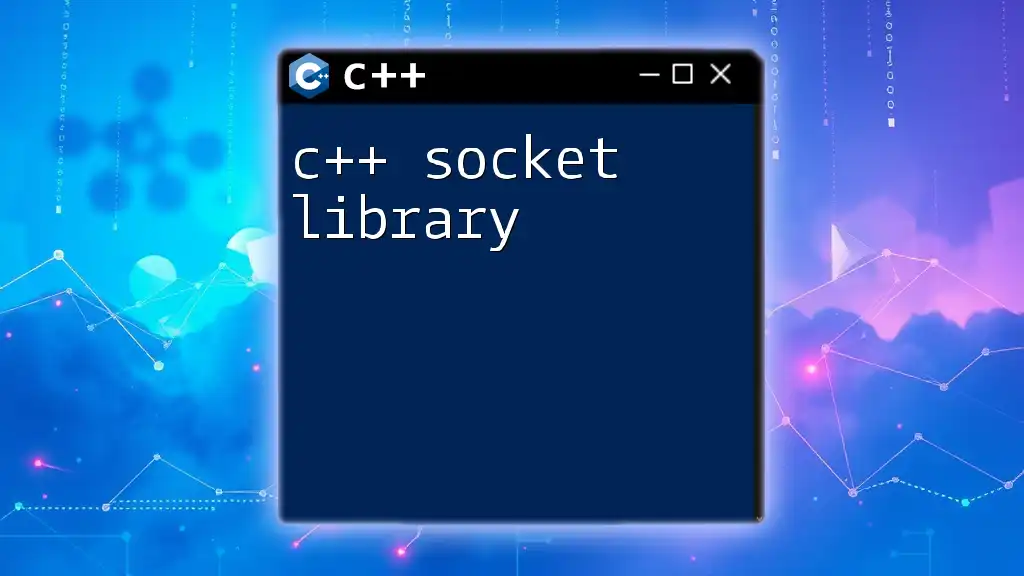
Additional Resources
To further enhance your skills in using C++ plotting libraries, consider exploring online resources such as:
- Library documentation: Most libraries come with comprehensive guides.
- Tutorials and forums: Engaging with community platforms like GitHub or Stack Overflow can provide valuable insights and troubleshooting assistance.
- Books on C++ and data visualization: Investing in literature can deepen your knowledge and skill set.
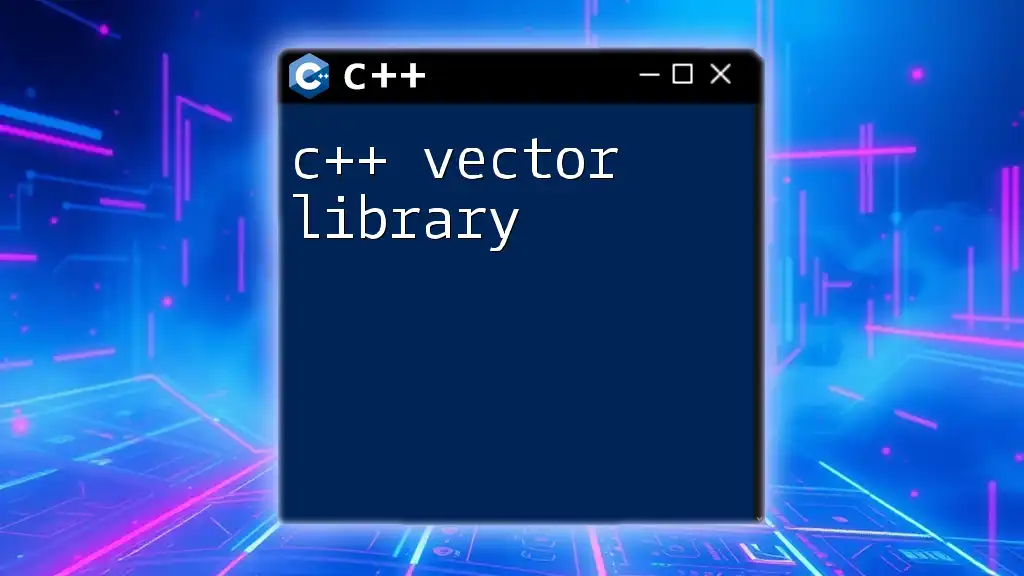
Conclusion
In summary, C++ plotting libraries offer powerful tools for visualizing data efficiently while integrating seamlessly into existing C++ projects. By leveraging these libraries, developers can enhance their data representation capabilities, leading to better insights and informed decision-making. Embrace the exploration of various attributes and techniques offered by these libraries for sophisticated analysis and compelling presentations.
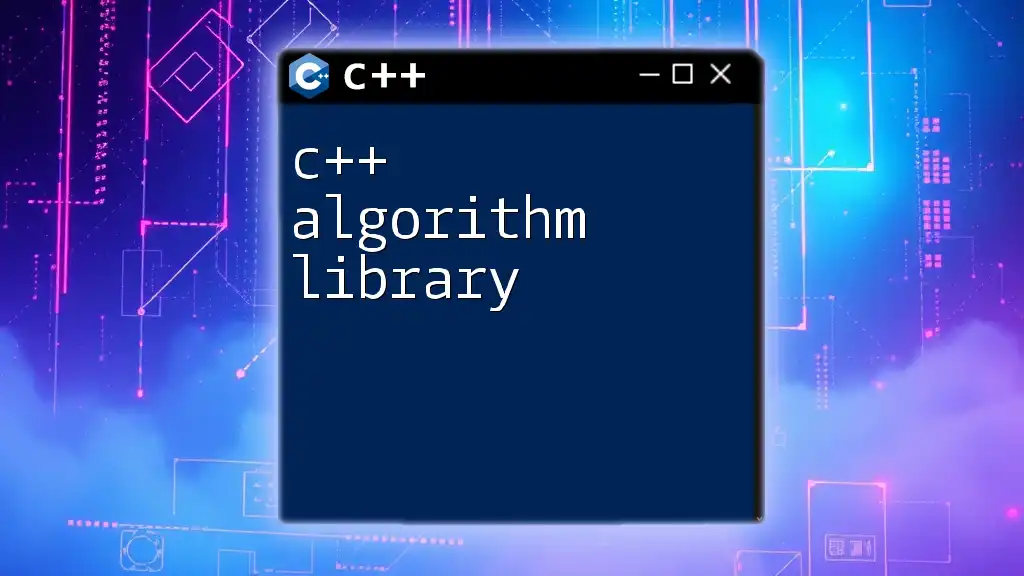
Call to Action
We encourage you to share your experiences using C++ plotting libraries! Whether it’s a specific question, a library experience, or a feature request, your feedback can help shape future content and discussions in this evolving field. Happy plotting!