A C++ graphics library provides tools and functions for creating visual output in applications, enabling developers to render shapes, images, and animations more easily.
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "C++ Graphics Example");
sf::CircleShape shape(50);
shape.setFillColor(sf::Color::Green);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
What Are Graphics Libraries?
Graphics libraries are collections of pre-written code that simplify the process of drawing graphics in applications. They provide a set of functions for performing common graphics tasks, which significantly reduces the amount of boilerplate code developers must write.
Using a C++ graphics library enables programmers to focus on building their applications rather than dealing with the lower-level details of graphics rendering. This is particularly critical in fields such as game development, simulation, and scientific visualization, where efficient rendering of graphics is essential.
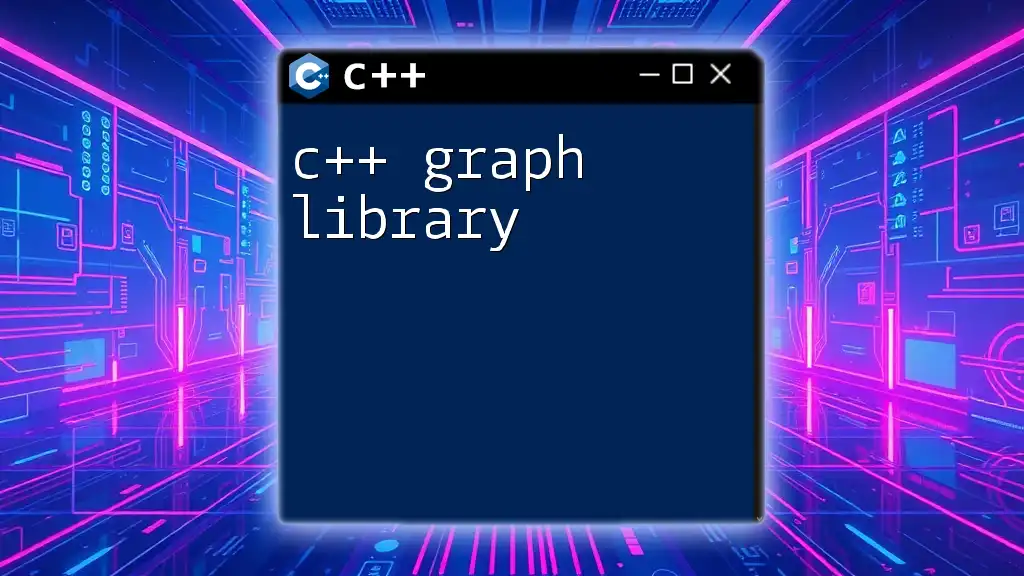
Overview of Popular C++ Graphics Libraries
Several C++ graphics libraries cater to different needs and types of applications. Understanding these libraries can guide you in choosing the right tool for your project.
SDL (Simple DirectMedia Layer)
SDL is a widely-used library that provides a simple interface to various multimedia functionalities, including graphics, audio, and input devices. It is particularly favorable for game developers and those working on multimedia applications.
Features include:
- Cross-platform compatibility.
- Support for 2D graphics and basic 3D functionalities.
- Ease of integration with other libraries.
SFML (Simple and Fast Multimedia Library)
SFML is another user-friendly library that excels in 2D graphics. It provides a straightforward interface to handle windows, graphics, audio, and network functionalities.
Advantages of SFML:
- Object-oriented design, making it easier to use and understand.
- Built-in support for various graphics elements like shapes, sprites, and text.
- Great for beginners and small to medium-sized projects.
OpenGL
OpenGL is a powerful graphics API for rendering 2D and 3D vector graphics. It is more complex than SDL or SFML but offers extensive capabilities and flexibility for advanced graphics applications.
Use cases for OpenGL:
- Video games and simulations where performance is critical.
- Applications requiring fine control over rendering and effects.
- Professional graphic applications such as CAD software.
Allegro
Allegro is a cross-platform library that provides various functionalities for creating multimedia applications. It's often favored for its simplicity and suitability for game development.
Features of Allegro include:
- Support for 2D graphics, sound, and input handling.
- Good documentation and community support.
- Eases the process of creating games or graphics-intensive applications.
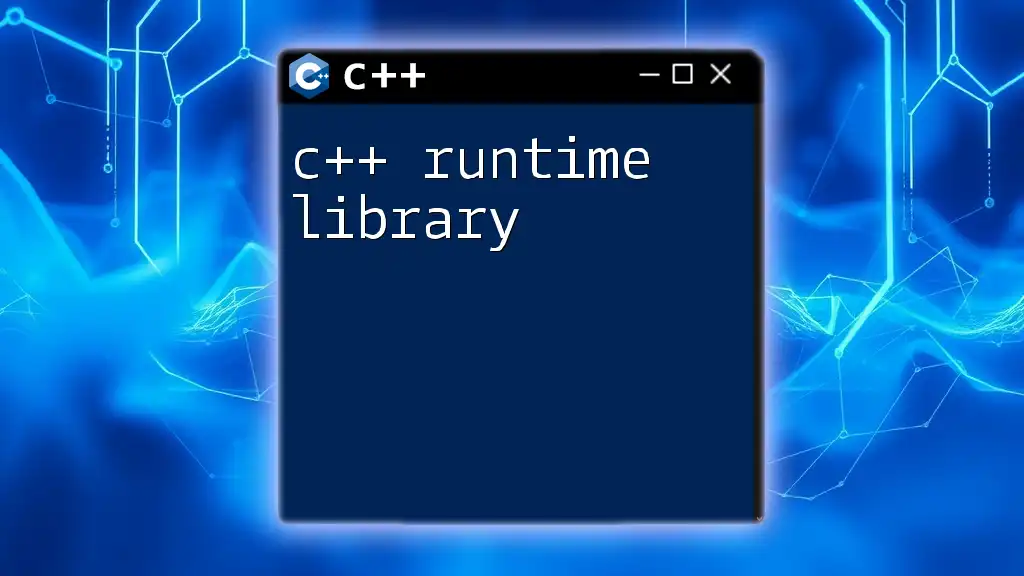
Setting Up Your Environment for C++ Graphics
To develop applications using any C++ graphics library, you first need to set up your environment correctly.
Required Software
Before starting, ensure you have a C++ compiler installed. Popular choices include GCC and MSVC. Additionally, you might want to use an Integrated Development Environment (IDE) like Visual Studio or Code::Blocks, which simplifies the coding process and management of libraries.
Installing Essential Libraries
The installation process varies depending on the library you choose. Below are the steps you would typically follow for SDL, SFML, and OpenGL.
- SDL: Visit the [SDL website](https://libsdl.org) to download and follow the installation instructions for your operating system.
- SFML: You can access the SFML library from its [official site](https://www.sfml-dev.org). Installation typically includes downloading the library and setting up linker paths, include paths, and library directories within your IDE.
- OpenGL: For OpenGL, you often need to install additional libraries like GLEW or GLFW. Make sure to check their documentation for installation guides.
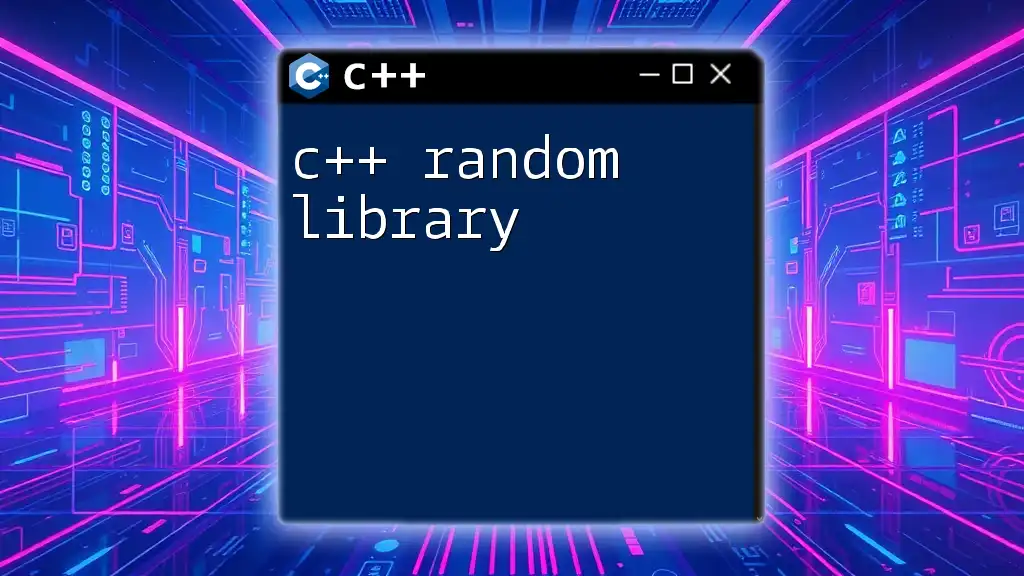
Getting Started with SDL
Basic SDL Initialization
Getting started with SDL involves initializing the library before you can perform any graphics rendering. Below is a simple code snippet to initialize SDL:
#include <SDL.h>
int main(int argc, char* argv[]) {
if (SDL_Init(SDL_INIT_VIDEO) != 0) {
// Handle error
return 1;
}
// Your code here
SDL_Quit();
return 0;
}
In this code, the initialization function `SDL_Init` sets up the SDL video subsystem. If the initialization fails, it's essential to handle the error accordingly before proceeding. Finally, `SDL_Quit()` is called to clean up resources when done.
Drawing Shapes with SDL
Once you've initialized SDL, you can create a window and start rendering shapes. The following code snippet demonstrates how to create a window and draw a simple line:
SDL_Window* window = SDL_CreateWindow("Hello SDL", 100, 100, 640, 480, SDL_WINDOW_SHOWN);
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, 0);
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255);
SDL_RenderDrawLine(renderer, 0, 0, 640, 480);
SDL_RenderPresent(renderer);
In this example, you create a window titled "Hello SDL" at coordinates (100, 100) with a width of 640 pixels and a height of 480 pixels. You then set the draw color to red (RGB values) and draw a line from one corner to the opposite. The call to `SDL_RenderPresent` updates the window to display the rendered content.
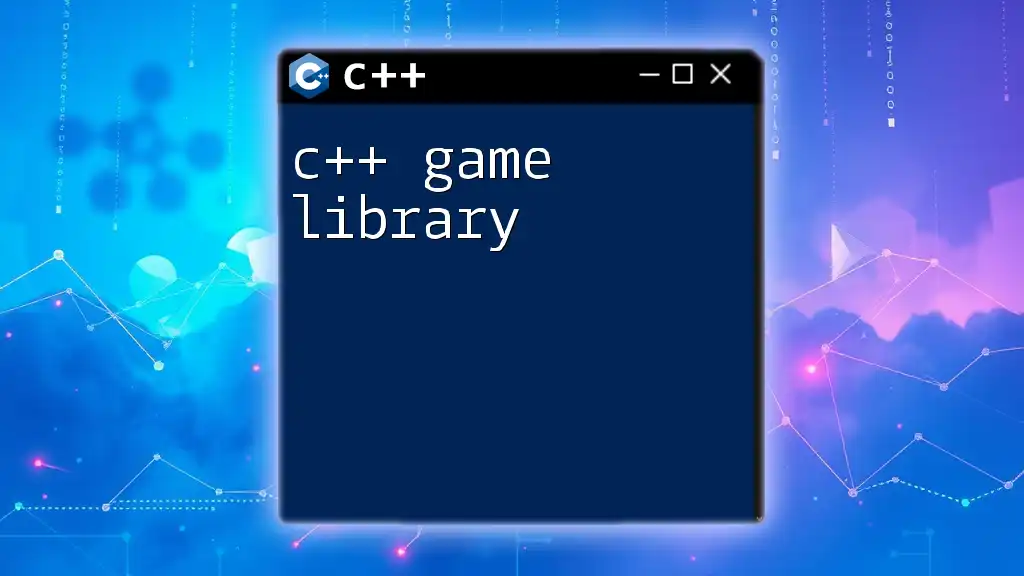
Getting Started with SFML
Library Initialization
To get started with SFML, you need to include the SFML headers and create a basic window. Below is a simple example:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML works!");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
Here, we create a window of size 800x600 pixels. The loop listens for events (like closing the window) and clears the window for new rendering. Finally, `window.display()` shows the updated frame.
Drawing Shapes and Text
SFML allows you to draw various shapes and text effortlessly. Here is how you can draw a rectangle and display text:
sf::RectangleShape rectangle(sf::Vector2f(120, 50));
rectangle.setFillColor(sf::Color::Green);
window.draw(rectangle);
In this snippet, a green rectangle of 120x50 pixels is created. You can customize its position, size, and color easily. For text rendering, SFML also provides a robust text class, allowing you to display any string with a custom font.
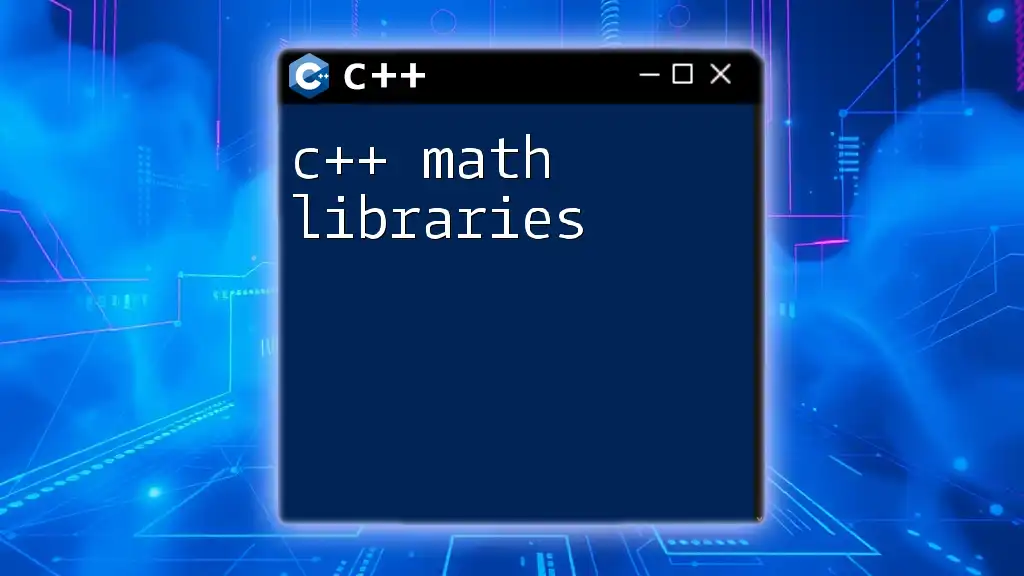
Harnessing the Power of OpenGL
Introduction to OpenGL Basics
OpenGL stands out as one of the most robust graphics APIs available, providing extensive control over rendering 2D and 3D graphics. Understanding OpenGL often requires a deeper knowledge of graphics programming and concepts such as shaders, buffers, and rendering contexts.
Setting Up OpenGL with GLFW
Before using OpenGL, you often need to set up a context, which GLFW helps facilitate. A simple initialization might look like this:
#include <GL/glew.h>
#include <GLFW/glfw3.h>
int main() {
glfwInit();
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
GLFWwindow* window = glfwCreateWindow(800, 600, "Hello OpenGL", nullptr, nullptr);
if (window == nullptr) {
// Handle error
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// OpenGL setup code here
glfwTerminate();
return 0;
}
This code sets up the GLFW library, specifies the desired OpenGL version, and creates a window. It's crucial to make the OpenGL context current with `glfwMakeContextCurrent`, enabling you to execute OpenGL commands.
Rendering a Triangle in OpenGL
Rendering a simple triangle is often the first step in learning OpenGL. You'll need to set up vertex data and use shaders:
// Vertex data for a triangle
GLfloat vertices[] = {
0.0f, 0.5f, 0.0f,
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f
};
// Shader setup code here (vertex and fragment shaders)
// Rendering loop
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
// Draw the triangle here using OpenGL commands
glfwSwapBuffers(window);
glfwPollEvents();
}
Within this setup, you declare an array of vertices for a triangle and clear the screen before each draw call. The loop continues until the window is instructed to close, allowing for continuous rendering.
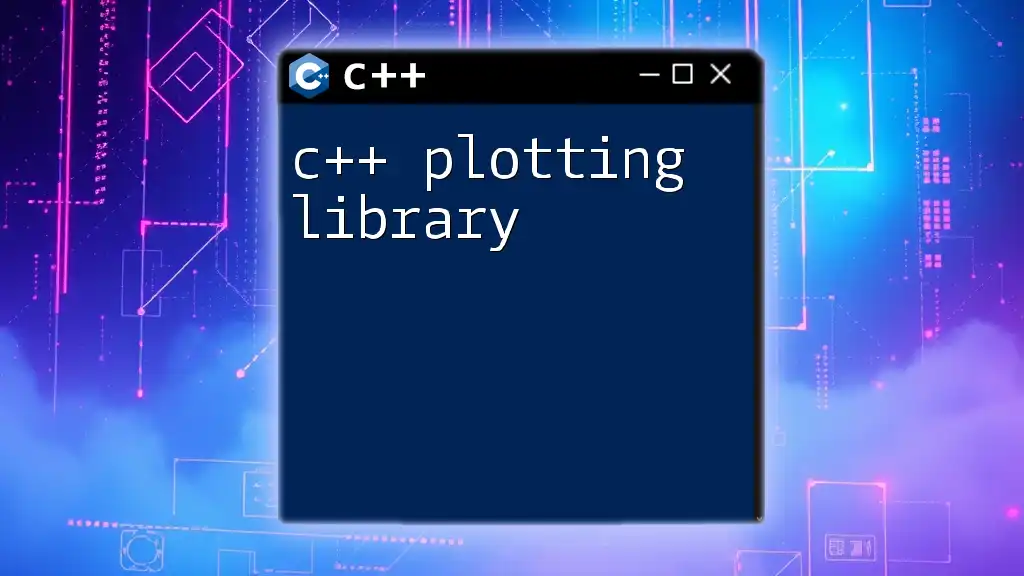
Best Practices for Graphics Programming in C++
Performance Optimization Tips
When working with C++ graphics libraries, optimizing performance is essential. To enhance your application’s graphical performance, consider the following techniques:
- Minimizing Draw Calls: Batch your rendering where possible by combining objects or drawing them in fewer calls. This dramatically reduces CPU workload.
- Resource Management: Load textures and other resources upfront rather than during rendering. This approach minimizes stutter and delays during real-time rendering.
Debugging Graphics Applications
Debugging can be particularly challenging in graphics programming due to the visual nature of the output. Utilize tools such as RenderDoc or graphics API-specific debugging tools to trace rendering issues effectively. Additionally, adding logging to your application can help identify where problems may originate.
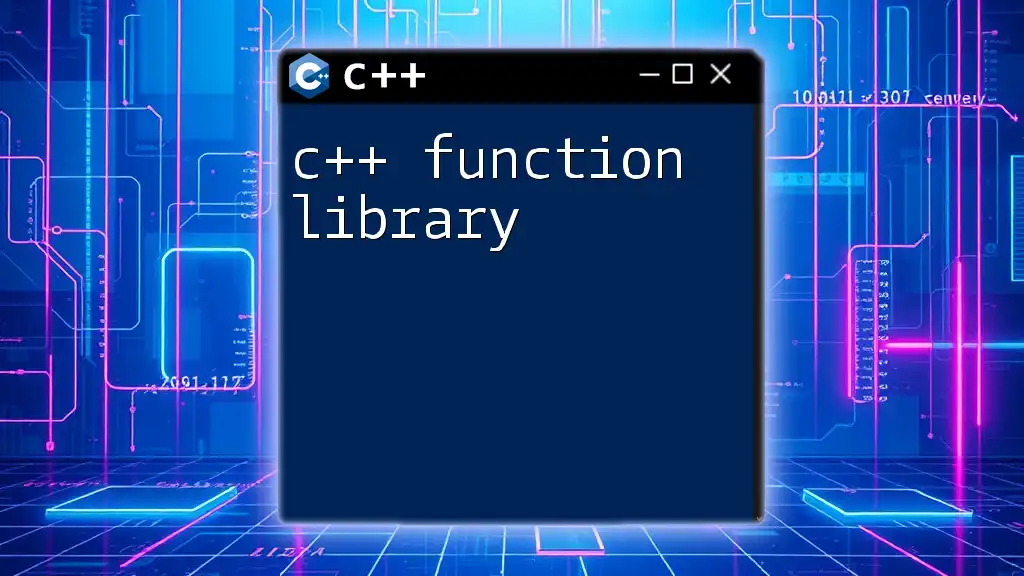
Conclusion
C++ graphics libraries offer developers the tools they need to create stunning graphics, whether in 2D or 3D. These libraries like SDL, SFML, OpenGL, and Allegro provide developers with options that cater to various needs, from simple applications to complex graphics projects.
This article covered the essential aspects of graphics libraries in C++, including popular choices, initial setup, programming examples, and best practices. With this knowledge, you are well-equipped to begin your journey into graphics programming using C++. Embrace the challenge and start exploring these powerful tools to mold your creative visions into reality!
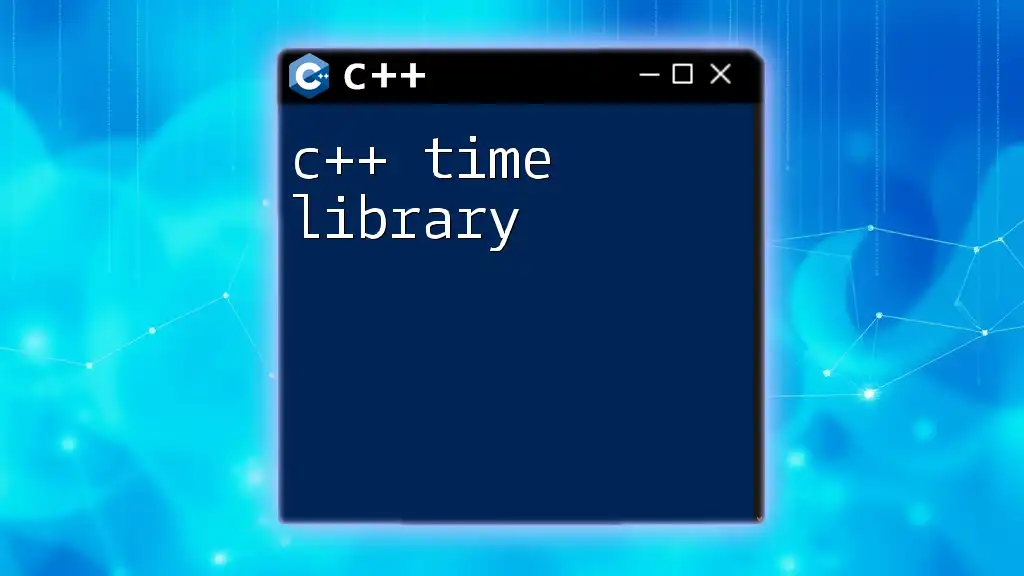
Additional Resources
For deeper learning, consider exploring:
- Official documentation and tutorials for SDL, SFML, and OpenGL.
- Community forums for discussions and troubleshooting in C++ graphics programming.
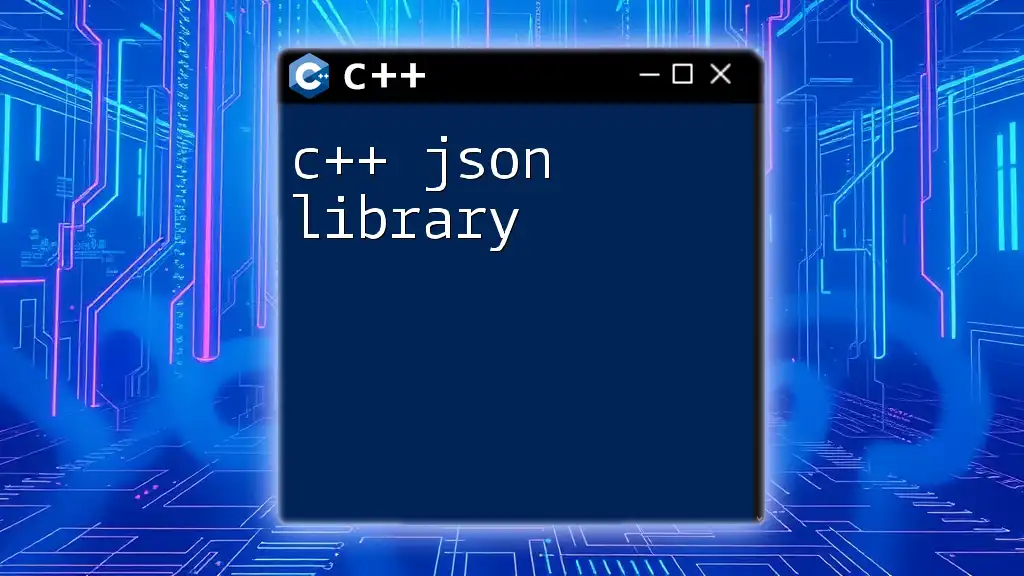
References
Make sure to refer to comprehensive books and articles related to graphics programming in C++ for further insights and advanced techniques. Happy coding!