The C++ Eigen library is a powerful linear algebra library that provides simple yet efficient matrix and vector operations for high-performance computing.
Here's a simple example of using the Eigen library to perform matrix multiplication:
#include <iostream>
#include <Eigen/Dense>
int main() {
Eigen::Matrix2d matA;
Eigen::Matrix2d matB;
matA << 1, 2,
3, 4;
matB << 5, 6,
7, 8;
Eigen::Matrix2d matC = matA * matB;
std::cout << "Result of matrix multiplication:\n" << matC << std::endl;
return 0;
}
What is the Eigen Library?
The C++ Eigen library is a high-performance library for linear algebra, including operations on vectors and matrices. It is designed with a focus on simplicity, offering an intuitive and consistent API that's easy to integrate. Eigen supports a variety of operations, making it ideal for applications in fields such as robotics, computer graphics, physics simulations, and much more.
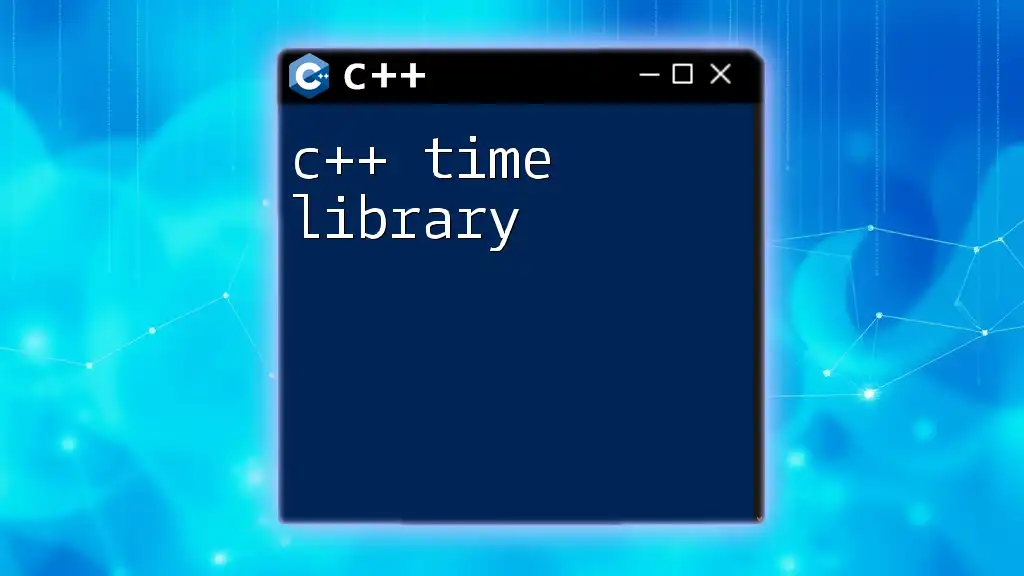
Why Use the Eigen Library?
One of the key reasons to adopt the Eigen library is its high performance. Eigen leverages several advanced optimization techniques, allowing it to efficiently handle large datasets with minimal overhead. Additionally, the library is header-only, which simplifies installation and usage; just include the headers to start working with it.
Use Cases of Eigen in Real-World Applications
Eigen is frequently employed in diverse areas such as:
- Computer Vision: For tasks like image transformation and 3D reconstruction.
- Machine Learning: Especially for implementing algorithms requiring matrix manipulations.
- Physics Engines: To compute vectors and forces in simulations.
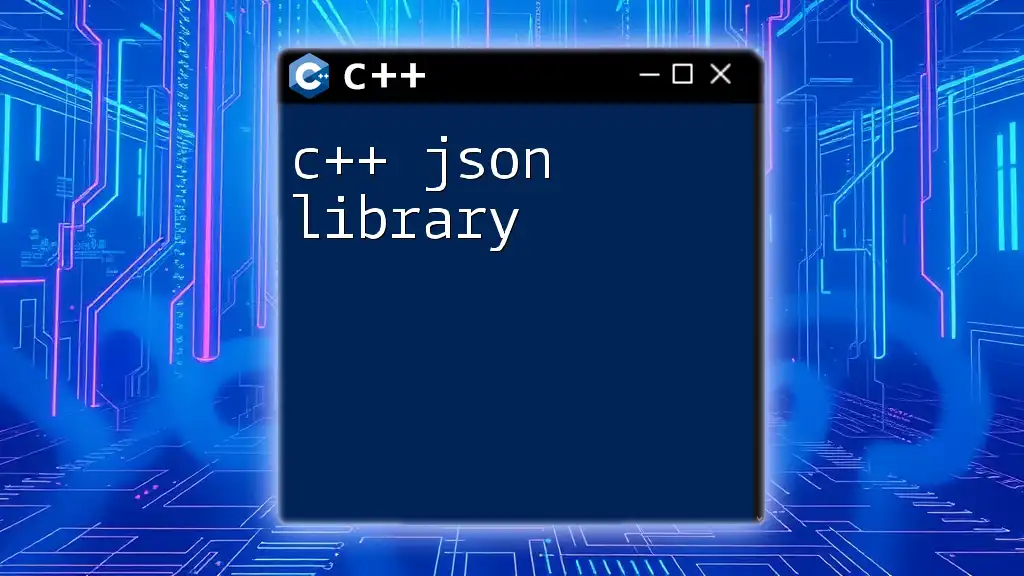
Installation and Setup
To get started with the C++ Eigen library, you first need to download it. You can find the latest version on the [official Eigen website](http://eigen.tuxfamily.org/).
Step-by-Step Guide to Installing Eigen
- Download the Eigen archive from the official site.
- Extract the contents to a directory of your choice.
- Include the Eigen header files in your C++ project like this:
#include <Eigen/Dense>
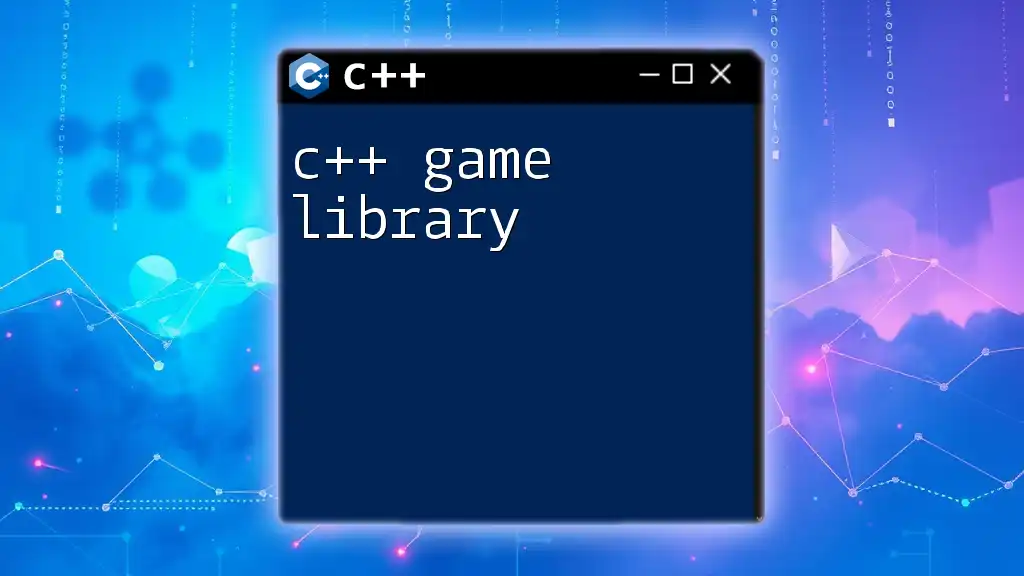
Basic Structure and Organization
Understanding the structure of Eigen can significantly enhance your productivity. It contains several modules categorized into namespaces, such as:
- Eigen::Matrix: For working with matrices.
- Eigen::Vector: For handling vectors, which are a special case of matrices.
- Eigen::Array: For operations that involve arrays rather than matrices or vectors.
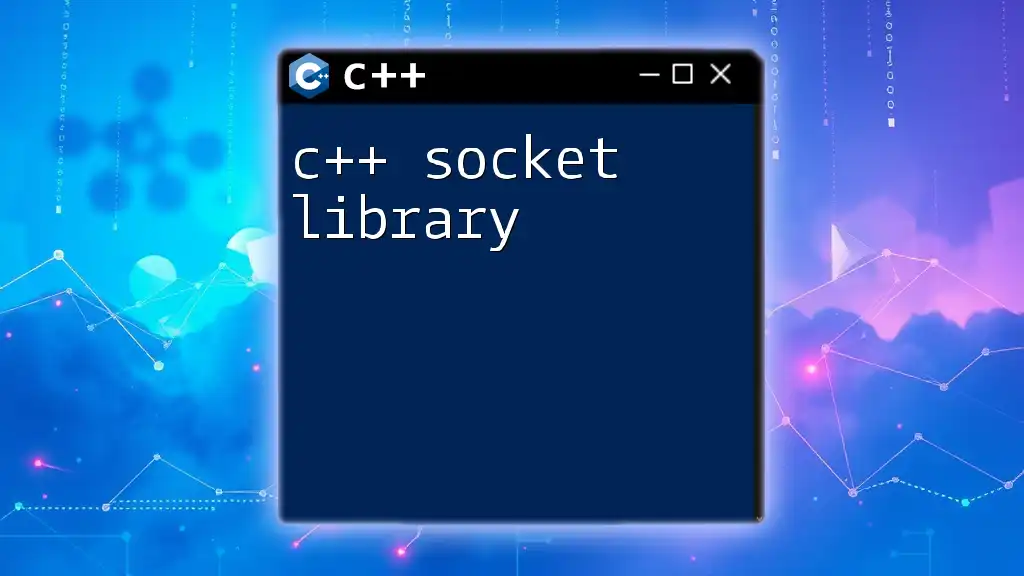
Matrices and Vectors
Creating Matrices and Vectors
Eigen allows for straightforward initialization of matrices and vectors. Here’s how you initialize them:
Eigen::MatrixXd mat(2, 2); // 2x2 matrix of doubles
mat << 1, 2,
3, 4; // Initializing values
Eigen::Vector2d vec; // 2D vector of doubles
vec << 5, 6; // Initializing values
Basic Operations
With Eigen, performing arithmetic operations is as simple as using standard operators:
Eigen::MatrixXd result = mat + mat; // Addition
Eigen::Vector2d difference = vec - vec; // Subtraction
Eigen::MatrixXd product = mat * mat; // Multiplication
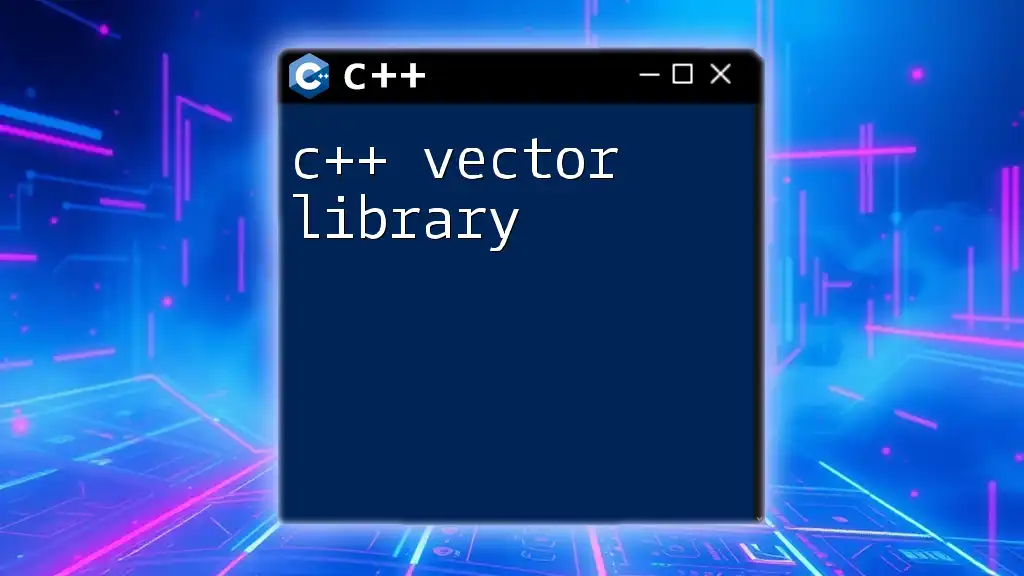
Advanced Matrix Operations
Determinants and Inverses
Eigen also provides functionalities to compute the determinant and inverse of matrices. Here’s how you can do it:
double det = mat.determinant(); // Computing determinant
Eigen::MatrixXd inv = mat.inverse(); // Computing the inverse
Eigenvalues and Eigenvectors
To find the eigenvalues and eigenvectors of a matrix:
Eigen::EigenSolver<Eigen::MatrixXd> solver(mat);
Eigen::VectorXd eigenvalues = solver.eigenvalues().real();
Eigen::MatrixXd eigenvectors = solver.eigenvectors().real();
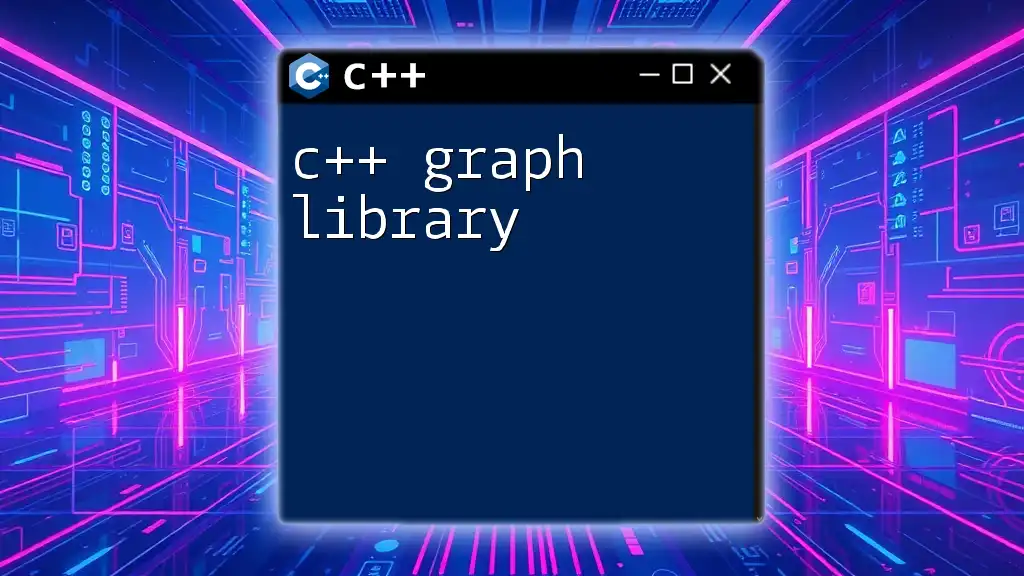
Matrix Decompositions
LU Decomposition
LU decomposition is critical in solving linear equations and can be executed as follows:
Eigen::PartialPivLU<Eigen::MatrixXd> lu_decomp(mat);
Eigen::VectorXd b(2);
b << 1, 2; // Sample right-hand side for Ax = b
Eigen::VectorXd x = lu_decomp.solve(b); // Solving Ax = b
QR and SVD Decomposition
Eigen simplifies QR and Singular Value Decomposition (SVD) processes too:
Eigen::HouseholderQR<Eigen::MatrixXd> qr(mat);
Eigen::MatrixXd qr_result = qr.householderQ() * qr.matrixQR();
Eigen::JacobiSVD<Eigen::MatrixXd> svd(mat);
Eigen::MatrixXd svd_result = svd.singularValues();
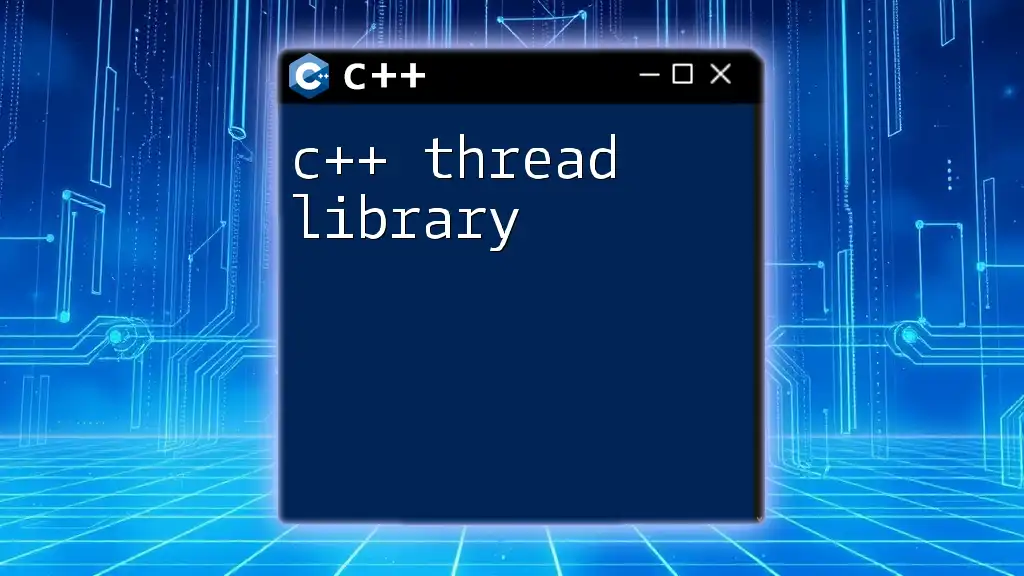
Using Eigen's Convenience Features
Expression Templates
Eigen utilizes expression templates to optimize performance significantly. This means operations are computed at compile-time, which reduces runtime overhead. For example, chaining operations is efficient:
Eigen::MatrixXd result = mat * mat.transpose() + vec * vec.transpose();
Automatic Differentiation
Automatic differentiation capabilities in Eigen allow for reverse-mode operations, which are particularly useful in machine learning applications. This feature is a boon when implementing optimization algorithms.
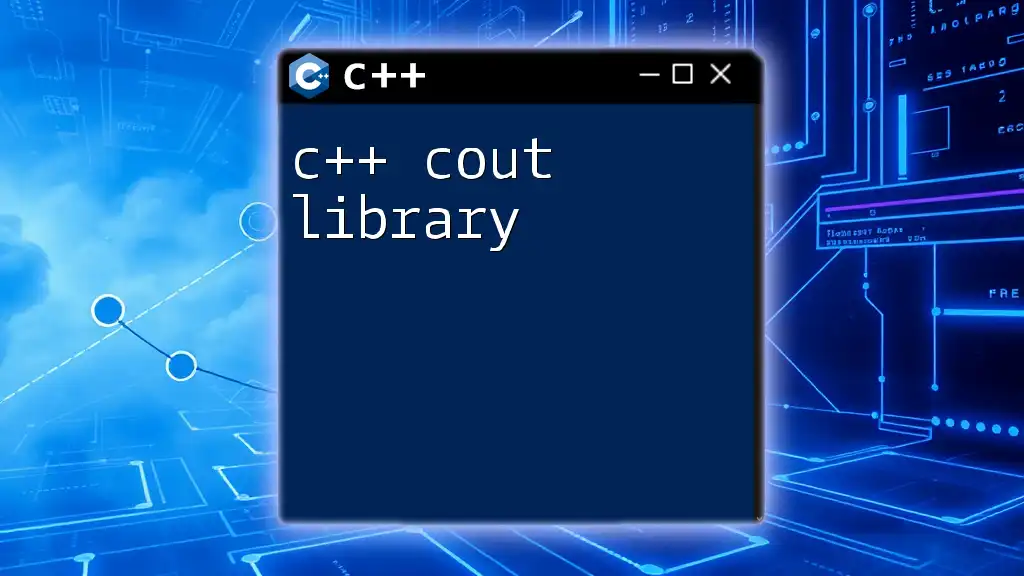
Performance Tips When Using Eigen
Optimization Techniques
To maximize performance when using the Eigen library, consider the following strategies:
- Use fixed-size matrices and vectors whenever possible, as they lead to better optimization opportunities.
- Memory alignment impacts performance; ensure optimal memory layouts when working with large numerical data.
Compile-Time Options
Eigen provides several compile-time configuration options to tweak performance characteristics. You can enable vectorization, for instance, through configuration defines:
#define EIGEN_USE_BLAS
#define EIGEN_USE_LAPACK
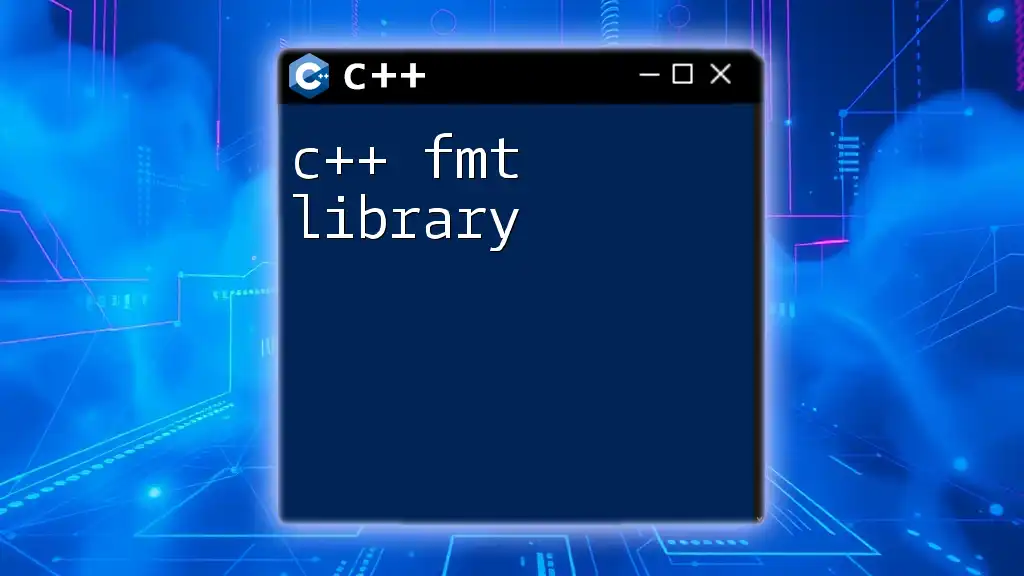
Practical Examples Using Eigen
Example: Linear Regression
Implementing linear regression is straightforward with Eigen. Below is a simple implementation:
Eigen::MatrixXd X; // Feature matrix
Eigen::VectorXd y; // Target vector
Eigen::MatrixXd beta = (X.transpose() * X).inverse() * X.transpose() * y; // Calculating coefficients
Example: Solving Linear Systems
You can quickly solve linear systems using Eigen's solvers:
Eigen::MatrixXd A; // Coefficient matrix
Eigen::VectorXd b; // Right-hand side vector
Eigen::VectorXd x = A.lu().solve(b); // Solve Ax = b
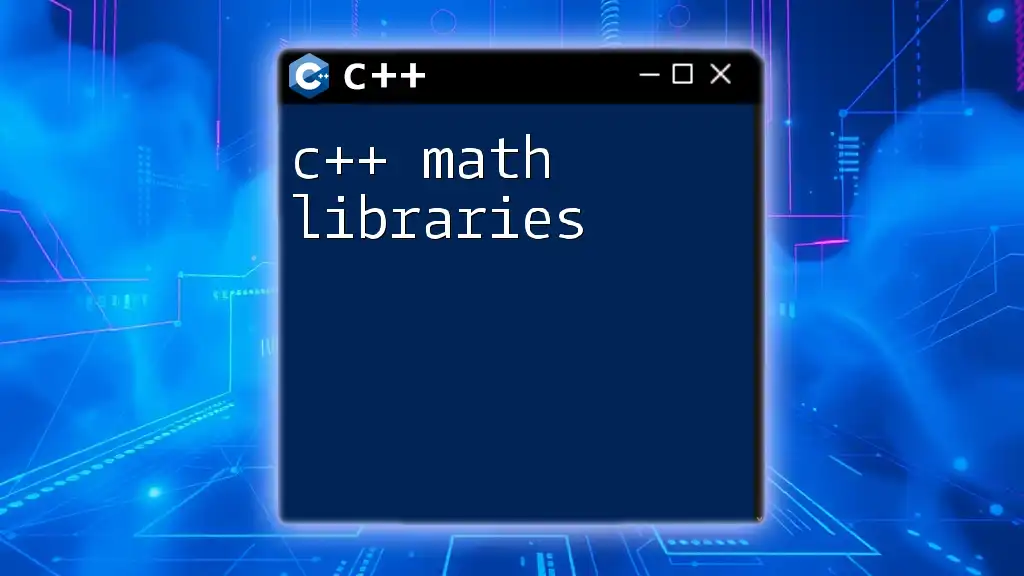
Common Pitfalls and How to Avoid Them
When using the C++ Eigen library, pay attention to:
- Matrix dimensions: Ensure dimensions are compatible when performing operations or you will encounter runtime errors.
- Using the wrong precision: For instance, using `float` instead of `double` can lead to numerical inaccuracies in calculations.
Best Practices
- Write clear code with comments to maintain readability.
- Use assertions to check matrix sizes before performing operations.
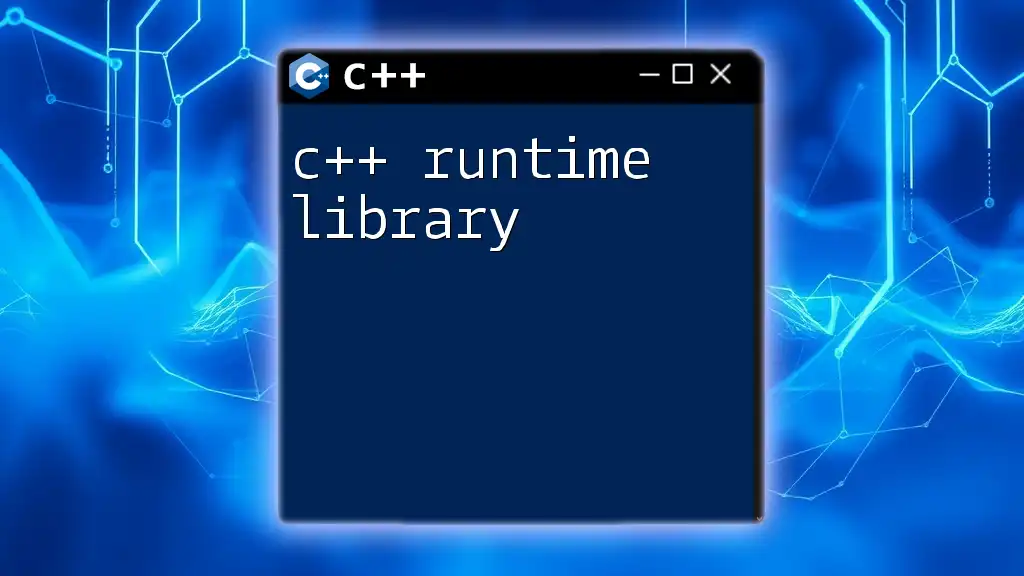
Conclusion
The C++ Eigen library stands as a powerful ally for developers needing efficient and robust linear algebra capabilities. With a user-friendly interface, extensive documentation, and high performance, Eigen is worth mastering for any C++ programmer.
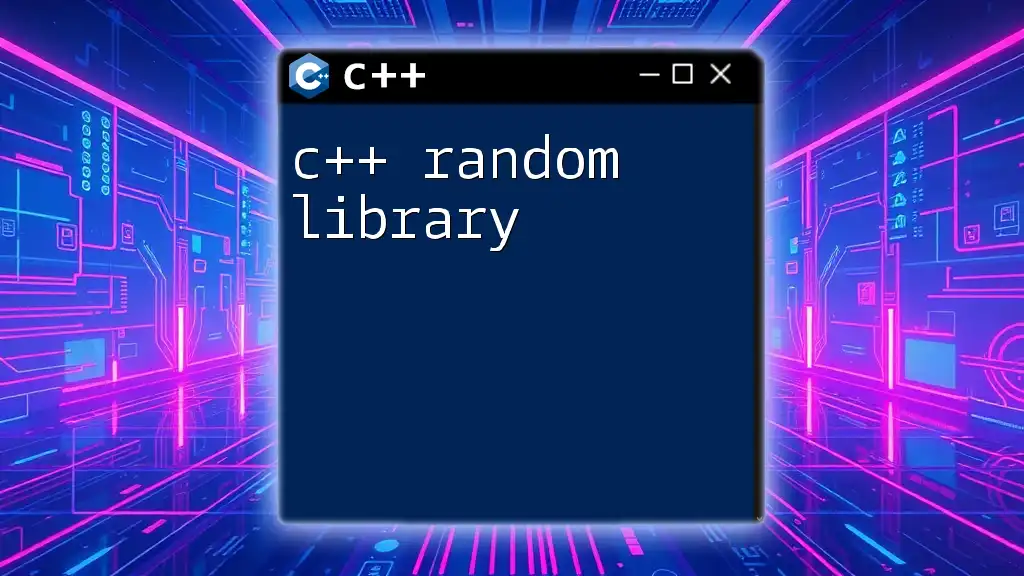
Further Resources for Learning Eigen
To deepen your understanding of Eigen, consider exploring:
- The [official Eigen documentation](http://eigen.tuxfamily.org/dox/index.html)
- Online courses focused on numerical methods and algorithms
- Community forums and GitHub repositories for practical insights and real-world applications.
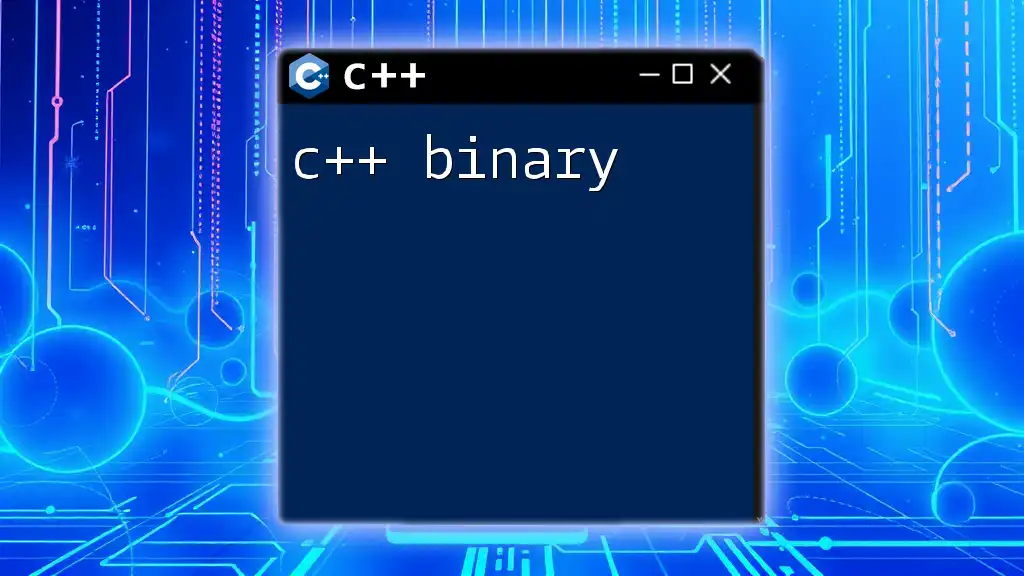
Encouragement to Start Using Eigen
The vast potential of the C++ Eigen library is just a few lines of code away. Start exploring its features today and elevate your C++ projects with advanced linear algebra capabilities!
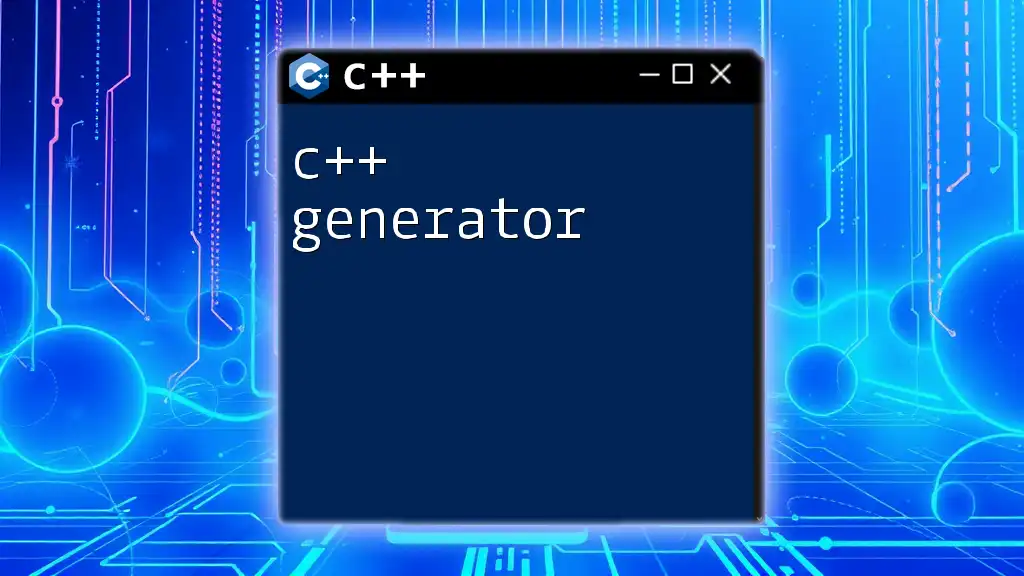
Additional Code Snippets and Examples
Feel free to refer to the code snippets provided in this guide as a reference to kickstart your journey with Eigen!
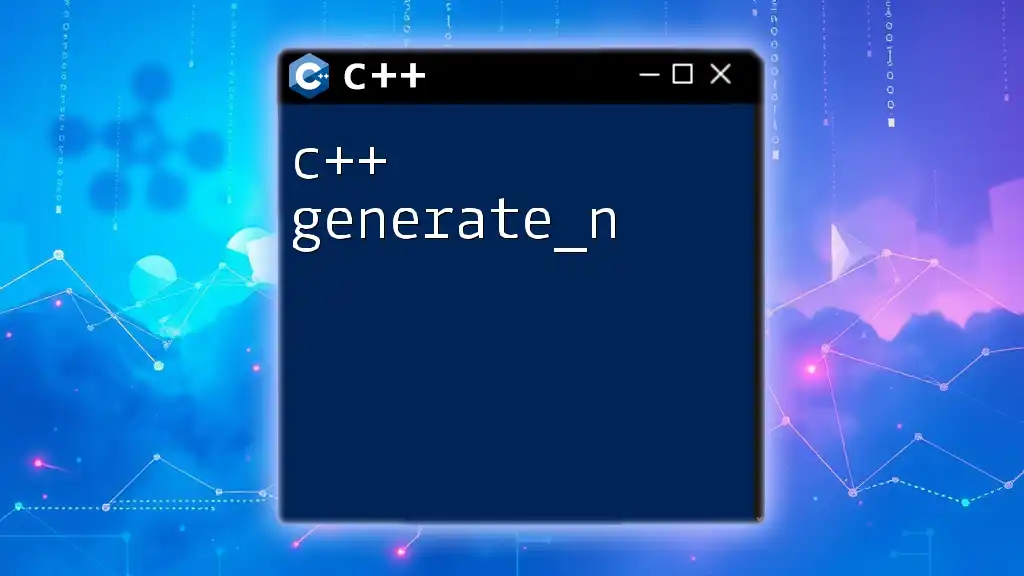
Frequently Asked Questions
If you have further inquiries or need clarification about using the Eigen library, consult the FAQs or visit community forums dedicated to C++ and Eigen-specific discussions.