A C++ WebSocket library enables real-time, bidirectional communication between clients and servers over the WebSocket protocol, allowing for efficient data exchange.
Here's a simple example using the WebSocket++ library:
#include <websocketpp/config/asio_no_tls.hpp>
#include <websocketpp/server.hpp>
typedef websocketpp::server<websocketpp::config::asio> server;
void on_message(websocketpp::connection_hdl hdl, server::message_ptr msg) {
std::cout << "Received: " << msg->get_payload() << std::endl;
}
int main() {
server echo_server;
echo_server.set_message_handler(bind(&on_message, ::_1, ::_2));
echo_server.init_asio();
echo_server.set_reuse_addr(true);
echo_server.listen(9002);
echo_server.start_accept();
echo_server.run();
}
Understanding WebSocket Protocol
WebSockets are a communication protocol that provides full-duplex communication channels over a single TCP connection. The significance of WebSocket lies in its capability to enable real-time exchanges between client and server, making it a perfect fit for applications requiring instant data updates, such as chat applications and online gaming.
Advantages of WebSockets over traditional HTTP protocols include:
- Low Latency: WebSockets maintain an open connection, reducing the delay in message delivery.
- Full-duplex Communication: Both client and server can send messages independently at any time.
- Resource Efficiency: WebSockets require fewer resources than repeatedly opening and closing HTTP connections.
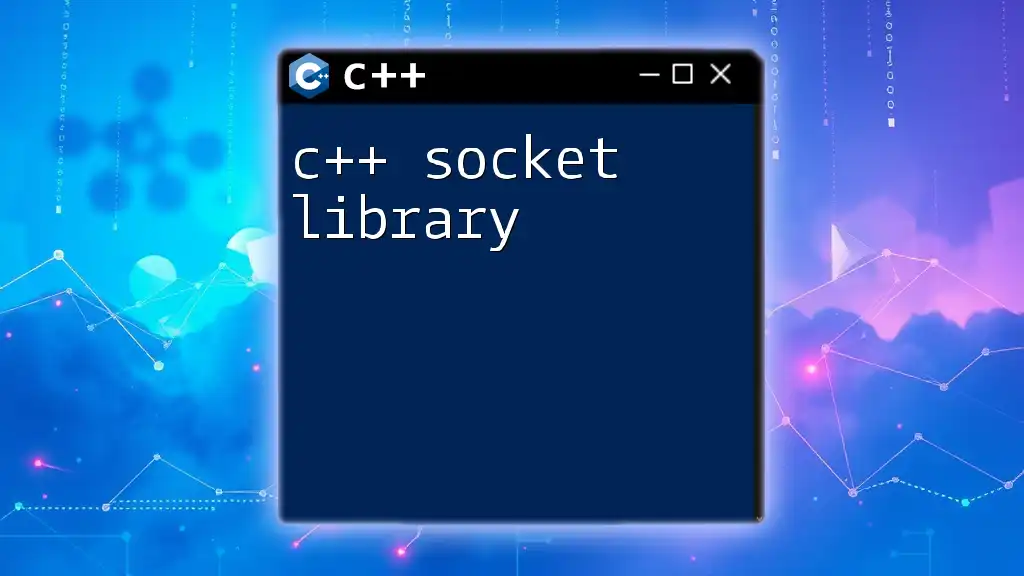
Use Cases for C++ WebSocket
C++ WebSocket libraries find applications in various domains:
- Real-time applications: WebSockets enable instant messaging in chat applications or multiplayer games, ensuring smooth interaction among users.
- Streaming Data Processing: Applications that require continuous data flow, such as stock price updates or sensor data from IoT devices, benefit from WebSockets.
- IoT Devices Communication: WebSockets facilitate communication between various IoT devices and central servers, making data transfer efficient and responsive.
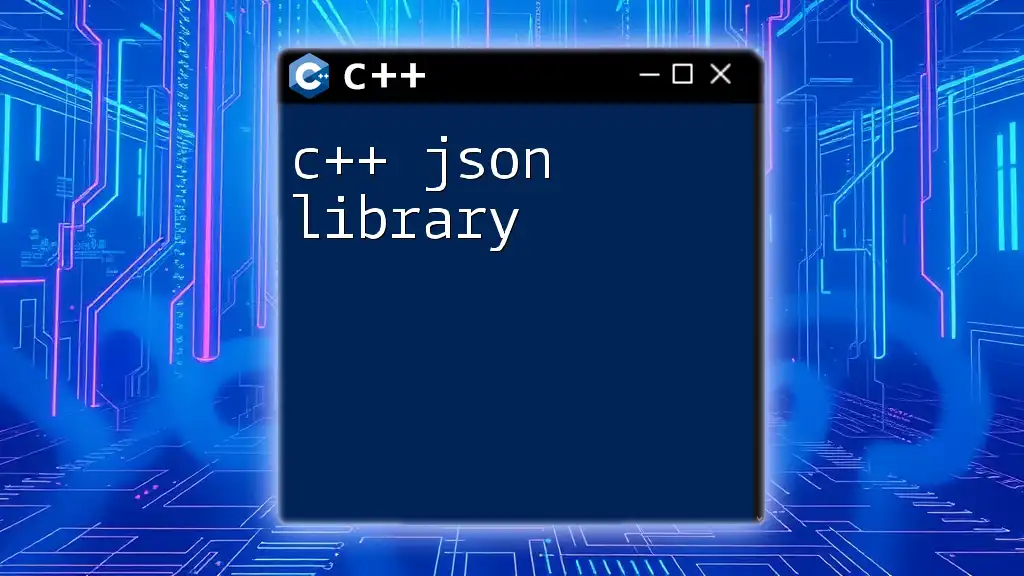
Choosing the Right C++ WebSocket Library
With several libraries available for implementing WebSockets in C++, it is crucial to choose one that fits your project requirements. Here are some of the most popular libraries:
WebSocket++
WebSocket++ is a standalone C++ library enabling WebSocket client and server functionalities. It is lightweight and easy to integrate into existing applications.
- Main Features:
- Supports various transport protocols (TCP/SSL).
- Asynchronous and synchronous modes of operation.
- Extensible for custom application protocols.
Boost.Beast
Part of the Boost C++ Libraries, Boost.Beast provides both HTTP and WebSocket functionality and is ideal for users familiar with Boost.
- Advantages:
- High-performance and powerful tools for HTTP and WebSocket handling.
- Strong documentation and community support.
- Fits seamlessly with other Boost components.
Qt WebSockets
For developers already using the Qt framework, Qt WebSockets provides a straightforward and reliable way to implement WebSockets.
- Benefits:
- Built-in integration with Qt applications.
- Easy to use with Qt's existing event loop framework.
- Comprehensive documentation available for Qt users.
Factors to Consider When Choosing
When selecting a C++ WebSocket library, consider the following:
- Ease of Use: Choose a library that simplifies development and minimizes setup time.
- Performance and Scalability: Evaluate the library's ability to handle high load and multiple connections simultaneously.
- Community and Documentation Resources: A well-documented library with an active community ensures that you can find support and solutions to potential issues quickly.
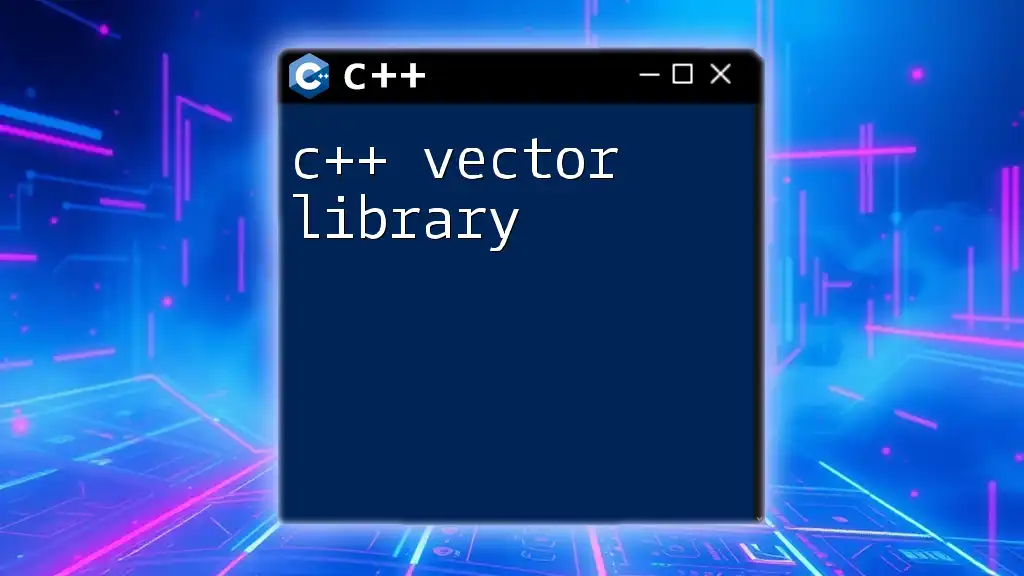
Getting Started with C++ WebSocket Library
Installation Process
WebSocket++
To install WebSocket++, you will follow these steps:
- Clone the WebSocket++ repository from GitHub.
- Configure CMake to include the library in your project.
Here’s an example of a basic project setup using CMake:
cmake_minimum_required(VERSION 3.5)
project(WebSocketExample)
find_package(Boost REQUIRED)
include_directories(${Boost_INCLUDE_DIRS} ${CMAKE_SOURCE_DIR}/path/to/websocketpp)
add_executable(WebSocketClient main.cpp)
target_link_libraries(WebSocketClient ${Boost_LIBRARIES})
Boost.Beast
To set up Boost.Beast:
- Ensure you have the Boost library installed.
- Include the necessary Boost headers in your project.
Example of setting up a Boost project with WebSockets:
cmake_minimum_required(VERSION 3.5)
project(BoostWebSocketExample)
find_package(Boost REQUIRED COMPONENTS system thread)
include_directories(${Boost_INCLUDE_DIRS})
add_executable(BoostWebSocket main.cpp)
target_link_libraries(BoostWebSocket ${Boost_LIBRARIES})
Qt WebSockets
For Qt WebSockets:
- Make sure Qt is installed.
- Include the WebSockets module in your .pro file.
Example:
QT += websockets
SOURCES += main.cpp
Setting Up Your First WebSocket Client
To create your first WebSocket client with WebSocket++, follow these instructions. Here's a complete example of a basic WebSocket client implementation:
#include <websocketpp/config/asio_no_tls.hpp>
#include <websocketpp/client.hpp>
typedef websocketpp::client<websocketpp::config::asio_client> client;
class websocket_client {
public:
websocket_client() {
m_endpoint.init_asio();
m_endpoint.set_open_handler(bind(&websocket_client::on_open, this, ::_1));
m_endpoint.set_close_handler(bind(&websocket_client::on_close, this, ::_1));
m_endpoint.set_message_handler(bind(&websocket_client::on_message, this, ::_1, ::_2));
}
void run(std::string uri) {
websocketpp::lib::error_code ec;
client::connection_ptr con = m_endpoint.get_connection(uri, ec);
m_endpoint.connect(con);
m_endpoint.run();
}
private:
void on_open(websocketpp::connection_hdl hdl) {
std::cout << "WebSocket connection opened!" << std::endl;
}
void on_close(websocketpp::connection_hdl hdl) {
std::cout << "WebSocket connection closed!" << std::endl;
}
void on_message(websocketpp::connection_hdl, client::message_ptr msg) {
std::cout << "Message received: " << msg->get_payload() << std::endl;
}
client m_endpoint;
};
Running the WebSocket Client
To compile and run the client:
- Compile your program using the build tools configured in your project.
- Execute the client and pass the WebSocket URI as an argument.
- Expected Output: Upon connecting, the client should print confirmation messages indicating successful connection and incoming messages when a server responds.
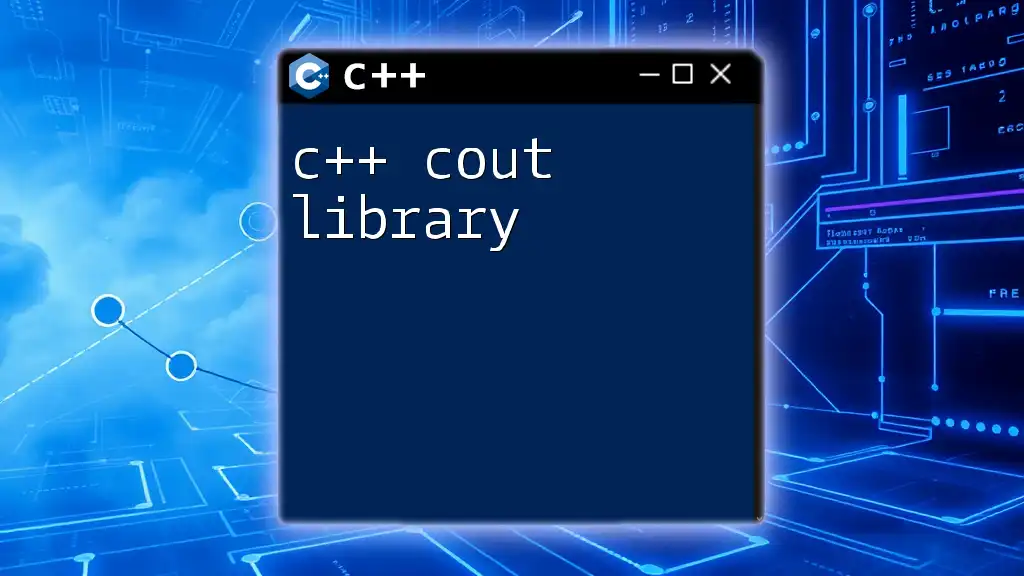
Building a WebSocket Server in C++
Creating a WebSocket server allows your application to handle incoming connections and distribute messages to clients. Here’s an overview of a simple WebSocket server using WebSocket++.
Server Implementation Overview
A WebSocket server listens for connections, accepts, and manages client communications. The server receives and processes messages, offering services like message broadcasting or echoing messages back to clients.
Code Snippet: Basic Server Implementation
#include <websocketpp/config/asio_no_tls.hpp>
#include <websocketpp/server.hpp>
typedef websocketpp::server<websocketpp::config::asio> server;
class websocket_server {
public:
websocket_server() {
m_server.init_asio();
m_server.set_open_handler(bind(&websocket_server::on_open, this, ::_1));
m_server.set_close_handler(bind(&websocket_server::on_close, this, ::_1));
m_server.set_message_handler(bind(&websocket_server::on_message, this, ::_1, ::_2));
}
void start(uint16_t port) {
m_server.set_reuse_addr(true);
m_server.listen(port);
m_server.start_accept();
m_server.run();
}
private:
void on_open(websocketpp::connection_hdl hdl) {
std::cout << "New client connected!" << std::endl;
}
void on_close(websocketpp::connection_hdl hdl) {
std::cout << "Client disconnected!" << std::endl;
}
void on_message(websocketpp::connection_hdl hdl, server::message_ptr msg) {
std::cout << "Message from client: " << msg->get_payload() << std::endl;
m_server.send(hdl, "Echo: " + msg->get_payload(), websocketpp::frame::opcode::text);
}
server m_server;
};
Running the WebSocket Server
To run the server:
- Compile the server code.
- Run the server on a specified port.
- Expected Interactions: Test the server by connecting a client and sending messages. The server should echo back any received message.
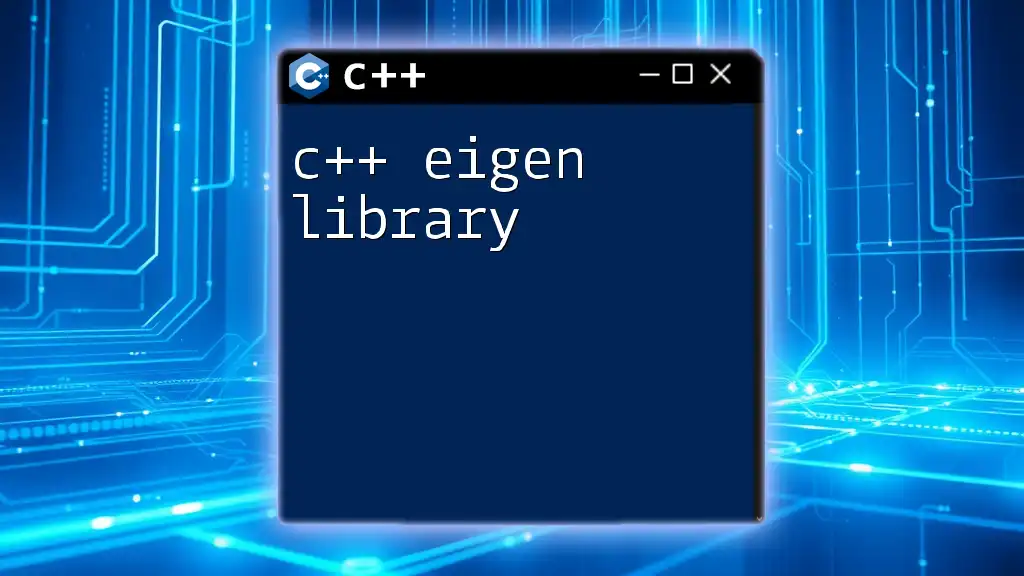
Advanced WebSocket Features
Handling Binary Data
In addition to text messages, WebSockets can manage binary data. This feature is essential for applications like image sharing or video streaming. When sending binary data, ensure the correct `opcode` is set to facilitate proper processing by clients.
Example of handling binary messages:
void on_message(websocketpp::connection_hdl, server::message_ptr msg) {
if (msg->get_opcode() == websocketpp::frame::opcode::binary) {
std::cout << "Binary message received!" << std::endl;
} else {
std::cout << "Text message received: " << msg->get_payload() << std::endl;
}
}
Security Considerations
Securing WebSocket communications is vital, especially for applications handling sensitive data. Here are key points to consider:
- WSS Protocol: Use the secure WebSocket protocol (WSS) to encrypt data in transit.
- SSL/TLS Implementation: Ensure that your server uses SSL/TLS to authenticate and encrypt connections.
Integrating SSL into your WebSocket server generally involves using a suitable library to manage certificates and encryption protocols, such as OpenSSL.
Scaling WebSocket Applications
To efficiently manage numerous connections and maintain performance, consider the following:
- Horizontal Scaling: Distribute the load across several servers using a load balancer.
- Vertical Scaling: Increase the hardware specifications (CPU, memory) of your existing infrastructure.
Adopting these strategies enhances your WebSocket application’s performance and ability to handle increasing user volumes.
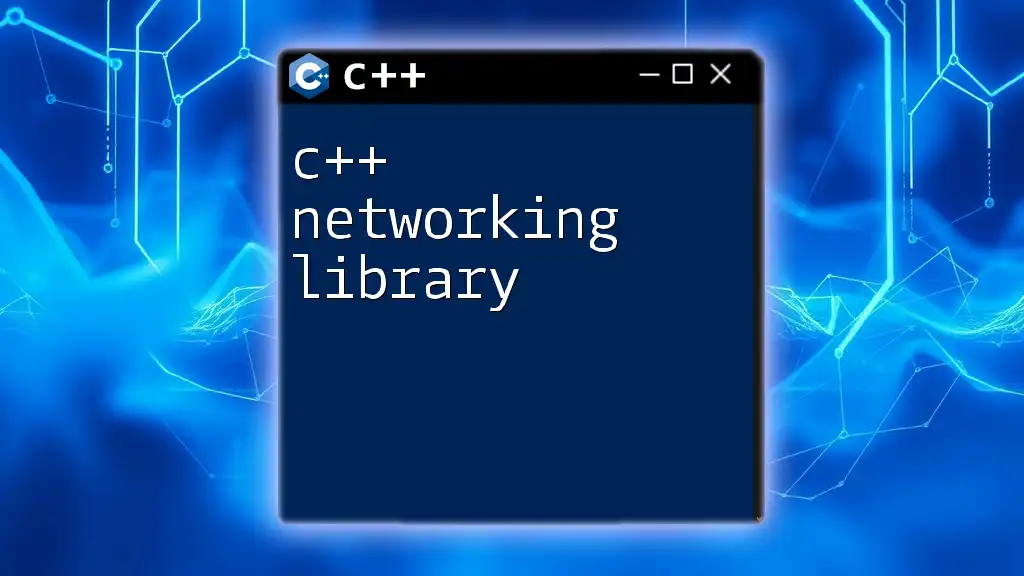
Debugging and Testing WebSockets in C++
Common Issues and Solutions
Common issues arise during the WebSocket connection phase:
- Connection Refused: Verify server availability and that it's listening on the expected port.
- Timeouts: Investigate network configurations to eliminate latency issues.
Testing with WebSocket Clients
Various tools exist for testing WebSocket servers and clients:
- Postman: Allows you to send and receive messages over WebSocket connections.
- WebSocket Client Apps: Dedicated applications available across platforms (e.g., Smart Websocket Client for Chrome).
Automate testing by simulating multiple connections to gauge the server's performance and ensure it handles various scenarios gracefully.
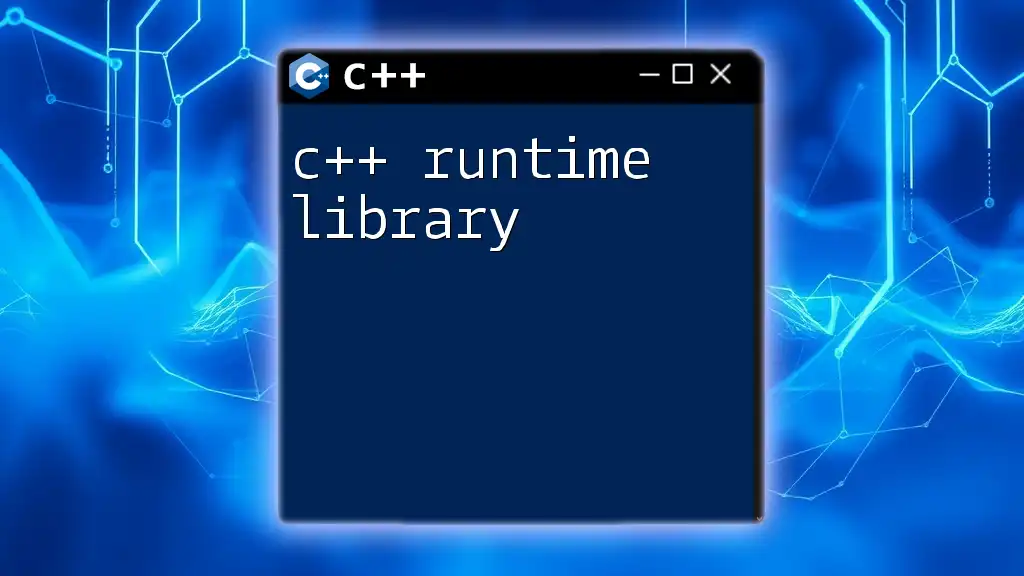
Conclusion
In summary, leveraging a C++ WebSocket library significantly boosts your ability to develop real-time applications. Whether you adopt WebSocket++, Boost.Beast, or Qt WebSockets, the capability for seamless communication is at your fingertips.
As you explore these libraries, experiment with the example code provided and begin building your own applications. The future of interactive and responsive software is right here with WebSocket technology!