A C++ networking library simplifies the process of implementing network protocols and communications in C++ applications, allowing developers to easily send and receive data over the network.
Here’s a simple example using the Boost.Asio library for asynchronous network communication:
#include <boost/asio.hpp>
#include <iostream>
using boost::asio::ip::tcp;
int main() {
boost::asio::io_context io_context;
tcp::resolver resolver(io_context);
tcp::resolver::results_type endpoints = resolver.resolve("example.com", "80");
tcp::socket socket(io_context);
boost::asio::connect(socket, endpoints);
// Send an HTTP request
const std::string request = "GET / HTTP/1.1\r\nHost: example.com\r\n\r\n";
boost::asio::write(socket, boost::asio::buffer(request));
// Read the response
boost::asio::streambuf response;
boost::asio::read_until(socket, response, "\r\n");
std::cout << "Response received:\n";
std::cout << &response;
return 0;
}
Understanding C++ Network Libraries
What is a C++ Networking Library?
A C++ networking library is a collection of pre-written code that simplifies the process of implementing network communication within C++ applications. These libraries abstract the complexities of socket programming, making it easier for developers to send and receive data over networks. By leveraging a networking library, you can:
- Save time spent on lower-level socket programming.
- Streamline your networking code, making it more readable and maintainable.
- Focus on functionality rather than the intricacies of network protocols.
When selecting a C++ networking library, consider factors such as performance, ease of use, community support, and available features.
Popular C++ Networking Libraries
Boost.Asio
Boost.Asio is part of the larger Boost library collection and provides a framework for asynchronous I/O. It is known for its high performance and versatility. Key features of Boost.Asio include:
- Cross-platform support for different operating systems.
- Synchronization and signal handling for efficient I/O operations.
- Support for both TCP and UDP, providing flexibility as per application requirements.
Here's a basic code example to demonstrate asynchronous networking with Boost.Asio:
#include <iostream>
#include <boost/asio.hpp>
void async_connect(boost::asio::ip::tcp::socket& socket, const std::string& host, const std::string& service) {
boost::asio::ip::tcp::resolver resolver(socket.get_executor());
boost::asio::ip::tcp::resolver::results_type endpoints = resolver.resolve(host, service);
boost::asio::async_connect(socket, endpoints,
[](const boost::system::error_code& error, const boost::asio::ip::tcp::endpoint&) {
if (!error) {
std::cout << "Connected successfully!" << std::endl;
} else {
std::cout << "Error in connection: " << error.message() << std::endl;
}
});
socket.get_executor().context().run(); // Run the context to process events
}
POCO C++ Libraries
POCO stands for "POrtable COmponents" and offers a variety of libraries, including networking capabilities. Its main advantages include:
- High-level abstractions for developing network applications quickly.
- Built-in support for creating RESTful web services and HTTP servers.
- Simplicity in setup and deployment.
Here's an example of creating a simple HTTP server using POCO:
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPServerRequest.h>
#include <Poco/Net/HTTPServerResponse.h>
#include <Poco/Net/HTTPRequestHandler.h>
#include <Poco/Net/ServerSocket.h>
#include <Poco/Thread.h>
class HelloWorldRequestHandler : public Poco::Net::HTTPRequestHandler {
public:
void handleRequest(Poco::Net::HTTPServerRequest& request, Poco::Net::HTTPServerResponse& response) {
response.setContentType("text/plain");
response.setStatus(Poco::Net::HTTPResponse::HTTP_OK);
std::ostream& out = response.send();
out << "Hello, World!";
}
};
void startServer() {
Poco::Net::HTTPServer srv(new Poco::Net::HTTPRequestHandlerFactoryImpl<HelloWorldRequestHandler>(), 8080);
srv.start();
std::cout << "HTTP Server is running on port 8080\nPress Ctrl+C to stop." << std::endl;
Poco::Thread::sleep(Poco::Timespan(10, 0)); // Keep server running for demonstration
srv.stop();
}
Qt Network
Qt Network is part of the Qt framework that provides a comprehensive set of classes for network programming, including TCP and UDP support. Its strengths lie in:
- Signals and slots mechanism that simplifies event-driven programming.
- Extensive and documented API.
- Built-in classes for both high-level and low-level networking.
Here's an example of a TCP client with Qt:
#include <QCoreApplication>
#include <QTcpSocket>
#include <QTextStream>
class MyClient : public QObject {
Q_OBJECT
public:
MyClient() {
QTcpSocket* socket = new QTcpSocket(this);
connect(socket, &QTcpSocket::connected, this, [&]() {
QTextStream(stdout) << "Connected to server" << endl;
socket->write("Hello Server!");
});
connect(socket, &QTcpSocket::readyRead, this, [&]() {
QTextStream(stdout) << "Received from server: " << socket->readAll() << endl;
});
socket->connectToHost("localhost", 8080);
}
};
int main(int argc, char *argv[]) {
QCoreApplication app(argc, argv);
MyClient client;
return app.exec();
}
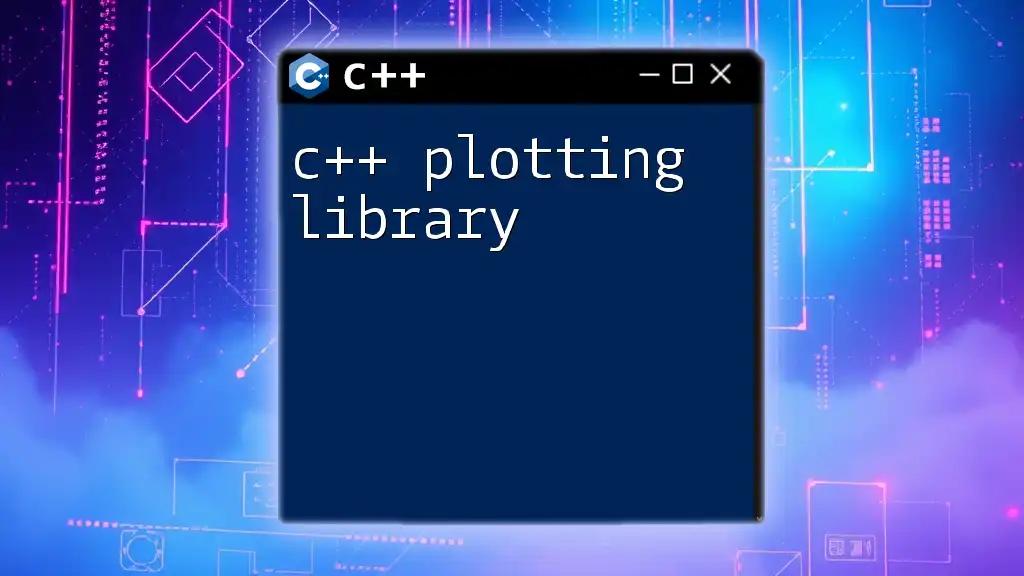
Setting Up Your C++ Networking Environment
Prerequisites
Before diving into C++ networking, ensure you have a suitable development environment. The essential tools include:
- A modern C++ compiler (GCC, Clang, or MSVC).
- An IDE (like Visual Studio, Code::Blocks, or Visual Studio Code).
- Access to the internet for downloading libraries and dependencies.
Installing C++ Networking Libraries
The installation process varies based on the library choice:
- Boost.Asio: Install Boost and include the relevant headers in your project.
- POCO Libraries: Download and compile POCO libraries or use a package manager like vcpkg.
- Qt: Install the Qt SDK, which includes the networking module.
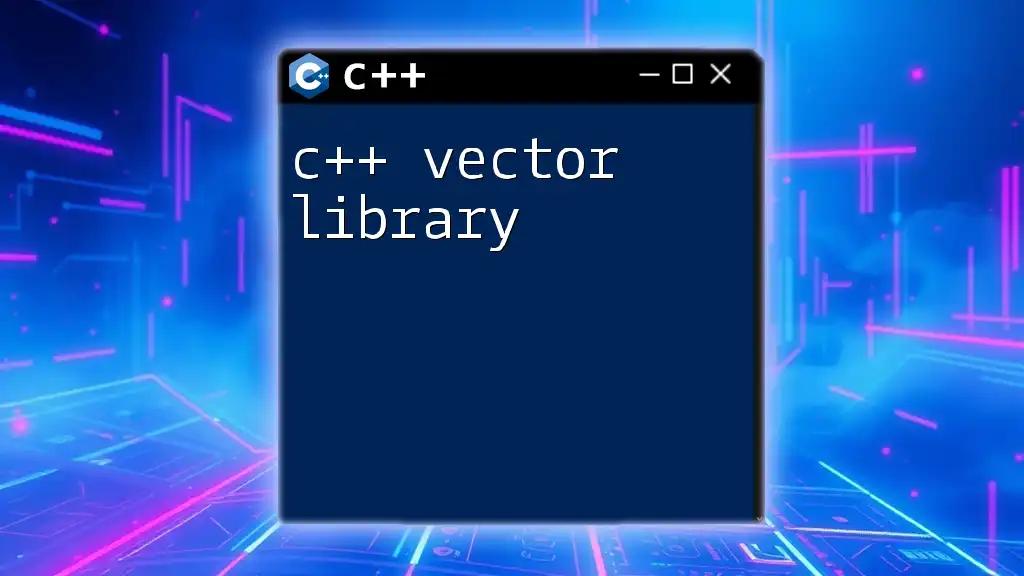
Getting Started with C++ Networking
Basic Networking Concepts
Socket Programming Basics
Sockets serve as endpoints for sending and receiving data in a network. Here are the crucial points to consider:
- TCP (Transmission Control Protocol): Reliable, connection-oriented communication.
- UDP (User Datagram Protocol): Connectionless communication suitable for fast data transfer.
A simple implementation of a basic TCP socket can be done as follows:
#include <iostream>
#include <boost/asio.hpp>
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::socket socket(io_context);
boost::asio::ip::tcp::endpoint endpoint(boost::asio::ip::address::from_string("127.0.0.1"), 8080);
socket.connect(endpoint);
std::cout << "Connected to the server!" << std::endl;
return 0;
}
Client-Server Model
Understanding the client-server model is vital for networking. In this model, the client initiates requests, and the server responds. Below is an example of a basic echo server and client.
Echo Server Using Boost.Asio:
#include <boost/asio.hpp>
#include <iostream>
void session(boost::asio::ip::tcp::socket socket) {
for (;;) {
char data[1024];
boost::system::error_code error;
size_t len = socket.read_some(boost::asio::buffer(data), error);
if (error == boost::asio::error::eof) {
break; // Connection closed cleanly
} else if (error) {
throw boost::system::system_error(error); // Some other error
}
boost::asio::write(socket, boost::asio::buffer(data, len)); // Echo back
}
}
Echo Client:
#include <boost/asio.hpp>
#include <iostream>
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::socket socket(io_context);
boost::asio::ip::tcp::resolver resolver(io_context);
auto endpoints = resolver.resolve("127.0.0.1", "8080");
boost::asio::connect(socket, endpoints);
const std::string msg = "Hello, Echo Server!";
boost::asio::write(socket, boost::asio::buffer(msg));
char reply[1024];
size_t reply_length = boost::asio::read(socket, boost::asio::buffer(reply, msg.size()));
std::cout << "Reply from server: ";
std::cout.write(reply, reply_length);
return 0;
}
Making Your First Network Request
Using Boost.Asio, you can make a simple GET request. Here’s a code example:
#include <boost/asio.hpp>
#include <iostream>
void make_get_request(const std::string& server, const std::string& path) {
boost::asio::io_context io_context;
boost::asio::ip::tcp::socket socket(io_context);
boost::asio::ip::tcp::resolver resolver(io_context);
auto endpoints = resolver.resolve(server, "80");
boost::asio::connect(socket, endpoints);
std::string request = "GET " + path + " HTTP/1.0\r\nHost: " + server + "\r\n\r\n";
boost::asio::write(socket, boost::asio::buffer(request));
char reply[1024];
boost::system::error_code error;
size_t len = socket.read_some(boost::asio::buffer(reply), error);
if (error == boost::asio::error::eof) {
std::cout << "Connection closed by server." << std::endl;
} else if (error) {
throw boost::system::system_error(error);
}
std::cout << "Response: ";
std::cout.write(reply, len);
}
Handling Responses and Errors in Network Requests
Properly handling responses and errors is crucial in networking applications. Always implement checks for network errors, read timeouts, and connection closures to ensure a smooth user experience. Using try-catch blocks around I/O operations can help manage errors effectively.
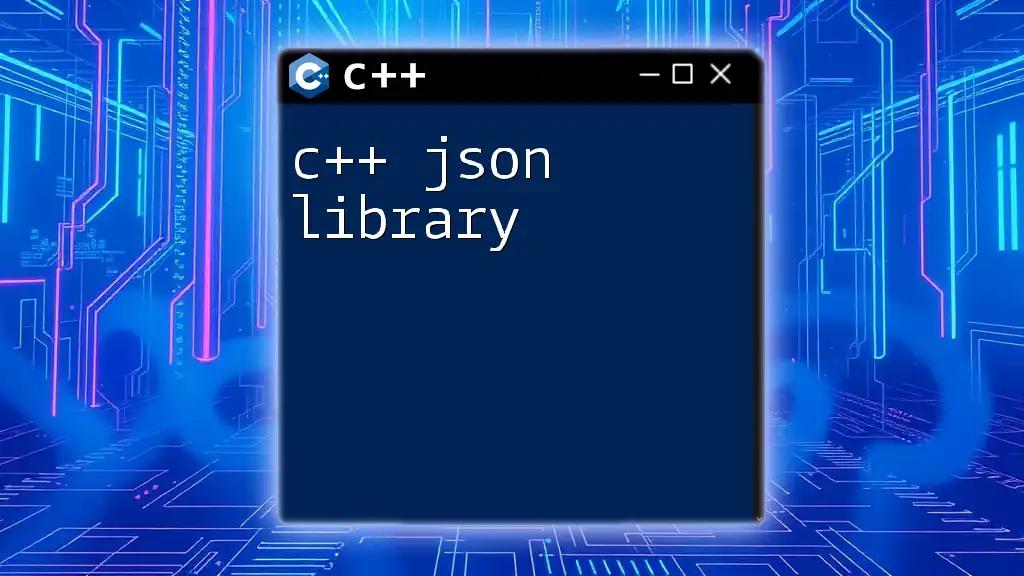
Advanced Networking Techniques
Asynchronous Networking
Asynchronous programming is a powerful feature of C++ networking, allowing you to manage multiple simultaneous operations without blocking the application. This approach significantly enhances performance and responsiveness.
Here’s a code example demonstrating asynchronous TCP client functionality in Boost.Asio:
void async_read(boost::asio::ip::tcp::socket& socket) {
char buffer[1024];
boost::asio::async_read(socket, boost::asio::buffer(buffer),
[&](const boost::system::error_code& error, std::size_t bytes_transferred) {
if (!error) {
std::cout << "Received: " << std::string(buffer, bytes_transferred) << std::endl;
} else {
std::cerr << "Read error: " << error.message() << std::endl;
}
});
}
Multithreading in Networking
Using multiple threads can help optimize your server's responsiveness and scalability. When implementing multithreading, consider thread safety principles. Here's an example of a thread-safe TCP server:
#include <iostream>
#include <thread>
#include <boost/asio.hpp>
void handle_client(boost::asio::ip::tcp::socket socket) {
try {
char buffer[1024];
while (true) {
boost::system::error_code error;
size_t len = socket.read_some(boost::asio::buffer(buffer), error);
if (error) throw boost::system::system_error(error);
boost::asio::write(socket, boost::asio::buffer(buffer, len));
}
} catch (std::exception& e) {
std::cerr << "Exception in thread: " << e.what() << std::endl;
}
}
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::acceptor acceptor(io_context, {boost::asio::ip::tcp::v4(), 8080});
while (true) {
boost::asio::ip::tcp::socket socket(io_context);
acceptor.accept(socket);
std::thread(handle_client, std::move(socket)).detach(); // Detach the thread
}
}
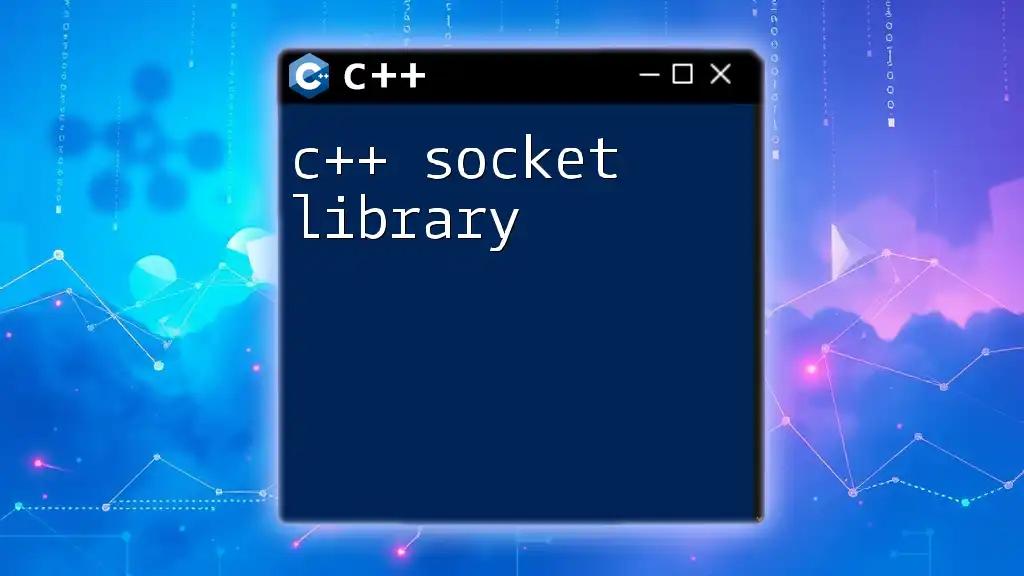
Security Considerations
Securing Network Connections
Security in networking is paramount, particularly when handling sensitive data. Implementing SSL/TLS is essential for encrypting data transmitted over the network.
Using POCO, here's a basic setup for creating a secure HTTP server:
#include <Poco/Net/SSLServerSocket.h>
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPServerRequest.h>
#include <Poco/Net/HTTPServerResponse.h>
#include <Poco/Net/SSLManager.h>
#include <Poco/Net/SSLException.h>
void startSecureServer() {
try {
Poco::Net::SSLManager::instance().initializeServerDefaults();
Poco::Net::SSLServerSocket s(/*SSL port*/ 443);
Poco::Net::HTTPServer server(new Poco::Net::HTTPRequestHandlerFactoryImpl<HelloWorldRequestHandler>(), s);
server.start();
} catch (Poco::Net::SSLException& e) {
std::cerr << "SSL Error: " << e.what() << std::endl;
}
}
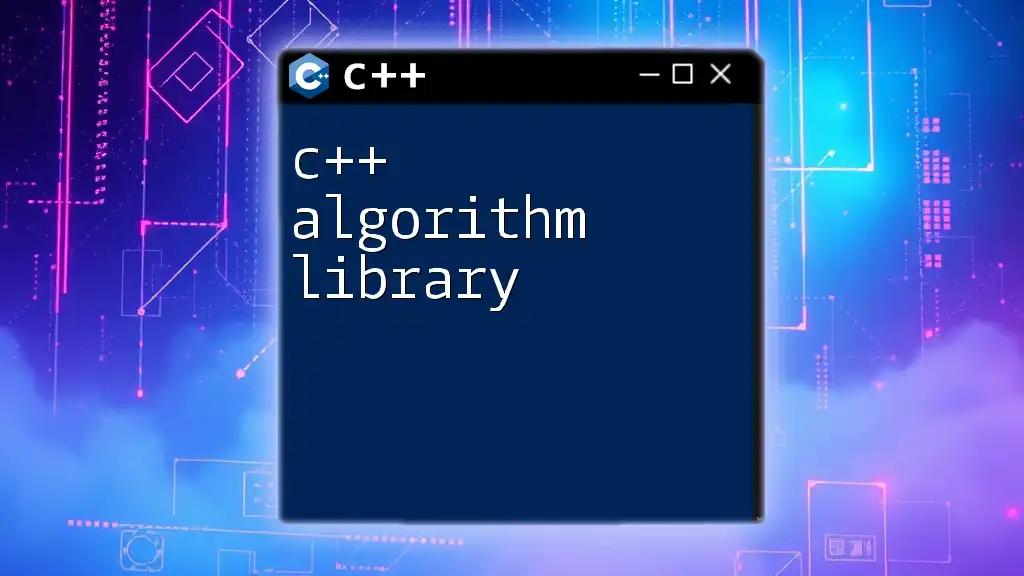
Best Practices for C++ Networking
Performance Optimization
When optimizing for performance in a C++ networking library, consider these practices:
- Reduce latency by minimizing the number of system calls.
- Implement connection pooling to reuse existing connections rather than creating new ones.
- Use appropriate buffer sizes to reduce the frequency of I/O operations.
Error Handling and Logging
Effective error handling and logging are crucial for maintaining robust applications. Implement comprehensive error handling across all network operations and log crucial events for later analysis. This practice can help you troubleshoot issues quickly and improve the reliability of your networking applications.
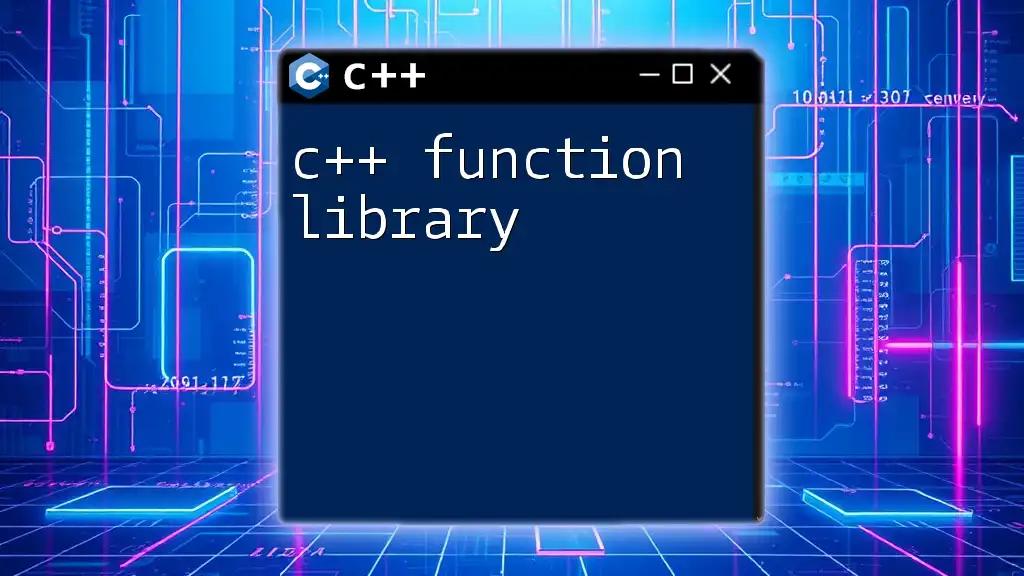
Conclusion
A C++ networking library serves as a vital tool for developers looking to implement networking features efficiently. By utilizing popular libraries such as Boost.Asio, POCO, or Qt, you can enhance your applications with robust network communication capabilities. Always prioritize security and best practices in networking, as these ensure your applications are both performant and safe. Embrace the world of C++ networking and create powerful, connected applications!

References
- Boost.Asio Documentation
- POCO C++ Libraries Documentation
- Qt Network Module Documentation

Additional Resources
- "C++ Networking and Socket Programming" by John Doe
- Online forums like Stack Overflow and C++ networking groups