Networking in C++ involves using libraries like Boost.Asio to manage and implement asynchronous network communications efficiently.
Here’s a simple code snippet demonstrating a TCP server in C++ using Boost.Asio:
#include <iostream>
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
int main() {
boost::asio::io_context io_context;
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 12345));
while (true) {
tcp::socket socket(io_context);
acceptor.accept(socket);
std::cout << "Client connected!" << std::endl;
}
return 0;
}
Understanding Socket Programming
What is Socket Programming?
Socket programming is a crucial aspect of networking in C++. It enables communication between two endpoints, whether on the same machine or across networks. A socket acts as a communication endpoint, allowing programs to send and receive data. There are two primary types of sockets:
- Stream Sockets (TCP): These sockets provide a reliable, connection-oriented communication channel. They ensure that data packets are delivered in the same order and without any loss.
- Datagram Sockets (UDP): These sockets operate on a connectionless basis, sending data without establishing a dedicated end-to-end connection. While they are faster, they do not guarantee the reliability of delivery or order.
Setting Up a Socket
Creating a Socket
To start networking in C++, you first need to create a socket using the `socket()` function. This function sets up the communication endpoint and returns a socket descriptor.
Here is a simple example of creating a TCP socket:
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
#include <iostream>
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Socket creation failed");
return -1;
}
std::cout << "Socket created successfully!" << std::endl;
return 0;
}
This code snippet creates a TCP socket for communication using the Internet Protocol (IPv4).
Binding a Socket
Once you have a socket, the next step is to bind it to a specific address and port using the `bind()` function. This association allows the operating system to route incoming data to your socket.
Here’s how to bind a socket to a port:
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = INADDR_ANY;
serv_addr.sin_port = htons(8080);
if (bind(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
perror("Bind failed");
return -1;
}
std::cout << "Socket bound to port 8080!" << std::endl;
In this example, we bind to all available interfaces on port 8080.
Listening and Accepting Connections
Listening for Connections
After binding, a server needs to listen for incoming connections using the `listen()` function. This function tells the operating system to accept incoming requests.
Here’s a code snippet for listening on the socket:
if (listen(sockfd, 5) < 0) {
perror("Listen failed");
return -1;
}
std::cout << "Listening for connections..." << std::endl;
The second parameter specifies the maximum length of the queue for pending connections.
Accepting Connections
Once your socket is listening, you can accept incoming connection requests with the `accept()` function. This function creates a new socket for the established connection, allowing communication with the client.
Example code to accept a connection:
struct sockaddr_in client_addr;
socklen_t addr_len = sizeof(client_addr);
int new_sock = accept(sockfd, (struct sockaddr *)&client_addr, &addr_len);
if (new_sock < 0) {
perror("Accept failed");
return -1;
}
std::cout << "Connection accepted!" << std::endl;
This code creates a new socket that handles communication with the connected client.
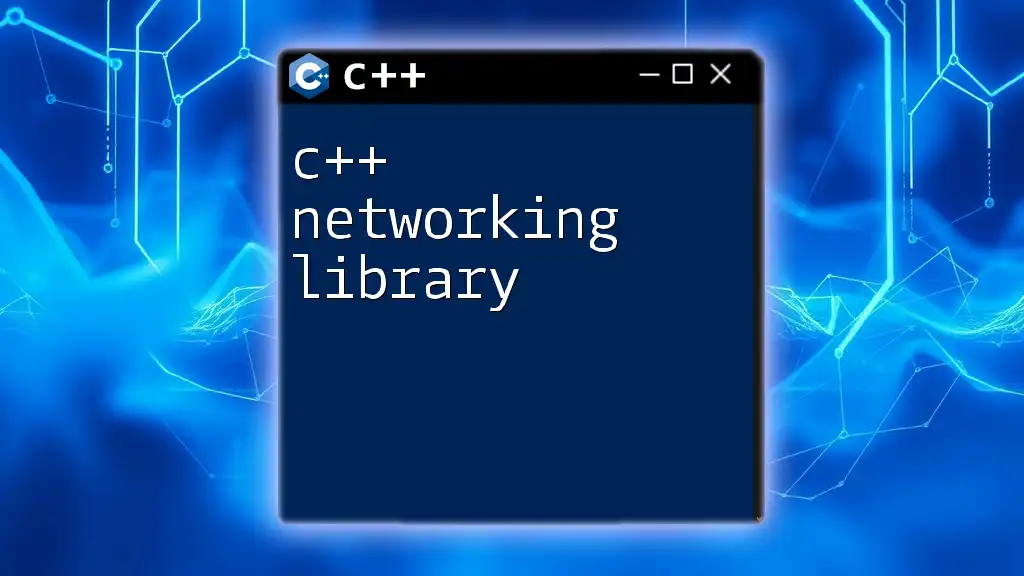
Client-Server Model
Overview of Client-Server Architecture
The client-server architecture is a fundamental model used in networking. In this model, the server waits and listens for incoming client connections. The client, on the other hand, initiates communication with the server. This connection allows the client to send requests for data, while the server processes these requests and sends back responses.
Writing a Simple Server Program
To implement a simple server in C++, we can combine the concepts discussed above. Here's a basic TCP server program:
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Socket creation failed");
return -1;
}
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = INADDR_ANY;
serv_addr.sin_port = htons(8080);
if (bind(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
perror("Bind failed");
return -1;
}
listen(sockfd, 5);
std::cout << "Server is listening on port 8080..." << std::endl;
struct sockaddr_in client_addr;
socklen_t addr_len = sizeof(client_addr);
int new_sock = accept(sockfd, (struct sockaddr *)&client_addr, &addr_len);
if (new_sock < 0) {
perror("Accept failed");
return -1;
}
std::cout << "Connection accepted!" << std::endl;
// Handle connection (e.g., send/receive data)
close(new_sock);
close(sockfd);
return 0;
}
This code creates a simple server that listens on port 8080 and waits for client connections.
Writing a Simple Client Program
For the client, we will use a similar approach, connecting to the server:
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
#include <string.h>
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Socket creation failed");
return -1;
}
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(8080);
inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr); // Connect to localhost
if (connect(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
perror("Connection failed");
return -1;
}
std::cout << "Connected to server!" << std::endl;
// Handle communication (e.g., send/receive data)
close(sockfd);
return 0;
}
This client program connects to the server running on the same machine.
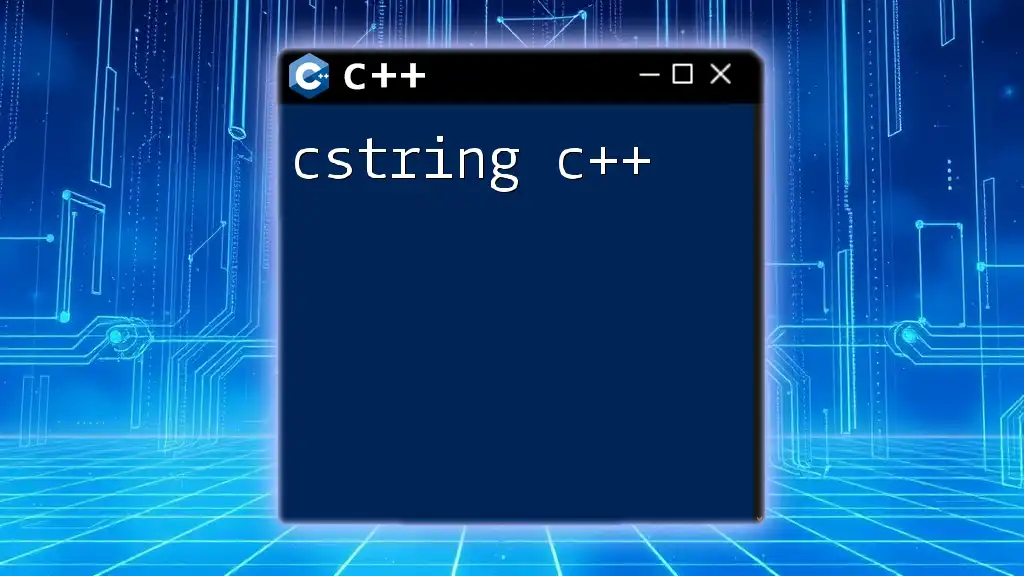
Networking with C++: Using Libraries
Introduction to Boost.Asio
For more complex networking features, many developers choose to use libraries like Boost.Asio. Boost.Asio is a cross-platform C++ library providing asynchronous input/output, networking functionality, and a rich set of features for building efficient and high-performance networked applications.
Connecting with Boost.Asio
Setting Up Boost.Asio
To get started with Boost.Asio, you first need to install the Boost library. You can find detailed instructions on the official Boost website.
Once installed, here’s a basic example of a TCP client using Boost.Asio:
#include <iostream>
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
int main() {
boost::asio::io_context io_context;
tcp::socket socket(io_context);
tcp::resolver resolver(io_context);
boost::asio::connect(socket, resolver.resolve("127.0.0.1", "8080"));
std::cout << "Connected to server using Boost.Asio!" << std::endl;
// Handle communication
return 0;
}
This demonstrates how to connect to a server with Boost.Asio, simplifying many underlying networking tasks.
Handling Asynchronous Operations
One of the strengths of Boost.Asio is its ability to handle asynchronous operations, allowing your program to efficiently manage multiple tasks without blocking. This is particularly beneficial in network programming.
Here is a basic structure showing how to receive async data:
void on_read(const boost::system::error_code& error, std::size_t bytes_transferred) {
if (!error) {
// Process the data
}
}
socket.async_read_some(boost::asio::buffer(data), on_read);
In this example, once data is available, the `on_read` function is triggered, providing a non-blocking event-driven model.
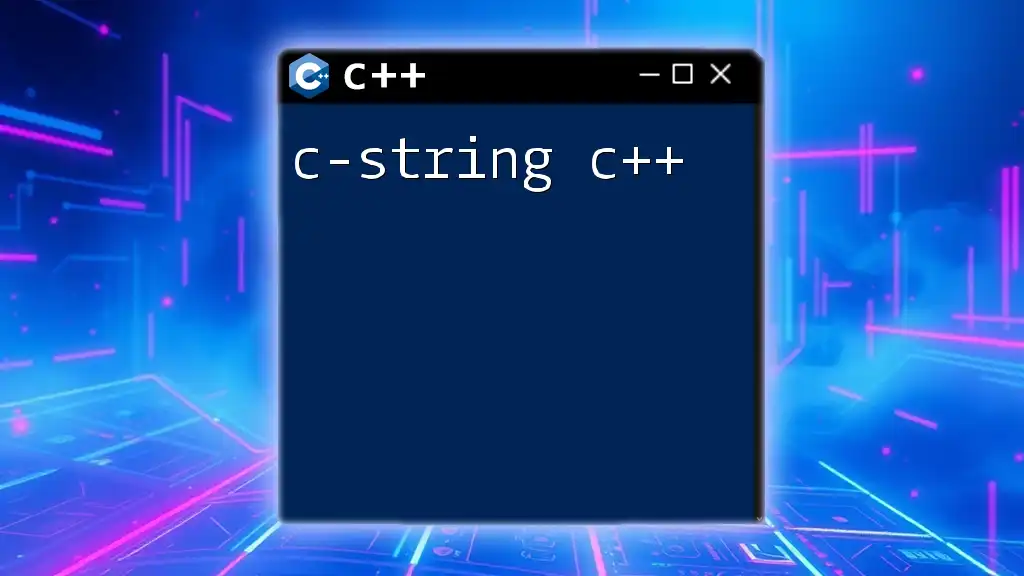
Error Handling in C++ Networking
Common Networking Errors
Error handling is essential in networking to deal with various issues, such as connection failures, timeouts, and resource limits. Typical networking errors in C++ might include:
- Socket creation failures
- Connection refusals
- Timeouts during communication
Implementing Error Handling
Implement error handling using appropriate checks after every significant networking call to catch and manage errors smoothly. Here's how to implement basic error handling:
if (error_occurred) {
perror("An error occurred");
close(sockfd);
}
This approach ensures that you can gracefully handle issues without crashing your application.
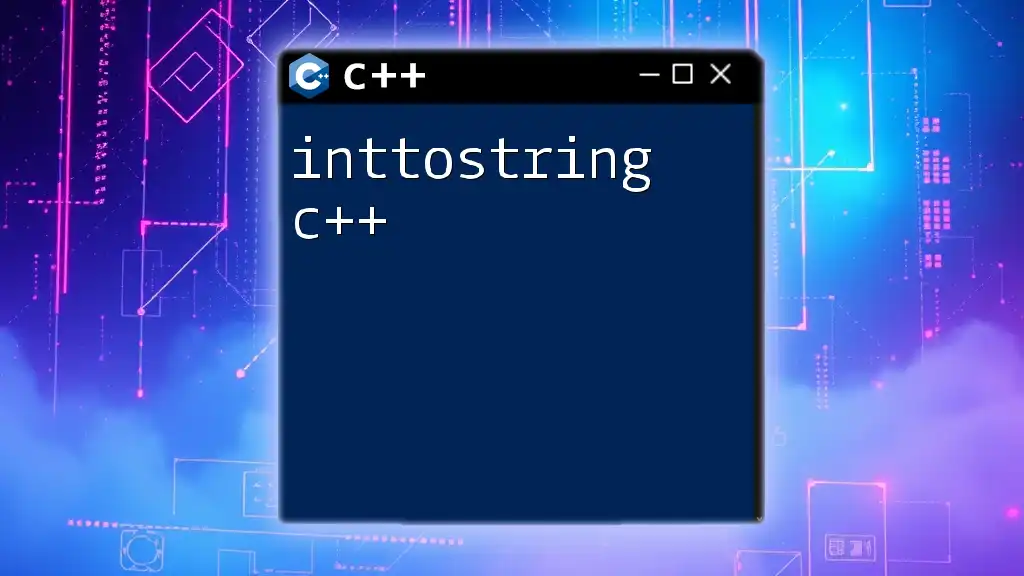
Conclusion
In summary, networking in C++ provides a robust framework for building network applications, enabling real-time communication over the internet. By leveraging socket programming and libraries like Boost.Asio, developers can create efficient client-server applications. Practice implementing the various components discussed, and you will deepen your understanding of networking concepts in C++. For further exploration, consider examining documentation and tutorials dedicated to more advanced features and best practices in C++ networking.
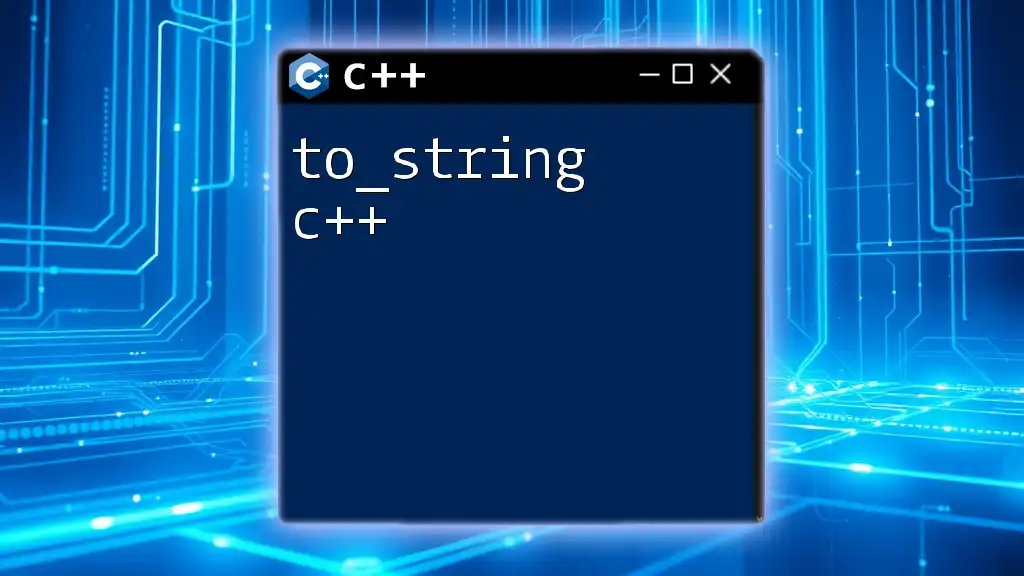
Additional Resources
For those interested in deepening their knowledge of networking in C++, consider checking out online resources, forums, and documentation that focus specifically on socket programming and the Boost libraries. Engaging with community discussions can also facilitate continuous learning and application of new techniques.