If the `getline` function in C++ isn't working as expected, it might be due to leftover newline characters in the input buffer, which can be resolved by using `std::cin.ignore()` beforehand.
Here’s a code snippet that demonstrates this:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin.ignore(); // Clear the input buffer
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
Understanding getline
What is getline?
The `getline` function in C++ is used to read a line of text from an input stream and store it in a string. It is particularly useful for gathering input that may contain spaces, such as names or full sentences, which other input methods like `cin` would fail to capture properly.
Syntax of getline
The syntax for `getline` is as follows:
getline(std::istream& is, std::string& str, char delim = '\n')
- `is`: This parameter is the input stream from which you're reading, typically `std::cin` for console input.
- `str`: This is the string variable where the input will be stored.
- `delim`: This optional parameter specifies the delimiter that marks the end of the input. By default, it is set to a newline character `\n`.
Example of Using getline
Here’s a simple example that demonstrates how `getline` works:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
This code prompts the user for their name and displays a greeting.
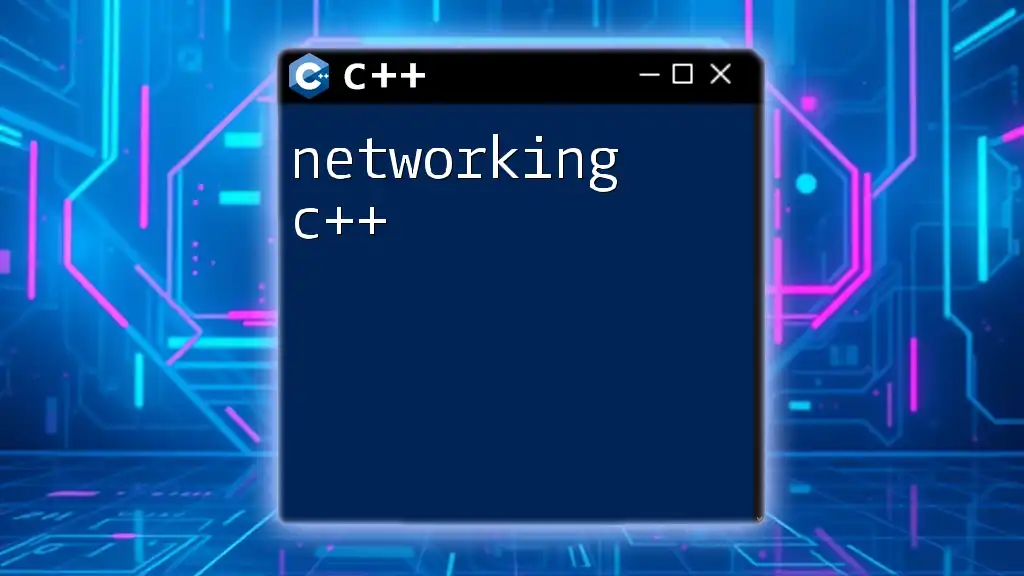
Common Issues With getline
Issue 1: Previous Input Conflicts
A common problem that programmers encounter is when using `getline` after preceding input operations like `cin`. The issue arises due to input buffering. After a call to `cin`, the newline character (which is not consumed by `cin`) remains in the input stream, causing `getline` to read this EOF-like state instead of waiting for new input.
Here's an example illustrating this problem:
#include <iostream>
#include <string>
int main() {
int age;
std::string name;
std::cout << "Enter your age: ";
std::cin >> age; // Input read here
std::cout << "Enter your name: ";
std::getline(std::cin, name); // getline may not read input correctly
std::cout << "Age: " << age << ", Name: " << name << std::endl;
return 0;
}
To fix this, you can use `std::cin.ignore()` right after `cin` to clear the newline character from the buffer.
Issue 2: Handling Leading Newline Characters
When you encounter issues with `getline`, it's often because of leading newline characters left in the input buffer. To resolve this, add the following line before your `getline` call:
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
This code will ignore any leftover characters in the input buffer until it reaches a newline, ensuring no remnants interfere with subsequent `getline` calls.
Issue 3: Unexpected Behavior with Delimiters
Understanding how to control the end of input is crucial. While `getline` uses the newline character by default, you can specify a different delimiter, such as a comma. Here's a short example:
#include <iostream>
#include <string>
int main() {
std::string data;
std::cout << "Enter data (comma-separated): ";
getline(std::cin, data, ',');
std::cout << "Data entered: " << data << std::endl;
return 0;
}
This code will stop reading input once it encounters a comma, demonstrating how you can adapt `getline` to your needs.
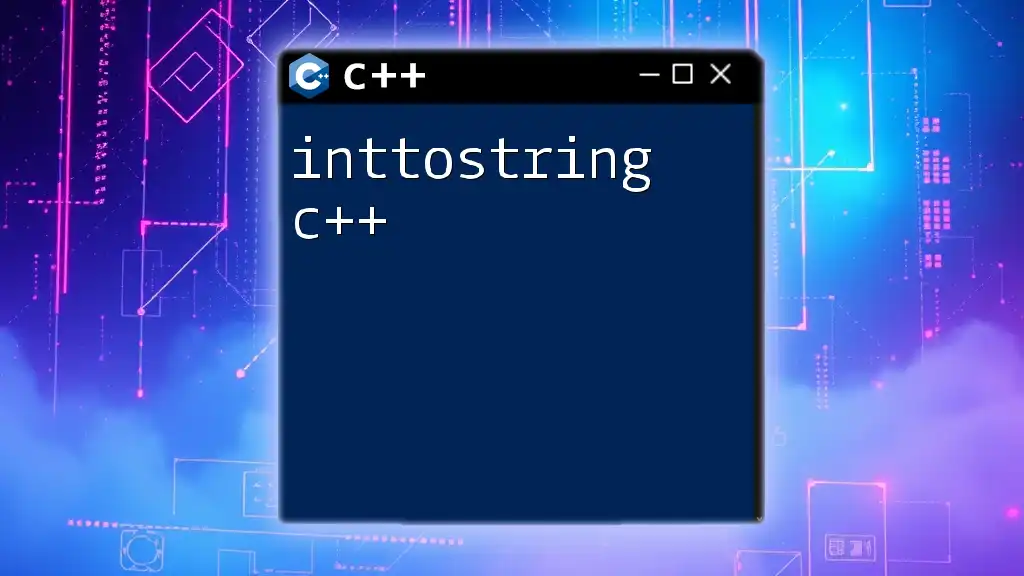
Debugging Techniques
Using Debugging Statements
When `getline` isn't working as expected, add print statements to verify the values of variables and the flow of execution. For example:
std::cout << "Current value of name: " << name << std::endl;
This simple technique can provide insight into whether `getline` reads the expected value.
Checking for Fail States
Using `std::cin.fail()` allows you to check if the last input operation encountered an error. For instance, it could look like this:
if (std::cin.fail()) {
std::cerr << "Input error! Please try again." << std::endl;
std::cin.clear(); // Clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discard invalid input
}
This code ensures that any erroneous input does not break your program.
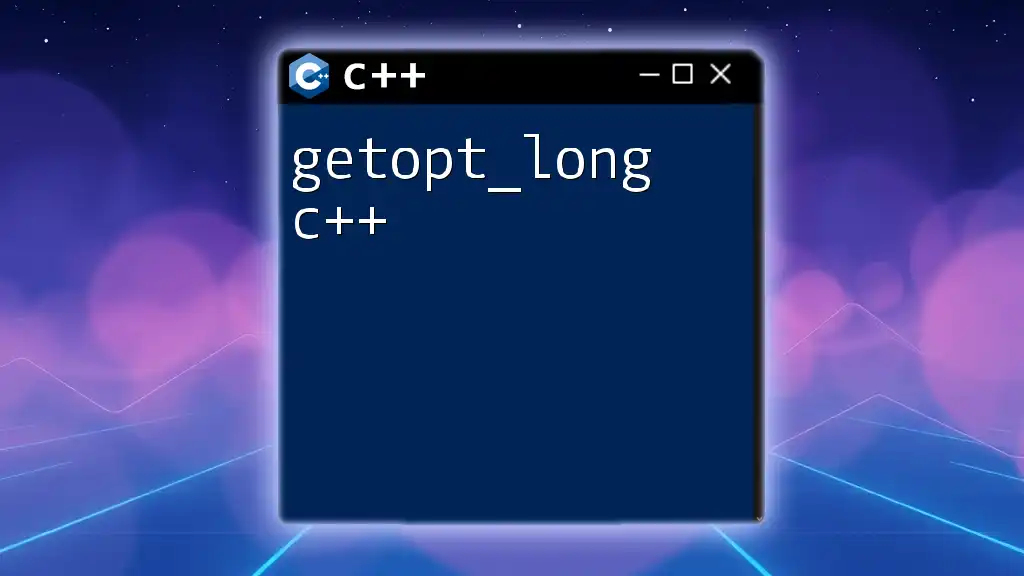
Best Practices for Using getline
Always Clear Input Buffer
After using `cin`, especially when reading integers or other formatted input, always clear the input buffer before calling `getline`. As mentioned, `std::cin.ignore()` is useful here and helps you avoid unexpected behavior.
Always Check Input Validity
It’s essential to validate the input you receive. After using `getline`, you can determine if the input was successful with the following condition:
if (std::getline(std::cin, name)) {
// Process input
} else {
// Handle error
}
This helps capture any unexpected input scenarios and allows for graceful error handling.
Handle Edge Cases
Prepare for cases such as empty inputs. Prompting for re-entry or checking if the string is empty right after acquiring input can improve user experience:
if (name.empty()) {
std::cerr << "No input provided. Please enter a valid name." << std::endl;
}
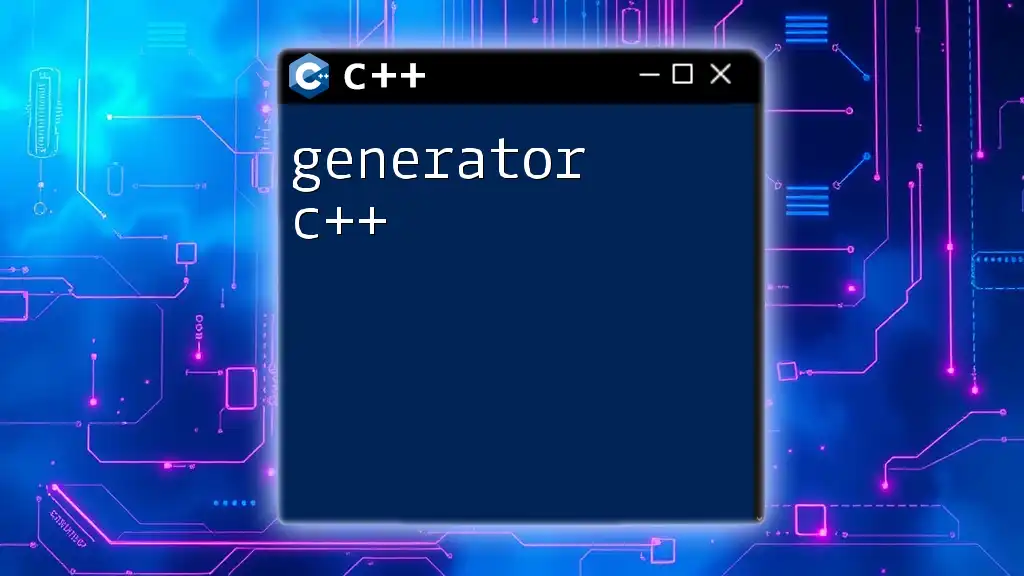
Conclusion
In summary, the `getline not working c++` issue is often linked to input stream conflicts and misunderstandings about how it operates with various data types and characters. By ensuring you clear the input buffer, checking fail states, and handling cases properly, you can effectively use `getline` without it causing roadblocks in user input handling. Practice employing these techniques, and don't hesitate to experiment with different approaches!
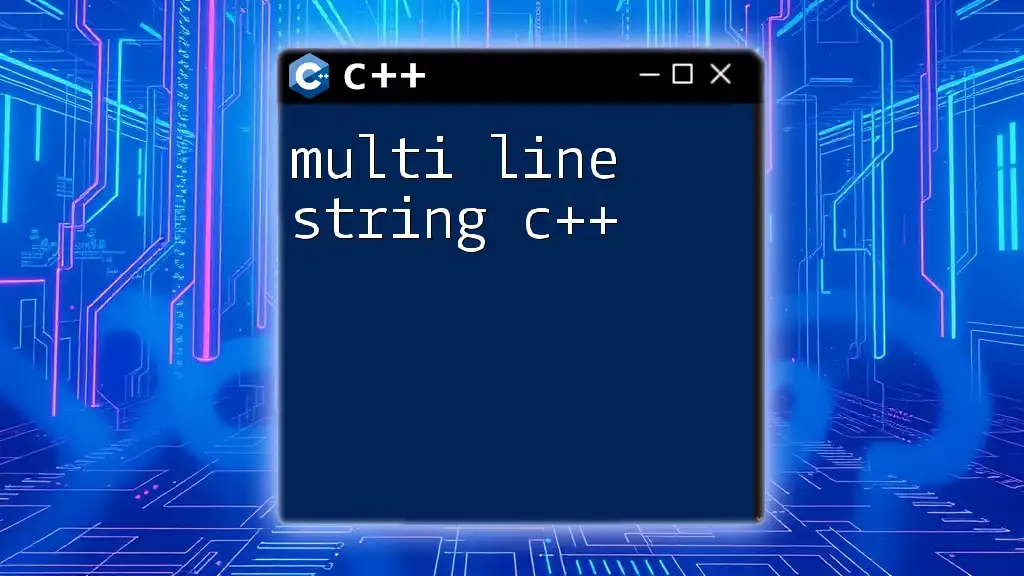
Additional Resources
For those looking to delve deeper into C++ input handling, the following resources can be immensely helpful:
- The official C++ documentation on `getline`.
- Recommended programming books and online tutorials focusing on input/output in C++.
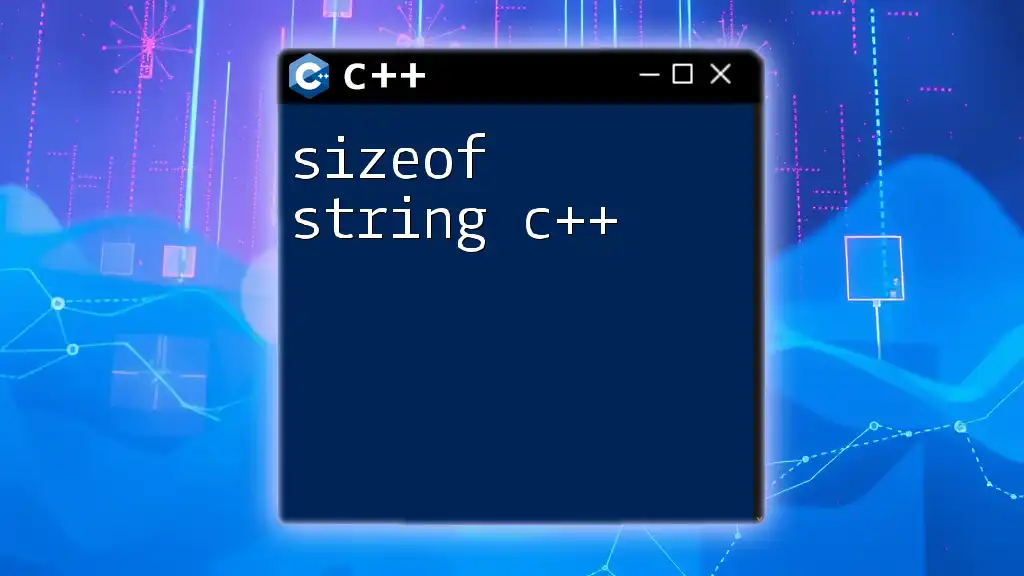
FAQs
FAQ 1: Why does getline skip the first input after cin?
This happens because the newline character left in the input buffer from the previous `cin` call is picked up by `getline`, making it seem like `getline` is not functioning. Always clear the input buffer if you're switching between `cin` and `getline`.
FAQ 2: Can getline read from files as well?
Yes, `getline` is versatile and works with file streams. For example:
std::ifstream file("data.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
This code effectively reads each line from a file and outputs it to the console.
FAQ 3: What if I want to read multiple strings separated by spaces?
You can use `istringstream` to achieve this. By reading the entire line first and then parsing it, you can easily handle multiple tokens:
#include <sstream>
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter your name and age: ";
std::getline(std::cin, line);
std::istringstream iss(line);
std::string name;
int age;
iss >> name >> age;
std::cout << "Hello, " << name << ", Age: " << age << std::endl;
return 0;
}
This approach allows you to split input based on spaces while still using `getline` for the initial read.