To reverse a substring in C++, you can use the `std::reverse` function from the `<algorithm>` header, which allows you to efficiently reverse the order of characters in the specified range of the string.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin() + 7, str.end()); // reverses "World!" to "!dlroW"
std::cout << str << std::endl; // Output: Hello, !dlroW
return 0;
}
Understanding Substrings
What is a Substring?
A substring is a contiguous sequence of characters within a string. In C++, substrings are fundamental for various text manipulations and analyses. For instance, given a string `str = "Hello, World!"`, `"Hello"` and `"World"` are both valid substrings. Understanding substrings is crucial in many programming applications, such as searching, parsing, and text formatting.
Importance of Substrings in Programming
Manipulating substrings allows programmers to efficiently handle and process strings. This includes tasks such as:
- Searching for specific patterns within strings.
- Modifying existing strings for formatting and reports.
- Data extraction for analysis or presentation.

C++ String Handling Basics
Using the Standard Library
C++ provides a rich Standard Library that simplifies string manipulations through the `std::string` class. This class offers utilities for managing string content effectively without needing to manage memory manually. It includes functions for concatenation, comparison, and various manipulations, making it easier to work with strings compared to older C-style strings.
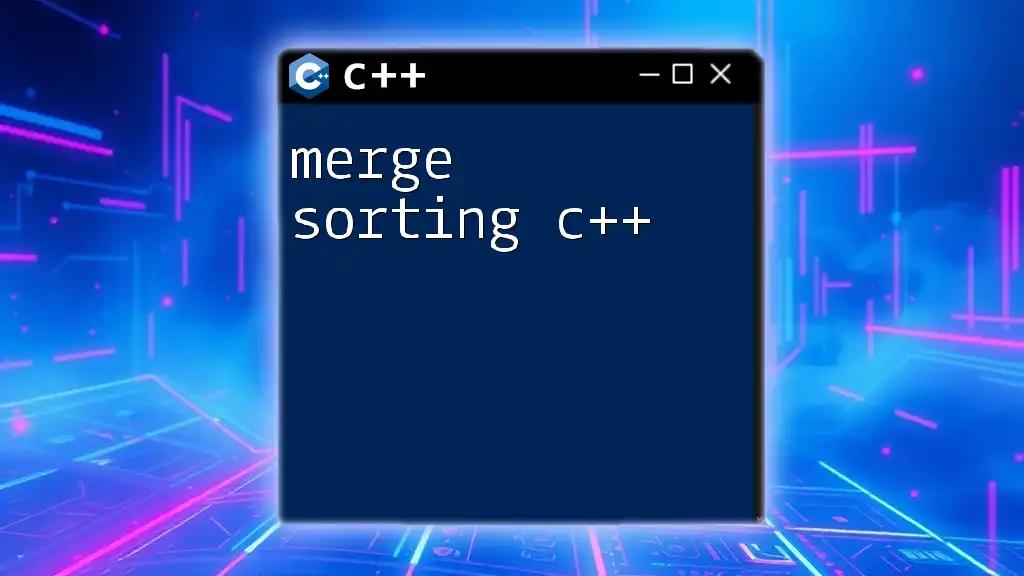
Techniques for Reversing a Substring
Extracting a Substring
To manipulate a substring in C++, we can use the `substr()` method available in the `std::string` class. This function allows you to extract a portion of the original string based on starting position and length.
Here's the syntax:
std::string substr(size_t start, size_t length);
Example of using `substr()`:
std::string original = "Hello, World!";
std::string sub = original.substr(0, 5); // "Hello"
In this example, `sub` comprises the first five characters of `original`.
Reversing a String
To reverse a string or a substring, we can utilize the `reverse()` function from the `<algorithm>` library. This function rearranges the elements in the given range, allowing us to reverse strings efficiently.
Example of reversing a string:
#include <algorithm>
std::string str = "Hello";
std::reverse(str.begin(), str.end());
// str is now "olleH"
By calling `std::reverse()` on the string `str`, we transform it into its reverse.
Combining Substring and Reverse
Creating a Function to Reverse a Substring
We can combine the techniques of extracting and reversing a substring into a cohesive function. Here’s how to create a function that reverses a specific substring within a larger string:
std::string reverseSubstring(std::string str, int start, int length) {
std::string sub = str.substr(start, length);
std::reverse(sub.begin(), sub.end());
return str.replace(start, length, sub);
}
// Example usage
std::string result = reverseSubstring("Hello, World!", 0, 5); // Output: "olleH, World!"
In this example, `reverseSubstring()` takes the original string, extracts the specified substring, reverses it, and then replaces the original substring with the reversed version.

Variations in Implementation
Reversing All Substrings
Suppose we want to reverse multiple substrings throughout a string. We can loop through the string indices, applying the reverse operation to each found substring. This allows for broader manipulations.
Reversing Without Using Built-in Functions
In cases where built-in functions are not desired, we can write a function to reverse a substring manually. Here’s an example of how to achieve this:
std::string manualReverse(std::string str, int start, int length) {
std::string reversed;
for (int i = start + length - 1; i >= start; --i) {
reversed += str[i];
}
return str.replace(start, length, reversed);
}
This `manualReverse()` function constructs the reversed substring manually by iterating backward through the indices of the original string.
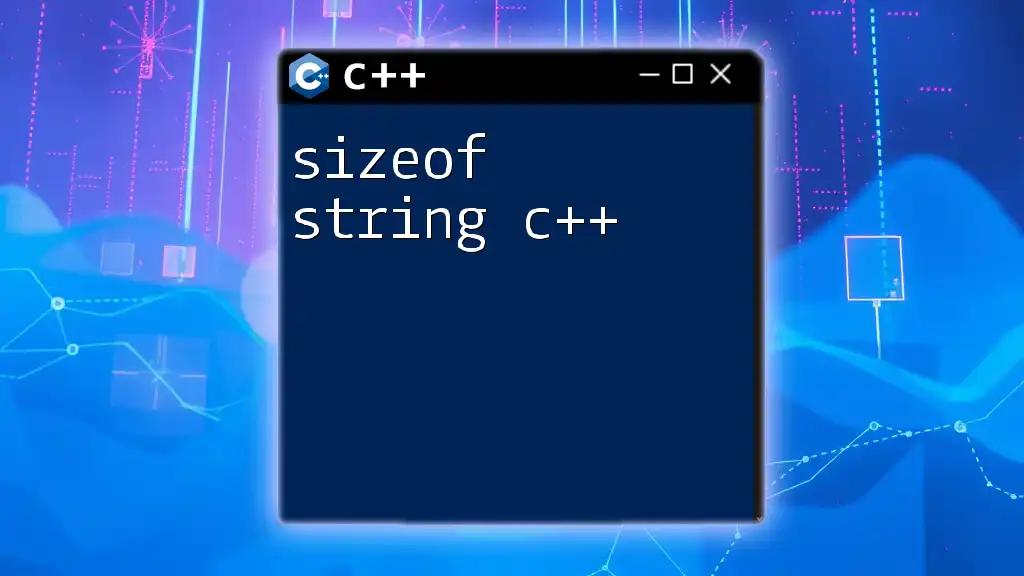
Practical Examples
Use Case 1: Palindrome Checking
Reversing substrings can be pivotal in algorithms for checking palindromes. By reversing a section of a string and comparing it to the original, we can efficiently determine if a word or phrase reads the same backward as forward.
Use Case 2: DNA Sequence Analysis
In bioinformatics, reversing substrings can be crucial for analyzing DNA sequences. For example, finding reverse complements of sequences may require reversing substrings, making this operation a valuable tool in genetic research.

Common Pitfalls
Off-by-One Errors
When manipulating substrings, developers often encounter off-by-one errors, particularly with indexing. It is vital to ensure the starting point and length are correctly calculated to avoid unexpected results or out-of-bounds errors.
Performance Considerations
Performance can be an essential factor when manipulating large strings. While C++ handles string manipulations efficiently, excessive copying and alterations can lead to overhead. Therefore, it is vital to consider performance implications when designing algorithms that involve multiple string operations.
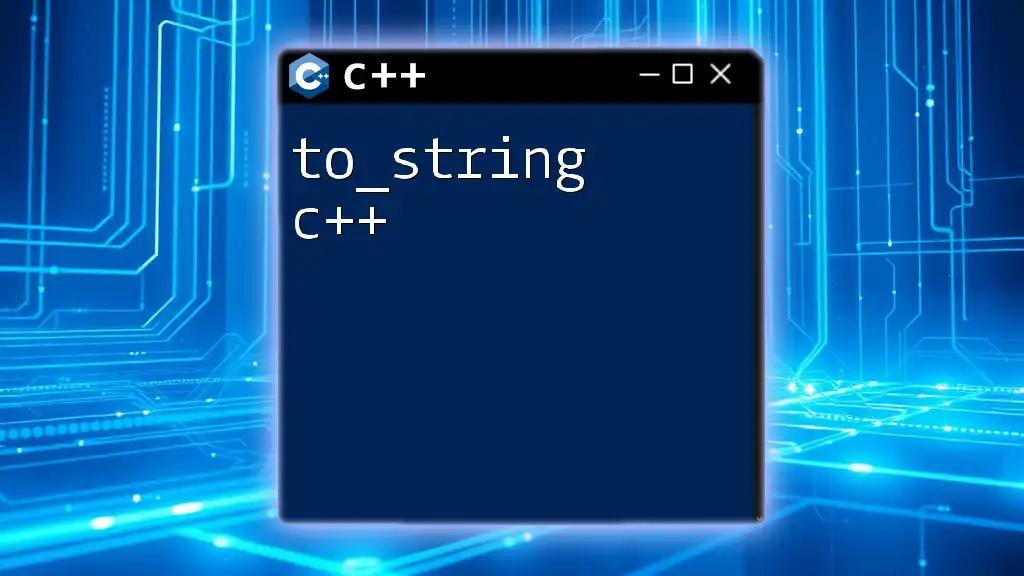
Conclusion
In conclusion, understanding how to reverse a substring in C++ is instrumental for effective string manipulation. This comprehensive guide covered the basics of substrings, illustrated practical techniques for reversing them, and highlighted real-world applications. By mastering these operations, you enhance your programming capabilities and prepare for more complex string manipulations.