In C++, the `const` keyword specifies that the value of a variable, like a `string`, cannot be modified after its initialization, ensuring safety and preventing accidental changes to the data.
const std::string greeting = "Hello, World!";
// greeting = "Hi!"; // This line would cause a compilation error
Understanding the Basics of C++
C++ is a powerful programming language that has gained immense popularity for its performance and flexibility. At its core, C++ is built around fundamental data types that allow developers to create efficient and effective software. Strings, as a data type, play a crucial role in handling text data and user inputs.
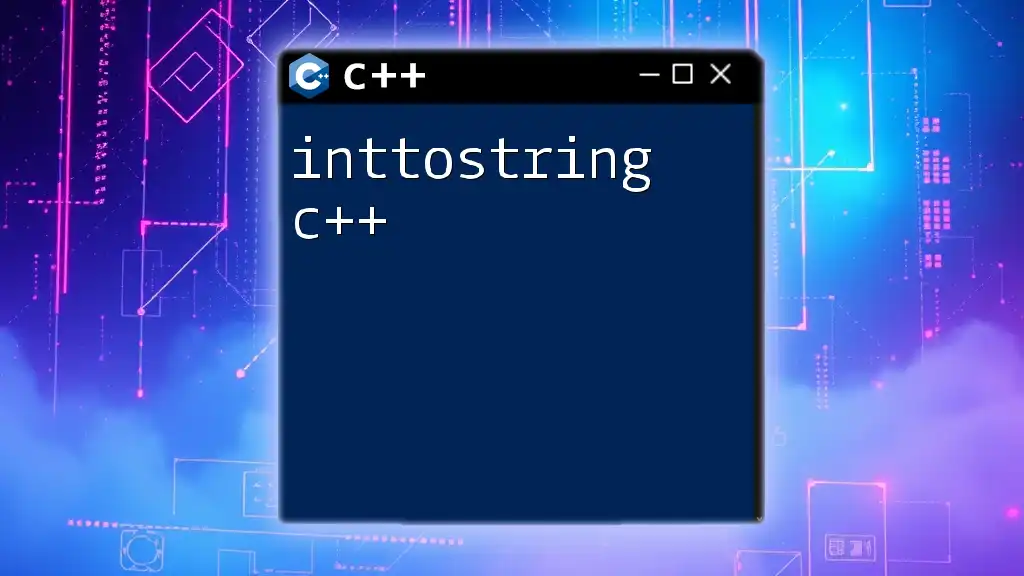
What is a String in C++?
In C++, a string can be defined as a sequence of characters. Unlike traditional character arrays, which are limited in functionality and ease of use, the `std::string` class offers a wealth of features, including dynamic resizing and various built-in functions.
String vs. Character Array
While both strings and character arrays can store text, `std::string` provides multiple methods that enhance functionality, such as concatenation and substring manipulation. This makes it a preferred choice in modern C++ programming.
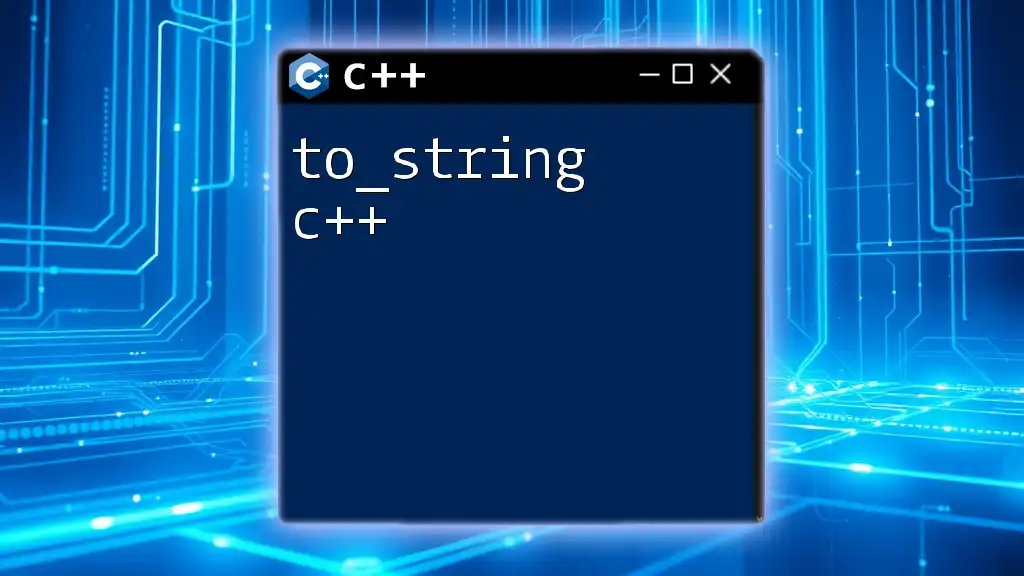
What is `const` in C++?
The `const` keyword in C++ serves as a qualifier that indicates immutability. When applied to variables, it protects them from being modified post-declaration.
Benefits of Using `const`
Utilizing `const` brings numerous advantages:
- Enhancing Code Readability: It makes the intention of the developer clear, indicating which variables should remain unchanged.
- Preventing Accidental Data Modification: This reduces bugs related to unintentional value changes.
- Potential Performance Optimizations: The compiler can make better decisions when it knows certain values will not change.
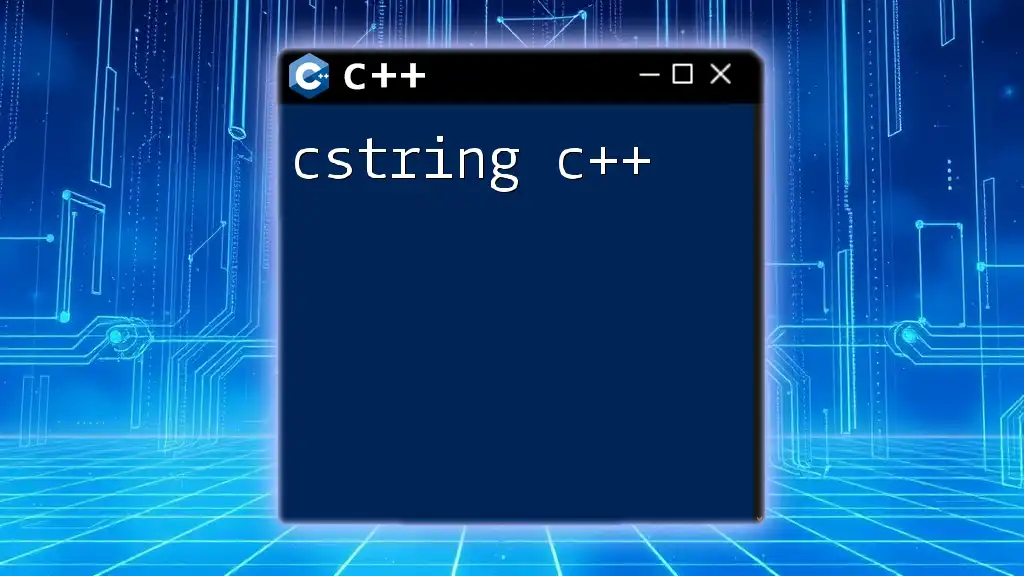
Introduction to `const string`
A `const string` in C++ allows you to declare a string that cannot be modified after its initial assignment. This is particularly useful in scenarios where immutability is desired, enhancing both security and clarity in your code.
Syntax and Declaration
Declaring a constant string in C++ is straightforward. The syntax is as follows:
const std::string myString = "Hello, World!";
Here, `myString` is declared as a constant, meaning any attempt to reassign it later will result in a compilation error.
When to Use `const string`
There are several circumstances where using a `const string` becomes advantageous:
- Immutability in Function Parameters: Whenever a string is passed into a function and should not be altered, `const string` is ideal.
- Function Returns: Returning a `const string` can guarantee that the string returned is not accidentally changed by the calling function.
- Multi-threaded Environments: By ensuring that string values remain constant, you can safely share them across threads without fear of unintended modifications.
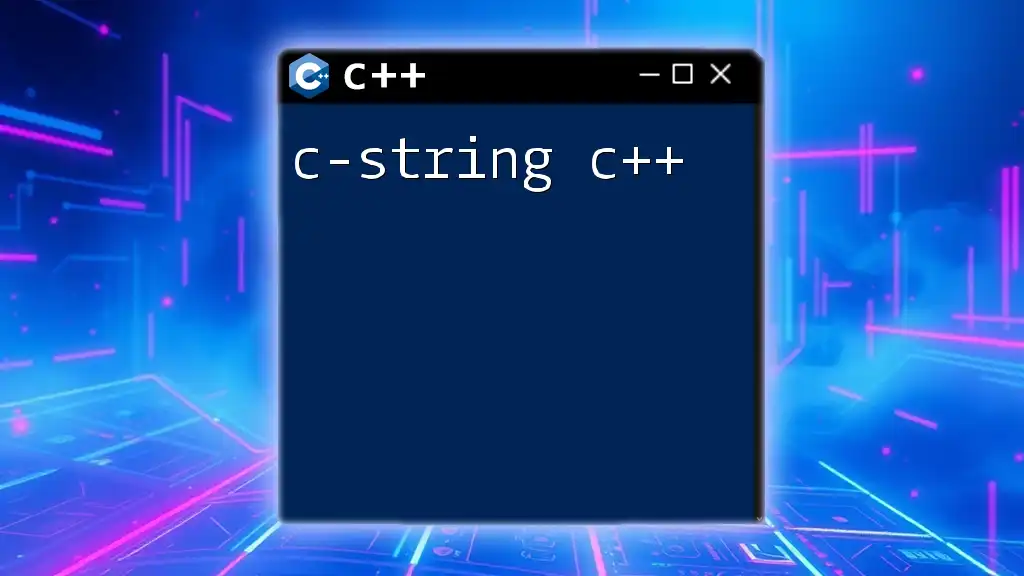
Examples of `const string` Usage
Basic Example
Let’s look at a simple application of `const string`:
const std::string greeting = "Hello, C++!";
std::cout << greeting << std::endl; // Output: Hello, C++!
In this example, `greeting` is a constant string that is printed to the console. Trying to change `greeting` later in the code would result in an error.
Passing `const string` to Functions
Using `const string` as function parameters is a common practice. Here’s how it can be achieved:
void printMessage(const std::string& message) {
std::cout << message << std::endl;
}
In this case, the function `printMessage` accepts a reference to a constant string, ensuring the original string remains intact while allowing efficient access to its contents.
Returning `const string` from Functions
Functions that return `const string` also provide safety. Here's an example:
const std::string getGreeting() {
return "Welcome to C++ programming!";
}
Returning a constant string guarantees that the caller cannot alter the string after it has been provided.
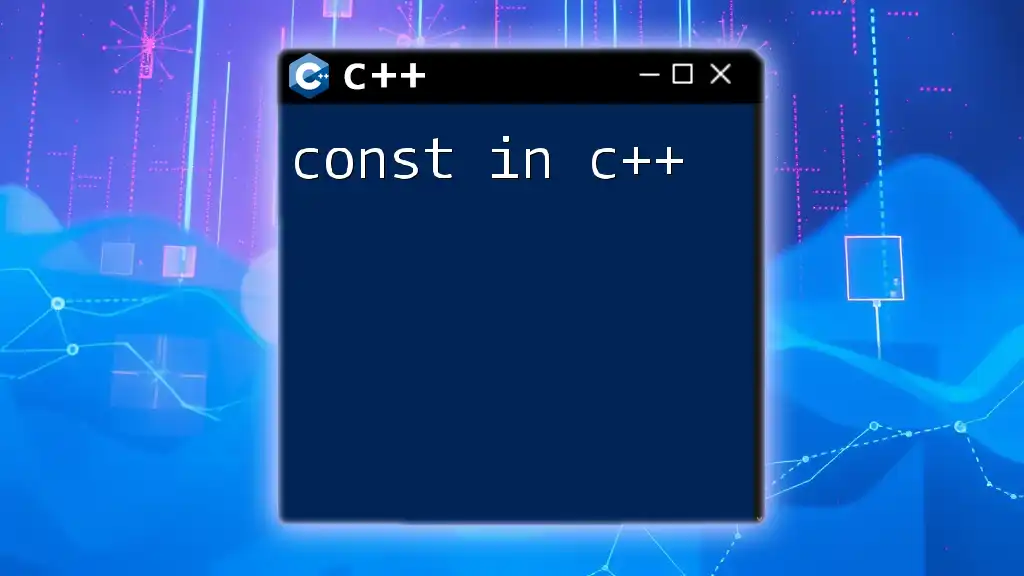
Advanced Concepts with `const string`
Constness and Member Functions
When using classes, it is useful to understand how `const` interacts with member functions:
class Greeting {
public:
const std::string message;
Greeting(const std::string& msg) : message(msg) {}
void display() const {
std::cout << message << std::endl; // Can use `message` as it is `const`
}
};
In this code, `message` is a `const` string member of the `Greeting` class. The `display` function is marked as `const`, meaning it cannot modify any member variables, which is essential for maintaining the integrity of the object's state.
Compatibility with String Manipulation Functions
Even when you're working with `const string`, numerous standard library functions will work efficiently. For example:
std::string appendToGreeting(const std::string& base) {
return base + ", C++ World!";
}
In this snippet, `appendToGreeting` takes a `const string` as an input and appends additional text to it, illustrating that you can manipulate copies while preserving the original string's constness.
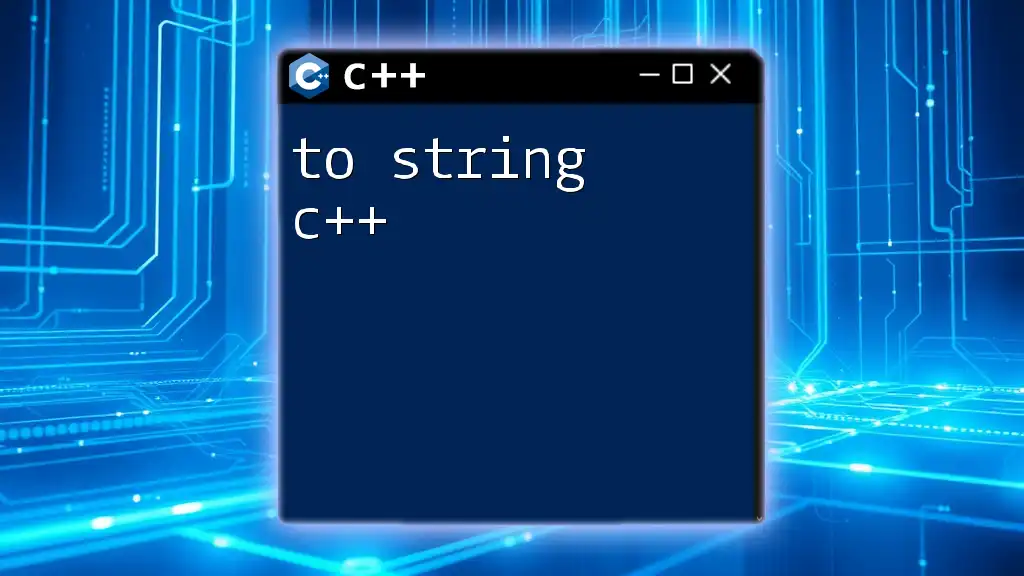
Common Pitfalls to Avoid with `const string`
Misunderstanding Constness
A common mistake is confusing a `const` string's behavior. For example, trying to reassign a `const string` will lead to errors. Here’s a simple mistake:
const std::string msg = "Hello";
// msg = "Hi"; // This will cause a compilation error
Remember that `const` ensures the variable it modifies cannot change after initialization.
Performance Considerations
While `const string` has its benefits, excessive use can lead to performance slowdowns if not used judiciously. Whenever possible, assess whether a string really needs to be `const` or if it can be modified instead.
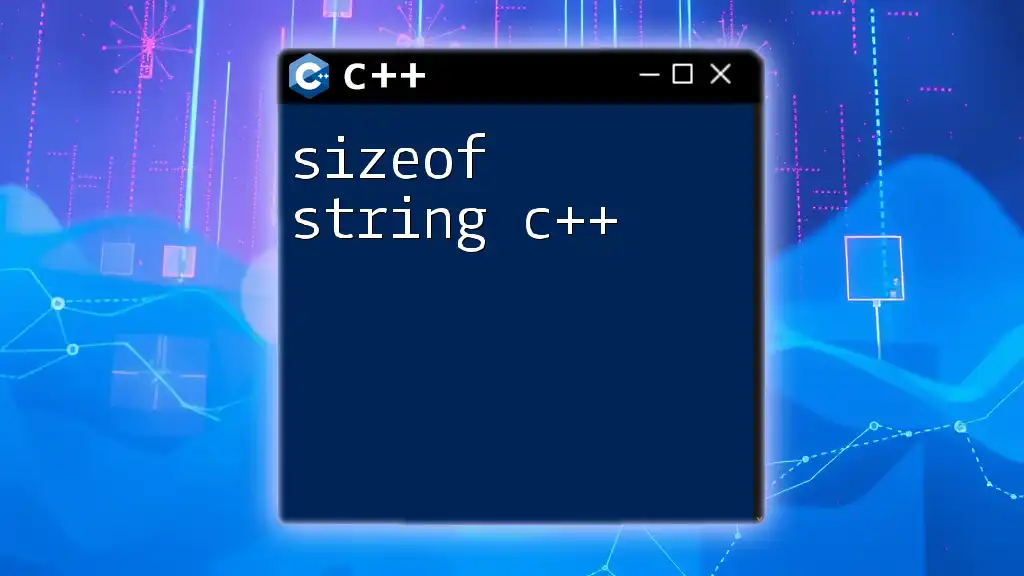
Conclusion
In conclusion, understanding `const string` in C++ is important for writing secure, efficient, and maintainable code. It promotes code clarity and prevents unintentional modifications, offering significant benefits both in development and runtime.
As you continue your C++ journey, exploring more complex scenarios involving `const string` will enhance your programming skills and deepen your understanding of C++. Start practicing today, and consider diving into further courses for more advanced C++ topics to fully leverage the capabilities of this powerful language.