In C++, the `const` keyword is used to define variables whose values cannot be modified after initialization, promoting safer and more predictable code.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
int main() {
const int maxScore = 100; // maxScore cannot be changed
std::cout << "The maximum score is " << maxScore << std::endl;
// maxScore = 90; // This line would cause a compilation error
return 0;
}
What is Const?
The const keyword in C++ is used to define constant variables and objects, which means the values they hold cannot be modified after initialization. This is crucial for maintaining the integrity of data throughout your program.
When a variable is defined with the const qualifier, it signals to both the compiler and anyone reading the code that the variable’s value should remain constant, improving the readability and reliability of the code.
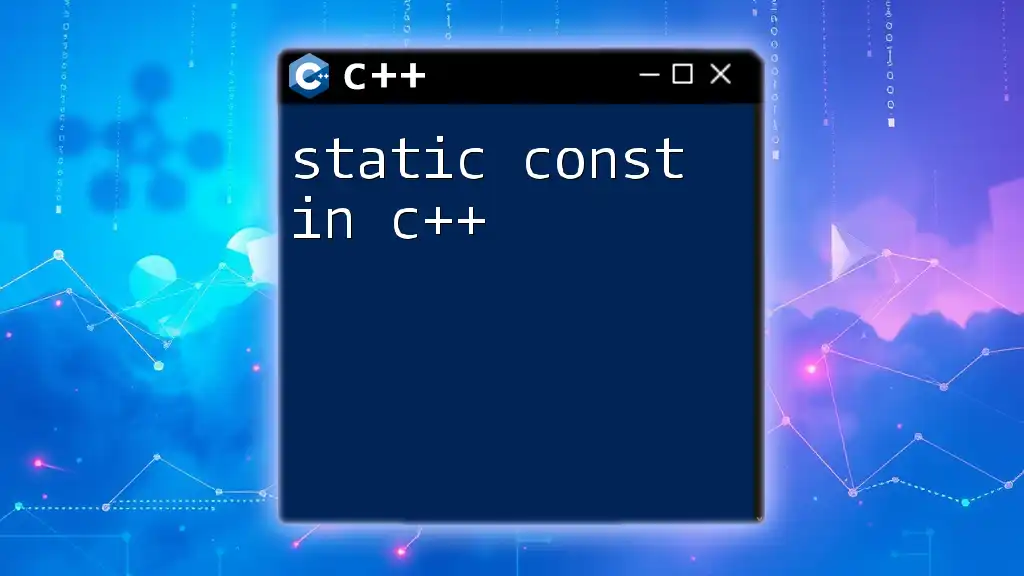
Understanding Constness
Value vs. Reference Constness
Constness can be categorized into two types: value constness and reference constness.
-
Value Constness: When you declare a variable as const, you're stating that its value should not change. For example:
const int maxValue = 100; // The value of maxValue cannot be changed
-
Reference Constness: When a reference is declared as const, it cannot be altered to refer to another object. This ensures that the reference remains tied to its original object throughout its lifetime.
Const vs. Non-const Variables
It’s important to understand the distinction between const and non-const variables. Non-const variables allow for modifications while const variables do not.
For instance:
int regularVar = 10;
const int constVar = 20;
regularVar = 15; // Allowed
constVar = 25; // Error: cannot assign a value to a const variable
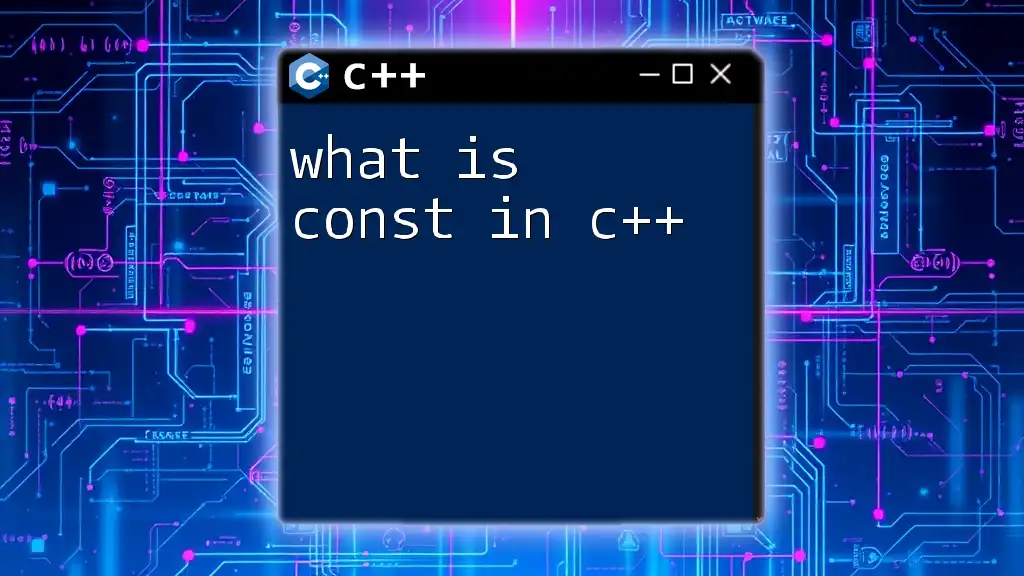
Syntax of Const in C++
Declaring Const Variables
To declare a variable as const, you place the const keyword before the type. Here’s the syntax:
const type variableName;
For example, declaring a constant integer:
const int maxConnections = 100; // Constant integer
Using const with character arrays can look like this:
const char* greeting = "Hello, World!"; // Constant character array
Usage of the Const Keyword
The const keyword can be used in various contexts throughout your code, from simple variable declarations to defining complex data structures.
To illustrate:
const double pi = 3.14159;
const std::string name = "Const Example";
In these examples, both `pi` and `name` are immutable; any attempt to change their values will lead to a compilation error.
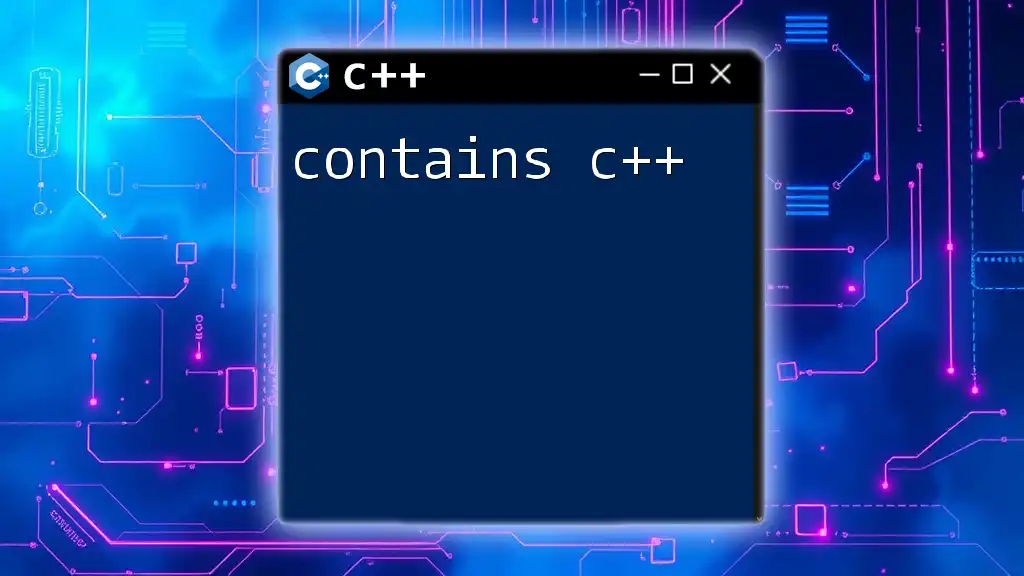
Using Const with Pointers
Const Pointers
Pointers can also be made constant, which is critical in many scenarios. There are two variations to consider:
-
Pointer to a Const Value: This means you cannot change the value being pointed to:
int value = 42; const int* ptrValue = &value; // Pointer to a const integer
-
Const Pointer: In this scenario, you cannot change where the pointer is pointing:
int* const ptrConst = &value; // Const pointer to an integer
Const with Pointers to Const Data
When utilizing pointers to const data, you ensure that neither the pointer itself nor the data it points to can be modified. This is important for protecting data integrity.
Example:
const int* ptrConstValue = &value; // Pointer to a const value
This setup ensures that the integer value `42` cannot be tampered with through `ptrConstValue`.
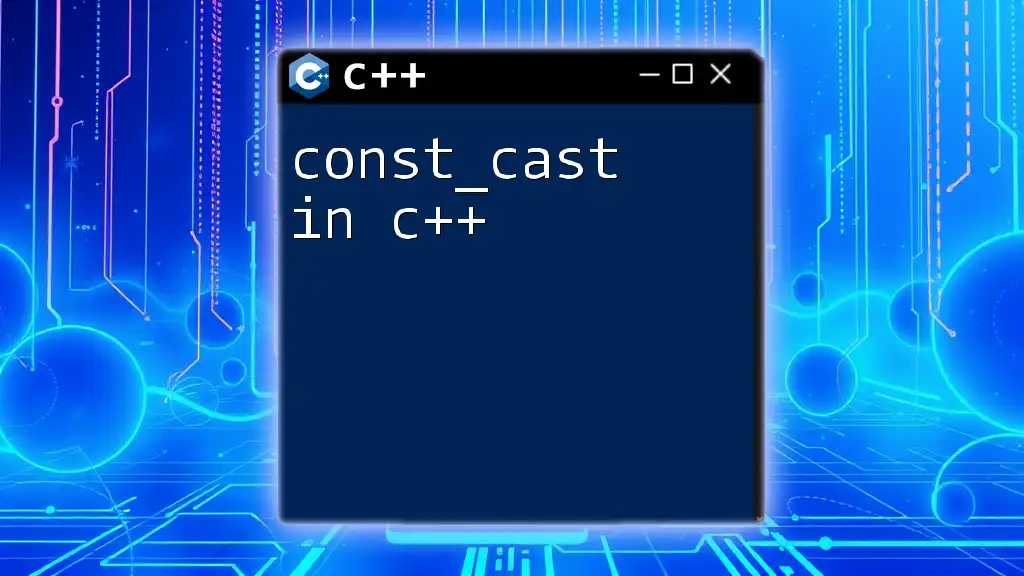
Const Member Functions
In C++, class member functions can also be declared as const, which indicates that such functions will not modify any member variables of the object they belong to. This feature promotes safer code practices.
The syntax for a const member function is straightforward:
class Example {
public:
void display() const { // Const member function
// Implementation that does not modify member variables
}
};
Within a const member function, you can access member variables but cannot alter them, which helps in generating consistent behaviors while assuring the caller about the immutability of the method.
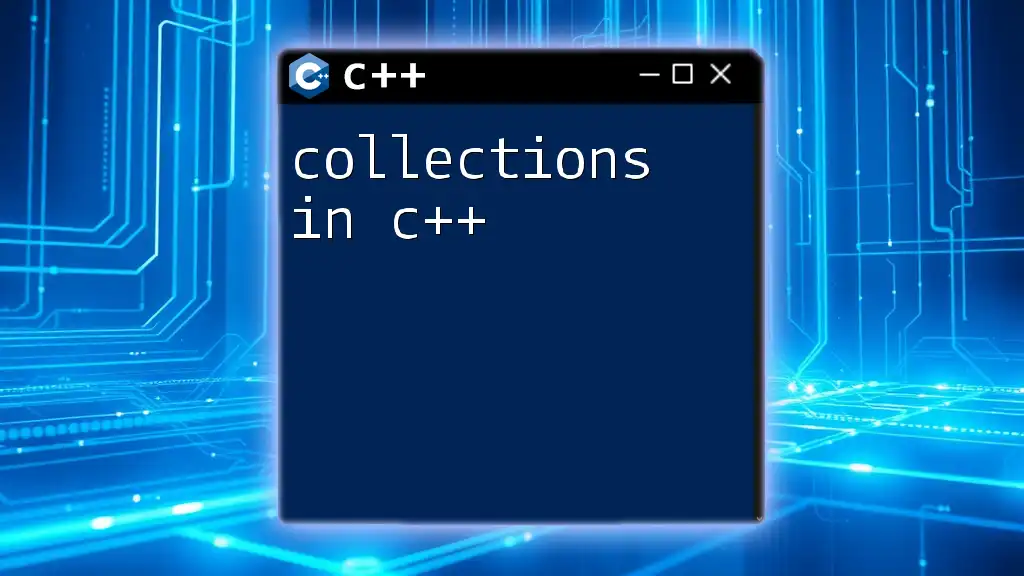
Benefits of Using Const in C++
Const for Code Safety
Using const effectively reduces the risk of accidental modifications throughout your code, making it much safer. By defining variables as constants, you can protect critical values and maintain data integrity. This practice aids in identifying potential logical errors during compilation rather than runtime, preventing bugs from proliferating.
Const and Performance Optimization
Moreover, utilizing const can lead to performance optimizations by enabling the compiler to make more aggressive assumptions about the code, potentially leading to more efficient machine code generation. For instance, when a function parameter is declared as const, the compiler can assume that the data won't change, allowing it to optimize the function's implementation.
Consider the following:
void processData(const DataStructure& data) {
// The compiler knows 'data' won't change, allowing optimizations
}
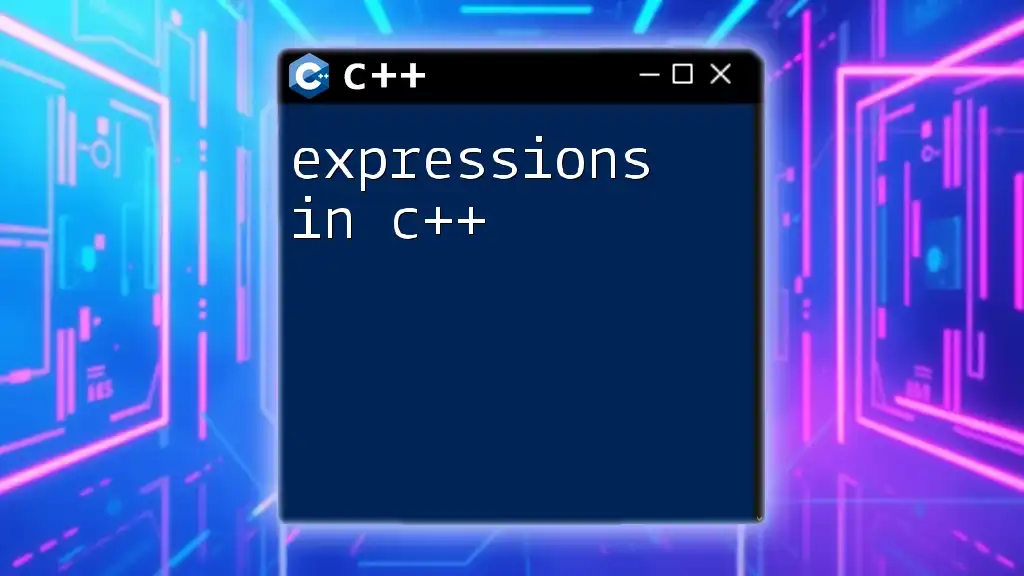
Best Practices for Using Const
To maximize the benefits of const, consider these best practices:
- Use const whenever possible, especially for function parameters and return types.
- Prefer `const` to protect data integrity and signal intent to other developers.
- Be cautious of overusing const inappropriately, which may lead to complex code or hinder clarity. Use const when you have a legitimate reason to enforce immutability.
In some cases, you might find it useful to create `const` versions of overloaded functions, which can help clarify intent without impacting code readability.
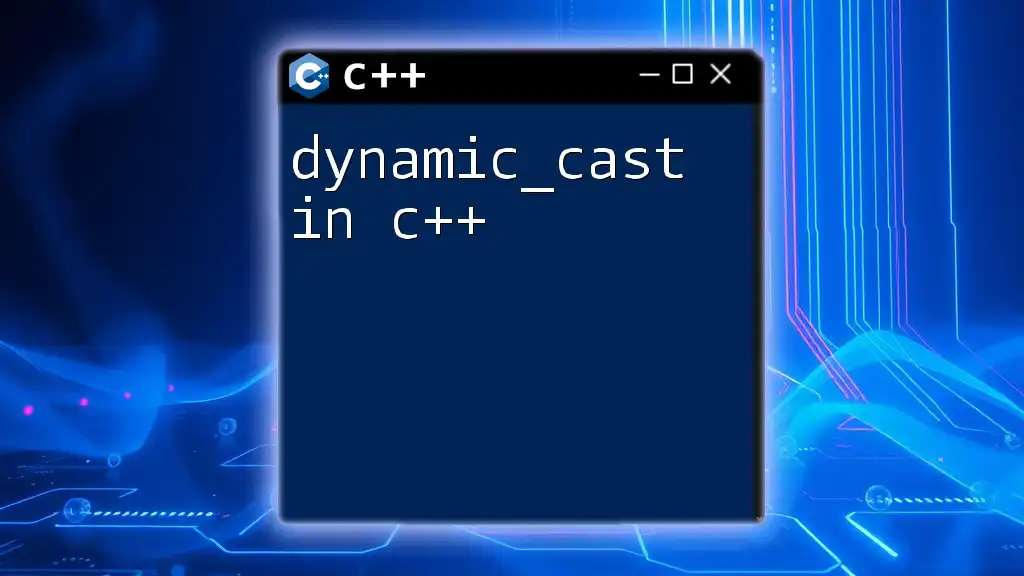
Summary
Throughout this guide, we've established that const in C++ plays a vital role in improving code safety, optimizing performance, and clarifying intentions. By making effective use of the const keyword, programmers can write robust, reliable, and maintainable code.
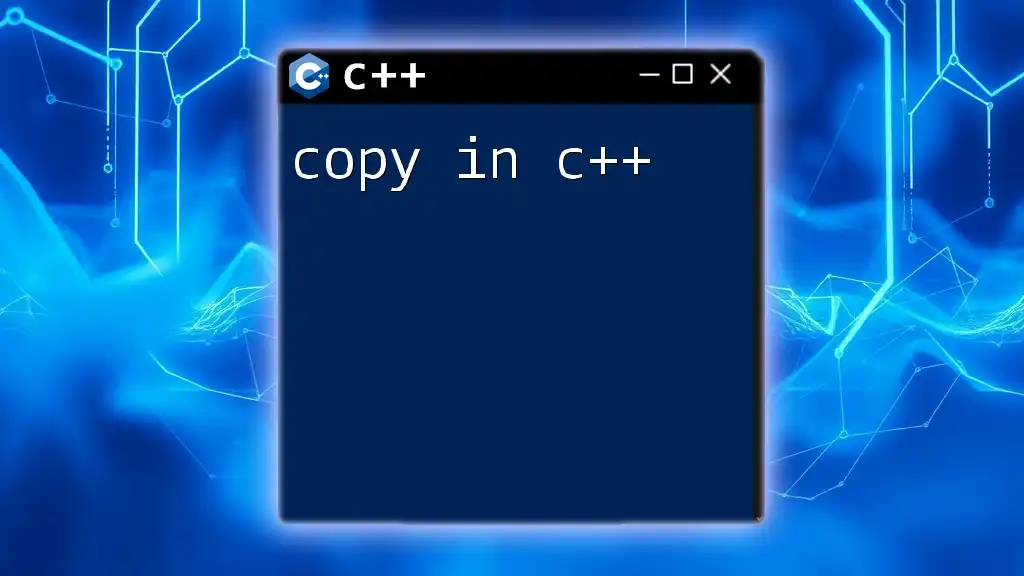
Conclusion
Mastering the const keyword in C++ will not only help you write cleaner and safer code but also encourage better programming practices. By consistently applying `const` where appropriate, you cultivate a codebase that is easier to understand, maintain, and optimize.
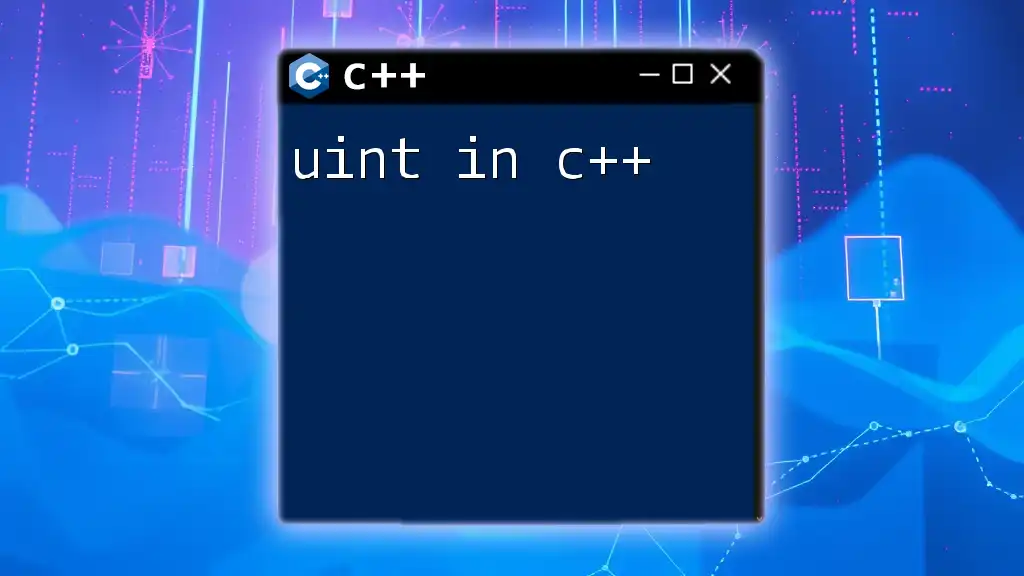
Additional Resources
For more insights into const in C++, consider referring to the official C++ documentation and exploring coding exercises that reinforce the principles discussed in this article. Engaging with practical examples will significantly improve your comprehension and application of const in your programming projects.