In C++, a script typically refers to a sequence of commands or code that can be executed, often to automate tasks or perform simple operations, as demonstrated in the following example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ Scripting
C++ scripting is a powerful way to utilize the C++ programming language beyond traditional application development. Unlike loosely-typed scripting languages such as Python or JavaScript, C++ combines speed and efficiency, making it a compelling option for relatively resource-intensive tasks. In this guide, we'll explore the foundations of scripting in C++, how to set up your environment, and advanced concepts that will enhance your scripting skills.

Understanding C++ Scripts
What is a C++ Script?
A script in C++ is a collection of C++ code that automates tasks or runs specific functionalities. These scripts often serve tasks like data processing, gaming logic, or even extensive computational simulations. The primary characteristics of C++ scripts include efficiency, support for object-oriented programming, and a syntactically rich framework that offers robust functionality.
C++ vs. Traditional Scripting Languages
When comparing C++ to traditional scripting languages, one notable advantage is performance. C++ code compiles into efficient machine code, leading to faster execution times for intensive tasks. Additionally, C++ is more type-safe than dynamic scripting languages, reducing the likelihood of runtime errors due to unexpected data types.
However, opting for C++ scripting often involves trade-offs in terms of complexity. While it provides greater control over system resources and performance, developers may face a steeper learning curve and longer development times than with scripting languages designed for rapid prototyping.
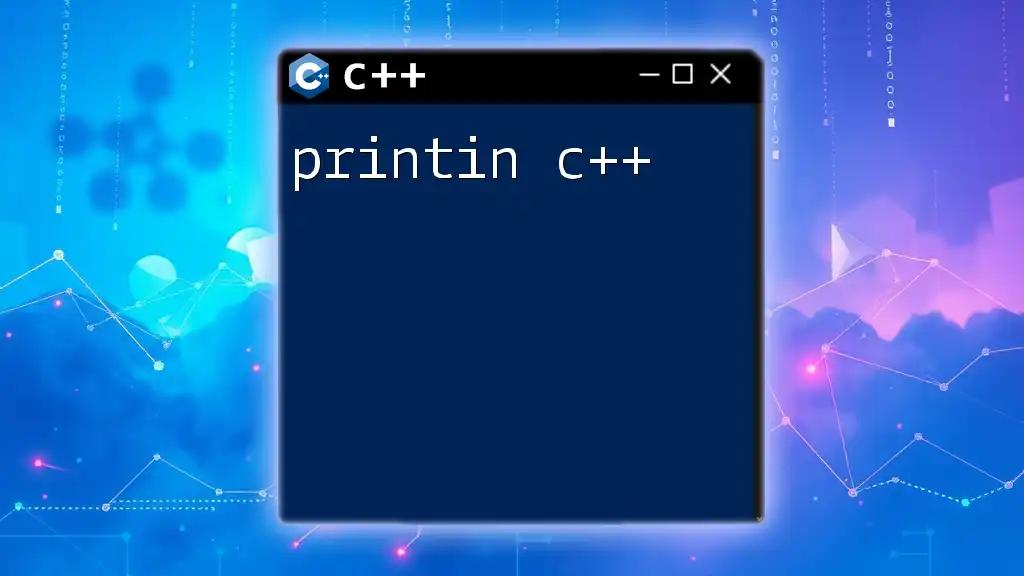
Setting Up Your C++ Environment
Installing a C++ Compiler
To begin scripting in C++, you first need to install a C++ compiler. Some popular options include:
- GCC: A widely used open-source compiler available on multiple platforms.
- Clang: Known for its performance and modern features, Clang is also a great choice for C++ compilation.
- MSVC: The Microsoft Visual C++ compiler, commonly used in Windows environments.
To install GCC, for instance, you would typically run the following command on a Unix-based system:
sudo apt-get install g++
For other operating systems, refer to the relevant installation guides.
Choosing an Integrated Development Environment (IDE)
The next step is choosing an IDE that suits your needs. Some popular IDEs for C++ scripting include:
- Visual Studio: Feature-rich, ideal for Windows users with extensive debugging tools.
- Code::Blocks: Lightweight and customizable, it's excellent for beginners.
- CLion: A professional IDE with a robust feature set, though it requires a subscription.
Once you've downloaded an IDE, follow the setup instructions to create your development environment.
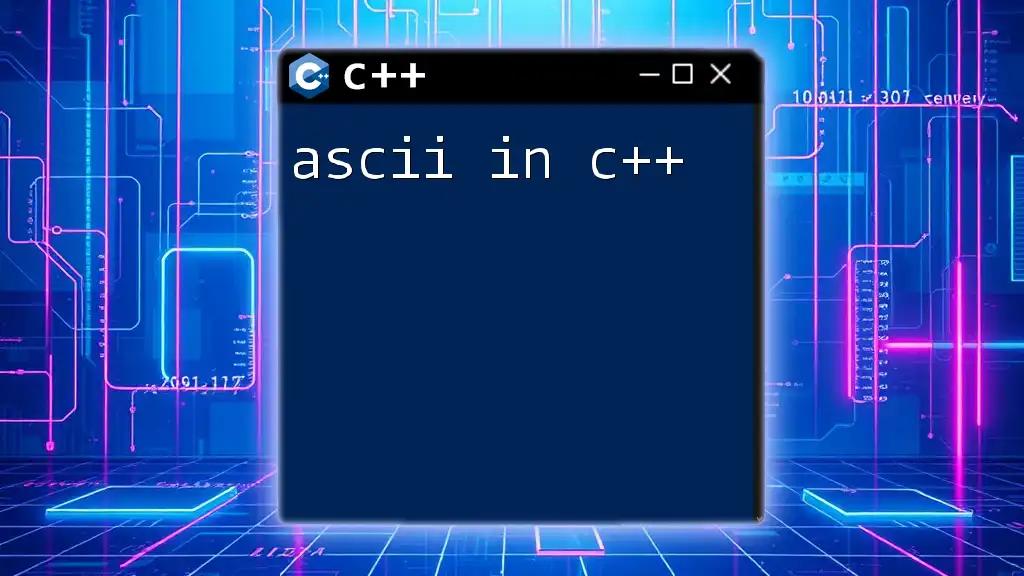
Writing Your First C++ Script
Basic Structure of a C++ Script
Every C++ script typically begins with the inclusion of header files and defining the `main()` function. Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this script, we include the `<iostream>` library for input-output operations. The `main()` function is the entry point of any C++ program. The `std::cout` statement outputs text to the console.
Compiling and Running Your C++ Script
Compiling your C++ script can be done through the command line or the IDE itself. For example, if you save the script above as `hello.cpp`, you can compile it using the following command:
g++ hello.cpp -o hello
To run the compiled script, you would enter:
./hello
If you encounter any errors during compilation, the compiler will provide feedback, allowing you to troubleshoot and correct issues effectively.

Key Features of C++ Scripting
Variables and Data Types
C++ supports a variety of variables and data types. Understanding these is crucial for effective scripting. You can declare variables as follows:
int number = 5;
double pi = 3.14;
char letter = 'A';
Knowing how to utilize different data types will enhance your scripting capabilities and ensure efficient memory usage.
Control Structures
Control structures govern the logic flow of your scripts. C++ supports several essential structures such as `if` statements and loops. Here’s an example of a loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop will print numbers 0 through 4 to the console. By mastering control structures, you gain the ability to manage how your script runs and interacts with input data effectively.
Functions and Scope
Functions break down your script into manageable parts and enhance reusability. You can define a simple function like this:
void greet() {
std::cout << "Welcome to C++ scripting!" << std::endl;
}
By understanding function scope—how variables are accessed within the function versus outside of it—you can write more efficient, organized code.

Advanced C++ Scripting Concepts
Object-Oriented Programming (OOP) in C++
C++ is inherently supportive of Object-Oriented Programming, allowing you to create classes and objects. Here’s a brief example of a simple class:
class Animal {
public:
void sound() {
std::cout << "Animal sounds!" << std::endl;
}
};
Utilizing OOP principles such as inheritance and polymorphism enables you to design better-structured scripts, enhancing maintainability and scalability.
Using Libraries and Frameworks
Integrating libraries can significantly expand your scripting capabilities. Libraries like Boost and SFML provide pre-built functions for various functionalities, ranging from data handling to graphics. To include a library in your script, you typically add:
#include <boost/algorithm/string.hpp>
Make sure to follow the library’s installation instructions to ensure proper linkage in your script.
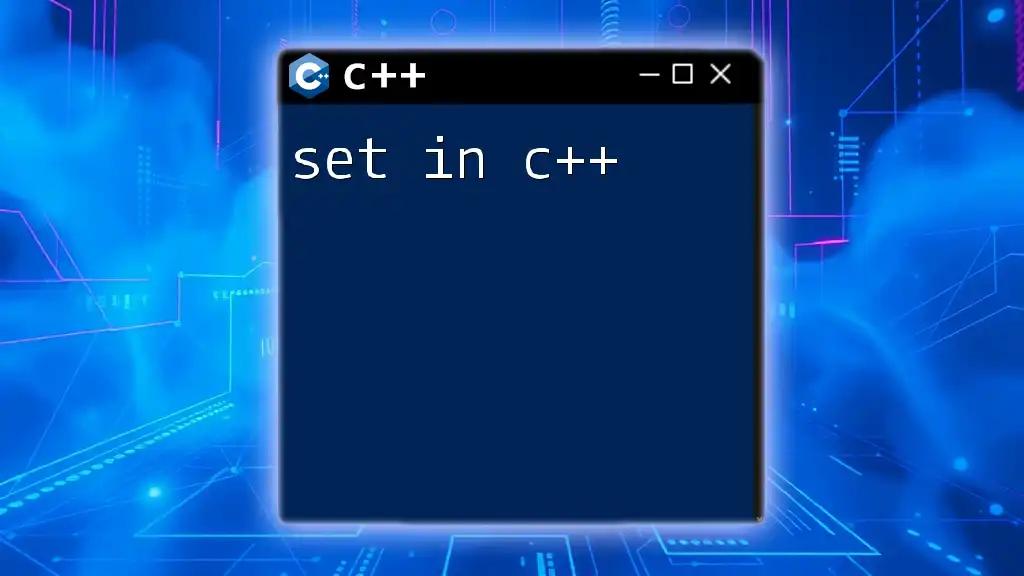
Best Practices for C++ Scripting
Writing Clean and Efficient Code
Clean coding practices are essential for maintainable scripts. Use meaningful variable names, consistent indentation, and ample comments to explain your code. For instance:
// This function calculates the area of a rectangle
double calculateArea(double width, double height) {
return width * height;
}
This level of commented clarity makes it easier for others (and future you) to understand the intentions of the code.
Debugging and Testing Your Scripts
Debugging is a crucial skill for any developer. Make use of debugging tools available in your IDE or command line, such as `gdb` for GNU or the built-in debugger in Visual Studio. Learning to write unit tests ensures your script behaves as expected—both during development and later maintenance.
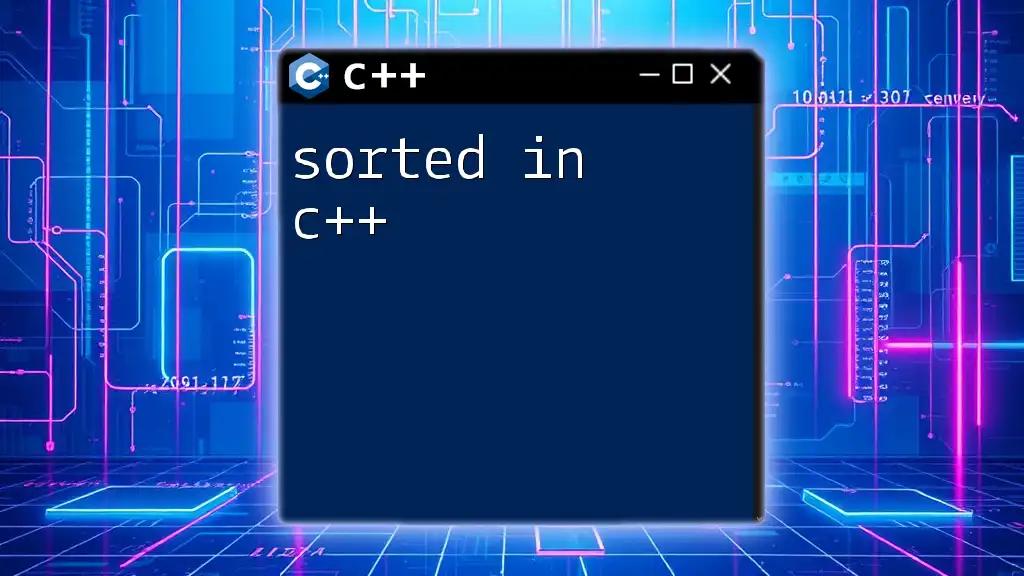
Conclusion
In summary, scripting in C++ opens up a variety of options for developers interested in enhancing their skill set. By setting up your environment properly, understanding key scripting principles, and following best practices, you can effectively harness the power of C++ for your scripting needs. We encourage you to practice, experiment, and explore the rich capabilities of C++ scripting to truly unlock your potential in programming.

Additional Resources
For further learning, consider diving into books like "The C++ Programming Language" by Bjarne Stroustrup or taking online courses focused on C++ programming and scripting. Joining communities such as Stack Overflow or Reddit's r/cpp can also provide valuable insights and support as you navigate your scripting journey.