JavaScript and C++ differ in syntax and structure, and here’s a quick example of a simple function in both languages that adds two numbers together:
int add(int a, int b) {
return a + b;
}
Understanding the Basics of JavaScript and C++
When discussing the transition from JavaScript to C++, it's essential to understand the purpose and use cases of both languages. JavaScript is primarily used for web development, enabling interactive elements in frontend applications. In contrast, C++ is renowned for its role in systems programming, game development, and applications that require high performance and close-to-hardware manipulation.
Syntax Differences
Although both languages are popular, their syntax and style can differ significantly. JavaScript uses a more flexible syntax that permits dynamic typing, whereas C++ requires static typing, demanding explicit declaration of variable types. This difference can initially be confusing for developers transitioning from JavaScript to C++, as the expectation of flexibility in JavaScript might lead to unintended errors in C++.
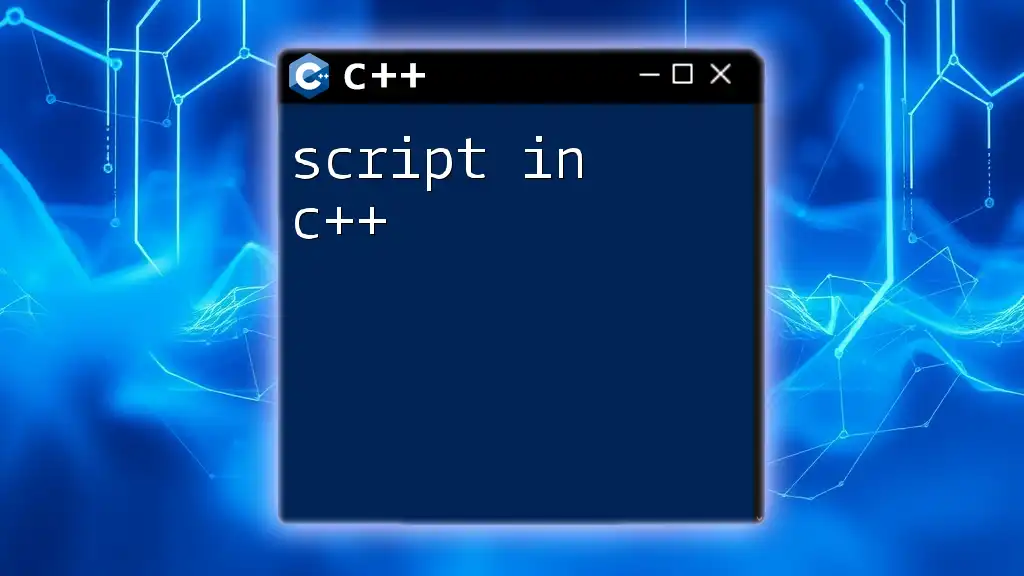
Setting Up Your Environment
To effectively start programming in C++, you need the right tools. You can choose from various Integrated Development Environments (IDEs) such as:
- Visual Studio
- CLion
- Code::Blocks
These IDEs simplify the experience with features like syntax highlighting and code suggestions.
Next, install the C++ compilers: GCC for Linux/Mac users or MSVC for Windows users. This setup forms the foundation for compiling and running your C++ programs.
Writing and Compiling Your First C++ Program
Once your environment is ready, let's create a simple "Hello, World!" program to get a feel for C++ syntax.
Here’s how you can do it:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This program demonstrates the basic structure of a C++ application, including headers, the `main` function, and output to the console.
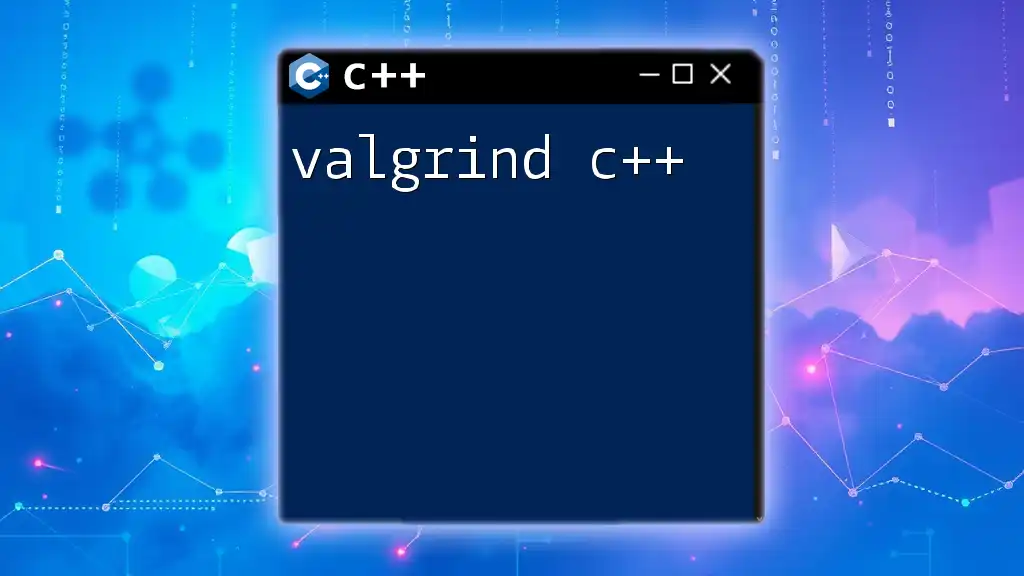
Language Fundamentals: Core Concepts
Variables and Data Types
One of the first fundamental concepts to grasp in transitioning from JavaScript to C++ is how variables and data types are defined and used in both languages.
JavaScript Variables
In JavaScript, variables can be declared using `var`, `let`, or `const`, and the language supports dynamic typing.
Example:
let number = 5;
Here, `number` is implicitly defined as an integer.
C++ Variables
In C++, however, you must declare the type of a variable explicitly, as the language employs static typing, which can help catch type-related errors at compile time.
Example:
int number = 5;
This declaration ensures that the variable `number` is specifically of type `int`.
Control Structures
Control structures are essential for dictating the flow of a program, and both JavaScript and C++ provide similar constructs, though they have different syntax.
Conditional Statements
In JavaScript, you can create conditional statements using if-else constructs. Here’s a typical example:
if (x > 5) {
// do something
} else {
// do something else
}
In C++, the syntax is quite similar, maintaining a logical flow:
if (x > 5) {
// do something
} else {
// do something else
}
Loops
Both JavaScript and C++ support loops, such as for-loops and while-loops, albeit with different syntax nuances.
JavaScript For Loop Example:
for (let i = 0; i < 5; i++) {
// loop body
}
C++ For Loop Example:
for (int i = 0; i < 5; i++) {
// loop body
}
You can see that the syntax is quite similar, making this transition relatively smooth.
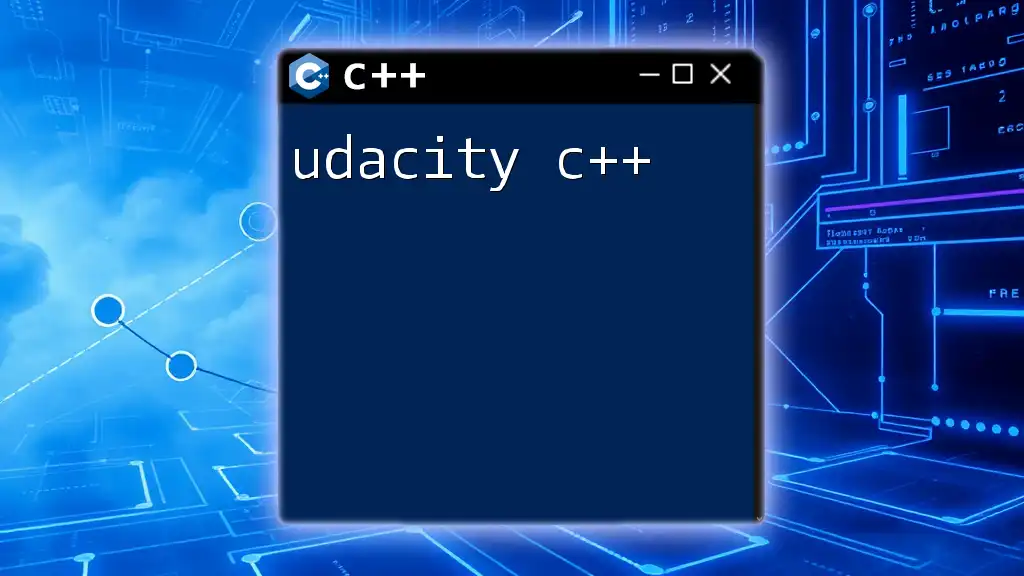
Advanced Topics: Object-Oriented Programming
Understanding Object-Oriented Programming is crucial when making the shift from JavaScript to C++. Both languages support OOP; however, their implementations can differ drastically.
Classes and Objects
JavaScript Class Syntax
In JavaScript, you define classes using the `class` keyword and work with prototype-based inheritance. An example looks like this:
class Person {
constructor(name) {
this.name = name;
}
}
C++ Class Syntax
C++ class syntax is similarly straightforward, but it differs in providing public and private access specifiers and the need for explicit constructors.
Example:
class Person {
public:
string name;
Person(string n) : name(n) {}
};
Here, the constructor is explicitly defined to initialize the `name` variable.
Constructors and Destructors
Both languages utilize constructors, but C++ also includes destructors for managing memory. In C++, you might have:
~Person() {
// destructor code
}
This destructor ensures proper cleanup of resources when an object is no longer needed, which is especially important in C++ due to its manual memory management requirements.
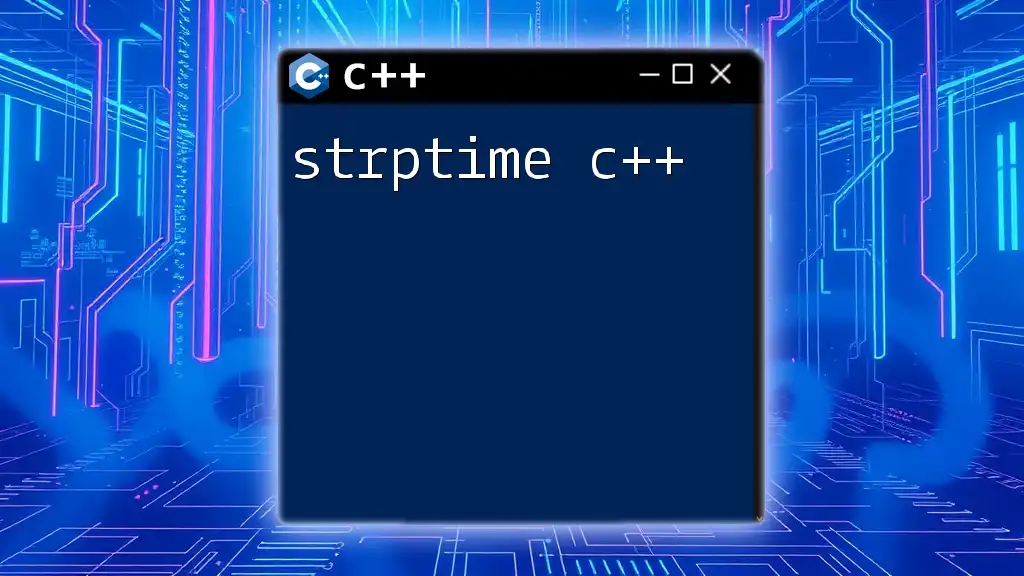
Function Differences Between JavaScript and C++
Functions serve as the building blocks of any programming language. Comparing them reveals more differences between JavaScript and C++.
Function Declaration
JavaScript Function Syntax
JavaScript functions can be defined in several ways, but here’s a classic syntax using the `function` keyword:
function greet() {
// code
}
C++ Function Syntax
In C++, functions require an explicit return type defined before the function name:
void greet() {
// code
}
This distinction underscores the necessity of specifying return types in C++ for robust type-checking.
Parameters and Return Types
Function parameters and return types also exhibit differences. While JavaScript allows ease of flexibility, with default parameters and no strict type checks, C++ requires matching the types precisely defined in the function declaration. For example:
JavaScript Example:
function add(a, b) {
return a + b;
}
C++ Equivalent:
int add(int a, int b) {
return a + b;
}
In C++, failure to match parameter types will lead to compile-time errors.
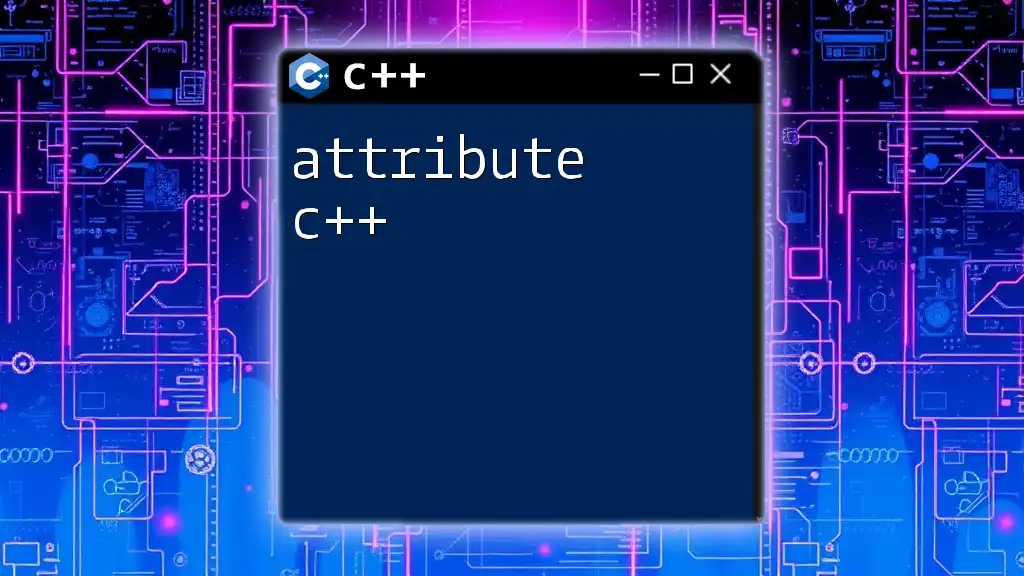
Memory Management
One of the most significant distinctions between JavaScript and C++ is memory management. JavaScript employs an automatic garbage collection mechanism, which helps manage memory for you. In contrast, C++ demands a more hands-on approach.
Manual vs Automatic
In C++, you allocate and deallocate memory manually using `new` and `delete`, ensuring optimal performance. With this comes the responsibility of managing memory efficiently to prevent memory leaks.
For instance, you might allocate memory as follows:
int* p = new int(5); // dynamically allocated memory
delete p; // free the memory
Failing to free the memory leads to memory leaks, which can severely impact application performance.
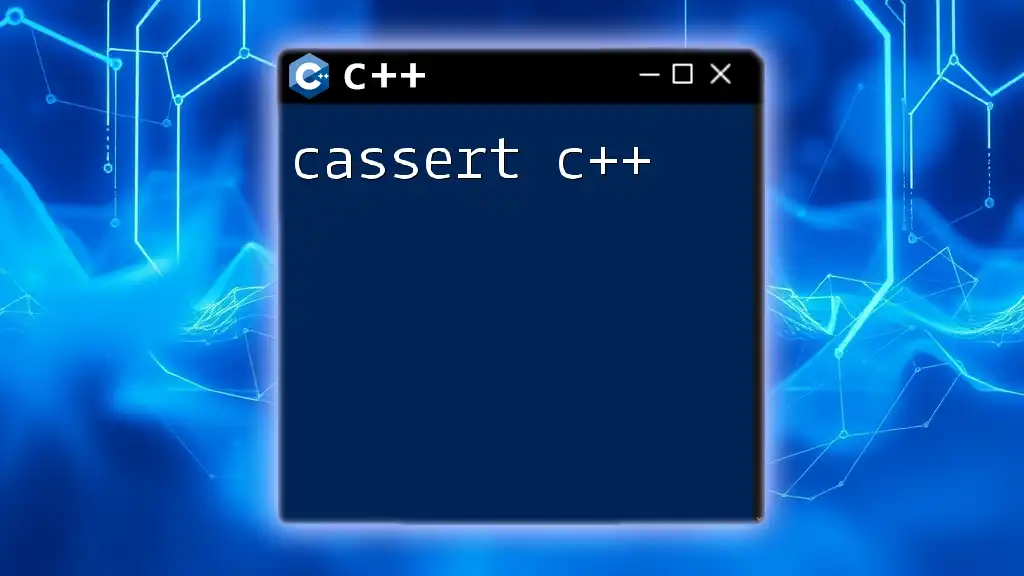
Conclusion
Transitioning from JavaScript to C++ opens up new opportunities in software development, from performance-critical applications to system-level programming. While there are significant differences in syntax and features between the two languages, understanding these contrasts can empower JavaScript developers to master C++.
The journey will require practice and continuous learning. By leveraging the resources discussed, you can solidify your understanding and become proficient in C++. Happy coding!