Java and C++ share similar syntax and object-oriented principles, but differ significantly in memory management and platform independence. Here’s a basic example demonstrating a simple class definition in both languages:
// C++
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
// Java
class Dog {
void bark() {
System.out.println("Woof!");
}
}
Overview of C++ and Java
What is C++?
C++ is a powerful, high-performance programming language originally developed by Bjarne Stroustrup in the late 1970s. It builds upon the C programming language and introduces additional features such as object-oriented programming (OOP). C++ is widely respected for its ability to manage system resources, offering developers fine-grained control over memory and performance.
Key features of C++ include:
- Object-Oriented Features: Support for classes, inheritance, polymorphism, and encapsulation.
- Performance Capabilities: Known for its speed, C++ is commonly used in applications where performance is critical, such as gaming, real-time simulations, and system software.
Here is an example of creating a simple class in C++:
#include <iostream>
using namespace std;
class Animal {
public:
void sound() {
cout << "Animal sound" << endl;
}
};
What is Java?
Java, developed by Sun Microsystems in 1995, is an object-oriented programming language designed to be platform-independent. It allows developers to write code once and run it anywhere, thanks to the Java Virtual Machine (JVM). Java emphasizes security and portability, making it the language of choice for a large number of web and enterprise applications.
Key features of Java include:
- Platform Independence: Java code is compiled into bytecode, which can be executed on any device that has a JVM.
- Memory Management and Garbage Collection: Java automates memory management, reducing the risk of memory leaks.
Here's a simple example of class definition in Java:
class Animal {
void sound() {
System.out.println("Animal sound");
}
}
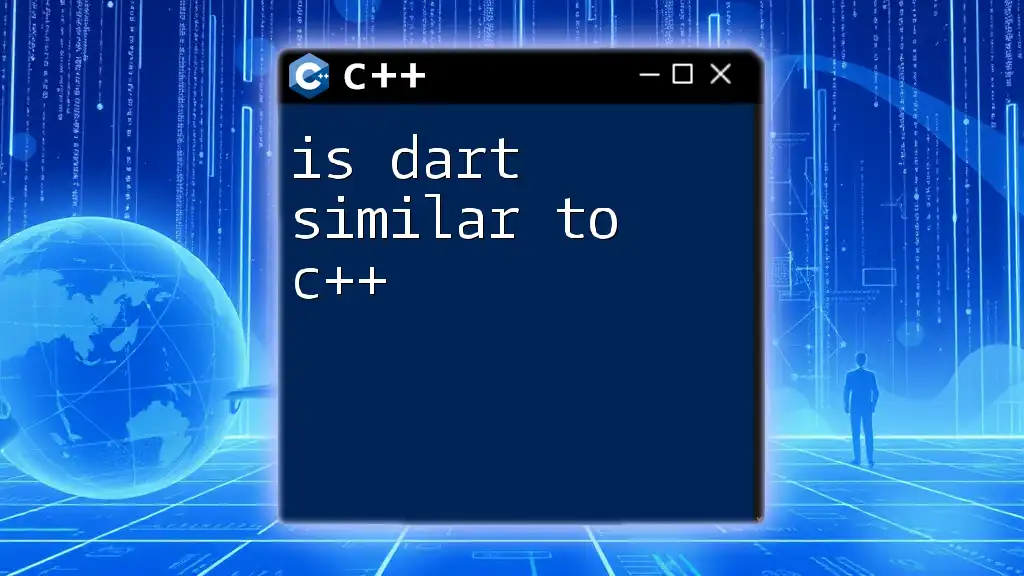
Language Syntax and Structure
Basic Syntax Comparison
Both C++ and Java share a similar syntax derived from C, making it relatively easier for programmers to transition between the two languages. For instance, class definitions, control structures, and comments look quite similar. However, there are notable differences in specifics such as method declarations and data types.
Variable Declarations and Data Types
In both languages, variables must be declared before they are used, but they differ in some aspects:
- Data Type Declarations: C++ has built-in primitive types such as `int`, `char`, `float`, etc., while Java also possesses similar primitive types but includes wrapper classes for each type to allow for object-oriented programming.
Here’s a comparison of variable declarations in both languages:
C++ Example
int a = 5;
float b = 5.5;
char c = 'A';
Java Example
int a = 5;
float b = 5.5f; // Note the 'f' suffix
char c = 'A';
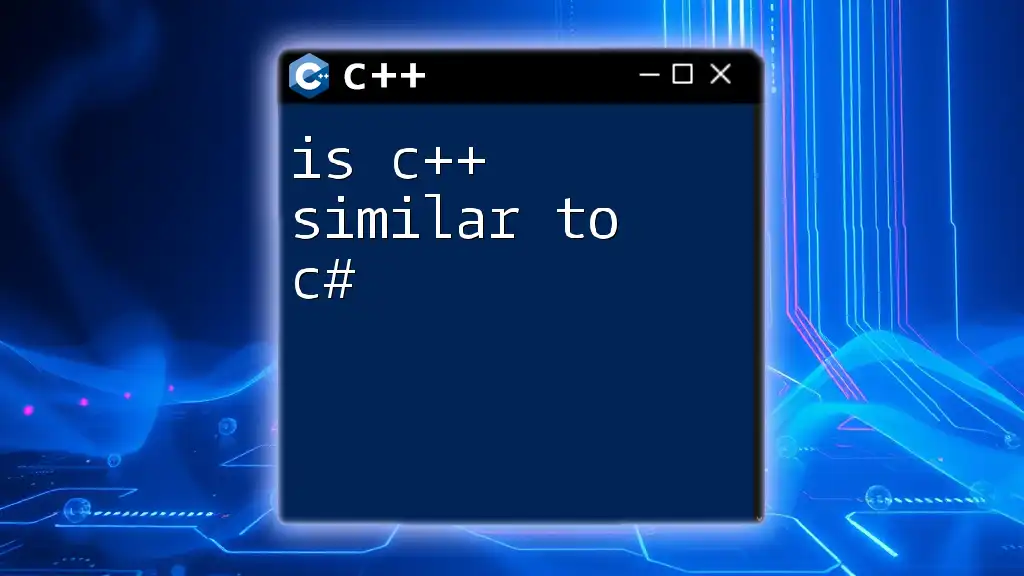
Object-Oriented Principles
Key Object-Oriented Concepts
When discussing whether Java is similar to C++, it is crucial to highlight their foundations in object-oriented programming. Both languages support the four primary principles of OOP:
- Encapsulation: Bundling the data (attributes) and methods (functions) that operate on the data within one unit (class).
- Inheritance: The ability of one class to acquire properties and methods of another.
- Polymorphism: The capability to treat objects of different classes through a common interface, often implemented via method overriding.
Here's a comparison of inheritance in both languages:
C++ Example of Inheritance
class Dog : public Animal {
public:
void sound() {
cout << "Bark" << endl;
}
};
Java Example of Inheritance
class Dog extends Animal {
void sound() {
System.out.println("Bark");
}
}
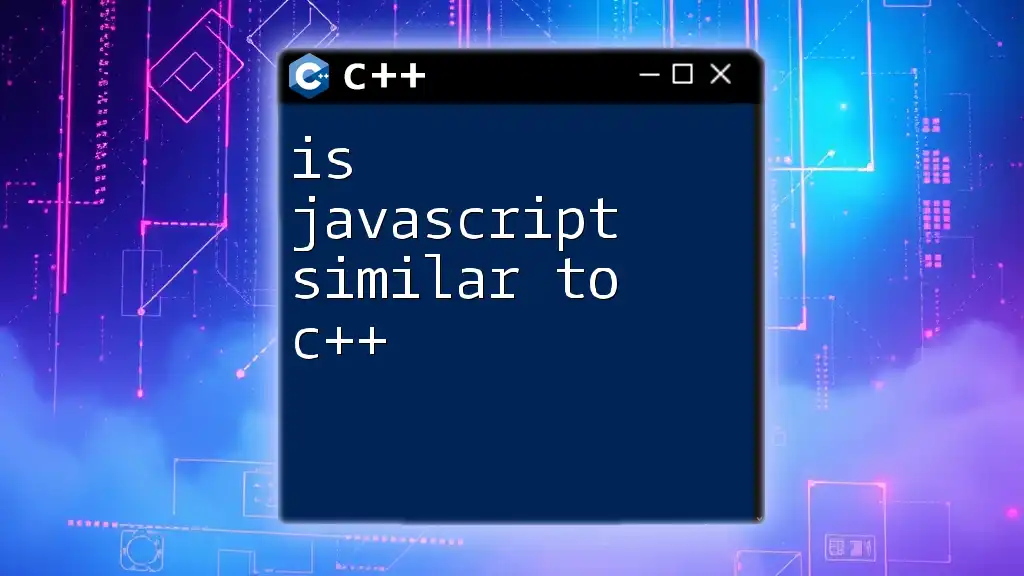
Memory Management
C++ Memory Management
In C++, memory management is a manual process. Developers must allocate and deallocate memory using `new` and `delete`. While this gives developers great control, it also increases the risk of memory leaks if not managed properly.
Example of memory management in C++:
int* arr = new int[5]; // Dynamically allocated array
delete[] arr; // Must be deleted manually
Java Memory Management
Java simplifies memory management through automatic garbage collection. This means that developers do not need to manually free memory, as the JVM handles this process. While this automation reduces the risk of memory leaks, it can introduce some overhead for the garbage collector.
Example of Java memory management:
int[] arr = new int[5]; // Dynamically allocated array
// No need to explicitly free memory
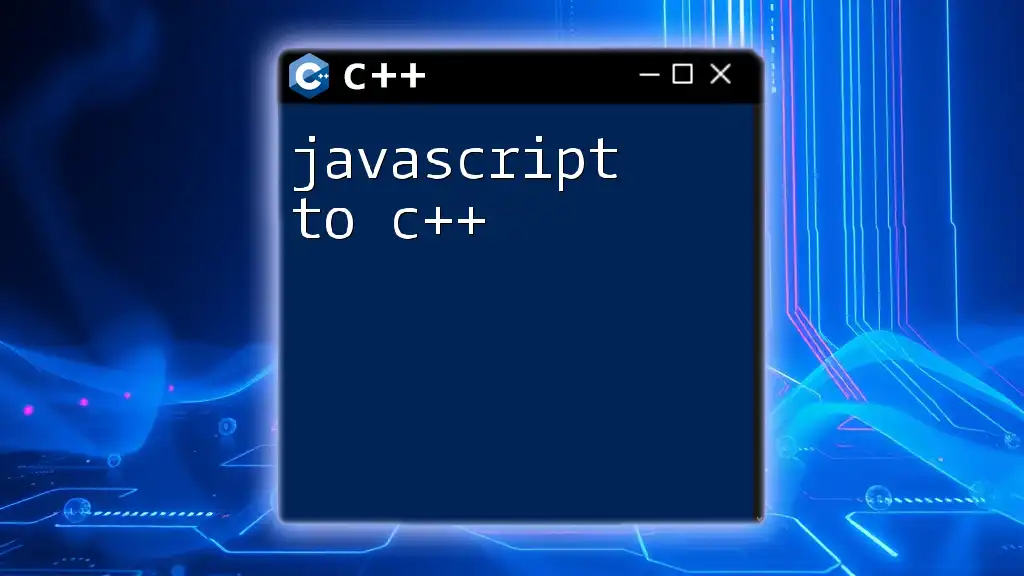
Performance Considerations
Speed and Efficiency
When considering whether Java is similar to C++, performance often comes into play. C++ is known for its execution speed and efficiency, attributed to its compiled nature, which converts code to machine language directly. This makes it suitable for performance-intensive applications.
In contrast, Java, while generally slower than C++, offers faster development times and ease of use, thanks to automatic memory management and extensive libraries. The choice often depends on the specific needs of a project.
Use Cases for C++ and Java
- C++ is used for systems programming, real-time simulation, and game development, where performance and resource management is critical.
- Java is predominantly used in web applications, mobile applications (Android), and enterprise-level applications, thriving in environments where cross-platform compatibility and rapid development are essential.
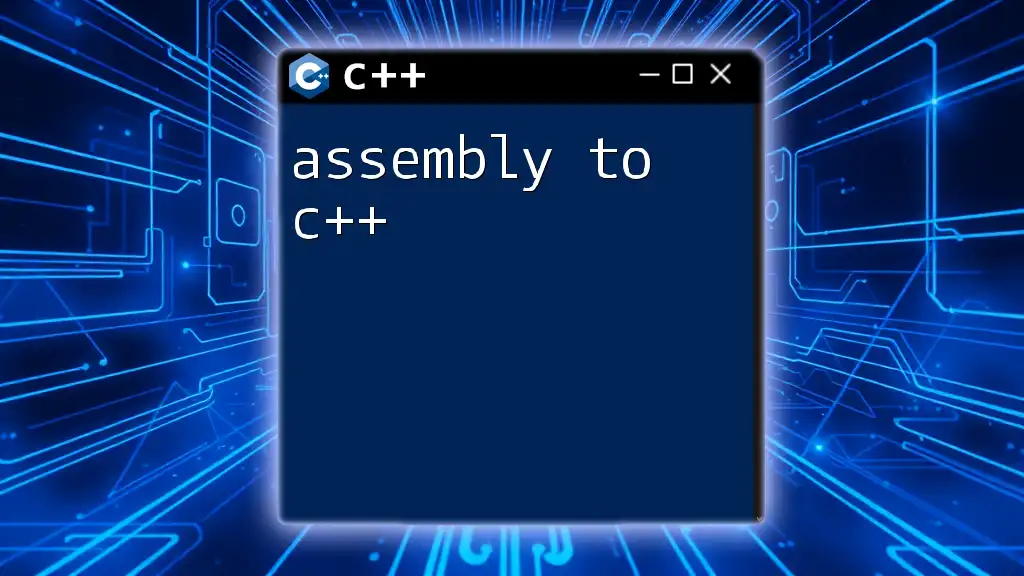
Community and Ecosystem
Libraries and Frameworks
Both languages boast a robust ecosystem of libraries and frameworks, enhancing their capabilities:
- C++ frameworks include Boost for general-purpose utilities and Qt for GUI development.
- Java frameworks such as Spring for enterprise applications and Hibernate for ORM facilitate rapid development and productivity.
Learning Resources and Support
Both C++ and Java communities are vibrant, with an abundance of resources available for learners. You can find extensive documentation, online courses, forums, and tutorials that cater to various levels of expertise in both languages.
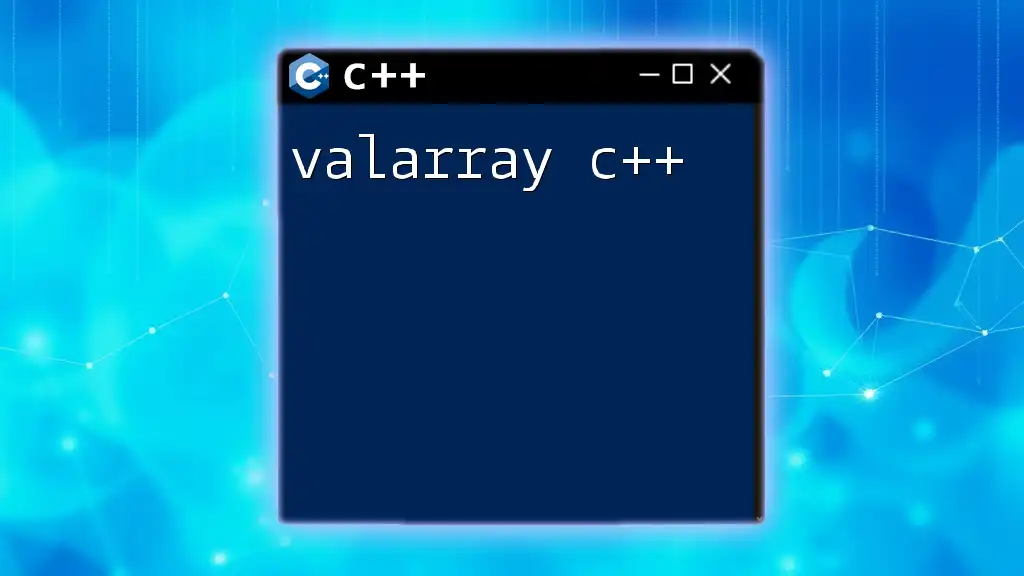
Conclusion
In summary, while there are notable similarities in syntax and object-oriented principles between C++ and Java, substantial differences exist in memory management, performance, and typical use cases. Understanding these nuances is crucial for developers deciding which language to pursue based on their project needs. Each has its strengths, making them valuable tools in the modern programming landscape. Exploring both languages can provide insights that enrich your programming career and broaden your skill set.