Here's a concise explanation along with a code snippet:
When transitioning from MATLAB to C++, simple array manipulations and mathematical operations can be implemented using standard C++ libraries, as shown below:
#include <iostream>
#include <vector>
int main() {
std::vector<double> A = {1.0, 2.0, 3.0}; // MATLAB: A = [1; 2; 3]
for (size_t i = 0; i < A.size(); ++i) {
A[i] = A[i] * 2; // MATLAB: A = A * 2
}
for (const auto& val : A) {
std::cout << val << " "; // Output: 2 4 6
}
return 0;
}
Understanding MATLAB and C++
What is MATLAB?
MATLAB, short for MATrix LABoratory, is a high-level programming language and environment designed primarily for numerical computing and data visualization. It simplifies matrix manipulations and provides a rich set of built-in functions, making it an excellent choice for engineers, scientists, and researchers who require efficient computations.
Key features of MATLAB include:
- An extensive library of functions for mathematical operations
- Powerful data visualization tools
- Ease of use with a focus on matrix operations
What is C++?
C++ is a versatile, high-performance programming language that supports procedural, object-oriented, and generic programming paradigms. Initially developed as an extension of the C programming language, C++ provides greater control over system resources and allows for intricate data structures.
Key features of C++ include:
- Enhanced performance due to lower-level programming capabilities
- Fine-grained control over memory management
- Support for object-oriented programming
Why Convert MATLAB to C++?
The transition from MATLAB to C++ can provide several benefits:
- Performance advantages: C++ can execute code much faster than interpreted MATLAB scripts, especially for computationally intensive operations.
- Integration with other systems: C++ can seamlessly interface with hardware and external libraries, making it ideal for embedded systems and applications requiring high efficiency.
- Need for portability: C++ code can be compiled across different platforms, while MATLAB relies on its specific environment.
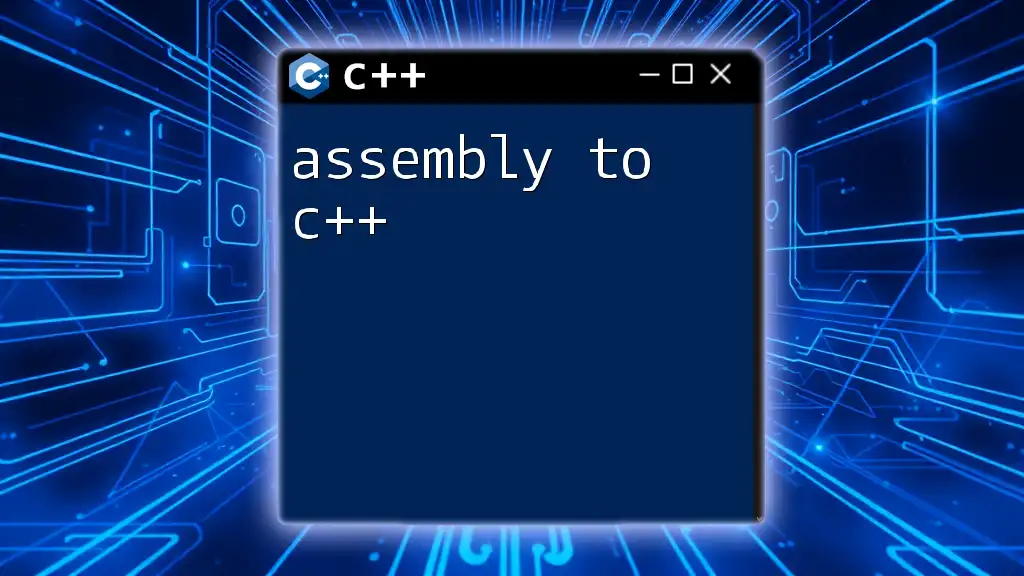
Fundamental Differences Between MATLAB and C++
Syntax Differences
MATLAB syntax is designed to be intuitive for users, particularly when dealing with arrays and matrices. C++, in contrast, has a more complex syntax that requires more explicit declarations.
For example, in MATLAB, declaring and initializing a variable could look like this:
a = 5;
b = [1, 2, 3; 4, 5, 6];
In C++, the equivalent would require explicit data types and semicolons:
int a = 5;
int b[2][3] = {{1, 2, 3}, {4, 5, 6}};
Data Types and Structures
MATLAB simplifies the handling of data types, primarily focusing on matrices. C++ offers a broader range of data types, including primitives (like `int`, `float`) and complex data structures (like `std::vector`).
Example: Converting a MATLAB matrix to a C++ `std::vector` might look like this:
A = [1, 2, 3; 4, 5, 6];
Translating this into C++ would involve:
#include <vector>
std::vector<std::vector<int>> A {{1, 2, 3}, {4, 5, 6}};
Control Structures
The control flow mechanisms in both languages are somewhat similar, but with differences in syntax.
In MATLAB, a basic for loop may be structured as follows:
for i = 1:5
disp(i);
end
This translates to C++ as:
#include <iostream>
for (int i = 1; i <= 5; i++) {
std::cout << i << std::endl;
}
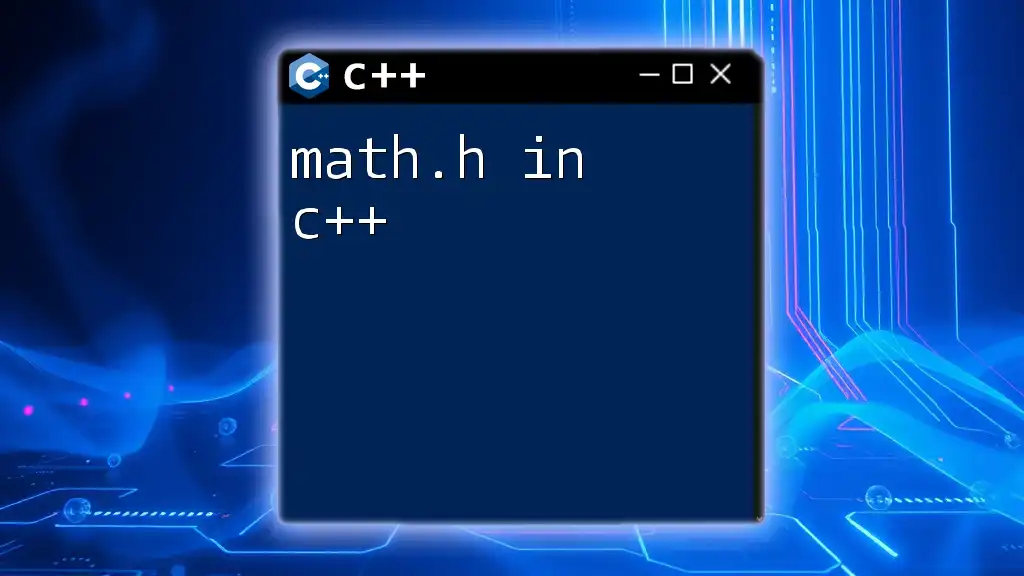
Translating Basic MATLAB Commands to C++
Variables and Arrays
In both MATLAB and C++, you need to declare variables, but C++ requires specifying types explicitly. The initialization of arrays differs as well.
Example: Creating an array in MATLAB:
arr = [1, 2, 3, 4];
Would look like this in C++:
int arr[] = {1, 2, 3, 4};
Functions
Defining functions in MATLAB is straightforward:
function result = factorial(n)
if n == 0
result = 1;
else
result = n * factorial(n - 1);
end
end
In C++, the same factorial function would be written as:
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
Control Flow Constructs
Similar to basic loops, conditional constructs can easily be converted.
For instance, an if-statement in MATLAB:
if a > b
disp('A is greater than B');
else
disp('A is not greater than B');
end
In C++:
if (a > b) {
std::cout << "A is greater than B" << std::endl;
} else {
std::cout << "A is not greater than B" << std::endl;
}
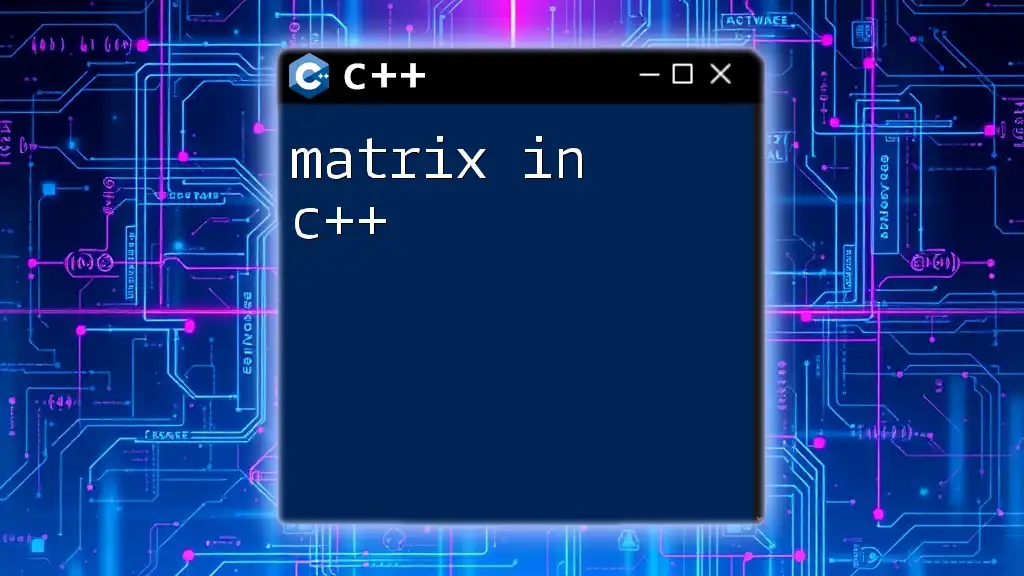
Advanced Topics in Conversion
Object-Oriented Programming Concepts
While both languages support object-oriented programming, their implementations differ. In MATLAB, defining a class is more streamlined, focusing on the data-centric model. Contrarily, C++ requires explicit declarations of member functions and attributes.
Example of a simple class in MATLAB:
classdef MyClass
properties
Value;
end
methods
function obj = MyClass(v)
obj.Value = v;
end
function displayValue(obj)
disp(obj.Value);
end
end
end
The corresponding class in C++ looks like:
class MyClass {
public:
int Value;
MyClass(int v) : Value(v) {}
void displayValue() {
std::cout << Value << std::endl;
}
};
Using Libraries
MATLAB offers various built-in toolboxes, whereas C++ relies on external libraries. A powerful library for matrix operations in C++ is Eigen. To use it, you must install and include the library:
#include <Eigen/Dense>
using namespace Eigen;
int main() {
MatrixXd m(2, 2);
m(0, 0) = 3;
m(1, 0) = 2.5;
m(0, 1) = -1;
m(1, 1) = 0.5;
std::cout << m << std::endl;
}
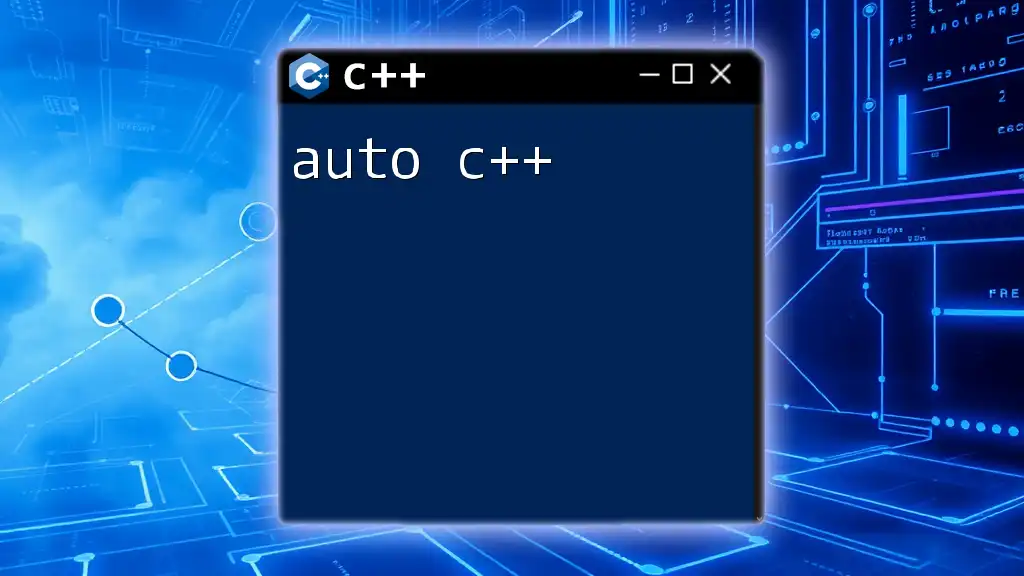
Performance Optimization Techniques
Profiling and Benchmarking
To optimize performance in both environments, profiling is essential. MATLAB provides the `profile` function, while C++ can utilize tools like gprof or Valgrind. Profiling helps identify bottlenecks in code, allowing for targeted improvements.
Memory Management
C++ requires more attention to memory management than MATLAB. While MATLAB automatically manages memory, C++ developers must allocate and deallocate memory manually.
For example, manual memory management might look like this in C++:
int* arr = new int[5]; // Dynamic allocation
// Use arr...
delete[] arr; // Manual deallocation
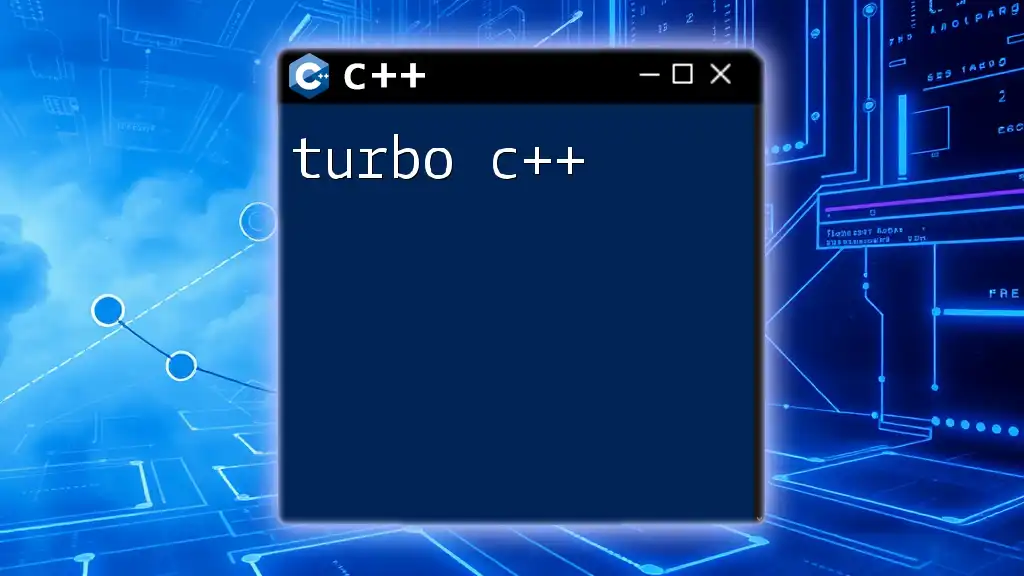
Common Pitfalls to Avoid
Type Compatibility Issues
Common mistakes arise from type compatibility, especially when dealing with integer and floating-point types. Be cautious of division operations; in C++, dividing two integers will result in an integer. To avoid unintended truncation, ensure at least one operand is a floating-point number:
double result = a / static_cast<double>(b); // Correct approach
Debugging Differences
Debugging in MATLAB is more user-friendly due to its interactive environment. In C++, developers utilize debuggers like gdb. Familiarize yourself with debugging tools in C++ to effectively troubleshoot your code.
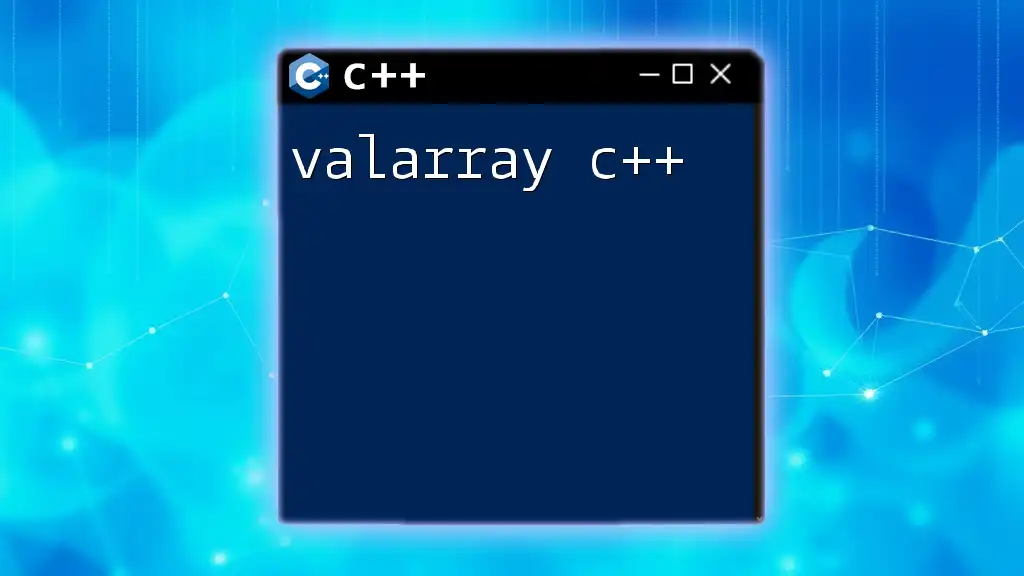
Conclusion
Transitioning from MATLAB to C++ can be challenging but rewarding. By understanding the fundamental differences in syntax, data structures, and programming paradigms, you can successfully convert your MATLAB scripts into efficient C++ code.
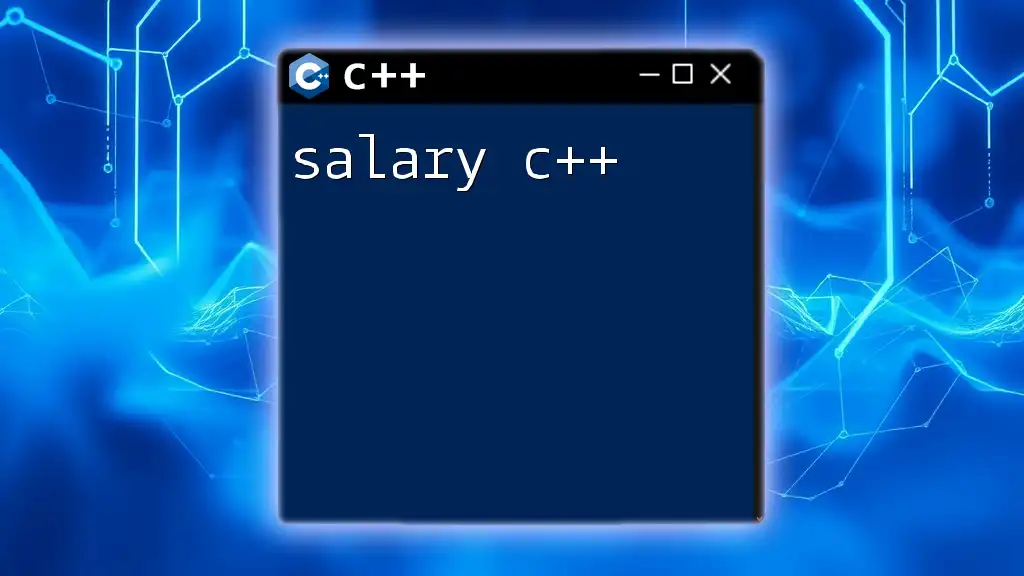
Additional Resources
To deepen your understanding of converting MATLAB code to C++, consider exploring relevant textbooks, online courses, and tutorials dedicated to both languages. Additionally, utilizing online compilers can help you practice and refine your skills in real-time.
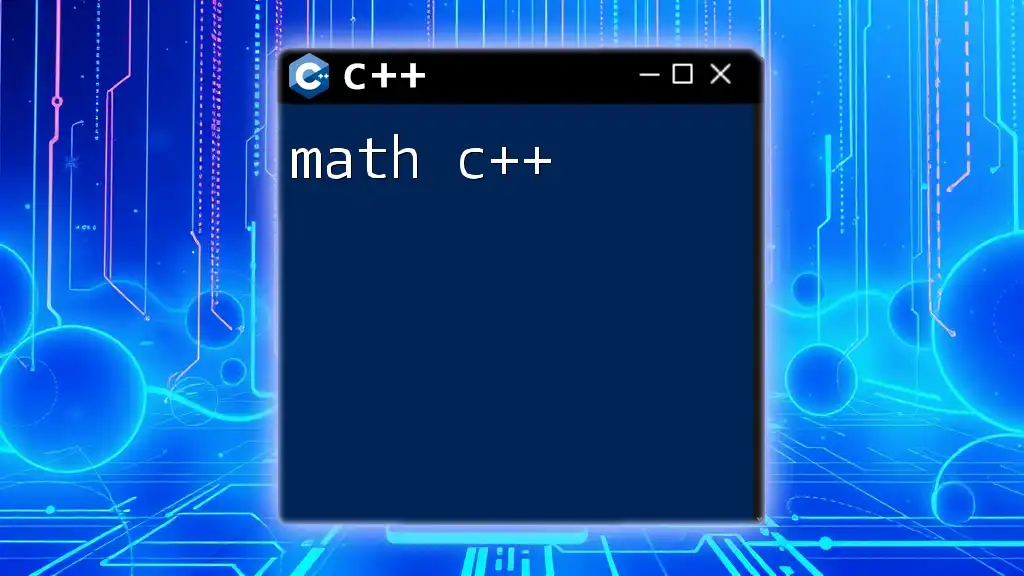
Call to Action
If you found this guide helpful and want to continue mastering the transition from MATLAB to C++, be sure to subscribe to our blog for more tutorials and insights. Join our upcoming courses to enhance your coding expertise and tackle complex programming challenges!