In C++, mathematical operations can be performed using built-in operators for addition, subtraction, multiplication, and division, as demonstrated in the following code snippet:
#include <iostream>
int main() {
int a = 10;
int b = 5;
std::cout << "Addition: " << (a + b) << "\n";
std::cout << "Subtraction: " << (a - b) << "\n";
std::cout << "Multiplication: " << (a * b) << "\n";
std::cout << "Division: " << (a / b) << "\n";
return 0;
}
Understanding the C++ Math Library
The C++ Math Library provides essential mathematical functions that can help with various calculations in programming. It is important to include this library to access a wide range of functions that can be utilized in mathematical computations. You can include the library in your C++ program like this:
#include <cmath>
Components of the Math Library
The math library is rich in mathematical functions that allow for a variety of operations, such as trigonometric calculations, powers, logarithms, and more. Understanding these components is critical for any programming task that involves mathematical computations.
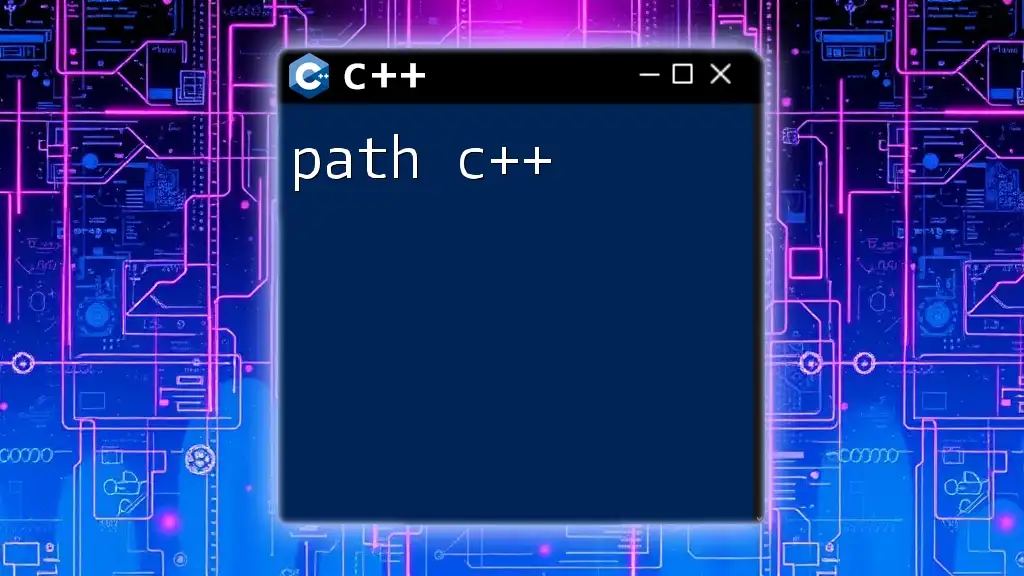
Basic Mathematical Operations in C++
Arithmetic Operations
In C++, basic arithmetic operations are straightforward and can be performed using standard operators: `+`, `-`, `*`, and `/`.
For example, consider the following code snippet that demonstrates these operations:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 5;
cout << "Addition: " << (a + b) << endl;
cout << "Subtraction: " << (a - b) << endl;
cout << "Multiplication: " << (a * b) << endl;
cout << "Division: " << (a / b) << endl;
return 0;
}
This program clearly shows how to perform basic mathematical operations in C++.
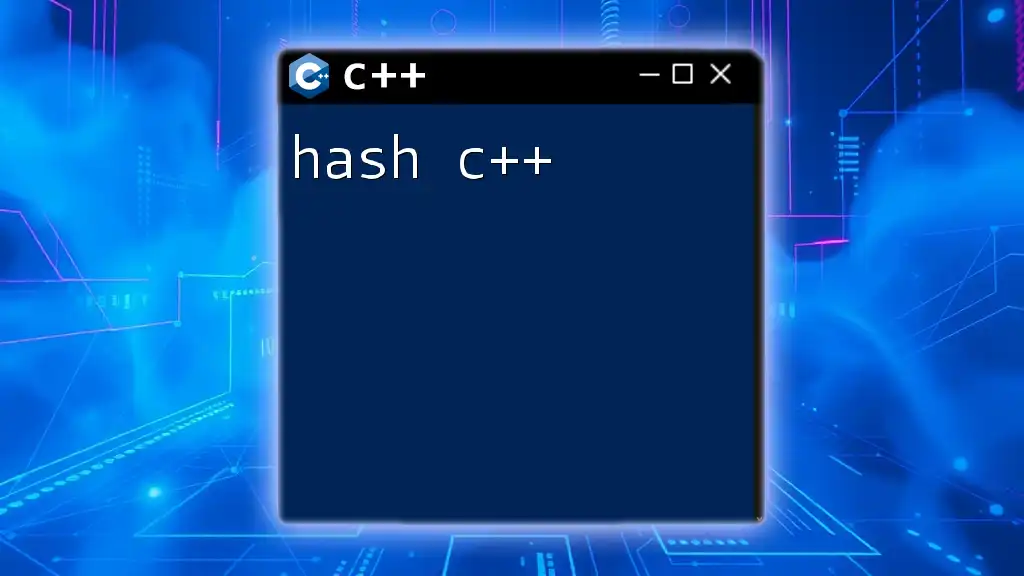
Common C++ Mathematical Functions
Overview of Common Functions
The C++ math library includes a variety of mathematical functions that are essential for performing complex calculations. Some of the most common functions include:
- sqrt(x) – Calculates the square root of x.
- pow(base, exponent) – Raises the base to the power of the exponent.
- sin(x), cos(x), and tan(x) – Calculate the sine, cosine, and tangent of x.
These functions make it easier to handle common mathematical calculations without needing to implement them manually.
Utilizing Math Functions in C++
Square Root Function
The square root function `sqrt` is particularly useful when dealing with quadratic equations or calculations involving areas. Here’s a simple example:
double num = 25.0;
cout << "Square root of " << num << " is " << sqrt(num) << endl;
In this case, the program computes the square root of 25 and outputs the result.
Power Function
Another powerful function is `pow`, which allows raising numbers to a specific power. For instance:
double base = 2.0, exponent = 3.0;
cout << base << " raised to the power of " << exponent << " is " << pow(base, exponent) << endl;
This snippet calculates 2 raised to the power of 3, resulting in 8.
Advanced Trigonometric Functions
C++ provides trigonometric functions that allow us to perform calculations involving angles, which are critical in fields like physics and engineering. Functions such as `sin`, `cos`, and `tan` take an angle in radians. To convert degrees to radians, you can use the formula `radians = degrees * (M_PI / 180.0)`.
Here is an example that demonstrates how to use the sine function:
double angle = 30.0; // angle in degrees
double radians = angle * (M_PI / 180.0); // converting degrees to radians
cout << "Sine of " << angle << " degrees is " << sin(radians) << endl;
The above code will output the sine value of 30 degrees.
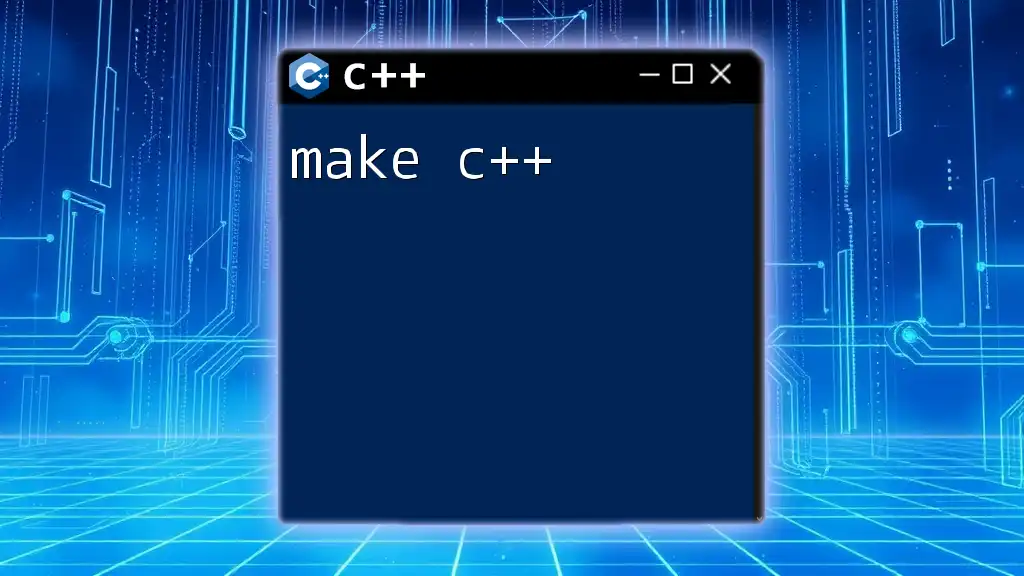
Handling Special Mathematical Cases
Dealing with Negative and Zero Values
When utilizing mathematical functions, special cases such as negative numbers and zero must be addressed, as they can lead to errors or unexpected results. For example, attempting to calculate the square root of a negative number will yield an undefined result.
Here’s how you can handle such cases in your code:
double num = -25.0;
if (num < 0) {
cout << "Cannot compute square root of negative number!" << endl;
} else {
cout << "Square root: " << sqrt(num) << endl;
}
The snippet above checks if the number is negative before attempting to calculate the square root.
NaN (Not a Number) and Infinity
When performing mathematical operations, situations can arise where the result is not defined, such as dividing zero by zero. In these cases, C++ returns NaN (Not a Number). Here’s a quick demonstration:
cout << "0 divided by 0 gives: " << 0.0 / 0.0 << endl; // Outputs NaN
Handling these special cases is crucial for ensuring that your program runs smoothly without errors.
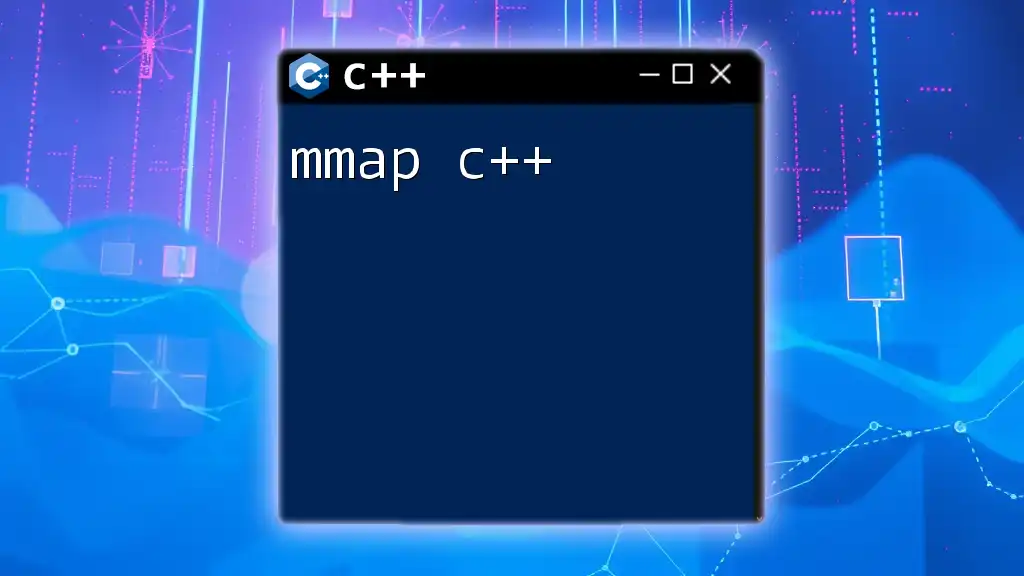
Building a Simple Math Application
Concept of a Calculator in C++
One of the practical applications of the math functions is to build a simple calculator that performs basic arithmetic operations. Such a project can help you solidify your understanding of how to utilize the math functions effectively in C++.
Example Code for a Basic Calculator
Below is an example of a basic command-line calculator that accepts user input for two numbers and an operator. It demonstrates the use of several math functions based on user input:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
char operation;
double num1, num2;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter an operator (+, -, *, /, ^): ";
cin >> operation;
cout << "Enter second number: ";
cin >> num2;
switch(operation) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if (num2 != 0)
cout << num1 / num2;
else
cout << "Division by zero is not allowed!";
break;
case '^':
cout << pow(num1, num2);
break;
default:
cout << "Invalid operator!";
}
return 0;
}
This code allows users to perform addition, subtraction, multiplication, division, and exponentiation, showcasing the use of mathematical functions in a practical context.
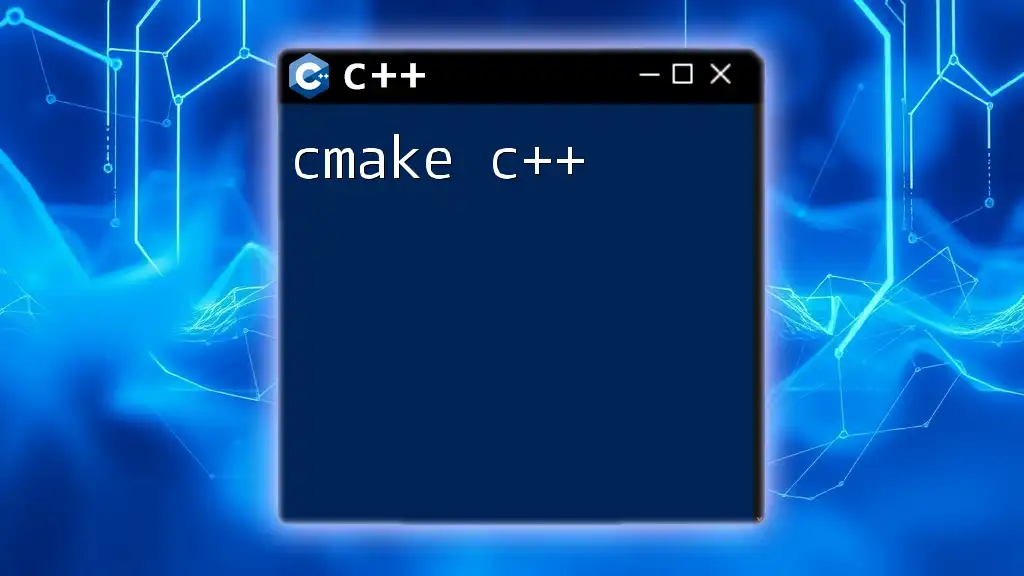
Conclusion
In summary, understanding math in C++ is crucial for anyone looking to enhance their programming skills. The C++ math library provides a robust set of functions that can simplify a wide array of mathematical computations, making it an invaluable asset in your programming toolkit. Experiment with these functions and consider building your own applications to gain hands-on experience.
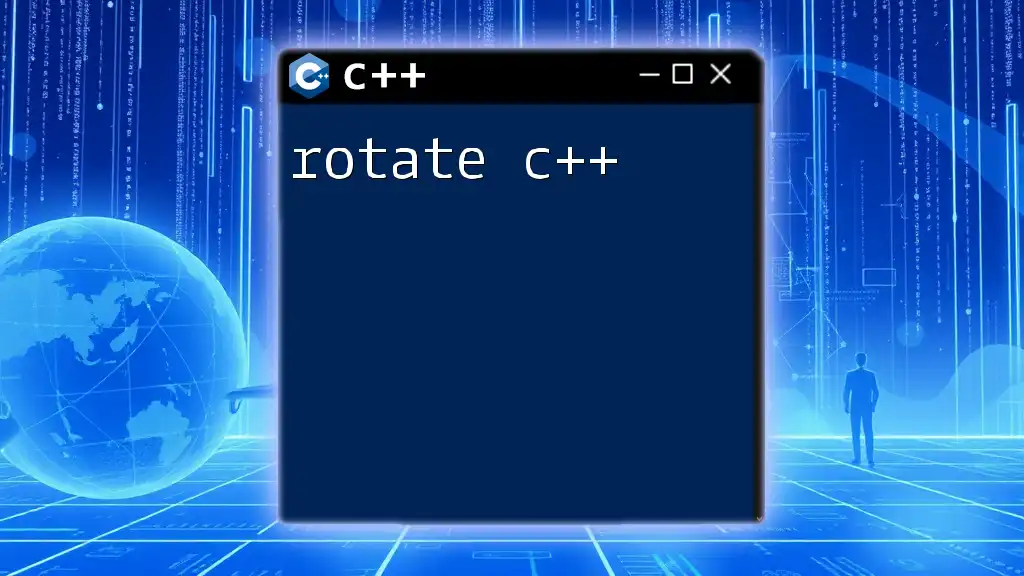
Additional Resources
For deeper exploration, refer to the official C++ documentation on the math library and seek out books or online courses aimed at furthering your knowledge of C++ mathematics. With these tools and knowledge, you’ll be well-equipped to tackle a variety of programming challenges that require mathematical understanding and application. Happy coding!