In C++, the `std::filesystem::path` class is utilized for manipulating and representing file paths in a portable manner across various operating systems.
Here's a simple example of how to create and manipulate a file path using the `std::filesystem` library:
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path filePath = "/home/user/documents/example.txt";
std::cout << "Filename: " << filePath.filename() << std::endl;
std::cout << "Parent Path: " << filePath.parent_path() << std::endl;
return 0;
}
What is a Path in C++?
A path in C++ refers to the string representation of the location of a file or directory in a file system. Understanding paths is crucial as they help developers locate resources, navigate the file structure, and manage file I/O operations effectively. A correctly used path ensures that your code is portable, reliable, and efficient, which is especially important when sharing your code across different operating systems or development environments.
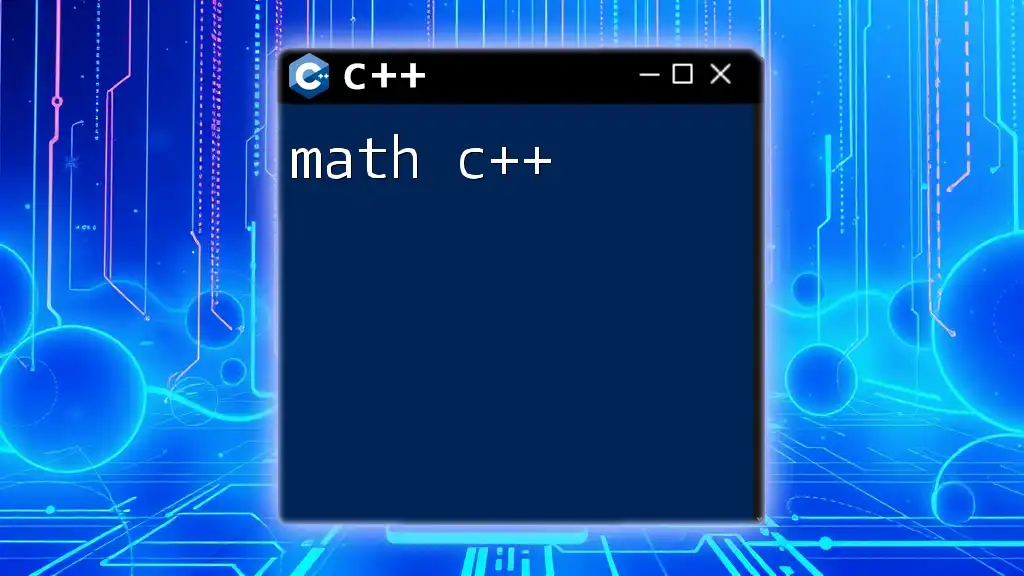
Why Understanding Paths is Essential
Having a strong grasp of how paths work can enhance project organization, allowing developers to manage files and directories efficiently. Proper path management also helps to avoid common pitfalls associated with file handling, such as file not found errors or access denied issues. By mastering path usage, you can make your applications robust and user-friendly.
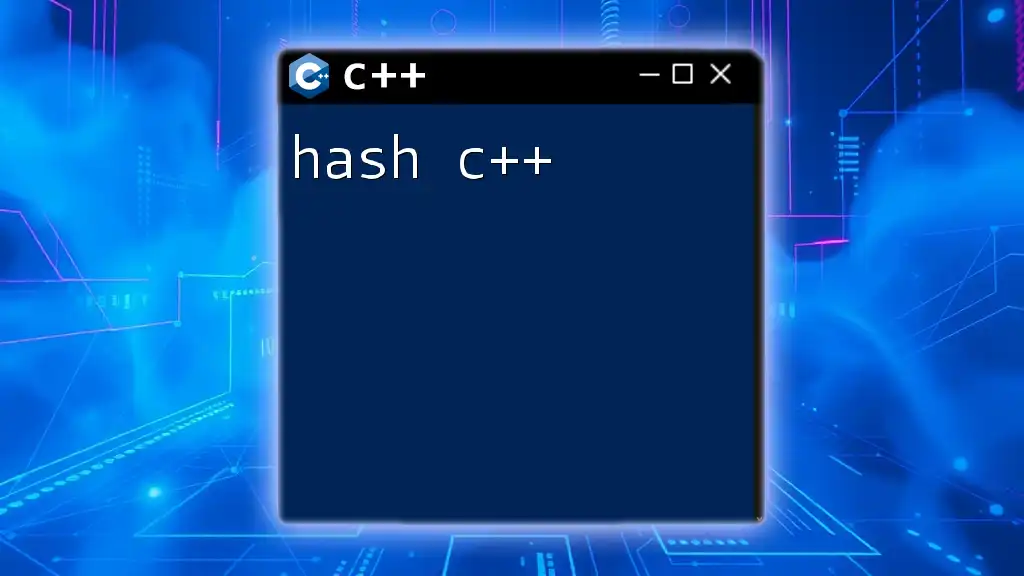
Types of Paths in C++
Absolute and Relative Paths
Understanding Absolute Paths
An absolute path provides the complete directory location from the root directory to the specific file. This path remains constant regardless of the current working directory. For example, on a Windows system, an absolute path might look like:
C:\Users\Username\Documents\file.txt
On a Unix-like system, an absolute path would be:
/home/username/documents/file.txt
Understanding Relative Paths
In contrast, a relative path specifies the location of a file in relation to the current working directory. This path can change depending on where the execution context of the program is. For example, in a C++ project, a relative path can be specified as follows from the main file of your project:
std::ifstream file("data/input.txt");
Here, the compiler will look for the `input.txt` file inside the `data` directory located relative to the working directory.
File System Paths
Directory Paths and Their Structure
Paths are structured differently across operating systems. Understanding this structure is essential for navigating and manipulating files effectively. For example, Windows uses backslashes (`\`) while Unix-like systems use forward slashes (`/`). Properly managing these differences can greatly simplify cross-platform development.
Special Folders in Path Management
Using Special Directory Keywords
The `..` refers to the parent directory, and `.` refers to the current directory. These keywords can be incredibly useful for navigating the directory structure without needing to specify the full path. For instance, you can access a file in the parent directory like this:
std::ifstream file("../data/input.txt");
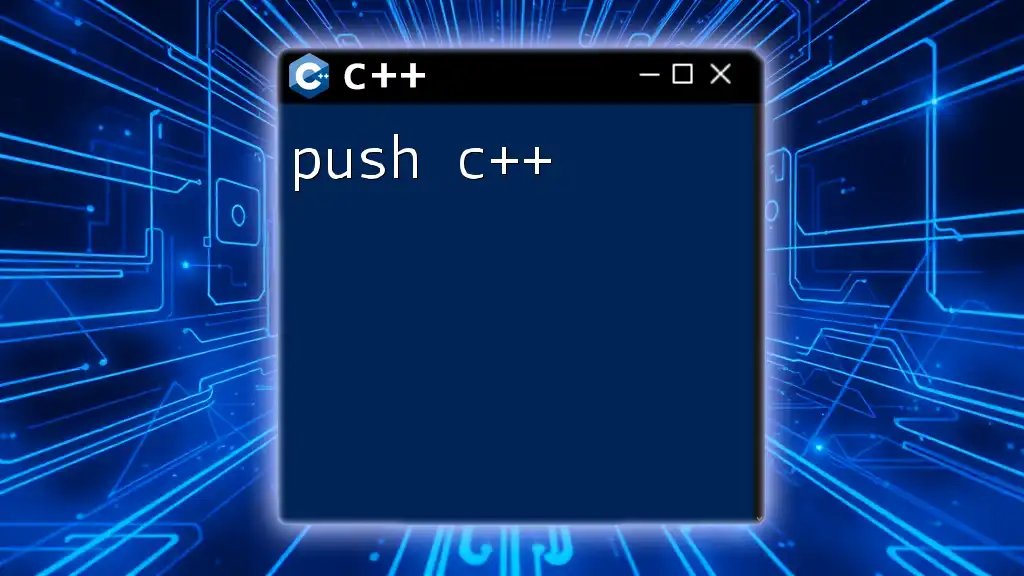
Working with Paths in C++
Including Libraries
Essential Libraries for Path Manipulation
In modern C++ (C++17 and later), the `<filesystem>` library offers a suite of powerful tools for path management. Utilizing this library simplifies working with paths, enabling you to perform manipulations and checks easily without falling into the intricacies of manual string operations.
Common Path Operations
Creating Paths
Creating a path using the `<filesystem>` library is simple. You can initialize a new path object as shown below:
#include <filesystem>
namespace fs = std::filesystem;
fs::path p("example.txt");
Manipulating Paths
With the `<filesystem>` library, manipulating paths becomes straightforward. You can retrieve the `parent_path`, `filename`, and `extension` of a file as follows:
fs::path p("/home/user/documents/example.txt");
std::cout << "Filename: " << p.filename() << std::endl; // Output: example.txt
Checking Path Properties
To determine if a path exists or to check whether it points to a file or a directory, you can use built-in features of the filesystem:
if (fs::exists(p)) {
std::cout << "Path exists!" << std::endl;
}
Advanced Path Operations
Iterating Through Directories
If you need to traverse a directory to list files or directories, you can leverage file iterators. Here's how you can do this:
for (const auto& entry : fs::directory_iterator("/path/to/directory"))
std::cout << entry.path() << std::endl;
Path Comparison and Equality
Comparing paths can be important for ensuring that your application behaves correctly. Using the equality operator, you can determine if two paths are identical:
fs::path path1("/home/user/docs");
fs::path path2("/home/user/docs/");
if (path1 == path2) {
std::cout << "Paths are equal." << std::endl;
}
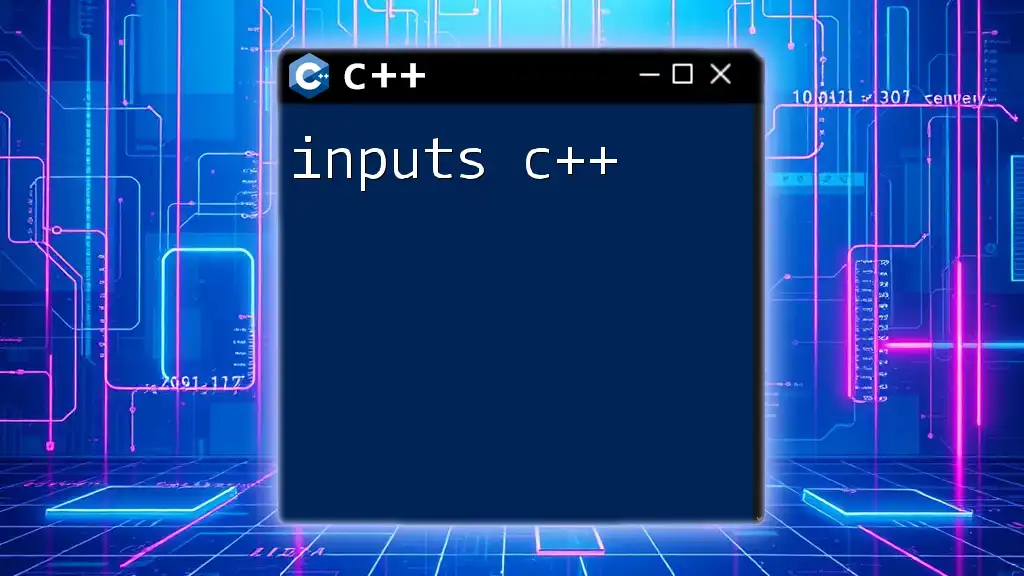
Best Practices for Managing Paths in C++
Cross-Platform Considerations
Writing code that works across different operating systems requires special attention to path formats. Use the `<filesystem>` library for compatibility, and always test your code in the environments where it will be used. This ensures that your application will work as expected, preventing headaches related to path manipulation.
Using Path Constants
To improve readability and maintainability, consider defining constants for frequently accessed directories. For example:
const fs::path DATA_PATH = "data";
const fs::path CONFIG_PATH = "config";
This practice helps standardize paths throughout your application, making it easier to update them in the future.
Error Handling and Exceptions
When working with paths, proper error handling is crucial. Use exception handling to catch errors that may occur during file operations. The `<filesystem>` library can throw exceptions, and acknowledging these can improve the robustness of your application. For example:
try {
fs::path p("non_existent_file.txt");
std::ifstream file(p);
file.exceptions(std::ifstream::failbit | std::ifstream::badbit);
file.open(p);
} catch (const fs::filesystem_error& e) {
std::cerr << "Filesystem error: " << e.what() << std::endl;
}
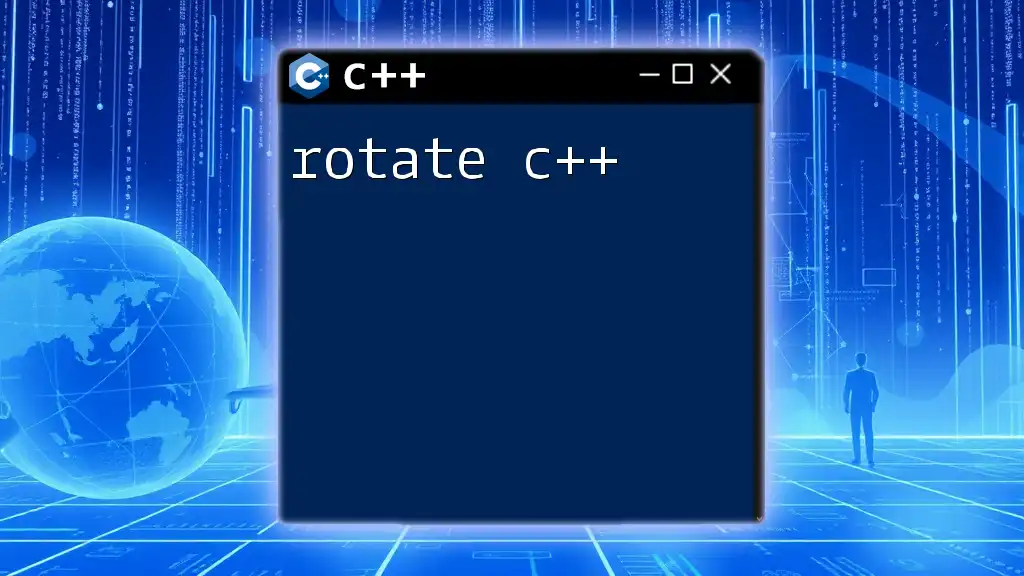
Conclusion
Understanding paths in C++ is essential to managing files and directories reliably within your applications. This comprehension not only aids in preventing errors but also promotes better code organization and portability across different systems. As you dive deeper into path management, remember to practice these concepts through hands-on exercises, and don't hesitate to explore external resources for further learning.
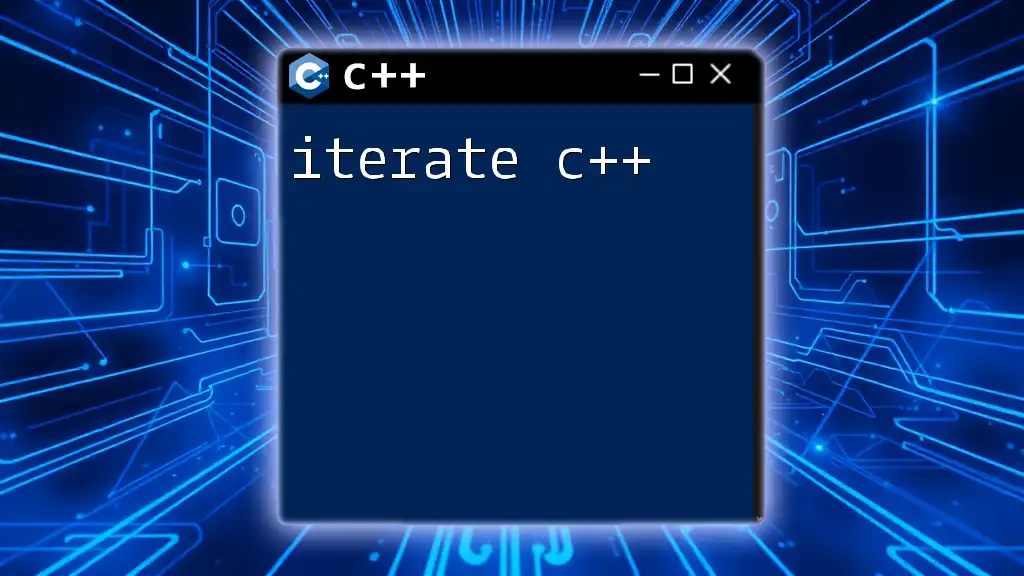
Additional Resources
For those interested in expanding their knowledge on paths in C++, consider exploring books, articles, and the official C++ documentation regarding the `<filesystem>` library. Engaging with community forums can also provide valuable insights and practical examples to enhance your skills in path manipulation.