In C++, an empty vector can be created using the `std::vector` class from the Standard Template Library (STL), which serves as a dynamic array that can grow in size as needed.
#include <vector>
std::vector<int> emptyVector; // Creating an empty vector of integers
Introduction to C++ Vectors
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. It is part of the Standard Template Library (STL) and provides several benefits, including ease of use and flexible memory management. Vectors are widely used throughout modern C++ programming for storing collections of elements efficiently.
The Concept of an Empty Vector
An empty vector is a vector that has been declared but contains no elements. This is often an important concept, especially when you need to initialize structures or prepare for incoming data.
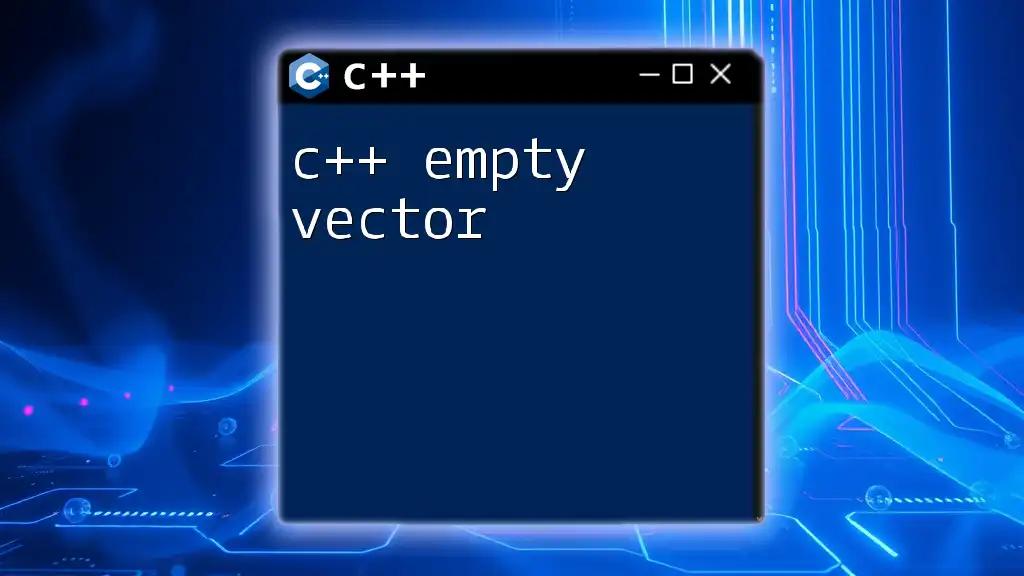
Creating an Empty C++ Vector
Syntax for Defining an Empty Vector
To create an empty vector, you can simply declare it without initializing any elements. Here’s how you can do this:
std::vector<int> myVector; // Default constructor
In this case, `myVector` is an empty vector of integers. When you declare a vector in this way, it is initialized, but it does not contain any elements.
Using the `std::vector` Constructor
You can explicitly create an empty vector using the constructor as shown below:
std::vector<int> myVector = std::vector<int>();
This line has the same effect as the previous declaration, explicitly creating a vector that is currently empty.
C++ Vector with Specified Type
Vectors can store different types of elements, not just integers. Here is how you can create an empty vector of strings:
std::vector<std::string> stringVector;
Now, `stringVector` is an empty vector ready to hold string elements, which you can add later as needed.
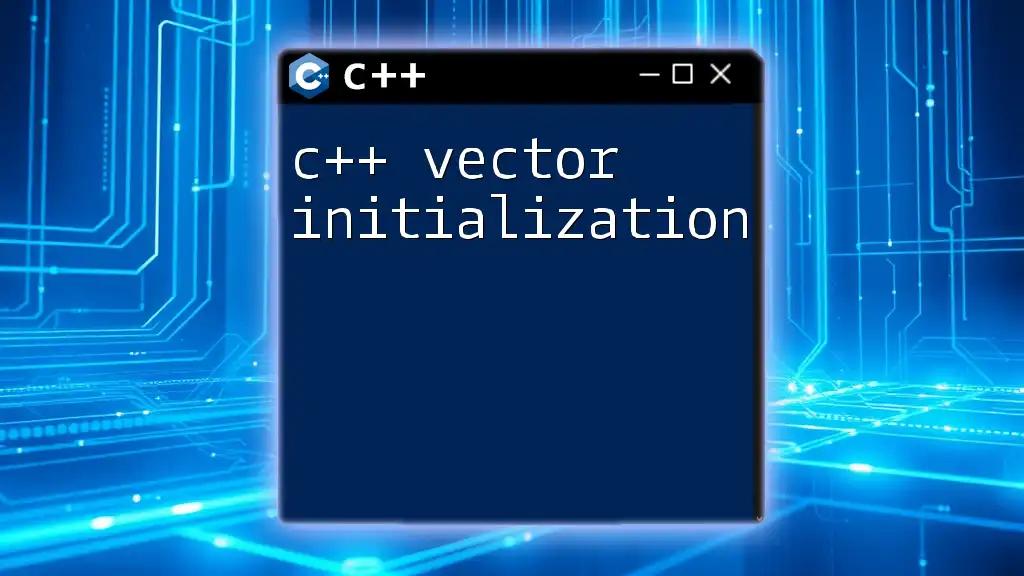
Checking if a Vector is Empty
Why You Need to Check for Empty Vectors
Checking whether a vector is empty can help avoid unnecessary errors in your program. If you try to access elements in an empty vector, you can run into runtime errors or undefined behavior. Thus, validating whether a vector is empty before proceeding is crucial for robust programming.
Using the `empty()` Method
C++ provides the `empty()` method to easily check if a vector is empty. Here’s how you can use it:
if(myVector.empty()) {
std::cout << "The vector is empty." << std::endl;
}
This code checks if `myVector` contains any elements. If it doesn’t, it will print "The vector is empty."
Understanding the Return Value
The `empty()` method returns a Boolean value: `true` if the vector is empty and `false` otherwise. This simplification allows for straightforward conditional checks within your code.
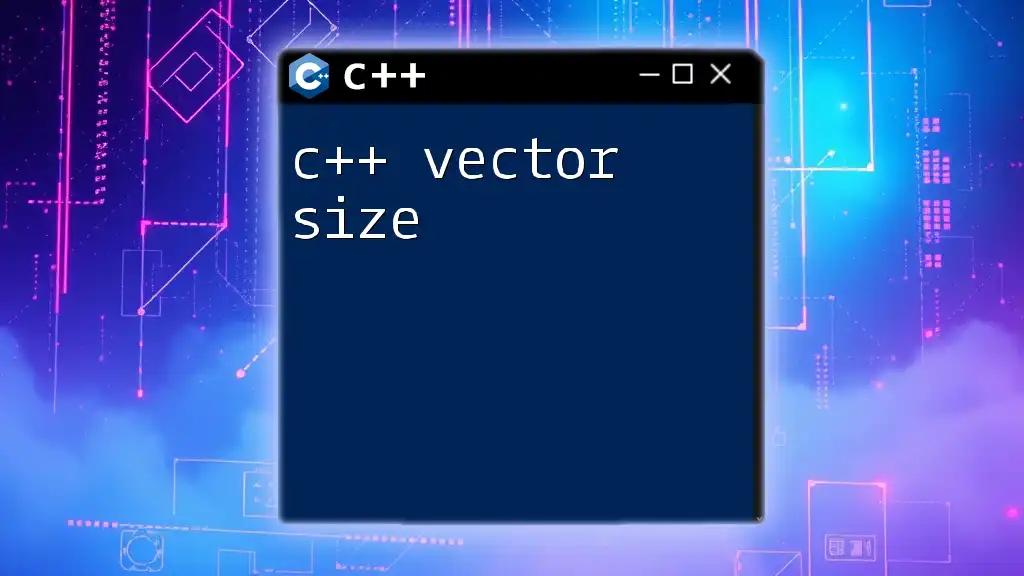
Best Practices for Working with Empty C++ Vectors
Initializing Vectors Before Use
Always initialize your vectors before you use them. Leaving vectors uninitialized might lead to logical errors, causing your program to behave unpredictably. For example, initializing an empty vector at the start of a function can set a clear state for subsequent operations.
Clearing Vectors Properly
When a vector is no longer needed, it’s a good practice to clear it. This can help manage memory efficiently. You can do this using:
myVector.clear();
Clearing a vector removes all elements but keeps the vector itself available for new elements to be added later. This is especially useful in scenarios where you may reuse the vector instead of reallocating a new one.
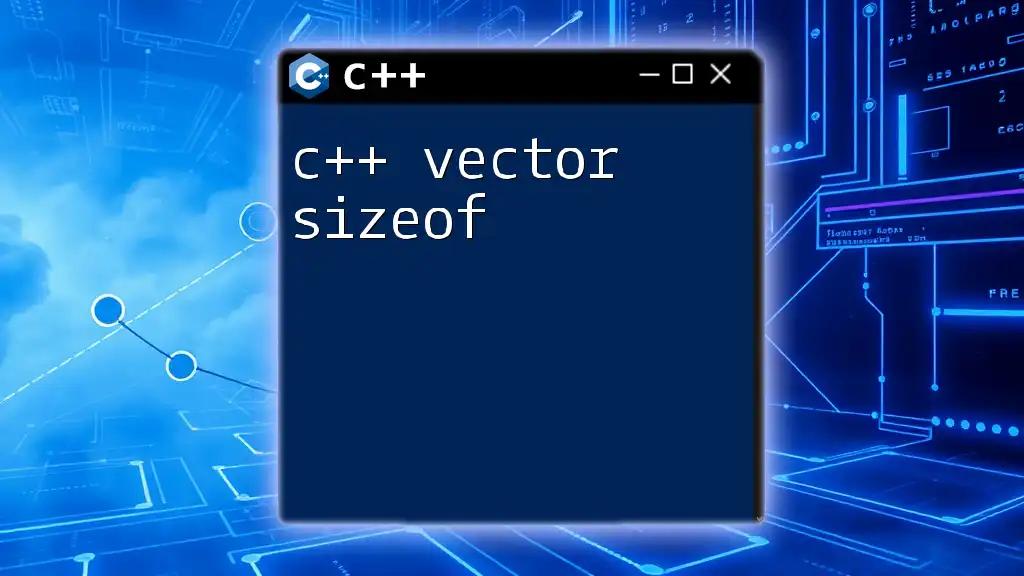
Modifying an Empty Vector
Adding Elements to an Empty Vector
Once you have an empty vector, you can begin populating it with elements using methods like `push_back()`. Here’s an example:
myVector.push_back(10); // Now it contains one element
After executing this line, `myVector` is no longer empty; it contains one integer element.
Resizing an Empty Vector
You can resize an empty vector to define how many elements it should hold, even if they are default-constructed values. For instance:
myVector.resize(5); // Creates a vector with 5 default-constructed elements.
After resizing, `myVector` will now hold five elements, all initialized to the default value for the specified type (in this case, integers initialized to zero).
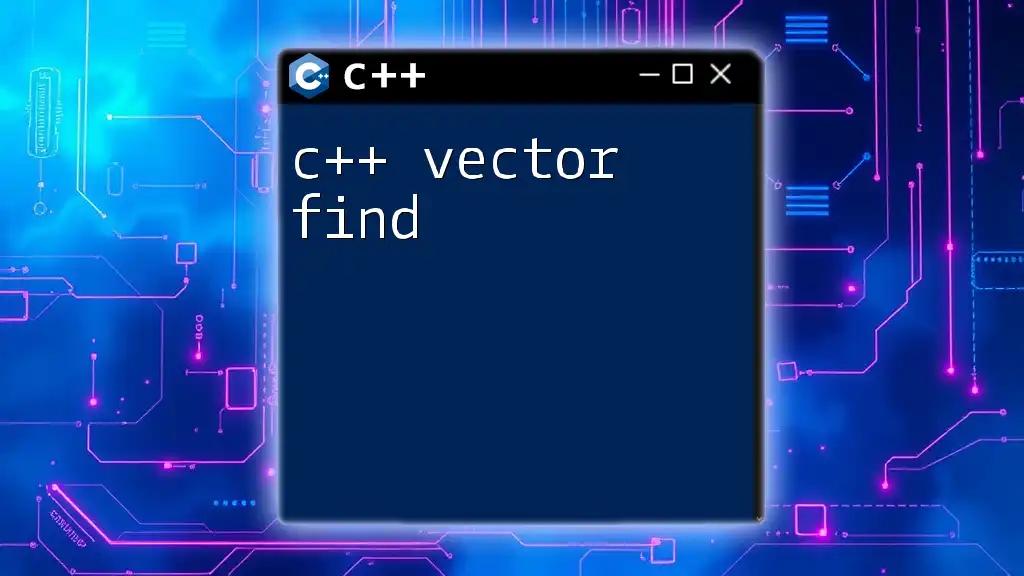
Common Errors and Debugging Tips
Misunderstandings About Vector Size vs. Capacity
It’s essential to distinguish between a vector’s size and its capacity. The size represents how many elements are currently in the vector, while capacity indicates how many elements the vector can hold before needing to allocate more memory.
std::cout << "Size: " << myVector.size() << " Capacity: " << myVector.capacity() << std::endl;
Understanding these terms can help avoid performance issues and potential bugs in applications.
Examples of Runtime Errors
Common mistakes include attempting to access or modify elements when a vector is empty. Always check if a vector is empty using `empty()` before accessing its elements or performing other operations that assume the presence of elements.
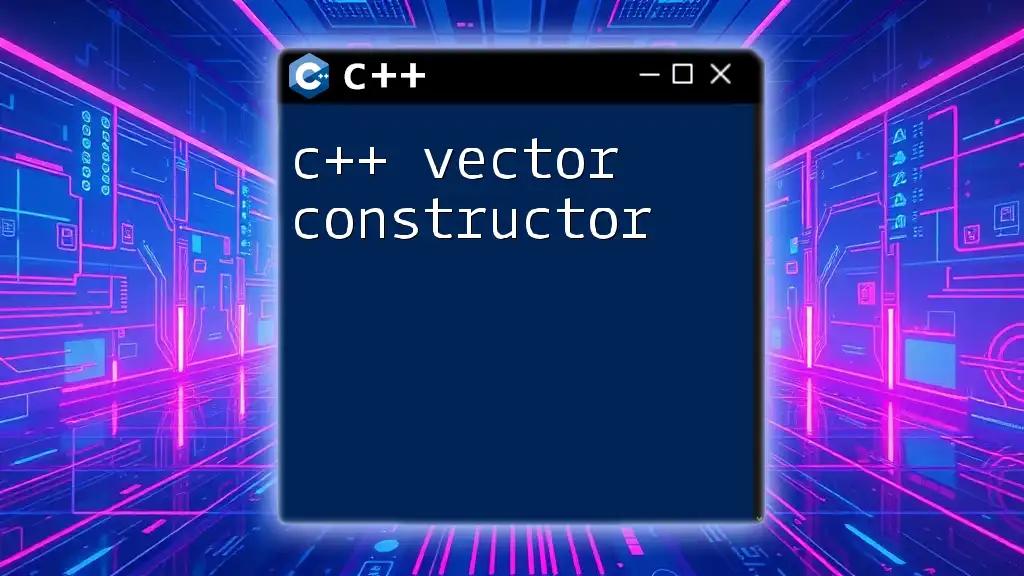
Conclusion
In summary, the empty C++ vector is a fundamental concept that can have a significant impact on how you manage data in your applications. By understanding how to create, manipulate, and check for empty vectors, you can write more flexible and error-free C++ code. Practice these techniques in your projects and see how they contribute to cleaner, more efficient code.