In C++, an empty vector is a vector that has been declared but contains no elements, which can be useful for initializing storage that will be populated later.
Here's how to declare an empty vector in C++:
#include <vector>
std::vector<int> myEmptyVector; // This creates an empty vector of integers.
Introduction to C++ Vectors
A vector in C++ is a dynamic array that can grow and shrink in size as needed, making it a versatile choice for managing collections of data. Unlike traditional arrays, which have a fixed size, vectors can be resized, allowing for more flexibility when the size of the data set is not known in advance.
Empty vectors are particularly important for initializing collections where data may be added later. Starting with an empty vector provides a clean slate to manage data effectively, ensuring that your program can adapt to changing data conditions.
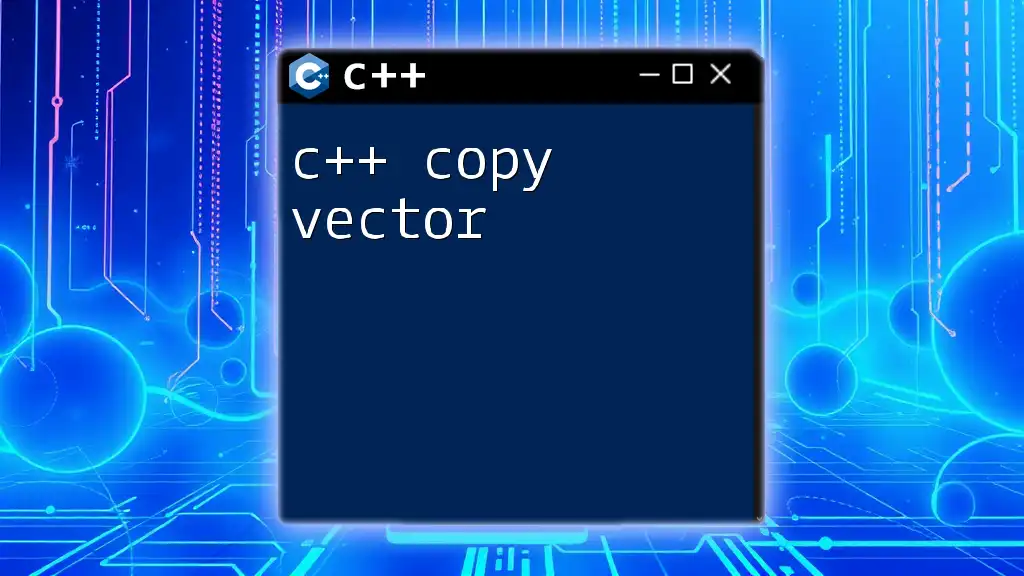
Creating an Empty Vector in C++
Creating an empty vector is straightforward. In C++, you first need to include the vector library before you can use it.
Basic Syntax for Vector Creation
You can create an empty vector by using the following syntax:
#include <vector>
std::vector<int> myEmptyVector; // Declaring an empty vector of integers
This line of code declares an empty vector `myEmptyVector` that can hold integers. The vector is initialized but contains no elements at the moment.
Vector Types
Vectors can be declared for various data types, making them highly versatile. You can create empty vectors for integers, strings, custom objects, and more.
-
Integer Vectors: Great for storing numerical values.
std::vector<int> myIntVector; // Empty vector for integers
-
String Vectors: Useful for storing sequences of characters.
std::vector<std::string> myStringVector; // Empty vector for strings
-
Custom Object Vectors: These can store instances of user-defined classes.
class MyClass { // Custom class definition }; std::vector<MyClass> myObjectVector; // Empty vector for custom objects
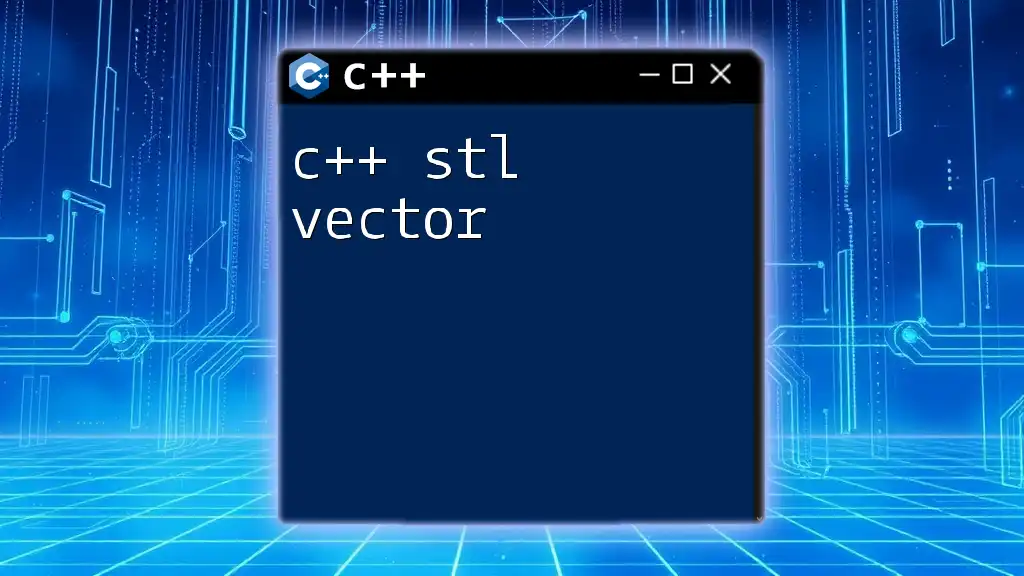
Checking If a Vector is Empty
Knowing whether a vector is empty is crucial before attempting to access its elements or perform operations on it.
Using the `empty()` Method
The `empty()` method allows you to check if a vector has any elements. It returns `true` if the vector is empty and `false` otherwise.
if (myEmptyVector.empty()) {
std::cout << "Vector is empty." << std::endl;
}
This code snippet checks if `myEmptyVector` contains any elements and prints a message if it does not.
Using the `size()` Method
An alternative way to check if a vector is empty is to use the `size()` method, which returns the number of elements in the vector. If the size is zero, the vector is empty.
if (myEmptyVector.size() == 0) {
std::cout << "Vector is empty." << std::endl;
}
Both methods are effective, but `empty()` is often preferred for simplicity and readability.
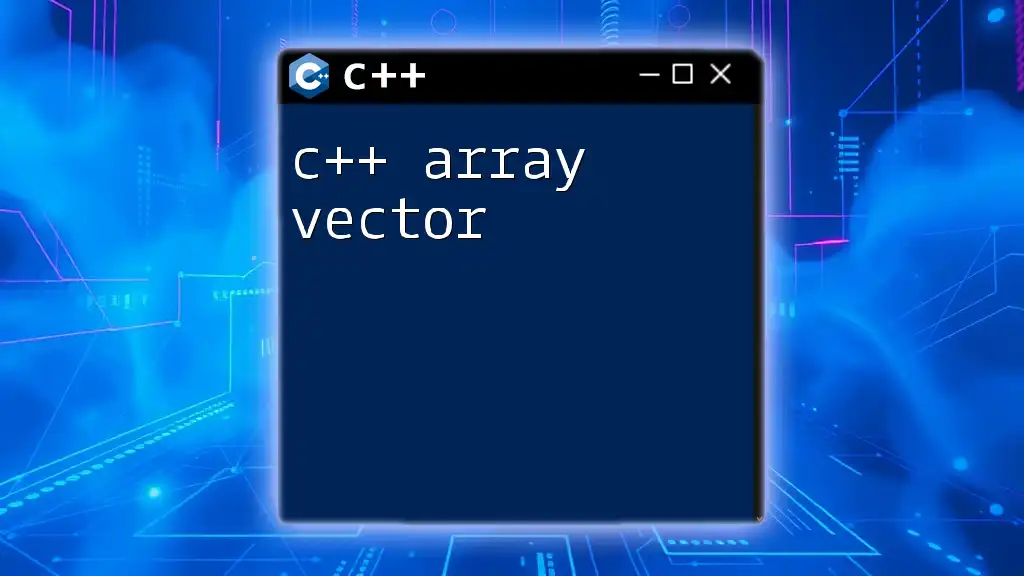
Adding Elements to an Empty Vector
Once you have an empty vector, adding elements to it is seamless.
Pushing Back Elements
The most common way to add elements to a vector is using the `push_back()` method. This method appends an element to the end of the vector.
myEmptyVector.push_back(10);
myEmptyVector.push_back(20);
In this example, `10` and `20` are added to `myEmptyVector`, increasing its size dynamically.
Inserting Elements at Specific Positions
You can also insert elements at specified positions using the `insert()` method.
myEmptyVector.insert(myEmptyVector.begin(), 5); // Inserting at the beginning
This line of code inserts the element `5` at the start of `myEmptyVector`, pushing the existing elements to the right.
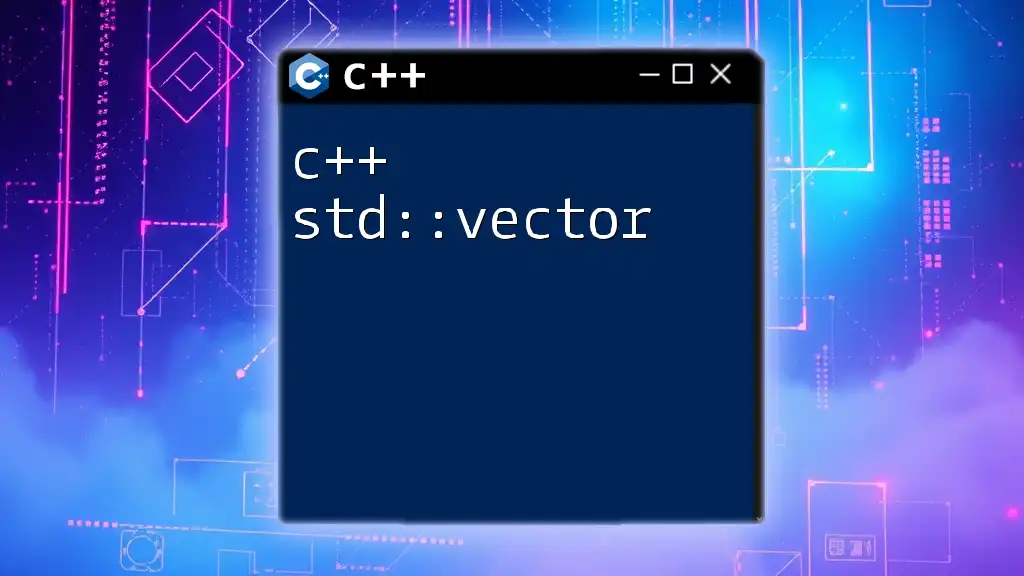
Accessing Elements of a Vector
Accessing elements in a vector can be done safely through several methods.
Using the Index Operator
You can access elements in a vector using the index operator, which allows direct access based on the element's position.
int firstElement = myEmptyVector[0]; // Accessing the first element
However, be cautious, as accessing an index out of bounds can lead to undefined behavior.
Using the `at()` Method
A safer alternative is to use the `at()` method, which checks for bounds and throws an exception if the index is invalid.
int secondElement = myEmptyVector.at(1); // Safely accessing the second element
Using `at()` helps prevent runtime errors, making your code more robust.
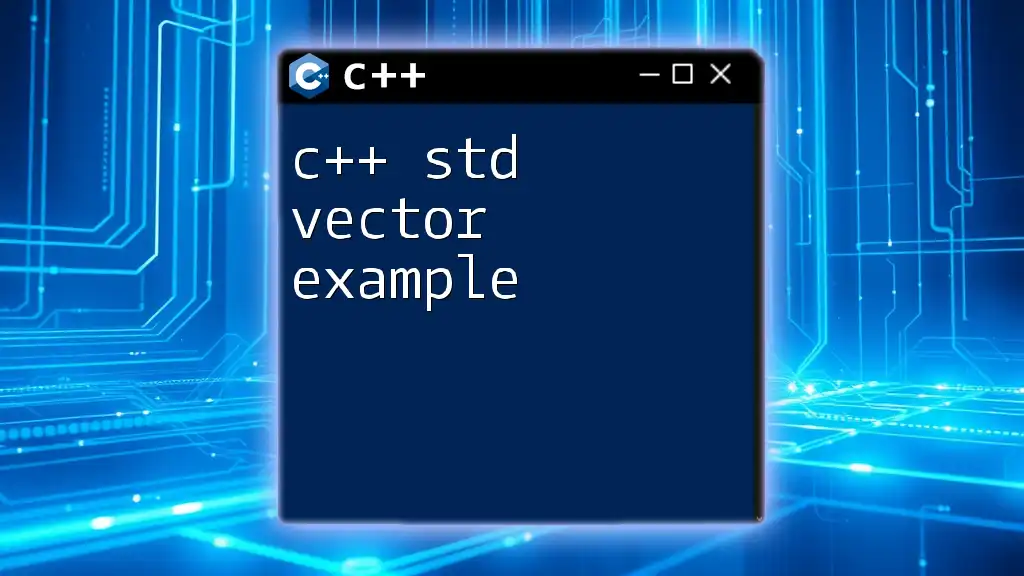
Best Practices When Working with Empty Vectors
Initialize Vectors Appropriately
Whenever possible, initialize your vectors explicitly to avoid confusion. Declaring a vector without initializing its elements can lead to unpredicted behavior.
Avoiding Memory Management Issues
Starting with an empty vector allows you to manage memory efficiently, reducing the chance of encountering errors such as out-of-bounds accesses. Always ensure your vectors are populated before accessing their elements.
Performance Considerations
If you know in advance the maximum size your vector will reach, you can use the `reserve()` method to allocate memory ahead of time:
myEmptyVector.reserve(100); // Reserve space for 100 elements
This helps optimize performance by reducing the number of memory reallocations that occur as elements are added.
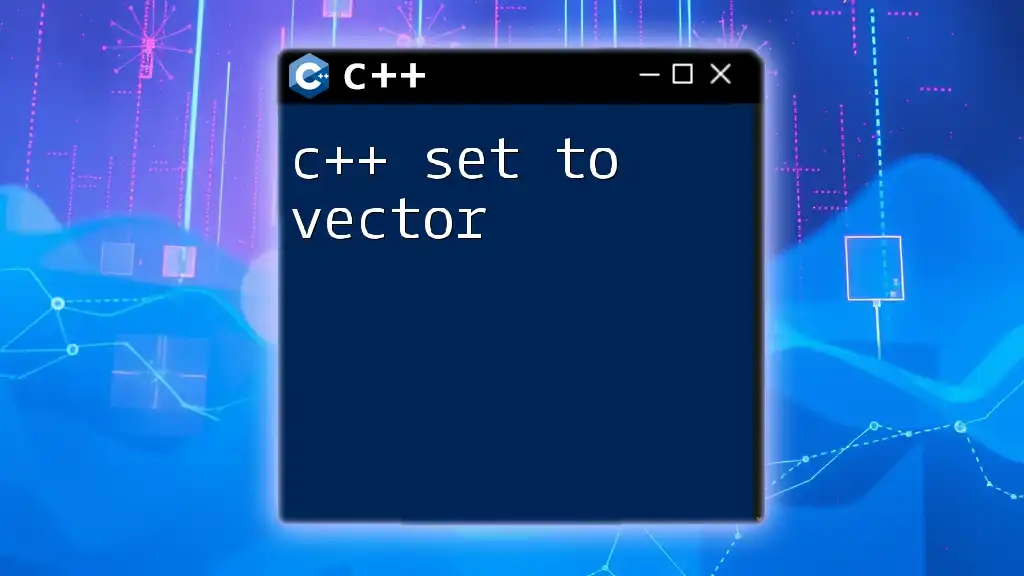
Common Use Cases for Empty Vectors
Dynamic Data Structure Usage
Empty vectors are invaluable in functions where data cannot be predetermined. They permit the collection of data as it's received, making them adaptable in various programming scenarios.
Data Collection Scenarios
Vectors are frequently used in data processing applications where data is captured over time, such as reading user input or processing file data. An empty vector can dynamically grow as data arrives.
Algorithm Implementation
Many algorithms require temporary storage for their operations, making empty vectors an excellent choice for managing intermediate data. Whether sorting, searching, or other computational tasks, empty vectors play a key role in algorithm efficiency.
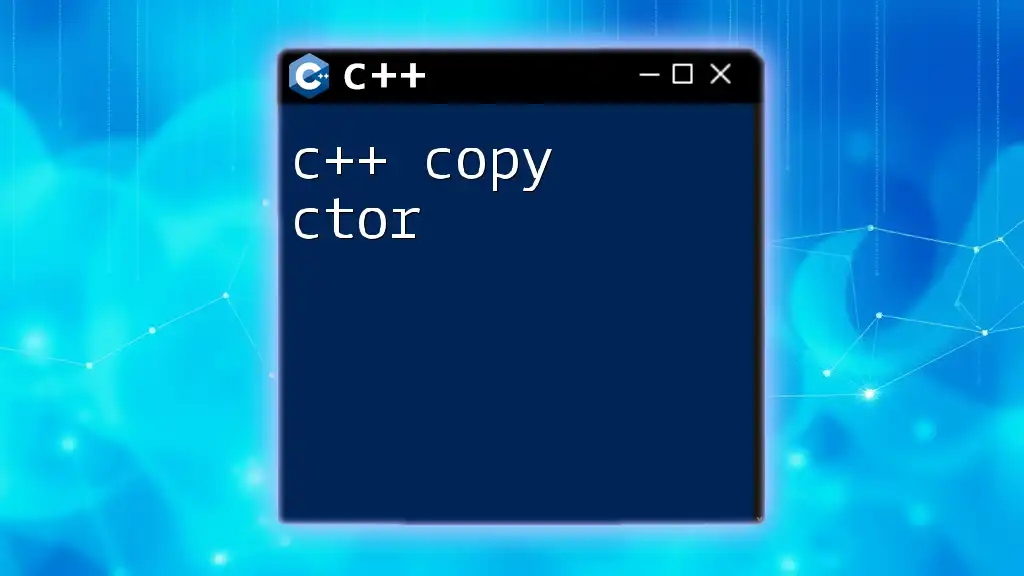
Conclusion
Understanding the C++ empty vector is critical for any programmer working with dynamic data structures. They provide flexibility and adaptability in data management, allowing for ease of use and performance optimization through careful initialization and memory management. Further exploration of C++ vectors opens the door to mastering more advanced programming techniques.