In C++, the `vector` class from the Standard Template Library (STL) is a dynamic array that can resize itself automatically when elements are added or removed.
Here’s a simple example demonstrating how to use a `vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Create a vector of integers
numbers.push_back(10); // Add elements to the vector
numbers.push_back(20);
numbers.push_back(30);
for (int num : numbers) { // Iterate and print the elements
std::cout << num << " ";
}
return 0;
}
What is a Vector in C++?
A vector in C++ is a dynamic array that allows costly operations, such as adding and removing elements, to be handled with greater efficiency compared to traditional arrays. While arrays have a fixed size, vectors can grow or shrink as needed, which makes them an essential data structure for many C++ programs.
The importance of the C++ class vector lies in its robust feature set, which simplifies memory management while providing flexible storage solutions.

Key Features of the C++ Vector Class
Vectors come with several key features that enhance their usefulness:
- Dynamic Size: Unlike arrays, vectors can grow or shrink in size at runtime.
- Memory Management: Vectors manage memory automatically, relieving developers from manual memory allocation and deallocation.
- Capacity vs Size: The size of a vector refers to the number of elements it contains, while the capacity refers to the amount of storage that has been allocated for it. This distinction is essential for optimizing performance.
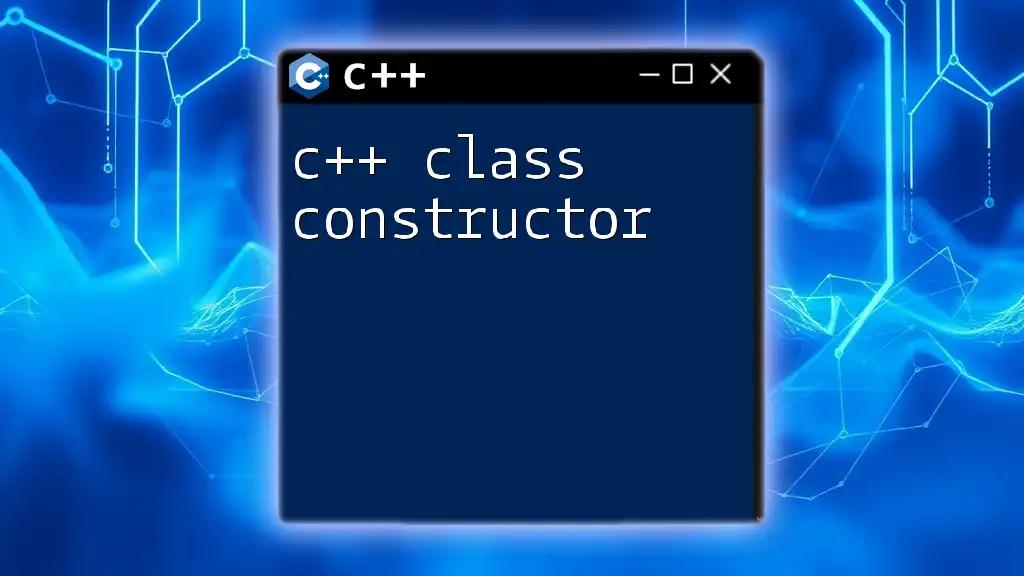
Basic Operations with Vectors
Creating a Vector
You can create a vector using the following syntax:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Creates an empty vector of integers
return 0;
}
Vectors can also be initialized directly with specified values:
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Initializes a vector with values
Adding Elements to a Vector
To add elements to a vector, you can use the `push_back()` method or the `insert()` method.
numbers.push_back(10); // Adds 10 to the end of the vector
numbers.insert(numbers.begin() + 1, 15); // Inserts 15 at position 1
These methods enable you to manipulate your vector easily, even if it is initially empty.
Accessing and Modifying Vector Elements
Accessing Elements
Elements in a vector can be accessed using the subscript operator `[]` or the `.at()` method. The latter provides bounds checking, which can help avoid out-of-bounds errors.
int firstElement = numbers[0]; // Accessing first element
int safeElement = numbers.at(0); // Also accesses first element but with bounds checking
Modifying Elements
You can modify vector elements directly through indexing:
numbers[0] = 20; // Changes the first element to 20
This overwrites the original value, demonstrating how vectors provide an effective means for dynamic data manipulation.
Vector Class Member Functions
Size and Capacity
Two crucial functions of the C++ class vector are `.size()` and `.capacity()`. The `.size()` function returns the number of elements currently held by the vector, while `.capacity()` tells you how many elements the vector can hold before needing to allocate more memory.
std::cout << "Size: " << numbers.size() << std::endl; // Outputs the current size
std::cout << "Capacity: " << numbers.capacity() << std::endl; // Outputs the capacity
You can also use `.resize()` to change the size of the vector, which is particularly useful for managing the vector data dynamically.
Removing Elements from a Vector
Removing elements can be done using `pop_back()`, `erase()`, and `clear()`. Each serves a different purpose—`pop_back()` removes the last element, `erase()` can remove specific elements, and `clear()` empties the vector entirely.
numbers.pop_back(); // Removes the last element
numbers.erase(numbers.begin() + 1); // Removes the element at position 1
These operations enhance data management, ensuring you can maintain your vector's integrity as required.
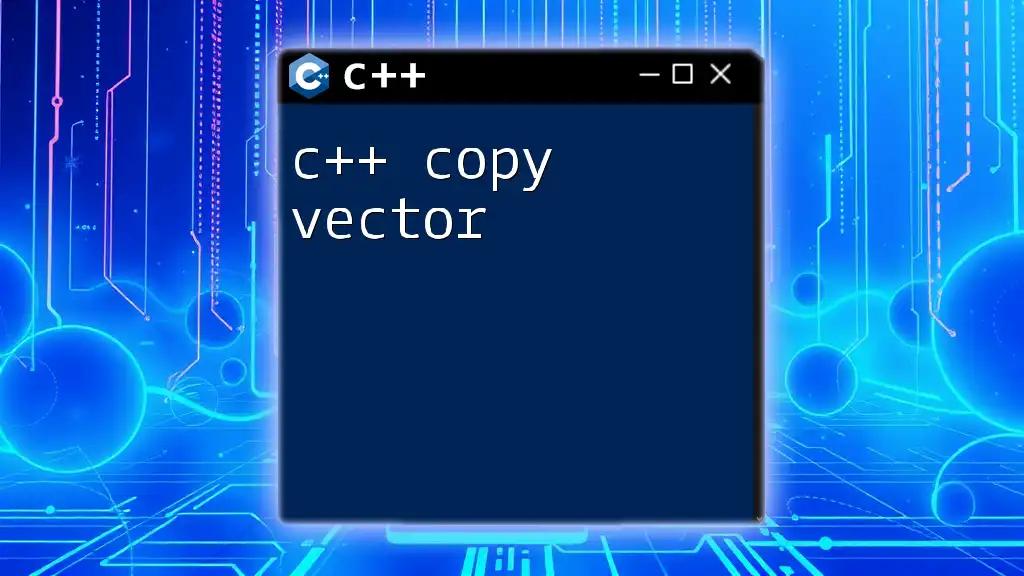
Advanced Features of the C++ Vector Class
Understanding Vector Iterators
Vectors support iterators, which provide a way to traverse the elements. Using iterators, you can write clean and efficient loops.
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " "; // Prints each element in the vector
}
Range-based For Loops
C++11 introduced range-based for loops, making it easier to iterate through vectors without directly handling iterators.
for (const auto &num : numbers) {
std::cout << num << " "; // Prints each element using range-based for loop
}
Sorting and Searching in Vectors
The Standard Library provides functions such as `std::sort` and `std::find`, allowing for convenient sorting and searching of vector contents.
#include <algorithm>
std::sort(numbers.begin(), numbers.end()); // Sorts the vector in ascending order
Using these algorithms enhances the functionality of vectors and sets the stage for more complex data manipulation.
Creating and Using 2D Vectors
The C++ class vector also supports multidimensional vectors, such as 2D vectors. This can be useful for applications such as matrices.
std::vector<std::vector<int>> matrix(3, std::vector<int>(3, 0)); // Creates a 3x3 matrix initialized to 0
Accessing a specific element in a 2D vector can be done as follows:
matrix[1][1] = 5; // Sets the element at row 1, column 1 to 5
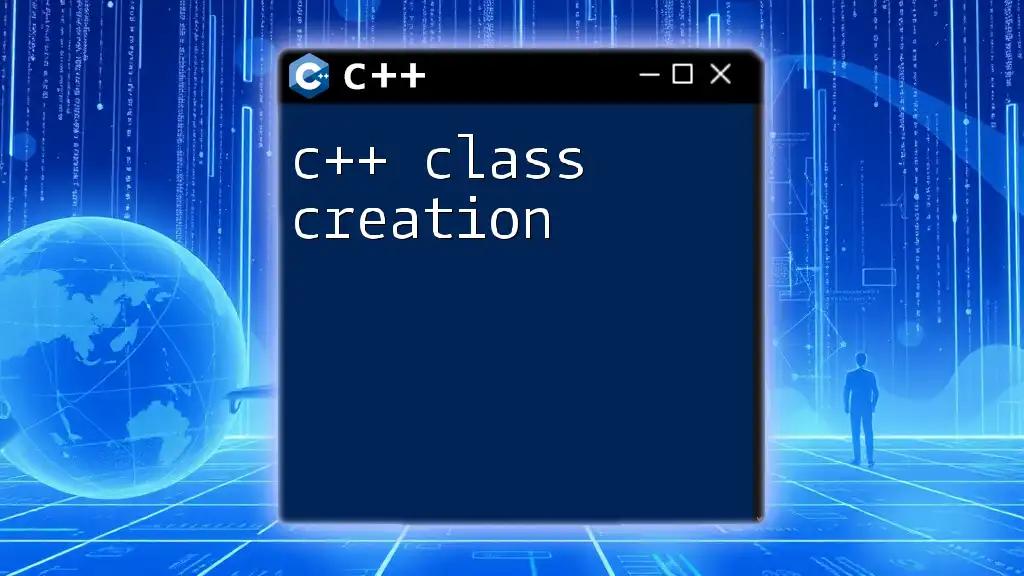
Performance Considerations
Memory Management and Efficiency
Understanding how memory is managed in vectors is crucial. Vectors utilize dynamic memory allocation, allowing for flexible data size. However, it's essential to be mindful of how frequently you resize a vector, as this can lead to performance overhead.
Comparing Vectors with Other Data Structures
Choosing between vectors and other data structures (like lists or arrays) depends on your application's requirements. Vectors perform well when:
- Random access is needed.
- The size of the collection changes frequently.
Conversely, lists might be more efficient when constant-time insertions and deletions are required.

Common Mistakes and How to Avoid Them
Avoiding Out-of-Bounds Errors
Out-of-bounds access can lead to run-time crashes. Use `.at()` instead of `[]` to benefit from bounds checking.
Memory Leaks with Vectors
While vectors manage memory automatically, failing to properly manage references or pointers can lead to memory leaks. Always ensure that any dynamically allocated memory is correctly released.
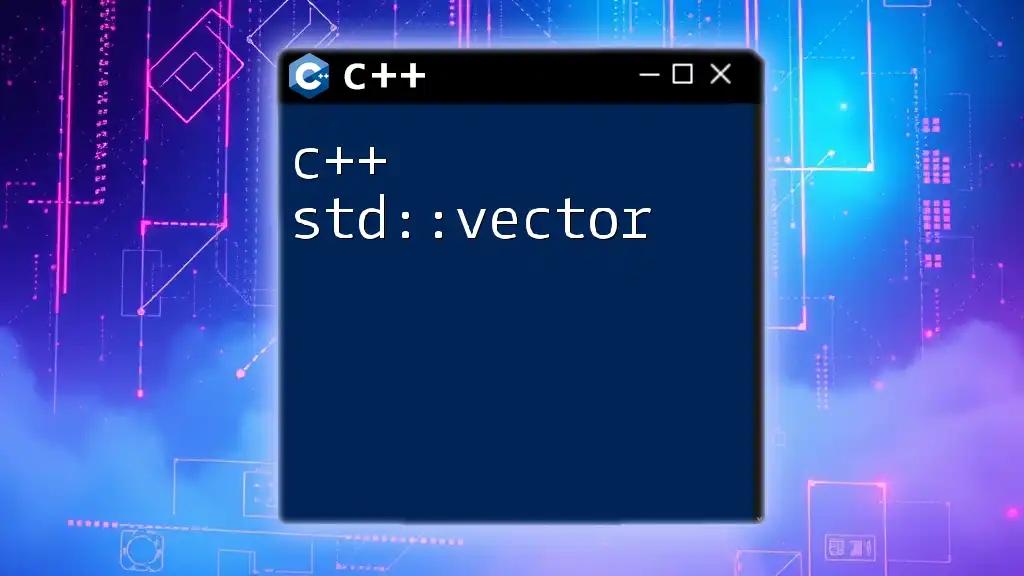
Recap of Key Points
The C++ class vector provides a flexible and powerful way to manipulate dynamic collections of data. Its key features, such as dynamic sizing, memory management, and built-in algorithms, make it an indispensable tool for modern C++ programming.
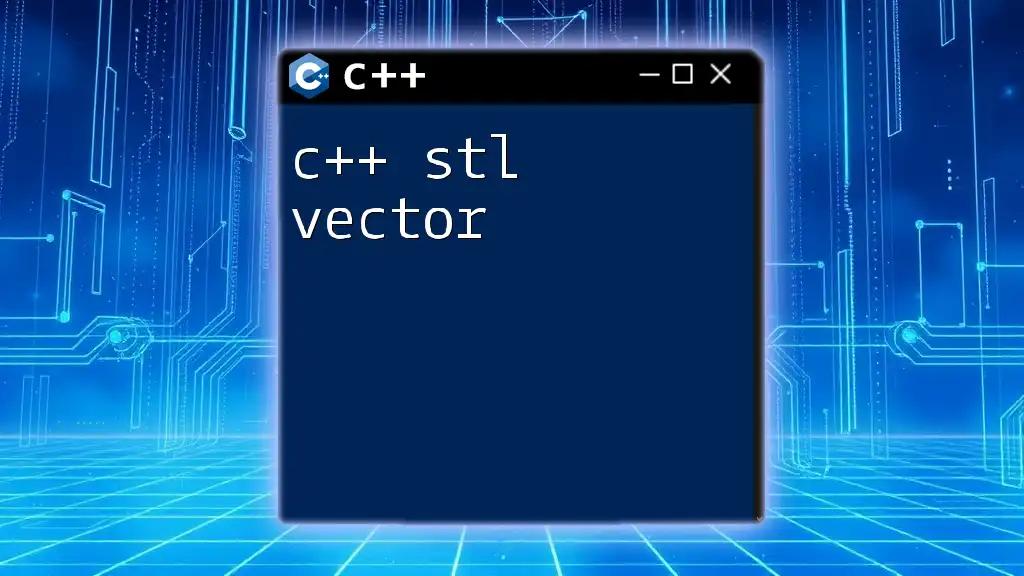
Resources for Further Learning
To deepen your understanding, consider exploring books focused on C++ programming, online tutorials, and participating in C++ communities and forums where you can ask questions, share experiences, and engage with fellow programming enthusiasts.
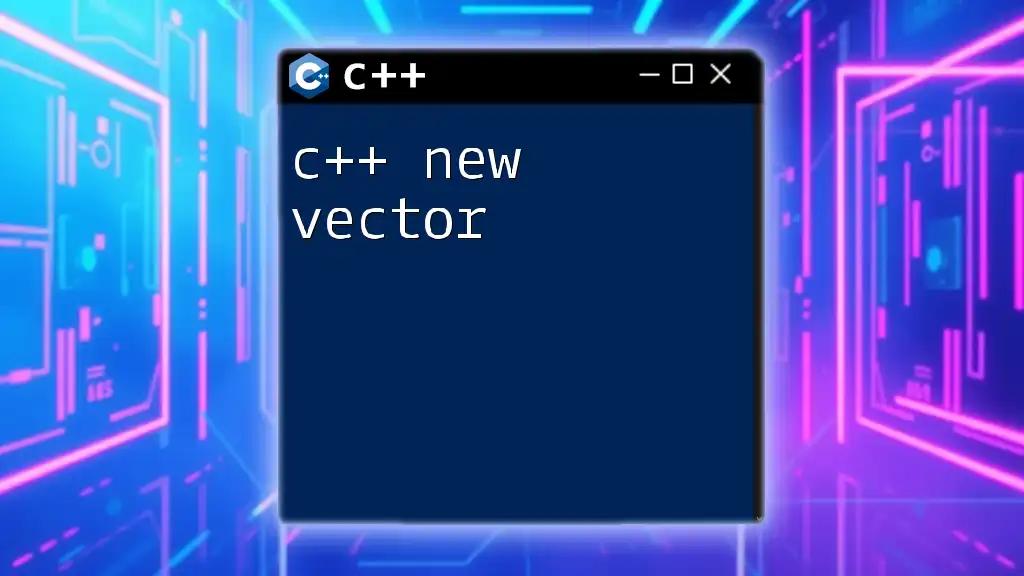
Encouragement to Practice
Don’t hesitate to experiment with the examples provided here. Practice is crucial for mastering the C++ class vector, so try to write some small applications that utilize vectors effectively!