In C++, you can copy a vector using the assignment operator or the `std::copy` algorithm, allowing you to create an identical copy of the original vector.
Here’s a code snippet demonstrating both methods:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
// Method 1: Using assignment operator
std::vector<int> copy1 = original;
// Method 2: Using std::copy
std::vector<int> copy2(original.size());
std::copy(original.begin(), original.end(), copy2.begin());
// Output to verify the copies
for(int n : copy1) std::cout << n << " "; // Output: 1 2 3 4 5
std::cout << std::endl;
for(int n : copy2) std::cout << n << " "; // Output: 1 2 3 4 5
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a dynamic array that can resize itself automatically when elements are added or removed. Unlike traditional arrays, which have a fixed size, vectors can grow or shrink to accommodate their elements. This flexibility is a key advantage, making vectors a popular choice for many programming tasks. They are part of the Standard Template Library (STL) and offer various member functions to facilitate operations such as insertion, deletion, and access to elements.
Why Copy a Vector?
Copying a vector in C++ can be crucial for several reasons:
- Function Arguments: When passing vectors to functions, especially for operations that require the original data to remain unchanged.
- Data Manipulation: Sometimes, you may need to work on a duplicate of the original vector, allowing you to modify it without impacting the initial data.
- Concurrency: When working with multithreading, having copies of vectors can prevent race conditions and ensure data integrity.

Methods to Copy a Vector in C++
Using the Assignment Operator
One of the simplest ways to copy a vector in C++ is by using the assignment operator. This method allows you to create a copy of the vector instantly.
Consider the following code snippet that demonstrates this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> copy = original; // Copy vector
for (int val : copy) {
std::cout << val << " ";
}
return 0;
}
In this example, the `copy` vector takes a complete replica of the `original` vector. Importantly, if you modify the `copy`, the `original` remains unchanged. This method is straightforward and efficient, especially for smaller vectors.
Using the `std::vector` Copy Constructor
Another way to perform a vector copy is through the use of the copy constructor. This constructor creates a new vector that is a copy of an existing vector when you initialize it.
Here’s how it looks in code:
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> copy(original); // Copy constructor
for (int val : copy) {
std::cout << val << " ";
}
return 0;
}
In this case, you are constructing a new vector `copy` directly from `original`. As with the assignment operator, this creates a separate instance of the vector with its own memory. Any changes made to `copy` will not affect `original`.
Using the `assign` Method
The `assign` member function offers another way to copy vectors, particularly useful when you want to copy elements from a different vector or a specific range of elements.
Consider the following code and notice how `assign` works:
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> copy;
copy.assign(original.begin(), original.end()); // Using assign
for (int val : copy) {
std::cout << val << " ";
}
return 0;
}
In this example, the `assign` method takes the beginning and end iterators of the `original` vector and fills the `copy` vector with those elements. This method is especially useful when you want to copy elements selectively or all at once.
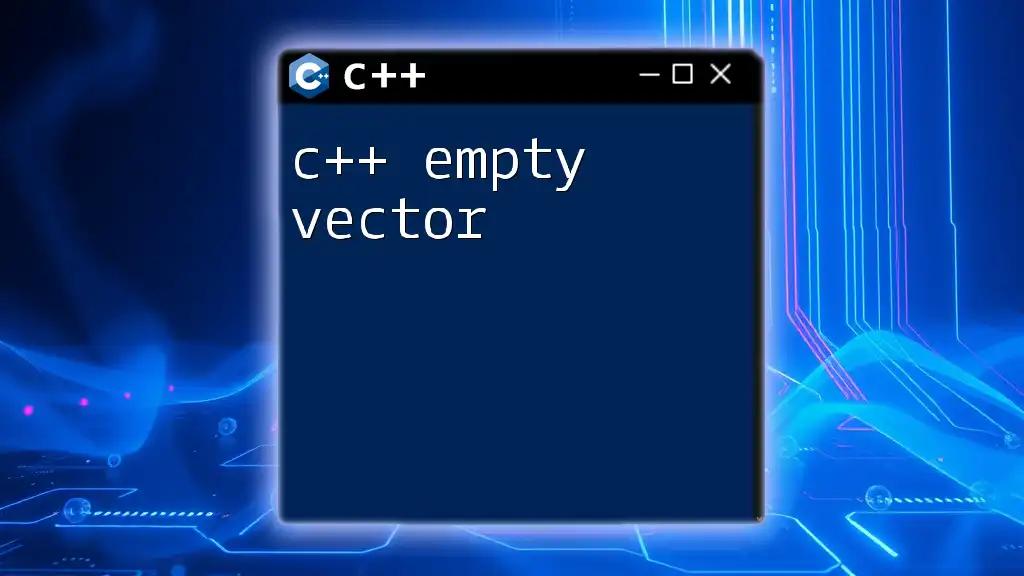
Copying a Subvector
Copying a Portion of a Vector
You may not always need to copy the entire vector; sometimes, copying a subvector suffices. You can do this easily using iterator pairs to define the range.
Here’s a practical example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> subVector(original.begin() + 1, original.begin() + 4); // Copy elements 2 to 4
for (int val : subVector) {
std::cout << val << " ";
}
return 0;
}
In this case, `subVector` contains a range from `original` starting at index 1 and ending before index 4. The copied elements will be `{2, 3, 4}`, illustrating how easy it is to create a subvector while maintaining the integrity of the original vector.
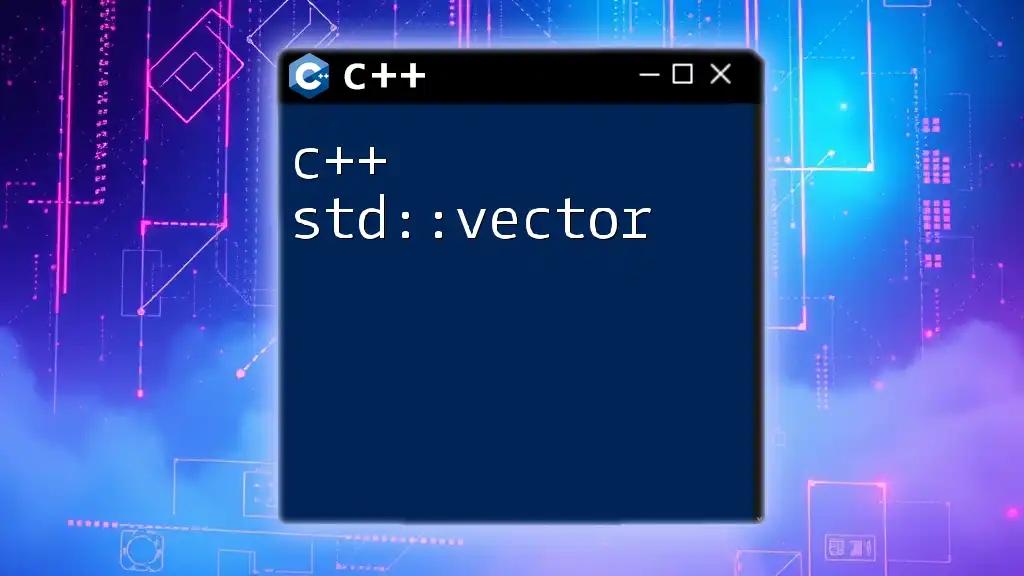
Performance Considerations
Copying Large Vectors
When dealing with large vectors, copying can introduce significant overhead. The costs associated with memory allocation and data copying can lead to performance bottlenecks in your application. Therefore, you should assess the necessity of copying a vector, especially when working with vast amounts of data.
Optimizing Vector Copies
One effective way to optimize vector copies is to utilize move semantics introduced in C++11. By using `std::move`, you can transfer ownership rather than copying data, which significantly reduces overhead. Here’s a brief example:
#include <iostream>
#include <vector>
#include <utility> // For std::move
int main() {
std::vector<int> original = {1, 2, 3, 4, 5};
std::vector<int> movedVector = std::move(original); // Move semantics
// Original vector is now in a valid but unspecified state
for (int val : movedVector) {
std::cout << val << " ";
}
return 0;
}
Using `std::move`, `movedVector` takes over the resources of `original`, leaving `original` in a valid but unspecified state. This technique is especially useful when you no longer need the original vector.
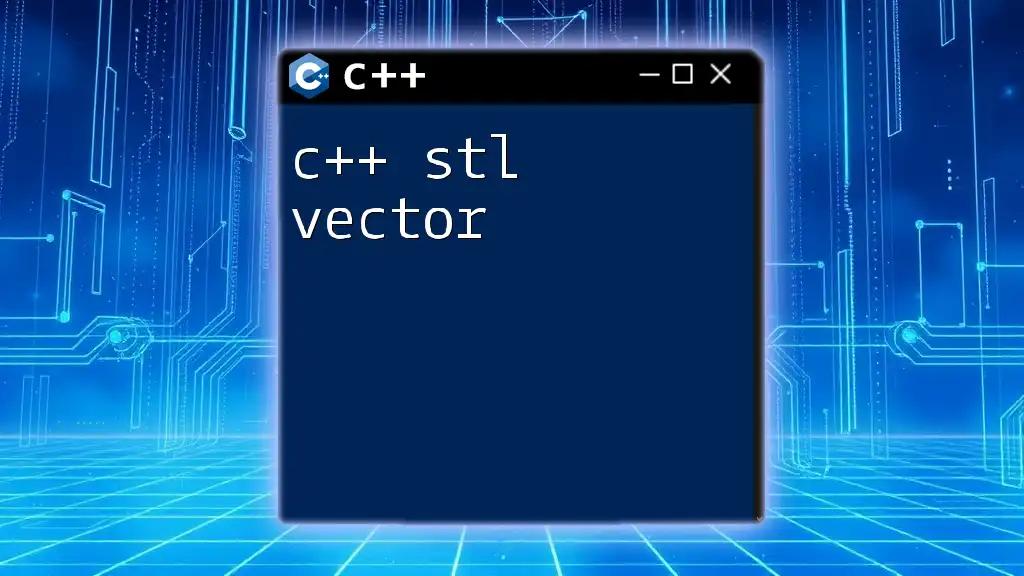
Common Mistakes to Avoid
Forgetting to Resize
One common mistake when copying vectors involves forgetting to resize the destination vector before assignment or copying. If you attempt to copy over a smaller vector without resizing, you may end up with incomplete data or cause undefined behavior. Always ensure that your destination vector is appropriately set up to accommodate the copied data.
Undefined Behavior
Another critical issue arises from shallow copies, especially when dealing with vectors of pointers or resources. If a vector holds pointers and you copy it using the assignment operator, both vectors will point to the same memory addresses. This can lead to undefined behavior if one vector is modified or destroyed, affecting the other. Understanding how memory management works in C++ is essential to avoid such pitfalls.

Conclusion
In summary, the ability to c++ copy vector is a fundamental skill every C++ programmer should master. Each of the discussed methods—using the assignment operator, copy constructor, and `assign` method—offers different advantages and should be used according to the specific requirements of your application.
By understanding the implications of copying, particularly in terms of performance and memory management, you can write more efficient and effective C++ code. Always consider the context in which you're copying vectors and choose the appropriate method accordingly.
Feel free to practice with the examples and explore various scenarios in your development work. There's a wealth of opportunities to enhance your understanding and application of vector copying in C++.