In C++, the `std::vector` is a dynamic array that can grow and shrink in size, providing convenient management of collections of data.
Here's a simple code snippet to demonstrate how to create a new vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Create a new vector of integers
numbers.push_back(10); // Add an element to the vector
numbers.push_back(20); // Add another element to the vector
std::cout << "Vector size: " << numbers.size() << std::endl; // Output: Vector size: 2
return 0;
}
What is a Vector?
A vector in C++ is a part of the Standard Template Library (STL) that implements a dynamic array. Unlike standard arrays, which have a fixed size, vectors can grow and shrink in size as elements are added or removed. This makes vectors a flexible option for handling collections of data.
When comparing vectors to arrays, it’s essential to note that vectors manage their memory automatically. This dynamic management allows developers to focus on functionality rather than complex memory handling often associated with arrays.
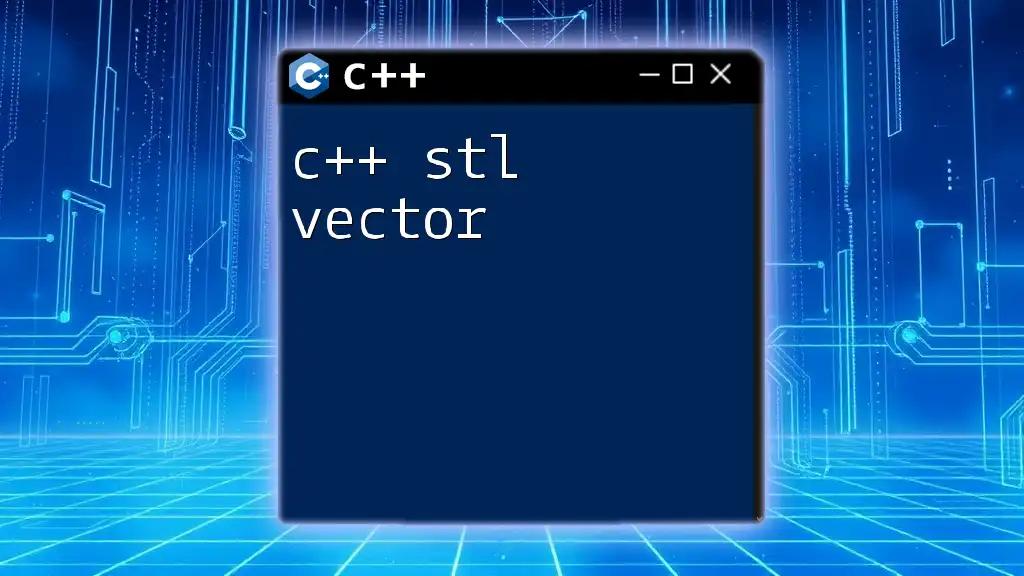
Creating a Vector
To begin using a vector, you need to declare it first. The syntax for declaring a vector is:
std::vector<Type> vectorName;
For example, to declare a vector of integers, you would write:
std::vector<int> myVector;
Once declared, you can initialize a vector in various ways:
- Using initializer lists: This method allows you to populate the vector with initial values directly upon declaration. For example:
std::vector<int> myVector = {1, 2, 3};
- Specifying size and default value: You can create a vector with a predefined size, filling it with a default value. Here’s how to create a vector with 5 elements, all initialized to 0:
std::vector<int> myVector(5, 0);
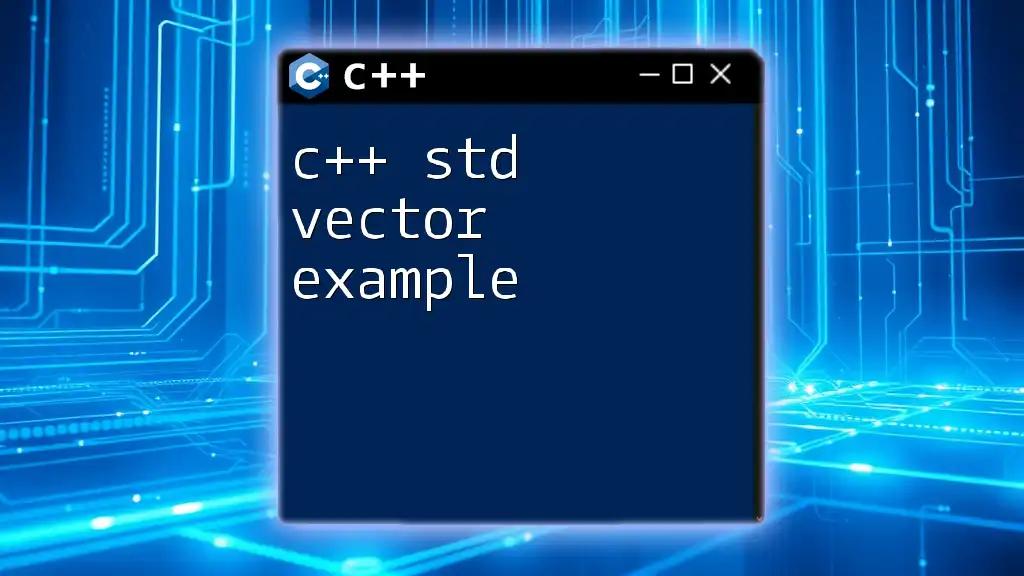
Accessing Elements in a Vector
Accessing elements in a vector can be done through various methods.
- Using the subscript operator: This is the most common way to access elements. For instance, you can retrieve the first element like this:
myVector[0];
- Using the `.at()` method: This method provides bounds checking, which prevents accessing out-of-bounds elements. It throws an `out_of_range` exception if the index does not exist. Here’s an example:
myVector.at(1);
Using `.at()` is generally safer than the subscript operator, especially in scenarios where the vector size may change dynamically during runtime.
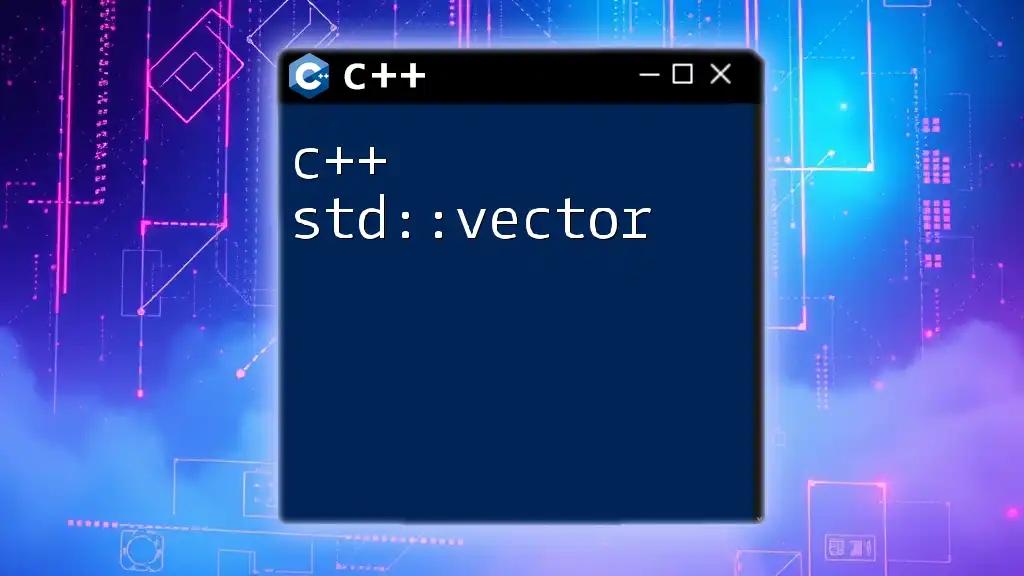
Common Vector Operations
Vectors provide several functions to manipulate the elements they contain.
-
Adding Elements:
- The `push_back()` method adds elements to the end of the vector. For example:
myVector.push_back(4);
- The `insert()` method allows you to insert elements at specific positions. For instance, if you wanted to insert the number 5 at the second position:
myVector.insert(myVector.begin() + 1, 5);
-
Removing Elements:
- To remove the last element in a vector, you can use `pop_back()`:
myVector.pop_back();
- To remove a specific element, `erase()` is utilized. This example would remove the third element:
myVector.erase(myVector.begin() + 2);
-
Clearing the Vector: To remove all elements and free up memory, the `clear()` method is used. It ensures that no memory leaks occur:
myVector.clear();
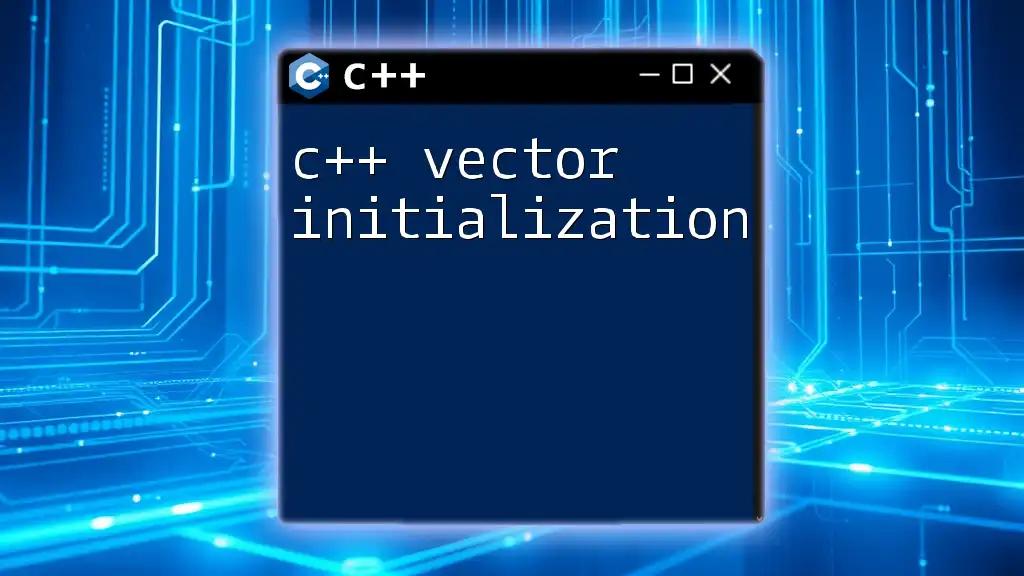
Resizing and Reserving
Managing the capacity of a vector is crucial for performance.
- Resizing a Vector: You can change the size of the vector with the `resize()` method. This method adjusts the size by adding or removing elements. It can also fill new elements with a default value. For example, to resize `myVector` to contain 10 elements:
myVector.resize(10, 0);
- Reserving Space: When you know the approximate number of elements in advance, use `reserve()` to allocate memory. This helps to avoid frequent reallocations. Here’s how you can reserve space for 20 elements:
myVector.reserve(20);
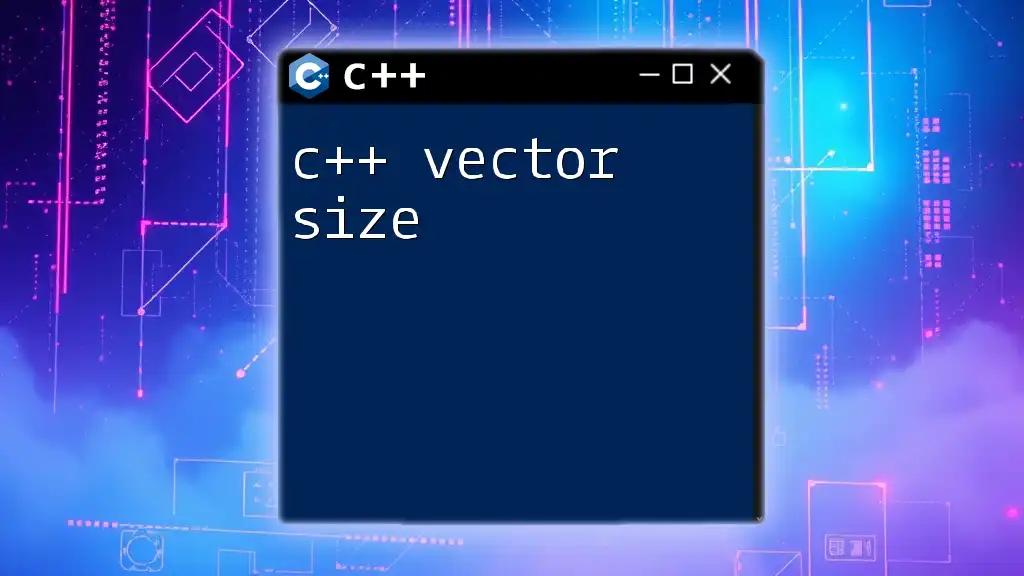
Looping Through a Vector
Iterating over vectors can be done in multiple ways:
- Using a Range-Based For Loop: This is the simplest and most readable way to iterate through elements:
for (const auto& value : myVector) {
std::cout << value << " ";
}
- Using a Traditional For Loop: If you need access to the index, you might prefer the traditional `for` loop:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " ";
}
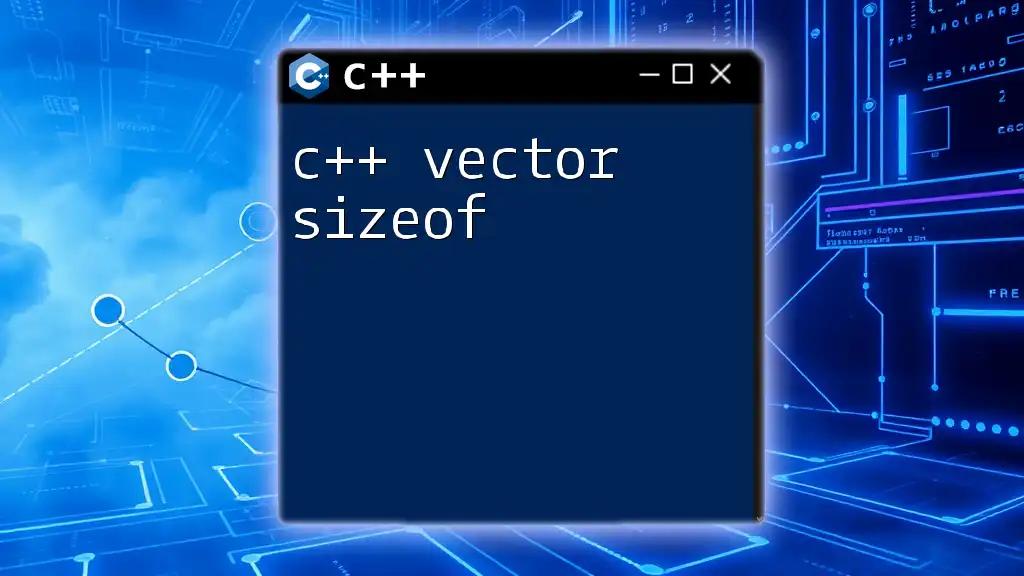
Advanced Vector Features
Exploring advanced features can increase the power of vectors in C++.
- Sorting a Vector: The `std::sort()` function allows sorting in ascending order. Ensure to include the `<algorithm>` header. Here’s how to sort:
std::sort(myVector.begin(), myVector.end());
- Searching for an Element: Use `std::find()` from `<algorithm>` to search for elements. Example code:
auto it = std::find(myVector.begin(), myVector.end(), 2);
if (it != myVector.end()) {
std::cout << "Found 2!";
}
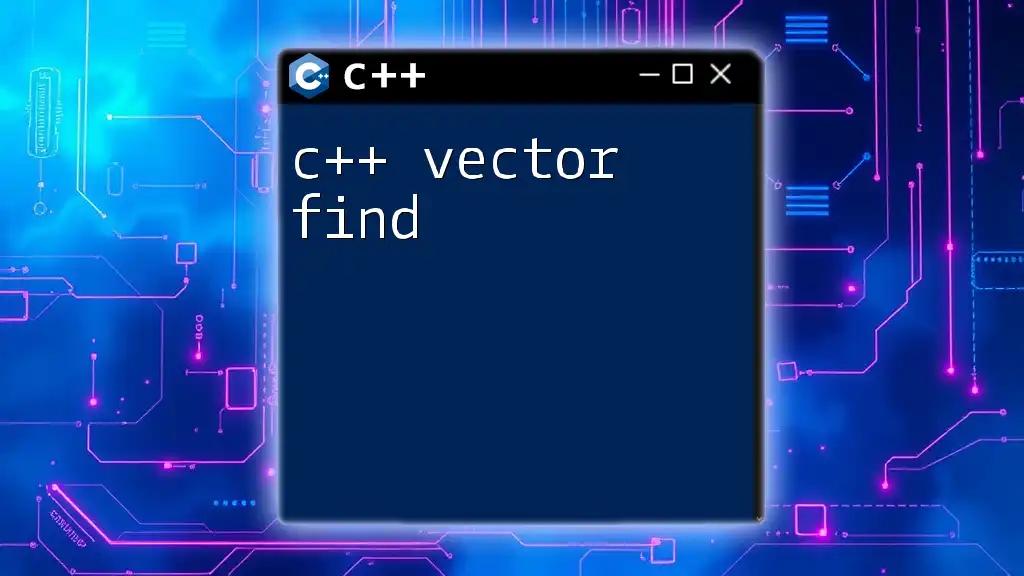
Common Pitfalls and Best Practices
While vectors are powerful, understanding their nuances can prevent headaches later:
-
Overusing `push_back()`: Each call may cause reallocations if the vector’s current capacity is exceeded. It’s wise to use `reserve()` when you know the number of items ahead of time.
-
Understanding vector capacity vs size: Capacity refers to the allocated storage, while size refers to the number of elements. Recognizing this difference can help you write optimized code.
-
Memory management tips: Always clear vectors when they are no longer needed to prevent memory leaks and ensure optimal performance.
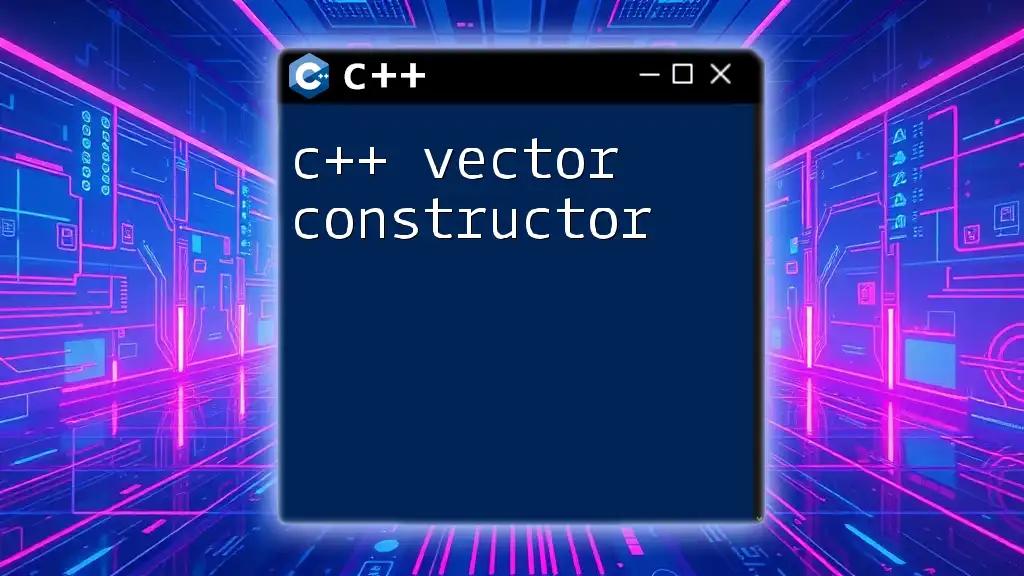
Conclusion
In summary, mastering the C++ new vector functionality equips you with a strong tool for managing collections of data dynamically and efficiently. Vectors offer flexibility, ease of use, and powerful features, enabling developers to handle a wide range of programming scenarios with confidence. Now is the time to experiment with the examples provided and deepen your understanding of vectors in C++.