The `begin()` function in C++ vectors returns an iterator pointing to the first element of the vector, allowing you to traverse the container from the start.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
auto it = nums.begin();
std::cout << *it << std::endl; // Output: 1
return 0;
}
Understanding the `begin` Function
Definition and Purpose of `begin`
The `begin` function is a member function of the C++ Standard Library's `vector` class. This function returns an iterator pointing to the first element of the vector. This is particularly important for iteration, as it serves as a starting point when accessing the contents of the vector.
Syntax of `begin`
The syntax for using the `begin` function is straightforward:
vector_name.begin()
This syntax will be used consistently throughout the article when dealing with vectors.
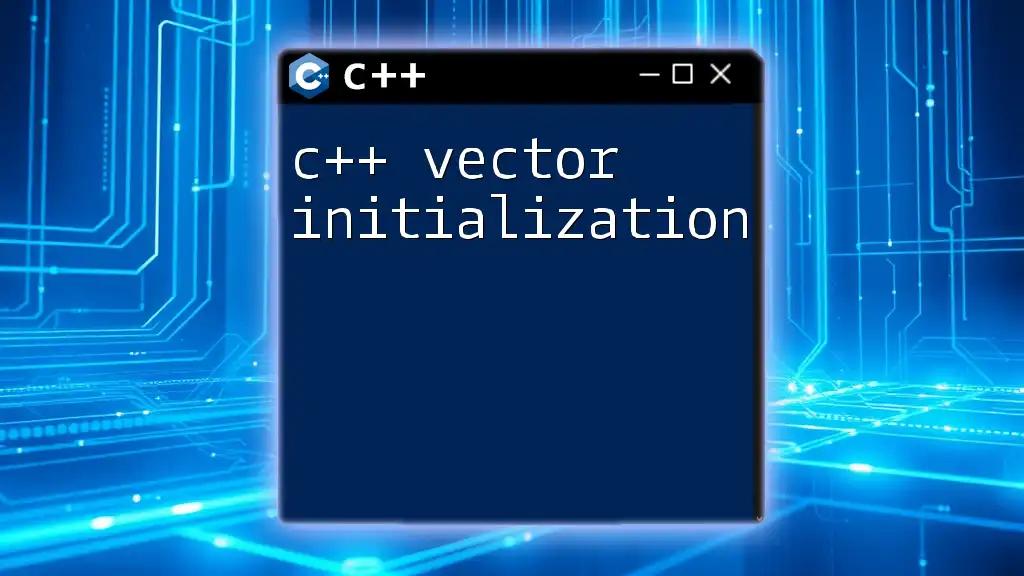
How to Use `begin`
Getting the First Element
One of the simplest uses of `begin` is to access the first element of a vector. By dereferencing the iterator returned by `begin`, you can retrieve the value stored at that position.
Here’s a brief example to illustrate this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40};
std::cout << "First element: " << *numbers.begin() << std::endl; // Output: 10
return 0;
}
In this example, `*numbers.begin()` dereferences the iterator to obtain the first element (in this case, `10`).
Using `begin` with Iterators
Iterators are a critical concept in C++. They provide a flexible way to traverse elements in a vector. By employing a `for-loop`, you can easily access each element starting from the position returned by `begin`.
The following code snippet demonstrates this:
#include <iostream>
#include <vector>
int main() {
std::vector<std::string> fruits = {"apple", "banana", "cherry"};
for (auto it = fruits.begin(); it != fruits.end(); ++it) {
std::cout << *it << std::endl;
}
return 0;
}
In this example, the loop iterates through the vector `fruits`, printing each fruit by dereferencing the iterator `it`, starting from `fruits.begin()` and continuing until `fruits.end()`, which denotes the position just past the last element.
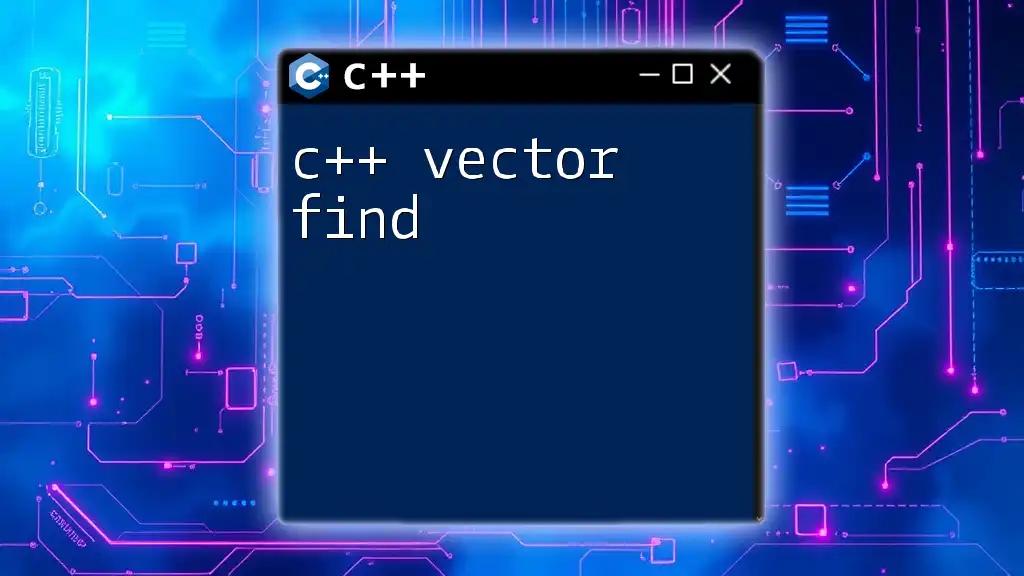
Differences Between `begin()` and `cbegin()`
Understanding `cbegin()`
While `begin` returns a regular iterator that allows modification of the elements in the vector, `cbegin` returns a constant iterator, which is read-only. Using `cbegin` helps prevent accidental modifications to the elements of the vector, thus maintaining data integrity.
Example Comparison
Let’s consider how the two functions can be used:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4};
auto it = numbers.cbegin(); // Constant iterator
// *it = 5; // Uncommenting this line will produce an error: cannot modify value
std::cout << "First element using cbegin: " << *it << std::endl;
return 0;
}
In this example, `numbers.cbegin()` allows you to retrieve the first element without the ability to modify it. Attempting to change the value through `cbegin()` will lead to a compilation error.
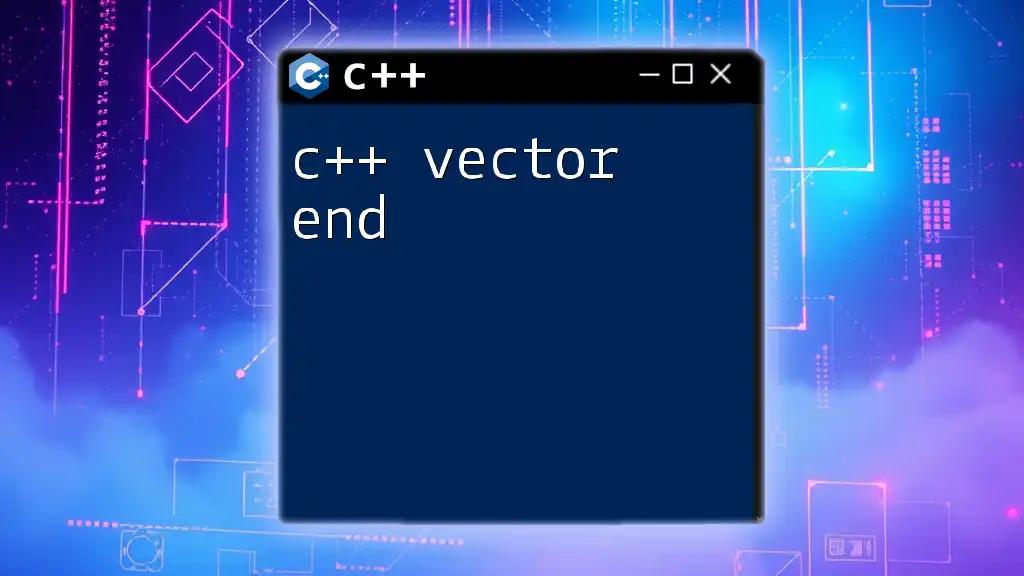
Common Use Cases for `begin`
Iterating Through a Vector
Using `begin` is a best practice for iterating through vectors. It provides a clear and expressive way to access elements. Simply combining `begin` with `end` helps in writing clean, readable code.
Finding Elements
Another powerful use of `begin` lies in finding specific elements within the vector. The Standard Library provides algorithms, such as `std::find`, that simplify this process. Here’s how you can use `begin` to search within a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> data = {10, 20, 30, 40};
auto it = std::find(data.begin(), data.end(), 30); // Looking for 30
if (it != data.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
In this code snippet, `std::find` uses `data.begin()` and `data.end()` to look for the element `30`. If found, it prints the value; otherwise, it indicates that the element is not present.
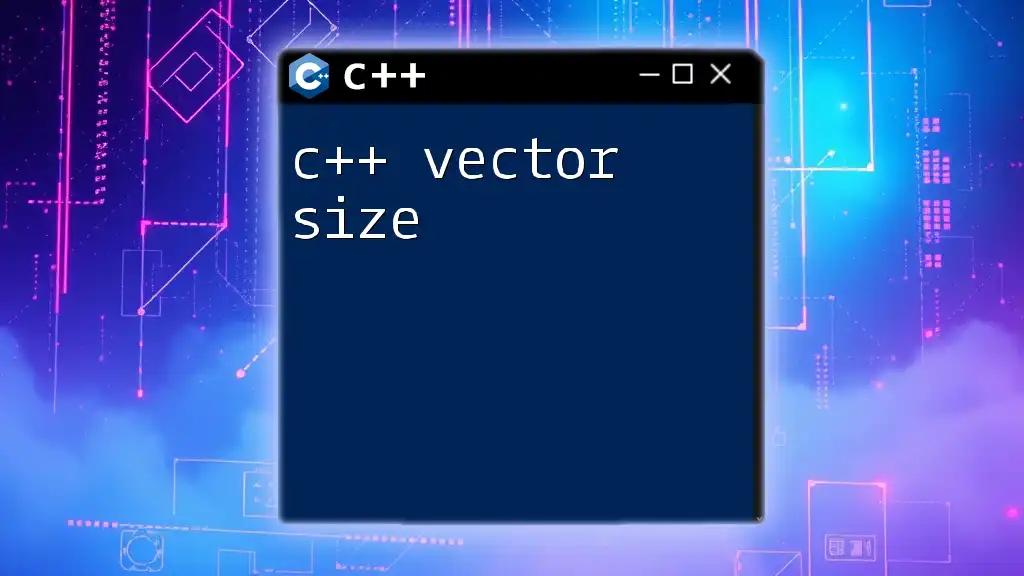
Potential Pitfalls When Using `begin`
Dereferencing Null Iterators
One common mistake developers can make is dereferencing iterators that equal `end()`. If you attempt to dereference such an iterator, it can lead to undefined behavior. Always ensure that the iterator is valid before usage.
Out of Bounds Issues
Never assume a vector is non-empty before calling `begin()`. Always check to see if the vector has elements.
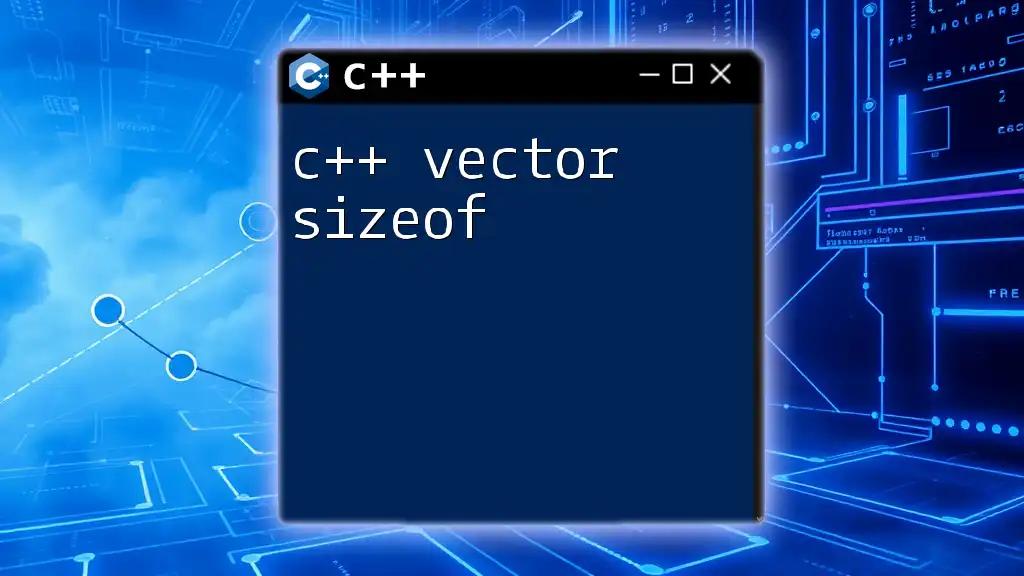
Performance Considerations
Efficiency of `begin`
The `begin` function is highly efficient, particularly when compared to manual index-based loops or raw arrays. It optimizes the access and iteration processes in a way that is not only faster but also cleaner in syntax.
When Not to Use Vectors
While vectors are versatile, there are scenarios where they may not be the best choice. For example, if you require constant time insertion or deletion at arbitrary positions, a linked list might be a better alternative due to its unique characteristics.
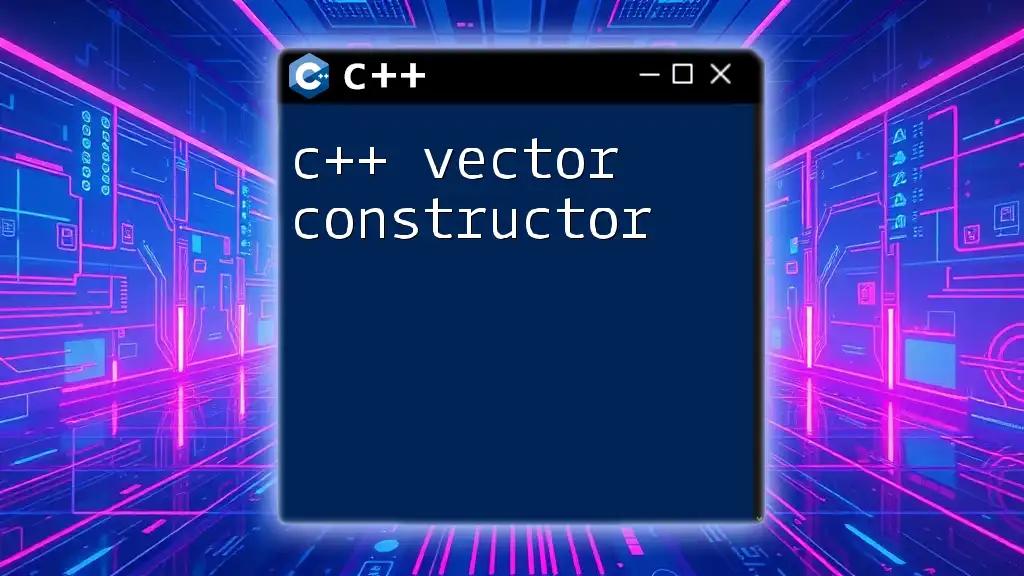
Conclusion
In summary, mastering the `c++ vector begin` function is crucial for effective C++ programming. It simplifies access to elements and allows for easy iteration, making your code cleaner and more efficient.
For further exploration on this topic, consider reading literature focusing on the Standard Template Library (STL) and C++ programming practices. It enhances your understanding and helps you harness the full capabilities of C++ vectors.
Call to Action
We encourage you to experiment with the examples provided and share your experience using `begin` and iterators with vectors. Engaging with the community can enhance your learning and expertise in C++.