In C++, a vector is a dynamic array that can resize itself automatically to accommodate new elements, providing flexibility and ease of use for managing collections of integers.
Here's a simple example of declaring and manipulating a vector of integers in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Declare and initialize a vector
numbers.push_back(6); // Add an element to the end
for (int num : numbers) { // Iterate through the vector
std::cout << num << " ";
}
return 0;
}
Understanding the Basics of `std::vector`
What is a Vector?
In C++, a vector is a dynamic array that allows you to store a sequence of elements. Unlike traditional arrays, the size of vectors can grow and shrink during the runtime of a program. This means you do not need to know the number of elements in advance, making vectors highly flexible and convenient for developers.
What is `std::vector<int>`?
`std::vector<int>` is a specific instantiation of the vector class provided by the Standard Template Library (STL) in C++. This declaration indicates that the vector will only contain integers. It automatically manages storage, enabling you to add or remove integers without needing to manage memory manually.
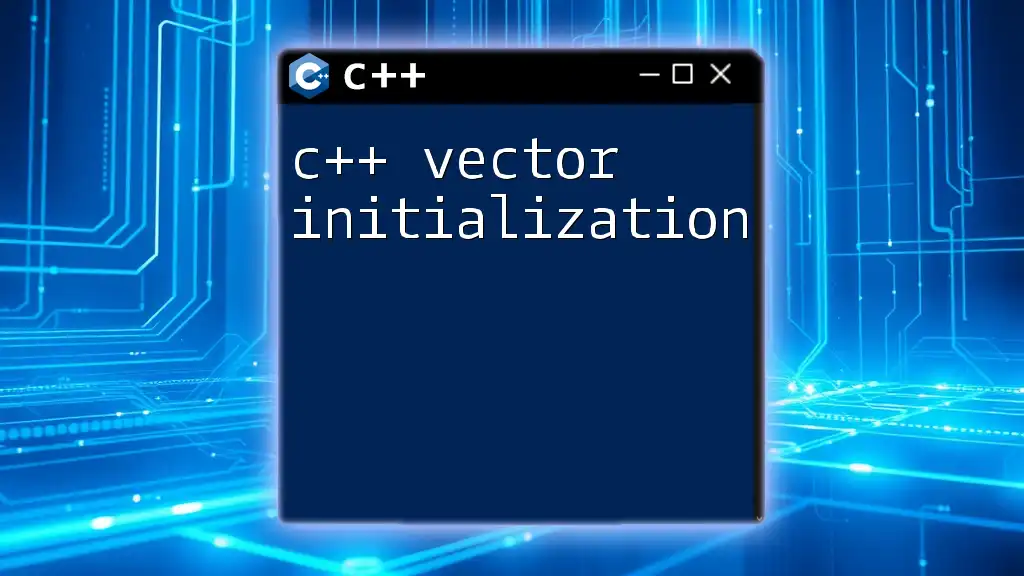
Basic Operations with `std::vector<int>`
Creating a Vector
Creating a `std::vector<int>` is straightforward. The following syntax initializes an empty vector ready to store integers:
#include <vector>
std::vector<int> myVector; // Creating an empty vector of integers
Adding Elements
To add elements to the vector, you can use the `push_back()` method. This method appends an element to the end of the vector, increasing its size dynamically.
myVector.push_back(10); // Adding the integer 10
myVector.push_back(20); // Adding the integer 20
Accessing Elements
Elements in a vector can be accessed using two primary methods: `at()` and operator[]. Using `at()` provides bounds checking and throws an exception if the index is out of range, making it safer.
int firstElement = myVector.at(0); // Safe access using at()
int secondElement = myVector[1]; // Direct access using operator[]
Removing Elements
To remove elements, you can use the `pop_back()` method, which removes the last element from the vector. Alternatively, you can use the `erase()` method to remove an element at a specific position.
myVector.pop_back(); // Removes the last element
myVector.erase(myVector.begin()); // Removes the first element
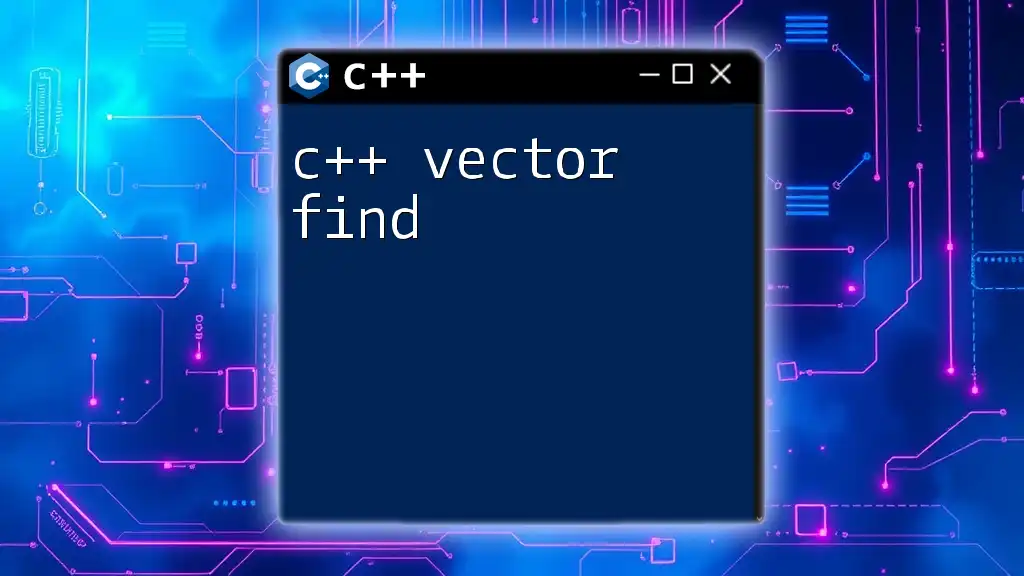
Advanced Operations in `std::vector<int>`
Modifying Elements
Changing the value of an element in a vector is done the same way you would in an array. You simply access the desired index and assign a new value.
myVector[0] = 15; // Changing the first element from 10 to 15
Iterating Through a Vector
When you want to traverse a vector, a range-based for loop is often the most straightforward approach. This loop automatically handles the index management for you.
for (int num : myVector) {
std::cout << num << " "; // Outputting each element
}
Sorting a Vector
Vectors can be sorted easily using the `std::sort()` function from the `<algorithm>` header. This allows you to arrange the elements in ascending order.
#include <algorithm>
std::sort(myVector.begin(), myVector.end()); // Sorting the vector in ascending order
Clearing a Vector
If you want to remove all elements from a vector, you can employ the `clear()` method. This method sets the size of the vector to zero while destroying all contained elements.
myVector.clear(); // Removes all elements from the vector
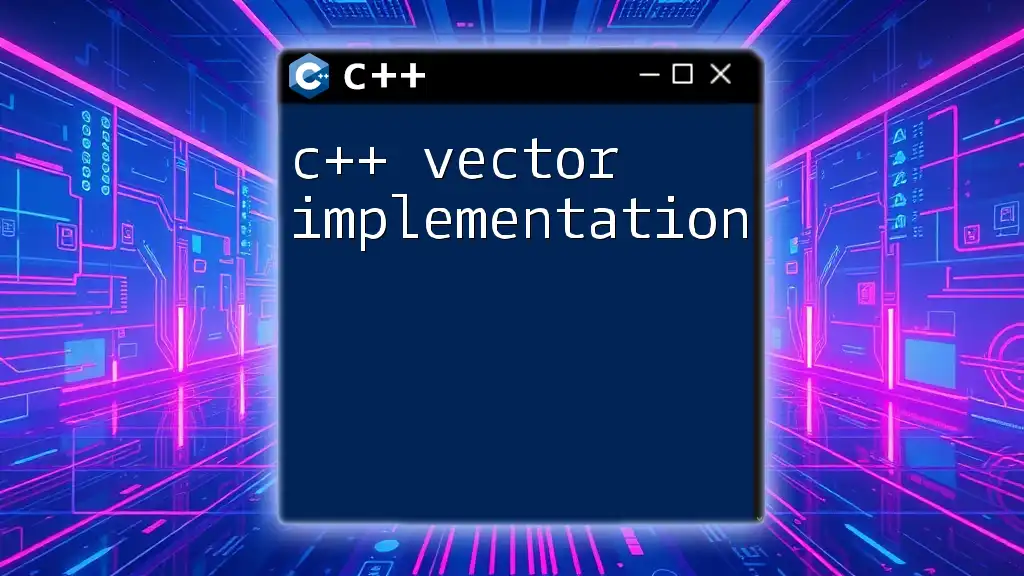
Performance Considerations
Memory Management
One of the key advantages of vectors is their ability to manage memory automatically. They have a concept of capacity, which reflects the amount of memory allocated, and size, which reflects the number of elements currently in the vector. Resizing might involve reallocating memory, which can impact performance.
Time Complexity of Vector Operations
Understanding the time complexity of vector operations can help you write more efficient code. For example, appending elements using `push_back()` and removing elements using `pop_back()` generally run in O(1) time. However, operations like `insert()` and `erase()` can be more costly, operating at O(n) complexity.
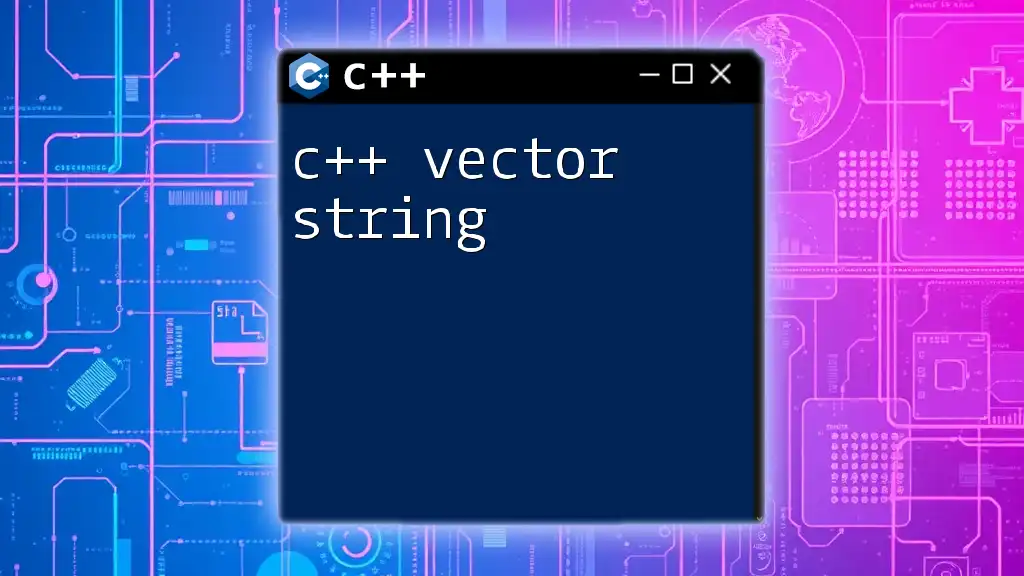
Common Use Cases for `std::vector<int>`
Applications in Competitive Programming
Vectors are widely used in competitive programming due to their efficiency in managing collections of data. They allow for quick access, modification, and dynamic resizing, matching the fast-paced nature of competitive coding.
Use Cases in Real World Applications
In real-world software development, `std::vector<int>` can be employed for a variety of tasks, including data processing where you may need to handle an unspecified number of outcomes, gaming applications for managing scores or health points, and simulations where data size can vary dramatically.
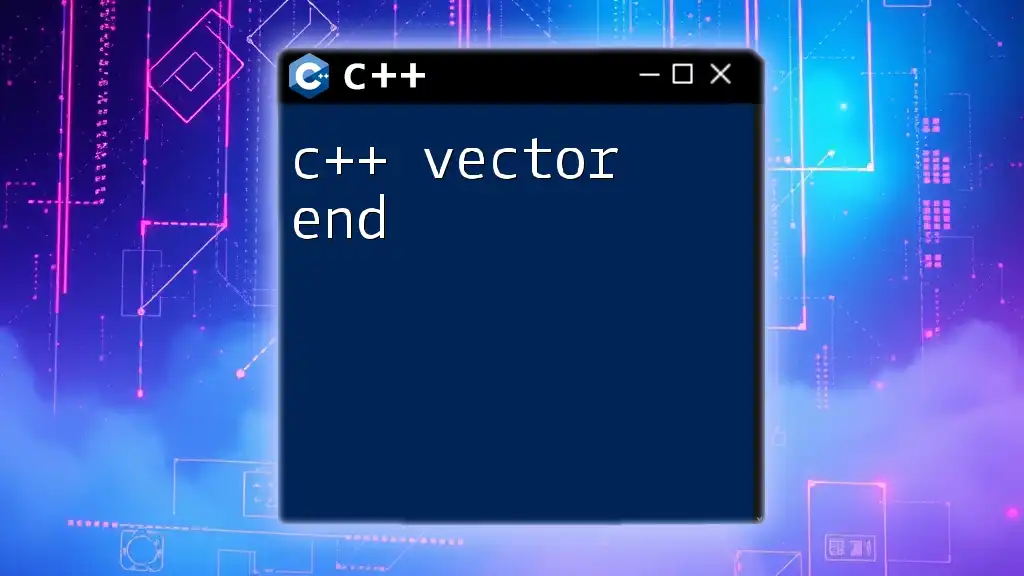
Error Handling with `std::vector<int>`
Handling Out-of-Bounds Access
Out-of-bounds access is a common source of bugs in C++. While using operator[] can lead to undefined behavior if the index is out of range, the `at()` method provides safe access with error throwing capabilities. Always prefer `at()` in scenarios where indices might be variable or uncertain.
Memory Leaks
Although vectors manage their own memory, programmers should still be cautious. Using smart pointers along with vectors when dealing with dynamically allocated objects can prevent memory leaks and ensure resources are appropriately released.
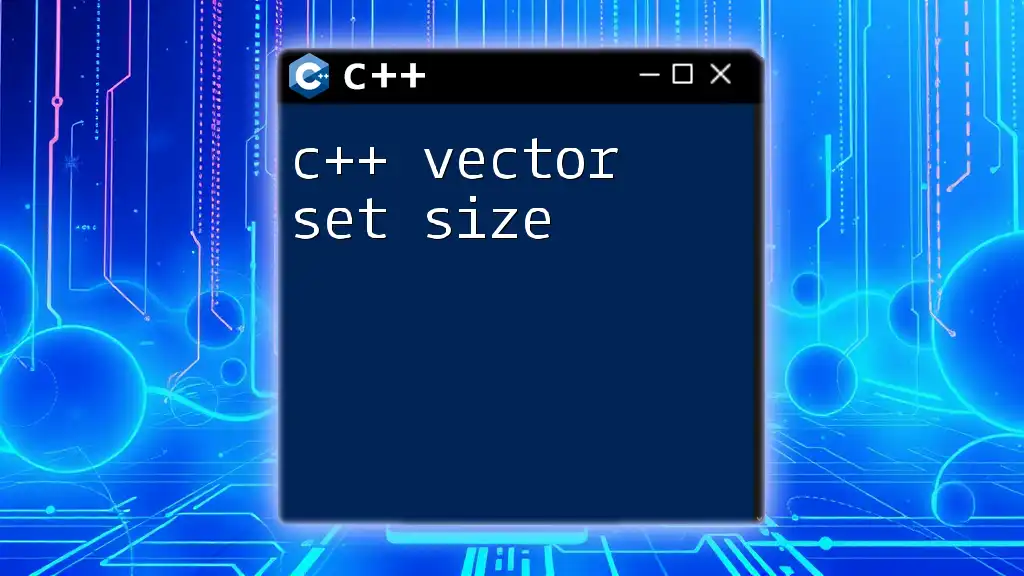
Conclusion
Mastering `std::vector<int>` is essential for any C++ developer. Understanding how to utilize and manipulate vectors enhances your programming capabilities and allows for more efficient and effective code. With dynamic resizing, intuitive operations, and robust performance, `std::vector<int>` stands out as an indispensable component of C++ programming. Embrace the power of vectors, and continue your journey in exploring the rich functionalities provided by C++ STL.
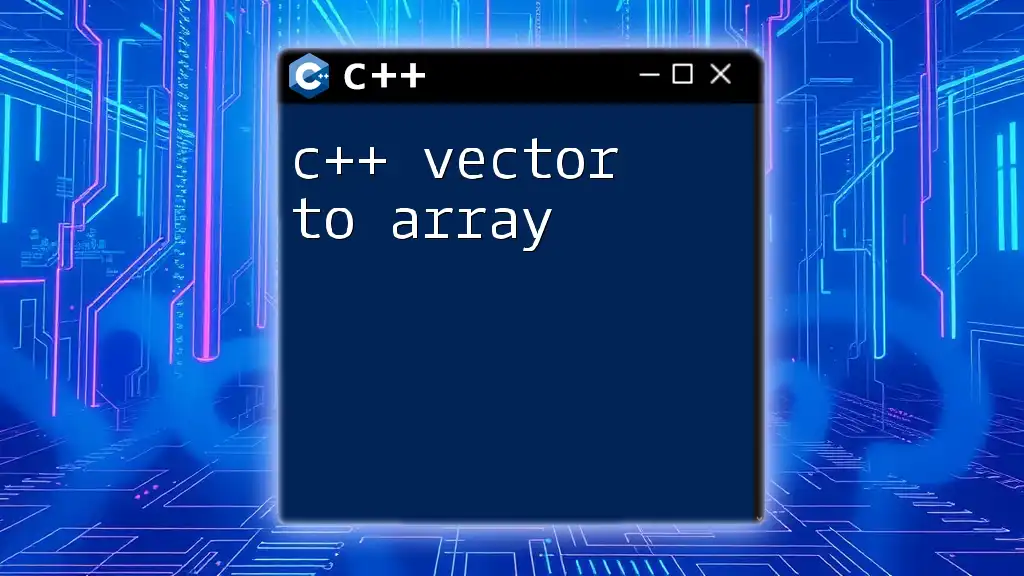
Additional Resources
For further learning, consider consulting the official C++ documentation on `std::vector`. Explore coding platforms to practice vector-related problems, or dive into specialized books that cover advanced C++ topics to deepen your understanding.