In C++, you can convert a `std::vector<std::string>` to a single `std::string` by joining its elements with a specified delimiter, such as a comma. Here's how you can do it:
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
std::string vectorToString(const std::vector<std::string>& vec, const std::string& delimiter) {
std::string result;
for (const auto& str : vec) {
if (!result.empty()) result += delimiter;
result += str;
}
return result;
}
int main() {
std::vector<std::string> vec = {"Hello", "world", "from", "C++"};
std::string joinedString = vectorToString(vec, ", ");
std::cout << joinedString << std::endl; // Output: Hello, world, from, C++
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a dynamic array that is part of the Standard Template Library (STL). Unlike traditional arrays, vectors can grow and shrink in size, making them a versatile option for managing collections of data. This adaptability comes with the benefit of automatic memory management, allowing developers to handle larger datasets without worrying about exceeding predefined limits.
Vectors hold elements of the same type and support a variety of operations, including:
- Adding elements with `push_back()`
- Removing elements with `pop_back()`
- Accessing elements using the subscript operator (`[]`)
Why Convert a Vector to a String?
Converting a C++ vector to a string is essential in several scenarios:
- Logging: String representations of vectors are often required when generating logs or alerts.
- Data Serialization: In applications where data needs to be transmitted over a network, converting vectors to a string format can make serialization easier.
- User Output: Displaying data in a user-friendly format often necessitates string conversion, especially in console applications.
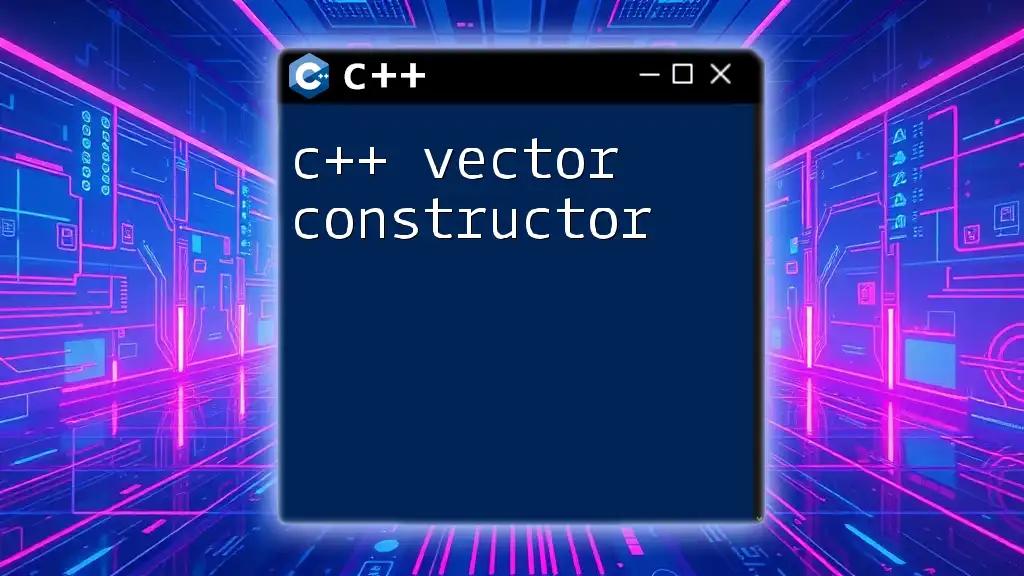
Methods for Converting a C++ Vector to a String
Using `std::ostringstream`
`std::ostringstream` is a powerful utility for string manipulation in C++. It allows you to construct a string progressively by streaming data into it.
Code Example:
#include <iostream>
#include <vector>
#include <sstream>
#include <string>
std::string vectorToString(const std::vector<int>& vec) {
std::ostringstream oss; // Create an output string stream
for (const auto& item : vec) {
oss << item << " "; // Stream elements to the output
}
return oss.str(); // Convert stream to string
}
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::string result = vectorToString(myVector);
std::cout << "Vector to string: " << result << std::endl; // Output the result
return 0;
}
In this example, an output string stream (`oss`) is used to concatenate each element of the vector followed by a space. After all elements are processed, `oss.str()` retrieves the complete string representation.
Using `std::string` and `std::accumulate`
The `std::accumulate` function from the `<numeric>` header enables a streamlined aggregation of vector elements. This method employs a lambda function to append each element to an existing string.
Code Example:
#include <iostream>
#include <vector>
#include <string>
#include <numeric>
std::string vectorToString(const std::vector<int>& vec) {
return std::accumulate(vec.begin(), vec.end(), std::string{},
[](const std::string& a, int b) {
return a + (a.empty() ? "" : " ") + std::to_string(b); // Handle spacing
});
}
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::string result = vectorToString(myVector);
std::cout << "Vector to string: " << result << std::endl; // Output the result
return 0;
}
In this code, `std::accumulate` operates over the vector, accumulating each integer into a string while managing spaces through the lambda function. This method is efficient, concise, and highlights the power of functional programming in C++.
Using Range-based for Loop with String Concatenation
The range-based for loop provides a clean syntax for iterating through vectors, making it an excellent choice for simple conversions.
Code Example:
#include <iostream>
#include <vector>
#include <string>
std::string vectorToString(const std::vector<int>& vec) {
std::string result; // Initialize an empty string
for (const auto& item : vec) {
result += std::to_string(item) + " "; // Concatenate each item
}
return result; // Return the final string
}
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::string result = vectorToString(myVector);
std::cout << "Vector to string: " << result << std::endl; // Output the result
return 0;
}
This approach is straightforward but can be less performant for larger vectors due to continuous memory reallocations. However, for small vectors or simpler use cases, it’s effective and easy to read.
Handling Different Data Types in Vectors
Using Templates for Generalization
To make the conversion function more versatile, you can utilize C++ templates. This allows the same function to work with vectors of various data types.
Code Example:
#include <iostream>
#include <vector>
#include <string>
#include <sstream>
template <typename T>
std::string vectorToString(const std::vector<T>& vec) {
std::ostringstream oss;
for (const auto& item : vec) {
oss << item << " "; // Stream each item into the string
}
return oss.str(); // Return the resulting string
}
int main() {
std::vector<double> myVector = {1.5, 2.3, 3.1};
std::string result = vectorToString(myVector);
std::cout << "Vector to string: " << result << std::endl; // Output the result
return 0;
}
Templates enhance reusability, allowing the same conversion function to handle integers, doubles, strings, and other types seamlessly.
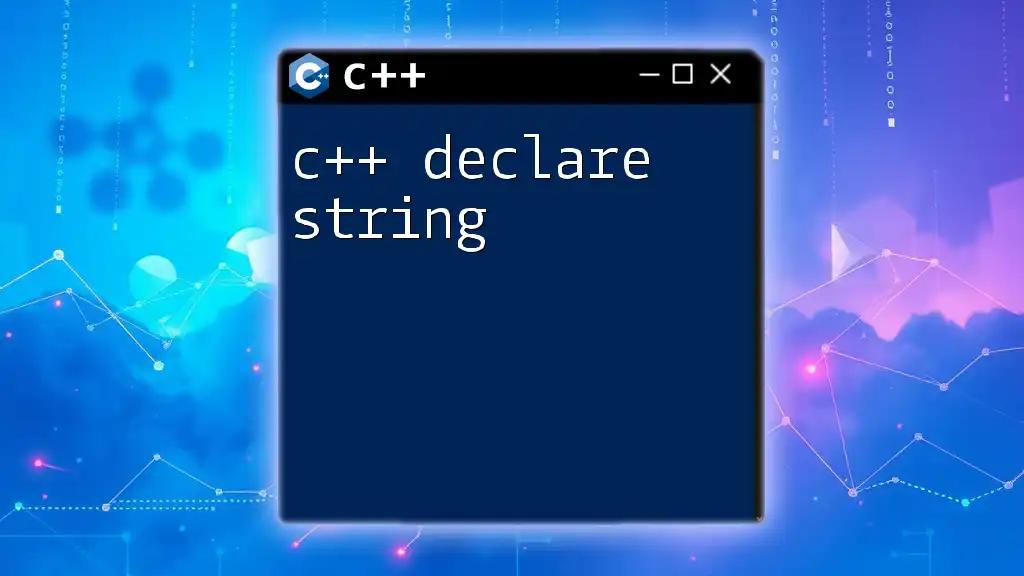
Best Practices
Optimal Performance Tips
When converting a vector to a string, consider the implications of repeated string concatenations, which can lead to performance issues—especially in large datasets. Using `std::ostringstream` or pre-reserved strings (via `resize()`) can mitigate such performance hits.
Readability and Maintainability
Readable code is as crucial as functional code. Use clear variable names and maintain organized structures. Commenting your code helps others (and yourself) understand the logic at a glance, ensuring maintainability.

Conclusion
In summary, converting a C++ vector to a string can be achieved through various methods, each suited to different needs and scenarios. Whether using `std::ostringstream`, `std::accumulate`, or a basic range-based for loop, developing a solid understanding of these methods can streamline your programming process.
Experiment with different data types and implementations to enhance your skills and ensure your C++ programs efficiently handle vector-to-string conversions. Should you have any questions or experiences to share, feel free to leave a comment below!