In C++, a vector of strings is a dynamic array that can store multiple string elements, allowing for easy manipulation and access, as demonstrated in the code snippet below:
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> fruits = {"Apple", "Banana", "Cherry"};
for (const auto& fruit : fruits) {
std::cout << fruit << std::endl;
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow or shrink in size. Unlike traditional arrays, which have a fixed size, vectors manage their own memory, allowing you to add or remove elements easily.
Vectors are part of the C++ Standard Library, providing a convenient and efficient way to handle collections of data. When it comes to a vector of strings, it offers an exceptional solution for storing a list of textual data with flexibility and ease of manipulation.
Why Use Vectors for Strings?
Choosing vectors for strings brings several advantages:
- Dynamic Sizing: You can easily add or remove strings without needing to know the size in advance.
- Built-in Methods: The `std::vector` class in C++ comes with numerous built-in functions that make it easier to deal with collections.
- Ease of Manipulation: You can quickly access, modify, and iterate over strings stored in the vector.
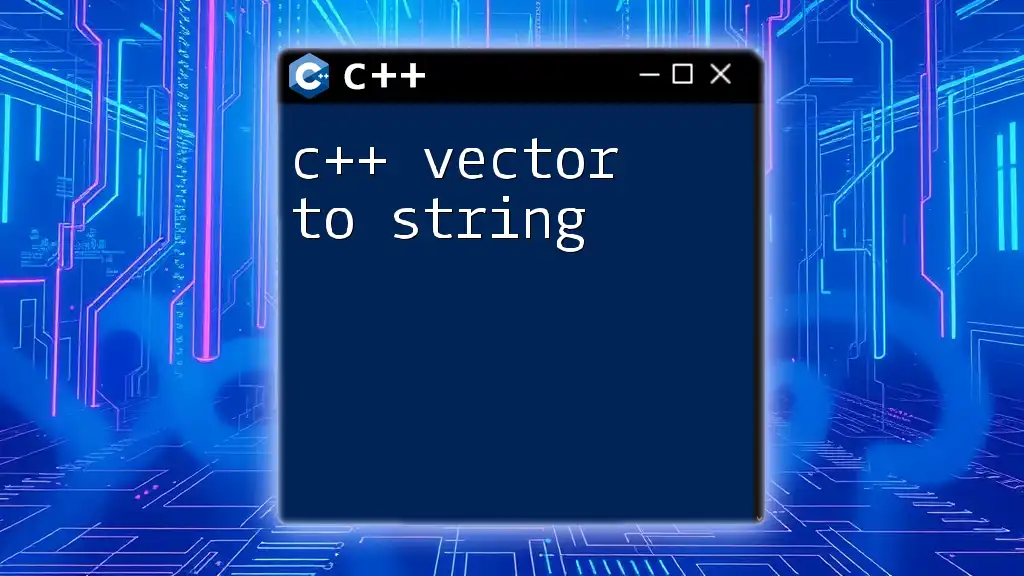
Declaring and Initializing a Vector of Strings
Basic Declaration
To declare a vector of strings in C++, you can use the following syntax:
std::vector<std::string> stringVector;
This creates an empty vector called `stringVector` that will be capable of holding strings.
Initializing a Vector of Strings
You can initialize a vector of strings using list initialization. Here’s how you can do it:
std::vector<std::string> stringVector = {"apple", "banana", "cherry"};
In this example, the vector is initialized with three string elements, which can later be manipulated as needed.
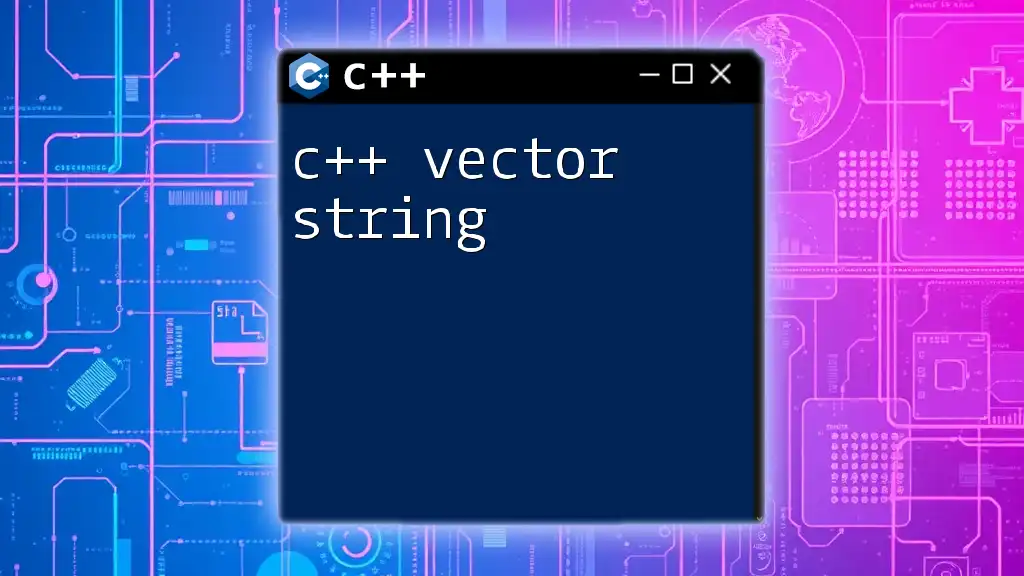
Common Operations on a Vector of Strings
Adding and Removing Strings
Adding and removing elements from a vector of strings is straightforward. To add an element to the end of the vector, use `push_back()` like this:
stringVector.push_back("date");
If you want to remove the last element, you can use `pop_back()`:
stringVector.pop_back(); // This will remove "date"
Accessing Elements
When you want to access elements in a vector, you can either use the `[]` operator or the `.at()` method. Here's a simple usage:
std::cout << stringVector[0]; // Outputs "apple"
std::cout << stringVector.at(1); // Outputs "banana"
Using `.at()` offers benefits in the form of bounds checking, which helps avoid out-of-bounds access that can lead to errors.
Iterating Through a Vector of Strings
Iterating through a vector can be performed in multiple ways, with the range-based for loop being particularly elegant:
for (const auto& str : stringVector) {
std::cout << str << std::endl; // Prints each string in the vector
}
This loop automatically handles the size of the vector, making it safer and more readable.
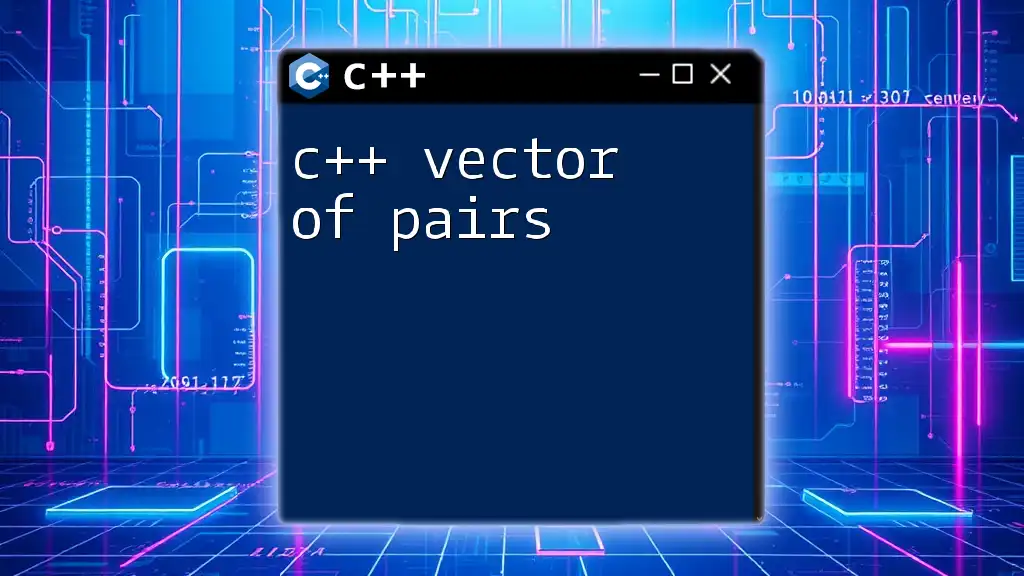
Useful Vector Functions for Strings
Sorting a Vector of Strings
Sorting is a common operation. You can sort a vector of strings using the `std::sort()` function:
#include <algorithm> // Required for std::sort
std::sort(stringVector.begin(), stringVector.end());
This will sort the strings in ascending order, allowing for easy organization of data.
Finding Elements
To find a specific element in a vector, you can use the `std::find()` function from the `<algorithm>` header:
#include <algorithm> // Required for std::find
auto it = std::find(stringVector.begin(), stringVector.end(), "banana");
if (it != stringVector.end()) {
std::cout << "Found banana!";
}
This method returns an iterator to the found element or the end iterator if the element is not found.
Vector Size and Capacity
To gauge how many elements are in a vector, you can use the `.size()` method:
std::cout << "Size: " << stringVector.size(); // Number of elements
To check the current allocated space for the vector, use `.capacity()`:
std::cout << "Capacity: " << stringVector.capacity(); // Current allocated space
Understanding these metrics helps in managing memory effectively, especially in performance-critical applications.
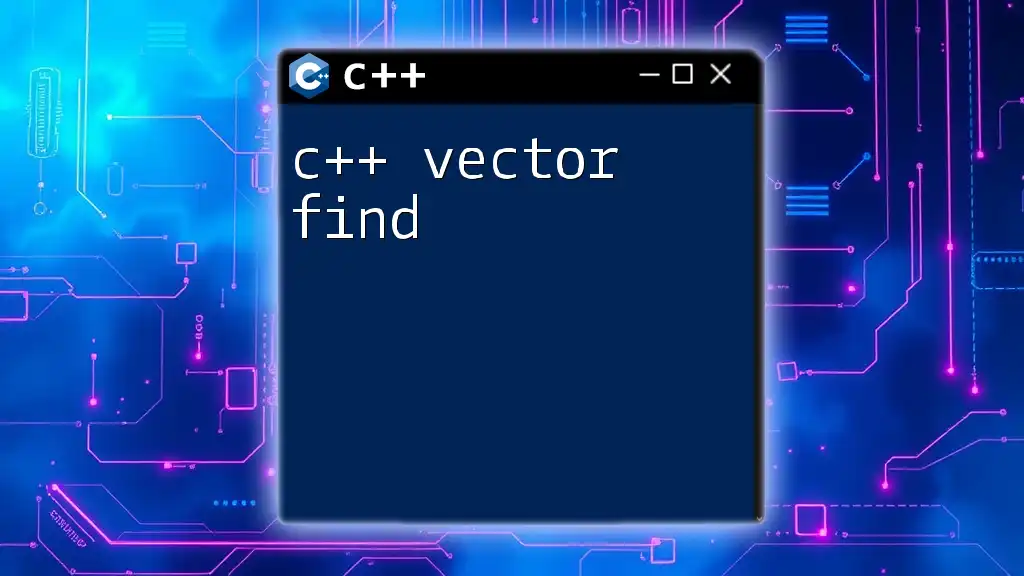
Practical Applications of a Vector of Strings
Storing User Input
A practical application of a vector of strings could be to collect names from user input. Here’s how you can do it:
std::string name;
while (std::cin >> name) {
stringVector.push_back(name); // Collects names until EOF
}
This loop continually takes inputs until the end of the file (EOF) is reached, dynamically storing them in the vector.
Managing a List of Files or URLs
Vectors are also beneficial when managing lists, such as file names or URLs. You can easily store, access, and manipulate these entities in a vector of strings, maintaining clarity and efficiency throughout your codebase.
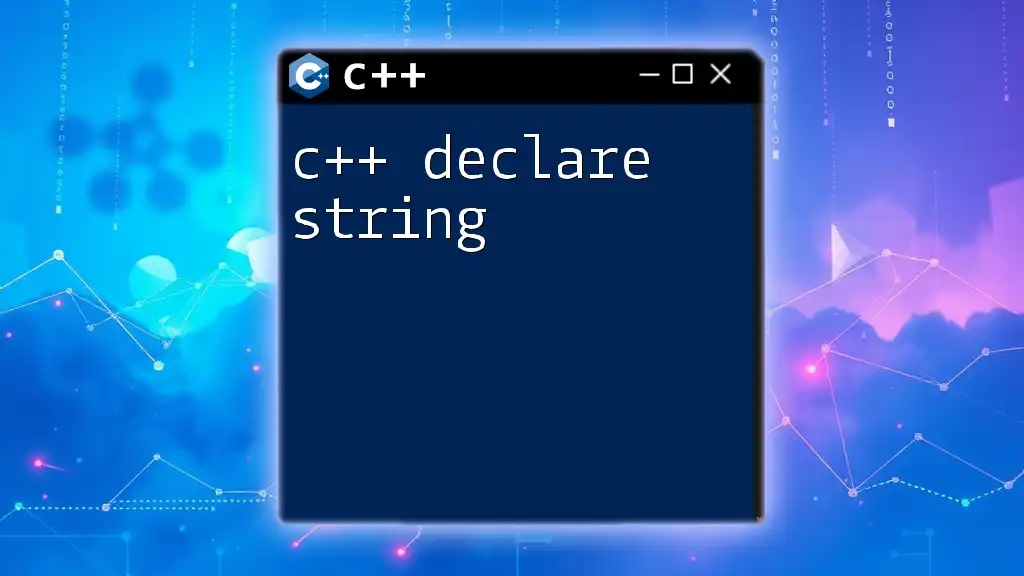
Best Practices for Using Vector of Strings
Memory Considerations
While vectors are dynamic, understanding when to use them versus other data structures is crucial for memory efficiency. Avoid excessive growth or shrinking, as this can lead to fragmentation and reduced performance.
Efficiency
To enhance performance when you know the approximate number of elements the vector will contain, consider using the `.reserve()` method:
stringVector.reserve(100); // Reserves space for 100 strings
This preallocates memory, minimizing the need for multiple reallocations and improving the efficiency of adding elements.
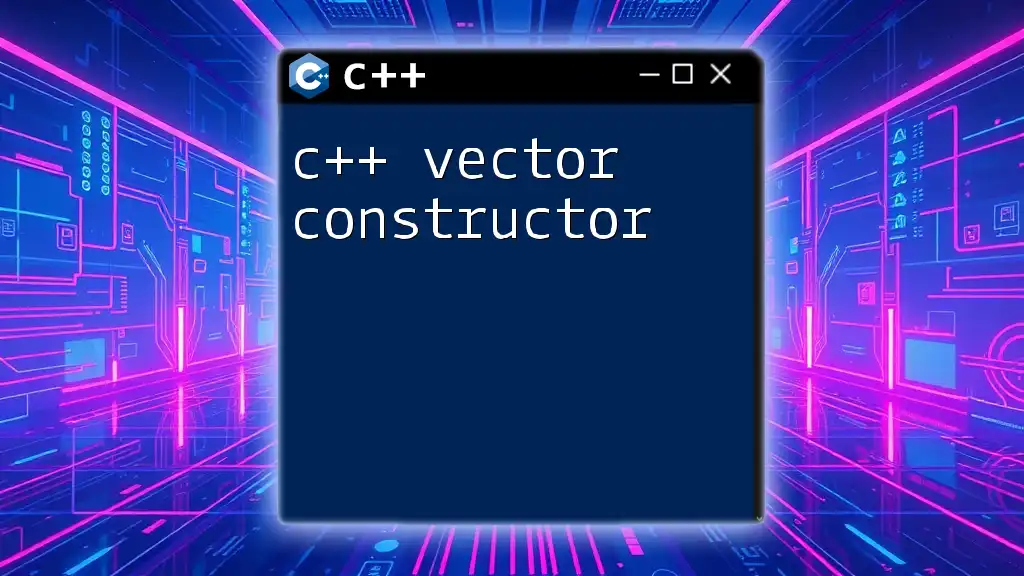
Conclusion
Using a C++ vector of strings can significantly simplify the management and manipulation of string data within your applications. By understanding the advantages, operations, and best practices associated with vectors, you can harness the full potential of dynamic collection types in C++. As you practice with these operations and explore their varied applications, you'll enhance your overall C++ programming proficiency.
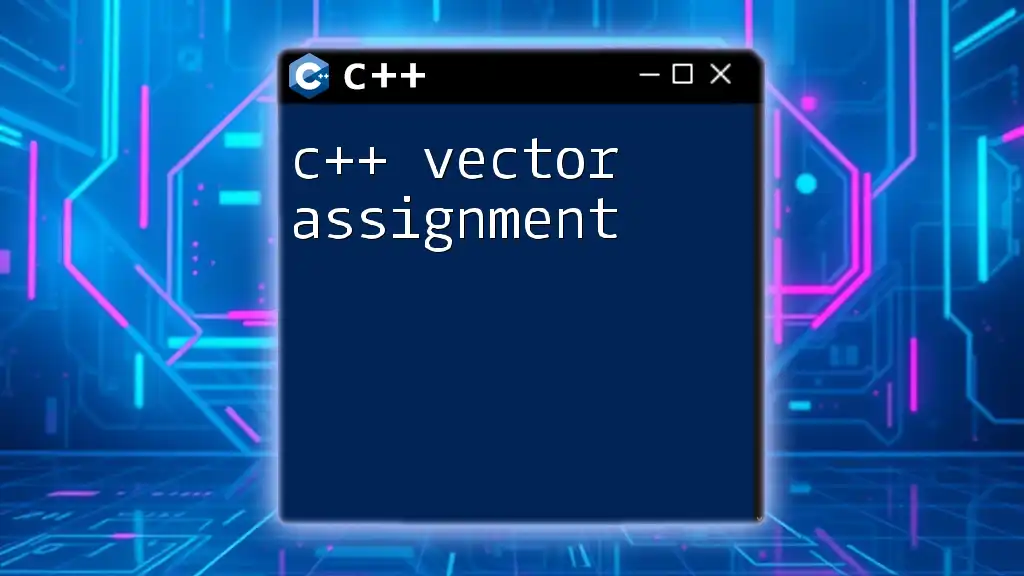
Additional Resources
For a deeper understanding of C++ vectors and the Standard Library, consider checking the official documentation, exploring recommended books, or enrolling in online courses that cover C++ fundamentals and advanced topics. Practicing with real-world examples will solidify your knowledge and prepare you for more complex programming challenges.