A C++ vector of iterators is a dynamic array that stores iterators pointing to elements in another container, allowing for flexible and efficient access and manipulation of those elements. Here's a quick example:
#include <iostream>
#include <vector>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
std::vector<std::list<int>::iterator> vecIterators;
for (auto it = myList.begin(); it != myList.end(); ++it) {
vecIterators.push_back(it);
}
// Access and print elements using the vector of iterators
for (auto it : vecIterators) {
std::cout << *it << " ";
}
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a part of the Standard Template Library (STL) and represents a dynamic array that can resize itself automatically when elements are added or removed. Unlike traditional arrays, C++ vectors provide the following advantages:
- Dynamic Size: They can grow and shrink in size, consuming only the memory they need.
- Random Access: Elements can be accessed using an index, just like an array.
- Member Functions: Vectors come with multiple member functions that simplify element management, such as `push_back()`, `pop_back()`, and `insert()`.
C++ vectors are implemented as templates, allowing them to hold elements of any data type.
Basic Operations on C++ Vectors
Working with C++ vectors involves several common operations. Below are some basic operations, along with code examples:
- Adding elements: You can add elements to a vector using `push_back()`.
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec;
vec.push_back(10);
vec.push_back(20);
vec.push_back(30);
for(int i : vec) {
std::cout << i << " ";
}
return 0;
}
- Removing elements: Elements can be removed using `pop_back()` or `erase()`.
vec.pop_back(); // Removes the last element
vec.erase(vec.begin()); // Removes the first element
- Accessing elements using indices: Elements can be accessed directly using their index.
std::cout << vec[1]; // Accesses the second element
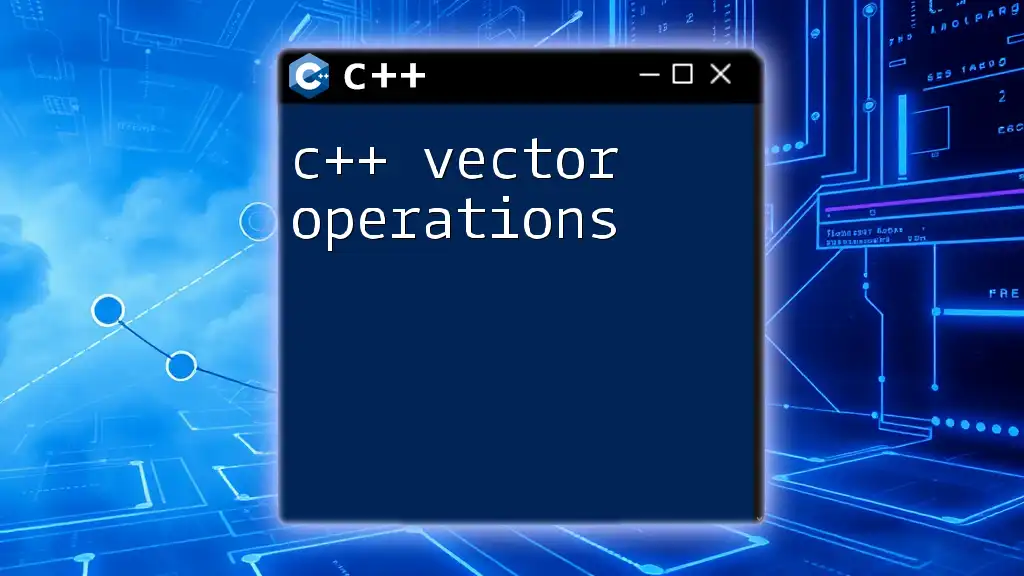
Introduction to Iterators
What is an Iterator?
An iterator in C++ is an abstract pointer that allows a programmer to traverse a container like a vector, list, or map. Unlike raw pointers, iterators provide a more generalized and safer way to navigate through the data structure.
The Importance of Iterators in C++
Using iterators over direct indexing provides several benefits:
- Generic Traversal: They offer a uniform way to traverse any STL container.
- Safety: Iterators prevent out-of-bounds errors that may occur with traditional indexing.
- Cleaner Syntax: Using iterators often leads to cleaner, more readable code.
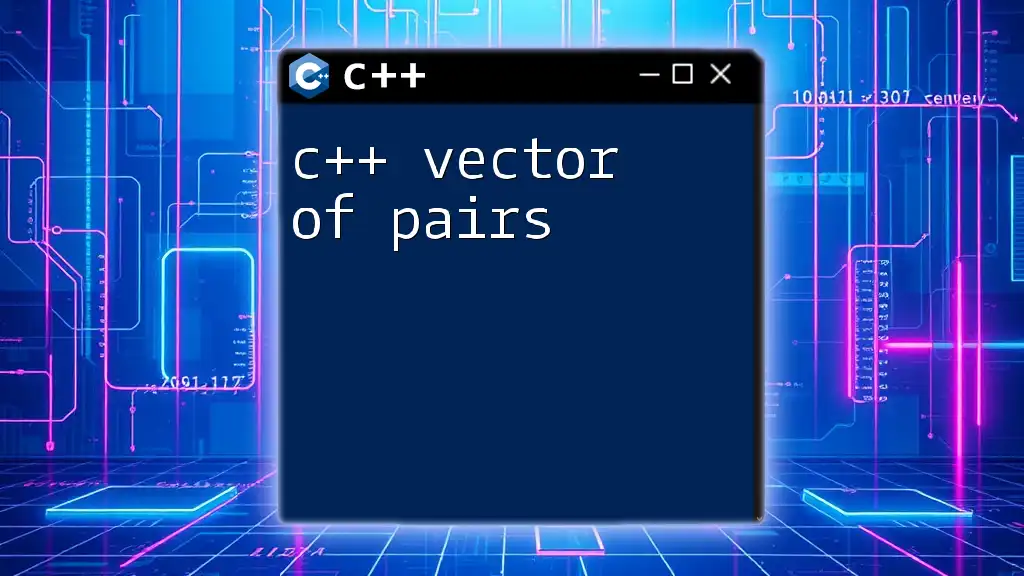
C++ Vector Iterators
Types of Iterators for Vectors
Begin and End Iterators
Vectors in C++ provide two essential functions: `begin()` and `end()`. The `begin()` function returns an iterator pointing to the first element of the vector, while the `end()` function returns an iterator pointing to one past the last element.
Example:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for(auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " "; // Dereferencing iterator to access value
}
return 0;
}
Const Iterators
Const iterators allow read-only access to the elements of a vector, ensuring that the elements cannot be modified through the iterator.
Example:
void printVector(const std::vector<int>& vec) {
for(auto it = vec.cbegin(); it != vec.cend(); ++it) {
std::cout << *it << " ";
}
}
C++ `std::vector` Iterators vs Other Iterators
While `std::vector` iterators are commonly used, each container type has its own iterator characteristics. For example, `std::list` provides bidirectional iterators, while `std::set` has unique sorted iterators.
The differences become apparent through operations. Here's an example contrasting the two:
#include <vector>
#include <list>
#include <iostream>
int main() {
std::list<int> lst = {1, 2, 3};
std::vector<int> vec = {4, 5, 6};
for(auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
for(auto it = lst.begin(); it != lst.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
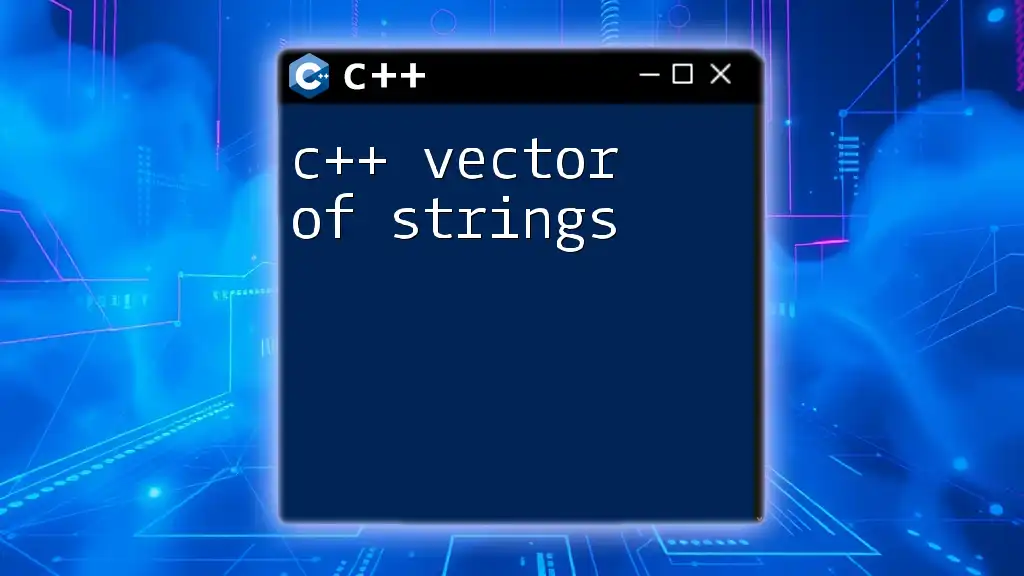
Creating a Vector of Iterators in C++
What is a Vector of Iterators?
A vector of iterators is a vector that stores iterators pointing to elements of another container. This can be particularly useful for operations like sorting, where you want to manage primarily references to elements rather than the elements themselves.
Implementing a Vector of Iterators
To create a vector of iterators, one can use the following code snippet:
#include <vector>
#include <list>
#include <iostream>
int main() {
std::list<int> myList = {10, 20, 30, 40};
std::vector<std::list<int>::iterator> iterators;
for(auto it = myList.begin(); it != myList.end(); ++it) {
iterators.push_back(it);
}
for(auto it : iterators) {
std::cout << *it << " ";
}
return 0;
}
Traversing a Vector of Iterators
Once you have a vector of iterators, you can traverse it easily:
for(auto it : iterators) {
std::cout << *it << " "; // Accessing the value pointed to by the iterator
}
This displays the contents of the list referred to by the vector of iterators.
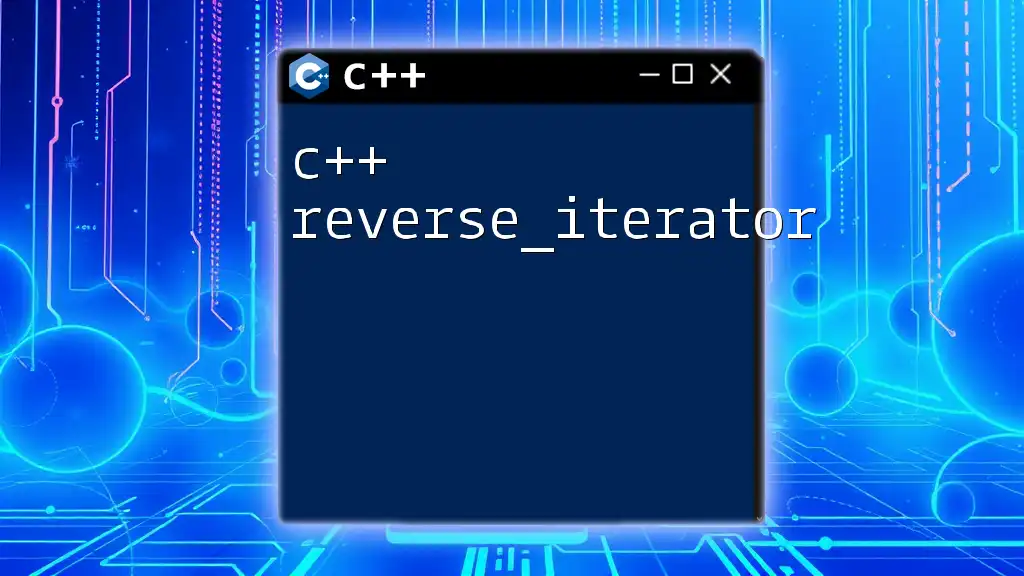
Managing Iterators in a Vector
Manipulating Iterators
Iterators within a vector can be manipulated using standard functions provided by STL, such as `insert()`, `erase()`, and `remove_if`. Here’s how to use several of these:
- Insert an Iterator: You can insert a new iterator at a specific position within the vector.
iterators.insert(iterators.begin() + 1, myList.begin());
- Erase an Iterator: This removes an iterator from the vector.
iterators.erase(iterators.begin() + 1); // Removes the second iterator
Common Pitfalls with Iterators in Vectors
While working with iterators in vectors, a few common pitfalls may arise:
- Invalidating Iterators: Modifying the underlying data structure (like inserting or deleting elements) can invalidate existing iterators.
- Dereferencing Invalid Iterators: Always check bounds and conditions to prevent dereferencing an invalid iterator.
To avoid these issues, consider using `const_iterator` for read-only operations and ensure that the vector of iterators is updated after structural changes to the underlying container.
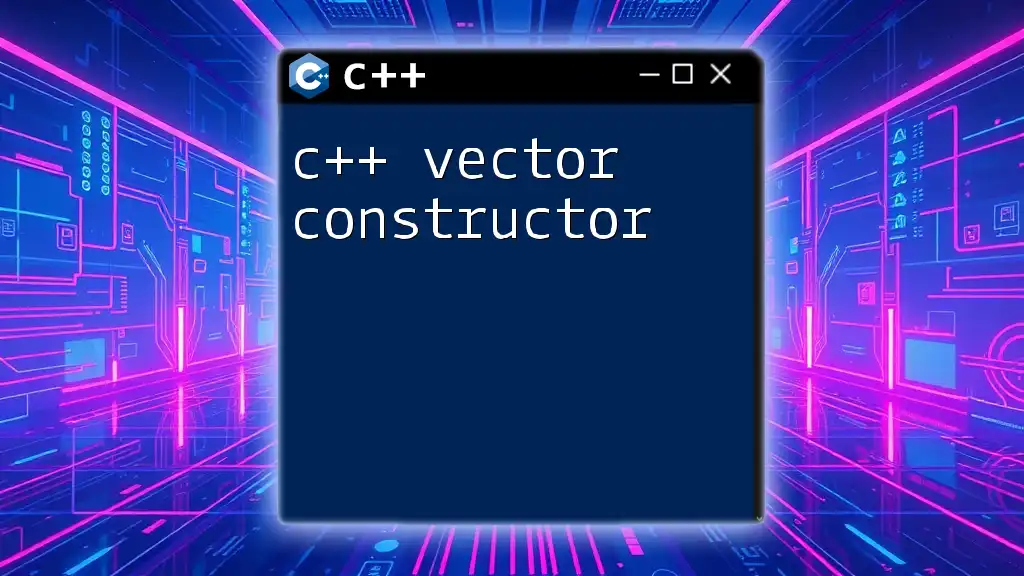
Real-World Applications of Vector Iterators
Use Cases for Vector of Iterators
Vector of iterators has numerous practical applications, especially in algorithms involving sorting, filtering, or manipulating collections. For instance, when sorting a list of elements, storing iterators in a vector allows you to sort references without rigorous overhead of copying elements.
Performance Considerations
Although vector iterators offer significant flexibility, performance must be considered. Access by iterators is generally as efficient as direct indexing when iterating through the data directly. However, in scenarios involving complex manipulations, care should be taken to avoid excessive overhead or invalidation of iterators.
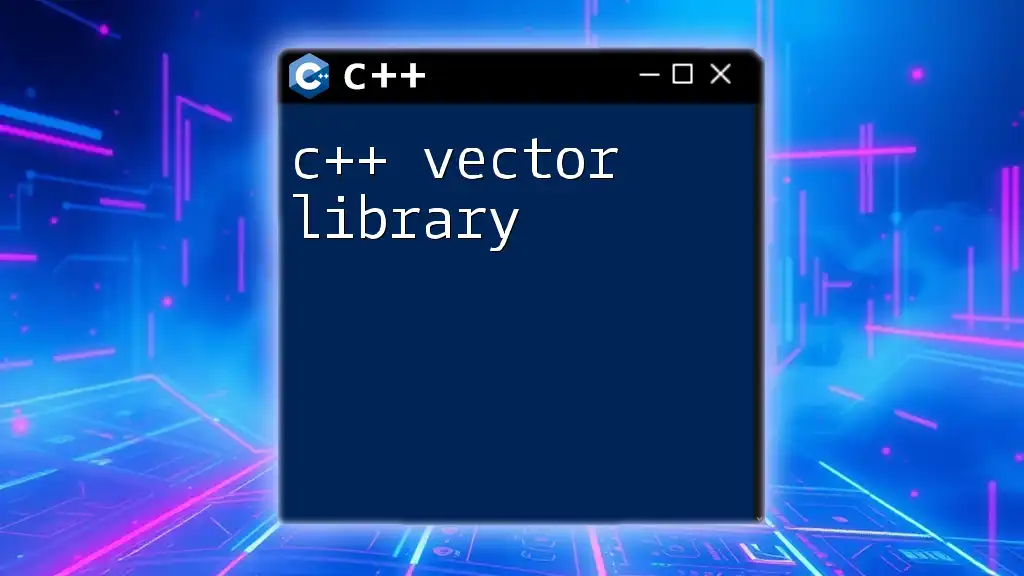
Conclusion
Understanding and implementing a C++ vector of iterators unlocks new possibilities for data management in STL, allowing for more efficient and readable code. By utilizing these powerful tools, programmers can efficiently manipulate collections and optimize performance in various applications. As you continue to practice and apply these concepts, you will enhance your proficiency in C++ programming significantly.
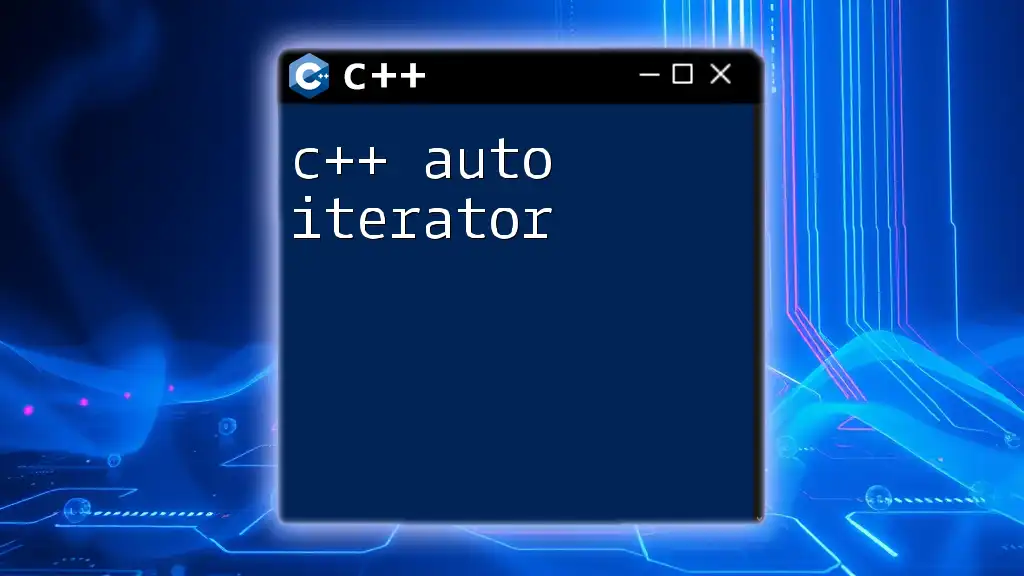
Additional Resources
To further your knowledge on C++ vectors and iterators, consider exploring the following resources:
- [C++ Standard Library Documentation](https://en.cppreference.com/w/cpp/container)
- [Learn C++ - STL Section](https://www.learncpp.com/cpp-tutorial/stl-what-is-the-standard-template-library/)
- [C++ Iterator Concepts](https://www.learncpp.com/cpp-tutorial/iterators/)
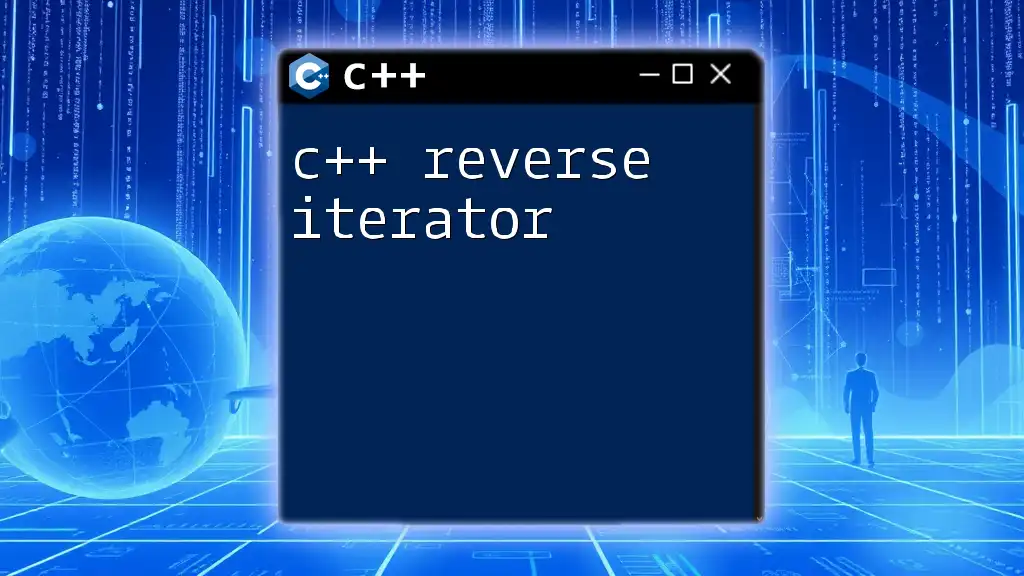
Frequently Asked Questions
Q: Can I iterate over a vector of iterators in reverse?
Yes, using `rbegin()` and `rend()` allows you to traverse in reverse order.
Q: What happens if I access an invalidated iterator?
Dereferencing an invalidated iterator leads to undefined behavior, which can crash your program. Always check the state of your iterators after modifying the underlying vector or its contents.